mpqc::lcao::gaussian::AtomicBasis Class Reference
Collaboration diagram for mpqc::lcao::gaussian::AtomicBasis:
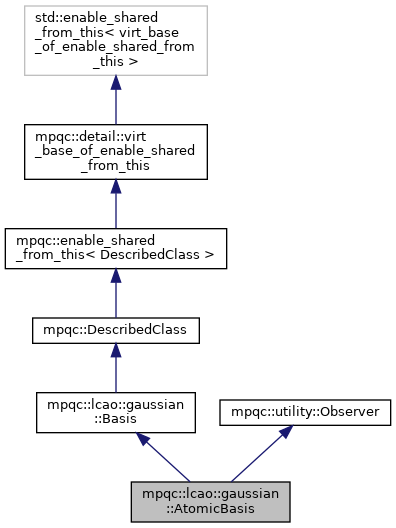
Documentation
AtomicBasis is a Basis constructed from a collection of atoms, i.e. a Molecule( or a UnitCell). Updating the Molecule object updates this basis as well. Clustering of AtomicBasis does not have to correspond to the cluster structure of Molecule (e.g. as a result of reblocking; furthermore, Molecule is clustered recursively, whereas Basis is nonrecursive).
- Warning
- Shells currently appear in the order of increasing atom index (see Molecule::atoms() for the definition of atom index). However, this may change in the future and should not be assumed in new code.
Public Types | |
using | Shell = ::mpqc::lcao::gaussian::Shell |
![]() | |
using | Shell = ::mpqc::lcao::gaussian::Shell |
![]() | |
typedef std::shared_ptr< DescribedClass >(* | keyval_ctor_wrapper_type) (const KeyVal &) |
Public Member Functions | |
AtomicBasis (const KeyVal &kv) | |
the KeyVal constructor for AtomicBasis More... | |
AtomicBasis (const std::shared_ptr< const Molecule > &atoms, const std::string &name, madness::World *world=mpqc::get_default_world(), int reblock_size=0) | |
contructs an AtomicBasis object from atoms + basis name More... | |
std::shared_ptr< const Factory > | factory () const |
std::shared_ptr< const Molecule > | atoms () const |
const std::string & | name () const |
const std::vector< std::vector< size_t > > & | shell_to_atom () const |
![]() | |
Basis () | |
creates an empty Basis More... | |
virtual | ~Basis () |
Basis (Basis const &) | |
Basis (Basis &&) | |
Basis & | operator= (Basis const &) |
Basis & | operator= (Basis &&) |
Basis (std::vector< ShellVec > shells) | |
construct a Basis from a clustered sequence of shells More... | |
std::vector< ShellVec > const & | cluster_shells () const |
ShellVec const & | cluster_shells (std::size_t cluster) const |
const std::vector< Shell > & | flattened_shells () const |
const std::vector< std::int64_t > & | bf2shell () const |
TiledArray::TiledRange1 | create_trange1 () const |
TiledArray::TiledRange1 | make_trange1 () const |
TiledArray::TiledRange1 | make_shell_trange1 () const |
TiledArray::TiledRange1 | make_trange1 (std::size_t cluster, std::size_t lobound=std::numeric_limits< std::size_t >::max()) const |
TiledArray::TiledRange1::range_type | make_range1 (std::size_t cluster, std::size_t lobound=std::numeric_limits< std::size_t >::max()) const |
int64_t | max_nprim () const |
int64_t | max_am () const |
int64_t | nfunctions () const |
int64_t | nshells () const |
int64_t | nclusters () const |
template<typename Archive > | |
std::enable_if< madness::archive::is_output_archive< Archive >::value >::type | serialize (const Archive &ar) |
template<typename Archive > | |
std::enable_if< madness::archive::is_input_archive< Archive >::value >::type | serialize (const Archive &ar) |
![]() | |
DescribedClass ()=default | |
virtual | ~DescribedClass () |
std::string | class_key () const |
![]() | |
virtual | ~enable_shared_from_this ()=default |
std::shared_ptr< DescribedClass > | shared_from_this () |
returns the pointer to this object More... | |
std::shared_ptr< std::add_const_t< DescribedClass > > | shared_from_this () const |
returns the pointer to this object More... | |
![]() | |
virtual | ~virt_base_of_enable_shared_from_this ()=default |
bool | shared_from_this_possible () const |
template<typename Target , typename = std::enable_if_t<!std::is_const_v<Target>>> | |
std::shared_ptr< Target > | cast_shared_from_this_to () |
returns the pointer to this cast to a particular type More... | |
template<typename Target > | |
std::shared_ptr< std::add_const_t< Target > > | cast_shared_from_this_to () const |
returns the pointer to this cast to a particular type More... | |
![]() | |
Observer ()=default | |
template<typename Observee > | |
Observer (Observee *observee, std::function< void()> message) | |
Observer (const Observer &other)=delete | |
Observer & | operator= (const Observer &other)=delete |
Observer (Observer &&other)=default | |
Observer & | operator= (Observer &&other)=default |
virtual | ~Observer () |
Additional Inherited Members | |
![]() | |
static keyval_ctor_wrapper_type | type_to_keyval_ctor (const std::string &type_name) |
template<typename T > | |
static void | register_keyval_ctor () |
template<typename T > | |
static bool | is_registered () |
template<typename T > | |
static std::string | class_key () |
static const keyval_ctor_registry_type & | keyval_ctor_registry () |
returns const ref to the keyval ctor registry More... | |
![]() | |
void | init_trange1_hashmarks () const |
![]() | |
template<typename Observee > | |
void | register_message (Observee *observee, std::function< void()> message) |
void | clear_messages () |
![]() | |
std::vector< ShellVec > | shells_ |
std::vector< std::size_t > | trange1_hashmarks_ |
std::vector< Shell > | flattened_shells_ |
std::vector< int64_t > | bf2shell_ |
int64_t | max_nprim_ = -1 |
int64_t | max_am_ = -1 |
int64_t | nfunctions_ = -1 |
int64_t | nshells_ = -1 |
Member Typedef Documentation
◆ Shell
Constructor & Destructor Documentation
◆ AtomicBasis() [1/2]
mpqc::lcao::gaussian::AtomicBasis::AtomicBasis | ( | const KeyVal & | kv | ) |
the KeyVal constructor for AtomicBasis
- Parameters
-
kv the KeyVal object. kv
will be queried for all keywords of Basis::Factory, as well as the following additional keywords:
Keyword | Type | Default | Description |
---|---|---|---|
"atoms" | Molecule or UnitCell | none | the centers |
"reblock" | int | 0 (i.e., no reblocking) | size to reblock basis |
|"$:world"
| pointer | the value returned by mpqc::get_default_world() | the default madness::World object to use |
◆ AtomicBasis() [2/2]
mpqc::lcao::gaussian::AtomicBasis::AtomicBasis | ( | const std::shared_ptr< const Molecule > & | atoms, |
const std::string & | name, | ||
madness::World * | world = mpqc::get_default_world() , |
||
int | reblock_size = 0 |
||
) |
contructs an AtomicBasis object from atoms + basis name
Member Function Documentation
◆ atoms()
std::shared_ptr< const Molecule > mpqc::lcao::gaussian::AtomicBasis::atoms | ( | ) | const |
◆ factory()
std::shared_ptr< const Basis::Factory > mpqc::lcao::gaussian::AtomicBasis::factory | ( | ) | const |
◆ name()
|
inline |
◆ shell_to_atom()
|
inline |
Maps shells to the ordinal index of the Atom on which it is located (see Molecule::atoms() for the definition of ordinal indices).
- Returns
- shell->atom map
- See also
- Molecule::atoms
- Warning
- Shells currently appear in the order of increasing atom index (see Molecule::atoms() for the definition of atom index). However, this may change in the future and should not be assumed in new code.
The documentation for this class was generated from the following files: