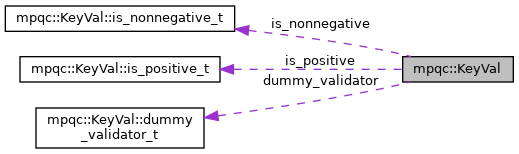
Documentation
KeyVal specifies C++ primitive data (booleans, integers, reals, string) and user-defined objects obtained from JSON/XML/INFO input or by programmatic construction.
KeyVal is a (sub)tree of Key=Value pairs implemented with Boost.PropertyTree. KeyVal extends the standard JSON/XML/INFO syntax to allow references as well as specification of registered C++ objects. See The KeyVal Library for the rationale, examples, and other details.
KeyVal has reference semantics, i.e., copying KeyVal produces a KeyVal that refers to the same PropertyTree object and shares the class registry object with the original KeyVal object.
Since KeyVal is default-constructible and directly mutable, this obsoletes sc::AssignedKeyVal
from MPQC v3 and older. Its behavior resembles sc::PrefixKeyVal
by combining prefix with a const tree. Hence this version of KeyVal roughly can be viewed as an assignable PrefixKeyVal
of MPQC v3 and older, but supporting input from more modern formats like XML and JSON.
Classes | |
struct | bad_input |
KeyVal exception. More... | |
struct | dummy_validator_t |
struct | is_nonnegative_t |
struct | is_positive_t |
Public Types | |
enum | InputFormat { InputFormat::invalid = -1, InputFormat::json = 0, InputFormat::xml = 1, InputFormat::info = 2, InputFormat::first = json, InputFormat::last = info } |
using | ptree = boost::property_tree::iptree |
data type for representing a property tree More... | |
using | key_type = ptree::key_type |
using | value_type = ptree::data_type |
Public Member Functions | |
KeyVal () | |
creates empty KeyVal More... | |
KeyVal (const KeyVal &other)=default | |
copy ctor More... | |
KeyVal (KeyVal &&other)=default | |
move ctor More... | |
virtual | ~KeyVal ()=default |
KeyVal & | operator= (const KeyVal &other)=default |
copy assignment More... | |
KeyVal & | operator= (KeyVal &&other)=default |
move assignment More... | |
KeyVal | clone () const |
creates a deep copy of this object More... | |
virtual KeyVal | keyval (const key_type &path) const |
construct a KeyVal representing a subtree located at the given path More... | |
std::shared_ptr< ptree > | top_tree () const |
returns a shared_ptr to the (top) tree More... | |
std::shared_ptr< ptree > | tree () const |
returns a shared_ptr to this (sub)tree More... | |
template<typename T , typename = std::enable_if_t<Describable<T>::value>> | |
KeyVal & | set_default_class_key (const std::string &key) |
obtains default class key for class T More... | |
template<typename T > | |
std::string | default_class_key () const |
obtains default class key for class T More... | |
bool | exists (const key_type &path) const |
template<typename Target = const DescribedClass> | |
bool | exists_object (const key_type &path) const noexcept |
bool | exists_class (const key_type &path) const |
std::optional< size_t > | count (const key_type &path) const |
template<typename T > | |
std::enable_if_t< not KeyVal::is_sequence_v< T >, KeyVal & > | assign (const key_type &path, const T &value) & |
assign simple value at the given path (overwrite, if necessary) More... | |
template<typename T > | |
std::enable_if_t< not KeyVal::is_sequence_v< T >, KeyVal > | assign (const key_type &path, const T &value) && |
template<typename SequenceContainer > | |
std::enable_if_t< KeyVal::is_sequence_v< SequenceContainer >, KeyVal & > | assign (const key_type &path, const SequenceContainer &value, bool json_style=true) & |
Assign a sequence container at the given path (overwrite, if necessary). More... | |
template<typename SequenceContainer > | |
std::enable_if_t< KeyVal::is_sequence_v< SequenceContainer >, KeyVal > | assign (const key_type &path, const SequenceContainer &value, bool json_style=true) && |
template<typename T > | |
KeyVal & | assign (const key_type &path, std::initializer_list< T > value, bool json_style=true) & |
