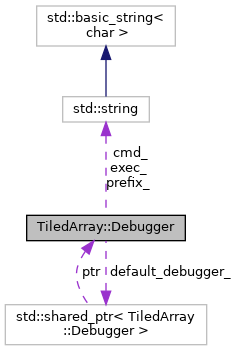
Documentation
The Debugger class describes what should be done when a catastrophic error causes unexpected program termination. It can try things such as start a debugger running where the program died or it can attempt to produce a stack traceback showing roughly where the program died. These attempts will not always succeed.
Public Member Functions | |
Debugger (const char *exec=nullptr) | |
Programmatic construction of Debugger. More... | |
virtual | ~Debugger () |
virtual void | debug (const char *reason) |
virtual void | traceback (const char *reason) |
virtual void | set_debug_on_signal (int) |
Turn on or off debugging on a signel. The default is on. More... | |
virtual void | set_traceback_on_signal (int) |
Turn on or off traceback on a signel. The default is on. More... | |
virtual void | set_exit_on_signal (int) |
Turn on or off exit after a signel. The default is on. More... | |
virtual void | set_wait_for_debugger (int) |
virtual void | handle (int sig) |
The Debugger will be activated when sig is caught. More... | |
virtual void | release (int sig) |
virtual void | handle_defaults () |
This calls handle(int) with all of the major signals. More... | |
virtual void | set_prefix (const char *p) |
This sets a prefix which preceeds all messages printing by Debugger. More... | |
virtual void | set_prefix (int p) |
Set the prefix to the decimal represention of p followed by a ": ". More... | |
virtual void | set_cmd (const char *) |
virtual void | default_cmd () |
Calls set_cmd with a hopefully suitable default. More... | |
virtual void | set_exec (const char *) |
virtual void | got_signal (int sig) |
Called when signal sig is received. This is mainly for internal use. More... | |
Static Public Member Functions | |
static void | set_default_debugger (const std::shared_ptr< Debugger > &) |
Set the global default debugger. The initial value is null. More... | |
static std::shared_ptr< Debugger > | default_debugger () |
Return the global default debugger. More... | |
Protected Member Functions | |
void | init () |
Static Protected Member Functions | |
static void | __traceback (const std::string &prefix, const char *reason=nullptr) |
Protected Attributes | |
std::string | prefix_ |
std::string | exec_ |
std::string | cmd_ |
volatile int | debugger_ready_ |
bool | debug_ |
bool | traceback_ |
bool | exit_on_signal_ |
bool | sleep_ |
bool | wait_for_debugger_ |
bool | handle_sigint_ |
int * | mysigs_ |
Static Protected Attributes | |
static std::shared_ptr< Debugger > | default_debugger_ |
Constructor & Destructor Documentation
◆ Debugger()
|
explicit |
◆ ~Debugger()
Member Function Documentation
◆ __traceback()
|
staticprotected |
prints out a backtrace
- Parameters
-
prefix this string will be prepended at the beginning of each line of Backtrace reason optional string specifying the reason for traceback
- Returns
- backtrace
Definition at line 328 of file bug.cpp.
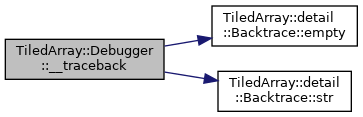

◆ debug()
|
virtual |
◆ default_cmd()
|
virtual |
◆ default_debugger()
|
static |
◆ got_signal()
|
virtual |
◆ handle()
|
virtual |
◆ handle_defaults()
|
virtual |
This calls handle(int) with all of the major signals.
Definition at line 120 of file bug.cpp.


◆ init()
|
protected |
◆ release()
|
virtual |
◆ set_cmd()
|
virtual |
Sets the command to be exectuted when debug is called. The character sequence "$(EXEC)" is replaced by the executable name (see set_exec), "$(PID)" is replaced by the current process id, and "$(PREFIX)" is replaced by the prefix.
Definition at line 194 of file bug.cpp.

◆ set_debug_on_signal()
|
virtual |
◆ set_default_debugger()
|
static |
◆ set_exec()
|
virtual |
◆ set_exit_on_signal()
|
virtual |
◆ set_prefix() [1/2]
|
virtual |
◆ set_prefix() [2/2]
|
virtual |
◆ set_traceback_on_signal()
|
virtual |
◆ set_wait_for_debugger()
|
virtual |
◆ traceback()
|
virtual |
The traceback member attempts to produce a Backtrace for the current process. A symbol table must be saved for the executable if any sense is to be made of the traceback. This feature is available on platforms with (1) libunwind, (2) backtrace, or (3) certain platforms with hardwired unwinding.
- Parameters
-
reason optional string specifying the reason for traceback
Definition at line 324 of file bug.cpp.
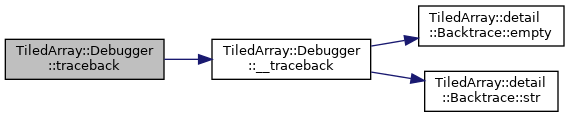

Member Data Documentation
◆ cmd_
◆ debug_
◆ debugger_ready_
◆ default_debugger_
|
staticprotected |
◆ exec_
◆ exit_on_signal_
◆ handle_sigint_
◆ mysigs_
◆ prefix_
◆ sleep_
◆ traceback_
◆ wait_for_debugger_
The documentation for this class was generated from the following files: