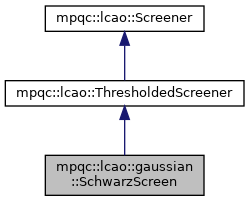
Documentation
Class for Schwarz based screening.
We will assume that these screeners are replicated for the integrals they need and so will not bother with serialization of them.
SchwarzScreen has support for up to 4 different bases, currently there are no optimizations made for all four bases being equal to each other.
Public Types | |
using | norm_op_type = Q2Matrix::norm_op_type |
Public Member Functions | |
SchwarzScreen ()=default | |
SchwarzScreen (SchwarzScreen const &)=default | |
SchwarzScreen (SchwarzScreen &&)=default | |
SchwarzScreen & | operator= (SchwarzScreen const &)=default |
SchwarzScreen & | operator= (SchwarzScreen &&)=default |
virtual | ~SchwarzScreen () noexcept=default |
SchwarzScreen (std::shared_ptr< Q2Matrix > Q2bra, std::shared_ptr< Q2Matrix > Q2ket, double thresh, double thresh_cluster) | |
Constructor which requires two Q matrices. More... | |
SchwarzScreen (std::shared_ptr< Q2Matrix > Q2bra, std::shared_ptr< Q2Matrix > Q2ket, double thresh) | |
The ctor version that uses same threshold for shell and cluster skipping. More... | |
void | set_skip_threshold (double thresh) override |
void | set_skip_threshold_cluster (double thresh) override |
Q2Matrix const & | Q2bra () const |
Returns the screening matrix for the Bra indices. More... | |
Q2Matrix const & | Q2ket () const |
Returns the screening matrix for the Ket indices. More... | |
const std::shared_ptr< const Basis > & | basis (std::size_t i=0) const |
std::size_t | nbases () const |
norm_op_type * | norm_op () const |
std::tuple< TA::Tensor< float >, bool > | norm_estimate (madness::World &world, BasisRefVector const &bs_array, TA::Pmap const &pmap, bool replicate=false) const override |
returns an estimate of shape norms for the given basis vector, in presence of symmetry described by a math::PetiteList object. More... | |
virtual double | estimate (int64_t a) const |
compute estimate of squared norm of a shellset/cluster of integrals More... | |
virtual double | estimate (int64_t a, int64_t b) const |
compute estimate of squared norm of a shellset/cluster of integrals More... | |
virtual double | estimate (int64_t a, int64_t b, int64_t c) const |
compute estimate of squared norm of a shellset/cluster of integrals More... | |
virtual double | estimate (int64_t a, int64_t b, int64_t c, int64_t d) const |
compute estimate of squared norm of a shellset/cluster of integrals More... | |
virtual double | estimate_shell (int64_t a) const |
compute estimate of squared norm of a shellset/cluster of integrals More... | |
virtual double | estimate_shell (int64_t a, int64_t b) const |
compute estimate of squared norm of a shellset/cluster of integrals More... | |
virtual double | estimate_shell (int64_t a, int64_t b, int64_t c) const |
compute estimate of squared norm of a shellset/cluster of integrals More... | |
virtual double | estimate_shell (int64_t a, int64_t b, int64_t c, int64_t d) const |
compute estimate of squared norm of a shellset/cluster of integrals More... | |
virtual double | estimate_cluster (int64_t a) const |
compute estimate of squared norm of a shellset/cluster of integrals More... | |
virtual double | estimate_cluster (int64_t a, int64_t b) const |
compute estimate of squared norm of a shellset/cluster of integrals More... | |
virtual double | estimate_cluster (int64_t a, int64_t b, int64_t c) const |
compute estimate of squared norm of a shellset/cluster of integrals More... | |
virtual double | estimate_cluster (int64_t a, int64_t b, int64_t c, int64_t d) const |
compute estimate of squared norm of a shellset/cluster of integrals More... | |
overrides of Screener::skip{,_shell,_cluster} functions | |
bool | skip (int64_t) const override |
bool | skip (int64_t, int64_t) const override |
bool | skip (int64_t, int64_t, int64_t) const override |
bool | skip (int64_t a, int64_t b, int64_t c, double D) const override |
bool | skip (int64_t, int64_t, int64_t, int64_t) const override |
bool | skip (int64_t, int64_t, int64_t, int64_t, double) const override |
bool | skip_shell (int64_t) const override |
bool | skip_shell (int64_t, int64_t) const override |
bool | skip_shell (int64_t, int64_t, int64_t) const override |
bool | skip_shell (int64_t a, int64_t b, int64_t c, double D) const override |
bool | skip_shell (int64_t, int64_t, int64_t, int64_t) const override |
bool | skip_shell (int64_t, int64_t, int64_t, int64_t, double D) const override |
bool | skip_cluster (int64_t) const override |
bool | skip_cluster (int64_t, int64_t) const override |
bool | skip_cluster (int64_t, int64_t, int64_t) const override |
bool | skip_cluster (int64_t a, int64_t b, int64_t c, double D) const override |
bool | skip_cluster (int64_t, int64_t, int64_t, int64_t) const override |
bool | skip_cluster (int64_t, int64_t, int64_t, int64_t, double D) const override |
![]() | |
ThresholdedScreener ()=default | |
ThresholdedScreener (ThresholdedScreener const &)=default | |
ThresholdedScreener (ThresholdedScreener &&)=default | |
ThresholdedScreener & | operator= (ThresholdedScreener &&)=default |
ThresholdedScreener & | operator= (ThresholdedScreener const &)=default |
virtual | ~ThresholdedScreener () noexcept=default |
ThresholdedScreener (double thresh, double thresh_cluster) | |
ThresholdedScreener (double thresh) | |
virtual double | skip_threshold () const |
virtual double | skip_threshold_cluster () const |
![]() | |
Screener ()=default | |
Screener (Screener const &)=default | |
Screener (Screener &&)=default | |
Screener & | operator= (Screener &&)=default |
Screener & | operator= (Screener const &)=default |
virtual | ~Screener () noexcept=default |
Protected Member Functions | |
double | skip_threshold_squared () const |
double | skip_threshold_cluster_squared () const |
template<typename... IDX> | |
bool | skip_ (IDX &&... idx) const |
template<typename... IDX> | |
bool | skip_shell_ (IDX &&... idx) const |
template<typename... IDX> | |
bool | skip_cluster_ (IDX &&... idx) const |
template<typename... IDX> | |
bool | skip_ (double D, IDX... idx) const |
template<typename... IDX> | |
bool | skip_shell_ (double D, IDX... idx) const |
template<typename... IDX> | |
bool | skip_cluster_ (double D, IDX... idx) const |
Member Typedef Documentation
◆ norm_op_type
Constructor & Destructor Documentation
◆ SchwarzScreen() [1/5]
|
default |
◆ SchwarzScreen() [2/5]
|
default |
◆ SchwarzScreen() [3/5]
|
default |
◆ ~SchwarzScreen()
|
virtualdefaultnoexcept |
◆ SchwarzScreen() [4/5]
mpqc::lcao::gaussian::SchwarzScreen::SchwarzScreen | ( | std::shared_ptr< Q2Matrix > | Q2bra, |
std::shared_ptr< Q2Matrix > | Q2ket, | ||
double | thresh, | ||
double | thresh_cluster | ||
) |
Constructor which requires two Q matrices.
- Parameters
-
[in] Q2bra is the Q2Matrix that describes the bra in screened integrals, e.g. it represents (cd| in (ab|cd), or (X| in (X|cd) [in] Q2ket is the Q2Matrix that describes the ket in screened integrals, e.g. it represents |cd) in (ab|cd), or |X) in (ab|X) [in] thresh is the screening threshold used when evaluating whether or not the skip() or skip_shell() function returns true for Q2bra.value(a,b)*Q2ket.value(c,d)<=skip_threshold()
. The norm types ofQ2bra
andQ2ket
are defined by their constructors.[in] thresh_cluster is the screening threshold used when evaluating whether or not the skip_cluster() function returns true for Q2bra.value_cluster(a,b)*Q2ket.value_cluster(c,d)<=skip_threshold_cluster()
. The norm types ofQ2bra
andQ2ket
are defined by their constructors.
◆ SchwarzScreen() [5/5]
mpqc::lcao::gaussian::SchwarzScreen::SchwarzScreen | ( | std::shared_ptr< Q2Matrix > | Q2bra, |
std::shared_ptr< Q2Matrix > | Q2ket, | ||
double | thresh | ||
) |
The ctor version that uses same threshold for shell and cluster skipping.
Member Function Documentation
◆ basis()
const std::shared_ptr< const Basis > & mpqc::lcao::gaussian::SchwarzScreen::basis | ( | std::size_t | i = 0 | ) | const |
- Parameters
-
[in] i basis index, any value between [0, nbases())
- Returns
- basis
i
◆ estimate() [1/4]
|
virtual |
compute estimate of squared norm of a shellset/cluster of integrals
◆ estimate() [2/4]
|
virtual |
compute estimate of squared norm of a shellset/cluster of integrals
Reimplemented in mpqc::lcao::gaussian::SQVlScreen.
◆ estimate() [3/4]
|
virtual |
compute estimate of squared norm of a shellset/cluster of integrals
Reimplemented in mpqc::lcao::gaussian::SQVlScreen.
◆ estimate() [4/4]
|
virtual |
compute estimate of squared norm of a shellset/cluster of integrals
◆ estimate_cluster() [1/4]
|
virtual |
compute estimate of squared norm of a shellset/cluster of integrals
◆ estimate_cluster() [2/4]
|
virtual |
compute estimate of squared norm of a shellset/cluster of integrals
◆ estimate_cluster() [3/4]
|
virtual |
compute estimate of squared norm of a shellset/cluster of integrals
◆ estimate_cluster() [4/4]
|
virtual |
compute estimate of squared norm of a shellset/cluster of integrals
◆ estimate_shell() [1/4]
|
virtual |
compute estimate of squared norm of a shellset/cluster of integrals
◆ estimate_shell() [2/4]
|
virtual |
compute estimate of squared norm of a shellset/cluster of integrals
Reimplemented in mpqc::lcao::gaussian::SQVlScreen.
◆ estimate_shell() [3/4]
|
virtual |
compute estimate of squared norm of a shellset/cluster of integrals
Reimplemented in mpqc::lcao::gaussian::SQVlScreen.
◆ estimate_shell() [4/4]
|
virtual |
compute estimate of squared norm of a shellset/cluster of integrals
◆ nbases()
std::size_t mpqc::lcao::gaussian::SchwarzScreen::nbases | ( | ) | const |
- Returns
- number of basis sets
◆ norm_estimate()
|
overridevirtual |
returns an estimate of shape norms for the given basis vector, in presence of symmetry described by a math::PetiteList object.
This function will only compute the estimate for tiles which are considered local by the pmap, the user will be responsible for using the world based constructor for the TA::Shape.
Reimplemented from mpqc::lcao::Screener.
◆ norm_op()
|
inline |
- Returns
- the norm op function
◆ operator=() [1/2]
|
default |
◆ operator=() [2/2]
|
default |
◆ Q2bra()
|
inline |
Returns the screening matrix for the Bra indices.
◆ Q2ket()
|
inline |
Returns the screening matrix for the Ket indices.
◆ set_skip_threshold()
|
overridevirtual |
Sets the threshold being used for skipping shell-sets ofintegrals
- Parameters
-
[in] thresh the threshold to be used to skipping shell-sets of integrals
Reimplemented from mpqc::lcao::ThresholdedScreener.
◆ set_skip_threshold_cluster()
|
overridevirtual |
Sets the threshold being used for skipping cluster-sets ofintegrals
- Parameters
-
[in] thresh the threshold to be used to skipping cluster-sets of integrals
Reimplemented from mpqc::lcao::ThresholdedScreener.
◆ skip() [1/6]
|
overridevirtual |
Reimplemented from mpqc::lcao::Screener.
◆ skip() [2/6]
|
overridevirtual |
Reimplemented from mpqc::lcao::Screener.
◆ skip() [3/6]
|
overridevirtual |
Reimplemented from mpqc::lcao::Screener.
Reimplemented in mpqc::lcao::gaussian::SQVlScreen.
◆ skip() [4/6]
|
overridevirtual |
Reimplemented from mpqc::lcao::Screener.
Reimplemented in mpqc::lcao::gaussian::SQVlScreen.
◆ skip() [5/6]
|
overridevirtual |
Reimplemented from mpqc::lcao::Screener.
◆ skip() [6/6]
|
overridevirtual |
Reimplemented from mpqc::lcao::Screener.
◆ skip_() [1/2]
|
inlineprotected |
◆ skip_() [2/2]
|
inlineprotected |
◆ skip_cluster() [1/6]
|
overridevirtual |
Reimplemented from mpqc::lcao::Screener.
◆ skip_cluster() [2/6]
|
overridevirtual |
Reimplemented from mpqc::lcao::Screener.
◆ skip_cluster() [3/6]
|
overridevirtual |
Reimplemented from mpqc::lcao::Screener.
◆ skip_cluster() [4/6]
|
overridevirtual |
Reimplemented from mpqc::lcao::Screener.
◆ skip_cluster() [5/6]
|
overridevirtual |
Reimplemented from mpqc::lcao::Screener.
◆ skip_cluster() [6/6]
|
overridevirtual |
Reimplemented from mpqc::lcao::Screener.
◆ skip_cluster_() [1/2]
|
inlineprotected |
◆ skip_cluster_() [2/2]
|
inlineprotected |
◆ skip_shell() [1/6]
|
overridevirtual |
Reimplemented from mpqc::lcao::Screener.
◆ skip_shell() [2/6]
|
overridevirtual |
Reimplemented from mpqc::lcao::Screener.
◆ skip_shell() [3/6]
|
overridevirtual |
Reimplemented from mpqc::lcao::Screener.
Reimplemented in mpqc::lcao::gaussian::SQVlScreen.
◆ skip_shell() [4/6]
|
overridevirtual |
Reimplemented from mpqc::lcao::Screener.
Reimplemented in mpqc::lcao::gaussian::SQVlScreen.
◆ skip_shell() [5/6]
|
overridevirtual |
Reimplemented from mpqc::lcao::Screener.
◆ skip_shell() [6/6]
|
overridevirtual |
Reimplemented from mpqc::lcao::Screener.
◆ skip_shell_() [1/2]
|
inlineprotected |
◆ skip_shell_() [2/2]
|
inlineprotected |
◆ skip_threshold_cluster_squared()
|
inlineprotected |
◆ skip_threshold_squared()
|
inlineprotected |
The documentation for this class was generated from the following files:
- mpqc/chemistry/qc/lcao/integrals/screening/schwarz.h
- mpqc/chemistry/qc/lcao/integrals/screening/schwarz.cpp