Assign a sequence container at the given path (overwrite, if necessary). More... | |
template<typename T > | |
KeyVal | assign (const key_type &path, std::initializer_list< T > value, bool json_style=true) && |
template<typename T = DescribedClass> | |
std::enable_if_t< Describable< T >::value, KeyVal & > | assign (const key_type &path, const std::shared_ptr< T > &value) & |
template<typename T = DescribedClass> | |
std::enable_if_t< Describable< T >::value, KeyVal > | assign (const key_type &path, const std::shared_ptr< T > &value) && |
template<typename T = DescribedClass> | |
std::enable_if_t< Describable< T >::value, const KeyVal & > | assign (const key_type &path, const std::shared_ptr< T > &value) const |
KeyVal & | erase (const key_type &path) |
const KeyVal & | erase_object (const key_type &path) const |
const KeyVal & | erase_class (const key_type &path) const |
template<typename T , typename Validator = dummy_validator_t> | |
std::enable_if_t< not KeyVal::is_sequence_v< T > &¬ utility::meta::can_construct< T, const KeyVal & >::value, T > | value (const key_type &path, Validator &&validator=Validator{}, std::enable_if_t< KeyVal::is_valid_validator_v< Validator, T >> *=nullptr) const |
template<typename T , typename Validator = dummy_validator_t> | |
std::enable_if_t< not KeyVal::is_sequence_v< T > &&utility::meta::can_construct< T, const KeyVal & >::value, T > | value (const key_type &path, Validator &&validator=Validator{}, std::enable_if_t< is_valid_validator_v< Validator, T >> *=nullptr) const |
template<typename SequenceContainer , typename Validator = dummy_validator_t> | |
std::enable_if_t< KeyVal::is_mutable_sequence_v< SequenceContainer >, SequenceContainer > | value (const key_type &path, Validator &&validator=Validator{}, std::enable_if_t< KeyVal::is_valid_validator_v< Validator, std::decay_t< typename SequenceContainer::value_type >>> *=nullptr) const |
template<typename SequenceContainer , typename Validator = dummy_validator_t> | |
std::enable_if_t< KeyVal::is_mutable_sequence_v< SequenceContainer >, SequenceContainer > | value (const key_type &path, const key_type &deprecated_path, Validator &&validator=Validator{}, std::enable_if_t< KeyVal::is_valid_validator_v< Validator, std::decay_t< typename SequenceContainer::value_type >>> *=nullptr) const |
template<typename T , typename Validator = dummy_validator_t> | |
std::enable_if_t< not KeyVal::is_sequence_v< T >, T > | value (const key_type &path, const T &default_value, const key_type &deprecated_path, Validator &&validator=Validator{}, std::enable_if_t< KeyVal::is_valid_validator_v< Validator, T >> *=nullptr) const |
template<typename T , typename Validator = dummy_validator_t> | |
std::enable_if_t< not KeyVal::is_sequence_v< T >, T > | value (const key_type &path, const T &default_value, Validator &&validator=Validator{}, std::enable_if_t< KeyVal::is_valid_validator_v< Validator, T >> *=nullptr) const |
template<typename T = DescribedClass, typename Enabler = std::enable_if_t<Describable<T>::value>> | |
std::shared_ptr< T > | object (const key_type &path=key_type(), bool bypass_registry=false, bool disable_bad_input=false) const |
return a pointer to an object at the given path More... | |
template<typename T = DescribedClass, typename = std::enable_if_t<Describable<T>::value>> | |
std::shared_ptr< T > | class_ptr (const key_type &path=key_type(), bool bypass_registry=false, bool disable_bad_input=false) const |
std::vector< key_type > | dc_registry_paths () const |
dumps paths of all objects in the DescribedClass object registry More... | |
std::string | path () const |
key_type | to_absolute_path (const key_type &path) const |
bool | operator== (const KeyVal &other) const |
void | write_xml (std::basic_ostream< typename key_type::value_type > &os) const |
write to stream in XML form More... | |
void | read_xml (std::basic_istream< typename key_type::value_type > &is) |
void | write_json (std::basic_ostream< key_type::value_type > &os) const |
write to stream in JSON form More... | |
void | read_json (std::basic_istream< key_type::value_type > &is) |
void | write_info (std::basic_ostream< typename key_type::value_type > &os) const |
write to stream in INFO form More... | |
void | read_info (std::basic_istream< typename key_type::value_type > &is) |
Static Public Member Functions | |
static bool | throw_if_deprecated_path () |
static void | set_throw_if_deprecated_path (bool f) |
static key_type | concat_path (const key_type &prefix, const key_type &postfix) |
Static Public Attributes | |
constexpr static char | separator = ':' |
static constexpr dummy_validator_t | dummy_validator {} |
static const is_nonnegative_t | is_nonnegative {} |
static const is_positive_t | is_positive {} |
Protected Member Functions | |
virtual boost::optional< const ptree & > | get_subtree (const key_type &path) const |
Member Typedef Documentation
◆ key_type
using mpqc::KeyVal::key_type = ptree::key_type |
◆ ptree
using mpqc::KeyVal::ptree = boost::property_tree::iptree |
data type for representing a property tree
◆ value_type
using mpqc::KeyVal::value_type = ptree::data_type |
Member Enumeration Documentation
◆ InputFormat
|
strong |
the format of the input file, described in Boost.PropertyTree docs.
Enumerator | |
---|---|
invalid | |
json | |
xml | |
info | |
first | |
last |
Constructor & Destructor Documentation
◆ KeyVal() [1/3]
mpqc::KeyVal::KeyVal | ( | ) |
creates empty KeyVal
◆ KeyVal() [2/3]
|
default |
copy ctor
Since this class has reference semantics, the underlying data structures (ptree and class registry) are not copied.
- Note
- to clone top-level tree use KeyVal::clone()
◆ KeyVal() [3/3]
|
default |
move ctor
◆ ~KeyVal()
|
virtualdefault |
Member Function Documentation
◆ assign() [1/9]
|
inline |
Assign a sequence container at the given path (overwrite, if necessary).
- Template Parameters
-
SequenceContainer any container for which KeyVal::is_sequence_v<SequenceContainer> is true, currently any of the following is allowed: std::array
,std::vector
,std::list
, andstd::initializer_list
. Can be a recursive sequence (unless initializer_list), i.e. sequence of sequence.
- Parameters
-
path the target path value the sequence to put at the path json_style if true, use empty keys so that JSON arrays are produced by KeyVal::write_json, else use 0-based integer keys, e.g. the first element will have key path:0
, the second –path:1
, etc. (this is similar to how array elements were addressed in MPQC3)
◆ assign() [2/9]
|
inline |
◆ assign() [3/9]
|
inline |
assign the given pointer to a DescribedClass object at the given path (overwrite, if necessary). This Keyval will keep track of the given object via a weak_ptr (i.e. KeyVal will not own it).
- Template Parameters
-
T a class directly derived from DescribedClass
- Parameters
-
path the path to value
value the object pointer to assign to path path
- Note
- redirects to the const version of this function; this (non-const version) is needed to be able to chain with other variants
- Warning
- these key/value pairs are not part of ptree, hence cannot be written to JSON/XML
◆ assign() [4/9]
|
inline |
◆ assign() [5/9]
|
inline |
assign the given pointer to a DescribedClass object at the given path (overwrite, if necessary). This Keyval will keep track of the given object via a weak_ptr (i.e. KeyVal will not own it).
- Template Parameters
-
T a class directly derived from DescribedClass
- Parameters
-
path the path to value
value the object pointer to assign to path path
(it is asserted to be nonnull)
- Note
- this is legitimately const since the registry contents are mutable (see KeyVal::object ).
- Warning
- these key/value pairs are not part of ptree, hence cannot be written to JSON/XML
◆ assign() [6/9]
|
inline |
assign simple value
at the given path (overwrite, if necessary)
- Parameters
-
value a "simple" value, i.e. it can be converted to a KeyVal::key_type using a std::basic_ostream<KeyVal::key_type::value_type>
◆ assign() [7/9]
|
inline |
◆ assign() [8/9]
|
inline |
Assign a sequence container at the given path (overwrite, if necessary).
- Template Parameters
-
T any type
- Parameters
-
path the target path value a sequence to put at the path json_style if true, use empty keys so that JSON arrays are produced by KeyVal::write_json, else use 0-based integer keys, e.g. the first element will have key path:0
, the second –path:1
, etc. (this is similar to how array elements were addressed in MPQC3)
◆ assign() [9/9]
|
inline |
◆ class_ptr()
|
inline |
◆ clone()
KeyVal mpqc::KeyVal::clone | ( | ) | const |
creates a deep copy of this object
- Note
- the DescribedClass object registry is not copied
◆ concat_path()
|
inlinestatic |
Concatenates two paths
- Returns
- a path obtained by concatenating
prefix
andpostfix
;
◆ count()
|
inline |
counts the number of children of the node at this path
- Parameters
-
path the path
- Returns
- an optional that does not contain a value if
path
does not exist or it points to a simple keyword, otherwise containing the number of elements (>=0) ifpath
points to an array or a keyword group
◆ dc_registry_paths()
|
inline |
dumps paths of all objects in the DescribedClass object registry
- Returns
- vector of absolute paths for the objects in the DescribedClass registry
◆ default_class_key()
|
inline |
obtains default class key for class T
- Template Parameters
-
T a class
- Returns
- the default class key to assume in KeyVal::object<T>() ; if the default class key has not been specified previously, returns an empty string
- See also
- set_default_class_key
◆ erase()
erases entries located under path
. This does not touch the DescribedClass object registry (
- See also
- KeyVal::erase_object ).
- Parameters
-
path the target path
◆ erase_class()
◆ erase_object()
erases the entry in the DescribedClass object registry at path
. This does not touch the keyword tree (
- See also
- KeyVal::erase ).
- Note
- this is const since the registry contents are mutable (see KeyVal::object ).
◆ exists()
|
inline |
checks whether the given path exists
- Parameters
-
path the path
- Returns
- true if
path
exists
◆ exists_class()
|
inline |
◆ exists_object()
|
inlinenoexcept |
check whether the given DescribedClass object exists in the registry already.
- Template Parameters
-
Target if it is not DescribedClass will only return true if the found object can be converted to this type Target
- Parameters
-
path the path
- Returns
- true if an object of type
Target
atpath
class exists
◆ get_subtree()
|
inlineprotectedvirtual |
returns a reference to a subtree located at (absolute or relative) path.
- Parameters
-
path the path to the subtree
◆ keyval()
construct a KeyVal representing a subtree located at the given path
Since this class has reference semantics, the result refers to the same underlying data structures (ptree and class registry).
corresponds to the old MPQC's PrefixKeyVal
- Parameters
-
path the path to the subtree; absolute (within the top_tree) and relative (with respect to this KeyVal's subtree) paths are allowed.
Reimplemented in mpqc::detail::SubTreeKeyVal.
◆ object()
|
inline |
return a pointer to an object at the given path
Constructs a smart pointer to object of type T
. If user provided keyword "type" in this KeyVal object its value will be used for the object type. Otherwise, if T
is a concrete type, this will construct object of type T
. In either case, the type of constructed object must have DescribedClass as its public base and registered.
- Template Parameters
-
T a class derived from DescribedClass Enabler a dummy enabler class; defaults to std::enable_if_t<Describable<T>::value>
; override to force object construction (this is useful to construct objects of classes that do not derive from DescribedClass programmatically), but must set bypass_registry to true
- Parameters
-
path the path; if not provided, use the empty path bypass_registry if true
will not query the registry for the existence of the object and will not place the newly-constructed object in the registrydisable_bad_input if true
will return a null pointer instead of throwing KeyVal::bad_input
- Returns
- a std::shared_ptr to
T
; null pointer will be returned ifpath
does not exist.
- Exceptions
-
KeyVal::bad_input Unless disable_bad_input
is true, will throw this if any of the following is true:- the value of keyword "type" is not a registered class key;
- the user did not specify keyword "type" and class T is abstract or is not registered.
- an object has been previosuly constructed, but cannot be cast to T
- Note
object<T>("path")
is equivalent tokeyval("path").object<T>()
- the contents of the registry are by definition mutable so that this can be const
◆ operator=() [1/2]
copy assignment
Since this class has reference semantics, the underlying data structures (ptree and class registry) are not copied.
◆ operator=() [2/2]
◆ operator==()
|
inline |
comparison operator
- Returns
- true if
this
andother
point to the same location in the same ptree (+ other impl details are the same)
◆ path()
|
inline |
- Returns
- the path from the root of top tree to this subtree
◆ read_info()
|
inline |
read from a std::istream in INFO form
- Parameters
-
[in,out] is a std::istream
- Exceptions
-
KeyVal::bad_input if failed to parse the contents of is
as INFO
◆ read_json()
|
inline |
read from a std::istream in JSON form
- Parameters
-
[in,out] is a std::istream
- Exceptions
-
KeyVal::bad_input if failed to parse the contents of is
as JSON
◆ read_xml()
|
inline |
read from a std::istream in XML form
- Parameters
-
[in,out] is a std::istream
- Exceptions
-
KeyVal::bad_input if failed to parse the contents of is
as XML
◆ set_default_class_key()
|
inline |
obtains default class key for class T
- Template Parameters
-
T a class derived from DescribedClass
- Returns
- the default class key to assume in KeyVal::object<T>() ; if the default class key has not been specified previously, returns an empty string
- See also
- set_default_class_key
◆ set_throw_if_deprecated_path()
|
inlinestatic |
sets the flag controlling whether reading a deprecated path results in an exception
- Parameters
-
f the new value of the flag
◆ throw_if_deprecated_path()
|
inlinestatic |
reads the flag controlling whether reading a deprecated path results in an exception
- Returns
- a boolean
◆ to_absolute_path()
computes an absolute path from a given (absolute or relative) path
- Parameters
-
path an absolute path (starts with "$:") or a relative path (the leading "$" is dropped)
- Returns
- the absolute path
- Note
- this does not resolve references
- Exceptions
-
KeyVal::bad_input if path is invalid; an example is ".." .
◆ top_tree()
|
inline |
returns a shared_ptr to the (top) tree
◆ tree()
std::shared_ptr< KeyVal::ptree > mpqc::KeyVal::tree | ( | ) | const |
returns a shared_ptr to this (sub)tree
- Note
- the result is aliased against the top tree
◆ value() [1/6]
std::enable_if_t< KeyVal::is_mutable_sequence_v< SequenceContainer >, SequenceContainer > mpqc::KeyVal::value | ( | const key_type & | path, |
const key_type & | deprecated_path, | ||
Validator && | validator = Validator{} , |
||
std::enable_if_t< KeyVal::is_valid_validator_v< Validator, std::decay_t< typename SequenceContainer::value_type >>> * | = nullptr |
||
) | const |
find value corresponding to a path and returns it as a SequenceContainer
- Template Parameters
-
SequenceContainer the desired sequence conatiner type Validator the type of validator
- Parameters
-
path the path deprecated_path a deprecated path will only be queried if it's not validator a callable for which validator(T)
returns a boolean. Before returning a value, it will be validated byvalidator
. The default is a dummy validator that always returnstrue
.
- Exceptions
-
KeyVal::bad_input if path
is bad, if validation failed, or if KeyVal::throw_if_deprecated_path returns true anddeprecated_path
was read.
- Returns
- value of type
SequenceContainer
◆ value() [2/6]
|
inline |
return value corresponding to a path and convert to the desired type.
- Template Parameters
-
T the desired value type Validator the type of validator
- Parameters
-
path the path to the value; ignored if empty default_value the default value will be returned if path
is not found.deprecated_path a deprecated path will only be queried if it's not empty and path
is empty or not found; if its value is used a message will be added toExEnv::err0()
. The default is an empty string.validator a callable for which validator(T)
returns a boolean. Before returning a value, it will be validated byvalidator
. The default is a dummy validator that always returnstrue
.
- Returns
- value of type
T
- Exceptions
-
KeyVal::bad_input if validation failed, or if KeyVal::throw_if_deprecated_path returns true and deprecated_path
was read.
◆ value() [3/6]
|
inline |
return value corresponding to a path and convert to the desired type.
- Template Parameters
-
T the desired value type Validator the type of validator
- Parameters
-
path the path to the value default_value the default value will be returned if path
is not found.validator a callable for which validator(T)
returns a boolean. Before returning a value, it will be validated byvalidator
. The default is a dummy validator that always returnstrue
.
- Returns
- value of type
T
- Exceptions
-
KeyVal::bad_input if validation failed.
◆ value() [4/6]
std::enable_if_t< not KeyVal::is_sequence_v< T > &&utility::meta::can_construct< T, const KeyVal & >::value, T > mpqc::KeyVal::value | ( | const key_type & | path, |
Validator && | validator = Validator{} , |
||
std::enable_if_t< is_valid_validator_v< Validator, T >> * | = nullptr |
||
) | const |
return the result of converting the value at the given path to the desired type.
- Template Parameters
-
T the desired value type, must be a "simple" type that can be accepted by KeyVal::assign() Validator the type of validator
- Parameters
-
path the path to the value validator a callable for which validator(T)
returns a boolean. Before returning a value, it will be validated byvalidator
. The default is a dummy validator that always returnstrue
.
- Returns
- value stored at
path
converted to typeT
- Exceptions
-
KeyVal::bad_input if path not found or cannot convert value KeyVal::bad_input if validation failed. representation to the desired type
◆ value() [5/6]
std::enable_if_t< KeyVal::is_mutable_sequence_v< SequenceContainer >, SequenceContainer > mpqc::KeyVal::value | ( | const key_type & | path, |
Validator && | validator = Validator{} , |
||
std::enable_if_t< KeyVal::is_valid_validator_v< Validator, std::decay_t< typename SequenceContainer::value_type >>> * | = nullptr |
||
) | const |
find value corresponding to a path and returns it as a SequenceContainer
- Template Parameters
-
SequenceContainer the desired sequence conatiner type Validator the type of validator
- Parameters
-
path the path validator a callable for which validator(T)
returns a boolean. Before returning a value, it will be validated byvalidator
. The default is a dummy validator that always returnstrue
.
- Exceptions
-
KeyVal::bad_input if path
is bad, or if validation failed.
- Returns
- value of type
T
◆ value() [6/6]
|
inline |
return the result of converting the value at the given path to the desired type.
- Template Parameters
-
T the desired value type, must be a "simple" type that can be accepted by KeyVal::assign() Validator the type of validator
- Parameters
-
path the path to the value validator a callable for which validator(T)
returns a boolean. Before returning a value, it will be validated byvalidator
. The default is a dummy validator that always returnstrue
.
- Returns
- value stored at
path
converted to typeT
- Exceptions
-
KeyVal::bad_input if path not found or cannot convert value representation to the desired type KeyVal::bad_input if validation failed.
◆ write_info()
|
inline |
write to stream in INFO form
◆ write_json()
|
inline |
write to stream in JSON form
◆ write_xml()
|
inline |
write to stream in XML form
read/write KeyVal specified in one of accepted Boost.PropertyTree formats (see Boost.PropertyTree docs)
Member Data Documentation
◆ dummy_validator
|
staticconstexpr |
◆ is_nonnegative
|
static |
◆ is_positive
|
static |
◆ separator
|
staticconstexpr |
The documentation for this class was generated from the following files:
- mpqc/util/keyval/keyval.h
- mpqc/util/keyval/keyval.cpp