#include <TiledArray/shape.h>
#include <TiledArray/type_traits.h>
#include <TiledArray/util/function.h>
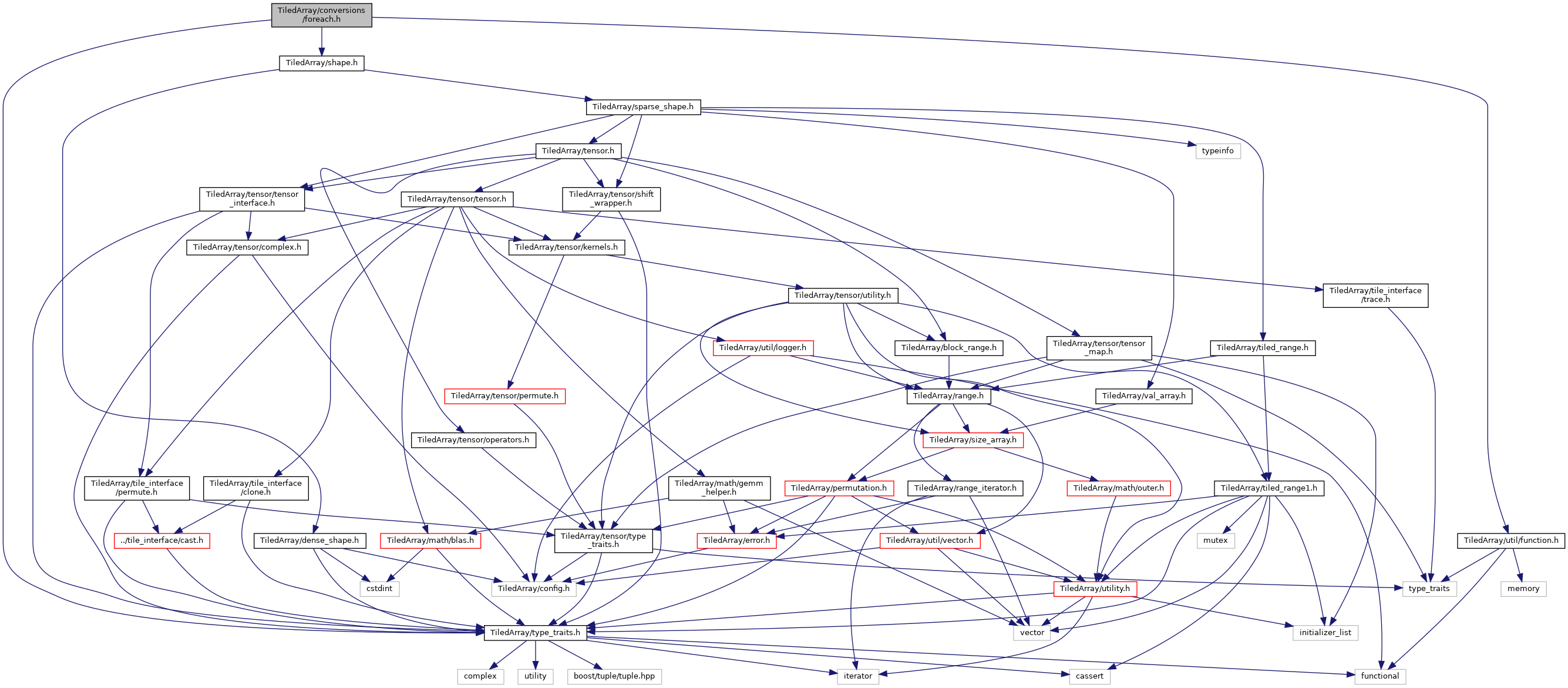
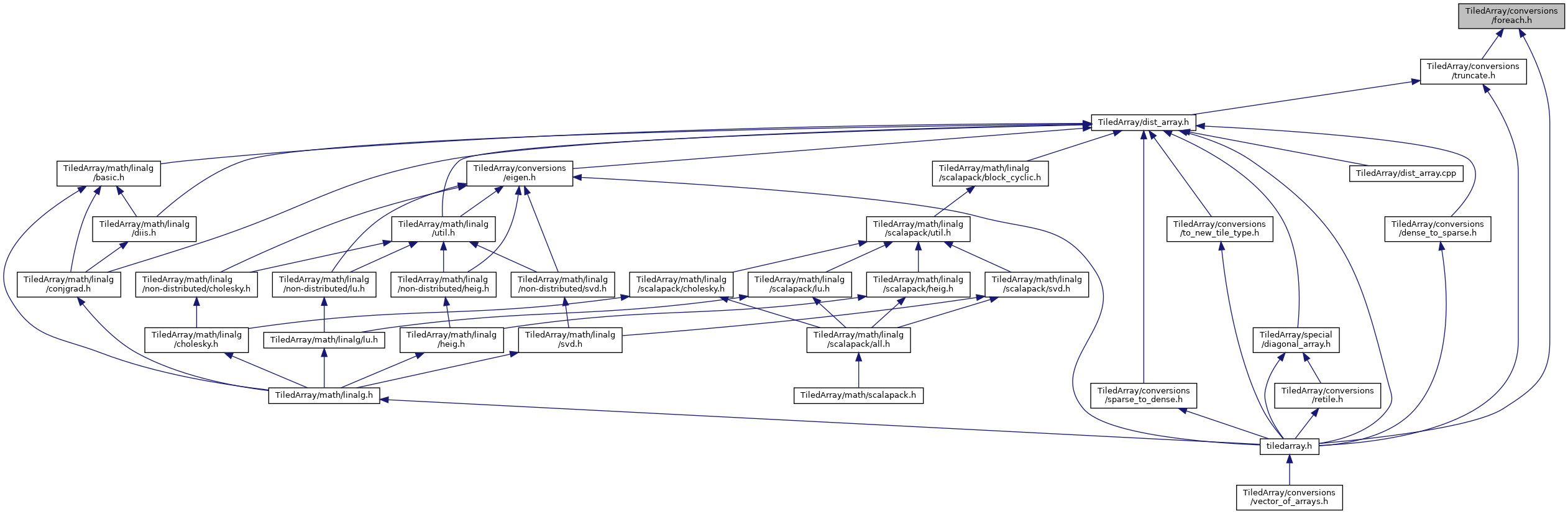
Namespaces | |
Eigen | |
Forward declarations. | |
TiledArray | |
TiledArray::detail | |
Enumerations | |
enum | TiledArray::ShapeReductionMethod { TiledArray::ShapeReductionMethod::Union, TiledArray::ShapeReductionMethod::Intersect } |
Functions | |
template<bool inplace = false, typename Op , typename ResultTile , typename ArgTile , typename Policy , typename... ArgTiles> | |
std::enable_if_t< is_dense_v< Policy >, DistArray< ResultTile, Policy > > | TiledArray::detail::foreach (Op &&op, const_if_t< not inplace, DistArray< ArgTile, Policy >> &arg, const DistArray< ArgTiles, Policy > &... args) |
base implementation of dense TiledArray::foreach More... | |
template<bool inplace = false, typename Op , typename ResultTile , typename ArgTile , typename Policy , typename... ArgTiles> | |
std::enable_if_t<!is_dense_v< Policy >, DistArray< ResultTile, Policy > > | TiledArray::detail::foreach (Op &&op, const ShapeReductionMethod shape_reduction, const_if_t< not inplace, DistArray< ArgTile, Policy >> &arg, const DistArray< ArgTiles, Policy > &... args) |
base implementation of sparse TiledArray::foreach More... | |
foreach/foreach_inplace functions | |
void(ResultTile&, const ArgTiles&...);
For sparse arrays void(ResultTile&, const ArgTiles&...);
or as real(ResultTile&, const ArgTiles&...);
where
| |
template<typename ResultTile , typename ArgTile , typename Policy , typename Op , typename = typename std::enable_if< !std::is_same<ResultTile, ArgTile>::value>::type> | |
std::enable_if_t< is_dense_v< Policy >, DistArray< ResultTile, Policy > > | TiledArray::foreach (const DistArray< ArgTile, Policy > &arg, Op &&op) |
Apply a function to each tile of a dense Array. More... | |
template<typename Tile , typename Policy , typename Op > | |
