Typedefs | |
template<typename Tensor > | |
using | default_permutation_t = typename default_permutation< Tensor >::type |
template<typename T , typename U = void> | |
using | enable_if_trace_is_defined_t = std::enable_if_t< trace_is_defined_v< T >, U > |
SFINAE type for enabling code when the trace operation is defined. More... | |
template<typename T , typename U = void> | |
using | enable_if_numeric_t = std::enable_if_t< is_numeric_v< T >, U > |
SFINAE type for enabling code when T is a numeric type. More... | |
template<typename T > | |
using | numeric_t = typename TiledArray::detail::numeric_type< T >::type |
numeric_t<T> is an alias for numeric_type<T>::type More... | |
template<typename T > | |
using | scalar_t = typename TiledArray::detail::scalar_type< T >::type |
scalar_t<T> is an alias for scalar_type<T>::type More... | |
template<typename T > | |
using | remove_cvr_t = typename remove_cvr< T >::type |
template<bool B, typename T > | |
using | const_if_t = typename std::conditional< B, const T, T >::type |
prepends const to T if B is true More... | |
template<typename U > | |
using | param_type = typename param< U >::type |
template<class T > | |
using | iterator_t = decltype(std::begin(std::declval< T & >())) |
template<class T > | |
using | value_t = remove_cvr_t< decltype(*std::begin(std::declval< T & >()))> |
template<typename Scalar1 , typename Scalar2 > | |
using | add_t = decltype(std::declval< Scalar1 >()+std::declval< Scalar2 >()) |
template<typename Scalar1 , typename Scalar2 > | |
using | subt_t = decltype(std::declval< Scalar1 >() - std::declval< Scalar2 >()) |
template<typename Scalar1 , typename Scalar2 > | |
using | mult_t = decltype(std::declval< Scalar1 >() *std::declval< Scalar2 >()) |
template<typename T > | |
using | trange_t = typename T::trange_type |
template<typename T > | |
using | shape_t = typename T::shape_type |
template<typename T > | |
using | pmap_t = typename T::pmap_interface |
template<typename T > | |
using | policy_t = typename T::policy_type |
template<typename T > | |
using | vector_il = std::initializer_list< T > |
template<typename T > | |
using | matrix_il = std::initializer_list< vector_il< T > > |
template<typename T > | |
using | tensor3_il = std::initializer_list< matrix_il< T > > |
template<typename T > | |
using | tensor4_il = std::initializer_list< tensor3_il< T > > |
template<typename T > | |
using | tensor5_il = std::initializer_list< tensor4_il< T > > |
template<typename T > | |
using | tensor6_il = std::initializer_list< tensor5_il< T > > |
template<typename T > | |
using | enable_if_can_make_random_t = std::enable_if_t< can_make_random_v< T > > |
Functions | |
template<typename Impl > | |
bool | operator== (const TileReference< Impl > &a, const TileReference< Impl > &b) |
comparison operator for TileReference objects More... | |
template<typename Impl > | |
bool | operator!= (const TileReference< Impl > &a, const TileReference< Impl > &b) |
inequality operator for TileReference objects More... | |
template<typename Impl > | |
std::ostream & | operator<< (std::ostream &os, const TileReference< Impl > &a) |
redirect operator to std::ostream for TileReference objects More... | |
template<typename Impl > | |
bool | operator== (const TileConstReference< Impl > &a, const TileConstReference< Impl > &b) |
comparison operator for TileConstReference objects More... | |
template<typename Impl > | |
bool | operator!= (const TileConstReference< Impl > &a, const TileConstReference< Impl > &b) |
inequality operator for TileConstReference objects More... | |
template<typename Impl > | |
std::ostream & | operator<< (std::ostream &os, const TileConstReference< Impl > &a) |
redirect operator to std::ostream for TileConstReference objects More... | |
template<typename B > | |
void | swap (Bitset< B > &b0, Bitset< B > &b1) |
template<typename Block > | |
Bitset< Block > | operator& (Bitset< Block > left, const Bitset< Block > &right) |
Bitwise and operator of bitset. More... | |
template<typename Block > | |
Bitset< Block > | operator| (Bitset< Block > left, const Bitset< Block > &right) |
Bitwise or operator of bitset. More... | |
template<typename Block > | |
Bitset< Block > | operator^ (Bitset< Block > left, const Bitset< Block > &right) |
Bitwise xor operator of bitset. More... | |
template<typename Block > | |
std::ostream & | operator<< (std::ostream &os, const Bitset< Block > &bitset) |
template<typename DistArray_ , typename BTAS_Tensor_ > | |
void | counted_btas_subtensor_to_tensor (const BTAS_Tensor_ *src, DistArray_ *dst, const typename DistArray_::ordinal_type i, madness::AtomicInt *counter) |
template<typename TA_Tensor_ , typename BTAS_Tensor_ > | |
void | counted_tensor_to_btas_subtensor (const TA_Tensor_ &src, BTAS_Tensor_ *dst, madness::AtomicInt *counter) |
Task function for assigning a tensor to an Eigen submatrix. More... | |
template<bool sparse> | |
auto | make_shape (World &world, const TiledArray::TiledRange &trange) |
template<> | |
auto | make_shape< true > (World &world, const TiledArray::TiledRange &trange) |
template<> | |
auto | make_shape< false > (World &, const TiledArray::TiledRange &) |
template<typename A , typename Derived > | |
void | counted_eigen_submatrix_to_tensor (const Eigen::MatrixBase< Derived > *matrix, A *array, const typename A::ordinal_type i, madness::AtomicInt *counter) |
Task function for converting Eigen submatrix to a tensor. More... | |
template<typename Derived , typename T > | |
void | counted_tensor_to_eigen_submatrix (const T &tensor, Eigen::MatrixBase< Derived > *matrix, madness::AtomicInt *counter) |
Task function for assigning a tensor to an Eigen submatrix. More... | |
template<bool inplace = false, typename Op , typename ResultTile , typename ArgTile , typename Policy , typename... ArgTiles> | |
std::enable_if_t< is_dense_v< Policy >, DistArray< ResultTile, Policy > > | foreach (Op &&op, const_if_t< not inplace, DistArray< ArgTile, Policy >> &arg, const DistArray< ArgTiles, Policy > &... args) |
base implementation of dense TiledArray::foreach More... | |
template<bool inplace = false, typename Op , typename ResultTile , typename ArgTile , typename Policy , typename... ArgTiles> | |
std::enable_if_t<!is_dense_v< Policy >, DistArray< ResultTile, Policy > > | foreach (Op &&op, const ShapeReductionMethod shape_reduction, const_if_t< not inplace, DistArray< ArgTile, Policy >> &arg, const DistArray< ArgTiles, Policy > &... args) |
base implementation of sparse TiledArray::foreach More... | |
TA::TiledRange | prepend_dim_to_trange (std::size_t array_rank, const TiledArray::TiledRange &array_trange, std::size_t block_size=1) |
prepends an extra dimension to a TRange More... | |
template<typename Tile > | |
TA::SparseShape< float > | fuse_vector_of_shapes_tiles (madness::World &global_world, const std::vector< TA::DistArray< Tile, TA::SparsePolicy >> &arrays, const std::size_t array_rank, const TA::TiledRange &fused_trange) |
fuses the SparseShape objects of a tilewise-round-robin distributed vector of Arrays into single SparseShape object, with the vector index forming the first dimension. More... | |
template<typename Tile > | |
TA::DenseShape | fuse_vector_of_shapes_tiles (madness::World &, const std::vector< TA::DistArray< Tile, TA::DensePolicy >> &arrays, const std::size_t array_rank, const TA::TiledRange &fused_trange) |
fuses the DenseShape objects of a tilewise-round-robin distributed vector of Arrays into single DenseShape object, with the vector index forming the first dimension. More... | |
TA::SparseShape< float > | subshape_from_fused_tile (const TA::TiledRange &split_trange, const TA::SparsePolicy::shape_type &shape, const std::size_t tile_idx, const std::size_t split_ntiles, const std::size_t tile_size) |
extracts the shape of a slice of a fused array created with fuse_vector_of_arrays More... | |
TA::DenseShape | subshape_from_fused_tile (const TA::TiledRange &split_trange, const TA::DensePolicy::shape_type &shape, const std::size_t tile_idx, const std::size_t split_ntiles, const std::size_t tile_size) |
extracts the shape of a subarray of a fused array created with fuse_vector_of_arrays More... | |
const TiledArray::Range & | make_ta_range (const TiledArray::Range &range) |
template<::blas::Layout Order, typename... Args> | |
TiledArray::Range | make_ta_range (const btas::RangeNd< Order, Args... > &range) |
makes TiledArray::Range from a btas::RangeNd More... | |
template<typename Perm , typename Arg , typename Result , typename = std::enable_if_t<is_permutation_v<Perm>>> | |
void | permute_array (const Perm &perm, const Arg &arg, Result &result) |
Create a permuted copy of an array. More... | |
template<typename T , typename Container > | |
bool | operator== (const RangeIterator< T, Container > &left_it, const RangeIterator< T, Container > &right_it) |
Equality operator. More... | |
template<typename T , typename Container > | |
bool | operator!= (const RangeIterator< T, Container > &left_it, const RangeIterator< T, Container > &right_it) |
Inequality operator. More... | |
template<typename T > | |
std::vector< T > | operator* (const Permutation &perm, const SizeArray< T > &orig) |
template<typename T > | |
std::ostream & | operator<< (std::ostream &os, const SizeArray< T > &size_array) |
Range | diagonal_range (Range const &rng) |
Computes a range of the diagonal elements (if any) in a rank-d Range. More... | |
template<typename T > | |
Tensor< float > | diagonal_shape (TiledRange const &trange, T val) |
computes shape data (i.e. Frobenius norms of the tiles) for a constant diagonal tensor More... | |
template<typename RandomAccessIterator > | |
std::enable_if_t< is_iterator< RandomAccessIterator >::value, Tensor< float > > | diagonal_shape (TiledRange const &trange, RandomAccessIterator diagonals_begin, RandomAccessIterator diagonals_end={}) |
computes shape data (i.e. Frobenius norms of the tiles) for a non-constant diagonal tensor More... | |
template<typename Array , typename T > | |
void | write_diag_tiles_to_array_val (Array &A, T val) |
Writes tiles of a constant diagonal array. More... | |
template<typename Array , typename RandomAccessIterator > | |
std::enable_if_t< is_iterator< RandomAccessIterator >::value, void > | write_diag_tiles_to_array_rng (Array &A, RandomAccessIterator diagonals_begin) |
Writes tiles of a nonconstant diagonal array. More... | |
template<typename R , typename std::enable_if< is_numeric_v< R > &&!is_complex< R >::value >::type * = nullptr> | |
TILEDARRAY_FORCE_INLINE R | conj (const R r) |
Wrapper function for std::conj More... | |
template<typename R > | |
TILEDARRAY_FORCE_INLINE std::complex< R > | conj (const std::complex< R > z) |
Wrapper function for std::conj. More... | |
template<typename L , typename R , typename std::enable_if< is_numeric_v< L > &&!is_complex< L >::value >::type * = nullptr> | |
TILEDARRAY_FORCE_INLINE auto | inner_product (const L l, const R r) |
Inner product of a real value and a numeric value. More... | |
template<typename R , typename std::enable_if< is_numeric_v< R > &&!is_complex< R >::value >::type * = nullptr> | |
TILEDARRAY_FORCE_INLINE R | norm (const R r) |
Wrapper function for std::norm More... | |
template<typename R > | |
TILEDARRAY_FORCE_INLINE R | norm (const std::complex< R > z) |
Compute the norm of a complex number z More... | |
template<typename S > | |
ComplexConjugate< S > | conj_op (const S factor) |
ComplexConjugate operator factory function. More... | |
ComplexConjugate< void > | conj_op () |
ComplexConjugate operator factory function. More... | |
template<typename L , typename R > | |
TILEDARRAY_FORCE_INLINE auto | operator* (const L value, const ComplexConjugate< R > op) |
template<typename L > | |
TILEDARRAY_FORCE_INLINE auto | operator* (const L value, const ComplexConjugate< void > &) |
template<typename L > | |
TILEDARRAY_FORCE_INLINE auto | operator* (const L value, const ComplexConjugate< ComplexNegTag > &) |
template<typename L , typename R > | |
TILEDARRAY_FORCE_INLINE auto | operator* (const ComplexConjugate< L > op, const R value) |
template<typename R > | |
TILEDARRAY_FORCE_INLINE auto | operator* (const ComplexConjugate< void >, const R value) |
template<typename R > | |
TILEDARRAY_FORCE_INLINE auto | operator* (const ComplexConjugate< ComplexNegTag >, const R value) |
template<typename L , typename R , typename std::enable_if<!std::is_void< R >::value >::type * = nullptr> | |
TILEDARRAY_FORCE_INLINE L & | operator*= (L &value, const ComplexConjugate< R > op) |
template<typename L > | |
TILEDARRAY_FORCE_INLINE L & | operator*= (L &value, const ComplexConjugate< void > &) |
template<typename L > | |
TILEDARRAY_FORCE_INLINE L & | operator*= (L &value, const ComplexConjugate< ComplexNegTag > &) |
template<typename T > | |
auto | abs (const ComplexConjugate< T > &a) |
int | abs (const ComplexConjugate< void > &a) |
template<typename TR , typename Op , typename T1 , typename... Ts, typename std::enable_if< is_tensor< TR, T1, Ts... >::value||is_tensor_of_tensor< TR, T1, Ts... >::value >::type * = nullptr> | |
TR | tensor_op (Op &&op, const T1 &tensor1, const Ts &... tensors) |
Tensor operations with contiguous data. More... | |
template<typename TR , typename Op , typename T1 , typename... Ts, typename std::enable_if<(is_tensor< T1, Ts... >::value||is_tensor_of_tensor< TR, T1, Ts... >::value) &&is_contiguous_tensor< T1, Ts... >::value >::type * = nullptr> | |
TR | tensor_op (Op &&op, const Permutation &perm, const T1 &tensor1, const Ts &... tensors) |
Tensor permutation operations with contiguous data. More... | |
template<typename Op , typename TR , typename... Ts, typename std::enable_if< is_tensor< TR, Ts... >::value &&is_contiguous_tensor< TR, Ts... >::value >::type * = nullptr> | |
void | inplace_tensor_op (Op &&op, TR &result, const Ts &... tensors) |
In-place tensor operations with contiguous data. More... | |
template<typename InputOp , typename OutputOp , typename TR , typename T1 , typename... Ts, typename std::enable_if< is_tensor< TR, T1, Ts... >::value &&is_contiguous_tensor< TR, T1, Ts... >::value >::type * = nullptr> | |
void | inplace_tensor_op (InputOp &&input_op, OutputOp &&output_op, const Permutation &perm, TR &result, const T1 &tensor1, const Ts &... tensors) |
In-place tensor permutation operations with contiguous data. More... | |
template<typename Op , typename TR , typename... Ts, typename std::enable_if< is_tensor< TR, Ts... >::value &&is_contiguous_tensor< TR, Ts... >::value >::type * = nullptr> | |
void | tensor_init (Op &&op, TR &result, const Ts &... tensors) |
Initialize tensor with contiguous tensor arguments. More... | |
template<typename Op , typename TR , typename T1 , typename... Ts, typename std::enable_if< is_tensor< TR, T1, Ts... >::value >::type * = nullptr> | |
void | tensor_init (Op &&op, const Permutation &perm, TR &result, const T1 &tensor1, const Ts &... tensors) |
Initialize tensor with permuted tensor arguments. More... | |
template<typename Op , typename TR , typename T1 , typename... Ts, typename std::enable_if< is_tensor< TR, T1, Ts... >::value &&is_contiguous_tensor< TR >::value &&!is_contiguous_tensor< T1, Ts... >::value >::type * = nullptr> | |
void | tensor_init (Op &&op, TR &result, const T1 &tensor1, const Ts &... tensors) |
Initialize tensor with one or more non-contiguous tensor arguments. More... | |
template<typename ReduceOp , typename JoinOp , typename Scalar , typename T1 , typename... Ts, typename std::enable_if_t< is_tensor< T1, Ts... >::value &&is_contiguous_tensor< T1, Ts... >::value &&!is_reduce_op_v< std::decay_t< ReduceOp >, std::decay_t< Scalar >, std::decay_t< T1 >, std::decay_t< Ts >... >> * = nullptr> | |
Scalar | tensor_reduce (ReduceOp &&reduce_op, JoinOp &&join_op, Scalar identity, const T1 &tensor1, const Ts &... tensors) |
Reduction operation for contiguous tensors. More... | |
template<typename SizeType , typename ExtentType > | |
void | fuse_dimensions (SizeType *MADNESS_RESTRICT const fused_size, SizeType *MADNESS_RESTRICT const fused_weight, const ExtentType *MADNESS_RESTRICT const size, const Permutation &perm) |
Compute the fused dimensions for permutation. More... | |
template<typename InputOp , typename OutputOp , typename Result , typename Perm , typename Arg0 , typename... Args, typename = std::enable_if_t<detail::is_permutation_v<Perm>>> | |
void | permute (InputOp &&input_op, OutputOp &&output_op, Result &result, const Perm &perm, const Arg0 &arg0, const Args &... args) |
Construct a permuted tensor copy. More... | |
template<typename Left , typename Right > | |
bool | is_range_congruent (const Left &left, const ShiftWrapper< Right > &right) |
Check for congruent range objects with a shifted tensor. More... | |
template<typename T , typename Range , typename OpResult > | |
bool | operator== (const TensorInterface< T, Range, OpResult > &first, const TensorInterface< T, Range, OpResult > &second) |
Shallow comparison operator. More... | |
template<typename T , typename std::enable_if< is_contiguous_tensor< T >::value >::type * = nullptr> | |
auto | clone_range (const T &tensor) |
Create a copy of the range of the tensor. More... | |
template<typename T , typename std::enable_if< !is_contiguous_tensor< T >::value >::type * = nullptr> | |
Range | clone_range (const T &tensor) |
Create a contiguous copy of the range of the tensor. More... | |
template<typename T1 , typename T2 , typename std::enable_if<!(is_shifted< T1 >::value||is_shifted< T2 >::value)>::type * = nullptr> | |
bool | is_range_congruent (const T1 &tensor1, const T2 &tensor2) |
Test that the ranges of a pair of tensors are congruent. More... | |
template<typename T1 , typename T2 , typename std::enable_if<!(is_shifted< T1 >::value||is_shifted< T2 >::value)>::type * = nullptr> | |
bool | is_range_congruent (const T1 &tensor1, const T2 &tensor2, const Permutation &perm) |
Test that the ranges of a pair of permuted tensors are congruent. More... | |
template<typename T > | |
constexpr bool | is_range_set_congruent (const Permutation &perm, const T &tensor) |
Test that the ranges of a permuted tensor is congruent with itself. More... | |
template<typename T1 , typename T2 , typename... Ts> | |
bool | is_range_set_congruent (const Permutation &perm, const T1 &tensor1, const T2 &tensor2, const Ts &... tensors) |
Test that the ranges of a permuted set of tensors are congruent. More... | |
template<typename T > | |
constexpr bool | is_range_set_congruent (const T &tensor) |
Test that the ranges of a tensor is congruent with itself. More... | |
template<typename T1 , typename T2 , typename... Ts> | |
bool | is_range_set_congruent (const T1 &tensor1, const T2 &tensor2, const Ts &... tensors) |
Test that the ranges of a set of tensors are congruent. More... | |
template<typename T > | |
T::size_type | inner_size_helper (const T &tensor) |
Get the inner size. More... | |
template<typename T1 , typename T2 > | |
T1::size_type | inner_size_helper (const T1 &tensor1, const T2 &tensor2) |
Get the inner size of two tensors. More... | |
template<typename T1 , typename T2 , typename std::enable_if<!is_contiguous_tensor< T1 >::value &&is_contiguous_tensor< T2 >::value >::type * = nullptr> | |
T1::size_type | inner_size (const T1 &tensor1, const T2 &) |
Get the inner size of two tensors. More... | |
template<typename T , typename std::enable_if< !is_contiguous_tensor< T >::value >::type * = nullptr> | |
T::size_type | inner_size (const T &tensor) |
Get the inner size. More... | |
constexpr bool | empty () |
Test for empty tensors in an empty list. More... | |
template<typename T1 , typename... Ts> | |
bool | empty (const T1 &tensor1, const Ts &... tensors) |
Test for empty tensors. More... | |
template<typename T > | |
Tile< T > | make_tile (T &&t) |
Factory function for tiles. More... | |
template<typename Iter1 , typename Iter2 , typename Op > | |
BinaryTransformIterator< Iter1, Iter2, Op > | make_tran_it (Iter1 it1, Iter2 it2, Op op) |
Binary Transform iterator factory. More... | |
template<typename Iter , typename Op > | |
UnaryTransformIterator< Iter, Op > | make_tran_it (Iter it, Op op) |
Unary Transform iterator factory. More... | |
template<std::size_t I, typename T > | |
auto | get (T &&t) |
template<typename GeneralizedPair , typename = std::enable_if_t<is_gpair_v<GeneralizedPair>>> | |
decltype(auto) | at (GeneralizedPair &&v, std::size_t idx) |
at(pair, i) extracts i-th element from gpair More... | |
std::string | dummy_annotation (unsigned int n_outer_size, unsigned int n_inner_size=0) |
auto | remove_whitespace (std::string s) |
auto | tokenize_index (const std::string &s, char delim) |
bool | is_valid_index (const std::string &idx) |
bool | is_tot_index (const std::string &idx) |
auto | split_index (const std::string &idx) |
template<typename A > | |
void | print_array (std::ostream &out, const A &a, const std::size_t n) |
Print the content of an array like object. More... | |
template<typename A > | |
void | print_array (std::ostream &out, const A &a) |
Print the content of an array like object. More... | |
std::atomic< bool > & | ignore_tile_position_accessor () |
template<typename T > | |
std::ostream & | operator<< (std::ostream &os, const ValArray< T > &val_array) |
Variables | |
template<typename T > | |
constexpr const bool | is_btas_tensor_v = is_btas_tensor<T>::value |
template<typename... Ts> | |
constexpr const bool | is_tensor_v = is_tensor<Ts...>::value |
template<typename... Ts> | |
constexpr const bool | is_tensor_of_tensor_v = is_tensor_of_tensor<Ts...>::value |
template<typename T > | |
constexpr const bool | is_ta_tensor_v = is_ta_tensor<T>::value |
template<typename... Ts> | |
constexpr const bool | is_contiguous_tensor_v |
template<typename... Ts> | |
constexpr const bool | is_shifted_v = is_shifted<Ts...>::value |
template<typename ReduceOp , typename Result , typename... Args> | |
constexpr const bool | is_reduce_op_v |
template<typename T > | |
constexpr auto | trace_is_defined_v = TraceIsDefined<T>::value |
template<typename T > | |
constexpr const bool | is_type_v = is_type<T>::value |
template<typename T > | |
constexpr const bool | is_complete_type_v = is_complete_type<T>::value |
template<class From , class To > | |
constexpr const bool | has_conversion_operator_v |
template<class From , class To > | |
constexpr const bool | is_explicitly_convertible_v |
template<class From , class To > | |
constexpr const bool | is_implicitly_convertible_v |
template<class From , class To > | |
constexpr const bool | is_convertible_v = is_convertible<From, To>::value |
template<typename T > | |
constexpr const bool | is_complex_v = is_complex<T>::value |
is_complex_v<T> is an alias for is_complex<T>::value More... | |
template<typename T > | |
constexpr const bool | is_numeric_v = is_numeric<T>::value |
is_numeric_v<T> is an alias for is_numeric<T>::value More... | |
template<typename T > | |
constexpr const bool | is_scalar_v = is_scalar<T>::value |
is_scalar_v<T> is an alias for is_scalar_v<T> More... | |
template<typename T > | |
constexpr const bool | is_array_tile_v = is_array_tile<T>::value |
is_array_tile_v<T> is an alias for is_array_tile<T>::value More... | |
template<typename T > | |
constexpr const bool | is_non_array_lazy_tile_v |
template<typename T > | |
constexpr const bool | is_strictly_ordered_v = is_strictly_ordered<T>::value |
is_strictly_ordered_v<T> is an alias for is_strictly_ordered<T>::value More... | |
template<typename... Ts> | |
constexpr const bool | is_integral_list_v = is_integral_list<Ts...>::value |
is_integral_list_v<T> is an alias for is_integral_list<T>::value More... | |
template<typename T > | |
constexpr const bool | is_tuple_v = is_tuple<T>::value |
is_tuple_v<T> is an alias for is_tuple<T>::value More... | |
template<std::size_t I, typename T > | |
constexpr const bool | is_std_gettable_v = is_std_gettable<I, T>::value |
template<std::size_t I, typename T > | |
constexpr const bool | is_boost_gettable_v = is_boost_gettable<I, T>::value |
template<std::size_t I, typename T > | |
constexpr const bool | is_gettable_v |
template<typename T > | |
constexpr const bool | is_gettable_pair_v = is_gettable_pair<T>::value |
is_gettable_pair_v<T> is an alias for is_gettable_pair<T>::value More... | |
template<typename T > | |
constexpr const bool | is_integral_pair_v = is_integral_pair<T>::value |
is_integral_pair_v<T> is an alias for is_integral_pair<T>::value More... | |
template<typename... Ts> | |
constexpr const bool | is_integral_pair_list_v |
template<typename T > | |
constexpr const bool | is_integral_tuple_v = is_integral_tuple<T>::value |
is_integral_tuple_v<T> is an alias for is_integral_tuple<T>::value More... | |
template<typename T > | |
constexpr const bool | is_pair_v = is_pair<T>::value |
is_pair_v<T> is an alias for is_pair<T>::value More... | |
Typedef Documentation
◆ add_t
using TiledArray::detail::add_t = typedef decltype(std::declval<Scalar1>() + std::declval<Scalar2>()) |
Definition at line 1153 of file type_traits.h.
◆ const_if_t
using TiledArray::detail::const_if_t = typedef typename std::conditional<B, const T, T>::type |
prepends const
to T
if B
is true
Definition at line 966 of file type_traits.h.
◆ default_permutation_t
using TiledArray::detail::default_permutation_t = typedef typename default_permutation<Tensor>::type |
Definition at line 290 of file type_traits.h.
◆ enable_if_can_make_random_t
using TiledArray::detail::enable_if_can_make_random_t = typedef std::enable_if_t<can_make_random_v<T> > |
◆ enable_if_numeric_t
using TiledArray::detail::enable_if_numeric_t = typedef std::enable_if_t<is_numeric_v<T>, U> |
SFINAE type for enabling code when T
is a numeric type.
Definition at line 649 of file type_traits.h.
◆ enable_if_trace_is_defined_t
using TiledArray::detail::enable_if_trace_is_defined_t = typedef std::enable_if_t<trace_is_defined_v<T>, U> |
◆ iterator_t
using TiledArray::detail::iterator_t = typedef decltype(std::begin(std::declval<T&>())) |
- Template Parameters
-
T a range type iterator_t<T>
is the iterator type, i.e. the type returned bystd::begin(T&)
- Warning
- will be replaced by C++20 ranges::iterator_t
Definition at line 1106 of file type_traits.h.
◆ matrix_il
using TiledArray::detail::matrix_il = typedef std::initializer_list<vector_il<T> > |
Definition at line 381 of file initializer_list.h.
◆ mult_t
using TiledArray::detail::mult_t = typedef decltype(std::declval<Scalar1>() * std::declval<Scalar2>()) |
Definition at line 1159 of file type_traits.h.
◆ numeric_t
using TiledArray::detail::numeric_t = typedef typename TiledArray::detail::numeric_type<T>::type |
numeric_t<T>
is an alias for numeric_type<T>::type
Definition at line 730 of file type_traits.h.
◆ param_type
using TiledArray::detail::param_type = typedef typename param<U>::type |
Definition at line 991 of file type_traits.h.
◆ pmap_t
using TiledArray::detail::pmap_t = typedef typename T::pmap_interface |
Definition at line 1177 of file type_traits.h.
◆ policy_t
using TiledArray::detail::policy_t = typedef typename T::policy_type |
Definition at line 1180 of file type_traits.h.
◆ remove_cvr_t
using TiledArray::detail::remove_cvr_t = typedef typename remove_cvr<T>::type |
Definition at line 962 of file type_traits.h.
◆ scalar_t
using TiledArray::detail::scalar_t = typedef typename TiledArray::detail::scalar_type<T>::type |
scalar_t<T>
is an alias for scalar_type<T>::type
Definition at line 760 of file type_traits.h.
◆ shape_t
using TiledArray::detail::shape_t = typedef typename T::shape_type |
Definition at line 1174 of file type_traits.h.
◆ subt_t
using TiledArray::detail::subt_t = typedef decltype(std::declval<Scalar1>() - std::declval<Scalar2>()) |
Definition at line 1156 of file type_traits.h.
◆ tensor3_il
using TiledArray::detail::tensor3_il = typedef std::initializer_list<matrix_il<T> > |
Definition at line 385 of file initializer_list.h.
◆ tensor4_il
using TiledArray::detail::tensor4_il = typedef std::initializer_list<tensor3_il<T> > |
Definition at line 389 of file initializer_list.h.
◆ tensor5_il
using TiledArray::detail::tensor5_il = typedef std::initializer_list<tensor4_il<T> > |
Definition at line 393 of file initializer_list.h.
◆ tensor6_il
using TiledArray::detail::tensor6_il = typedef std::initializer_list<tensor5_il<T> > |
Definition at line 397 of file initializer_list.h.
◆ trange_t
using TiledArray::detail::trange_t = typedef typename T::trange_type |
Definition at line 1171 of file type_traits.h.
◆ value_t
using TiledArray::detail::value_t = typedef remove_cvr_t<decltype(*std::begin(std::declval<T&>()))> |
- Template Parameters
-
T a range type value_t<T>
is the value type, i.e. the type to whichstd::begin(T&)
dereferences to
- Warning
- will be replaced by C++20 ranges::value_t
Definition at line 1113 of file type_traits.h.
◆ vector_il
using TiledArray::detail::vector_il = typedef std::initializer_list<T> |
Definition at line 377 of file initializer_list.h.
Function Documentation
◆ abs() [1/2]
|
inline |
◆ abs() [2/2]
|
inline |
◆ at()
decltype(auto) TiledArray::detail::at | ( | GeneralizedPair && | v, |
std::size_t | idx | ||
) |
at(pair, i)
extracts i-th element from gpair
Definition at line 1268 of file type_traits.h.
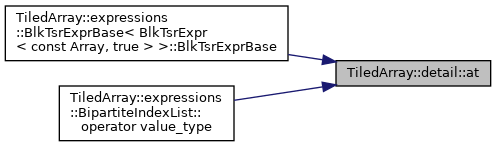
◆ clone_range() [1/2]
|
inline |
Create a copy of the range of the tensor.
- Template Parameters
-
T The tensor type
- Parameters
-
tensor The tensor with the range to be cloned
- Returns
- A contiguous range with the same lower and upper bounds as the range of
tensor
.
Definition at line 47 of file utility.h.
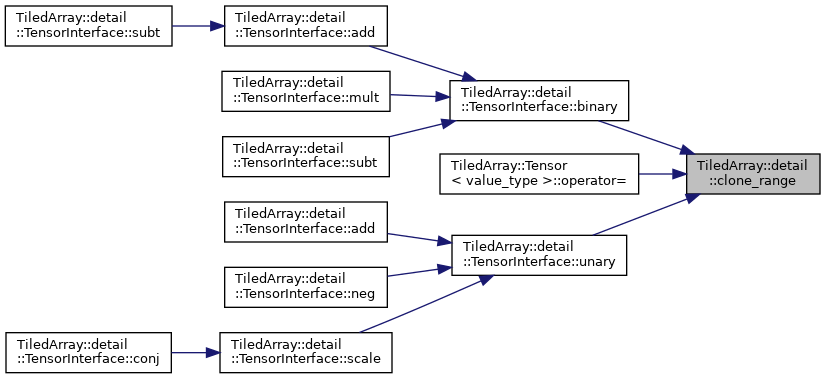
◆ clone_range() [2/2]
|
inline |
◆ conj() [1/2]
TILEDARRAY_FORCE_INLINE R TiledArray::detail::conj | ( | const R | r | ) |
Wrapper function for std::conj
This function disables the call to std::conj
for real values to prevent the result from being converted into a complex value.
- Template Parameters
-
R A real scalar type
- Parameters
-
r The real scalar
- Returns
r
Definition at line 45 of file complex.h.
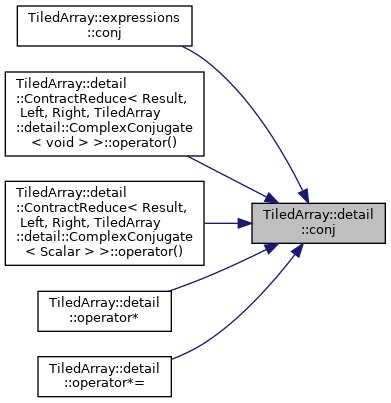
◆ conj() [2/2]
TILEDARRAY_FORCE_INLINE std::complex<R> TiledArray::detail::conj | ( | const std::complex< R > | z | ) |
◆ conj_op() [1/2]
|
inline |
ComplexConjugate operator factory function.
- Returns
- A complex conjugate operator
◆ conj_op() [2/2]
|
inline |
ComplexConjugate operator factory function.
- Template Parameters
-
S The scalar type
- Parameters
-
factor The scaling factor
- Returns
- A scaling complex conjugate operator
Definition at line 204 of file complex.h.
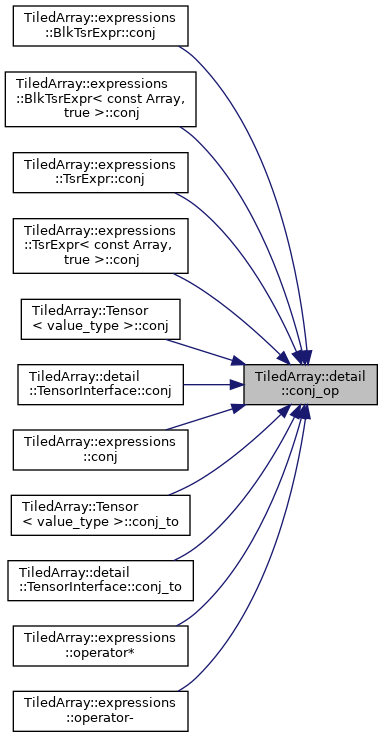
◆ counted_btas_subtensor_to_tensor()
void TiledArray::detail::counted_btas_subtensor_to_tensor | ( | const BTAS_Tensor_ * | src, |
DistArray_ * | dst, | ||
const typename DistArray_::ordinal_type | i, | ||
madness::AtomicInt * | counter | ||
) |
Task function for converting btas::Tensor subblock to a TiledArray::DistArray
- Template Parameters
-
DistArray_ a TiledArray::DistArray type TArgs the type pack in btas::Tensor<TArgs...> type
- Parameters
-
src The btas::Tensor object whose block will be copied dst The array that will hold the result i The index of the tile to be copied counter The task counter
Definition at line 126 of file btas.h.

◆ counted_eigen_submatrix_to_tensor()
void TiledArray::detail::counted_eigen_submatrix_to_tensor | ( | const Eigen::MatrixBase< Derived > * | matrix, |
A * | array, | ||
const typename A::ordinal_type | i, | ||
madness::AtomicInt * | counter | ||
) |
Task function for converting Eigen submatrix to a tensor.
- Template Parameters
-
A Array type Derived The matrix type
- Parameters
-
matrix The matrix that will be copied array The array that will hold the result i The index of the tile to be copied counter The task counter
Definition at line 338 of file eigen.h.

◆ counted_tensor_to_btas_subtensor()
void TiledArray::detail::counted_tensor_to_btas_subtensor | ( | const TA_Tensor_ & | src, |
BTAS_Tensor_ * | dst, | ||
madness::AtomicInt * | counter | ||
) |
Task function for assigning a tensor to an Eigen submatrix.
- Template Parameters
-
Tensor_ a TiledArray::Tensor type TArgs the type pack in btas::Tensor<TArgs...> type
- Parameters
-
src The source tensor dst The destination tensor counter The task counter
Definition at line 143 of file btas.h.
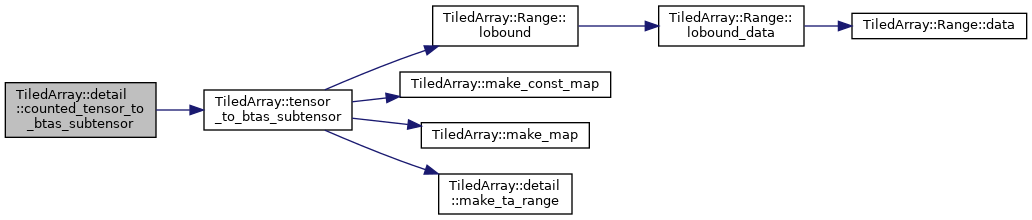
◆ counted_tensor_to_eigen_submatrix()
void TiledArray::detail::counted_tensor_to_eigen_submatrix | ( | const T & | tensor, |
Eigen::MatrixBase< Derived > * | matrix, | ||
madness::AtomicInt * | counter | ||
) |
Task function for assigning a tensor to an Eigen submatrix.
- Template Parameters
-
Derived The matrix type T Tensor type
- Parameters
-
matrix The matrix to be assigned tensor The tensor to be copied counter The task counter
Definition at line 356 of file eigen.h.


◆ diagonal_range()
Computes a range of the diagonal elements (if any) in a rank-d Range.
computes [min,max) describing the diagonal elements that rank-d Range contains; if the input Range contains no diagonal elements this return an empty Range
- Parameters
-
[in] rng an input (rank-d) Range
- Returns
- the range of diagonal elements, as a rank-1 Range
Definition at line 45 of file diagonal_array.h.
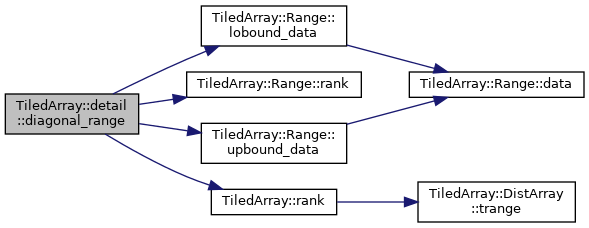
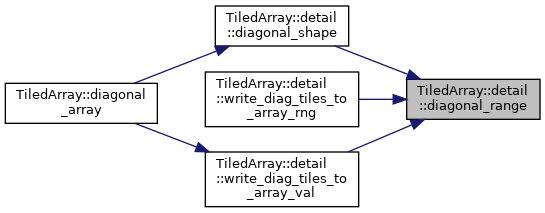
◆ diagonal_shape() [1/2]
std::enable_if_t<is_iterator<RandomAccessIterator>::value, Tensor<float> > TiledArray::detail::diagonal_shape | ( | TiledRange const & | trange, |
RandomAccessIterator | diagonals_begin, | ||
RandomAccessIterator | diagonals_end = {} |
||
) |
computes shape data (i.e. Frobenius norms of the tiles) for a non-constant diagonal tensor
- Template Parameters
-
RandomAccessIterator an iterator over the range of diagonal elements
- Parameters
-
[in] trange a TiledRange of the result [in] diagonals_begin the begin iterator of the range of the diagonals [in] diagonals_end the end iterator of the range of the diagonals; if not given, default initialized and thus will not be checked
- Returns
- a Tensor<float> containing the Frobenius norms of the tiles of a DistArray with
val
on the diagonal and zeroes elsewhere
Definition at line 110 of file diagonal_array.h.
◆ diagonal_shape() [2/2]
Tensor<float> TiledArray::detail::diagonal_shape | ( | TiledRange const & | trange, |
T | val | ||
) |
computes shape data (i.e. Frobenius norms of the tiles) for a constant diagonal tensor
- Template Parameters
-
T a numeric type
- Parameters
-
trange a TiledRange of the result val value of the diagonal elements
- Returns
- a Tensor<float> containing the Frobenius norms of the tiles of a DistArray with
val
on the diagonal and zeroes elsewhere
Definition at line 70 of file diagonal_array.h.
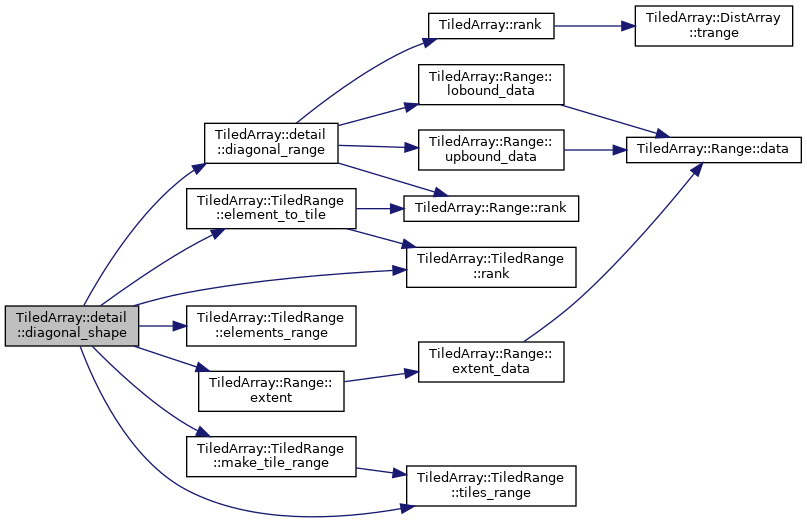

◆ dummy_annotation()
|
inline |
◆ empty() [1/2]
|
inlineconstexpr |
◆ empty() [2/2]
|
inline |
Test for empty tensors.
- Template Parameters
-
T1 The first tensor type Ts The remaining tensor types
- Parameters
-
tensor1 The first tensor to test tensors The remaining tensors to test
- Returns
true
if one or more tensors are empty
Definition at line 330 of file utility.h.

