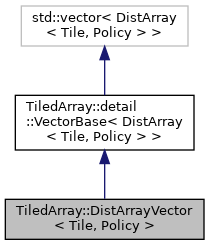
Documentation
template<typename Tile, typename Policy>
class TiledArray::DistArrayVector< Tile, Policy >
A vector of DistArray objects.
Each DistArray object is distributed over the same World, i.e. the vector is not distributed. If you need a vector distributed across a world, with each DistArray object belonging to a local world, see TiledArray/vector_of_arrays.h .
Rationale: sometimes it is more convenient to represent a sequence (vector) of arrays as a vector, rather than a "fused" array with an extra dimension. The latter makes sense when operations are homogeneous across the extra dimension. If extents of modes will depend on the vector index then fusing the arrays becomes cumbersome (N.B.: if the "inner" arrays do not need to be distributed then you should use a tensor-of-tensor, i.e. DistArray<Tensor<Tensor<T>>>)
- Template Parameters
-
T a DistArray type
Public Types | |
using | base_type = detail::VectorBase< DistArray< Tile, Policy > > |
using | array_type = TiledArray::DistArray< Tile, Policy > |
using | eval_type = typename array_type::eval_type |
using | policy_type = Policy |
using | value_type = array_type |
typedef array_type::element_type | element_type |
using | T = array_type |
Public Member Functions | |
DistArrayVector ()=default | |
DistArrayVector (std::size_t i) | |
template<typename... Args, typename = std::enable_if_t< (std::is_same_v<std::remove_reference_t<Args>, T> && ...)>> | |
DistArrayVector (Args &&..._t) | |
DistArrayVector (const std::vector< T > &t_pack) | |
DistArrayVector (std::vector< T > &&t_pack) | |
template<typename B , typename E > | |
DistArrayVector (B &&begin, E &&end) | |
operator bool () const | |
World & | world () const |
const TiledRange & | trange () const |
void | truncate () |
bool | is_initialized () |
ShapeVector< typename array_type::shape_type > | shape () const |
TiledArray::expressions::ExprVector< TiledArray::expressions::TsrExpr< const T, true > > | operator() (const std::string &annotation) const |
Create a tensor expression. More... | |
TiledArray::expressions::ExprVector< TiledArray::expressions::TsrExpr< T, true > > | operator() (const std::string &annotation) |
Create a tensor expression. More... | |
DistArray< Tile, Policy > | vector_sum () const |
![]() | |
VectorBase ()=default | |
VectorBase (std::size_t i) | |
VectorBase (Args &&..._t) | |
VectorBase (const std::vector< DistArray< Tile, Policy > > &t_pack) | |
VectorBase (std::vector< DistArray< Tile, Policy > > &&t_pack) | |
VectorBase (B &&begin, E &&end) | |
operator DistArray< Tile, Policy > & () & | |
operator const DistArray< Tile, Policy > & () const & | |
operator DistArray< Tile, Policy > && () && | |
operator bool () const | |
Public Attributes | |
decltype(norm2(std::declval< array_type >())) typedef | scalar_type |
Member Typedef Documentation
◆ array_type
using TiledArray::DistArrayVector< Tile, Policy >::array_type = TiledArray::DistArray<Tile, Policy> |
◆ base_type
using TiledArray::DistArrayVector< Tile, Policy >::base_type = detail::VectorBase<DistArray<Tile, Policy> > |
◆ element_type
typedef array_type::element_type TiledArray::DistArrayVector< Tile, Policy >::element_type |
◆ eval_type
using TiledArray::DistArrayVector< Tile, Policy >::eval_type = typename array_type::eval_type |
◆ policy_type
using TiledArray::DistArrayVector< Tile, Policy >::policy_type = Policy |
◆ T
using TiledArray::DistArrayVector< Tile, Policy >::T = array_type |
◆ value_type
using TiledArray::DistArrayVector< Tile, Policy >::value_type = array_type |
Constructor & Destructor Documentation
◆ DistArrayVector() [1/6]
|
default |
◆ DistArrayVector() [2/6]
|
inline |
◆ DistArrayVector() [3/6]
|
inline |
◆ DistArrayVector() [4/6]
|
inline |
◆ DistArrayVector() [5/6]
|
inline |
◆ DistArrayVector() [6/6]
|
inline |
Member Function Documentation
◆ is_initialized()
|
inline |
Calls is_initalized() on each element and return true if all
- Returns
- a bool true if all elemments are initialized
- Exceptions
-
TiledArray::Exception if this is null
◆ operator bool()
|
inlineexplicit |
- Returns
- true is nonempty and each element is nonnull (i.e., is initialized)
◆ operator()() [1/2]
|
inline |
Create a tensor expression.
- Parameters
-
annotation An annotation string
- Returns
- A non-const tensor expression object
◆ operator()() [2/2]
|
inline |
Create a tensor expression.
- Parameters
-
annotation An annotation string
- Returns
- A const tensor expression object
◆ shape()
|
inline |
returns the shapes of the member arrays
- Returns
- vector of shapes
- Exceptions
-
TiledArray::Exception if this is null
◆ trange()
|
inline |
- Returns
- a TiledRange object describing all objects
- Exceptions
-
TiledArray::Exception if this is null
◆ truncate()
|
inline |
Calls truncate() on each element
- Exceptions
-
TiledArray::Exception if this is null
◆ vector_sum()
|
inline |
- Returns
- A DistArray that is the sum of elements in DistArrayVector
◆ world()
|
inline |
- Returns
- a World to which all DistArrays belong
- Exceptions
-
TiledArray::Exception if this is null
Member Data Documentation
◆ scalar_type
decltype(norm2(std::declval<array_type>())) typedef TiledArray::DistArrayVector< Tile, Policy >::scalar_type |
The documentation for this class was generated from the following file:
- mpqc/math/external/tiledarray/dist_array_vector.h