std::enable_if_t< is_dense_v< Policy >, DistArray< Tile, Policy > > | TiledArray::foreach (const DistArray< Tile, Policy > &arg, Op &&op) |
Apply a function to each tile of a dense Array. More... | |
template<typename Tile , typename Policy , typename Op , typename = typename std::enable_if<!TiledArray::detail::is_array< typename std::decay<Op>::type>::value>::type> | |
std::enable_if_t< is_dense_v< Policy >, void > | TiledArray::foreach_inplace (DistArray< Tile, Policy > &arg, Op &&op, bool fence=true) |
Modify each tile of a dense Array. More... | |
template<typename ResultTile , typename ArgTile , typename Policy , typename Op , typename = typename std::enable_if< !std::is_same<ResultTile, ArgTile>::value>::type> | |
std::enable_if_t<!is_dense_v< Policy >, DistArray< ResultTile, Policy > > | TiledArray::foreach (const DistArray< ArgTile, Policy > arg, Op &&op) |
Apply a function to each tile of a sparse Array. More... | |
template<typename Tile , typename Policy , typename Op > | |
std::enable_if_t<!is_dense_v< Policy >, DistArray< Tile, Policy > > | TiledArray::foreach (const DistArray< Tile, Policy > &arg, Op &&op) |
Apply a function to each tile of a sparse Array. More... | |
template<typename Tile , typename Policy , typename Op , typename = typename std::enable_if<!TiledArray::detail::is_array< typename std::decay<Op>::type>::value>::type> | |
std::enable_if_t<!is_dense_v< Policy >, void > | TiledArray::foreach_inplace (DistArray< Tile, Policy > &arg, Op &&op, bool fence=true) |
Modify each tile of a sparse Array. More... | |
template<typename ResultTile , typename LeftTile , typename RightTile , typename Policy , typename Op , typename = typename std::enable_if< !std::is_same<ResultTile, LeftTile>::value>::type> | |
std::enable_if_t< is_dense_v< Policy >, DistArray< ResultTile, Policy > > | TiledArray::foreach (const DistArray< LeftTile, Policy > &left, const DistArray< RightTile, Policy > &right, Op &&op) |
