List of all members |
Classes |
Static Public Member Functions |
Protected Member Functions |
Static Protected Member Functions
TiledArray::Singleton< Derived > Class Template Reference
Documentation
template<typename Derived>
class TiledArray::Singleton< Derived >
Singleton base class To create a singleton class A
do:
Here's how to use it:
A::set_instance(Args...); // this creates the first instance of A, if A is
not default-constructible, otherwise can skip this A& the_instance_ref =
A::get_instance(); // throws if the instance of A had not been created
A* the_instance_ptr = A::get_instance_ptr(); // returns nullptr if the
instance of A had not been created
// the instance of A will be destroyed with other static-linkage objects
Definition at line 55 of file singleton.h.
Static Public Member Functions | |
template<typename D = Derived> | |
static std::enable_if_t< Singleton< D >::derived_is_default_constructible, D & > | get_instance () |
template<typename D = Derived> | |
static D & | get_instance (std::enable_if_t<!Singleton< Derived >::derived_is_default_constructible > *=nullptr) |
template<typename D = Derived> | |
static std::enable_if_t< Singleton< D >::derived_is_default_constructible, D * > | get_instance_ptr () |
template<typename D = Derived> | |
static D * | get_instance_ptr (std::enable_if_t<!Singleton< Derived >::derived_is_default_constructible > *=nullptr) |
template<typename... Args> | |
static void | set_instance (Args &&... args) |
Protected Member Functions | |
template<typename... Args> | |
Singleton (Args &&... args) | |
Static Protected Member Functions | |
static auto & | instance_accessor () |
Constructor & Destructor Documentation
◆ Singleton()
template<typename Derived >
template<typename... Args>
|
inlineprotected |
Definition at line 128 of file singleton.h.
Member Function Documentation
◆ get_instance() [1/2]
template<typename Derived >
template<typename D = Derived>
|
inlinestatic |
- Returns
- reference to the instance
Definition at line 70 of file singleton.h.
◆ get_instance() [2/2]
template<typename Derived >
template<typename D = Derived>
|
inlinestatic |
- Returns
- reference to the instance
- Exceptions
-
std::logic_error if the reference has not been contructed (because Derived is not default-constructible and set_instance() had not been called)
Definition at line 82 of file singleton.h.
◆ get_instance_ptr() [1/2]
template<typename Derived >
template<typename D = Derived>
|
inlinestatic |
- Returns
- pointer to the instance, or nullptr if it has not yet been constructed
Definition at line 97 of file singleton.h.
◆ get_instance_ptr() [2/2]
template<typename Derived >
template<typename D = Derived>
|
inlinestatic |
- Returns
- pointer to the instance, or nullptr if it has not yet been constructed
Definition at line 107 of file singleton.h.
◆ instance_accessor()
template<typename Derived >
|
inlinestaticprotected |
◆ set_instance()
template<typename Derived >
template<typename... Args>
|
inlinestatic |
Constructs the instance. This must be called if Derived is not default-constructible.
- Template Parameters
-
Args a parameter pack type
- Parameters
-
args a parameter pack
Definition at line 120 of file singleton.h.
Here is the caller graph for this function:
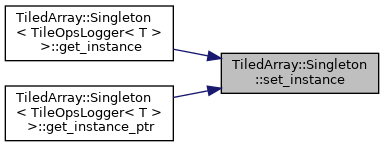
The documentation for this class was generated from the following file:
- TiledArray/util/singleton.h