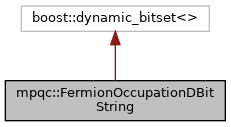
Documentation
A "dense" nullable string represents occupancies of a set of Ns states by a bitstring. The null string is distinct from a string in space of 0 states.
- Template Parameters
-
Ns the number of states
Public Types | |
using | parent_type = boost::dynamic_bitset<> |
using | base_type = parent_type |
using | state_index_type = orbital_index_type |
using | vectorofbool_t = boost::container::small_vector< bool, 128 > |
Member Typedef Documentation
◆ base_type
◆ parent_type
using mpqc::FermionOccupationDBitString::parent_type = boost::dynamic_bitset<> |
◆ state_index_type
◆ vectorofbool_t
using mpqc::FermionOccupationDBitString::vectorofbool_t = boost::container::small_vector<bool, 128> |
Constructor & Destructor Documentation
◆ FermionOccupationDBitString() [1/8]
|
inline |
constructs an invalid (null) string
◆ FermionOccupationDBitString() [2/8]
|
inline |
copy ctor
- Note
- does not copy memoized part of state
◆ FermionOccupationDBitString() [3/8]
|
default |
move ctor
◆ ~FermionOccupationDBitString()
|
default |
◆ FermionOccupationDBitString() [4/8]
|
inlineexplicit |
Constructs a set of N
states
- Parameters
-
[in] N the number of states [in] default_to_occupied if true, will make all states occupied
◆ FermionOccupationDBitString() [5/8]
|
inlineexplicit |
Constructs a string of N
states using bits encoded by an unsigned long
- Parameters
-
[in] N number of states represented by this string, asserted to be less than or equal to Ns
[in] ulong_bitset an unsigned long encoding the string
◆ FermionOccupationDBitString() [6/8]
|
inlineexplicit |
Constructs a set of states specified by a bitset
- Parameters
-
bs a bitset specifying the occupancies of the states (set bit indicates occupied states)
◆ FermionOccupationDBitString() [7/8]
|
inline |
Constructs FermionOccupationDBitString using a (possibly-empty) set of indices of occupied states
- Template Parameters
-
IntegralRange a range of integers
- Parameters
-
[in] N number of states represented by this string occupied_states the sequence of occupied states
◆ FermionOccupationDBitString() [8/8]
|
inline |
Constructs FermionOccupationDBitString using a (possibly-empty) set of indices of occupied states
- Template Parameters
-
Integer an integral type
- Parameters
-
[in] N number of states represented by this string occupied_states the sequence of occupied states
Member Function Documentation
◆ add()
|
inline |
Adds a particle to state to_.
- Parameters
-
to the state to which the particle will be added.
- Returns
- reference to this string, for chaining operations (e.g. a.remove(0).remove(7).add(13) etc.)
- Note
- if this object is null, this is noop
◆ clone()
|
inline |
- Returns
- clone of
*this
s
◆ count() [1/2]
|
inline |
Reports the number of occupied states
- Returns
- the number of occupied states
- Note
- asserts that this is nonnull
◆ count() [2/2]
|
inline |
counts the number of bits in (pos1,pos2) (assumes pos2 >= pos1)
- Parameters
-
pos1 integer in [-1,Ns), set to -1 to count bits in [0,pos2) pos2 integer in [0,Ns)
- Returns
- the number of bits in (pos1,pos2)
- Note
- asserts that this is nonnull
◆ empty()
|
inline |
are all states empty?
- Returns
- true if all states are empty
- Note
- asserts that this is nonnull
◆ find_first_occupied()
|
inline |
- Returns
- the lowest index
i
such as statei
is occupied, or-1
if*this
has no occupied states.
◆ find_next_occupied()
|
inline |
- Returns
- the lowest index
i
greater thanpos
such as statei
is occupied, or-1
if no such occupied states exist.
◆ flip()
|
inline |
flips the occupancies of all states
- Returns
- reference to
*this
- Note
- asserts that this is nonnull
◆ hash_value()
|
inline |
- Warning
- for 64 or fewer states this is optimized to return
this->to_ulong()
◆ occupied_states()
|
inline |
Reports the states that are occupied in this string
- Returns
- a range with the indices of all occupied states (in increasing order)
- Note
- asserts that this is nonnull
◆ operator bool()
|
inlineexplicit |
- Returns
- true of string is not in a null state
◆ operator!=()
|
inline |
- Parameters
-
[in] other another string
- Returns
- true if
(*this)==other is false
◆ operator&()
|
inline |
- Parameters
-
other another string
- Returns
- AND of
*this
withother
- Precondition
this->size() == other.size()
◆ operator=() [1/2]
|
inline |
copy assignment
- Note
- does not copy memoized part of state
◆ operator=() [2/2]
|
default |
move assignment
◆ operator==()
|
inline |
- Parameters
-
[in] other another string
- Returns
- true if both are null, or both are valid and equal
◆ operator^()
|
inline |
- Parameters
-
other another string
- Returns
- XOR of
*this
withother
- Precondition
this->size() == other.size()
◆ operator|()
|
inline |
- Parameters
-
other another string
- Returns
- OR of
*this
withother
- Precondition
this->size() == other.size()
◆ populated_states()
|
inline |
Reports the states in the order of their occupancy (occupied first, unoccupied second)
- Returns
- a const reference to (memoized) PopulatedSparseOrbitalRange object, with occupied orbitals preceding occupied orbitals
- Note
- asserts that this is nonnull
◆ remove()
|
inline |
Removes a particle from_ state from_.
- Parameters
-
from_ the state from_ which the particle will be removed.
- Returns
- reference to this string, for chaining operations (e.g. a.remove(0).remove(7).add(13) etc.)
- Note
- if this object is null, this is noop
◆ reset()
|
inline |
empties all states
◆ size()
|
inline |
Reports the total number of states
- Returns
- the total number states
- Note
- asserts that this is nonnull
◆ to_bitset()
|
inline |
converts to the bitset representation of this string
- Returns
- bitset representation of this string
- Note
- asserts that this is nonnull
◆ to_ulong()
|
inline |
converts to unsigned long
- Returns
- unsigned long representation of this string
- Note
- asserts that this is nonnull
◆ to_vectorofbool()
|
inline |
converts to the vector-of-bool representation of this string
- Returns
- vector-of-bool representation of this string
- Note
- asserts that this is nonnull
◆ unoccupied_states()
|
inline |
Reports the states that are unoccupied in this string
- Returns
- a range with the indices of all unoccupied states (in increasing order)
- Note
- asserts that this is nonnull
The documentation for this class was generated from the following file:
- mpqc/math/fock/string.h