template<typename LeftTile , typename RightTile , typename Policy , typename Op > | |
std::enable_if_t< is_dense_v< Policy >, DistArray< LeftTile, Policy > > | TiledArray::foreach (const DistArray< LeftTile, Policy > &left, const DistArray< RightTile, Policy > &right, Op &&op) |
template<typename LeftTile , typename RightTile , typename Policy , typename Op > | |
std::enable_if_t< is_dense_v< Policy >, void > | TiledArray::foreach_inplace (DistArray< LeftTile, Policy > &left, const DistArray< RightTile, Policy > &right, Op &&op, bool fence=true) |
This function takes two input tiles and put result into the left tile. More... | |
template<typename ResultTile , typename LeftTile , typename RightTile , typename Policy , typename Op , typename = typename std::enable_if< !std::is_same<ResultTile, LeftTile>::value>::type> | |
std::enable_if_t<!is_dense_v< Policy >, DistArray< ResultTile, Policy > > | TiledArray::foreach (const DistArray< LeftTile, Policy > &left, const DistArray< RightTile, Policy > &right, Op &&op, const ShapeReductionMethod shape_reduction=ShapeReductionMethod::Intersect) |
template<typename LeftTile , typename RightTile , typename Policy , typename Op > | |
std::enable_if_t<!is_dense_v< Policy >, DistArray< LeftTile, Policy > > | TiledArray::foreach (const DistArray< LeftTile, Policy > &left, const DistArray< RightTile, Policy > &right, Op &&op, const ShapeReductionMethod shape_reduction=ShapeReductionMethod::Intersect) |
template<typename LeftTile , typename RightTile , typename Policy , typename Op > | |
std::enable_if_t<!is_dense_v< Policy >, void > | TiledArray::foreach_inplace (DistArray< LeftTile, Policy > &left, const DistArray< RightTile, Policy > &right, Op &&op, const ShapeReductionMethod shape_reduction=ShapeReductionMethod::Intersect, bool fence=true) |
This function takes two input tiles and put result into the left tile. More... | |