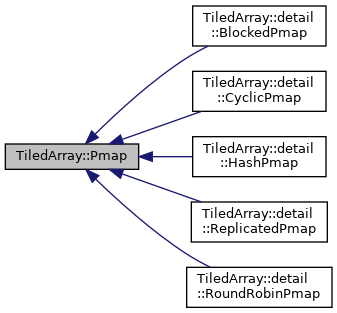
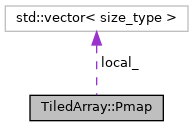
Documentation
Process map.
This is the base and interface class for other process maps. It provides access to the local tile iterator and basic process map information. Derived classes are responsible for distribution of tiles. The general idea of process map objects is to compute process owners with an O(1) algorithm to provide fast access to tile owner information and avoid storage of process map. A cached list of local tiles can be stored so that algorithms that need to iterate over local tiles can do so without computing the owner of all tiles. The algorithm to generate the cached list of local tiles and the memory requirement should scale as O(tiles/processes), if possible.
Classes | |
class | Iterator |
Pmap iterator type. More... | |
Public Types | |
typedef std::size_t | size_type |
Size type. More... | |
Public Member Functions | |
Pmap (World &world, const size_type size) | |
Process map constructor. More... | |
virtual | ~Pmap () |
virtual size_type | owner (const size_type tile) const =0 |
Maps tile to the processor that owns it. More... | |
virtual bool | is_local (const size_type tile) const =0 |
Check that the tile is owned by this process. More... | |
size_type | size () const |
Size accessor. More... | |
size_type | rank () const |
Process rank accessor. More... | |
size_type | procs () const |
Process count accessor. More... | |
virtual bool | known_local_size () const |
Queries whether local size is known. More... | |
size_type | local_size () const |
Local size accessor. More... | |
bool | empty () const |
Check if there are any local elements. More... | |
virtual bool | is_replicated () const |
Replicated array status. More... | |
Protected Attributes | |
const size_type | rank_ |
The rank of this process. More... | |
const size_type | procs_ |
The number of processes. More... | |
const size_type | size_ |
The number of tiles mapped among all processes. More... | |
std::vector< size_type > | local_ |
A list of local tiles (may be empty, if not needed) More... | |
size_type | local_size_ |
Iteration | |
typedef Iterator | const_iterator |
Iterator type. More... | |
class | Iterator |
Iterator type. More... | |
virtual const_iterator | begin () const |
Begin local element iterator. More... | |
virtual const_iterator | end () const |
End local element iterator. More... | |
const const_iterator | cbegin () const |
Begin local element iterator. More... | |
const const_iterator | cend () const |
End local element iterator. More... | |
Member Typedef Documentation
◆ const_iterator
◆ size_type
typedef std::size_t TiledArray::Pmap::size_type |
Constructor & Destructor Documentation
◆ Pmap()
|
inline |
◆ ~Pmap()
Member Function Documentation
◆ begin()
|
inlinevirtual |
Begin local element iterator.
- Returns
- An iterator that points to the beginning of the local element set
Reimplemented in TiledArray::detail::ReplicatedPmap, TiledArray::detail::CyclicPmap, and TiledArray::detail::BlockedPmap.
Definition at line 287 of file pmap.h.

◆ cbegin()
|
inline |
◆ cend()
|
inline |
◆ empty()
|
inline |
Check if there are any local elements.
- Returns
true
when there are no local tiles, otherwisefalse
.
- Warning
- if
size()>0
asserts thatknown_local_size()==true
Definition at line 133 of file pmap.h.

◆ end()
|
inlinevirtual |
End local element iterator.
- Returns
- An iterator that points to the beginning of the local element set
Reimplemented in TiledArray::detail::ReplicatedPmap, TiledArray::detail::CyclicPmap, and TiledArray::detail::BlockedPmap.
Definition at line 295 of file pmap.h.

◆ is_local()
|
pure virtual |
Check that the tile is owned by this process.
- Parameters
-
tile The tile to be checked
- Returns
true
iftile
is owned by this process, otherwisefalse
.
Implemented in TiledArray::detail::RoundRobinPmap, TiledArray::detail::ReplicatedPmap, TiledArray::detail::HashPmap, TiledArray::detail::CyclicPmap, and TiledArray::detail::BlockedPmap.
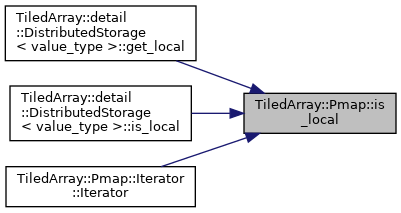
◆ is_replicated()
|
inlinevirtual |
Replicated array status.
- Returns
true
if the array is replicated, and false otherwise
Reimplemented in TiledArray::detail::ReplicatedPmap.
◆ known_local_size()
|
inlinevirtual |
Queries whether local size is known.
- Returns
- true if the number of local elements is known
- Note
- Override if it is too expensive to precompute
Reimplemented in TiledArray::detail::HashPmap.
Definition at line 118 of file pmap.h.

◆ local_size()
|
inline |
Local size accessor.
- Returns
- The number of local elements
- Warning
- if
size()>0
asserts thatknown_local_size()==true
Definition at line 124 of file pmap.h.

◆ owner()
Maps tile
to the processor that owns it.
- Parameters
-
tile The tile to be queried
- Returns
- Processor that logically owns
tile
Implemented in TiledArray::detail::RoundRobinPmap, TiledArray::detail::ReplicatedPmap, TiledArray::detail::HashPmap, TiledArray::detail::CyclicPmap, and TiledArray::detail::BlockedPmap.

◆ procs()
|
inline |
◆ rank()
|
inline |
◆ size()
|
inline |
Friends And Related Function Documentation
◆ Iterator
Member Data Documentation
◆ local_
|
protected |
◆ local_size_
|
protected |
◆ procs_
|
protected |
◆ rank_
|
protected |
◆ size_
|
protected |
The documentation for this class was generated from the following file:
- TiledArray/pmap/pmap.h