◆ foreach() [1/2]
|
inline |
base implementation of sparse TiledArray::foreach
- Template Parameters
-
Op the operation type, the following expression must be valid and return void
or be convertible toDistArray<ArgTile
, Policy>::shape_type::value_type :Op(ResultTile&, constArgTile&, const ArgTiles&...)
- Note
- can't autodeduce
ResultTile
fromvoid
Op(ResultTile,ArgTile)
Definition at line 243 of file foreach.h.
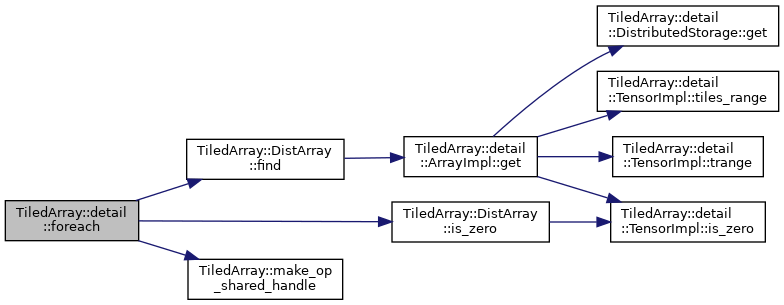
◆ foreach() [2/2]
|
inline |
base implementation of dense TiledArray::foreach
- Note
- can't autodeduce
ResultTile
fromvoid
Op(ResultTile,ArgTile)
Definition at line 173 of file foreach.h.
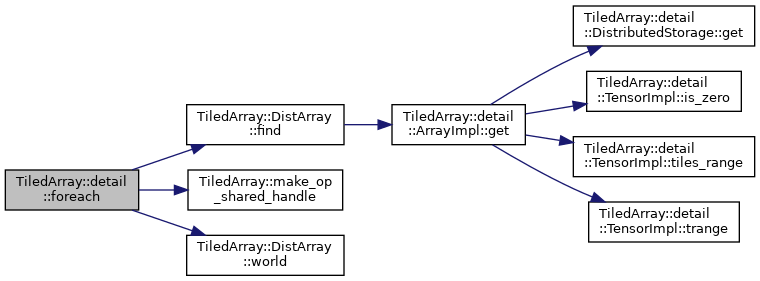
◆ fuse_dimensions()
|
inline |
Compute the fused dimensions for permutation.
This function will compute the fused dimensions of a tensor for use in permutation algorithms. The idea is to partition the stride 1 dimensions in both the input and output tensor, which yields a forth-order tensor (second- and third-order tensors have size of 1 and stride of 0 in the unused dimensions).
- Template Parameters
-
SizeType An unsigned integral type
- Parameters
-
[out] fused_size An array for the fused size output [out] fused_weight An array for the fused weight output [in] size An array that holds the unfused size information of the argument tensor [in] perm The permutation that will be applied to the argument tensor(s).
Definition at line 51 of file permute.h.

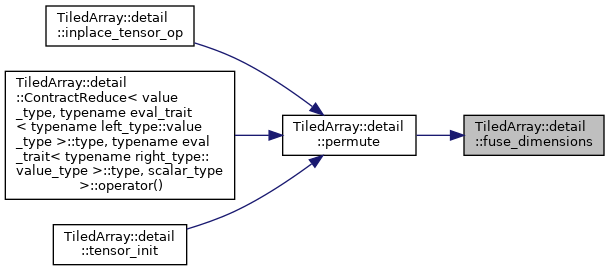
◆ fuse_vector_of_shapes_tiles() [1/2]
TA::DenseShape TiledArray::detail::fuse_vector_of_shapes_tiles | ( | madness::World & | , |
const std::vector< TA::DistArray< Tile, TA::DensePolicy >> & | arrays, | ||
const std::size_t | array_rank, | ||
const TA::TiledRange & | fused_trange | ||
) |
fuses the DenseShape objects of a tilewise-round-robin distributed vector of Arrays into single DenseShape object, with the vector index forming the first dimension.
This is the same as the sparse version above, but far simpler.
- Parameters
-
global_world the World object in which the new fused array will live [in] arrays a vector of DistArray objects; these are local components of a vector distributed tilewise in round-robin manner; each element of arrays
must have the same TiledRange;array_rank Sum of the sizes of arrays
on each rank (the size ofarrays
on each rank will depend on world.size)[in] fused_trange the TiledRange of the fused arrays
- Returns
- DenseShape of fused Array object
Definition at line 159 of file vector_of_arrays.h.
◆ fuse_vector_of_shapes_tiles() [2/2]
TA::SparseShape<float> TiledArray::detail::fuse_vector_of_shapes_tiles | ( | madness::World & | global_world, |
const std::vector< TA::DistArray< Tile, TA::SparsePolicy >> & | arrays, | ||
const std::size_t | array_rank, | ||
const TA::TiledRange & | fused_trange | ||
) |
fuses the SparseShape objects of a tilewise-round-robin distributed vector of Arrays into single SparseShape object, with the vector index forming the first dimension.
This is used to fuse shapes of a sequence of N-dimensional arrays into the shape of the fused (N+1)-dimensional array. The sequence is tiled, and the tiles are round-robin distributed. Hence for p ranks tile I will reside on processor Ip ; this tile includes shapes of arrays [I*b, (I+1)*b).
- Parameters
-
global_world the World object in which the new fused array will live [in] arrays a vector of DistArray objects; these are local components of a vector distributed tilewise in round-robin manner; each element of arrays
must have the same TiledRange;array_rank Sum of the sizes of arrays
on each rank (the size ofarrays
on each rank will depend on world.size)[in] fused_trange the TiledRange of the fused arrays
- Returns
- SparseShape of fused Array object TODO rename to fuse_tilewise_vector_of_shapes
Definition at line 71 of file vector_of_arrays.h.
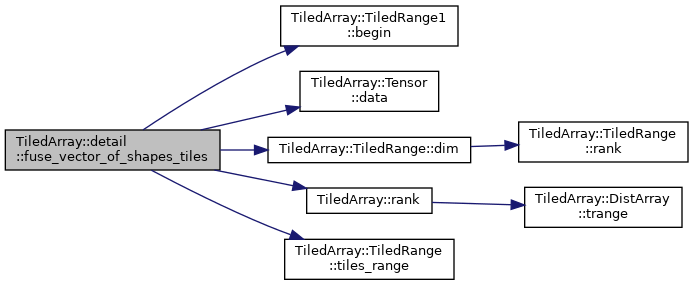

◆ get()
auto TiledArray::detail::get | ( | T && | t | ) |
Definition at line 858 of file type_traits.h.
◆ ignore_tile_position_accessor()
|
inline |
◆ inner_product()
TILEDARRAY_FORCE_INLINE auto TiledArray::detail::inner_product | ( | const L | l, |
const R | r | ||
) |
Inner product of a real value and a numeric value.
Inner product of a complex value and a numeric value.
- Template Parameters
-
L A real scalar type R A numeric type
- Returns
r
- Template Parameters
-
L A complex type R A numeric type
- Returns
r
Definition at line 67 of file complex.h.
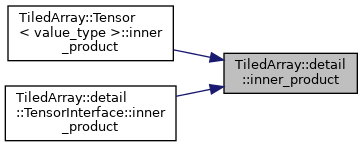
◆ inner_size() [1/2]
|
inline |
Get the inner size.
This function searches of the largest contiguous size in the range of a non-contiguous tensor. At a minimum, this is equal to the size of the stride-one dimension.
- Template Parameters
-
T A tensor type
- Parameters
-
tensor The tensor to be tested
- Returns
- The largest contiguous, inner-dimension size.
Definition at line 310 of file utility.h.

◆ inner_size() [2/2]
|
inline |
Get the inner size of two tensors.
This function searches of the largest, common contiguous size in the ranges of two non-contiguous tensors. At a minimum, this is equal to the size of the stride-one dimension.
- Template Parameters
-
T1 The first tensor type T2 The secont tensor type
- Parameters
-
tensor1 The first tensor to be tested
- Returns
- The largest contiguous, inner-dimension size.
This function searches of the largest, common contiguous size in the ranges of two non-contiguous tensors. At a minimum, this is equal to the size of the stride-one dimension.
- Template Parameters
-
T1 The first tensor type T2 The secont tensor type
- Parameters
-
tensor2 The second tensor to be tested
- Returns
- The largest contiguous, inner-dimension size.
This function searches of the largest, common contiguous size in the ranges of two non-contiguous tensors. At a minimum, this is equal to the size of the stride-one dimension.
- Template Parameters
-
T1 The first tensor type T2 The secont tensor type
- Parameters
-
tensor1 The first tensor to be tested tensor2 The second tensor to be tested
- Returns
- The largest common, contiguous inner-dimension size of the two tensors.
Definition at line 260 of file utility.h.

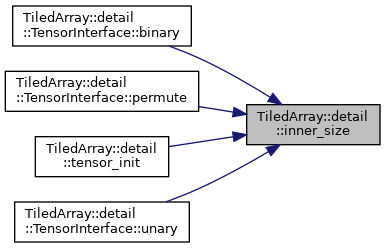
◆ inner_size_helper() [1/2]
|
inline |
Get the inner size.
This function searches of the largest contiguous size in the range of a non-contiguous tensor. At a minimum, this is equal to the size of the stride-one dimension.
- Template Parameters
-
T A tensor type
- Parameters
-
tensor The tensor to be tested
- Returns
- The largest contiguous, inner-dimension size.
Definition at line 192 of file utility.h.
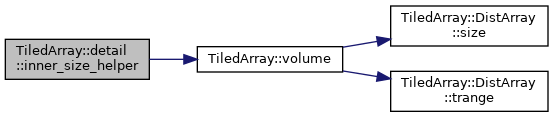
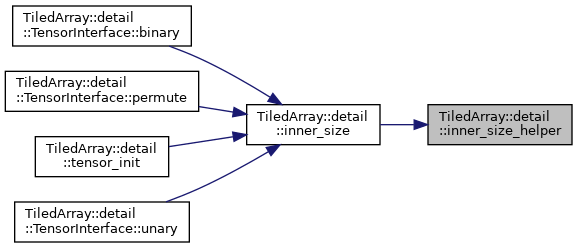
◆ inner_size_helper() [2/2]
|
inline |
Get the inner size of two tensors.
This function searches of the largest, common contiguous size in the ranges of two non-contiguous tensors. At a minimum, this is equal to the size of the stride-one dimension.
- Template Parameters
-
T1 The first tensor type T2 The secont tensor type
- Parameters
-
tensor1 The first tensor to be tested tensor2 The second tensor to be tested
- Returns
- The largest contiguous, inner-dimension size of the two tensors.
Definition at line 221 of file utility.h.

◆ inplace_tensor_op() [1/2]
|
inline |
In-place tensor permutation operations with contiguous data.
In-place tensor of tensors permutation operations with contiguous data.
This function sets the i
-th element of result
with the result of op(tensor1[i],tensors[i]...)
The expected signature of the input operations is:
The expected signature of the output operations is:
- Template Parameters
-
InputOp The input operation type OutputOp The output operation type TR The result tensor type T1 The first argument tensor type Ts The remaining argument tensor types
- Parameters
-
[in] input_op The operation that is used to generate the output value from the input arguments [in] output_op The operation that is used to set the value of the result tensor given the element pointer and the result value [in] perm The permutation applied to the argument tensors [in,out] result The result tensor [in] tensor1 The first argument tensor [in] tensors The remaining argument tensors
This function sets the i
-th element of result
with the result of op(tensor1[i], tensors[i]...)
The expected signature of the input operations is:
The expected signature of the output operations is:
- Template Parameters
-
InputOp The input operation type OutputOp The output operation type TR The result tensor type T1 The first argument tensor type Ts The remaining argument tensor types
- Parameters
-
[in] input_op The operation that is used to generate the output value from the input arguments [in] output_op The operation that is used to set the value of the result tensor given the element pointer and the result value [in] perm The permutation applied to the argument tensors [in,out] result The result tensor [in] tensor1 The first argument tensor [in] tensors The remaining argument tensors
Definition at line 262 of file kernels.h.
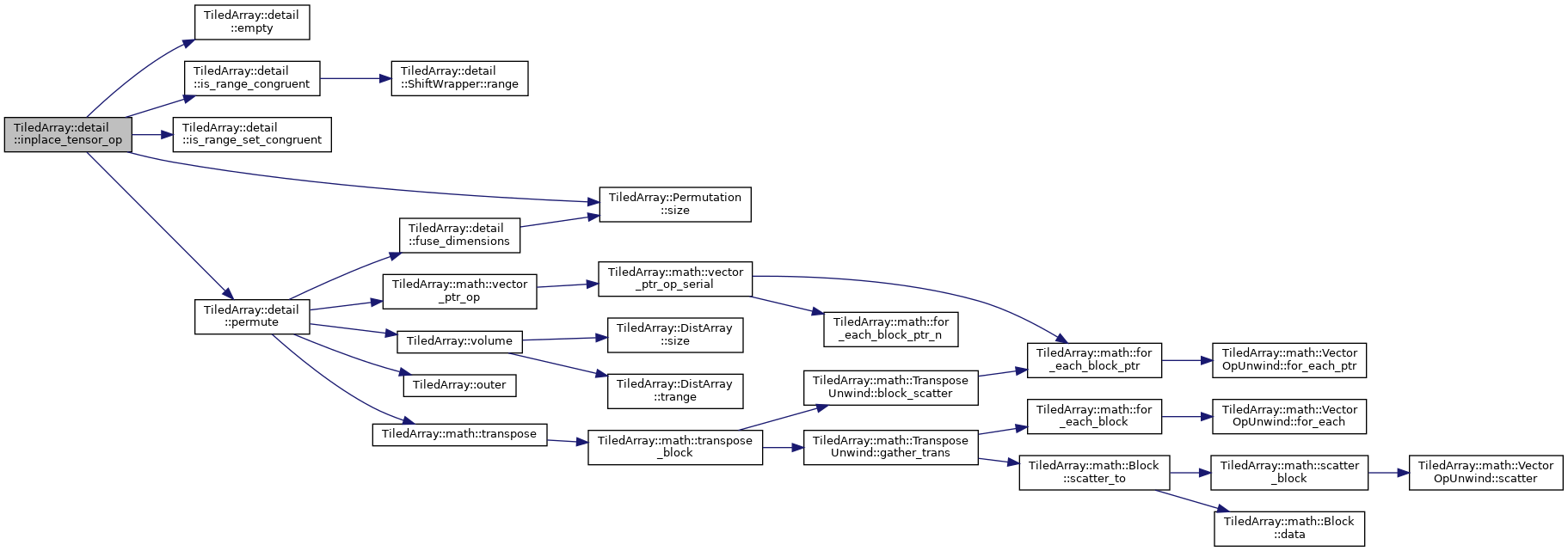
◆ inplace_tensor_op() [2/2]
|
inline |
In-place tensor operations with contiguous data.
In-place tensor of tensors operations with non-contiguous data.
In-place tensor operations with non-contiguous data.
In-place tensor of tensors operations with contiguous data.
This function sets the elements of result
with the result of op(tensors[i]...)
- Template Parameters
-
Op The element initialization operation type TR The result tensor type Ts The remaining argument tensor types
- Parameters
-
[in] op The result tensor element initialization operation [in,out] result The result tensor [in] tensors The argument tensors
This function sets the i
-th element of result
with the result of op(tensors[i]...)
- Template Parameters
-
Op The element initialization operation type TR The result tensor type Ts The remaining argument tensor types
- Parameters
-
[in] op The result tensor element initialization operation [in,out] result The result tensor [in] tensors The argument tensors
Definition at line 197 of file kernels.h.

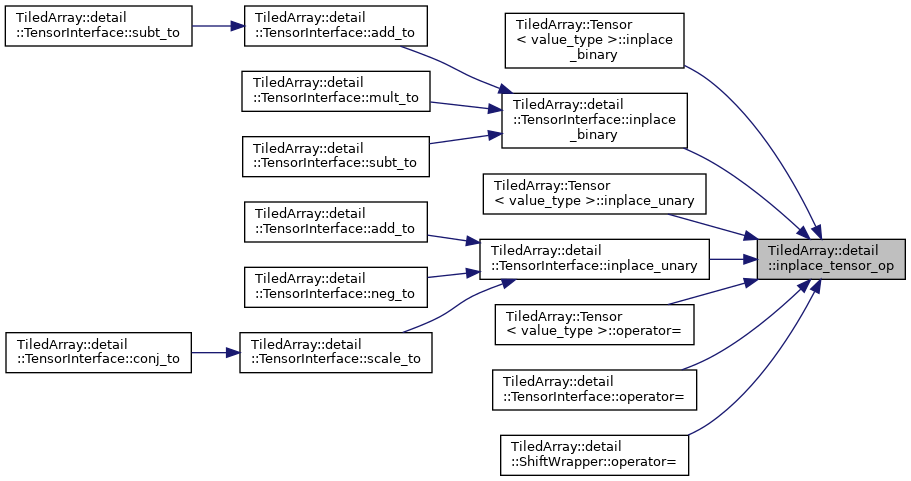
◆ is_range_congruent() [1/3]
|
inline |
Check for congruent range objects with a shifted tensor.
- Template Parameters
-
Left The left-hand tensor type Right The right-hand tensor type
- Parameters
-
left The left-hand tensor right The right-hand tensor
- Returns
true
if the lower and upper bounds of the left- and right-hand tensor ranges are equal, otherwisefalse
Definition at line 120 of file shift_wrapper.h.

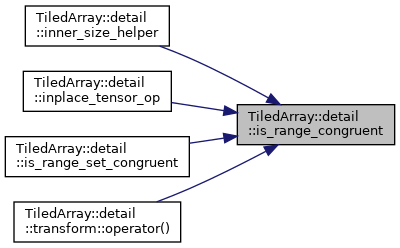
◆ is_range_congruent() [2/3]
|
inline |
Test that the ranges of a pair of tensors are congruent.
Test that the ranges of a pair of shifted tensors are congruent.
This function tests that the rank and extent of tensor1
are equal to those of tensor2
.
- Template Parameters
-
T1 The first tensor type T2 The second tensor type
- Parameters
-
tensor1 The first tensor to be compared tensor2 The second tensor to be compared
- Returns
true
if the rank and extent of the two tensors equal, otherwisefalse
.
This function tests that the extents of the two tensors are equal. One or both of the tensors may be shifted.
- Template Parameters
-
T1 The first tensor type T2 The second tensor type
- Parameters
-
tensor1 The first tensor to be compared tensor2 The second tensor to be compared
- Returns
true
if the rank and extent of the two tensors equal, otherwisefalse
.
Definition at line 76 of file utility.h.

◆ is_range_congruent() [3/3]
|
inline |
Test that the ranges of a pair of permuted tensors are congruent.
This function tests that the rank, lower bound, and upper bound of tensor1
is equal to that of the permuted range of tensor2
.
- Template Parameters
-
T1 The first tensor type T2 The second tensor type
- Parameters
-
tensor1 The first tensor to be compared tensor2 The second tensor to be compared perm The permutation to be applied to tensor2
- Returns
true
if the rank and extent of the two tensors equal, otherwisefalse
.
Definition at line 94 of file utility.h.

◆ is_range_set_congruent() [1/4]
|
inlineconstexpr |
Test that the ranges of a permuted tensor is congruent with itself.
This function is used as the termination step for the recursive is_range_set_congruent()
function, and to handle the case of a single tensor.
- Template Parameters
-
T The tensor type
- Parameters
-
perm The permutation tensor The tensor
- Returns
true
Definition at line 130 of file utility.h.
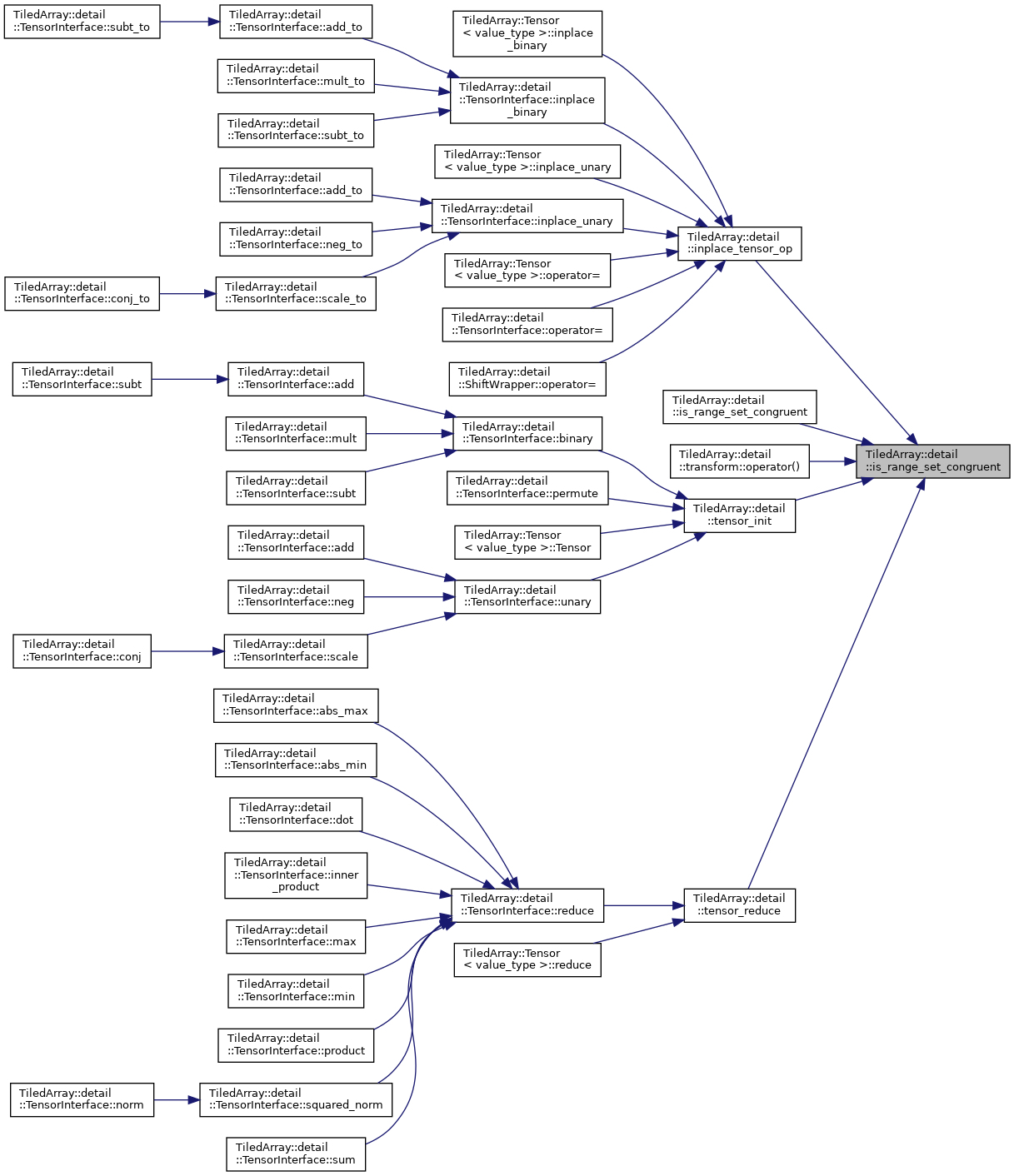
◆ is_range_set_congruent() [2/4]
|
inline |
Test that the ranges of a permuted set of tensors are congruent.
- Template Parameters
-
T1 The first tensor type T2 The second tensor type Ts The remaining tensor types
- Parameters
-
perm The permutation to be applied to tensor2
andtensors
...tensor1 The first tensor to be compared tensor2 The second tensor to be compared tensors The remaining tensor to be compared in recursive steps
- Returns
true
if all permuted tensors in the list are congruent with the first tensor in the set, otherwisefalse
.
Definition at line 147 of file utility.h.

◆ is_range_set_congruent() [3/4]
|
inlineconstexpr |
Test that the ranges of a tensor is congruent with itself.
This function is used as the termination step for the recursive is_range_set_congruent()
function, and to handle the case of a single tensor.
- Template Parameters
-
T The tensor type
- Parameters
-
tensor The tensor
- Returns
true
◆ is_range_set_congruent() [4/4]
|
inline |
Test that the ranges of a set of tensors are congruent.
- Template Parameters
-
T1 The first tensor type T2 The second tensor type Ts The remaining tensor types
- Parameters
-
tensor1 The first tensor to be compared tensor2 The second tensor to be compared tensors The remaining tensor to be compared in recursive steps
- Returns
true
if all tensors in the list are congruent with the first tensor in the set, otherwisefalse
.
Definition at line 177 of file utility.h.

◆ is_tot_index()
|
inline |
Defines what it means for a string index to be for a Tensor-of-Tensors
TiledArray defines an index as being for a tensor-of-tensors if:
- the index is valid
- the index contains a semicolon
- Parameters
-
[in] idx The index whose tensor-of-tensor-ness is in question.
- Returns
- True if
idx
is a valid tensor-of-tensor index and false otherwise.
- Exceptions
-
std::bad_alloc if is_valid_index throws while copying or splitting idx
. Strong throw guarantee.
Definition at line 160 of file annotation.h.
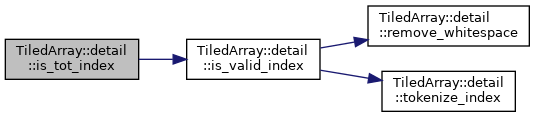

◆ is_valid_index()
|
inline |
Checks that the provided index is a valid TiledArray index
TiledArray defines a string as being a valid index if each character is one of the following:
- Roman letters A through Z (uppercase and lowercase are allowed)
- Base 10 numbers 0 through 9
- Whitespace
- underscore (
_
), prime ('
), comma (,
), or semicolon (;
)
Additionally the string can not:
- be only whitespace
- contain more than one semicolon
- have anonymous index name (i.e. can't have "i,,k" because the middle index has no name).
- Parameters
-
[in] idx The index whose validity is being questioned.
- Returns
- True if the string corresponds to a valid index and false otherwise.
- Note
- This function only tests that the characters making up the index are valid. The index may still be invalid for a particular tensor. For example if
idx
is an index for a matrix, but the actual tensor is rank 3, thenidx
would be an invalid index for that tensor despite being a valid index.
- Exceptions
-
std::bad_alloc if there is insufficient memory to copy idx
. Strong throw guarantee.std::bad_alloc if there is insufficient memory to split idx
into tokens. Strong throw guarantee.
Definition at line 122 of file annotation.h.
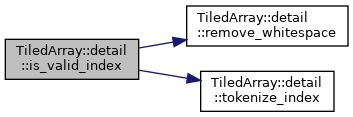

◆ make_shape()
auto TiledArray::detail::make_shape | ( | World & | world, |
const TiledArray::TiledRange & | trange | ||
) |
◆ make_shape< false >()
|
inline |
◆ make_shape< true >()
|
inline |
◆ make_ta_range() [1/2]
|
inline |
makes TiledArray::Range from a btas::RangeNd
- Parameters
-
[in] range a btas::RangeNd object
- Exceptions
-
TiledArray::Exception if range
is non-row-major
◆ make_ta_range() [2/2]
|
inline |
◆ make_tile()
Tile<T> TiledArray::detail::make_tile | ( | T && | t | ) |
◆ make_tran_it() [1/2]
UnaryTransformIterator<Iter, Op> TiledArray::detail::make_tran_it | ( | Iter | it, |
Op | op | ||
) |
Unary Transform iterator factory.
- Template Parameters
-
Iter The iterator type Op The binary transform type
- Parameters
-
it The iterator op The binary transform object
- Returns
- A unary transform iterator
Definition at line 437 of file transform_iterator.h.
◆ make_tran_it() [2/2]
BinaryTransformIterator<Iter1, Iter2, Op> TiledArray::detail::make_tran_it | ( | Iter1 | it1, |
Iter2 | it2, | ||
Op | op | ||
) |
Binary Transform iterator factory.
- Template Parameters
-
Iter1 First iterator type Iter2 Second iterator type Op The binary transform type
- Parameters
-
it1 First iterator it2 Second iterator op The binary transform object
- Returns
- A binary transform iterator
Definition at line 424 of file transform_iterator.h.
◆ norm() [1/2]
TILEDARRAY_FORCE_INLINE R TiledArray::detail::norm | ( | const R | r | ) |
Wrapper function for std::norm
This function disables the call to std::conj
for real values to prevent the result from being converted into a complex value.
- Template Parameters
-
R A real scalar type
- Parameters
-
r The real scalar
- Returns
r
Definition at line 93 of file complex.h.

◆ norm() [2/2]
TILEDARRAY_FORCE_INLINE R TiledArray::detail::norm | ( | const std::complex< R > | z | ) |
◆ operator!=() [1/3]
bool TiledArray::detail::operator!= | ( | const RangeIterator< T, Container > & | left_it, |
const RangeIterator< T, Container > & | right_it | ||
) |
Inequality operator.
Compares the iterators for inequality.
- Template Parameters
-
T The value type of the iterator Container The container that the iterator references
- Parameters
-
left_it The left-hand iterator to be compared right_it The right-hand iterator to be compared
- Returns
true
if the value or container are not equal for theleft_it
andright_it
, otherwisefalse
.
Definition at line 191 of file range_iterator.h.
◆ operator!=() [2/3]
bool TiledArray::detail::operator!= | ( | const TileConstReference< Impl > & | a, |
const TileConstReference< Impl > & | b | ||
) |
inequality operator for TileConstReference objects
Definition at line 188 of file array_impl.h.
◆ operator!=() [3/3]
bool TiledArray::detail::operator!= | ( | const TileReference< Impl > & | a, |
const TileReference< Impl > & | b | ||
) |
inequality operator for TileReference objects
Definition at line 126 of file array_impl.h.
◆ operator&()
◆ operator*() [1/7]
TILEDARRAY_FORCE_INLINE auto TiledArray::detail::operator* | ( | const ComplexConjugate< ComplexNegTag > | , |
const R | value | ||
) |
◆ operator*() [2/7]
TILEDARRAY_FORCE_INLINE auto TiledArray::detail::operator* | ( | const ComplexConjugate< L > | op, |
const R | value | ||
) |
◆ operator*() [3/7]
TILEDARRAY_FORCE_INLINE auto TiledArray::detail::operator* | ( | const ComplexConjugate< void > | , |
const R | value | ||
) |
◆ operator*() [4/7]
TILEDARRAY_FORCE_INLINE auto TiledArray::detail::operator* | ( | const L | value, |
const ComplexConjugate< ComplexNegTag > & | |||
) |
◆ operator*() [5/7]
TILEDARRAY_FORCE_INLINE auto TiledArray::detail::operator* | ( | const L | value, |
const ComplexConjugate< R > | op | ||
) |
◆ operator*() [6/7]
TILEDARRAY_FORCE_INLINE auto TiledArray::detail::operator* | ( | const L | value, |
const ComplexConjugate< void > & | |||
) |
◆ operator*() [7/7]
|
inline |
◆ operator*=() [1/3]
TILEDARRAY_FORCE_INLINE L& TiledArray::detail::operator*= | ( | L & | value, |
const ComplexConjugate< ComplexNegTag > & | |||
) |
◆ operator*=() [2/3]
TILEDARRAY_FORCE_INLINE L& TiledArray::detail::operator*= | ( | L & | value, |
const ComplexConjugate< R > | op | ||
) |
◆ operator*=() [3/3]
TILEDARRAY_FORCE_INLINE L& TiledArray::detail::operator*= | ( | L & | value, |
const ComplexConjugate< void > & | |||
) |
◆ operator<<() [1/5]
std::ostream& TiledArray::detail::operator<< | ( | std::ostream & | os, |
const Bitset< Block > & | bitset | ||
) |
◆ operator<<() [2/5]
|
inline |
◆ operator<<() [3/5]
std::ostream& TiledArray::detail::operator<< | ( | std::ostream & | os, |
const TileConstReference< Impl > & | a | ||
) |
redirect operator to std::ostream for TileConstReference objects
Definition at line 195 of file array_impl.h.

◆ operator<<() [4/5]
std::ostream& TiledArray::detail::operator<< | ( | std::ostream & | os, |
const TileReference< Impl > & | a | ||
) |
redirect operator to std::ostream for TileReference objects
Definition at line 132 of file array_impl.h.

◆ operator<<() [5/5]
|
inline |
◆ operator==() [1/4]
bool TiledArray::detail::operator== | ( | const RangeIterator< T, Container > & | left_it, |
const RangeIterator< T, Container > & | right_it | ||
) |
Equality operator.
Compares the iterators for equality. They must reference the same range object to be considered equal.
- Template Parameters
-
T The value type of the iterator Container The container that the iterator references
- Parameters
-
left_it The left-hand iterator to be compared right_it The right-hand iterator to be compared
- Returns
true
if the value and container are equal for theleft_it
andright_it
, otherwisefalse
.
Definition at line 175 of file range_iterator.h.

◆ operator==() [2/4]
bool TiledArray::detail::operator== | ( | const TensorInterface< T, Range, OpResult > & | first, |
const TensorInterface< T, Range, OpResult > & | second | ||
) |
Shallow comparison operator.
- Returns
- true if
first
andsecond
view the same data block through equivalent ranges
Definition at line 1143 of file tensor_interface.h.
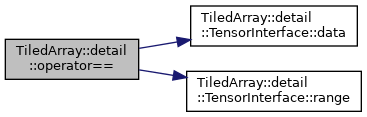
◆ operator==() [3/4]
bool TiledArray::detail::operator== | ( | const TileConstReference< Impl > & | a, |
const TileConstReference< Impl > & | b | ||
) |
comparison operator for TileConstReference objects
Definition at line 181 of file array_impl.h.

◆ operator==() [4/4]
bool TiledArray::detail::operator== | ( | const TileReference< Impl > & | a, |
const TileReference< Impl > & | b | ||
) |
comparison operator for TileReference objects
Definition at line 120 of file array_impl.h.

◆ operator^()
◆ operator|()
◆ permute()
|
inline |
Construct a permuted tensor copy.
The expected signature of the input operations is:
The expected signature of the output operations is:
- Template Parameters
-
InputOp The input operation type OutputOp The output operation type Result The result tensor type Arg0 The first tensor argument type Args The remaining tensor argument types
- Parameters
-
input_op The operation that is used to generate the output value from the input arguments output_op The operation that is used to set the value of the result tensor given the element pointer and the result value args The data pointers of the tensors to be permuted perm The permutation that will be applied to the copy
Definition at line 117 of file permute.h.
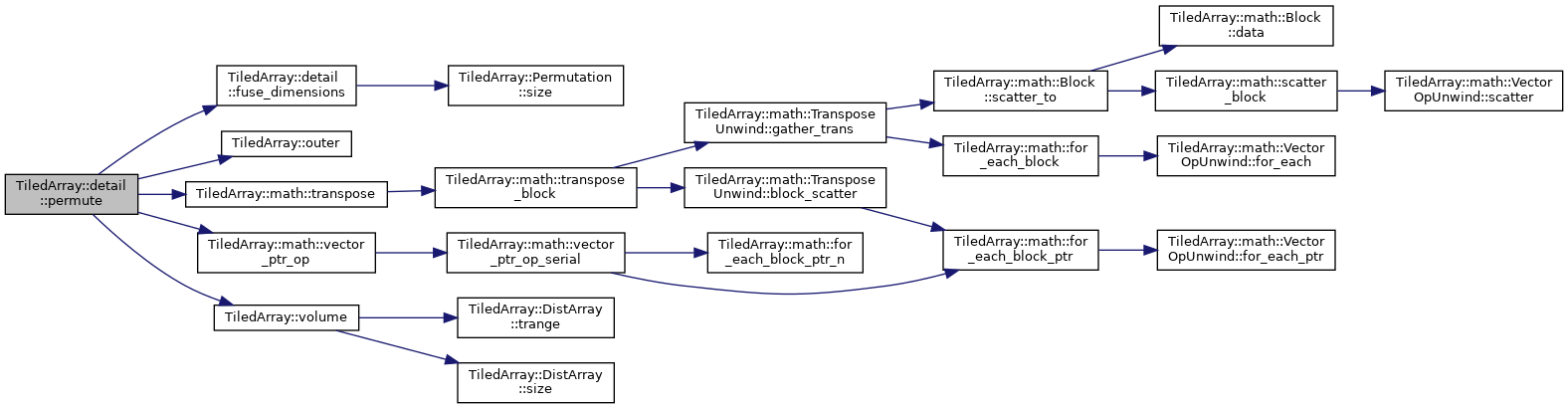
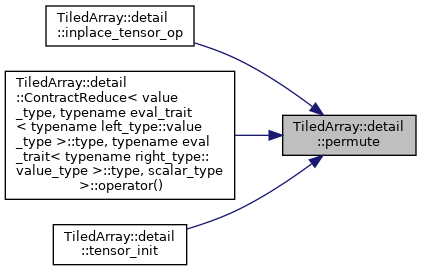
◆ permute_array()
|
inline |
Create a permuted copy of an array.
- Template Parameters
-
Perm The permutation type Arg The input array type Result The output array type
- Parameters
-
[in] perm The permutation [in] arg The input array to be permuted [out] result The output array that will hold the permuted array
Definition at line 66 of file permutation.h.
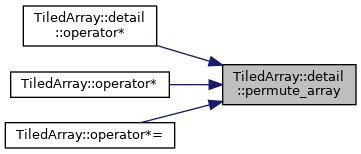
◆ prepend_dim_to_trange()
|
inline |
prepends an extra dimension to a TRange
The extra dimension will be the leading dimension, and will be blocked by block_size
- Parameters
-
array_rank extent of the leading dimension of the result array_trange the base trange block_size blocking range for the new dimension, the dimension being fused
- Returns
- TiledRange of fused Array object
make the new TiledRange1 for new dimension
make the new range for N+1 Array
Definition at line 24 of file vector_of_arrays.h.


◆ print_array() [1/2]
|
inline |
◆ print_array() [2/2]
|
inline |
◆ remove_whitespace()
|
inline |
This function removes all whitespace characters from a string.
- Parameters
-
[in] s The string we are removing whitespace from.
- Returns
s
, but without whitespace.
- Exceptions
-
none No throw guarantee.
Definition at line 52 of file annotation.h.

◆ split_index()
|
inline |
Splits and sanitizes a string labeling a tensor's modes.
This function encapsulates TiledArray's string index parsing. It is a free function to facilitate usage outside the BipartiteIndexList class. This function will take a string and separate it into the individual mode labels. The API is designed so that split_index
can be used with tensors-of-tensors as well as normal, non-nested tensors. By convention, tokenized indices of normal tensors are returned as "outer" indices. The resulting indices will be stripped of all whitespace to facilitate string comparisons.
- Note
- This function will ensure that
idx
is a valid string label. This entails requiring thatis_valid_index(idx)
is true. It does not take into the rank and/or partitioning of the tensor being labeled, i.e., it is the caller's responsibility to make sure the index makes sense for the tensor being labeled.
- Parameters
-
[in] idx The string label that should be tokenized
- Returns
- An std::pair such that the first element is a vector containing the tokenized outer indices and the second element of the std::pair is a std::vector with the tokenized inner indices. Inner indices will be an empty std::vector if
idx
is not a tensor-of-tensor index.
- Exceptions
-
TiledArray::Exception if idx
is not a valid string labeling. Strong throw guarantee.std::bad_alloc if there is insufficient memory to copy idx
or to create the returns. Strong throw guarantee.
Definition at line 191 of file annotation.h.

◆ subshape_from_fused_tile() [1/2]
|
inline |
extracts the shape of a subarray of a fused array created with fuse_vector_of_arrays
- Parameters
-
[in] fused_array a DistArray created with fuse_vector_of_arrays [in] i the index of the subarray whose Shape will be extracted (i.e. the index of the corresponding tile of the leading dimension) [in] split_trange TiledRange of the target subarray objct
- Returns
- the Shape of the
i
-th subarray
Definition at line 212 of file vector_of_arrays.h.
◆ subshape_from_fused_tile() [2/2]
|
inline |
extracts the shape of a slice of a fused array created with fuse_vector_of_arrays
- Parameters
-
[in] split_trange the TiledRange object of each "slice" array that was fused via fuse_vector_of_arrays [in] shape the shape of a DistArray created with fuse_vector_of_arrays [in] tile_idx the tile index of the leading mode that will be sliced off [in] split_ntiles the number of tiles in each "slice" array that was fused via fuse_vector_of_arrays [in] tile_size the size of the tile of the leading dimension of the fused array
- Returns
- the Shape of the
i
-th subarray
Definition at line 180 of file vector_of_arrays.h.
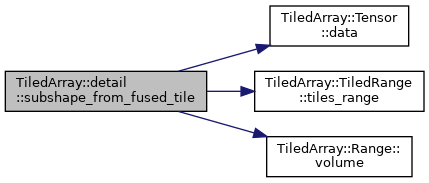

◆ swap()
◆ tensor_init() [1/3]
|
inline |
Initialize tensor with permuted tensor arguments.
Initialize tensor of tensors with permuted tensor arguments.
This function initializes the i
-th element of result
with the result of op(tensor1[i], tensors[i]...)
- Precondition
- The memory of
result
has been allocated but not initialized.
- Template Parameters
-
Op The element initialization operation type TR The result tensor type T1 The first argument tensor type Ts The argument tensor types
- Parameters
-
[in] op The result tensor element initialization operation [in] perm The permutation that will be applied to tensor2 [out] result The result tensor [in] tensor1 The first argument tensor [in] tensors The argument tensors
This function initializes the i
-th element of result
with the result of op(tensor1[i], tensors[i]...)
- Precondition
- The memory of
result
has been allocated but not initialized.
- Template Parameters
-
Op The element initialization operation type Perm A permutation type TR The result tensor type T1 The first argument tensor type Ts The argument tensor types
- Parameters
-
[in] op The result tensor element initialization operation [out] result The result tensor [in] tensor1 The first argument tensor [in] tensors The argument tensors
Definition at line 488 of file kernels.h.
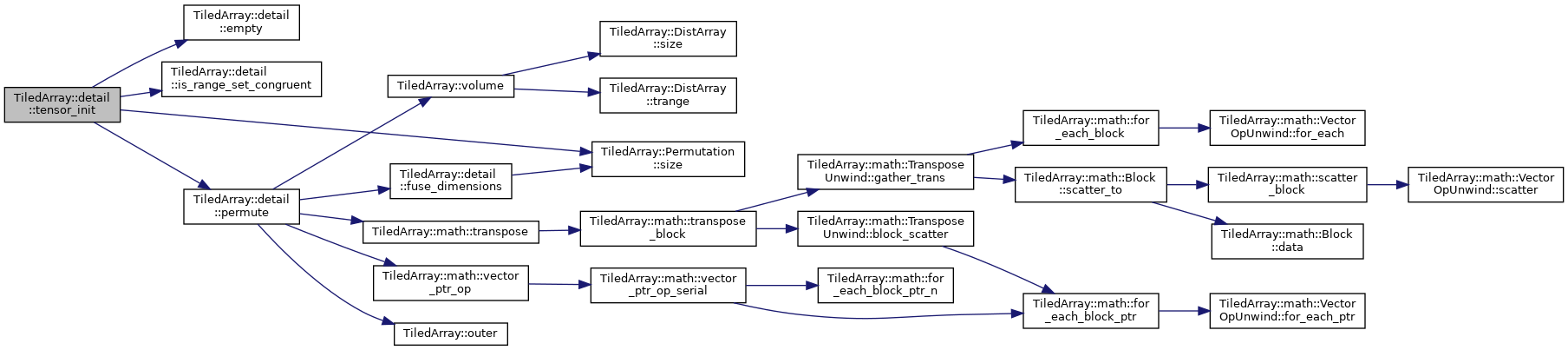
◆ tensor_init() [2/3]
|
inline |
Initialize tensor with one or more non-contiguous tensor arguments.
This function initializes the i
-th element of result
with the result of op(tensor1[i], tensors[i]...)
- Precondition
- The memory of
tensor1
has been allocated but not initialized.
- Template Parameters
-
Op The element initialization operation type T1 The result tensor type Ts The argument tensor types
- Parameters
-
[in] op The result tensor element initialization operation [out] result The result tensor [in] tensor1 The first argument tensor [in] tensors The argument tensors
This function initializes the i
-th element of result
with the result of op(tensor1[i],tensors[i]...)
- Precondition
- The memory of
tensor1
has been allocated but not initialized.
- Template Parameters
-
Op The element initialization operation type T1 The result tensor type Ts The argument tensor types
- Parameters
-
[in] op The result tensor element initialization operation [out] result The result tensor [in] tensor1 The first argument tensor [in] tensors The argument tensors
Definition at line 567 of file kernels.h.
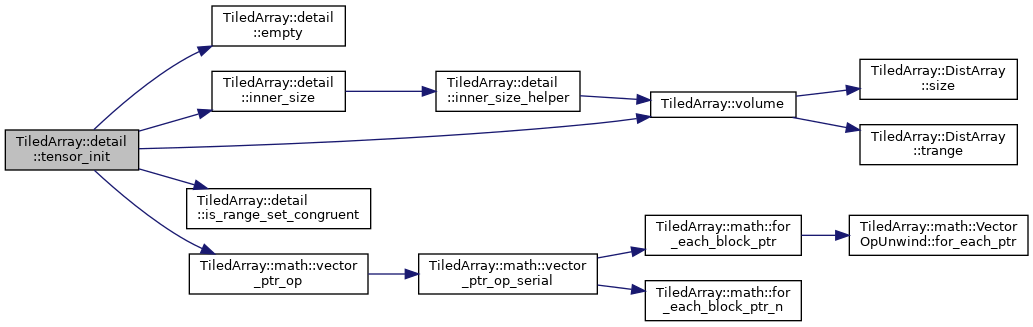
◆ tensor_init() [3/3]
|
inline |
Initialize tensor with contiguous tensor arguments.
Initialize tensor of tensors with contiguous tensor arguments.
This function initializes the i
-th element of result
with the result of op(tensors[i]...)
- Precondition
- The memory of
tensor1
has been allocated but not initialized.
- Template Parameters
-
Op The element initialization operation type TR The result tensor type Ts The argument tensor types
- Parameters
-
[in] op The result tensor element initialization operation [out] result The result tensor [in] tensors The argument tensors
Definition at line 421 of file kernels.h.
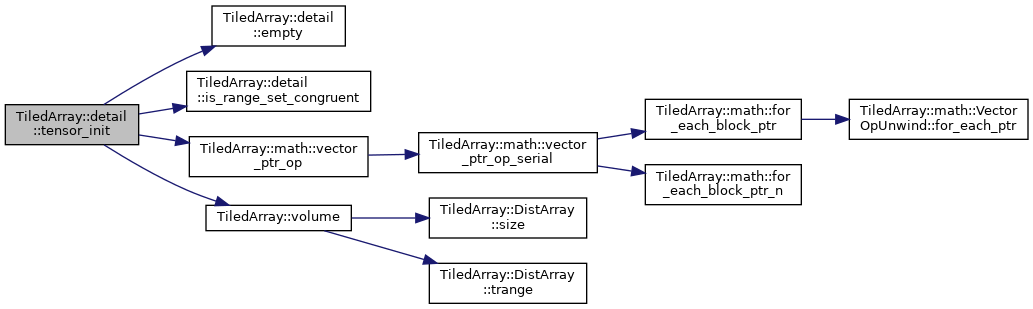
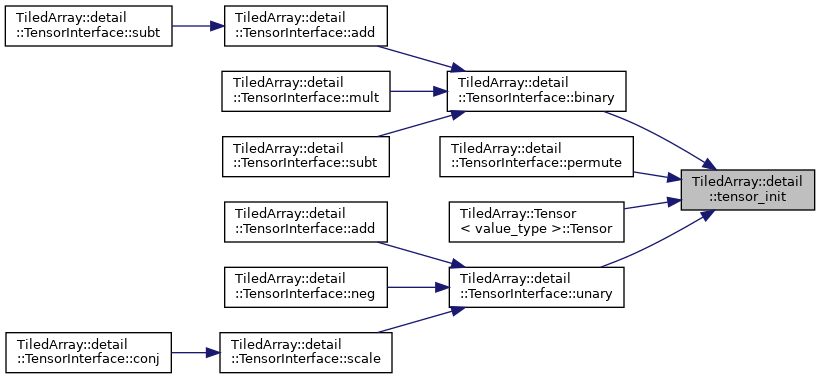
◆ tensor_op() [1/2]
|
inline |
Tensor permutation operations with contiguous data.
This function transforms argument tensors applying a callable directly (i.e., tensor-wise as result=op(perm,tensor1,tensors...)
), or by lowering to the elements (i.e., element-wise as result
[i]=op(perm,tensor1[i],tensors[i]...) )
- Template Parameters
-
TR The tensor result type Op A callable used to produce TR when called with the argument tensors, or produce TR's elements when called with the argument tensor's elements T1 The result tensor type Ts The argument tensor types
- Parameters
-
[in] op The operation that is used to compute the result value from the input arguments [in] perm The permutation applied to the argument tensors [in] tensor1 The first argument tensor [in] tensors The remaining argument tensors
◆ tensor_op() [2/2]
|
inline |
Tensor operations with contiguous data.
This function transforms argument tensors applying a callable directly (i.e., tensor-wise as result=op(tensor1,tensors...)
), or by lowering to the elements (i.e., element-wise as result
[i]=op(tensor1[i],tensors[i]...) )
- Template Parameters
-
TR The tensor result type Op A callable used to produce TR when called with the argument tensors, or produce TR's elements when called with the argument tensor's elements T1 The first argument tensor type Ts The remaining argument tensor types
- Parameters
-
op The result tensor element initialization operation tensor1 The first argument tensor tensors The remaining argument tensors
◆ tensor_reduce()
Scalar TiledArray::detail::tensor_reduce | ( | ReduceOp && | reduce_op, |
JoinOp && | join_op, | ||
Scalar | identity, | ||
const T1 & | tensor1, | ||
const Ts &... | tensors | ||
) |
Reduction operation for contiguous tensors.
Reduction operation for non-contiguous tensors of tensors.
Reduction operation for non-contiguous tensors.
Reduction operation for contiguous tensors of tensors.
Reduction operation for tensors.
Perform an element-wise reduction of the tensors by executing join_op(result, reduce_op(result, &tensor1[i], &tensors[i]...))
for each i
in the index range of tensor1
. result
is initialized to identity
. If HAVE_INTEL_TBB is defined, the reduction will be executed in an undefined order, otherwise will execute in the order of increasing i
.
- Template Parameters
-
ReduceOp The element-wise reduction operation type JoinOp The result operation type Scalar A scalar type T1 The first argument tensor type Ts The argument tensor types
- Parameters
-
reduce_op The element-wise reduction operation identity The initial value for the reduction and the result tensor1 The first tensor to be reduced tensors The other tensors to be reduced
- Returns
- The reduced value of the tensor(s)
Perform tensor-wise reduction of the tensors by executing reduce_op(result, &tensor1, &tensors...)
. result
is initialized to identity
.
- Template Parameters
-
ReduceOp The tensor-wise reduction operation type JoinOp The result operation type Scalar A scalar type T1 The first argument tensor type Ts The argument tensor types
- Parameters
-
reduce_op The element-wise reduction operation identity The initial value for the reduction and the result tensor1 The first tensor to be reduced tensors The other tensors to be reduced
- Returns
- The reduced value of the tensor(s)
Perform reduction of the tensor-of-tensors' elements by executing join_op(result, reduce_op(tensor1[i], tensors[i]...))
for each i
in the index range of tensor1
. result
is initialized to identity
. This will execute serially, in the order of increasing i
(each element's reduction can however be executed in parallel, depending on the element type).
- Template Parameters
-
ReduceOp The tensor-wise reduction operation type JoinOp The result operation type Scalar A scalar type T1 The first argument tensor type Ts The argument tensor types
- Parameters
-
reduce_op The element-wise reduction operation join_op The result join operation identity The initial value for the reduction and the result tensor1 The first tensor to be reduced tensors The other tensors to be reduced
- Returns
- The reduced value of the tensor(s)
Perform an element-wise reduction of the tensors by executing join_op(result, reduce_op(tensor1[i], tensors[i]...))
for each i
in the index range of tensor1
. result
is initialized to identity
. This will execute serially, in the order of increasing i
(each element-wise reduction can however be executed in parallel, depending on the element type).
- Template Parameters
-
ReduceOp The element-wise reduction operation type JoinOp The result operation type Scalar A scalar type T1 The first argument tensor type Ts The argument tensor types
- Parameters
-
reduce_op The element-wise reduction operation join_op The result join operation identity The initial value for the reduction and the result tensor1 The first tensor to be reduced tensors The other tensors to be reduced
- Returns
- The reduced value of the tensor(s)
Definition at line 665 of file kernels.h.
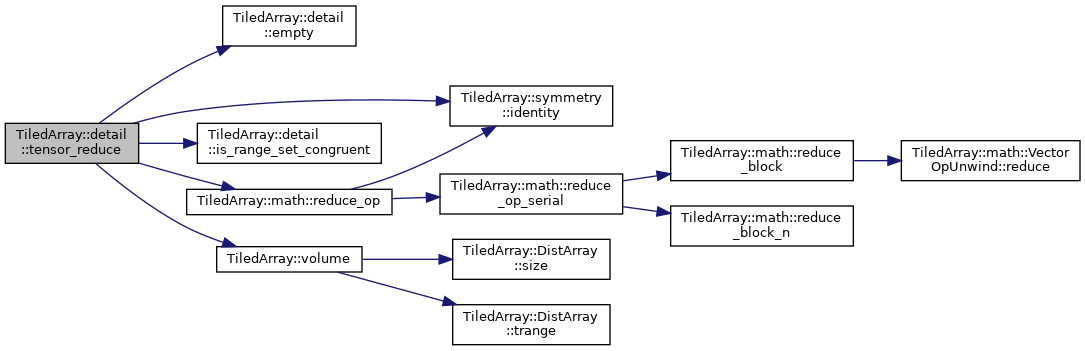
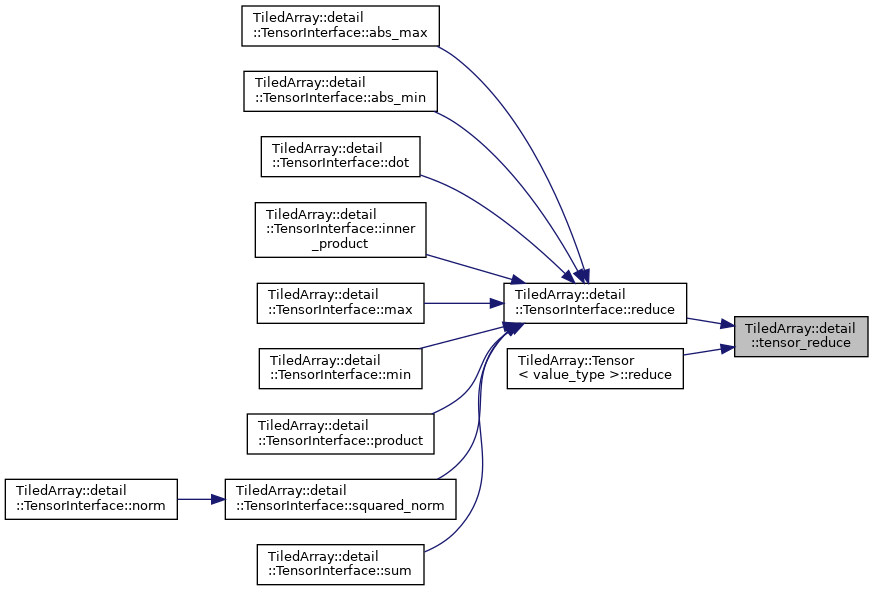
◆ tokenize_index()
|
inline |
Splits a string into tokens based on a character delimiter
This function assumes that the input string can be considered a series of delimiter separated tokens. It will split the string into tokens and return an std::vector of the tokens. This function does no additional string processing (such as removing spaces).
It's worth noting several edge cases:
- If
s
is an empty string the result vector will contain a single empty string. - If
s
starts/ends with a delimiter the result vector will start/end with an empty string - Adjacent delimiters are tokenized as delimiting the empty string
Downstream error checking relies on this edge case behavior.
- Parameters
-
[in] s The string we are splitting into tokens. [in] delim The character used to delimit tokens
- Returns
- A vector containing the tokens
- Exceptions
-
std::bad_alloc if there is insufficient memory to allocate the vector which will hold the return. Strong throw guarantee. std::bad_alloc if there is insufficient memory to allocate the tokens in the return. Strong throw guarantee.
Definition at line 81 of file annotation.h.

◆ write_diag_tiles_to_array_rng()
std::enable_if_t<is_iterator<RandomAccessIterator>::value, void> TiledArray::detail::write_diag_tiles_to_array_rng | ( | Array & | A, |
RandomAccessIterator | diagonals_begin | ||
) |
Writes tiles of a nonconstant diagonal array.
- Template Parameters
-
Array a DistArray type RandomAccessIterator an iterator over the range of diagonal elements
- Parameters
-
[in] A an Array object [in] diagonals_begin the begin iterator of the range of the diagonals
Definition at line 194 of file diagonal_array.h.
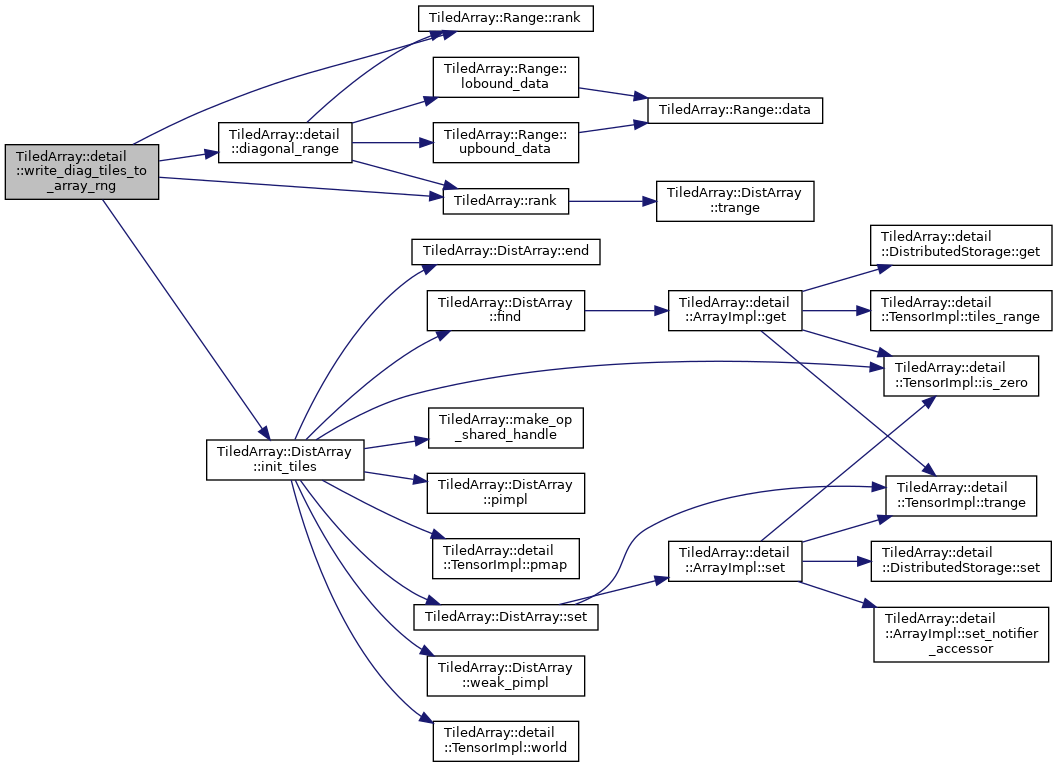
◆ write_diag_tiles_to_array_val()
void TiledArray::detail::write_diag_tiles_to_array_val | ( | Array & | A, |
T | val | ||
) |
Writes tiles of a constant diagonal array.
- Template Parameters
-
Array a DistArray type T a numeric type
- Parameters
-
[in] A an Array object [in] val the value of the diagonal elements of A
Definition at line 161 of file diagonal_array.h.
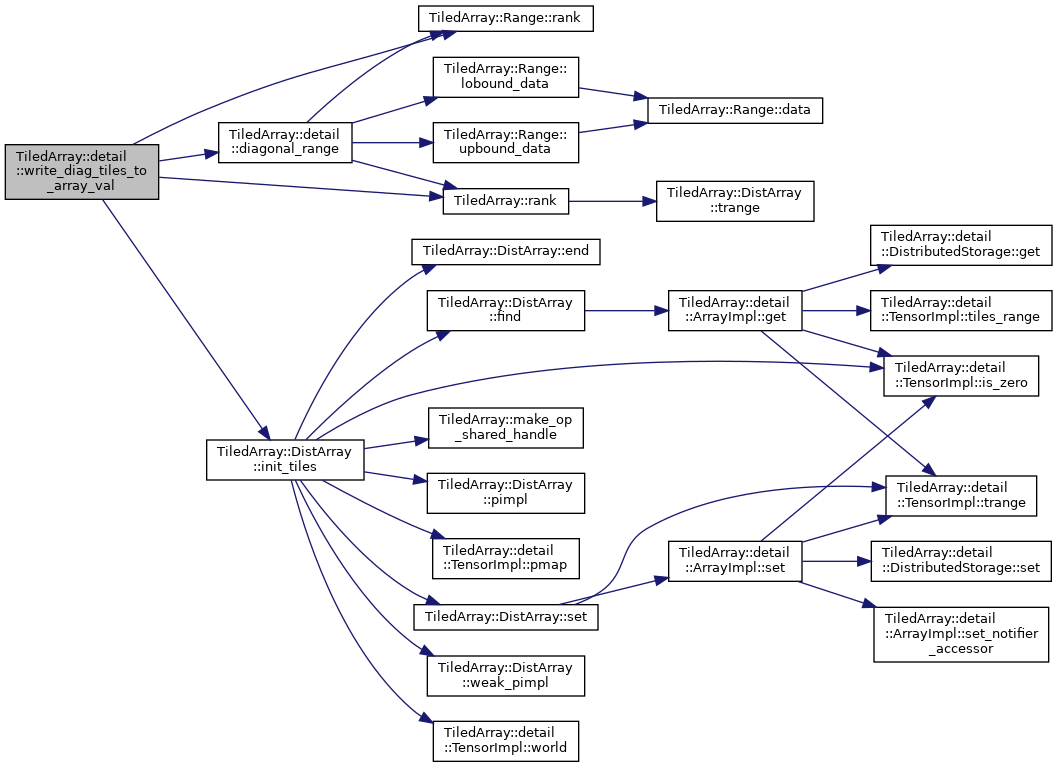

Variable Documentation
◆ has_conversion_operator_v
|
constexpr |
has_conversion_operator_v<From, To>
is an alias for has_conversion_operator<From, To>::value
Definition at line 496 of file type_traits.h.
◆ is_array_tile_v
|
constexpr |
is_array_tile_v<T>
is an alias for is_array_tile<T>::value
Definition at line 678 of file type_traits.h.
◆ is_boost_gettable_v
|
constexpr |
Definition at line 851 of file type_traits.h.
◆ is_btas_tensor_v
|
constexpr |
◆ is_complete_type_v
|
constexpr |
- Template Parameters
-
T a type is_complete_type_v<T>
is an alias foris_complete_type<T>::value
Definition at line 412 of file type_traits.h.
◆ is_complex_v
|
constexpr |
is_complex_v<T>
is an alias for is_complex<T>::value
Definition at line 632 of file type_traits.h.
◆ is_contiguous_tensor_v
|
constexpr |
- Template Parameters
-
Ts a parameter pack is_contiguous_tensor_v<Ts...>
is an alias foris_contiguous_tensor<Ts...>::value
Definition at line 211 of file type_traits.h.
◆ is_convertible_v
|
constexpr |
is_convertible_v<From, To>
is an alias for is_convertible<From
, To>::value
Definition at line 537 of file type_traits.h.
◆ is_explicitly_convertible_v
|
constexpr |
is_explicitly_convertible_v<From, To>
is an alias for is_explicitly_convertible<From, To>::value
Definition at line 508 of file type_traits.h.
◆ is_gettable_pair_v
|
constexpr |
is_gettable_pair_v<T>
is an alias for is_gettable_pair<T>::value
Definition at line 888 of file type_traits.h.
◆ is_gettable_v
|
constexpr |
Definition at line 854 of file type_traits.h.
◆ is_implicitly_convertible_v
|
constexpr |
is_implicitly_convertible_v<From, To>
is an alias for is_implicitly_convertible<From, To>::value
Definition at line 521 of file type_traits.h.
◆ is_integral_list_v
|
constexpr |
is_integral_list_v<T>
is an alias for is_integral_list<T>::value
Definition at line 812 of file type_traits.h.
◆ is_integral_pair_list_v
|
constexpr |
is_integral_pair_list_v<T>
is an alias for is_integral_pair_list<T>::value
Definition at line 939 of file type_traits.h.
◆ is_integral_pair_v
|
constexpr |
is_integral_pair_v<T>
is an alias for is_integral_pair<T>::value
Definition at line 909 of file type_traits.h.
◆ is_integral_tuple_v
|
constexpr |
is_integral_tuple_v<T>
is an alias for is_integral_tuple<T>::value
Definition at line 952 of file type_traits.h.
◆ is_non_array_lazy_tile_v
|
constexpr |
is_non_array_lazy_tile_v<T>
is an alias for is_non_array_lazy_tile<T>::value
Definition at line 696 of file type_traits.h.
◆ is_numeric_v
|
constexpr |
is_numeric_v<T>
is an alias for is_numeric<T>::value
Definition at line 645 of file type_traits.h.
◆ is_pair_v
|
constexpr |
is_pair_v<T>
is an alias for is_pair<T>::value
Definition at line 1203 of file type_traits.h.
◆ is_reduce_op_v
|
constexpr |
Definition at line 254 of file type_traits.h.
◆ is_scalar_v
|
constexpr |
is_scalar_v<T>
is an alias for is_scalar_v<T>
Definition at line 659 of file type_traits.h.
◆ is_shifted_v
|
constexpr |
- Template Parameters
-
Ts a parameter pack is_shifted_v<Ts...>
is an alias foris_shifted<Ts...>::value
Definition at line 240 of file type_traits.h.
◆ is_std_gettable_v
|
constexpr |
Definition at line 840 of file type_traits.h.
◆ is_strictly_ordered_v
|
constexpr |
is_strictly_ordered_v<T>
is an alias for is_strictly_ordered<T>::value
Definition at line 786 of file type_traits.h.
◆ is_ta_tensor_v
|
constexpr |
Definition at line 164 of file type_traits.h.
◆ is_tensor_of_tensor_v
|
constexpr |
- Template Parameters
-
Ts a parameter pack is_tensor_of_tensor_v<Ts...>
is an alias foris_tensor_of_tensor<Ts...>::value
Definition at line 155 of file type_traits.h.
◆ is_tensor_v
|
constexpr |
- Template Parameters
-
Ts a parameter pack is_tensor_v<Ts...>
is an alias foris_tensor<Ts...>::value
Definition at line 135 of file type_traits.h.
◆ is_tuple_v
|
constexpr |
is_tuple_v<T>
is an alias for is_tuple<T>::value
Definition at line 826 of file type_traits.h.
◆ is_type_v
|
constexpr |
- Template Parameters
-
T a type is_type_v<T>
is an alias foris_type<T>::value
Definition at line 393 of file type_traits.h.
◆ trace_is_defined_v
|
constexpr |
Helper variable for determining if the trace operation is defined for a tile of type T
This global variable is provided as a convenience for checking the value of TraceIsDefined<T>::value
.
- Template Parameters
-
T The type of the tile we are trying to take the trace of.