Namespaces | |
container | |
detail | |
eigen | |
expressions | |
math | |
meta | |
symmetry | |
tile_interface | |
Classes | |
class | AbsMaxReduction |
Maxabs tile reduction. More... | |
class | AbsMinReduction |
Minabs tile reduction. More... | |
class | Add |
Add tile operation. More... | |
class | AddTo |
Add-to tile operation. More... | |
class | BipartitePermutation |
Permutation of a bipartite set. More... | |
class | BlockRange |
Range that references a subblock of another range. More... | |
class | Cast |
Tile cast operation. More... | |
struct | Cast< TiledArray::Tensor< T, Allocator >, btas::Tensor< T, Range_, Storage > > |
converts a btas::Tensor to a TiledArray::Tensor More... | |
struct | Cast< TiledArray::Tensor< typename T::value_type, Allocator >, Tile< T >, std::void_t< decltype(std::declval< TiledArray::Cast< TiledArray::Tensor< typename T::value_type, Allocator >, T >>()(std::declval< const T & >()))> > |
class | Clone |
Create a deep copy of a tile. More... | |
struct | clone_trait |
Clone trait. More... | |
class | cpu_cuda_vector |
a vector that lives on either host or device side, or both More... | |
class | Debugger |
class | DensePolicy |
class | DenseShape |
Dense shape of an array. More... | |
class | DistArray |
Forward declarations. More... | |
class | DotReduction |
Vector dot product tile reduction. More... | |
struct | eval_trait |
Determine the object type used in the evaluation of tensor expressions. More... | |
class | Exception |
class | function_ref |
class | function_ref< R(Args...)> |
Specialization for function types. More... | |
struct | InitializerListRank |
Primary template for determining how many nested std::initializer_list's are in a type. More... | |
struct | InitializerListRank< std::initializer_list< T >, SizeType > |
Specialization of InitializerListRank used when the template type parameter is a std::initializer_list type. More... | |
class | InnerProductReduction |
Vector inner product tile reduction. More... | |
class | Irrep |
Irrep of an ![]() | |
struct | is_consumable_tile |
Consumable tile type trait. More... | |
struct | is_consumable_tile< ZeroTensor > |
struct | is_dense |
Type trait to detect dense shape types. More... | |
struct | is_dense< DensePolicy > |
struct | is_dense< DenseShape > |
struct | is_dense< DistArray< Tile, Policy > > |
struct | is_lazy_tile |
Detect lazy evaluation tiles. More... | |
struct | is_lazy_tile< DistArray< Tile, Policy > > |
class | MaxReduction |
Maximum tile reduction. More... | |
class | MaxReduction< Tile, typename std::enable_if< detail::is_strictly_ordered< detail::numeric_t< Tile > >::value >::type > |
class | MinReduction |
Minimum tile reduction. More... | |
class | MinReduction< Tile, typename std::enable_if< detail::is_strictly_ordered< detail::numeric_t< Tile > >::value >::type > |
class | Permutation |
Permutation of a sequence of objects indexed by base-0 indices. More... | |
class | Permute |
Permute a tile. More... | |
struct | permute_trait |
Permute trait. More... | |
class | Pmap |
Process map. More... | |
class | ProductReduction |
Tile product reduction. More... | |
class | Range |
A (hyperrectangular) interval on ![]() ![]() | |
class | ScalAdd |
Add tile operation. More... | |
class | ScalAddTo |
Add-to and scale tile operation. More... | |
class | Scale |
Scale tile. More... | |
struct | scale_trait |
Scale trait. More... | |
class | shared_function |
analogous to std::function, but has shallow-copy semantics More... | |
class | Shift |
Shift the range of tile. More... | |
class | ShiftTo |
Shift the range of tile in place. More... | |
class | Singleton |
Singleton base class To create a singleton class A do: More... | |
class | SparsePolicy |
class | SparseShape |
Frobenius-norm-based sparse shape. More... | |
class | SquaredNormReduction |
Squared norm tile reduction. More... | |
class | SumReduction |
Tile sum reduction. More... | |
class | Tensor |
An N-dimensional tensor object. More... | |
class | Tile |
An N-dimensional shallow copy wrapper for tile objects. More... | |
class | TiledRange |
Range data of a tiled array. More... | |
class | TiledRange1 |
struct | TileOpsLogger |
class | TraceReduction |
Tile trace reduction. More... | |
struct | ZeroTensor |
Place-holder object for a zero tensor. More... | |
Typedefs | |
typedef Eigen::Matrix< double, Eigen::Dynamic, Eigen::Dynamic, Eigen::RowMajor > | EigenMatrixXd |
typedef Eigen::Matrix< float, Eigen::Dynamic, Eigen::Dynamic, Eigen::RowMajor > | EigenMatrixXf |
typedef Eigen::Matrix< std::complex< double >, Eigen::Dynamic, Eigen::Dynamic, Eigen::RowMajor > | EigenMatrixXcd |
typedef Eigen::Matrix< std::complex< float >, Eigen::Dynamic, Eigen::Dynamic, Eigen::RowMajor > | EigenMatrixXcf |
typedef Eigen::Matrix< int, Eigen::Dynamic, Eigen::Dynamic, Eigen::RowMajor > | EigenMatrixXi |
typedef Eigen::Matrix< long, Eigen::Dynamic, Eigen::Dynamic, Eigen::RowMajor > | EigenMatrixXl |
typedef Eigen::Matrix< double, Eigen::Dynamic, 1 > | EigenVectorXd |
typedef Eigen::Matrix< float, Eigen::Dynamic, 1 > | EigenVectorXf |
typedef Eigen::Matrix< std::complex< double >, 1, Eigen::Dynamic > | EigenVectorXcd |
typedef Eigen::Matrix< std::complex< float >, 1, Eigen::Dynamic > | EigenVectorXcf |
typedef Eigen::Matrix< int, Eigen::Dynamic, 1 > | EigenVectorXi |
typedef Eigen::Matrix< long, Eigen::Dynamic, 1 > | EigenVectorXl |
template<typename T , typename Range_ = Range, typename OpResult = Tensor<T>> | |
using | TensorMap = detail::TensorInterface< T, Range_, OpResult > |
template<typename T , typename Range_ = Range, typename OpResult = Tensor<T>> | |
using | TensorConstMap = detail::TensorInterface< typename std::add_const< T >::type, Range_, OpResult > |
template<typename T > | |
using | TensorView = detail::TensorInterface< T, BlockRange > |
template<typename T > | |
using | TensorConstView = detail::TensorInterface< typename std::add_const< T >::type, BlockRange > |
template<typename T > | |
using | result_of_trace_t = decltype(trace(std::declval< T >())) |
template<typename... T> | |
using | result_of_subt_t = decltype(subt(std::declval< T >()...)) |
template<typename... T> | |
using | result_of_subt_to_t = decltype(subt_to(std::declval< T >()...)) |
template<typename... T> | |
using | result_of_mult_t = decltype(mult(std::declval< T >()...)) |
template<typename... T> | |
using | result_of_mult_to_t = decltype(mult_to(std::declval< T >()...)) |
template<typename... T> | |
using | result_of_binary_t = decltype(binary(std::declval< T >()...)) |
template<typename... T> | |
using | result_of_inplace_binary_t = decltype(inplace_binary(std::declval< T >()...)) |
template<typename... T> | |
using | result_of_neg_t = decltype(neg(std::declval< T >()...)) |
template<typename... T> | |
using | result_of_neg_to_t = decltype(neg_to(std::declval< T >()...)) |
template<typename... T> | |
using | result_of_conj_t = decltype(conj(std::declval< T >()...)) |
template<typename... T> | |
using | result_of_conj_to_t = decltype(conj_to(std::declval< T >()...)) |
template<typename... T> | |
using | result_of_unary_t = decltype(unary(std::declval< T >()...)) |
template<typename... T> | |
using | result_of_inplace_unary_t = decltype(inplace_unary(std::declval< T >()...)) |
template<typename... T> | |
using | result_of_gemm_t = decltype(gemm(std::declval< T >()...)) |
template<typename T > | |
using | result_of_sum_t = decltype(sum(std::declval< T >())) |
template<typename T > | |
using | result_of_product_t = decltype(product(std::declval< T >())) |
template<typename T > | |
using | result_of_squared_norm_t = decltype(squared_norm(std::declval< T >())) |
template<typename T , typename ResultType = T> | |
using | result_of_norm_t = decltype(norm(std::declval< T >(), std::declval< ResultType & >())) |
template<typename T > | |
using | result_of_max_t = decltype(max(std::declval< T >())) |
template<typename T > | |
using | result_of_min_t = decltype(min(std::declval< T >())) |
template<typename T > | |
using | result_of_abs_max_t = decltype(abs_max(std::declval< T >())) |
template<typename T > | |
using | result_of_abs_min_t = decltype(abs_min(std::declval< T >())) |
template<typename L , typename R > | |
using | result_of_dot_t = decltype(dot(std::declval< L >(), std::declval< R >())) |
using | time_point = std::chrono::high_resolution_clock::time_point |
typedef Tensor< double, Eigen::aligned_allocator< double > > | TensorD |
typedef Tensor< int, Eigen::aligned_allocator< int > > | TensorI |
typedef Tensor< float, Eigen::aligned_allocator< float > > | TensorF |
typedef Tensor< long, Eigen::aligned_allocator< long > > | TensorL |
typedef Tensor< std::complex< double >, Eigen::aligned_allocator< std::complex< double > > > | TensorZ |
typedef Tensor< std::complex< float >, Eigen::aligned_allocator< std::complex< float > > > | TensorC |
template<typename T > | |
using | TArray = DistArray< Tensor< T, Eigen::aligned_allocator< T > >, DensePolicy > |
typedef TArray< double > | TArrayD |
typedef TArray< int > | TArrayI |
typedef TArray< float > | TArrayF |
typedef TArray< long > | TArrayL |
typedef TArray< std::complex< double > > | TArrayZ |
typedef TArray< std::complex< float > > | TArrayC |
template<typename T > | |
using | TSpArray = DistArray< Tensor< T, Eigen::aligned_allocator< T > >, SparsePolicy > |
typedef TSpArray< double > | TSpArrayD |
typedef TSpArray< int > | TSpArrayI |
typedef TSpArray< float > | TSpArrayF |
typedef TSpArray< long > | TSpArrayL |
typedef TSpArray< std::complex< double > > | TSpArrayZ |
typedef TSpArray< std::complex< float > > | TSpArrayC |
template<typename T , unsigned int = 0, typename Tile = Tensor<T, Eigen::aligned_allocator<T> >, typename Policy = DensePolicy> | |
using | Array = DistArray< Tile, Policy > |
Enumerations | |
enum | ShapeReductionMethod { ShapeReductionMethod::Union, ShapeReductionMethod::Intersect } |
enum | MemorySpace { MemorySpace::Null = 0b00, MemorySpace::CPU = 0b01, MemorySpace::CUDA = 0b10, MemorySpace::CUDA_UM = CPU | CUDA } |
enumerates the memory spaces More... | |
enum | ExecutionSpace { ExecutionSpace::CPU, ExecutionSpace::CUDA } |
enumerates the execution spaces More... | |
enum | OrdinalType { OrdinalType::RowMajor = -1, OrdinalType::ColMajor = 1, OrdinalType::Other = 0, OrdinalType::Invalid } |
Functions | |
bool | is_congruent (const BlockRange &r1, const BlockRange &r2) |
Test that two BlockRange objects are congruent. More... | |
bool | is_congruent (const BlockRange &r1, const Range &r2) |
Test that BlockRange and Range are congruent. More... | |
bool | is_congruent (const Range &r1, const BlockRange &r2) |
Test that Range and BlockRange are congruent. More... | |
bool | operator== (const BlockRange &r1, const BlockRange &r2) |
BlockRange equality comparison. More... | |
bool | is_contiguous (const BlockRange &range) |
template<typename T , typename Range_ , typename Storage_ , typename Tensor_ > | |
void | btas_subtensor_to_tensor (const btas::Tensor< T, Range_, Storage_ > &src, Tensor_ &dst) |
Copy a block of a btas::Tensor into a TiledArray::Tensor. More... | |
template<typename Tensor_ , typename T , typename Range_ , typename Storage_ > | |
void | tensor_to_btas_subtensor (const Tensor_ &src, btas::Tensor< T, Range_, Storage_ > &dst) |
Copy a block of a btas::Tensor into a TiledArray::Tensor. More... | |
template<typename DistArray_ , typename T , typename Range , typename Storage > | |
DistArray_ | btas_tensor_to_array (World &world, const TiledArray::TiledRange &trange, const btas::Tensor< T, Range, Storage > &src, bool replicated=false) |
Convert a btas::Tensor object into a TiledArray::DistArray object. More... | |
template<typename Tile , typename Policy , typename Storage = std::vector<typename Tile::value_type>> | |
btas::Tensor< typename Tile::value_type, btas::DEFAULT::range, Storage > | array_to_btas_tensor (const TiledArray::DistArray< Tile, Policy > &src, int target_rank=-1) |
Convert a TiledArray::DistArray object into a btas::Tensor object. More... | |
template<typename Tile , typename Policy > | |
DistArray< Tile, Policy > | clone (const DistArray< Tile, Policy > &arg) |
Create a deep copy of an array. More... | |
template<typename Tile , typename ResultPolicy = SparsePolicy, typename ArgPolicy > | |
std::enable_if_t<!is_dense_v< ResultPolicy > &&is_dense_v< ArgPolicy >, DistArray< Tile, ResultPolicy > > | to_sparse (DistArray< Tile, ArgPolicy > const &dense_array) |
Function to convert a dense array into a block sparse array. More... | |
template<typename Tile , typename Policy > | |
std::enable_if_t<!is_dense_v< Policy >, DistArray< Tile, Policy > > | to_sparse (DistArray< Tile, Policy > const &sparse_array) |
If the array is already sparse return a copy of the array. More... | |
template<typename T , Eigen::StorageOptions Storage = Eigen::RowMajor, std::enable_if_t< detail::is_contiguous_tensor_v< T >> * = nullptr> | |
Eigen::Map< const Eigen::Matrix< typename T::value_type, Eigen::Dynamic, Eigen::Dynamic, Storage >, Eigen::AutoAlign > | eigen_map (const T &tensor, const std::size_t m, const std::size_t n) |
Construct a const Eigen::Map object for a given Tensor object. More... | |
template<typename T , Eigen::StorageOptions Storage = Eigen::RowMajor, std::enable_if_t< detail::is_contiguous_tensor_v< T >> * = nullptr> | |
Eigen::Map< Eigen::Matrix< typename T::value_type, Eigen::Dynamic, Eigen::Dynamic, Storage >, Eigen::AutoAlign > | eigen_map (T &tensor, const std::size_t m, const std::size_t n) |
Construct an Eigen::Map object for a given Tensor object. More... | |
template<typename T , std::enable_if_t< detail::is_contiguous_tensor_v< T >> * = nullptr> | |
Eigen::Map< const Eigen::Matrix< typename T::value_type, Eigen::Dynamic, 1 >, Eigen::AutoAlign > | eigen_map (const T &tensor, const std::size_t n) |
Construct a const Eigen::Map object for a given Tensor object. More... | |
template<typename T , std::enable_if_t< detail::is_contiguous_tensor_v< T >> * = nullptr> | |
Eigen::Map< Eigen::Matrix< typename T::value_type, Eigen::Dynamic, 1 >, Eigen::AutoAlign > | eigen_map (T &tensor, const std::size_t n) |
Construct an Eigen::Map object for a given Tensor object. More... | |
template<typename T , Eigen::StorageOptions Storage = Eigen::RowMajor, std::enable_if_t< detail::is_contiguous_tensor_v< T >> * = nullptr> | |
Eigen::Map< const Eigen::Matrix< typename T::value_type, Eigen::Dynamic, Eigen::Dynamic, Storage >, Eigen::AutoAlign > | eigen_map (const T &tensor) |
Construct a const Eigen::Map object for a given Tensor object. More... | |
template<typename T , Eigen::StorageOptions Storage = Eigen::RowMajor, std::enable_if_t< detail::is_contiguous_tensor_v< T >> * = nullptr> | |
Eigen::Map< Eigen::Matrix< typename T::value_type, Eigen::Dynamic, Eigen::Dynamic, Storage >, Eigen::AutoAlign > | eigen_map (T &tensor) |
Construct an Eigen::Map object for a given Tensor object. More... | |
template<typename T , typename Derived , std::enable_if_t< detail::is_contiguous_tensor_v< T >> * = nullptr> | |
void | eigen_submatrix_to_tensor (const Eigen::MatrixBase< Derived > &matrix, T &tensor) |
Copy a block of an Eigen matrix into a tensor. More... | |
template<typename T , typename Derived , std::enable_if_t< detail::is_contiguous_tensor_v< T >> * = nullptr> | |
void | tensor_to_eigen_submatrix (const T &tensor, Eigen::MatrixBase< Derived > &matrix) |
Copy the content of a tensor into an Eigen matrix block. More... | |
template<typename A , typename Derived > | |
A | eigen_to_array (World &world, const typename A::trange_type &trange, const Eigen::MatrixBase< Derived > &matrix, bool replicated=false, std::shared_ptr< typename A::pmap_interface > pmap={}) |
Convert an Eigen matrix into an Array object. More... | |
template<typename Tile , typename Policy , unsigned int EigenStorageOrder = Eigen::ColMajor> | |
Eigen::Matrix< typename Tile::value_type, Eigen::Dynamic, Eigen::Dynamic, EigenStorageOrder > | array_to_eigen (const DistArray< Tile, Policy > &array) |
Convert an Array object into an Eigen matrix object. More... | |
template<typename A > | |
A | row_major_buffer_to_array (World &world, const typename A::trange_type &trange, const typename A::value_type::value_type *buffer, const std::size_t m, const std::size_t n, const bool replicated=false, std::shared_ptr< typename A::pmap_interface > pmap={}) |
Convert a row-major matrix buffer into an Array object. More... | |
template<typename A > | |
A | column_major_buffer_to_array (World &world, const typename A::trange_type &trange, const typename A::value_type::value_type *buffer, const std::size_t m, const std::size_t n, const bool replicated=false, std::shared_ptr< typename A::pmap_interface > pmap={}) |
Convert a column-major matrix buffer into an Array object. More... | |
template<typename Array , typename Op , typename std::enable_if< is_dense< Array >::value >::type * = nullptr> | |
Array | make_array (World &world, const detail::trange_t< Array > &trange, const std::shared_ptr< detail::pmap_t< Array > > &pmap, Op &&op) |
Construct dense Array. More... | |
template<typename Array , typename Op > | |
Array | make_array (World &world, const detail::trange_t< Array > &trange, Op &&op) |
Construct an Array. More... | |
template<typename TileType , typename PolicyType > | |
auto | retile (const DistArray< TileType, PolicyType > &tensor, const TiledRange &new_trange) |
template<typename Tile , typename ResultPolicy = DensePolicy, typename ArgPolicy > | |
std::enable_if_t< is_dense_v< ResultPolicy > &&!is_dense_v< ArgPolicy >, DistArray< Tile, ResultPolicy > > | to_dense (DistArray< Tile, ArgPolicy > const &sparse_array) |
template<typename Tile , typename Policy > | |
std::enable_if_t< is_dense_v< Policy >, DistArray< Tile, Policy > > | to_dense (DistArray< Tile, Policy > const &other) |
template<typename Tile , typename ConvTile = Tile, typename Policy , typename Op > | |
decltype(auto) | to_new_tile_type (DistArray< Tile, Policy > const &old_array, Op &&op) |
Function to convert an array to a new array with a different tile type. More... | |
template<typename Tile , typename Policy > | |
std::enable_if_t< is_dense_v< Policy >, void > | truncate (DistArray< Tile, Policy > &array, typename Policy::shape_type::value_type=0) |
Truncate a dense Array. More... | |
template<typename Tile , typename Policy > | |
std::enable_if_t<!is_dense_v< Policy >, void > | truncate (DistArray< Tile, Policy > &array, typename Policy::shape_type::value_type thresh=Policy::shape_type::threshold()) |
Truncate a sparse Array. More... | |
template<typename Tile , typename Policy > | |
TA::DistArray< Tile, Policy > | fuse_vector_of_arrays_tiles (madness::World &global_world, const std::vector< TA::DistArray< Tile, Policy >> &array_vec, const std::size_t fused_dim_extent, const TiledArray::TiledRange &array_trange, std::size_t block_size=1) |
fuses a vector of DistArray objects, each with the same TiledRange into a DistArray with 1 more dimensions More... | |
template<typename Tile , typename Policy > | |
void | subarray_from_fused_array (madness::World &local_world, const TA::DistArray< Tile, Policy > &fused_array, std::size_t tile_idx, std::vector< TA::DistArray< Tile, Policy >> &split_arrays, const TA::TiledRange &split_trange) |
extracts a subarray of a fused array created with fuse_vector_of_arrays and creates the array in local_world . More... | |
template<MemorySpace Space, typename T , typename HostAlloc , typename DeviceAlloc > | |
bool | in_memory_space (const cpu_cuda_vector< T, HostAlloc, DeviceAlloc > &vec) noexcept |
template<ExecutionSpace Space, typename T , typename HostAlloc , typename DeviceAlloc > | |
void | to_execution_space (cpu_cuda_vector< T, HostAlloc, DeviceAlloc > &vec, cudaStream_t stream=0) |
template<typename T > | |
void | make_device_storage (cpu_cuda_vector< T > &storage, std::size_t n, cudaStream_t stream=0) |
template<typename T > | |
T * | device_data (cpu_cuda_vector< T > &storage) |
template<typename T > | |
const T * | device_data (const cpu_cuda_vector< T > &storage) |
template<typename T > | |
void | mult_to_cuda_kernel_impl (T *result, const T *arg, std::size_t n, cudaStream_t stream, int device_id) |
result[i] = result[i] * arg[i] More... | |
template<typename T > | |
void | mult_cuda_kernel_impl (T *result, const T *arg1, const T *arg2, std::size_t n, cudaStream_t stream, int device_id) |
result[i] = arg1[i] * arg2[i] More... | |
template<typename T , typename ReduceOp > | |
T | reduce_cuda_kernel_impl (ReduceOp &&op, const T *arg, std::size_t n, T init, cudaStream_t stream, int device_id) |
T = reduce(T* arg) More... | |
template<typename T > | |
T | product_reduce_cuda_kernel_impl (const T *arg, std::size_t n, cudaStream_t stream, int device_id) |
template<typename T > | |
T | sum_reduce_cuda_kernel_impl (const T *arg, std::size_t n, cudaStream_t stream, int device_id) |
template<typename T > | |
T | max_reduce_cuda_kernel_impl (const T *arg, std::size_t n, cudaStream_t stream, int device_id) |
template<typename T > | |
T | min_reduce_cuda_kernel_impl (const T *arg, std::size_t n, cudaStream_t stream, int device_id) |
template<typename T > | |
T | absmax_reduce_cuda_kernel_impl (const T *arg, std::size_t n, cudaStream_t stream, int device_id) |
template<typename T > | |
T | absmin_reduce_cuda_kernel_impl (const T *arg, std::size_t n, cudaStream_t stream, int device_id) |
constexpr MemorySpace | operator& (MemorySpace space1, MemorySpace space2) |
constexpr MemorySpace | operator| (MemorySpace space1, MemorySpace space2) |
constexpr bool | overlap (MemorySpace space1, MemorySpace space2) |
constexpr bool | operator== (const DenseShape &a, const DenseShape &b) |
constexpr bool | operator!= (const DenseShape &a, const DenseShape &b) |
constexpr bool | is_replicated (World &world, const DenseShape &t) |
template<typename Tile , typename Policy > | |
std::ostream & | operator<< (std::ostream &os, const DistArray< Tile, Policy > &a) |
Add the tensor to an output stream. More... | |
template<typename Tile , typename Policy > | |
auto | rank (const DistArray< Tile, Policy > &a) |
template<typename Tile , typename Policy > | |
size_t | volume (const DistArray< Tile, Policy > &a) |
template<typename Tile , typename Policy > | |
auto | abs_min (const DistArray< Tile, Policy > &a) |
template<typename Tile , typename Policy > | |
auto | abs_max (const DistArray< Tile, Policy > &a) |
template<typename Tile , typename Policy > | |
auto | dot (const DistArray< Tile, Policy > &a, const DistArray< Tile, Policy > &b) |
template<typename Tile , typename Policy > | |
auto | inner_product (const DistArray< Tile, Policy > &a, const DistArray< Tile, Policy > &b) |
template<typename Tile , typename Policy > | |
auto | squared_norm (const DistArray< Tile, Policy > &a) |
template<typename Tile , typename Policy > | |
auto | norm2 (const DistArray< Tile, Policy > &a) |
void | exception_break () |
void | assert_failed (const std::string &m) |
bool | congruent (const Range &r1, const Range &r2) |
Test if the two ranges are congruent. More... | |
template<typename Perm > | |
std::enable_if<!TiledArray::detail::is_permutation_v< Perm >, TiledArray::Range >::type | permute (const TiledArray::Range &r, const Perm &p) |
void | set_default_world (World &world) |
Sets the default World to world . More... | |
World & | get_default_world () |
void | reset_default_world () |
std::unique_ptr< World, decltype(world_resetter)> | push_default_world (World &world) |
bool | initialized () |
bool | finalized () |
bool | operator== (const Permutation &p1, const Permutation &p2) |
Permutation equality operator. More... | |
std::ostream & | operator<< (std::ostream &output, const Permutation &p) |
Add permutation to an output stream. More... | |
template<typename T , std::size_t N> | |
std::array< T, N > | operator* (const Permutation &perm, const std::array< T, N > &a) |
Permute a std::array . More... | |
template<typename T , std::size_t N> | |
std::array< T, N > & | operator*= (std::array< T, N > &a, const Permutation &perm) |
In-place permute a std::array . More... | |
template<typename T , typename A > | |
std::vector< T > | operator* (const Permutation &perm, const std::vector< T, A > &v) |
permute a std::vector<T> More... | |
template<typename T , typename A > | |
std::vector< T, A > & | operator*= (std::vector< T, A > &v, const Permutation &perm) |
In-place permute a std::vector . More... | |
template<typename T > | |
std::vector< T > | operator* (const Permutation &perm, const T *MADNESS_RESTRICT const ptr) |
Permute a memory buffer. More... | |
bool | operator== (const BipartitePermutation &p1, const BipartitePermutation &p2) |
Permutation equality operator. More... | |
bool | operator!= (const Permutation &p1, const Permutation &p2) |
Permutation inequality operator. More... | |
bool | operator< (const Permutation &p1, const Permutation &p2) |
Permutation less-than operator. More... | |
Permutation | operator- (const Permutation &perm) |
Inverse permutation operator. More... | |
Permutation | operator* (const Permutation &p1, const Permutation &p2) |
Permutation multiplication operator. More... | |
Permutation & | operator*= (Permutation &p1, const Permutation &p2) |
return *this ^ other More... | |
Permutation | operator^ (const Permutation &perm, int n) |
Raise perm to the n-th power. More... | |
template<typename T , std::size_t N> | |
boost::container::small_vector< T, N > | operator* (const Permutation &perm, const boost::container::small_vector< T, N > &v) |
permute a boost::container::small_vector<T> More... | |
template<typename T , std::size_t N> | |
boost::container::small_vector< T, N > & | operator*= (boost::container::small_vector< T, N > &v, const Permutation &perm) |
In-place permute a boost::container::small_vector . More... | |
bool | operator!= (const BipartitePermutation &p1, const BipartitePermutation &p2) |
Permutation inequality operator. More... | |
auto | inner (const Permutation &p) |
auto | outer (const Permutation &p) |
auto | inner_size (const Permutation &p) |
auto | outer_size (const Permutation &p) |
auto | inner (const BipartitePermutation &p) |
auto | outer (const BipartitePermutation &p) |
auto | inner_size (const BipartitePermutation &p) |
auto | outer_size (const BipartitePermutation &p) |
void | swap (Range &r0, Range &r1) |
Exchange the values of the give two ranges. More... | |
Range | operator* (const Permutation &perm, const Range &r) |
Create a permuted range. More... | |
template<typename I , typename = std::enable_if_t<std::is_integral_v<I>>> | |
Range | permute (const Range &r, std::initializer_list< I > perm) |
Create a permuted range. More... | |
bool | operator== (const Range &r1, const Range &r2) |
Range equality comparison. More... | |
bool | operator!= (const Range &r1, const Range &r2) |
Range inequality comparison. More... | |
std::ostream & | operator<< (std::ostream &os, const Range &r) |
Range output operator. More... | |
bool | is_congruent (const Range &r1, const Range &r2) |
Test the two ranges are congruent. More... | |
bool | is_contiguous (const Range &range) |
template<typename T > | |
std::ostream & | operator<< (std::ostream &os, const SparseShape< T > &shape) |
Add the shape to an output stream. More... | |
template<typename T > | |
bool | is_replicated (World &world, const SparseShape< T > &shape) |
collective bitwise-compare-reduce for SparseShape objects More... | |
template<typename Array , typename T = double> | |
Array | diagonal_array (World &world, TiledRange const &trange, T val=1) |
Creates a constant diagonal DistArray. More... | |
template<typename Array , typename RandomAccessIterator > | |
std::enable_if_t< detail::is_iterator< RandomAccessIterator >::value, Array > | diagonal_array (World &world, TiledRange const &trange, RandomAccessIterator diagonals_begin, RandomAccessIterator diagonals_end={}) |
Creates a non-constant diagonal DistArray. More... | |
template<typename T1 , typename T2 , typename std::enable_if< detail::is_tensor< T1, T2 >::value||detail::is_tensor_of_tensor< T1, T2 >::value >::type * = nullptr> | |
auto | operator+ (const T1 &left, const T2 &right) |
Tensor plus operator. More... | |
template<typename T1 , typename T2 , typename std::enable_if< detail::is_tensor< T1, T2 >::value||detail::is_tensor_of_tensor< T1, T2 >::value >::type * = nullptr> | |
auto | operator- (const T1 &left, const T2 &right) |
Tensor minus operator. More... | |
template<typename T1 , typename T2 , typename std::enable_if< detail::is_tensor< T1, T2 >::value||detail::is_tensor_of_tensor< T1, T2 >::value >::type * = nullptr> | |
auto | operator* (const T1 &left, const T2 &right) |
Tensor multiplication operator. More... | |
template<typename T , typename N , typename std::enable_if<(detail::is_tensor< T >::value||detail::is_tensor_of_tensor< T >::value) &&detail::is_numeric_v< N >>::type * = nullptr> | |
auto | operator* (const T &left, N right) |
Create a copy of left that is scaled by right . More... | |
template<typename N , typename T , typename std::enable_if< detail::is_numeric_v< N > &&(detail::is_tensor< T >::value||detail::is_tensor_of_tensor< T >::value)>::type * = nullptr> | |
auto | operator* (N left, const T &right) |
Create a copy of right that is scaled by left . More... | |
template<typename T , typename std::enable_if< detail::is_tensor< T >::value||detail::is_tensor_of_tensor< T >::value >::type * = nullptr> | |
auto | operator- (const T &arg) -> decltype(arg.neg()) |
Create a negated copy of arg . More... | |
template<typename T , typename std::enable_if< detail::is_tensor< T >::value||detail::is_tensor_of_tensor< T >::value >::type * = nullptr> | |
auto | operator* (const Permutation &perm, const T &arg) |
Create a permuted copy of arg . More... | |
template<typename T1 , typename T2 , typename std::enable_if< detail::is_tensor< T1, T2 >::value||detail::is_tensor_of_tensor< T1, T2 >::value >::type * = nullptr> | |
auto | operator+= (T1 &left, const T2 &right) |
Tensor plus operator. More... | |
template<typename T1 , typename T2 , typename std::enable_if< detail::is_tensor< T1, T2 >::value||detail::is_tensor_of_tensor< T1, T2 >::value >::type * = nullptr> | |
auto | operator-= (T1 &left, const T2 &right) |
Tensor minus operator. More... | |
template<typename T1 , typename T2 , typename std::enable_if< detail::is_tensor< T1, T2 >::value||detail::is_tensor_of_tensor< T1, T2 >::value >::type * = nullptr> | |
auto | operator*= (T1 &left, const T2 &right) |
In place tensor multiplication. More... | |
template<typename T , typename N , typename std::enable_if<(detail::is_tensor< T >::value||detail::is_tensor_of_tensor< T >::value) &&detail::is_numeric_v< N >>::type * = nullptr> | |
auto | operator+= (T &left, N right) |
In place tensor add constant. More... | |
template<typename T , typename N , typename std::enable_if<(detail::is_tensor< T >::value||detail::is_tensor_of_tensor< T >::value) &&detail::is_numeric_v< N >>::type * = nullptr> | |
auto | operator-= (T &left, N right) |
In place tensor subtract constant. More... | |
template<typename T , typename N , typename std::enable_if<(detail::is_tensor< T >::value||detail::is_tensor_of_tensor< T >::value) &&detail::is_numeric_v< N >>::type * = nullptr> | |
auto | operator*= (T &left, N right) |
In place tensor scale. More... | |
template<typename T > | |
detail::ShiftWrapper< T > | shift (T &tensor) |
Shift a tensor from one range to another. More... | |
template<typename T > | |
detail::ShiftWrapper< const T > | shift (const T &tensor) |
Shift a tensor from one range to another. More... | |
template<typename T , typename A > | |
bool | operator== (const Tensor< T, A > &a, const Tensor< T, A > &b) |
template<typename T , typename A > | |
bool | operator!= (const Tensor< T, A > &a, const Tensor< T, A > &b) |
template<typename T , typename Range_ , typename OpResult , typename Index1 , typename Index2 > | |
void | remap (detail::TensorInterface< T, Range_, OpResult > &, T *const, const Index1 &, const Index2 &) |
template<typename T , typename Range_ , typename OpResult , typename Index1 , typename Index2 > | |
void | remap (detail::TensorInterface< const T, Range_, OpResult > &, T *const, const Index1 &, const Index2 &) |
template<typename T , typename Range_ , typename OpResult , typename Index1 , typename Index2 > | |
void | remap (detail::TensorInterface< T, Range_, OpResult > &, T *const, const std::initializer_list< Index1 > &, const std::initializer_list< Index2 > &) |
template<typename T , typename Range_ , typename OpResult , typename Index1 , typename Index2 > | |
void | remap (detail::TensorInterface< const T, Range_, OpResult > &, T *const, const std::initializer_list< Index1 > &, const std::initializer_list< Index2 > &) |
template<typename T , typename Range_ = Range, typename OpResult = Tensor<T>, typename Index > | |
TensorMap< T, Range_, OpResult > | make_map (T *const data, const Index &lower_bound, const Index &upper_bound) |
template<typename T , typename Range_ = Range, typename OpResult = Tensor<T>> | |
TensorMap< T, Range_, OpResult > | make_map (T *const data, const std::initializer_list< std::size_t > &lower_bound, const std::initializer_list< std::size_t > &upper_bound) |
template<typename T , typename Range_ , typename OpResult = Tensor<T>> | |
TensorMap< T, std::decay_t< Range_ >, OpResult > | make_map (T *const data, Range_ &&range) |
template<typename T , typename Range_ = Range, typename OpResult = Tensor<T>, typename Index > | |
TensorConstMap< T, Range_, OpResult > | make_map (const T *const data, const Index &lower_bound, const Index &upper_bound) |
template<typename T , typename Range_ = Range, typename OpResult = Tensor<T>> | |
TensorConstMap< T, Range_, OpResult > | make_map (const T *const data, const std::initializer_list< std::size_t > &lower_bound, const std::initializer_list< std::size_t > &upper_bound) |
template<typename T , typename Range_ , typename OpResult = Tensor<T>> | |
TensorConstMap< T, std::decay_t< Range_ >, OpResult > | make_map (const T *const data, Range_ &&range) |
template<typename T , typename Range_ = Range, typename OpResult = Tensor<T>, typename Index > | |
TensorConstMap< T, Range_, OpResult > | make_const_map (const T *const data, const Index &lower_bound, const Index &upper_bound) |
template<typename T , typename Range_ = Range, typename OpResult = Tensor<T>> | |
TensorConstMap< T, Range_, OpResult > | make_const_map (const T *const data, const std::initializer_list< std::size_t > &lower_bound, const std::initializer_list< std::size_t > &upper_bound) |
template<typename T , typename Range_ , typename OpResult = Tensor<T>> | |
TensorConstMap< T, std::decay_t< Range_ >, OpResult > | make_const_map (const T *const data, Range_ &&range) |
template<typename T , typename Range_ , typename OpResult = Tensor<T>, typename Index > | |
TensorConstMap< T, Range_, OpResult > | make_const_map (T *const data, const Index &lower_bound, const Index &upper_bound) |
template<typename T , typename Range_ , typename OpResult = Tensor<T>> | |
TensorConstMap< T, Range_, OpResult > | make_const_map (T *const data, const std::initializer_list< std::size_t > &lower_bound, const std::initializer_list< std::size_t > &upper_bound) |
template<typename T , typename Range_ , typename OpResult = Tensor<T>> | |
TensorConstMap< T, std::decay_t< Range_ >, OpResult > | make_const_map (T *const data, Range_ &&range) |
template<typename T , typename Range_ , typename OpResult , typename Index1 , typename Index2 > | |
void | remap (TensorMap< T, Range_, OpResult > &map, T *const data, const Index1 &lower_bound, const Index2 &upper_bound) |
For reusing map without allocating new ranges . . . maybe. More... | |
template<typename T , typename Range_ , typename OpResult , typename Index1 , typename Index2 , typename = std::enable_if_t<!std::is_const<T>::value>> | |
void | remap (TensorConstMap< T, Range_, OpResult > &map, T *const data, const Index1 &lower_bound, const Index2 &upper_bound) |
template<typename T , typename Range_ , typename OpResult , typename Index1 , typename Index2 > | |
void | remap (TensorMap< T, Range_, OpResult > &map, T *const data, const std::initializer_list< Index1 > &lower_bound, const std::initializer_list< Index2 > &upper_bound) |
template<typename T , typename Range_ , typename OpResult , typename Index1 , typename Index2 , typename = std::enable_if_t<!std::is_const<T>::value>> | |
void | remap (TensorConstMap< T, Range_, OpResult > &map, T *const data, const std::initializer_list< Index1 > &lower_bound, const std::initializer_list< Index2 > &upper_bound) |
template<typename T , typename std::enable_if< detail::is_tensor< T >::value &&detail::is_contiguous_tensor< T >::value >::type * = nullptr> | |
std::ostream & | operator<< (std::ostream &os, const T &t) |
Tensor output operator. More... | |
template<typename Arg > | |
Tile< Arg > | clone (const Tile< Arg > &arg) |
Create a copy of arg . More... | |
template<typename Arg > | |
bool | empty (const Tile< Arg > &arg) |
Check that arg is empty (no data) More... | |
template<typename Arg , typename Perm , typename = std::enable_if_t<detail::is_permutation_v<Perm>>> | |
decltype(auto) | permute (const Tile< Arg > &arg, const Perm &perm) |
Create a permuted copy of arg . More... | |
template<typename Arg , typename Index , typename = std::enable_if_t<detail::is_integral_range_v<Index>>> | |
decltype(auto) | shift (const Tile< Arg > &arg, const Index &range_shift) |
Shift the range of arg . More... | |
template<typename Arg , typename Index , typename = std::enable_if_t<std::is_integral_v<Index>>> | |
decltype(auto) | shift (const Tile< Arg > &arg, const std::initializer_list< Index > &range_shift) |
Shift the range of arg . More... | |
template<typename Arg , typename Index , typename = std::enable_if_t<detail::is_integral_range_v<Index>>> | |
Tile< Arg > & | shift_to (Tile< Arg > &arg, const Index &range_shift) |
Shift the range of arg in place. More... | |
template<typename Arg , typename Index , typename = std::enable_if_t<std::is_integral_v<Index>>> | |
Tile< Arg > & | shift_to (Tile< Arg > &arg, const std::initializer_list< Index > &range_shift) |
Shift the range of arg in place. More... | |
template<typename Left , typename Right > | |
decltype(auto) | add (const Tile< Left > &left, const Tile< Right > &right) |
Add tile arguments. More... | |
template<typename Left , typename Right , typename Scalar , typename std::enable_if< detail::is_numeric_v< Scalar >>::type * = nullptr> | |
decltype(auto) | add (const Tile< Left > &left, const Tile< Right > &right, const Scalar factor) |
Add and scale tile arguments. More... | |
template<typename Left , typename Right , typename Perm , typename = std::enable_if_t<detail::is_permutation_v<Perm>>> | |
decltype(auto) | add (const Tile< Left > &left, const Tile< Right > &right, const Perm &perm) |
Add and permute tile arguments. More... | |
template<typename Left , typename Right , typename Scalar , typename Perm , typename std::enable_if< detail::is_numeric_v< Scalar > &&detail::is_permutation_v< Perm >>::type * = nullptr> | |
decltype(auto) | add (const Tile< Left > &left, const Tile< Right > &right, const Scalar factor, const Perm &perm) |
