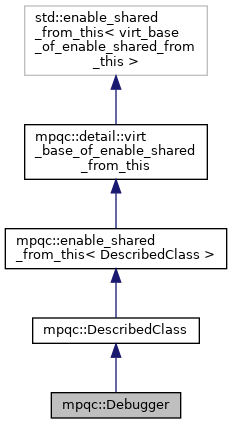
Documentation
The Debugger class describes what should be done when a catastrophic error causes unexpected program termination. It can try things such as start a debugger running where the program died or it can attempt to produce a stack traceback showing roughly where the program died. These attempts will not always succeed.
Public Member Functions | |
Debugger (const KeyVal &) | |
The KeyVal constructor. More... | |
Debugger (const char *exec=0) | |
Programmatic construction of Debugger. More... | |
~Debugger () | |
virtual void | debug (const char *reason=0) |
virtual void | traceback (const char *reason=0) |
virtual void | set_debug_on_signal (int) |
Turn on or off debugging on a signel. The default is on. More... | |
virtual void | set_traceback_on_signal (int) |
Turn on or off traceback on a signel. The default is on. More... | |
virtual void | set_exit_on_signal (int) |
Turn on or off exit after a signel. The default is on. More... | |
virtual void | set_wait_for_debugger (int) |
virtual void | handle (int sig) |
The Debugger will be actived when sig is caught. More... | |
virtual void | release (int sig) |
virtual void | handle_defaults () |
This calls handle(int) with all of the major signals. More... | |
virtual void | set_prefix (const char *p) |
This sets a prefix which preceeds all messages printing by Debugger. More... | |
virtual void | set_prefix (int p) |
Set the prefix to the decimal represention of p followed by a ": ". More... | |
virtual void | set_cmd (const char *) |
virtual void | default_cmd () |
Calls set_cmd with a hopefully suitable default. More... | |
virtual void | set_exec (const char *) |
virtual void | got_signal (int sig) |
Called when signal sig is received. This is mainly for internal use. More... | |
![]() | |
DescribedClass ()=default | |
virtual | ~DescribedClass () |
std::string | class_key () const |
![]() | |
virtual | ~enable_shared_from_this ()=default |
std::shared_ptr< DescribedClass > | shared_from_this () |
returns the pointer to this object More... | |
std::shared_ptr< std::add_const_t< DescribedClass > > | shared_from_this () const |
returns the pointer to this object More... | |
![]() | |
virtual | ~virt_base_of_enable_shared_from_this ()=default |
bool | shared_from_this_possible () const |
template<typename Target , typename = std::enable_if_t<!std::is_const_v<Target>>> | |
std::shared_ptr< Target > | cast_shared_from_this_to () |
returns the pointer to this cast to a particular type More... | |
template<typename Target > | |
std::shared_ptr< std::add_const_t< Target > > | cast_shared_from_this_to () const |
returns the pointer to this cast to a particular type More... | |
Static Public Member Functions | |
static void | set_default_debugger (const std::shared_ptr< Debugger > &) |
Set the global default debugger. The initial value is null. More... | |
static std::shared_ptr< Debugger > | default_debugger () |
Return the global default debugger. More... | |
![]() | |
static keyval_ctor_wrapper_type | type_to_keyval_ctor (const std::string &type_name) |
template<typename T > | |
static void | register_keyval_ctor () |
template<typename T > | |
static bool | is_registered () |
template<typename T > | |
static std::string | class_key () |
static const keyval_ctor_registry_type & | keyval_ctor_registry () |
returns const ref to the keyval ctor registry More... | |
Protected Member Functions | |
void | init () |
Static Protected Member Functions | |
static void | __traceback (const std::string &prefix, const char *reason=0) |
Protected Attributes | |
std::string | prefix_ |
std::string | exec_ |
std::string | cmd_ |
volatile int | debugger_ready_ |
bool | debug_ |
bool | traceback_ |
bool | exit_on_signal_ |
bool | sleep_ |
bool | wait_for_debugger_ |
bool | handle_sigint_ |
std::unique_ptr< int[]> | mysigs_ |
Static Protected Attributes | |
static std::shared_ptr< Debugger > | default_debugger_ |
Additional Inherited Members | |
![]() | |
typedef std::shared_ptr< DescribedClass >(* | keyval_ctor_wrapper_type) (const KeyVal &) |
Constructor & Destructor Documentation
◆ Debugger() [1/2]
mpqc::Debugger::Debugger | ( | const KeyVal & | keyval | ) |
The KeyVal constructor.
The KeyVal object will be queried for the following keywords:
debug
Try to start a debugger when an error occurs. Doesn't work on all machines. The default is true, if possible.
traceback
Try to print out a traceback extracting return addresses from the call stack. Doesn't work on most machines. The default is true, if possible.
exit
Exit on errors. The default is true.
wait_for_debugger
When starting a debugger go into an infinite loop to give the debugger a chance to attach to the process. The default is true.
sleep
When starting a debugger wait this many seconds to give the debugger a chance to attach to the process. The default is 0.
handle_defaults
Handle a standard set of signals such as SIGBUS, SIGSEGV, etc. The default is true.
prefix
Gives a string that is printed before each line that is printed by Debugger. The default is nothing.
cmd
Gives a command to be executed to start the debugger. If the environment variable
DISPLAY
is not set, the default is to skip launching the debugger, and to spin in a loop until the user attaches debugger to it manually. IfDISPLAY
is set, the default command is set tolldb_xterm
if MPQC compiled with Clang compiler, orgdb_xterm
otherwise, wherelldb_xterm
andgdb_xterm
are aliases forxterm -title "" -e lldb -p &
andxterm -title "" -e gdb &
, respectively; tokenis replaced by the value of keyword
prefix
,is replaced by the name of the current executable, and
is the process ID of each process. These commands launch one
xterm
per MPI rank, each running anlldb
orgdb
instance.
◆ Debugger() [2/2]
mpqc::Debugger::Debugger | ( | const char * | exec = 0 | ) |
◆ ~Debugger()
mpqc::Debugger::~Debugger | ( | ) |
Member Function Documentation
◆ __traceback()
|
staticprotected |
prints out a backtrace
- Parameters
-
prefix this string will be prepended at the beginning of each line of Backtrace reason optional string specifying the reason for traceback
- Returns
- backtrace
◆ debug()
|
virtual |
The debug member attempts to start a debugger running on the current process.
◆ default_cmd()
|
virtual |
Calls set_cmd with a hopefully suitable default.
◆ default_debugger()
|
static |
Return the global default debugger.
◆ got_signal()
|
virtual |
Called when signal sig is received. This is mainly for internal use.
◆ handle()
|
virtual |
The Debugger will be actived when sig is caught.
◆ handle_defaults()
|
virtual |
This calls handle(int) with all of the major signals.
◆ init()
|
protected |
◆ release()
|
virtual |
Reverts the effect of handle(sig)
, i.e. the Debugger will not be activated when sig
is caught.
◆ set_cmd()
|
virtual |
Sets the command to be exectuted when debug is called. The character sequence "$(EXEC)" is replaced by the executable name (see set_exec), "$(PID)" is replaced by the current process id, and "$(PREFIX)" is replaced by the prefix.
◆ set_debug_on_signal()
|
virtual |
Turn on or off debugging on a signel. The default is on.
◆ set_default_debugger()
|
static |
Set the global default debugger. The initial value is null.
◆ set_exec()
|
virtual |
◆ set_exit_on_signal()
|
virtual |
Turn on or off exit after a signel. The default is on.
◆ set_prefix() [1/2]
|
virtual |
This sets a prefix which preceeds all messages printing by Debugger.
◆ set_prefix() [2/2]
|
virtual |
Set the prefix to the decimal represention of p followed by a ": ".
◆ set_traceback_on_signal()
|
virtual |
Turn on or off traceback on a signel. The default is on.
◆ set_wait_for_debugger()
|
virtual |
Turn on or off running an infinite loop after the debugger is started. This loop gives the debugger a chance to attack to the process. The default is on.
◆ traceback()
|
virtual |
The traceback member attempts to produce a Backtrace for the current process. A symbol table must be saved for the executable if any sense is to be made of the traceback. This feature is available on platforms with (1) libunwind, (2) backtrace, or (3) certain platforms with hardwired unwinding.
- Parameters
-
reason optional string specifying the reason for traceback
Member Data Documentation
◆ cmd_
|
protected |
◆ debug_
|
protected |
◆ debugger_ready_
|
protected |
◆ default_debugger_
|
staticprotected |
◆ exec_
|
protected |
◆ exit_on_signal_
|
protected |
◆ handle_sigint_
|
protected |
◆ mysigs_
|
protected |
◆ prefix_
|
protected |
◆ sleep_
|
protected |
◆ traceback_
|
protected |
◆ wait_for_debugger_
|
protected |
The documentation for this class was generated from the following files: