Documentation
template<typename T, typename HostAlloc = std::allocator<T>, typename DeviceAlloc = thrust::device_allocator<T>>
class TiledArray::cpu_cuda_vector< T, HostAlloc, DeviceAlloc >
a vector that lives on either host or device side, or both
- Template Parameters
-
T the type of values this vector holds HostAlloc The allocator type used for host data DeviceAlloc The allocator type used for device data
Definition at line 19 of file cpu_cuda_vector.h.
Public Types | |
enum | state { state::none = 0x00, state::host = 0x01, state::device = 0x10, state::all = 0x11 } |
typedef T | value_type |
typedef T & | reference |
typedef const T & | const_reference |
typedef thrust::host_vector< T, HostAlloc >::size_type | size_type |
typedef thrust::host_vector< T, HostAlloc >::difference_type | difference_type |
typedef thrust::host_vector< T, HostAlloc >::iterator | iterator |
typedef thrust::host_vector< T, HostAlloc >::const_iterator | const_iterator |
Public Member Functions | |
cpu_cuda_vector () | |
creates an empty vector More... | |
cpu_cuda_vector (size_type size, state st=state::host) | |
cpu_cuda_vector (size_type size, T value, state st=state::host) | |
template<typename RandomAccessIterator > | |
cpu_cuda_vector (RandomAccessIterator begin, RandomAccessIterator end) | |
size_type | size () const |
void | resize (size_type new_size) |
void | to_device () const |
moves the data from the host to the device (even if it's there) More... | |
void | to_host () const |
moves the data from the device to the host (even if it's there) More... | |
const T * | host_data () const |
T * | host_data () |
const T * | device_data () const |
T * | device_data () |
const T * | data () const |
T * | data () |
iterator | begin () |
const_iterator | begin () const |
const_iterator | cbegin () const |
iterator | end () |
const_iterator | end () const |
const_iterator | cend () const |
const_reference | operator[] (std::size_t i) const |
reference | operator[] (std::size_t i) |
bool | on_host () const |
bool | on_device () const |
Member Typedef Documentation
◆ const_iterator
typedef thrust::host_vector<T, HostAlloc>::const_iterator TiledArray::cpu_cuda_vector< T, HostAlloc, DeviceAlloc >::const_iterator |
Definition at line 29 of file cpu_cuda_vector.h.
◆ const_reference
typedef const T& TiledArray::cpu_cuda_vector< T, HostAlloc, DeviceAlloc >::const_reference |
Definition at line 23 of file cpu_cuda_vector.h.
◆ difference_type
typedef thrust::host_vector<T, HostAlloc>::difference_type TiledArray::cpu_cuda_vector< T, HostAlloc, DeviceAlloc >::difference_type |
Definition at line 26 of file cpu_cuda_vector.h.
◆ iterator
typedef thrust::host_vector<T, HostAlloc>::iterator TiledArray::cpu_cuda_vector< T, HostAlloc, DeviceAlloc >::iterator |
Definition at line 27 of file cpu_cuda_vector.h.
◆ reference
typedef T& TiledArray::cpu_cuda_vector< T, HostAlloc, DeviceAlloc >::reference |
Definition at line 22 of file cpu_cuda_vector.h.
◆ size_type
typedef thrust::host_vector<T, HostAlloc>::size_type TiledArray::cpu_cuda_vector< T, HostAlloc, DeviceAlloc >::size_type |
Definition at line 24 of file cpu_cuda_vector.h.
◆ value_type
typedef T TiledArray::cpu_cuda_vector< T, HostAlloc, DeviceAlloc >::value_type |
Definition at line 21 of file cpu_cuda_vector.h.
Member Enumeration Documentation
◆ state
|
strong |
Enumerator | |
---|---|
none | |
host | |
device | |
all |
Definition at line 31 of file cpu_cuda_vector.h.
Constructor & Destructor Documentation
◆ cpu_cuda_vector() [1/4]
|
inline |
creates an empty vector
Definition at line 34 of file cpu_cuda_vector.h.
◆ cpu_cuda_vector() [2/4]
|
inline |
creates a vector with size
elements
- Parameters
-
st the target state of the vector
Definition at line 37 of file cpu_cuda_vector.h.

◆ cpu_cuda_vector() [3/4]
|
inline |
creates a vector with size
elements filled with value
- Parameters
-
value the value used to fill the vector st the target state of the vector
Definition at line 47 of file cpu_cuda_vector.h.
◆ cpu_cuda_vector() [4/4]
|
inline |
initializes on host side from an iterator range
- Template Parameters
-
RandomAccessIterator
Definition at line 58 of file cpu_cuda_vector.h.
Member Function Documentation
◆ begin() [1/2]
|
inline |
◆ begin() [2/2]
|
inline |
◆ cbegin()
|
inline |
◆ cend()
|
inline |
◆ data() [1/2]
|
inline |
◆ data() [2/2]
|
inline |
◆ device_data() [1/2]
|
inline |
◆ device_data() [2/2]
|
inline |
Definition at line 96 of file cpu_cuda_vector.h.


◆ end() [1/2]
|
inline |
◆ end() [2/2]
|
inline |
◆ host_data() [1/2]
|
inline |
◆ host_data() [2/2]
|
inline |
Definition at line 87 of file cpu_cuda_vector.h.


◆ on_device()
|
inline |
◆ on_host()
|
inline |
◆ operator[]() [1/2]
|
inline |
◆ operator[]() [2/2]
|
inline |
◆ resize()
|
inline |
Definition at line 66 of file cpu_cuda_vector.h.
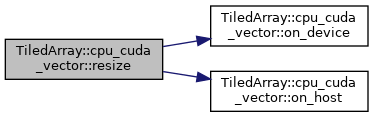

◆ size()
|
inline |
Definition at line 61 of file cpu_cuda_vector.h.
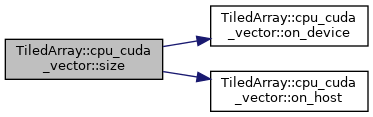
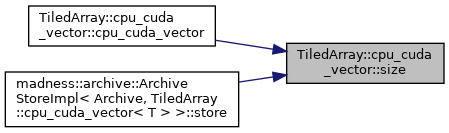
◆ to_device()
|
inline |
moves the data from the host to the device (even if it's there)
Definition at line 75 of file cpu_cuda_vector.h.


◆ to_host()
|
inline |
moves the data from the device to the host (even if it's there)
Definition at line 81 of file cpu_cuda_vector.h.


The documentation for this class was generated from the following file:
- TiledArray/cuda/cpu_cuda_vector.h