Add, scale, and permute tile arguments. More... | |
template<typename Arg , typename Scalar , typename std::enable_if< detail::is_numeric_v< Scalar >>::type * = nullptr> | |
decltype(auto) | add (const Tile< Arg > &arg, const Scalar value) |
Add a constant scalar to tile argument. More... | |
template<typename Arg , typename Scalar , typename Perm , typename std::enable_if< detail::is_numeric_v< Scalar > &&detail::is_permutation_v< Perm >>::type * = nullptr> | |
decltype(auto) | add (const Tile< Arg > &arg, const Scalar value, const Perm &perm) |
Add a constant scalar and permute tile argument. More... | |
template<typename Result , typename Arg > | |
Tile< Result > & | add_to (Tile< Result > &result, const Tile< Arg > &arg) |
Add to the result tile. More... | |
template<typename Result , typename Arg , typename Scalar , typename std::enable_if< detail::is_numeric_v< Scalar >>::type * = nullptr> | |
Tile< Result > & | add_to (Tile< Result > &result, const Tile< Arg > &arg, const Scalar factor) |
Add and scale to the result tile. More... | |
template<typename Result , typename Scalar , typename std::enable_if< detail::is_numeric_v< Scalar >>::type * = nullptr> | |
Tile< Result > & | add_to (Tile< Result > &result, const Scalar value) |
Add constant scalar to the result tile. More... | |
template<typename Left , typename Right > | |
decltype(auto) | subt (const Tile< Left > &left, const Tile< Right > &right) |
Subtract tile arguments. More... | |
template<typename Left , typename Right , typename Scalar , typename std::enable_if< detail::is_numeric_v< Scalar >>::type * = nullptr> | |
decltype(auto) | subt (const Tile< Left > &left, const Tile< Right > &right, const Scalar factor) |
Subtract and scale tile arguments. More... | |
template<typename Left , typename Right , typename Perm , typename = std::enable_if_t<detail::is_permutation_v<Perm>>> | |
decltype(auto) | subt (const Tile< Left > &left, const Tile< Right > &right, const Perm &perm) |
Subtract and permute tile arguments. More... | |
template<typename Left , typename Right , typename Scalar , typename Perm , typename std::enable_if< detail::is_numeric_v< Scalar > &&detail::is_permutation_v< Perm >>::type * = nullptr> | |
decltype(auto) | subt (const Tile< Left > &left, const Tile< Right > &right, const Scalar factor, const Perm &perm) |
Subtract, scale, and permute tile arguments. More... | |
template<typename Arg , typename Scalar , typename std::enable_if< detail::is_numeric_v< Scalar >>::type * = nullptr> | |
decltype(auto) | subt (const Tile< Arg > &arg, const Scalar value) |
Subtract a scalar constant from the tile argument. More... | |
template<typename Arg , typename Scalar , typename Perm , typename std::enable_if< detail::is_numeric_v< Scalar > &&detail::is_permutation_v< Perm >>::type * = nullptr> | |
decltype(auto) | subt (const Tile< Arg > &arg, const Scalar value, const Perm &perm) |
Subtract a constant scalar and permute tile argument. More... | |
template<typename Result , typename Arg > | |
Tile< Result > & | subt_to (Tile< Result > &result, const Tile< Arg > &arg) |
Subtract from the result tile. More... | |
template<typename Result , typename Arg , typename Scalar , typename std::enable_if< detail::is_numeric_v< Scalar >>::type * = nullptr> | |
Tile< Result > & | subt_to (Tile< Result > &result, const Tile< Arg > &arg, const Scalar factor) |
Subtract and scale from the result tile. More... | |
template<typename Result , typename Scalar , typename std::enable_if< detail::is_numeric_v< Scalar >>::type * = nullptr> | |
Tile< Result > & | subt_to (Tile< Result > &result, const Scalar value) |
Subtract constant scalar from the result tile. More... | |
template<typename Left , typename Right > | |
decltype(auto) | mult (const Tile< Left > &left, const Tile< Right > &right) |
Multiplication tile arguments. More... | |
template<typename Left , typename Right , typename Scalar , typename std::enable_if< detail::is_numeric_v< Scalar >>::type * = nullptr> | |
decltype(auto) | mult (const Tile< Left > &left, const Tile< Right > &right, const Scalar factor) |
Multiplication and scale tile arguments. More... | |
template<typename Left , typename Right , typename Perm , typename = std::enable_if_t<detail::is_permutation_v<Perm>>> | |
decltype(auto) | mult (const Tile< Left > &left, const Tile< Right > &right, const Perm &perm) |
Multiplication and permute tile arguments. More... | |
template<typename Left , typename Right , typename Scalar , typename Perm , typename std::enable_if< detail::is_numeric_v< Scalar > &&detail::is_permutation_v< Perm >>::type * = nullptr> | |
decltype(auto) | mult (const Tile< Left > &left, const Tile< Right > &right, const Scalar factor, const Perm &perm) |
Multiplication, scale, and permute tile arguments. More... | |
template<typename Result , typename Arg > | |
Tile< Result > & | mult_to (Tile< Result > &result, const Tile< Arg > &arg) |
Multiply to the result tile. More... | |
template<typename Result , typename Arg , typename Scalar , typename std::enable_if< detail::is_numeric_v< Scalar >>::type * = nullptr> | |
Tile< Result > & | mult_to (Tile< Result > &result, const Tile< Arg > &arg, const Scalar factor) |
Multiply and scale to the result tile. More... | |
template<typename Left , typename Right , typename Op > | |
decltype(auto) | binary (const Tile< Left > &left, const Tile< Right > &right, Op &&op) |
Binary element-wise transform producing a new tile. More... | |
template<typename Left , typename Right , typename Op , typename Perm , typename = std::enable_if_t<detail::is_permutation_v<Perm>>> | |
decltype(auto) | binary (const Tile< Left > &left, const Tile< Right > &right, Op &&op, const Perm &perm) |
Binary element-wise transform producing a new tile. More... | |
template<typename Left , typename Right , typename Op > | |
Tile< Left > & | inplace_binary (Tile< Left > &left, const Tile< Right > &right, Op &&op) |
Binary element-wise in-place transform. More... | |
template<typename Arg , typename Scalar , typename std::enable_if< detail::is_numeric_v< Scalar >>::type * = nullptr> | |
decltype(auto) | scale (const Tile< Arg > &arg, const Scalar factor) |
Scalar the tile argument. More... | |
template<typename Arg , typename Scalar , typename Perm , typename std::enable_if< detail::is_numeric_v< Scalar > &&detail::is_permutation_v< Perm >>::type * = nullptr> | |
decltype(auto) | scale (const Tile< Arg > &arg, const Scalar factor, const Perm &perm) |
Scale and permute tile argument. More... | |
template<typename Result , typename Scalar , typename std::enable_if< detail::is_numeric_v< Scalar >>::type * = nullptr> | |
Tile< Result > & | scale_to (Tile< Result > &result, const Scalar factor) |
Scale to the result tile. More... | |
template<typename Arg > | |
decltype(auto) | neg (const Tile< Arg > &arg) |
Negate the tile argument. More... | |
template<typename Arg , typename Perm , typename = std::enable_if_t<detail::is_permutation_v<Perm>>> | |
decltype(auto) | neg (const Tile< Arg > &arg, const Perm &perm) |
Negate and permute tile argument. More... | |
template<typename Result > | |
Tile< Result > & | neg_to (Tile< Result > &result) |
In-place negate tile. More... | |
template<typename Arg > | |
decltype(auto) | conj (const Tile< Arg > &arg) |
Create a complex conjugated copy of a tile. More... | |
template<typename Arg , typename Scalar , typename std::enable_if< TiledArray::detail::is_numeric_v< Scalar >>::type * = nullptr> | |
decltype(auto) | conj (const Tile< Arg > &arg, const Scalar factor) |
Create a complex conjugated and scaled copy of a tile. More... | |
template<typename Arg , typename Perm , typename = std::enable_if_t<detail::is_permutation_v<Perm>>> | |
decltype(auto) | conj (const Tile< Arg > &arg, const Perm &perm) |
Create a complex conjugated and permuted copy of a tile. More... | |
template<typename Arg , typename Scalar , typename Perm , typename std::enable_if< TiledArray::detail::is_numeric_v< Scalar > &&detail::is_permutation_v< Perm >>::type * = nullptr> | |
decltype(auto) | conj (const Tile< Arg > &arg, const Scalar factor, const Perm &perm) |
Create a complex conjugated, scaled, and permuted copy of a tile. More... | |
template<typename Result > | |
Tile< Result > & | conj_to (Tile< Result > &result) |
In-place complex conjugate a tile. More... | |
template<typename Result , typename Scalar , typename std::enable_if< TiledArray::detail::is_numeric_v< Scalar >>::type * = nullptr> | |
Tile< Result > & | conj_to (Tile< Result > &result, const Scalar factor) |
In-place complex conjugate and scale a tile. More... | |
template<typename Arg , typename Op > | |
decltype(auto) | unary (const Tile< Arg > &arg, Op &&op) |
Unary element-wise transform producing a new tile. More... | |
template<typename Arg , typename Op , typename Perm , typename = std::enable_if_t<detail::is_permutation_v<Perm>>> | |
decltype(auto) | unary (const Tile< Arg > &arg, Op &&op, const Perm &perm) |
Unary element-wise transform producing a new tile. More... | |
template<typename Result , typename Op > | |
Tile< Result > & | inplace_unary (Tile< Result > &arg, Op &&op) |
Unary element-wise in-place transform. More... | |
template<typename Left , typename Right , typename Scalar , typename std::enable_if< detail::is_numeric_v< Scalar >>::type * = nullptr> | |
decltype(auto) | gemm (const Tile< Left > &left, const Tile< Right > &right, const Scalar factor, const math::GemmHelper &gemm_config) |
Contract 2 tensors over head/tail modes and scale the product. More... | |
template<typename Result , typename Left , typename Right , typename Scalar , typename std::enable_if< detail::is_numeric_v< Scalar >>::type * = nullptr> | |
Tile< Result > & | gemm (Tile< Result > &result, const Tile< Left > &left, const Tile< Right > &right, const Scalar factor, const math::GemmHelper &gemm_config) |
template<typename Result , typename Left , typename Right , typename ElementMultiplyAddOp , typename std::enable_if< std::is_invocable_r_v< void, std::remove_reference_t< ElementMultiplyAddOp >, typename Result::value_type &, const typename Left::value_type &, const typename Right::value_type & >>::type * = nullptr> | |
Tile< Result > & | gemm (Tile< Result > &result, const Tile< Left > &left, const Tile< Right > &right, const math::GemmHelper &gemm_config, ElementMultiplyAddOp &&element_multiplyadd_op) |
template<typename Arg > | |
decltype(auto) | trace (const Tile< Arg > &arg) |
Sum the hyper-diagonal elements a tile. More... | |
template<typename Arg > | |
decltype(auto) | sum (const Tile< Arg > &arg) |
Sum the elements of a tile. More... | |
template<typename Arg > | |
decltype(auto) | product (const Tile< Arg > &arg) |
Multiply the elements of a tile. More... | |
template<typename Arg > | |
decltype(auto) | squared_norm (const Tile< Arg > &arg) |
Squared vector 2-norm of the elements of a tile. More... | |
template<typename Arg > | |
decltype(auto) | norm (const Tile< Arg > &arg) |
Vector 2-norm of a tile. More... | |
template<typename Arg , typename ResultType > | |
void | norm (const Tile< Arg > &arg, ResultType &result) |
Vector 2-norm of a tile. More... | |
template<typename Arg > | |
decltype(auto) | max (const Tile< Arg > &arg) |
Maximum element of a tile. More... | |
template<typename Arg > | |
decltype(auto) | min (const Tile< Arg > &arg) |
Minimum element of a tile. More... | |
template<typename Arg > | |
decltype(auto) | abs_max (const Tile< Arg > &arg) |
Absolute maximum element of a tile. More... | |
template<typename Arg > | |
decltype(auto) | abs_min (const Tile< Arg > &arg) |
Absolute mainimum element of a tile. More... | |
template<typename Left , typename Right > | |
decltype(auto) | dot (const Tile< Left > &left, const Tile< Right > &right) |
Vector dot product of a tile. More... | |
template<typename Left , typename Right > | |
decltype(auto) | inner_product (const Tile< Left > &left, const Tile< Right > &right) |
Vector inner product of a tile. More... | |
template<typename T > | |
std::ostream & | operator<< (std::ostream &os, const Tile< T > &tile) |
template<typename T1 , typename T2 > | |
bool | operator== (const Tile< T1 > &t1, const Tile< T2 > &t2) |
Tile equality comparison. More... | |
template<typename T1 , typename T2 > | |
bool | operator!= (const Tile< T1 > &t1, const Tile< T2 > &t2) |
Tile inequality comparison. More... | |
template<typename Left , typename Right > | |
auto | add (const Left &left, const Right &right) -> decltype(left.add(right)) |
Add tile arguments. More... | |
template<typename Left , typename Right , typename Scalar , typename std::enable_if< detail::is_numeric_v< Scalar >>::type * = nullptr> | |
auto | add (const Left &left, const Right &right, const Scalar factor) |
Add and scale tile arguments. More... | |
template<typename Left , typename Right , typename Perm , typename = std::enable_if_t<TiledArray::detail::is_permutation_v<Perm> && detail::has_member_function_add_anyreturn_v< const Left, const Right&, const Perm&>>> | |
auto | add (const Left &left, const Right &right, const Perm &perm) |
Add and permute tile arguments. More... | |
template<typename Left , typename Right , typename Scalar , typename Perm , typename std::enable_if< detail::is_numeric_v< Scalar > &&detail::is_permutation_v< Perm >>::type * = nullptr> | |
auto | add (const Left &left, const Right &right, const Scalar factor, const Perm &perm) |
Add, scale, and permute tile arguments. More... | |
template<typename Result , typename Arg > | |
Result & | add_to (Result &result, const Arg &arg) |
Add to the result tile. More... | |
template<typename Result , typename Arg , typename Scalar , typename std::enable_if< detail::is_numeric_v< Scalar >>::type * = nullptr> | |
Result & | add_to (Result &result, const Arg &arg, const Scalar factor) |
Add and scale to the result tile. More... | |
template<typename Arg , typename Result = typename TiledArray::eval_trait< madness::remove_fcvr_t<Arg>>::type> | |
auto | invoke_cast (Arg &&arg) |
template<typename Arg > | |
auto | clone (const Arg &arg) |
Create a copy of arg . More... | |
template<typename Arg , typename Perm , typename = std::enable_if_t<detail::is_permutation_v<Perm> && detail::has_member_function_permute_anyreturn_v< const Arg, const Perm&>>> | |
auto | permute (const Arg &arg, const Perm &perm) |
Create a permuted copy of arg . More... | |
template<typename Arg , typename Scalar , std::enable_if_t< TiledArray::detail::is_numeric_v< Scalar > &&!TiledArray::detail::is_array_v< Arg >> * = nullptr> | |
auto | scale (const Arg &arg, const Scalar factor) |
Scalar the tile argument. More... | |
template<typename Arg , typename Scalar , typename Perm , std::enable_if_t< TiledArray::detail::is_numeric_v< Scalar > &&TiledArray::detail::is_permutation_v< Perm >> * = nullptr> | |
auto | scale (const Arg &arg, const Scalar factor, const Perm &perm) |
Scale and permute tile argument. More... | |
template<typename Result , typename Scalar , std::enable_if_t< TiledArray::detail::is_numeric_v< Scalar >> * = nullptr> | |
Result & | scale_to (Result &result, const Scalar factor) |
Scale to the result tile. More... | |
template<typename Arg , typename Index , typename = std::enable_if_t<detail::is_integral_range_v<Index>>> | |
auto | shift (const Arg &arg, const Index &range_shift) |
Shift the range of arg . More... | |
template<typename Arg , typename Index , typename = std::enable_if_t<std::is_integral_v<Index>>> | |
auto | shift (const Arg &arg, const std::initializer_list< Index > &range_shift) |
Shift the range of arg . More... | |
template<typename Arg , typename Index , typename = std::enable_if_t<detail::is_integral_range_v<Index>>> | |
auto | shift_to (Arg &arg, const Index &range_shift) |
Shift the range of arg in place. More... | |
template<typename Arg , typename Index , typename = std::enable_if_t<std::is_integral_v<Index>>> | |
auto | shift_to (Arg &arg, const std::initializer_list< Index > &range_shift) |
Shift the range of arg in place. More... | |
template<typename T , typename = detail::enable_if_trace_is_defined_t<T>> | |
decltype(auto) | trace (const T &t) |
template<typename Left , typename Right > | |
auto | subt (const Left &left, const Right &right) |
Subtract tile arguments. More... | |
template<typename Left , typename Right , typename Scalar , typename std::enable_if< detail::is_numeric_v< Scalar >>::type * = nullptr> | |
auto | subt (const Left &left, const Right &right, const Scalar factor) |
Subtract and scale tile arguments. More... | |
template<typename Left , typename Right , typename Perm , typename = std::enable_if_t<detail::is_permutation_v<Perm>>> | |
auto | subt (const Left &left, const Right &right, const Perm &perm) |
Subtract and permute tile arguments. More... | |
template<typename Left , typename Right , typename Scalar , typename Perm , typename std::enable_if< detail::is_numeric_v< Scalar > &&detail::is_permutation_v< Perm >>::type * = nullptr> | |
auto | subt (const Left &left, const Right &right, const Scalar factor, const Perm &perm) |
Subtract, scale, and permute tile arguments. More... | |
template<typename Arg , typename Scalar , typename std::enable_if< detail::is_numeric_v< Scalar >>::type * = nullptr> | |
auto | subt (const Arg &arg, const Scalar value) |
Subtract a scalar constant from the tile argument. More... | |
template<typename Arg , typename Scalar , typename Perm , typename std::enable_if< detail::is_numeric_v< Scalar > &&detail::is_permutation_v< Perm >>::type * = nullptr> | |
auto | subt (const Arg &arg, const Scalar value, const Perm &perm) |
Subtract a constant scalar and permute tile argument. More... | |
template<typename Result , typename Arg > | |
Result & | subt_to (Result &result, const Arg &arg) |
Subtract from the result tile. More... | |
template<typename Result , typename Arg , typename Scalar , typename std::enable_if< detail::is_numeric_v< Scalar >>::type * = nullptr> | |
Result & | subt_to (Result &result, const Arg &arg, const Scalar factor) |
Subtract and scale from the result tile. More... | |
template<typename Result , typename Scalar , typename std::enable_if< detail::is_numeric_v< Scalar >>::type * = nullptr> | |
Result & | subt_to (Result &result, const Scalar value) |
Subtract constant scalar from the result tile. More... | |
template<typename Left , typename Right > | |
auto | mult (const Left &left, const Right &right) |
Multiplication tile arguments. More... | |
template<typename Left , typename Right , typename Scalar , std::enable_if_t< TiledArray::detail::is_numeric_v< Scalar >> * = nullptr> | |
auto | mult (const Left &left, const Right &right, const Scalar factor) |
Multiplication and scale tile arguments. More... | |
template<typename Left , typename Right , typename Perm , typename = std::enable_if_t<detail::is_permutation_v<Perm> && detail::has_member_function_mult_anyreturn_v< const Left, const Right&, const Perm&>>> | |
auto | mult (const Left &left, const Right &right, const Perm &perm) |
Multiplication and permute tile arguments. More... | |
template<typename Left , typename Right , typename Scalar , typename Perm , std::enable_if_t< TiledArray::detail::is_numeric_v< Scalar > &&detail::is_permutation_v< Perm >> * = nullptr> | |
auto | mult (const Left &left, const Right &right, const Scalar factor, const Perm &perm) |
Multiplication, scale, and permute tile arguments. More... | |
template<typename Result , typename Arg > | |
Result & | mult_to (Result &result, const Arg &arg) |
Multiply to the result tile. More... | |
template<typename Result , typename Arg , typename Scalar , std::enable_if_t< TiledArray::detail::is_numeric_v< Scalar >> * = nullptr> | |
Result & | mult_to (Result &result, const Arg &arg, const Scalar factor) |
Multiply and scale to the result tile. More... | |
template<typename Left , typename Right , typename Op > | |
decltype(auto) | binary (const Left &left, const Right &right, Op &&op) |
Binary element-wise transform producing a new tile. More... | |
template<typename Left , typename Right , typename Op , typename Perm , typename = std::enable_if_t<detail::is_permutation_v<Perm>>> | |
decltype(auto) | binary (const Left &left, const Right &right, Op &&op, const Perm &perm) |
Binary element-wise transform producing a new tile. More... | |
template<typename Left , typename Right , typename Op > | |
Left & | inplace_binary (Left &left, const Right &right, Op &&op) |
Binary element-wise in-place transform. More... | |
template<typename Arg > | |
auto | neg (const Arg &arg) |
Negate the tile argument. More... | |
template<typename Arg , typename Perm , typename = std::enable_if_t<detail::is_permutation_v<Perm>>> | |
auto | neg (const Arg &arg, const Perm &perm) |
Negate and permute tile argument. More... | |
template<typename Result > | |
Result & | neg_to (Result &result) |
Negate the tile argument in-place. More... | |
template<typename Arg > | |
auto | conj (const Arg &arg) |
Create a complex conjugated copy of a tile. More... | |
template<typename Arg , typename Scalar , typename std::enable_if< TiledArray::detail::is_numeric_v< Scalar >>::type * = nullptr> | |
auto | conj (const Arg &arg, const Scalar factor) |
Create a complex conjugated and scaled copy of a tile. More... | |
template<typename Arg , typename Perm , typename = std::enable_if_t<detail::is_permutation_v<Perm>>> | |
auto | conj (const Arg &arg, const Perm &perm) |
Create a complex conjugated and permuted copy of a tile. More... | |
template<typename Arg , typename Scalar , typename Perm , typename std::enable_if< TiledArray::detail::is_numeric_v< Scalar > &&detail::is_permutation_v< Perm >>::type * = nullptr> | |
auto | conj (const Arg &arg, const Scalar factor, const Perm &perm) |
Create a complex conjugated, scaled, and permuted copy of a tile. More... | |
template<typename Result > | |
Result & | conj_to (Result &result) |
In-place complex conjugate a tile. More... | |
template<typename Result , typename Scalar , typename std::enable_if< TiledArray::detail::is_numeric_v< Scalar >>::type * = nullptr> | |
Result & | conj_to (Result &result, const Scalar factor) |
In-place complex conjugate and scale a tile. More... | |
template<typename Arg , typename Op > | |
decltype(auto) | unary (const Arg &arg, Op &&op) |
Unary element-wise transform producing a new tile. More... | |
template<typename Arg , typename Op , typename Perm , typename = std::enable_if_t<detail::is_permutation_v<Perm>>> | |
decltype(auto) | unary (const Arg &arg, Op &&op, const Perm &perm) |
Unary element-wise transform producing a new tile. More... | |
template<typename Result , typename Op > | |
Result & | inplace_unary (Result &arg, Op &&op) |
Unary element-wise in-place transform. More... | |
template<typename Left , typename Right , typename Scalar , std::enable_if_t< TiledArray::detail::is_numeric_v< Scalar >> * = nullptr> | |
auto | gemm (const Left &left, const Right &right, const Scalar factor, const math::GemmHelper &gemm_config) |
Contract 2 tensors over head/tail modes and scale the product. More... | |
template<typename Result , typename Left , typename Right , typename Scalar , std::enable_if_t< TiledArray::detail::is_numeric_v< Scalar >> * = nullptr> | |
Result & | gemm (Result &result, const Left &left, const Right &right, const Scalar factor, const math::GemmHelper &gemm_config) |
template<typename Result , typename Left , typename Right , typename ElementMultiplyAddOp , std::enable_if_t< std::is_invocable_r_v< void, std::remove_reference_t< ElementMultiplyAddOp >, typename Result::value_type &, const typename Left::value_type &, const typename Right::value_type & >> * = nullptr> | |
Result & | gemm (Result &result, const Left &left, const Right &right, const math::GemmHelper &gemm_config, ElementMultiplyAddOp &&element_multiplyadd_op) |
template<typename Arg > | |
auto | sum (const Arg &arg) |
Sum the hyper-diagonal elements a tile. More... | |
template<typename Arg > | |
auto | product (const Arg &arg) |
Multiply the elements of a tile. More... | |
template<typename Arg > | |
auto | squared_norm (const Arg &arg) |
Squared vector 2-norm of the elements of a tile. More... | |
template<typename Arg > | |
auto | norm (const Arg &arg) |
Vector 2-norm of a tile. More... | |
template<typename Arg , typename ResultType > | |
void | norm (const Arg &arg, ResultType &result) |
Vector 2-norm of a tile. More... | |
template<typename Arg > | |
auto | max (const Arg &arg) |
Maximum element of a tile. More... | |
template<typename Arg > | |
auto | min (const Arg &arg) |
Minimum element of a tile. More... | |
template<typename Arg > | |
auto | abs_max (const Arg &arg) |
Absolute maximum element of a tile. More... | |
template<typename Arg > | |
auto | abs_min (const Arg &arg) |
Absolute mainimum element of a tile. More... | |
template<typename Left , typename Right > | |
auto | dot (const Left &left, const Right &right) |
Vector dot product of two tiles. More... | |
template<typename Left , typename Right > | |
auto | inner_product (const Left &left, const Right &right) |
Vector inner product of two tiles. More... | |
TiledRange | operator* (const Permutation &p, const TiledRange &r) |
TiledRange permutation operator. More... | |
void | swap (TiledRange &r0, TiledRange &r1) |
Exchange the content of the two given TiledRange's. More... | |
bool | operator== (const TiledRange &r1, const TiledRange &r2) |
Returns true when all tile and element ranges are the same. More... | |
bool | operator!= (const TiledRange &r1, const TiledRange &r2) |
std::ostream & | operator<< (std::ostream &out, const TiledRange &rng) |
void | swap (TiledRange1 &r0, TiledRange1 &r1) |
Exchange the data of the two given ranges. More... | |
bool | operator== (const TiledRange1 &r1, const TiledRange1 &r2) |
Equality operator. More... | |
bool | operator!= (const TiledRange1 &r1, const TiledRange1 &r2) |
Inequality operator. More... | |
std::ostream & | operator<< (std::ostream &out, const TiledRange1 &rng) |
TiledRange1 ostream operator. More... | |
TiledRange1 | concat (const TiledRange1 &r1, const TiledRange1 &r2) |
Concatenates two ranges. More... | |
bool | is_congruent (const TiledRange1 &r1, const TiledRange1 &r2) |
Test that two TiledRange1 objects are congruent. More... | |
void | launch_gdb_xterm () |
Use this to launch GNU debugger in xterm. More... | |
void | launch_lldb_xterm () |
Use this to launch LLVM debugger in xterm. More... | |
template<class F > | |
shared_function< std::decay_t< F > > | make_shared_function (F &&f) |
template<typename R , typename... Args> | |
constexpr void | swap (function_ref< R(Args...)> &lhs, function_ref< R(Args...)> &rhs) noexcept |
Swaps the referred callables of lhs and rhs . More... | |
template<typename Op > | |
auto | make_op_shared_handle (Op &&op) |
template<typename T , typename U = std::array<TiledRange1, initializer_list_rank_v<std::decay_t<T>>>> | |
auto | tiled_range_from_il (T &&il, U shape={}) |
Creates a TiledRange for the provided initializer list. More... | |
template<typename T , typename OutputItr > | |
auto | flatten_il (T &&il, OutputItr out_itr) |
Flattens the contents of a (possibly nested) initializer_list into the provided buffer. More... | |
template<typename T , typename U > | |
auto | get_elem_from_il (T idx, U &&il, std::size_t depth=0) |
Retrieves the specified element from an initializer_list. More... | |
template<typename ArrayType , typename T > | |
auto | array_from_il (World &world, const TiledRange &trange, T &&il) |
Converts an std::initializer_list into a tiled array. More... | |
template<typename ArrayType , typename T > | |
auto | array_from_il (World &world, T &&il) |
Converts an std::initializer_list into a single tile array. More... | |
time_point | now () |
std::chrono::system_clock::time_point | system_now () |
double | duration_in_s (time_point const &t0, time_point const &t1) |
int64_t | duration_in_ns (time_point const &t0, time_point const &t1) |
template<typename T , typename A > | |
std::ostream & | operator<< (std::ostream &os, const std::vector< T, A > &vec) |
Vector output stream operator. More... | |
void | ignore_tile_position (bool b) |
bool | ignore_tile_position () |
foreach/foreach_inplace functions | |
void(ResultTile&, const ArgTiles&...);
For sparse arrays void(ResultTile&, const ArgTiles&...);
or as real(ResultTile&, const ArgTiles&...);
where
| |
template<typename ResultTile , typename ArgTile , typename Policy , typename Op , typename = typename std::enable_if< !std::is_same<ResultTile, ArgTile>::value>::type> | |
std::enable_if_t< is_dense_v< Policy >, DistArray< ResultTile, Policy > > | foreach (const DistArray< ArgTile, Policy > &arg, Op &&op) |
Apply a function to each tile of a dense Array. More... | |
template<typename Tile , typename Policy , typename Op > | |
std::enable_if_t< is_dense_v< Policy >, DistArray< Tile, Policy > > | foreach (const DistArray< Tile, Policy > &arg, Op &&op) |
Apply a function to each tile of a dense Array. More... | |
template<typename Tile , typename Policy , typename Op , typename = typename std::enable_if<!TiledArray::detail::is_array< typename std::decay<Op>::type>::value>::type> | |
std::enable_if_t< is_dense_v< Policy >, void > | foreach_inplace (DistArray< Tile, Policy > &arg, Op &&op, bool fence=true) |
Modify each tile of a dense Array. More... | |
template<typename ResultTile , typename ArgTile , typename Policy , typename Op , typename = typename std::enable_if< !std::is_same<ResultTile, ArgTile>::value>::type> | |
std::enable_if_t<!is_dense_v< Policy >, DistArray< ResultTile, Policy > > | foreach (const DistArray< ArgTile, Policy > arg, Op &&op) |
Apply a function to each tile of a sparse Array. More... | |
template<typename Tile , typename Policy , typename Op > | |
std::enable_if_t<!is_dense_v< Policy >, DistArray< Tile, Policy > > | foreach (const DistArray< Tile, Policy > &arg, Op &&op) |
Apply a function to each tile of a sparse Array. More... | |
template<typename Tile , typename Policy , typename Op , typename = typename std::enable_if<!TiledArray::detail::is_array< typename std::decay<Op>::type>::value>::type> | |
std::enable_if_t<!is_dense_v< Policy >, void > | foreach_inplace (DistArray< Tile, Policy > &arg, Op &&op, bool fence=true) |
Modify each tile of a sparse Array. More... | |
template<typename ResultTile , typename LeftTile , typename RightTile , typename Policy , typename Op , typename = typename std::enable_if< !std::is_same<ResultTile, LeftTile>::value>::type> | |
std::enable_if_t< is_dense_v< Policy >, DistArray< ResultTile, Policy > > | foreach (const DistArray< LeftTile, Policy > &left, const DistArray< RightTile, Policy > &right, Op &&op) |
template<typename LeftTile , typename RightTile , typename Policy , typename Op > | |
std::enable_if_t< is_dense_v< Policy >, DistArray< LeftTile, Policy > > | foreach (const DistArray< LeftTile, Policy > &left, const DistArray< RightTile, Policy > &right, Op &&op) |
template<typename LeftTile , typename RightTile , typename Policy , typename Op > | |
std::enable_if_t< is_dense_v< Policy >, void > | foreach_inplace (DistArray< LeftTile, Policy > &left, const DistArray< RightTile, Policy > &right, Op &&op, bool fence=true) |
This function takes two input tiles and put result into the left tile. More... | |
template<typename ResultTile , typename LeftTile , typename RightTile , typename Policy , typename Op , typename = typename std::enable_if< !std::is_same<ResultTile, LeftTile>::value>::type> | |
std::enable_if_t<!is_dense_v< Policy >, DistArray< ResultTile, Policy > > | foreach (const DistArray< LeftTile, Policy > &left, const DistArray< RightTile, Policy > &right, Op &&op, const ShapeReductionMethod shape_reduction=ShapeReductionMethod::Intersect) |
template<typename LeftTile , typename RightTile , typename Policy , typename Op > | |
std::enable_if_t<!is_dense_v< Policy >, DistArray< LeftTile, Policy > > | foreach (const DistArray< LeftTile, Policy > &left, const DistArray< RightTile, Policy > &right, Op &&op, const ShapeReductionMethod shape_reduction=ShapeReductionMethod::Intersect) |
template<typename LeftTile , typename RightTile , typename Policy , typename Op > | |
std::enable_if_t<!is_dense_v< Policy >, void > | foreach_inplace (DistArray< LeftTile, Policy > &left, const DistArray< RightTile, Policy > &right, Op &&op, const ShapeReductionMethod shape_reduction=ShapeReductionMethod::Intersect, bool fence=true) |
This function takes two input tiles and put result into the left tile. More... | |
TiledArray initialization. | |
These functions initialize TiledArray and (if needed) MADWorld runtime.
| |
void | ta_abort () |
void | ta_abort (const std::string &m) |
void | finalize () |
TiledArray initialization. | |
These functions initialize TiledArray and (if needed) MADWorld runtime.
| |
World & | initialize (int &argc, char **&argv, const SafeMPI::Intracomm &comm, bool quiet=true) |
World & | initialize (int &argc, char **&argv, bool quiet=true) |
World & | initialize (int &argc, char **&argv, const MPI_Comm &comm, bool quiet=true) |
Variables | |
template<typename T > | |
constexpr const bool | is_dense_v = is_dense<T>::value |
template<typename T > | |
constexpr const bool | is_lazy_tile_v = is_lazy_tile<T>::value |
is_lazy_tile_v<T> is an alias for is_lazy_tile<T>::value More... | |
template<typename T > | |
constexpr const bool | is_consumable_tile_v = is_consumable_tile<T>::value |
is_consumable_tile_v<T> is an alias for is_consumable_tile<T>::value More... | |
template<typename T , typename SizeType = std::size_t> | |
constexpr auto | initializer_list_rank_v |
Helper variable for retrieving the degree of nesting for an std::initializer_list. More... | |
Typedef Documentation
◆ Array
using TiledArray::Array = typedef DistArray<Tile, Policy> |
Definition at line 117 of file tiledarray_fwd.h.
◆ EigenMatrixXcd
typedef Eigen::Matrix<std::complex<double>, Eigen::Dynamic, Eigen::Dynamic, Eigen::RowMajor> TiledArray::EigenMatrixXcd |
◆ EigenMatrixXcf
typedef Eigen::Matrix<std::complex<float>, Eigen::Dynamic, Eigen::Dynamic, Eigen::RowMajor> TiledArray::EigenMatrixXcf |
◆ EigenMatrixXd
typedef Eigen::Matrix<double, Eigen::Dynamic, Eigen::Dynamic, Eigen::RowMajor> TiledArray::EigenMatrixXd |
◆ EigenMatrixXf
typedef Eigen::Matrix<float, Eigen::Dynamic, Eigen::Dynamic, Eigen::RowMajor> TiledArray::EigenMatrixXf |
◆ EigenMatrixXi
typedef Eigen::Matrix<int, Eigen::Dynamic, Eigen::Dynamic, Eigen::RowMajor> TiledArray::EigenMatrixXi |
◆ EigenMatrixXl
typedef Eigen::Matrix<long, Eigen::Dynamic, Eigen::Dynamic, Eigen::RowMajor> TiledArray::EigenMatrixXl |
◆ EigenVectorXcd
typedef Eigen::Matrix<std::complex<double>, 1, Eigen::Dynamic> TiledArray::EigenVectorXcd |
◆ EigenVectorXcf
typedef Eigen::Matrix<std::complex<float>, 1, Eigen::Dynamic> TiledArray::EigenVectorXcf |
◆ EigenVectorXd
typedef Eigen::Matrix<double, Eigen::Dynamic, 1> TiledArray::EigenVectorXd |
◆ EigenVectorXf
typedef Eigen::Matrix<float, Eigen::Dynamic, 1> TiledArray::EigenVectorXf |
◆ EigenVectorXi
typedef Eigen::Matrix<int, Eigen::Dynamic, 1> TiledArray::EigenVectorXi |
◆ EigenVectorXl
typedef Eigen::Matrix<long, Eigen::Dynamic, 1> TiledArray::EigenVectorXl |
◆ result_of_trace_t
using TiledArray::result_of_trace_t = typedef decltype(trace(std::declval<T>())) |
◆ TArray
using TiledArray::TArray = typedef DistArray<Tensor<T, Eigen::aligned_allocator<T> >, DensePolicy> |
Definition at line 93 of file tiledarray_fwd.h.
◆ TArrayC
typedef TArray<std::complex<float> > TiledArray::TArrayC |
Definition at line 99 of file tiledarray_fwd.h.
◆ TArrayD
typedef TArray<double> TiledArray::TArrayD |
Definition at line 94 of file tiledarray_fwd.h.
◆ TArrayF
typedef TArray<float> TiledArray::TArrayF |
Definition at line 96 of file tiledarray_fwd.h.
◆ TArrayI
typedef TArray<int> TiledArray::TArrayI |
Definition at line 95 of file tiledarray_fwd.h.
◆ TArrayL
typedef TArray<long> TiledArray::TArrayL |
Definition at line 97 of file tiledarray_fwd.h.
◆ TArrayZ
typedef TArray<std::complex<double> > TiledArray::TArrayZ |
Definition at line 98 of file tiledarray_fwd.h.
◆ TensorC
typedef Tensor<std::complex<float>, Eigen::aligned_allocator<std::complex<float> > > TiledArray::TensorC |
Definition at line 59 of file tiledarray_fwd.h.
◆ TensorConstMap
using TiledArray::TensorConstMap = typedef detail::TensorInterface<typename std::add_const<T>::type, Range_, OpResult> |
Definition at line 48 of file tensor_map.h.
◆ TensorConstView
using TiledArray::TensorConstView = typedef detail::TensorInterface<typename std::add_const<T>::type, BlockRange> |
◆ TensorD
typedef Tensor<double, Eigen::aligned_allocator<double> > TiledArray::TensorD |
Definition at line 50 of file tiledarray_fwd.h.
◆ TensorF
typedef Tensor<float, Eigen::aligned_allocator<float> > TiledArray::TensorF |
Definition at line 52 of file tiledarray_fwd.h.
◆ TensorI
typedef Tensor<int, Eigen::aligned_allocator<int> > TiledArray::TensorI |
Definition at line 51 of file tiledarray_fwd.h.
◆ TensorL
typedef Tensor<long, Eigen::aligned_allocator<long> > TiledArray::TensorL |
Definition at line 53 of file tiledarray_fwd.h.
◆ TensorMap
using TiledArray::TensorMap = typedef detail::TensorInterface<T, Range_, OpResult> |
Definition at line 45 of file tensor_map.h.
◆ TensorView
using TiledArray::TensorView = typedef detail::TensorInterface<T, BlockRange> |
◆ TensorZ
typedef Tensor<std::complex<double>, Eigen::aligned_allocator<std::complex<double> > > TiledArray::TensorZ |
Definition at line 56 of file tiledarray_fwd.h.
◆ time_point
using TiledArray::time_point = typedef std::chrono::high_resolution_clock::time_point |
◆ TSpArray
using TiledArray::TSpArray = typedef DistArray<Tensor<T, Eigen::aligned_allocator<T> >, SparsePolicy> |
Definition at line 103 of file tiledarray_fwd.h.
◆ TSpArrayC
typedef TSpArray<std::complex<float> > TiledArray::TSpArrayC |
Definition at line 110 of file tiledarray_fwd.h.
◆ TSpArrayD
typedef TSpArray<double> TiledArray::TSpArrayD |
Definition at line 105 of file tiledarray_fwd.h.
◆ TSpArrayF
typedef TSpArray<float> TiledArray::TSpArrayF |
Definition at line 107 of file tiledarray_fwd.h.
◆ TSpArrayI
typedef TSpArray<int> TiledArray::TSpArrayI |
Definition at line 106 of file tiledarray_fwd.h.
◆ TSpArrayL
typedef TSpArray<long> TiledArray::TSpArrayL |
Definition at line 108 of file tiledarray_fwd.h.
◆ TSpArrayZ
typedef TSpArray<std::complex<double> > TiledArray::TSpArrayZ |
Definition at line 109 of file tiledarray_fwd.h.
Enumeration Type Documentation
◆ ExecutionSpace
|
strong |
◆ MemorySpace
|
strong |
enumerates the memory spaces
Enumerator | |
---|---|
Null | |
CPU | |
CUDA | |
CUDA_UM |
Definition at line 30 of file platform.h.
◆ OrdinalType
|
strong |
Specifies how coordinates are mapped to ordinal values
- RowMajor: stride decreases as mode index increases
- ColMajor: stride increases with the mode index
- Other: unknown or dynamic order
Enumerator | |
---|---|
RowMajor | |
ColMajor | |
Other | |
Invalid |
Definition at line 324 of file type_traits.h.
◆ ShapeReductionMethod
|
strong |
Function Documentation
◆ abs_max()
auto TiledArray::abs_max | ( | const DistArray< Tile, Policy > & | a | ) |
Definition at line 1635 of file dist_array.h.

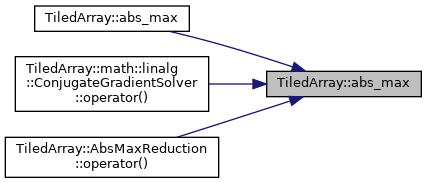
◆ abs_min()
auto TiledArray::abs_min | ( | const DistArray< Tile, Policy > & | a | ) |
Definition at line 1630 of file dist_array.h.

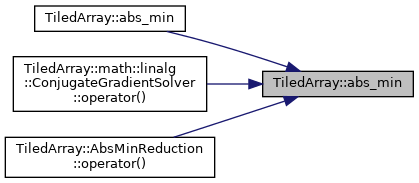
◆ absmax_reduce_cuda_kernel_impl()
T TiledArray::absmax_reduce_cuda_kernel_impl | ( | const T * | arg, |
std::size_t | n, | ||
cudaStream_t | stream, | ||
int | device_id | ||
) |
Definition at line 96 of file reduce_kernel_impl.h.
◆ absmin_reduce_cuda_kernel_impl()
T TiledArray::absmin_reduce_cuda_kernel_impl | ( | const T * | arg, |
std::size_t | n, | ||
cudaStream_t | stream, | ||
int | device_id | ||
) |
Definition at line 113 of file reduce_kernel_impl.h.
◆ add() [1/4]
|
inline |
◆ add() [2/4]
|
inline |
Add and permute tile arguments.
- Template Parameters
-
Left The left-hand tile type Right The right-hand tile type
- Parameters
-
left The left-hand argument to be added right The right-hand argument to be added perm The permutation to be applied to the result
- Returns
- A tile that is equal to
perm ^ (left + right)
◆ add() [3/4]
|
inline |
Add and scale tile arguments.
- Template Parameters
-
Left The left-hand tile type Right The right-hand tile type Scalar A scalar type
- Parameters
-
left The left-hand argument to be added right The right-hand argument to be added factor The scaling factor
- Returns
- A tile that is equal to
(left + right) * factor
◆ add() [4/4]
|
inline |
Add, scale, and permute tile arguments.
- Template Parameters
-
Left The left-hand tile type Right The right-hand tile type Scalar A scalar type
- Parameters
-
left The left-hand argument to be added right The right-hand argument to be added factor The scaling factor perm The permutation to be applied to the result
- Returns
- A tile that is equal to
perm ^ (left + right) * factor
◆ add_to() [1/2]
|
inline |
◆ add_to() [2/2]
|
inline |
Add and scale to the result tile.
- Template Parameters
-
Result The result tile type Arg The argument tile type Scalar A scalar type
- Parameters
-
result The result tile arg The argument to be added to result
factor The scaling factor
- Returns
- A tile that is equal to
(result[i] += arg[i]) *= factor
◆ array_from_il() [1/2]
auto TiledArray::array_from_il | ( | World & | world, |
const TiledRange & | trange, | ||
T && | il | ||
) |
Converts an std::initializer_list
into a tiled array.
This function encapsulates the process of turning an std::initializer_list
into a TiledArray array. The resulting tensor will have a tiling consistent with the provided TiledRange, trange
.
- Note
- This function will raise a static assertion if you try to construct a rank 0 tensor (i.e., you pass in a single element and not an
std::initializer_list
).
- Template Parameters
-
ArrayType The type of the array we are creating. Expected to be have an API akin to that of DistArray T The type of the provided std::initializer_list
.
- Parameters
-
[in] world The context in which the resulting tensor will live. [in] trange The tiling for the resulting tensor. [in] il The initializer_list containing the initial state of the tensor. il
is assumed to be non-empty and in row-major form. The nesting ofil
will be used to determine the rank of the resulting tensor.
- Returns
- A newly created instance of type
ArrayType
whose state is derived fromil
and exists in theworld
context.
- Exceptions
-
TiledArray::Exception if il
contains no elements. If an exception is raisedworld
,trange
, andil
are unchanged (strong throw guarantee).TiledArray::Exception If the provided std::initializer_list
is not rectangular (e.g., attempting to initialize a matrix with the value{{1, 2}, {3, 4, 5}}
). If an exception is raisedworld
,trange
, andil
are unchanged.
Definition at line 315 of file initializer_list.h.

◆ array_from_il() [2/2]
auto TiledArray::array_from_il | ( | World & | world, |
T && | il | ||
) |
Converts an std::initializer_list
into a single tile array.
This function encapsulates the process of turning an std::initializer_list
into a TiledArray array. The resulting tensor will consistent of a single tile which holds all of the values.
- Note
- This function will raise a static assertion if you try to construct a rank 0 tensor (i.e., you pass in a single element and not an
std::initializer_list
).
- Template Parameters
-
ArrayType The type of the array we are creating. Expected to be have an API akin to that of DistArray T The type of the provided std::initializer_list
.
- Parameters
-
[in] world The context in which the resulting tensor will live. [in] il The initializer_list containing the initial state of the tensor. il
is assumed to be non-empty and in row-major form. The nesting ofil
will be used to determine the rank of the resulting tensor.
- Returns
- A newly created instance of type
ArrayType
whose state is derived fromil
and exists in theworld
context.
- Exceptions
-
TiledArray::Exception if il
contains no elements. If an exception is raisedworld
andil
are unchanged (strong throw guarantee).TiledArray::Exception If the provided std::initializer_list
is not rectangular (e.g., attempting to initialize a matrix with the value{{1, 2}, {3, 4, 5}}
). If an exception is raisedworld
andil
are unchanged.
Definition at line 367 of file initializer_list.h.

