Search Results
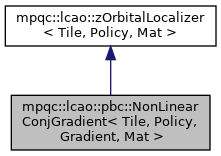
Documentation
template<typename Tile, typename Policy, typename Gradient, typename Mat>
class mpqc::lcao::pbc::NonLinearConjGradient< Tile, Policy, Gradient, Mat >
Implements non-linear conjugate gradient for a given gradient expression.
Public Member Functions | |
virtual ~NonLinearConjGradient ()=default | |
NonLinearConjGradient (const KeyVal &kv) | |
double inner_product (Mat const &bra_mat, Mat const &ket_mat) const | |
std::vector< Mat > rotate_W (const int nkpts, double mu, std::vector< Mat > const &Hn, std::vector< Mat > const &Wn) const | |
int find_max_idx (Eigen::VectorXd const &list, double const max_value) const | |
Eigen::VectorXd fit_data (Eigen::VectorXd const &mu_list, Eigen::VectorXd const &P_list) const | |
void evaluate_P_on_range (Eigen::VectorXd &mu_list, Eigen::VectorXd &P_list, const double lower_bound, const double upper_bound, const int p, const int nkpts, Eigen::Vector3i const &nk, std::vector< Mat > const &Hn, std::vector< Mat > const &Wn, std::vector< std::vector< Mat >> const &Q_list) const | |
double get_upper_bound (double const &lower_bound, double const &upper_bound, Eigen::VectorXd &mu_list, Eigen::VectorXd &P_list, const int p, const double P_initial, Eigen::Vector3i const &nk, std::vector< Mat > const &Hn, std::vector< Mat > const &Wn, std::vector< std::vector< Mat >> const &Q_list, const int nkpts) const | |
double solve_root (const double &lower_bound, double &upper_bound, const int p, const double P_initial, const int nkpts, Eigen::Vector3i const &nk, std::vector< Mat > const &Hn, std::vector< Mat > const &Wn, std::vector< std::vector< Mat >> const &Q_list) const | |
std::vector< Mat > update_W_fit_functional (const int nkpts, const int p, double const &lower_bound, double &upper_bound, double &P_initial, Eigen::Vector3i const &nk, std::vector< Mat > const &Hn, std::vector< Mat > const &Wn, std::vector< std::vector< Mat >> const &Q_list) const | |
std::vector< Mat > compute (std::vector< Mat > const &C, std::vector< Mat > &C_rotated, Eigen::Vector3i const &nk, int64_t nocc, size_t ncols_of_C_to_skip=0) const final | |
![]() | |
virtual ~zOrbitalLocalizer ()=default | |
Protected Member Functions | |
void init (std::shared_ptr< detail::zOrbitalLocalizationGradient< Mat >> gradient) | |
NonLinearConjGradient (int max_iter, double target_precision) | |
Protected Attributes | |
std::shared_ptr< WavefunctionWorld > wfn_world_ | |
std::shared_ptr< lcao::pbc::gaussian::AOFactory< TileD, Policy > > ao_factory_ | |
double convergence_threshold_ | |
size_t max_iter_ | |
std::string update_factor_ | |
std::string initial_W_ | |
std::shared_ptr< detail::zOrbitalLocalizationGradient< Mat > > gradient_ | |
Constructor & Destructor Documentation
◆ ~NonLinearConjGradient()
|
virtualdefault |
◆ NonLinearConjGradient() [1/2]
|
inlineexplicit |
KeyVal constructor for NonLinearConjGradient
- Parameters
-
kv the KeyVal object; it will be queried for the following keywords: Keyword Type Default Description wfn_world
class none the WavefunctionWorld convergence_threshold
double 1e-8 the non-linear conjugate gradient solver is converged when the norm of the gradient falls below this value max_iter
int 50 the maximum number of iterations update_factor
string sdsa the means of forming gamma
◆ NonLinearConjGradient() [2/2]
|
inlineprotected |
Member Function Documentation
◆ compute()
|
inlinefinalvirtual |
- Parameters
-
C input LCAOs [in] ncols_of_C_to_skip the number of columns of C to keep non-localized, presumably because they are already localized
- Returns
- transformation matrix
U
that convertsC
to localized LCAOs, i.e.computes the AO coefficients of localized MOs from the AO coefficients of input MOs";Cao("mu,k") * U("k,i")
Step 1: Get the maximum of all omega_k
Step 2: Determine the order q of the cost function
Step 3: Determine the value of Tmu = 2 pi / (q * max_omega)
Step 1: Get the maximum of all omega_k
Step 2: Determine the order q of the cost function
Step 3: Determine the value of Tmu = 2 pi / (q * max_omega)
Implements mpqc::lcao::zOrbitalLocalizer< Tile, Policy, Mat >.
◆ evaluate_P_on_range()
|
inline |
Step 5: Evaluate R(mu) at each mu_i
Step 6: For each R(mu), evaluate the PM functional
◆ find_max_idx()
|
inline |
Given an Eigen::VectorXd of items and the max item in that vector, find the index of the max item
◆ fit_data()
|
inline |
◆ get_upper_bound()
|
inline |
◆ init()
|
inlineprotected |
◆ inner_product()
|
inline |
◆ rotate_W()
|
inline |
I believe that this is the correct one to use Given an appropriate double mu and vector of matrices Hn, generate the rotated W matrices Rotate each W with the same mu but a different H
◆ solve_root()
|
inline |
◆ update_W_fit_functional()
|
inline |
Compute the optimal step size, \mu_{opt} and update W by fitting the P(mu) values and then finding the roots of the first derivative of this function
- Parameters
-
[in] nkpts the number of k points [in] p the order of the polynomial [in] Hn the current iteration's search descent direction: one matrix for each k point [in] Wn the current iteration's estimate of the orbital transformation matrix: one matrix for each k point
- Returns
- mu_n a vector containing the current optimal step size for each k point
Member Data Documentation
◆ ao_factory_
|
protected |
◆ convergence_threshold_
|
protected |
◆ gradient_
|
protected |
◆ initial_W_
|
protected |
◆ max_iter_
|
protected |
◆ update_factor_
|
protected |
◆ wfn_world_
|
protected |
The documentation for this class was generated from the following file:
- mpqc/chemistry/qc/lcao/scf/pbc/zorbital_localization.h