◆ array_to_btas_tensor()
btas::Tensor<typename Tile::value_type, btas::DEFAULT::range, Storage> TiledArray::array_to_btas_tensor | ( | const TiledArray::DistArray< Tile, Policy > & | src, |
int | target_rank = -1 |
||
) |
Convert a TiledArray::DistArray object into a btas::Tensor object.
This function will copy the contents of src
into a btas::Tensor
object. The copy operation is done in parallel, and this function will block until all elements of src
have been copied into the result array tiles. The size of src.world()
.size() must be equal to 1 or src
must be a replicated TiledArray::DistArray. Usage:
- Template Parameters
-
Tile the tile type of src
Policy the policy type of src
- Parameters
-
[in] src The TiledArray::DistArray<Tile,Policy> object whose contents will be copied to the result.
- Returns
- A
btas::Tensor
object that is a copy ofsrc
- Exceptions
-
TiledArray::Exception When world size is greater than 1 and src
is not replicated
- Parameters
-
[in] target_rank the rank on which to create the BTAS tensor containing the data of src
; iftarget_rank=-1
then create the BTAS tensor on every rank (this requires thatsrc.is_replicated()==true
)
- Returns
- BTAS tensor object containing the data of
src
, if my rank equalstarget_rank
ortarget_rank==-1
, default-initialized BTAS tensor otherwise.
Definition at line 301 of file btas.h.
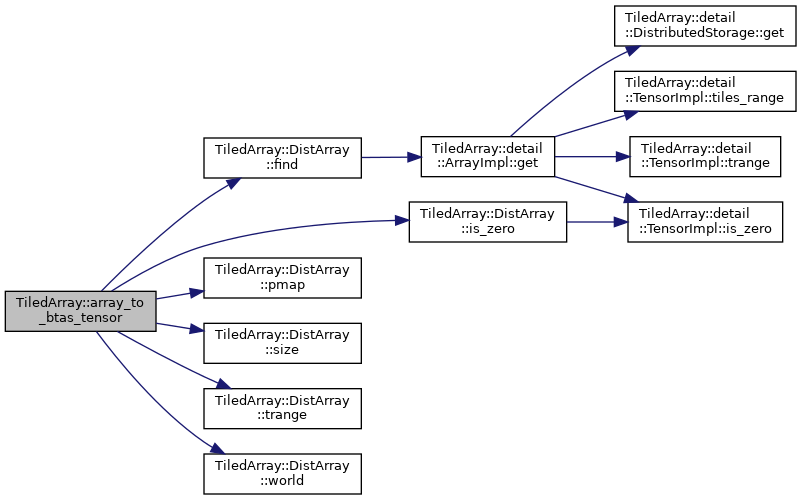
◆ array_to_eigen()
Eigen::Matrix<typename Tile::value_type, Eigen::Dynamic, Eigen::Dynamic, EigenStorageOrder> TiledArray::array_to_eigen | ( | const DistArray< Tile, Policy > & | array | ) |
Convert an Array object into an Eigen matrix object.
This function will copy the content of an Array
object into matrix. The copy operation is done in parallel, and this function will block until all elements of array
have been copied into the result matrix. The size of world must be exactly equal to 1, or array
must be a replicated object. Usage:
- Template Parameters
-
Tile The array tile type EigenStorageOrder The storage order of the resulting Eigen::Matrix object; the default is Eigen::ColMajor, i.e. the column-major storage
- Parameters
-
array The array to be converted. It must be replicated if using 2 or more World ranks.
- Returns
- an Eigen matrix; it will contain same data on each World rank.
- Exceptions
-
TiledArray::Exception When world size is greater than 1 and array
is not replicated.TiledArray::Exception When the number of dimensions of array
is not equal to 1 or 2.
Definition at line 496 of file eigen.h.
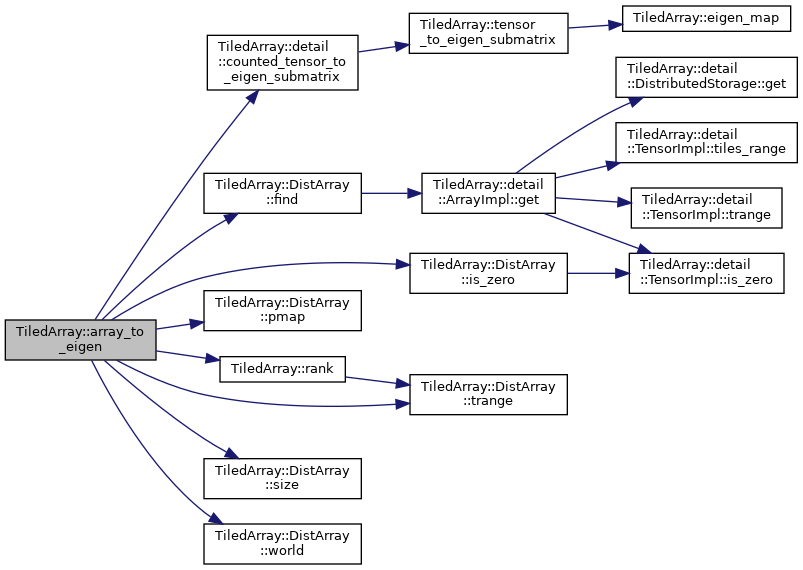
◆ assert_failed()
|
inline |
◆ btas_subtensor_to_tensor()
|
inline |
Copy a block of a btas::Tensor into a TiledArray::Tensor.
A block of btas::Tensor src
will be copied into TiledArray::Tensor dst
. The block dimensions will be determined by the dimensions of the range of dst
.
- Template Parameters
-
T The tensor element type Range_ The range type of the source btas::Tensor object Storage_ The storage type of the source btas::Tensor object Tensor_ A tensor type (e.g., TiledArray::Tensor or btas::Tensor, optionally wrapped into TiledArray::Tile)
- Parameters
-
[in] src The source object; its subblock defined by the {lower,upper} bounds {dst.lobound()
,dst.upbound()} will be copied todst
[out] dst The object that will contain the contents of the corresponding subblock of src
- Exceptions
-
TiledArray::Exception When the dimensions of src
anddst
do not match.
Definition at line 60 of file btas.h.
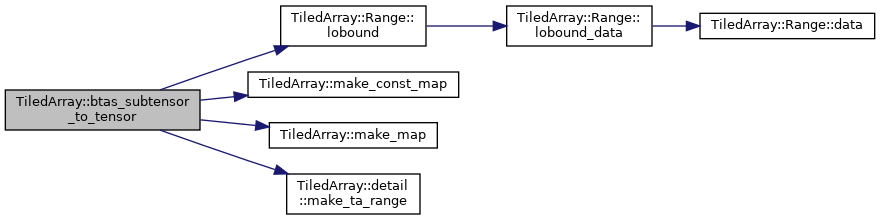

◆ btas_tensor_to_array()
DistArray_ TiledArray::btas_tensor_to_array | ( | World & | world, |
const TiledArray::TiledRange & | trange, | ||
const btas::Tensor< T, Range, Storage > & | src, | ||
bool | replicated = false |
||
) |
Convert a btas::Tensor object into a TiledArray::DistArray object.
This function will copy the contents of src
into a DistArray_
object that is tiled according to the trange
object. If the DistArray_
object has sparse policy, a sparse map with large norm is created to ensure all the values from src
copy to the DistArray_
object. The copy operation is done in parallel, and this function will block until all elements of src
have been copied into the result array tiles. The size of world.size()
must be equal to 1 or replicate
must be equal to true
. If replicate
is true
, it is your responsibility to ensure that the data in src
is identical on all nodes. Upon completion, if the DistArray_
object has sparse policy truncate() is called.
Usage:
- Template Parameters
-
DistArray_ a TiledArray::DistArray type TArgs the type pack in type btas::Tensor<TArgs...> of src
- Parameters
-
[in,out] world The world where the result array will live [in] trange The tiled range of the new array [in] src The btas::Tensor<TArgs..> object whose contents will be copied to the result. [in] replicated true
indicates that the result array should be a replicated array [default = false].
- Returns
- A
DistArray_
object that is a copy ofsrc
- Exceptions
-
TiledArray::Exception When world size is greater than 1
- Note
- If using 2 or more World ranks, set
replicated=true
and make surematrix
is the same on each rank!
Definition at line 212 of file btas.h.
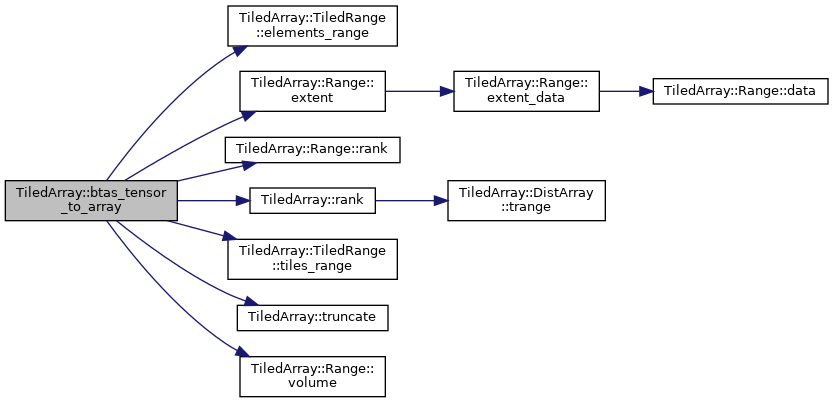
◆ clone() [1/2]
|
inline |
◆ clone() [2/2]
◆ column_major_buffer_to_array()
|
inline |
Convert a column-major matrix buffer into an Array object.
This function will copy the content of buffer
into an Array
object that is tiled according to the trange
object. The copy operation is done in parallel, and this function will block until all elements of matrix
have been copied into the result array tiles. Each tile is created using the local contents of matrix
, hence it is your responsibility to ensure that the data in matrix
is distributed correctly among the ranks. If in doubt, you should replicate matrix
among the ranks prior to calling this.
Usage:
- Template Parameters
-
A The array type
- Parameters
-
world The world where the array will live trange The tiled range of the new array buffer The row-major matrix buffer to be copied m The number of rows in the matrix n The number of columns in the matrix replicated if true, the result will be replicated [default = true]. pmap the process map object [default=null]; initialized to the default if replicated
is false, or a replicated pmap ifreplicated
is true; ignored ifreplicated
is true andworld.size()>1
- Returns
- An
Array
object that is a copy ofmatrix
- Exceptions
-
TiledArray::Exception When m
andn
are not equal to the number of rows or columns in tiled range.
◆ concat()
|
inline |
Concatenates two ranges.
Tiles of the second range are concatenated to the tiles of the first. For example:
- Parameters
-
r1 first range r2 second range
- Returns
- concatenated range
Definition at line 339 of file tiled_range1.h.
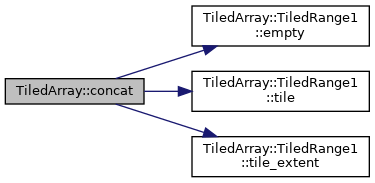
◆ congruent()
◆ device_data() [1/2]
const T* TiledArray::device_data | ( | const cpu_cuda_vector< T > & | storage | ) |
◆ device_data() [2/2]
T* TiledArray::device_data | ( | cpu_cuda_vector< T > & | storage | ) |
◆ diagonal_array() [1/2]
std::enable_if_t<detail::is_iterator<RandomAccessIterator>::value, Array> TiledArray::diagonal_array | ( | World & | world, |
TiledRange const & | trange, | ||
RandomAccessIterator | diagonals_begin, | ||
RandomAccessIterator | diagonals_end = {} |
||
) |
Creates a non-constant diagonal DistArray.
Creates an array whose only nonzero values are the (hyper)diagonal elements (i.e. (n,n,n, ..., n) ); the values of the diagonal elements are given by an input range
- Template Parameters
-
Array a DistArray type RandomAccessIterator an iterator over the range of diagonal elements
- Parameters
-
world The world for the array [in] trange The trange for the array [in] diagonals_begin the begin iterator of the range of the diagonals [in] diagonals_end the end iterator of the range of the diagonals; if not given, default initialized and thus will not be checked
- Returns
- a constant diagonal DistArray
Definition at line 262 of file diagonal_array.h.
◆ diagonal_array() [2/2]
Array TiledArray::diagonal_array | ( | World & | world, |
TiledRange const & | trange, | ||
T | val = 1 |
||
) |
Creates a constant diagonal DistArray.
Creates an array whose only nonzero values are the (hyper)diagonal elements (i.e. (n,n,n, ..., n) ), and they are all have the same value
- Template Parameters
-
Policy the policy type of the resulting DistArray T a numeric type
- Parameters
-
world The world for the array [in] trange The trange for the array [in] val The value of the diagonal elements
- Returns
- a constant diagonal DistArray
Definition at line 230 of file diagonal_array.h.
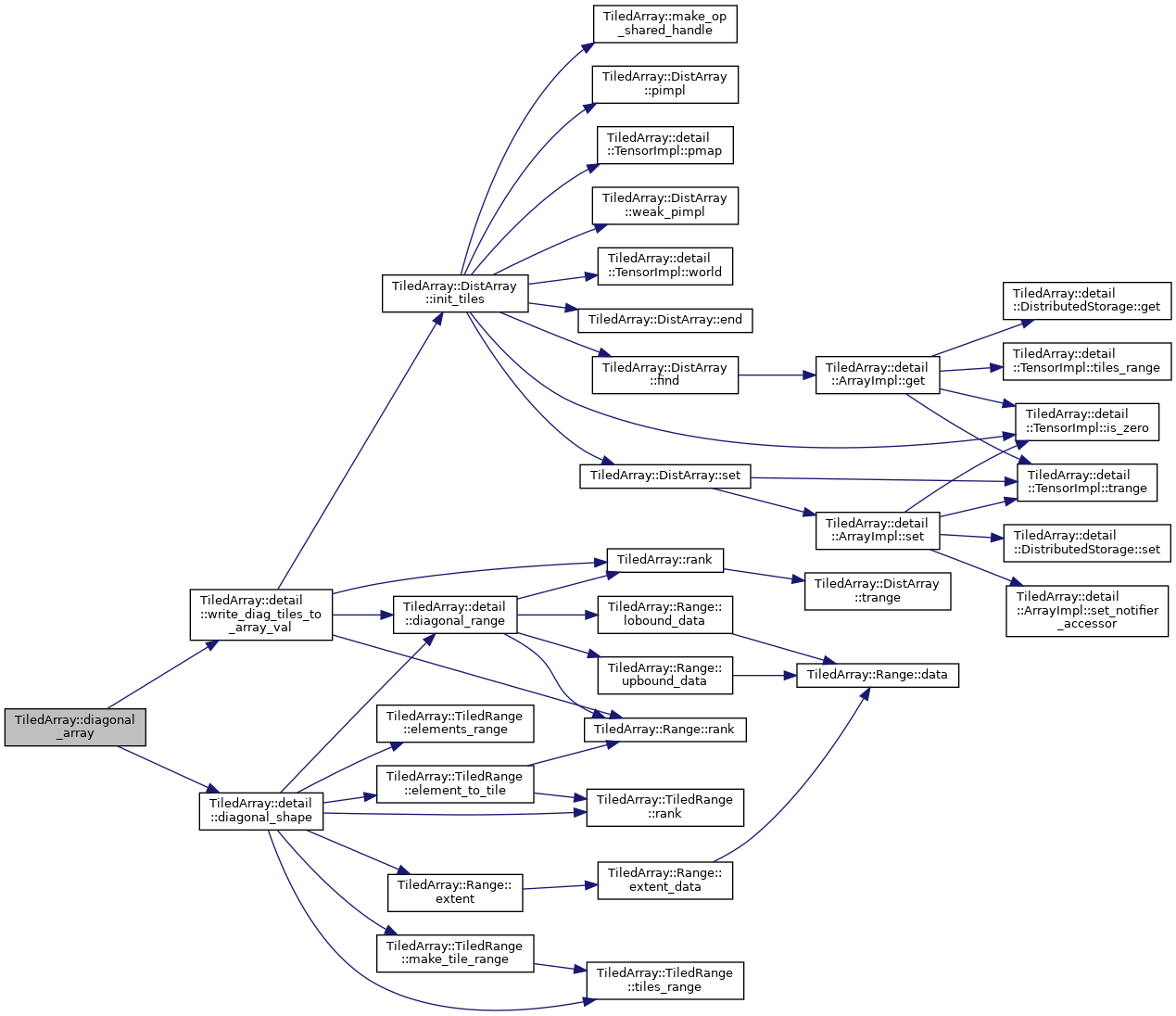
◆ dot()
auto TiledArray::dot | ( | const DistArray< Tile, Policy > & | a, |
const DistArray< Tile, Policy > & | b | ||
) |
Definition at line 1640 of file dist_array.h.
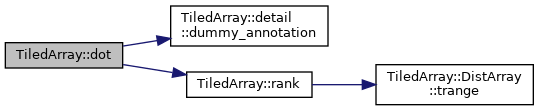
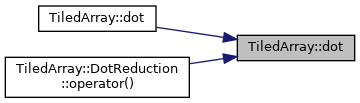
◆ duration_in_ns()
|
inline |
◆ duration_in_s()
|
inline |
◆ eigen_map() [1/6]
|
inline |
Construct a const Eigen::Map object for a given Tensor object.
The dimensions of the result tensor are extracted from the tensor itself
- Template Parameters
-
T A contiguous tensor type, e.g. TiledArray::Tensor ; namely, TiledArray::detail::is_contiguous_tensor_v<T>
must be trueStorage the tensor layout, either Eigen::RowMajor (default) or Eigen::ColMajor
- Parameters
-
tensor The tensor object, laid out according to Storage
- Returns
- An Eigen matrix map for
tensor
- Exceptions
-
TiledArray::Exception When tensor
dimensions are not equal to 2 or 1.
◆ eigen_map() [2/6]
|
inline |
Construct a const Eigen::Map object for a given Tensor object.
- Template Parameters
-
T A contiguous tensor type, e.g. TiledArray::Tensor ; namely, TiledArray::detail::is_contiguous_tensor_v<T>
must be trueStorage the tensor layout, either Eigen::RowMajor (default) or Eigen::ColMajor
- Parameters
-
tensor The tensor object, laid out according to Storage m The number of rows in the result matrix n The number of columns in the result matrix
- Returns
- An m x n Eigen matrix map for
tensor
- Exceptions
-
TiledArray::Exception When m * n is not equal to tensor
size
Definition at line 77 of file eigen.h.
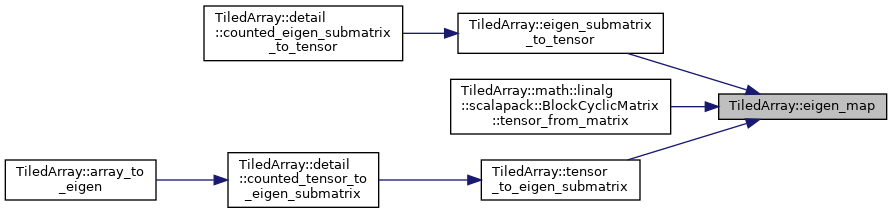
◆ eigen_map() [3/6]
|
inline |
Construct a const Eigen::Map object for a given Tensor object.
- Template Parameters
-
T A contiguous tensor type, e.g. TiledArray::Tensor ; namely, TiledArray::detail::is_contiguous_tensor_v<T>
must be true
- Parameters
-
tensor The tensor object n The number of elements in the result matrix
- Returns
- An n element Eigen vector map for
tensor
- Exceptions
-
TiledArray::Exception When n is not equal to tensor
size
◆ eigen_map() [4/6]
|
inline |
Construct an Eigen::Map object for a given Tensor object.
The dimensions of the result tensor are extracted from the tensor itself
- Template Parameters
-
T A contiguous tensor type, e.g. TiledArray::Tensor ; namely, TiledArray::detail::is_contiguous_tensor_v<T>
must be trueStorage the tensor layout, either Eigen::RowMajor (default) or Eigen::ColMajor
- Parameters
-
tensor The tensor object, laid out according to Storage
- Returns
- An Eigen matrix map for
tensor
- Exceptions
-
When tensor
dimensions are not equal to 2 or 1.
◆ eigen_map() [5/6]
|
inline |
Construct an Eigen::Map object for a given Tensor object.
- Template Parameters
-
T A contiguous tensor type, e.g. TiledArray::Tensor ; namely, TiledArray::detail::is_contiguous_tensor_v<T>
must be trueStorage the tensor layout, either Eigen::RowMajor (default) or Eigen::ColMajor
- Parameters
-
tensor The tensor object, laid out according to Storage m The number of rows in the result matrix n The number of columns in the result matrix
- Returns
- An m x n Eigen matrix map for
tensor
- Exceptions
-
TiledArray::Exception When m * n is not equal to tensor
size
◆ eigen_map() [6/6]
|
inline |
Construct an Eigen::Map object for a given Tensor object.
- Template Parameters
-
T A tensor type, e.g. TiledArray::Tensor
- Parameters
-
tensor The tensor object n The number of elements in the result matrix
- Returns
- An n element Eigen vector map for
tensor
- Exceptions
-
TiledArray::Exception When n is not equal to tensor
size
◆ eigen_submatrix_to_tensor()
|
inline |
Copy a block of an Eigen matrix into a tensor.
A block of matrix
will be copied into tensor
. The block dimensions will be determined by the dimensions of the tensor's range.
- Template Parameters
-
T A tensor type, e.g. TiledArray::Tensor Derived The derived type of an Eigen matrix
- Parameters
-
[in] matrix The object that will be assigned the content of tensor
[out] tensor The object that will be assigned the content of matrix
- Exceptions
-
TiledArray::Exception When the dimensions of tensor
are not equal to 1 or 2.TiledArray::Exception When the range of tensor
is outside the range ofmatrix
.
Definition at line 207 of file eigen.h.


◆ eigen_to_array()
A TiledArray::eigen_to_array | ( | World & | world, |
const typename A::trange_type & | trange, | ||
const Eigen::MatrixBase< Derived > & | matrix, | ||
bool | replicated = false , |
||
std::shared_ptr< typename A::pmap_interface > | pmap = {} |
||
) |
Convert an Eigen matrix into an Array object.
This function will copy the content of matrix
into an Array
object that is tiled according to the trange
object. The copy operation is done in parallel, and this function will block until all elements of matrix
have been copied into the result array tiles. Each tile is created using the local contents of matrix
, hence it is your responsibility to ensure that the data in matrix
is distributed correctly among the ranks. If in doubt, you should replicate matrix
among the ranks prior to calling this.
Usage:
- Template Parameters
-
A The array type Derived The Eigen matrix derived type
- Parameters
-
world The world where the array will live trange The tiled range of the new array matrix The Eigen matrix to be copied replicated if true, the result will be replicated [default = true]. pmap the process map object [default=null]; initialized to the default if replicated
is false, or a replicated pmap ifreplicated
is true; ignored ifreplicated
is true andworld.size()>1
- Returns
- An
Array
object that is a copy ofmatrix
◆ exception_break()
|
inline |
Place a break point on this function to stop before TiledArray exceptions are thrown.
Definition at line 62 of file error.h.

◆ finalize()
void TiledArray::finalize | ( | ) |
Finalizes TiledArray (and MADWorld runtime, if it had not been initialized when TiledArray::initialize was called).
Definition at line 118 of file tiledarray.cpp.

◆ finalized()
bool TiledArray::finalized | ( | ) |
- Returns
- true if TiledArray has been finalized at least once
Definition at line 66 of file tiledarray.cpp.

◆ flatten_il()
auto TiledArray::flatten_il | ( | T && | il, |
OutputItr | out_itr | ||
) |
Flattens the contents of a (possibly nested) initializer_list into the provided buffer.
This function is used to flatten a (possibly nested) std::initializer_list
into a buffer provided by the user. The flattening occurs by iterating over the layers of the std::initializer_list
in a depth-first manner. As the initializer_list is flattened the data is copied into the container associated with out_itr
. It is assumed that the container associated with out_itr
is already allocated or that out_itr
will internally allocate the memory on-the-fly (e.g. std::back_insert_iterator
).
This function works with empty std::initializer_list
instances (you will get back out_itr
unchanged and the corresponding container is unchanged) as well as single tensor elements (i.e., initializing a scalar); in the latter case the buffer corresponding to out_itr
must contain room for at least one element as the element will be copied to the buffer.
- Template Parameters
-
T Expected to be the type of a tensor element (i.e. float, double, etc.) or a (possibly nested) std::initializer_list
of tensor elements.OutputItr The type of an iterator which can be used to fill a container. It must satisfy the concept of Output Iterator.
- Parameters
-
[in] il The std::initializer_list
we are flattening.[in] out_itr An iterator pointing to the first element where data should be copied to. Memory in the destination container is assumed to be pre-allocated otherwise @
- Returns
out_itr
pointing to just past the last element inserted by this function.
- Exceptions
-
TiledArray::Exception If the provided std::initializer_list
is not rectangular (e.g., attempting to initialize a matrix with the value{{1, 2}, {3, 4, 5}}
). If an exception is thrownil
andout_itr
are in their original state (strong throw guarantee).
Definition at line 205 of file initializer_list.h.
◆ foreach() [1/8]
|
inline |
Apply a function to each tile of a dense Array.
This function uses an Array
object to generate a new Array
where the output tiles are a function of the input tiles. Users must provide a function/functor that initializes the tiles for the new Array
object. For example, if we want to create a new array with were each element is equal to the square root of the corresponding element of the original array:
The expected signature of the tile operation is:
- Template Parameters
-
ResultTile The tile type of the result array ArgTile The tile type of arg
Policy The policy type of arg
;is_dense_v<Policy>
must be trueOp Tile operation
- Parameters
-
op The tile function arg The argument array
◆ foreach() [2/8]
|
inline |
Apply a function to each tile of a sparse Array.
This function uses an Array
object to generate a new Array
where the output tiles are a function of the input tiles. Users must provide a function/functor that initializes the tiles for the new Array
object. For example, if we want to create a new array with were each element is equal to the square root of the corresponding element of the original array:
The expected signature of the tile operation is:
where in the case of standard Policy (i.e. SparsePolicy) the return value of op
is the 2-norm (Frobenius norm) of the result tile.
- Note
- This function should not be used to initialize the tiles of an array object.
- Template Parameters
-
ResultTile The tile type of the result Tile The tile type of arg
Policy The policy type of arg
;is_dense_v<Policy>
must be falseOp Tile operation
- Parameters
-
arg The argument array op The tile function
◆ foreach() [3/8]
|
inline |
◆ foreach() [4/8]
|
inline |
◆ foreach() [5/8]
|
inline |
◆ foreach() [6/8]
|
inline |
◆ foreach() [7/8]
|
inline |
◆ foreach() [8/8]
|
inline |
◆ foreach_inplace() [1/4]
|
inline |
◆ foreach_inplace() [2/4]
|
inline |
◆ foreach_inplace() [3/4]
|
inline |
Modify each tile of a dense Array.
This function modifies the tile data of Array
object. Users must provide a function/functor that modifies the tile data. For example, if we want to modify the elements of the array to be equal to the square root of the original value:
The expected signature of the tile operation is:
- Template Parameters
-
Tile The tile type of arg
Policy The policy type of arg
;is_dense_v<Policy>
must be trueOp Mutating tile operation
- Parameters
-
arg The argument array to be modified op The mutating tile function fence A flag that indicates fencing behavior. If true
this function will fence before data is modified.
- Warning
- This function fences by default to avoid data race conditions. Only disable the fence if you can ensure, the data is not being read by another thread.
-
If there is a another copy of
arg
that was created via (or arg was created by) theArray
copy constructor or copy assignment operator, this function will modify the data of that array since the data of a tile is held in astd::shared_ptr
. If you need to ensure other copies of the data are not modified or this behavior causes problems in your application, use theTiledArray::foreach
function instead.
Definition at line 476 of file foreach.h.

◆ foreach_inplace() [4/4]
|
inline |
Modify each tile of a sparse Array.
This function modifies the tile data of Array
object. Users must provide a function/functor that modifies the tile data in place. For example, if we want to modify the elements of the array to be equal to the square root of the original value:
The expected signature of the tile operation is:
where for the standard Policy (i.e. SparsePolicy) the return value of op
is the 2-norm (Frobenius norm) of the tile.
- Note
- This function should not be used to initialize the tiles of an array object.
- Template Parameters
-
Tile The tile type of arg
Policy The policy type of arg
;is_dense_v<Policy>
must be falseOp Tile operation
- Parameters
-
arg The argument array to be modified op The mutating tile function fence A flag that indicates fencing behavior. If true
this function will fence before data is modified.
- Warning
- This function fences by default to avoid data race conditions. Only disable the fence if you can ensure, the data is not being read by another thread.
-
If there is a another copy of
arg
that was created via (or arg was created by) theArray
copy constructor or copy assignment operator, this function will modify the data of that array since the data of a tile is held in astd::shared_ptr
. If you need to ensure other copies of the data are not modified or this behavior causes problems in your application, use theTiledArray::foreach
function instead.
Definition at line 584 of file foreach.h.

◆ fuse_vector_of_arrays_tiles()
TA::DistArray<Tile, Policy> TiledArray::fuse_vector_of_arrays_tiles | ( | madness::World & | global_world, |
const std::vector< TA::DistArray< Tile, Policy >> & | array_vec, | ||
const std::size_t | fused_dim_extent, | ||
const TiledArray::TiledRange & | array_trange, | ||
std::size_t | block_size = 1 |
||
) |
fuses a vector of DistArray objects, each with the same TiledRange into a DistArray with 1 more dimensions
The leading dimension of the resulting array is the vector dimension, and will be blocked by @block_size .
- Parameters
-
global_world the world in which the result will live and across which this is invoked. [in] array_vec a vector of DistArray objects; every element of arrays
must have the same TiledRange object and live in the same world.[in] fused_dim_extent the extent of the resulting (fused) mode; equals the total number of arrays in a fused arrays
(sum ofarrays.size()
on each rank)[in] block_size the block size for the "vector" dimension of the tiled range of the result
- Returns
arrays
fused into a DistArray
- Note
- This is a collective function. It assumes that it is invoked across
global_world
, but the subarrays are "local" to each rank and distributed in tilewise-round-robin fashion. The result will live inglobal_world
.
- See also
- detail::fuse_vector_of_shapes_tiles TODO rename to fuse_tilewise_vector_of_arrays
copy the data from a sequence of tiles
write to blocks of fused_array
Definition at line 289 of file vector_of_arrays.h.
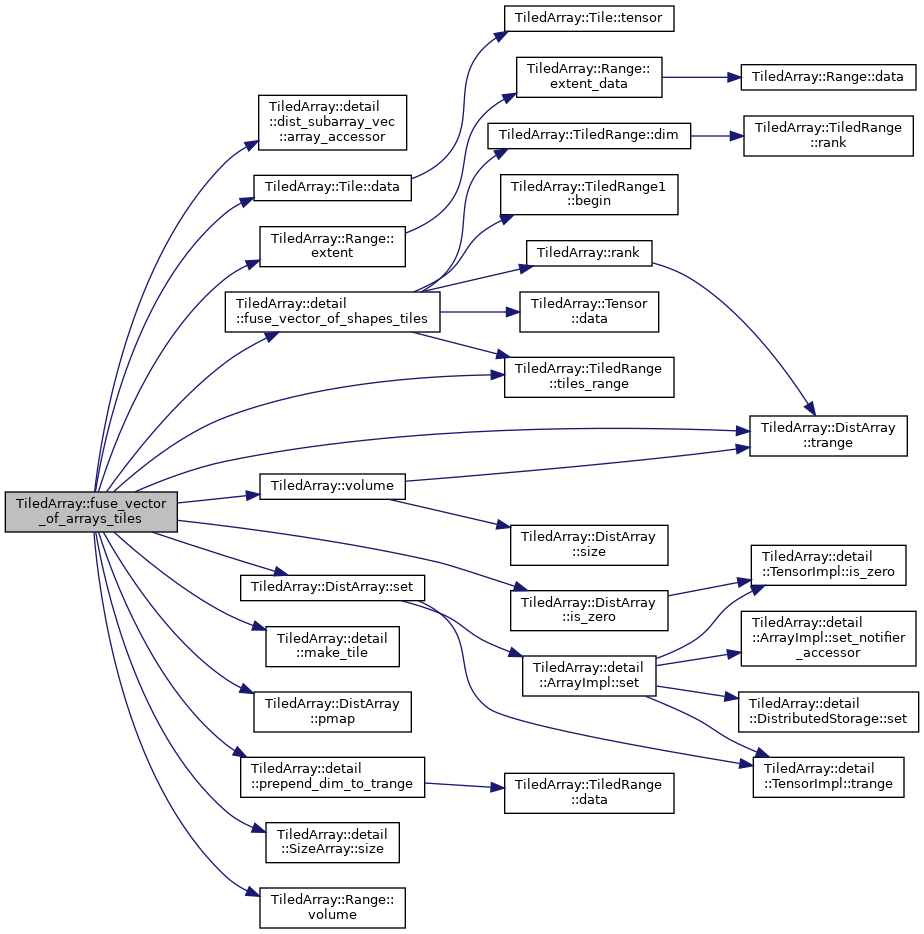
◆ get_default_world()
|
inline |
◆ get_elem_from_il()
auto TiledArray::get_elem_from_il | ( | T | idx, |
U && | il, | ||
std::size_t | depth = 0 |
||
) |
Retrieves the specified element from an initializer_list.
Given an initializer_list with nestings,
il
, and an element index,
idx
, this function will return the element which is offset idx[i]
along the -th mode of
il
.
- Template Parameters
-
T The type of the container holding the index. Assumed to be a random access container whose elements are of an integral type. U Assumed to be a scalar type (e.g. float, double, etc.) or a (possibly nested) std::initializer_list
of scalar types.
- Parameters
-
[in] idx The desired element's offsets along each mode. [in] il The initializer list we are retrieving the value from. [in] depth Used internally to keep track of how many levels of recursion have occurred. Defaults to 0 and should not be modified.
- Returns
- The requested element.
- Exceptions
-
TiledArray::Exception if the number of elements in idx
does not equal the nesting ofil
. Strong throw guarantee.TiledArray::Exception if the offset along a mode is greater than the length of the mode. Strong throw guarantee.
Definition at line 259 of file initializer_list.h.

◆ ignore_tile_position() [1/2]
|
inline |
Reports whether tile positions are checked in binary array operations. These checks are disabled if preprocessor symbol NDEBUG
is defined. By default, tile positions are checked.
- Returns
- if true, tile positions will be ignored in binary array operations.
Definition at line 90 of file utility.h.

◆ ignore_tile_position() [2/2]
|
inline |
Controls whether tile positions are checked in binary array operations. These checks are disabled if preprocessor symbol NDEBUG
is defined. By default, tile positions are checked.
- Parameters
-
[in] b if true, tile positions will be ignored in binary array operations.
- Warning
- this function should be called following a fence from the main thread only.
Definition at line 81 of file utility.h.

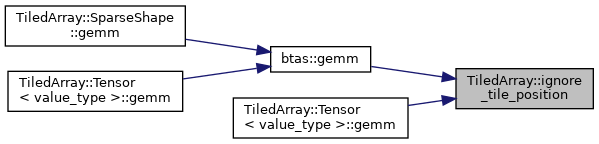
◆ in_memory_space()
|
noexcept |
◆ initialize() [1/3]
|
inline |
- Exceptions
-
TiledArray::Exception if TiledArray initialized MADWorld and TiledArray::finalize() had been called
Definition at line 38 of file initialize.h.

◆ initialize() [2/3]
|
inline |
- Exceptions
-
TiledArray::Exception if TiledArray initialized MADWorld and TiledArray::finalize() had been called
Definition at line 42 of file initialize.h.

◆ initialize() [3/3]
TiledArray::World & TiledArray::initialize | ( | int & | argc, |
char **& | argv, | ||
const SafeMPI::Intracomm & | comm, | ||
bool | quiet = true |
||
) |
- Exceptions
-
TiledArray::Exception if TiledArray initialized MADWorld and TiledArray::finalize() had been called
Definition at line 80 of file tiledarray.cpp.
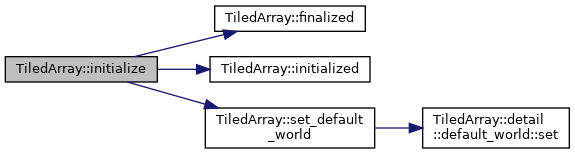

◆ initialized()
bool TiledArray::initialized | ( | ) |
- Returns
- true if TiledArray (and, necessarily, MADWorld runtime) is in an initialized state
Definition at line 63 of file tiledarray.cpp.
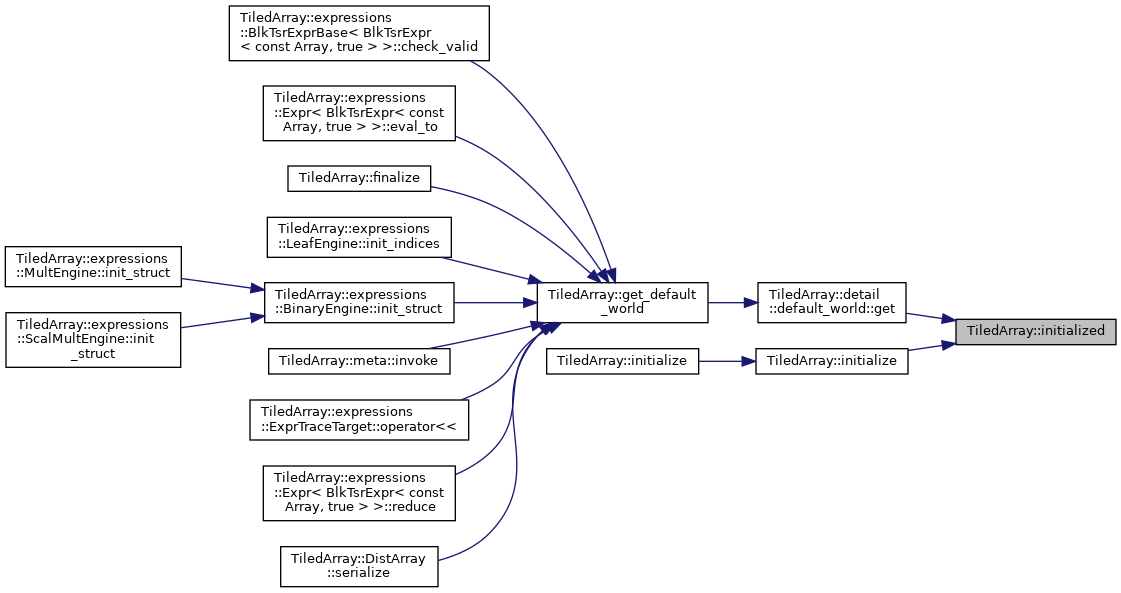
◆ inner() [1/2]
|
inline |
◆ inner() [2/2]
|
inline |
◆ inner_product()
auto TiledArray::inner_product | ( | const DistArray< Tile, Policy > & | a, |
const DistArray< Tile, Policy > & | b | ||
) |
Definition at line 1647 of file dist_array.h.

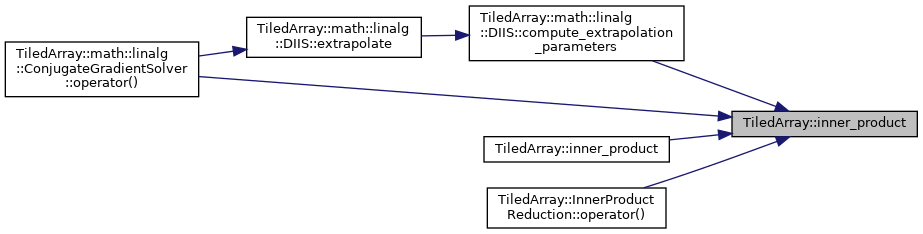
◆ inner_size() [1/2]
|
inline |
◆ inner_size() [2/2]
|
inline |
◆ invoke_cast()
auto TiledArray::invoke_cast | ( | Arg && | arg | ) |
Invokes TiledArray::Cast to cast/convert the argument to type Result. The operation may be nonblocking, if needed. The cast may involve zero, one, or more conversions, depending on the implementation of Cast<>, and the properties of types Arg and Result.
Definition at line 176 of file cast.h.

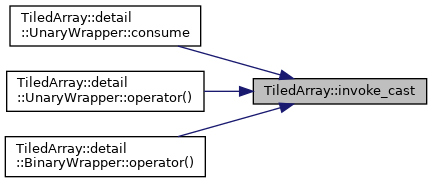
◆ is_congruent() [1/5]
|
inline |
Test that two BlockRange objects are congruent.
This function tests that the rank, extent of r1
is equal to that of r2
.
- Parameters
-
r1 The first BlockRange to compare r2 The second BlockRange to compare
Definition at line 400 of file block_range.h.
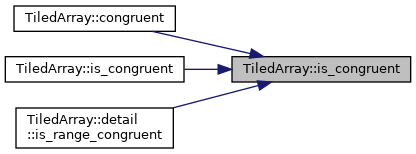
◆ is_congruent() [2/5]
|
inline |
Test that BlockRange and Range are congruent.
This function tests that the rank, extent of r1
is equal to that of r2
.
- Parameters
-
r1 The BlockRange to compare r2 The Range to compare
Definition at line 412 of file block_range.h.

◆ is_congruent() [3/5]
|
inline |
Test that Range and BlockRange are congruent.
This function tests that the rank, extent of r1
is equal to that of r2
.
- Parameters
-
r1 The Range to compare r2 The BlockRange to compare
Definition at line 423 of file block_range.h.

◆ is_congruent() [4/5]
◆ is_congruent() [5/5]
|
inline |
Test that two TiledRange1 objects are congruent.
This function tests that the tile sizes of the two ranges coincide.
- Template Parameters
-
Range The range type
- Parameters
-
r1 an TiledRange1 object r2 an TiledRange1 object
Definition at line 362 of file tiled_range1.h.
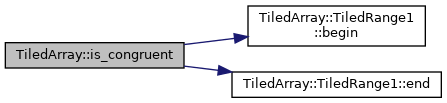
◆ is_contiguous() [1/2]
|
inline |
Tests whether a range is contiguous, i.e. whether its ordinal values form a contiguous range
- Parameters
-
range a BlockRange
- Returns
- true since TiledArray::BlockRange is contiguous by definition
Definition at line 443 of file block_range.h.
◆ is_contiguous() [2/2]
|
inline |
Tests whether a range is contiguous, i.e. whether its ordinal values form a contiguous range
- Parameters
-
range a Range
- Returns
- true since TiledArray::Range is contiguous by definition
◆ is_replicated() [1/2]
|
inlineconstexpr |
◆ is_replicated() [2/2]
bool TiledArray::is_replicated | ( | World & | world, |
const SparseShape< T > & | shape | ||
) |
collective bitwise-compare-reduce for SparseShape objects
- Parameters
-
world the World object [in] shape the SparseShape object
- Returns
- true if
shape
is bitwise identical acrossworld
- Note
- must be invoked on every rank of World
Definition at line 1622 of file sparse_shape.h.
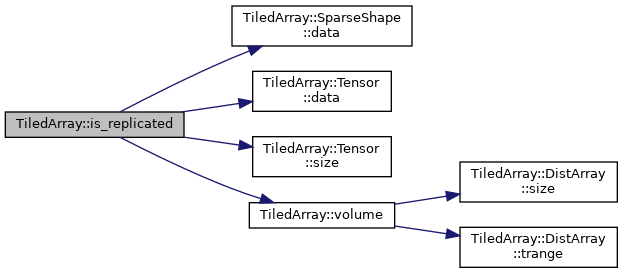
◆ launch_gdb_xterm()
void TiledArray::launch_gdb_xterm | ( | ) |
◆ launch_lldb_xterm()
void TiledArray::launch_lldb_xterm | ( | ) |
◆ make_array() [1/2]
|
inline |
Construct dense Array.
Construct sparse Array.
This function is used to construct a DistArray
object. Users must provide a world object, tiled range object, and function/functor that generates the tiles for the new array object. For example, if we want to create an array with were the elements are equal to 1
:
Note that the result is default constructed before (contains no data) and must be initialized inside the function/functor with the provided range object. The expected signature of the tile operation is:
where tile_t
and range_t
are your tile type and tile range type, respectively.
- Parameters
-
world The world where the array will live trange The tiled range of the array op The tile function/functor
- Returns
- An array object of type
Array
This function is used to construct a DistArray
object. Users must provide a world object, tiled range object, process map, and function/ functor that generates the tiles for the new array object. For example, if we want to create an array with all elements equal to 1
:
You may choose not to initialize a tile inside the tile initialization function (not shown in the example) by returning 0
for the tile norm. Note that the result is default constructed before (contains no data) and must be initialized inside the function/functor with the provided range object unless the returned tile norm is zero. The expected signature of the tile operation is:
where value_t
, tile_t
and range_t
are your tile value type, tile type, and tile range type, respectively.
- Parameters
-
world The world where the array will live trange The tiled range of the array pmap A shared pointer to the array process map op The tile function/functor
- Returns
- An array object of type
Array
Definition at line 73 of file make_array.h.
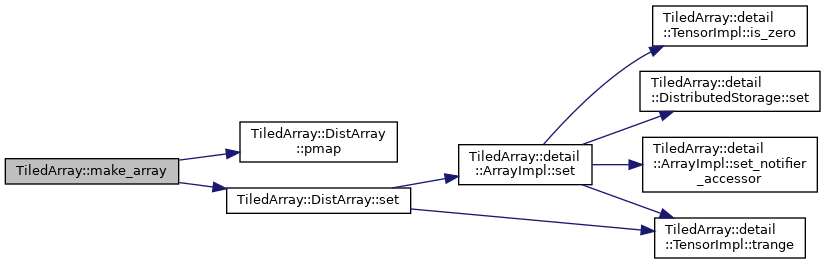

◆ make_array() [2/2]
|
inline |
Construct an Array.
This function is used to construct a DistArray
object. Users must provide a world object, tiled range object, and function/functor that generates the tiles for the new array object. For example, if we want to create an array with were the elements are equal to 1
:
For sparse arrays, you may choose not to initialize a tile inside the tile initialization (not shown in the example) by returning 0
for the tile norm. Note that the result is default constructed before (contains no data) and must be initialized inside the function/functor with the provided range object unless the returned tile norm is zero. The expected signature of the tile operation is:
where value_t
, tile_t
and range_t
are your tile value type, tile type, and tile range type, respectively.
- Parameters
-
world The world where the array will live trange The tiled range of the array op The tile function/functor
- Returns
- An array object of type
Array
Definition at line 222 of file make_array.h.
◆ make_const_map() [1/6]
|
inline |
◆ make_const_map() [2/6]
|
inline |
Definition at line 105 of file tensor_map.h.
◆ make_const_map() [3/6]
|
inline |
Definition at line 113 of file tensor_map.h.
◆ make_const_map() [4/6]
|
inline |
Definition at line 121 of file tensor_map.h.
◆ make_const_map() [5/6]
|
inline |
Definition at line 128 of file tensor_map.h.
◆ make_const_map() [6/6]
|
inline |
Definition at line 136 of file tensor_map.h.
◆ make_device_storage()
void TiledArray::make_device_storage | ( | cpu_cuda_vector< T > & | storage, |
std::size_t | n, | ||
cudaStream_t | stream = 0 |
||
) |
Definition at line 187 of file cpu_cuda_vector.h.
◆ make_map() [1/6]
|
inline |
Definition at line 74 of file tensor_map.h.
◆ make_map() [2/6]
|
inline |
Definition at line 82 of file tensor_map.h.
◆ make_map() [3/6]
|
inline |
Definition at line 90 of file tensor_map.h.
◆ make_map() [4/6]
|
inline |
◆ make_map() [5/6]
|
inline |
Definition at line 60 of file tensor_map.h.
◆ make_map() [6/6]
|
inline |
Definition at line 67 of file tensor_map.h.
◆ make_op_shared_handle()
auto TiledArray::make_op_shared_handle | ( | Op && | op | ) |
wraps Op into a shallow-copy copyable handle if Op is an rvalue ref makes an owning handle otherwise makes an non-owning handle
Definition at line 129 of file function.h.
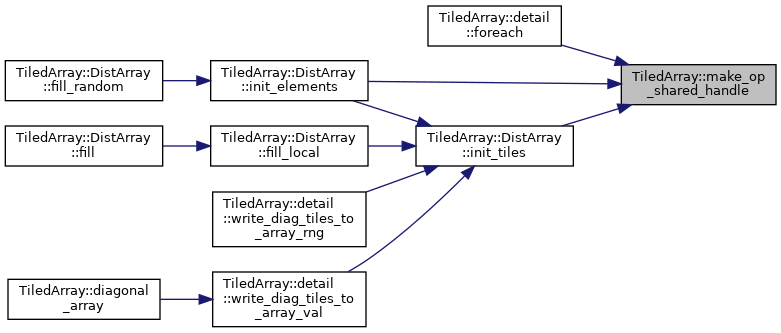
◆ make_shared_function()
shared_function<std::decay_t<F> > TiledArray::make_shared_function | ( | F && | f | ) |
Definition at line 39 of file function.h.
◆ max_reduce_cuda_kernel_impl()
T TiledArray::max_reduce_cuda_kernel_impl | ( | const T * | arg, |
std::size_t | n, | ||
cudaStream_t | stream, | ||
int | device_id | ||
) |
◆ min_reduce_cuda_kernel_impl()
T TiledArray::min_reduce_cuda_kernel_impl | ( | const T * | arg, |
std::size_t | n, | ||
cudaStream_t | stream, | ||
int | device_id | ||
) |
◆ mult_cuda_kernel_impl()
void TiledArray::mult_cuda_kernel_impl | ( | T * | result, |
const T * | arg1, | ||
const T * | arg2, | ||
std::size_t | n, | ||
cudaStream_t | stream, | ||
int | device_id | ||
) |
result[i] = arg1[i] * arg2[i]
Definition at line 48 of file mult_kernel_impl.h.
◆ mult_to_cuda_kernel_impl()
void TiledArray::mult_to_cuda_kernel_impl | ( | T * | result, |
const T * | arg, | ||
std::size_t | n, | ||
cudaStream_t | stream, | ||
int | device_id | ||
) |
result[i] = result[i] * arg[i]
Definition at line 35 of file mult_kernel_impl.h.
◆ norm2()
auto TiledArray::norm2 | ( | const DistArray< Tile, Policy > & | a | ) |
Definition at line 1660 of file dist_array.h.


◆ now()
|
inline |
◆ operator!=() [1/7]
|
inline |
Permutation inequality operator.
- Parameters
-
p1 The left-hand permutation to be compared p2 The right-hand permutation to be compared
- Returns
false
if all elements ofp1
andp2
are equal and the partition sizes match, otherwisetrue
.
Definition at line 806 of file permutation.h.
◆ operator!=() [2/7]
|
inlineconstexpr |
Definition at line 382 of file dense_shape.h.
◆ operator!=() [3/7]
◆ operator!=() [4/7]
◆ operator!=() [5/7]
◆ operator!=() [6/7]
|
inline |
◆ operator!=() [7/7]
|
inline |
Inequality operator.
Definition at line 318 of file tiled_range1.h.

◆ operator&()
|
constexpr |
- Returns
- intersection of
space1
andspace2
Definition at line 43 of file platform.h.
◆ operator*() [1/10]
|
inline |
TiledRange permutation operator.
This function will permute the range. Note: only tiles that are not being used by other objects will be permuted. The owner of those objects are
Definition at line 326 of file tiled_range.h.
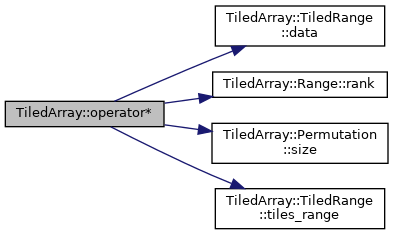
◆ operator*() [2/10]
|
inline |
permute a boost::container::small_vector<T>
- Template Parameters
-
T The element type of the vector N The max static size of the vector
- Parameters
-
perm The permutation v The vector to be permuted
- Returns
- A permuted copy of
v
- Exceptions
-
TiledArray::Exception When the dimension of the permutation is not equal to the size of v
.
Definition at line 563 of file permutation.h.
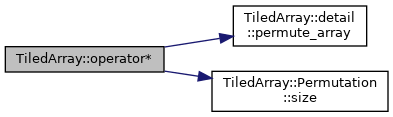
◆ operator*() [3/10]
|
inline |
◆ operator*() [4/10]
|
inline |
Permute a std::array
.
- Template Parameters
-
T The element type of the array N The size of the array
- Parameters
-
perm The permutation a The array to be permuted
- Returns
- A permuted copy of
a
- Exceptions
-
TiledArray::Exception When the dimension of the permutation is not equal to the size of a
.
Definition at line 492 of file permutation.h.
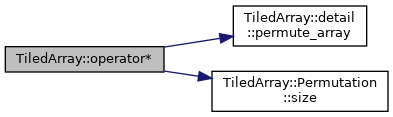
◆ operator*() [5/10]
|
inline |
permute a std::vector<T>
- Template Parameters
-
T The element type of the vector A The allocator type of the vector
- Parameters
-
perm The permutation v The vector to be permuted
- Returns
- A permuted copy of
v
- Exceptions
-
TiledArray::Exception When the dimension of the permutation is not equal to the size of v
.
Definition at line 528 of file permutation.h.
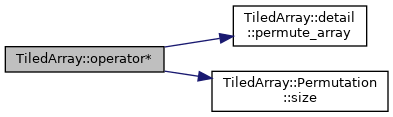
◆ operator*() [6/10]
|
inline |
Create a permuted copy of arg
.
- Template Parameters
-
T The argument tensor type
- Parameters
-
perm The permutation to be applied to arg
arg The argument tensor to be permuted
Definition at line 135 of file operators.h.

◆ operator*() [7/10]
|
inline |
Permute a memory buffer.
- Template Parameters
-
T The element type of the memory buffer
- Parameters
-
perm The permutation ptr A pointer to the memory buffer to be permuted
- Returns
- A permuted copy of the memory buffer as a
std::vector
Definition at line 595 of file permutation.h.

◆ operator*() [8/10]
|
inline |
Create a copy of left
that is scaled by right
.
Scale a tensor
- Template Parameters
-
T The left-hand tensor type N Numeric type
- Parameters
-
left The left-hand tensor argument right The right-hand scalar argument
- Returns
- A tensor where element
i
is equal toleft[i] * right
Definition at line 95 of file operators.h.

◆ operator*() [9/10]
|
inline |
Tensor multiplication operator.
Element-wise multiplication of two tensors
- Template Parameters
-
T1 The left-hand tensor type T2 The right-hand tensor type
- Parameters
-
left The left-hand tensor argument right The right-hand tensor argument
- Returns
- A tensor where element
i
is equal toleft[i] * right[i]
Definition at line 79 of file operators.h.

◆ operator*() [10/10]
|
inline |
Create a copy of right
that is scaled by left
.
- Template Parameters
-
N A numeric type T The right-hand tensor type
- Parameters
-
left The left-hand scalar argument right The right-hand tensor argument
- Returns
- A tensor where element
i
is equal toleft * right[i]
Definition at line 111 of file operators.h.

◆ operator*=() [1/5]
|
inline |
In-place permute a boost::container::small_vector
.
- Template Parameters
-
T The element type of the vector N The max static size of the vector
- Parameters
-
[out] v The vector to be permuted [in] perm The permutation
- Returns
- A reference to
v
- Exceptions
-
TiledArray::Exception When the dimension of the permutation is not equal to the size of v
.
Definition at line 581 of file permutation.h.

◆ operator*=() [2/5]
|
inline |
In-place permute a std::array
.
- Template Parameters
-
T The element type of the array N The size of the array
- Parameters
-
[out] a The array to be permuted [in] perm The permutation
- Returns
- A reference to
a
- Exceptions
-
TiledArray::Exception When the dimension of the permutation is not equal to the size of a
.
Definition at line 510 of file permutation.h.
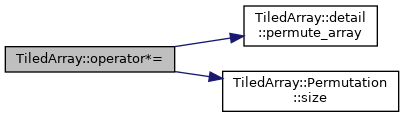
◆ operator*=() [3/5]
|
inline |
In-place permute a std::vector
.
- Template Parameters
-
T The element type of the vector A The allocator type of the vector
- Parameters
-
[out] v The vector to be permuted [in] perm The permutation
- Returns
- A reference to
v
- Exceptions
-
TiledArray::Exception When the dimension of the permutation is not equal to the size of v
.
Definition at line 546 of file permutation.h.

◆ operator*=() [4/5]
|
inline |
In place tensor scale.
Scale the elements of left
by right
- Template Parameters
-
T The left-hand tensor type N Numeric type
- Parameters
-
left The left-hand tensor argument right The right-hand scalar argument
- Returns
- A reference to
left
Definition at line 231 of file operators.h.

◆ operator*=() [5/5]
|
inline |
In place tensor multiplication.
Multiply the elements of left by that of right
- Template Parameters
-
T1 The left-hand tensor type T2 The right-hand tensor type
- Parameters
-
left The left-hand tensor argument right The right-hand tensor argument
- Returns
- A reference to
left
Definition at line 183 of file operators.h.

◆ operator+()
|
inline |
Tensor plus operator.
Add two tensors
- Template Parameters
-
T1 The left-hand tensor type T2 The right-hand tensor type
- Parameters
-
left The left-hand tensor argument right The right-hand tensor argument
- Returns
- A tensor where element
i
is equal toleft[i] + right[i]
Definition at line 47 of file operators.h.

◆ operator+=() [1/2]
|
inline |
In place tensor add constant.
Scale the elements of left
by right
- Template Parameters
-
T The left-hand tensor type N Numeric type
- Parameters
-
left The left-hand tensor argument right The right-hand scalar argument
- Returns
- A reference to
left
Definition at line 199 of file operators.h.

◆ operator+=() [2/2]
|
inline |
Tensor plus operator.
Add the elements of right
to that of left
- Template Parameters
-
T1 The left-hand tensor type T2 The right-hand tensor type
- Parameters
-
left The left-hand tensor argument right The right-hand tensor argument
- Returns
- A tensor where element
i
is equal toleft[i] + right[i]
Definition at line 151 of file operators.h.

◆ operator-() [1/2]
|
inline |
Create a negated copy of arg
.
- Template Parameters
-
T The element type of arg
- Parameters
-
arg The argument tensor
- Returns
- A tensor where element
i
is equal to-arg
[i]
Definition at line 123 of file operators.h.

◆ operator-() [2/2]
|
inline |
Tensor minus operator.
Subtract two tensors
- Template Parameters
-
T1 The left-hand tensor type T2 The right-hand tensor type
- Parameters
-
left The left-hand tensor argument right The right-hand tensor argument
- Returns
- A tensor where element
i
is equal toleft[i] - right[i]
Definition at line 63 of file operators.h.

◆ operator-=() [1/2]
|
inline |
In place tensor subtract constant.
Scale the elements of left
by right
- Template Parameters
-
T The left-hand tensor type N Numeric type
- Parameters
-
left The left-hand tensor argument right The right-hand scalar argument
- Returns
- A reference to
left
Definition at line 215 of file operators.h.

◆ operator-=() [2/2]
|
inline |
Tensor minus operator.
Subtract the elements of right
from that of left
- Template Parameters
-
T1 The left-hand tensor type T2 The right-hand tensor type
- Parameters
-
left The left-hand tensor argument right The right-hand tensor argument
- Returns
- A reference to
left
Definition at line 167 of file operators.h.
◆ operator<<() [1/7]
|
inline |
Add the tensor to an output stream.
This function will iterate through all tiles on node 0 and print non-zero tiles. It will wait for each tile to be evaluated (i.e. it is a blocking function). Tasks will continue to be processed.
- Parameters
-
os The output stream a The array to be put in the output stream
- Returns
- A reference to the output stream
Definition at line 1602 of file dist_array.h.
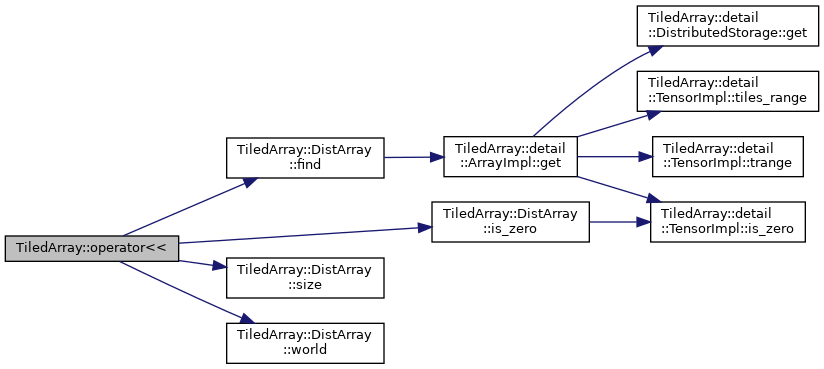
◆ operator<<() [2/7]
|
inline |
◆ operator<<() [3/7]
|
inline |
Add the shape to an output stream.
- Template Parameters
-
T the numeric type supporting the type of shape
- Parameters
-
os The output stream shape the SparseShape<T> object
- Returns
- A reference to the output stream
Definition at line 1609 of file sparse_shape.h.

◆ operator<<() [4/7]
|
inline |
◆ operator<<() [5/7]
|
inline |
◆ operator<<() [6/7]
|
inline |
◆ operator<<() [7/7]
|
inline |
TiledRange1 ostream operator.
Definition at line 323 of file tiled_range1.h.
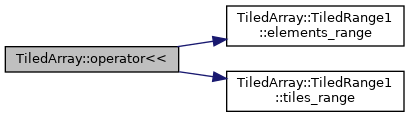
◆ operator==() [1/8]
|
inline |
Permutation equality operator.
- Parameters
-
p1 The left-hand permutation to be compared p2 The right-hand permutation to be compared
- Returns
true
if all elements ofp1
andp2
are equal and the partition sizes match, otherwisefalse
.
Definition at line 795 of file permutation.h.

◆ operator==() [2/8]
|
inline |
BlockRange equality comparison.
- Parameters
-
r1 The first range to be compared r2 The second range to be compared
- Returns
true
whenr1
represents the same range asr2
, otherwisefalse
.
Definition at line 433 of file block_range.h.

◆ operator==() [3/8]
|
inlineconstexpr |
Definition at line 378 of file dense_shape.h.
◆ operator==() [4/8]
◆ operator==() [5/8]
◆ operator==() [6/8]
◆ operator==() [7/8]
|
inline |
Returns true when all tile and element ranges are the same.
Definition at line 337 of file tiled_range.h.
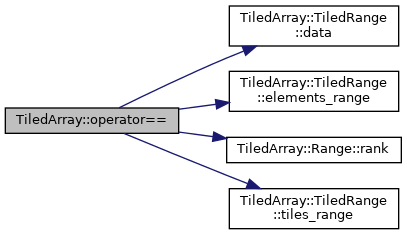
◆ operator==() [8/8]
|
inline |
Equality operator.
Definition at line 311 of file tiled_range1.h.
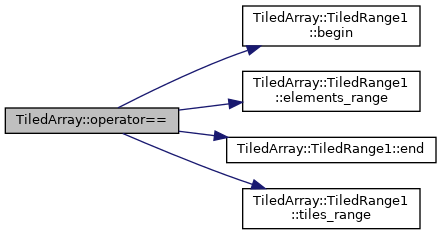
◆ operator|()
|
constexpr |
- Returns
- union of
space1
andspace2
Definition at line 48 of file platform.h.
◆ outer() [1/2]
|
inline |
◆ outer() [2/2]
|
inline |
◆ outer_size() [1/2]
|
inline |
◆ outer_size() [2/2]
|
inline |
◆ overlap()
|
constexpr |
- Returns
- true if intersection of
space1
andspace2
is nonnull
Definition at line 53 of file platform.h.

◆ permute() [1/3]
|
inline |
◆ permute() [2/3]
◆ permute() [3/3]
std::enable_if<!TiledArray::detail::is_permutation_v<Perm>, TiledArray::Range>::type TiledArray::permute | ( | const TiledArray::Range & | r, |
const Perm & | p | ||
) |
permute function for TiledArray::Range class with non-TiledArray Permutation object
Definition at line 803 of file btas.h.
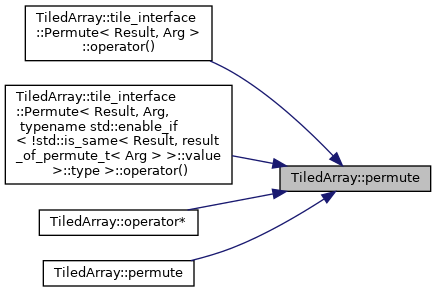
◆ product_reduce_cuda_kernel_impl()
T TiledArray::product_reduce_cuda_kernel_impl | ( | const T * | arg, |
std::size_t | n, | ||
cudaStream_t | stream, | ||
int | device_id | ||
) |
◆ push_default_world()
|
inline |
Sets the default World to world
and returns a smart pointer to the current default World. Releasing this pointer will automatically set the default World to the World that it points. Thus use this as follows:
- Parameters
-
world a World object which will become the new default
- Returns
- a smart pointer to the current default World (i.e. not
world
) whose deleter will reset the default World back to the stored value
Definition at line 117 of file madness.h.

◆ rank()
auto TiledArray::rank | ( | const DistArray< Tile, Policy > & | a | ) |
Definition at line 1617 of file dist_array.h.

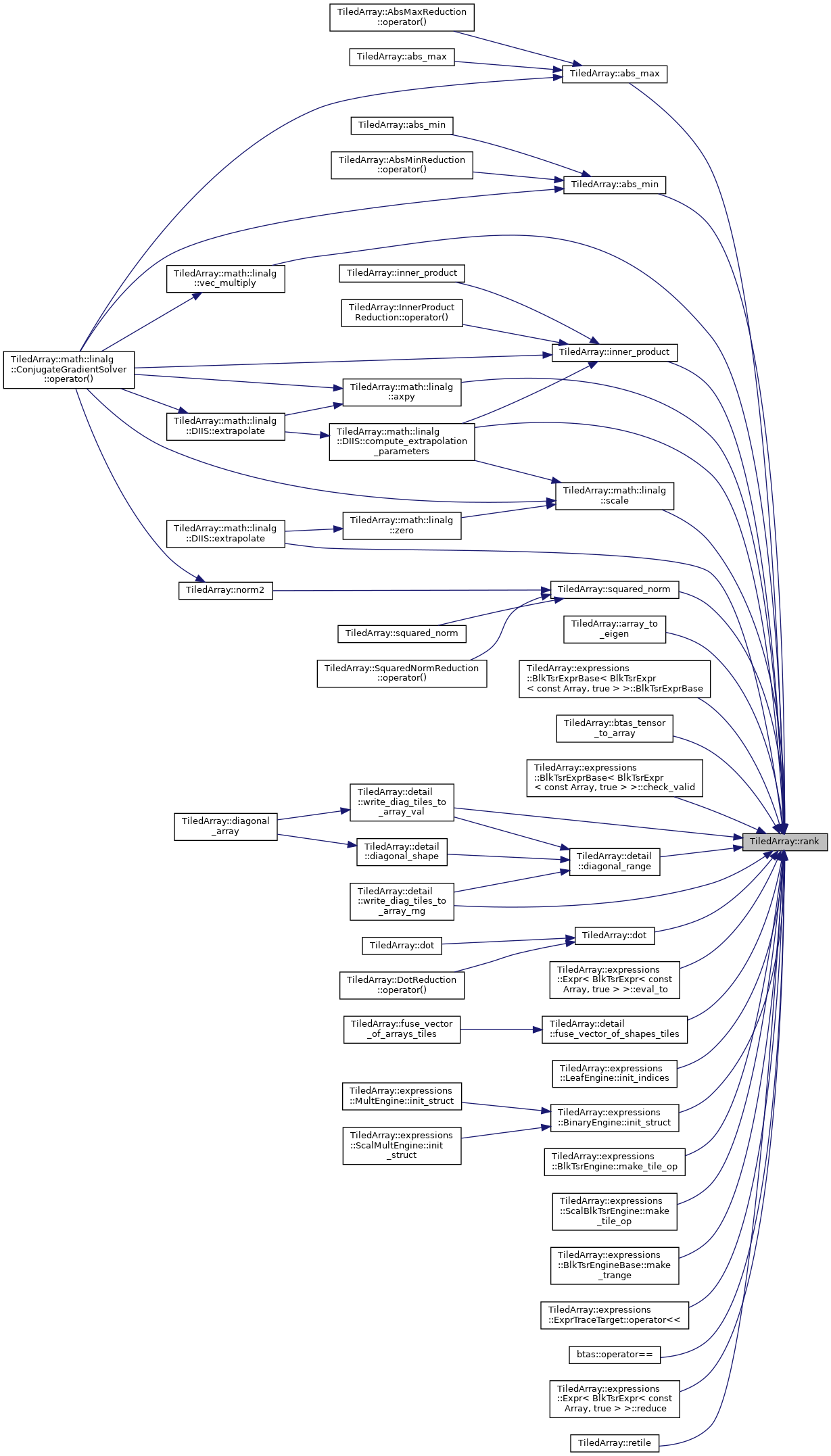
◆ reduce_cuda_kernel_impl()
T TiledArray::reduce_cuda_kernel_impl | ( | ReduceOp && | op, |
const T * | arg, | ||
std::size_t | n, | ||
T | init, | ||
cudaStream_t | stream, | ||
int | device_id | ||
) |
T = reduce(T* arg)
Definition at line 51 of file reduce_kernel_impl.h.
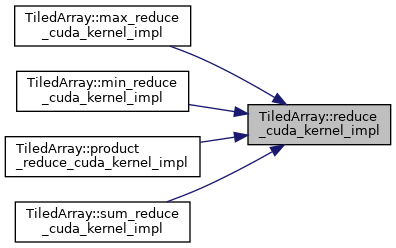
◆ remap() [1/8]
void TiledArray::remap | ( | detail::TensorInterface< const T, Range_, OpResult > & | , |
T * const | , | ||
const Index1 & | , | ||
const Index2 & | |||
) |
◆ remap() [2/8]
void TiledArray::remap | ( | detail::TensorInterface< const T, Range_, OpResult > & | , |
T * const | , | ||
const std::initializer_list< Index1 > & | , | ||
const std::initializer_list< Index2 > & | |||
) |
◆ remap() [3/8]
|
inline |
Definition at line 154 of file tensor_map.h.
◆ remap() [4/8]
|
inline |
Definition at line 172 of file tensor_map.h.
◆ remap() [5/8]
|
inline |
Definition at line 154 of file tensor_map.h.
◆ remap() [6/8]
|
inline |
Definition at line 172 of file tensor_map.h.
◆ remap() [7/8]
|
inline |
For reusing map without allocating new ranges . . . maybe.
Definition at line 145 of file tensor_map.h.
◆ remap() [8/8]
|
inline |
Definition at line 162 of file tensor_map.h.
◆ reset_default_world()
|
inline |
◆ retile()
auto TiledArray::retile | ( | const DistArray< TileType, PolicyType > & | tensor, |
const TiledRange & | new_trange | ||
) |
◆ row_major_buffer_to_array()
|
inline |
Convert a row-major matrix buffer into an Array object.
This function will copy the content of buffer
into an Array
object that is tiled according to the trange
object. The copy operation is done in parallel, and this function will block until all elements of matrix
have been copied into the result array tiles. Each tile is created using the local contents of matrix
, hence it is your responsibility to ensure that the data in matrix
is distributed correctly among the ranks. If in doubt, you should replicate matrix
among the ranks prior to calling this.
Usage:
- Template Parameters
-
A The array type
- Parameters
-
world The world where the array will live trange The tiled range of the new array buffer The row-major matrix buffer to be copied m The number of rows in the matrix n The number of columns in the matrix replicated if true, the result will be replicated [default = true]. pmap the process map object [default=null]; initialized to the default if replicated
is false, or a replicated pmap ifreplicated
is true; ignored ifreplicated
is true andworld.size()>1
- Returns
- An
Array
object that is a copy ofmatrix
- Exceptions
-
TiledArray::Exception When m
andn
are not equal to the number of rows or columns in tiled range.
◆ scale() [1/2]
|
inline |
Scalar the tile argument.
- Template Parameters
-
Arg A tile type Scalar A numeric type (i.e. TiledArray::detail::is_numeric_v<Scalar> is true)
- Parameters
-
arg The left-hand argument to be scaled factor The scaling factor
- Returns
- A tile that is equal to
arg * factor
◆ scale() [2/2]
|
inline |
Scale and permute tile argument.
- Template Parameters
-
Arg The tile argument type Scalar A scalar type
- Parameters
-
arg The left-hand argument to be scaled factor The scaling factor perm The permutation to be applied to the result
- Returns
- A tile that is equal to
perm ^ (arg * factor)
◆ scale_to()
|
inline |
◆ set_default_world()
|
inline |
Sets the default World to world
.
Expressions that follow this call will use world
as the default execution context (the use of default World can be overridden by explicit mechanisms for specifying the execution context, like Expr::set_world() or assigning an expression to a DistArray that has already been initialized with a World).
- Note
- set_default_world() and get_default_world() are only useful if 1 thread (usually, the main thread ) creates TiledArray expressions.
- Parameters
-
world a World object which will become the new default
Definition at line 85 of file madness.h.


◆ shift() [1/4]
|
inline |
◆ shift() [2/4]
|
inline |
◆ shift() [3/4]
detail::ShiftWrapper<const T> TiledArray::shift | ( | const T & | tensor | ) |
Shift a tensor from one range to another.
- Template Parameters
-
T A tensor type
- Parameters
-
tensor The tensor object to shift
- Returns
- A shifted tensor object
Definition at line 146 of file shift_wrapper.h.
◆ shift() [4/4]
detail::ShiftWrapper<T> TiledArray::shift | ( | T & | tensor | ) |
Shift a tensor from one range to another.
- Template Parameters
-
T A tensor type
- Parameters
-
tensor The tensor object to shift
- Returns
- A shifted tensor object
Definition at line 136 of file shift_wrapper.h.
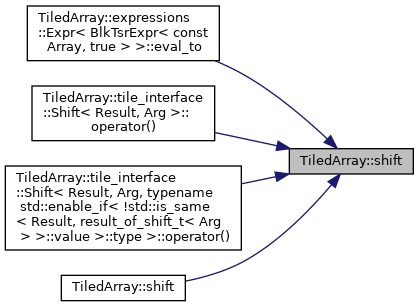
◆ shift_to() [1/2]
|
inline |
◆ shift_to() [2/2]
|
inline |
◆ squared_norm()
auto TiledArray::squared_norm | ( | const DistArray< Tile, Policy > & | a | ) |
Definition at line 1655 of file dist_array.h.

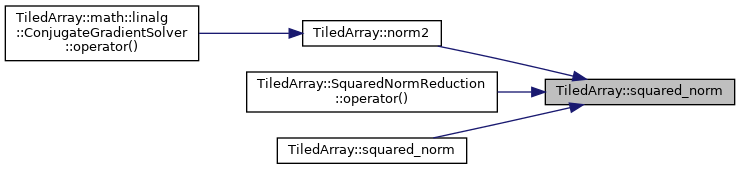
◆ subarray_from_fused_array()
void TiledArray::subarray_from_fused_array | ( | madness::World & | local_world, |
const TA::DistArray< Tile, Policy > & | fused_array, | ||
std::size_t | tile_idx, | ||
std::vector< TA::DistArray< Tile, Policy >> & | split_arrays, | ||
const TA::TiledRange & | split_trange | ||
) |
extracts a subarray of a fused array created with fuse_vector_of_arrays and creates the array in local_world
.
- Parameters
-
[in] local_world The World object where the @i -th subarray is created [in] fused_array a DistArray created with fuse_vector_of_arrays [in] i the index of the subarray to be extracted (i.e. the index of the corresponding element index of the leading dimension) [in] tile_of_i tile range information for tile i [in] split_trange TiledRange of the split Array object
- Returns
- the
i
-th subarray
- See also
- detail::subshape_from_fused_tile TODO rename to split_tilewise_fused_array
copy the data from tile
write to blocks of fused_array
Definition at line 393 of file vector_of_arrays.h.
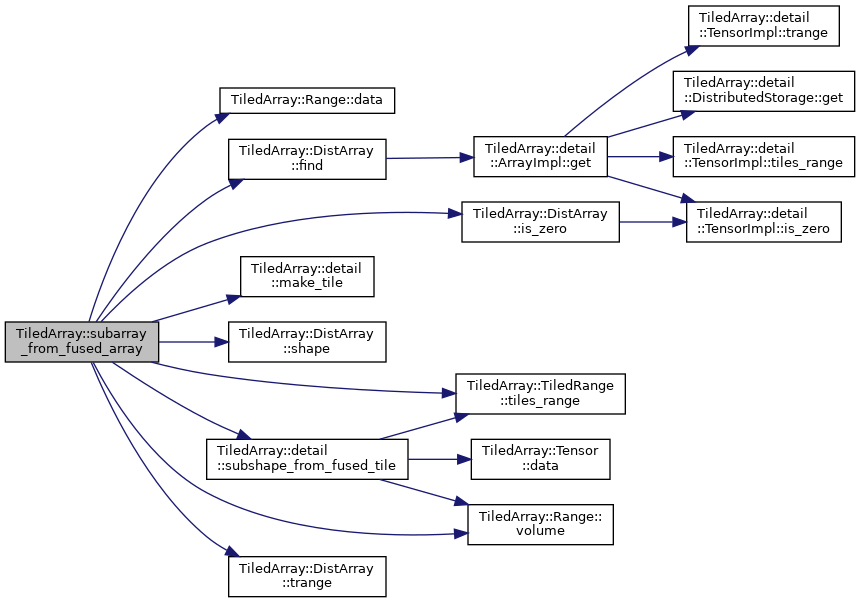
◆ sum_reduce_cuda_kernel_impl()
T TiledArray::sum_reduce_cuda_kernel_impl | ( | const T * | arg, |
std::size_t | n, | ||
cudaStream_t | stream, | ||
int | device_id | ||
) |
◆ swap() [1/4]
|
constexprnoexcept |
Swaps the referred callables of lhs
and rhs
.
Definition at line 120 of file function.h.

◆ swap() [2/4]
◆ swap() [3/4]
|
inline |
Exchange the content of the two given TiledRange's.
Definition at line 334 of file tiled_range.h.


◆ swap() [4/4]
|
inline |
Exchange the data of the two given ranges.
Definition at line 306 of file tiled_range1.h.


◆ system_now()
|
inline |
◆ ta_abort() [1/2]
void TiledArray::ta_abort | ( | ) |
Finalizes TiledArray (and MADWorld runtime, if it had not been initialized when TiledArray::initialize was called).
Definition at line 136 of file tiledarray.cpp.
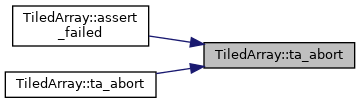
◆ ta_abort() [2/2]
void TiledArray::ta_abort | ( | const std::string & | m | ) |
Finalizes TiledArray (and MADWorld runtime, if it had not been initialized when TiledArray::initialize was called).
Definition at line 140 of file tiledarray.cpp.

◆ tensor_to_btas_subtensor()
|
inline |
Copy a block of a btas::Tensor into a TiledArray::Tensor.
TiledArray::Tensor src
will be copied into a block of btas::Tensor dst
. The block dimensions will be determined by the dimensions of the range of src
.
- Template Parameters
-
Tensor_ A tensor type (e.g., TiledArray::Tensor or btas::Tensor, optionally wrapped into TiledArray::Tile) T The tensor element type Range_ The range type of the destination btas::Tensor object Storage_ The storage type of the destination btas::Tensor object
- Parameters
-
[in] src The source object whose contents will be copied into a subblock of dst
[out] dst The destination object; its subblock defined by the {lower,upper} bounds {src.lobound()
,src.upbound()} will be overwritten with the content ofsrc
- Exceptions
-
TiledArray::Exception When the dimensions of src
anddst
do not match.
Definition at line 96 of file btas.h.
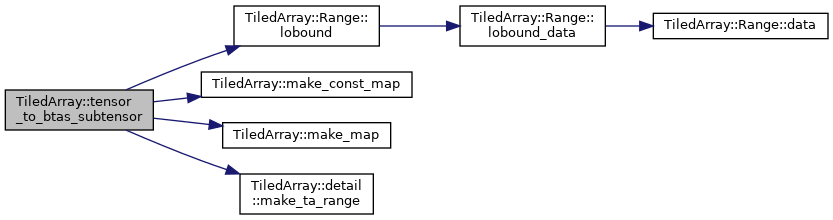

◆ tensor_to_eigen_submatrix()
|
inline |
Copy the content of a tensor into an Eigen matrix block.
The content of tensor will be copied into a block of matrix. The block dimensions will be determined by the dimensions of the tensor's range.
- Template Parameters
-
T A tensor type, e.g. TiledArray::Tensor Derived The derived type of an Eigen matrix
- Parameters
-
[in] tensor The object that will be copied to matrix
[out] matrix The object that will be assigned the content of tensor
- Exceptions
-
TiledArray::Exception When the dimensions of tensor
are not equal to 1 or 2.TiledArray::Exception When the range of tensor
is outside the range ofmatrix
.
Definition at line 274 of file eigen.h.


◆ tiled_range_from_il()
auto TiledArray::tiled_range_from_il | ( | T && | il, |
U | shape = {} |
||
) |
Creates a TiledRange for the provided initializer list.
Tensors which are constructed with initializer lists are assumed to be small enough that the data should reside in a single tile. This function will recurse through il
and create a TiledRange instance such that each rank is tiled from [0, n_i)
where n_i
is the length of the i
-th nested std::initializer_list
in il
.
- Template Parameters
-
T Expected to be the type of a tensor element (i.e. float, double, etc.) or a (possibly nested) std::initializer_list
of tensor elements.U The type of the container which will hold the TiledRange1 instances for each level of nesting in T
.U
must satisfy the concept of a random-access container.U
defaults tostd::array<TiledRange1, N>
whereN
is the degree of nesting ofil
.
- Parameters
-
[in] il The state we intend to initialize the tensor to. [in] shape A pre-allocated buffer that will be used to hold the TiledRange1 instances for each std::initializer_list
as this function recurses. The default value is anstd::array
of default constructed TiledRange1 instances, which should suffice for most purposes.
- Returns
- A TiledRange instance consistent with treating
il
as a tensor with a single tile.
- Exceptions
-
TiledArray::Exception if il
contains no elements. If an exception is raised this wayil
andshape
are guaranteed to be in the same state (strong throw guarantee).
Definition at line 131 of file initializer_list.h.

◆ to_dense() [1/2]
|
inline |
◆ to_dense() [2/2]
|
inline |
Definition at line 65 of file sparse_to_dense.h.
◆ to_execution_space()
void TiledArray::to_execution_space | ( | cpu_cuda_vector< T, HostAlloc, DeviceAlloc > & | vec, |
cudaStream_t | stream = 0 |
||
) |
◆ to_new_tile_type()
|
inline |
Function to convert an array to a new array with a different tile type.
- Template Parameters
-
Tile The array tile type ConvTile The tile type to which Tile can be converted to and for which Op(ConvTile)
is well-formedPolicy The array policy type Op The tile conversion operation type
- Parameters
-
array The array to be converted op The tile type conversion operation
Definition at line 71 of file to_new_tile_type.h.
◆ to_sparse() [1/2]
std::enable_if_t<!is_dense_v<ResultPolicy> && is_dense_v<ArgPolicy>, DistArray<Tile, ResultPolicy> > TiledArray::to_sparse | ( | DistArray< Tile, ArgPolicy > const & | dense_array | ) |
Function to convert a dense array into a block sparse array.
If the input array is dense then create a copy by checking the norms of the tiles in the dense array and then cloning the significant tiles into the sparse array.
Definition at line 17 of file dense_to_sparse.h.
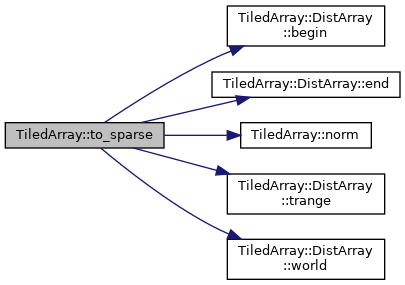
◆ to_sparse() [2/2]
std::enable_if_t<!is_dense_v<Policy>, DistArray<Tile, Policy> > TiledArray::to_sparse | ( | DistArray< Tile, Policy > const & | sparse_array | ) |
If the array is already sparse return a copy of the array.
Definition at line 54 of file dense_to_sparse.h.
◆ trace()
decltype(auto) TiledArray::trace | ( | const T & | t | ) |
Helper function for taking the trace of a tensor
This function works by creating a detail::Trace instance for the given tile type, T
. The implementation of the trace operation can be controlled by specializing Trace<T>. It simply returns the result of Trace<T>::operator()(const T&)const verbatim.
This function only participates in overload resolution if detail::trace_is_defined_v<T> is true.
- Template Parameters
-
T The type of tensor we are trying to take the trace of. <anonymous> Template type parameter used for SFINAE. Defaults to void when we know how to take the trace of a tensor type
- Parameters
-
[in] t The tensor instance we want the trace of.
- Returns
- The results of calling Trace<T>::operator() on
t
.
- Exceptions
-
??? Any exceptions thrown by Trace<T>::operator() will also be thrown by this function with the same throw guarantee.
◆ truncate() [1/2]
|
inline |
Truncate a sparse Array.
- Template Parameters
-
Tile The tile type of array
Policy The policy type of array
- Parameters
-
[in,out] array The array object to be truncated
Definition at line 56 of file truncate.h.
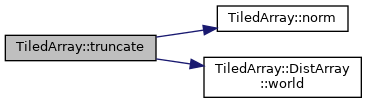
◆ truncate() [2/2]
|
inline |
Truncate a dense Array.
This is a no-op
- Template Parameters
-
Tile The tile type of array
Policy The policy type of array
- Parameters
-
[in,out] array The array object to be truncated
Definition at line 46 of file truncate.h.
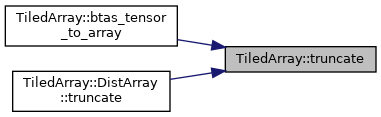
◆ volume()
size_t TiledArray::volume | ( | const DistArray< Tile, Policy > & | a | ) |
Definition at line 1622 of file dist_array.h.
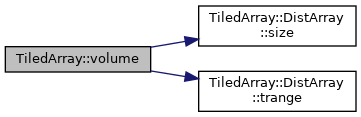
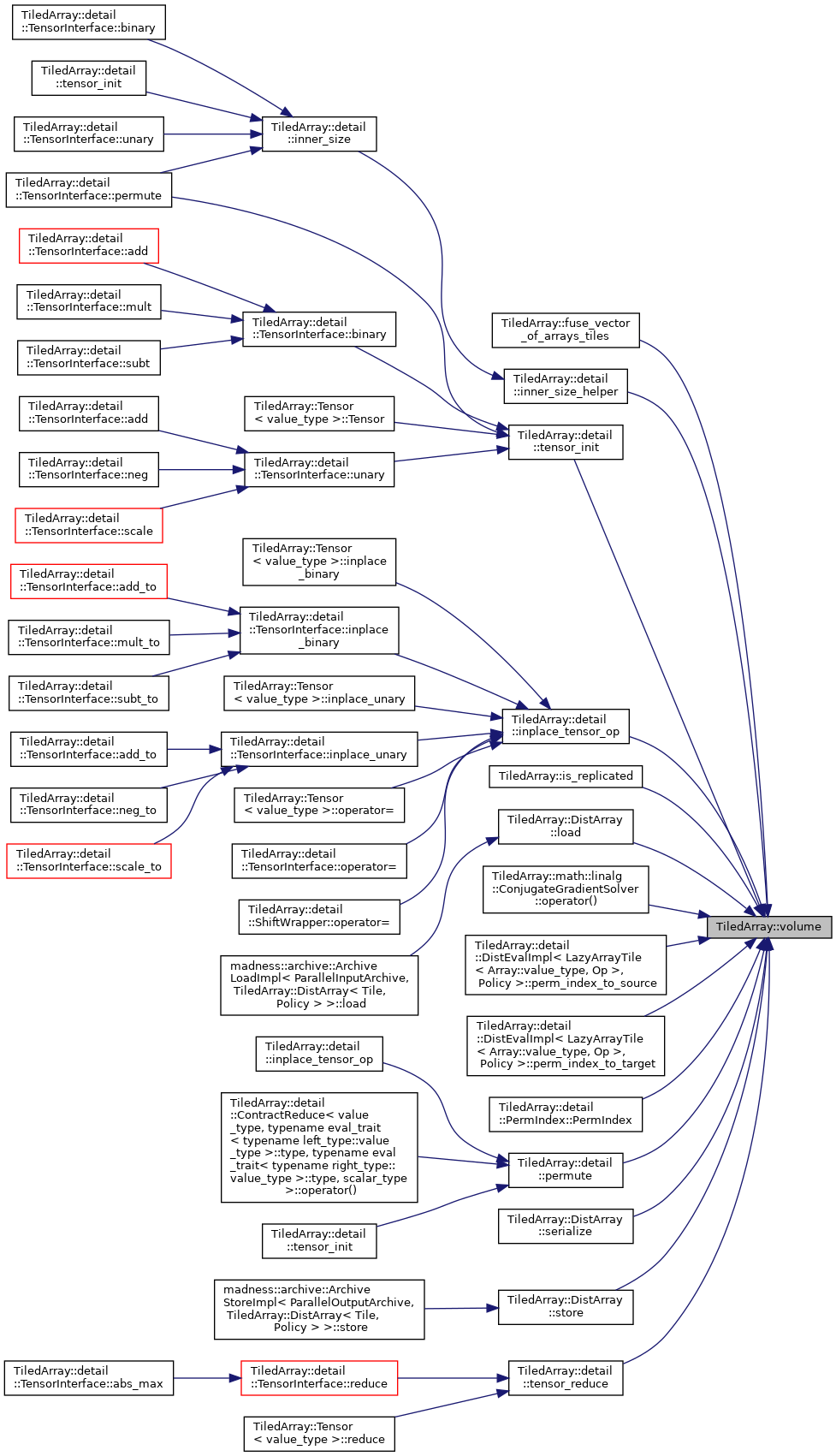
Variable Documentation
◆ initializer_list_rank_v
|
constexpr |
Helper variable for retrieving the degree of nesting for an std::initializer_list.
This helper variable creates a global variable which contains the value of InitializerListRank<T, SizeType> and is intended to be used as a (more) convenient means of retrieving the value.
- Template Parameters
-
T The type we are analyzing for its std::initializer_list-nested-ness SizeType the type to use for the value
member. Defaults tostd::size_t
.
Definition at line 71 of file initializer_list.h.