Documentation
Classes | |
struct | TiledArray::eval_trait< T > |
Determine the object type used in the evaluation of tensor expressions. More... | |
struct | TiledArray::is_lazy_tile< T > |
Detect lazy evaluation tiles. More... | |
struct | TiledArray::is_lazy_tile< DistArray< Tile, Policy > > |
struct | TiledArray::is_consumable_tile< T > |
Consumable tile type trait. More... | |
struct | TiledArray::is_consumable_tile< ZeroTensor > |
Functions | |
template<typename Arg > | |
Tile< Arg > | TiledArray::clone (const Tile< Arg > &arg) |
Create a copy of arg . More... | |
template<typename Arg > | |
bool | TiledArray::empty (const Tile< Arg > &arg) |
Check that arg is empty (no data) More... | |
template<typename Arg , typename Perm , typename = std::enable_if_t<detail::is_permutation_v<Perm>>> | |
decltype(auto) | TiledArray::permute (const Tile< Arg > &arg, const Perm &perm) |
Create a permuted copy of arg . More... | |
template<typename Arg , typename Index , typename = std::enable_if_t<detail::is_integral_range_v<Index>>> | |
decltype(auto) | TiledArray::shift (const Tile< Arg > &arg, const Index &range_shift) |
Shift the range of arg . More... | |
template<typename Arg , typename Index , typename = std::enable_if_t<std::is_integral_v<Index>>> | |
decltype(auto) | TiledArray::shift (const Tile< Arg > &arg, const std::initializer_list< Index > &range_shift) |
Shift the range of arg . More... | |
template<typename Arg , typename Index , typename = std::enable_if_t<detail::is_integral_range_v<Index>>> | |
Tile< Arg > & | TiledArray::shift_to (Tile< Arg > &arg, const Index &range_shift) |
Shift the range of arg in place. More... | |
template<typename Arg , typename Index , typename = std::enable_if_t<std::is_integral_v<Index>>> | |
Tile< Arg > & | TiledArray::shift_to (Tile< Arg > &arg, const std::initializer_list< Index > &range_shift) |
Shift the range of arg in place. More... | |
template<typename Left , typename Right > | |
decltype(auto) | TiledArray::add (const Tile< Left > &left, const Tile< Right > &right) |
Add tile arguments. More... | |
template<typename Left , typename Right , typename Scalar , typename std::enable_if< detail::is_numeric_v< Scalar >>::type * = nullptr> | |
decltype(auto) | TiledArray::add (const Tile< Left > &left, const Tile< Right > &right, const Scalar factor) |
Add and scale tile arguments. More... | |
template<typename Left , typename Right , typename Perm , typename = std::enable_if_t<detail::is_permutation_v<Perm>>> | |
decltype(auto) | TiledArray::add (const Tile< Left > &left, const Tile< Right > &right, const Perm &perm) |
Add and permute tile arguments. More... | |
template<typename Left , typename Right , typename Scalar , typename Perm , typename std::enable_if< detail::is_numeric_v< Scalar > &&detail::is_permutation_v< Perm >>::type * = nullptr> | |
decltype(auto) | TiledArray::add (const Tile< Left > &left, const Tile< Right > &right, const Scalar factor, const Perm &perm) |
Add, scale, and permute tile arguments. More... | |
template<typename Arg , typename Scalar , typename std::enable_if< detail::is_numeric_v< Scalar >>::type * = nullptr> | |
decltype(auto) | TiledArray::add (const Tile< Arg > &arg, const Scalar value) |
Add a constant scalar to tile argument. More... | |
template<typename Arg , typename Scalar , typename Perm , typename std::enable_if< detail::is_numeric_v< Scalar > &&detail::is_permutation_v< Perm >>::type * = nullptr> | |
decltype(auto) | TiledArray::add (const Tile< Arg > &arg, const Scalar value, const Perm &perm) |
Add a constant scalar and permute tile argument. More... | |
template<typename Result , typename Arg > | |
Tile< Result > & | TiledArray::add_to (Tile< Result > &result, const Tile< Arg > &arg) |
Add to the result tile. More... | |
template<typename Result , typename Arg , typename Scalar , typename std::enable_if< detail::is_numeric_v< Scalar >>::type * = nullptr> | |
Tile< Result > & | TiledArray::add_to (Tile< Result > &result, const Tile< Arg > &arg, const Scalar factor) |
Add and scale to the result tile. More... | |
template<typename Result , typename Scalar , typename std::enable_if< detail::is_numeric_v< Scalar >>::type * = nullptr> | |
Tile< Result > & | TiledArray::add_to (Tile< Result > &result, const Scalar value) |
Add constant scalar to the result tile. More... | |
template<typename Left , typename Right > | |
decltype(auto) | TiledArray::subt (const Tile< Left > &left, const Tile< Right > &right) |
Subtract tile arguments. More... | |
template<typename Left , typename Right , typename Scalar , typename std::enable_if< detail::is_numeric_v< Scalar >>::type * = nullptr> | |
decltype(auto) | TiledArray::subt (const Tile< Left > &left, const Tile< Right > &right, const Scalar factor) |
Subtract and scale tile arguments. More... | |
template<typename Left , typename Right , typename Perm , typename = std::enable_if_t<detail::is_permutation_v<Perm>>> | |
decltype(auto) | TiledArray::subt (const Tile< Left > &left, const Tile< Right > &right, const Perm &perm) |
Subtract and permute tile arguments. More... | |
template<typename Left , typename Right , typename Scalar , typename Perm , typename std::enable_if< detail::is_numeric_v< Scalar > &&detail::is_permutation_v< Perm >>::type * = nullptr> | |
decltype(auto) | TiledArray::subt (const Tile< Left > &left, const Tile< Right > &right, const Scalar factor, const Perm &perm) |
Subtract, scale, and permute tile arguments. More... | |
template<typename Arg , typename Scalar , typename std::enable_if< detail::is_numeric_v< Scalar >>::type * = nullptr> | |
decltype(auto) | TiledArray::subt (const Tile< Arg > &arg, const Scalar value) |
Subtract a scalar constant from the tile argument. More... | |
template<typename Arg , typename Scalar , typename Perm , typename std::enable_if< detail::is_numeric_v< Scalar > &&detail::is_permutation_v< Perm >>::type * = nullptr> | |
decltype(auto) | TiledArray::subt (const Tile< Arg > &arg, const Scalar value, const Perm &perm) |
Subtract a constant scalar and permute tile argument. More... | |
template<typename Result , typename Arg > | |
Tile< Result > & | TiledArray::subt_to (Tile< Result > &result, const Tile< Arg > &arg) |
Subtract from the result tile. More... | |
template<typename Result , typename Arg , typename Scalar , typename std::enable_if< detail::is_numeric_v< Scalar >>::type * = nullptr> | |
Tile< Result > & | TiledArray::subt_to (Tile< Result > &result, const Tile< Arg > &arg, const Scalar factor) |
Subtract and scale from the result tile. More... | |
template<typename Result , typename Scalar , typename std::enable_if< detail::is_numeric_v< Scalar >>::type * = nullptr> | |
Tile< Result > & | TiledArray::subt_to (Tile< Result > &result, const Scalar value) |
Subtract constant scalar from the result tile. More... | |
template<typename Left , typename Right > | |
decltype(auto) | TiledArray::mult (const Tile< Left > &left, const Tile< Right > &right) |
Multiplication tile arguments. More... | |
template<typename Left , typename Right , typename Scalar , typename std::enable_if< detail::is_numeric_v< Scalar >>::type * = nullptr> | |
decltype(auto) | TiledArray::mult (const Tile< Left > &left, const Tile< Right > &right, const Scalar factor) |
Multiplication and scale tile arguments. More... | |
template<typename Left , typename Right , typename Perm , typename = std::enable_if_t<detail::is_permutation_v<Perm>>> | |
decltype(auto) | TiledArray::mult (const Tile< Left > &left, const Tile< Right > &right, const Perm &perm) |
Multiplication and permute tile arguments. More... | |
template<typename Left , typename Right , typename Scalar , typename Perm , typename std::enable_if< detail::is_numeric_v< Scalar > &&detail::is_permutation_v< Perm >>::type * = nullptr> | |
decltype(auto) | TiledArray::mult (const Tile< Left > &left, const Tile< Right > &right, const Scalar factor, const Perm &perm) |
Multiplication, scale, and permute tile arguments. More... | |
template<typename Result , typename Arg > | |
Tile< Result > & | TiledArray::mult_to (Tile< Result > &result, const Tile< Arg > &arg) |
Multiply to the result tile. More... | |
template<typename Result , typename Arg , typename Scalar , typename std::enable_if< detail::is_numeric_v< Scalar >>::type * = nullptr> | |
Tile< Result > & | TiledArray::mult_to (Tile< Result > &result, const Tile< Arg > &arg, const Scalar factor) |
Multiply and scale to the result tile. More... | |
template<typename Left , typename Right , typename Op > | |
decltype(auto) | TiledArray::binary (const Tile< Left > &left, const Tile< Right > &right, Op &&op) |
Binary element-wise transform producing a new tile. More... | |
template<typename Left , typename Right , typename Op , typename Perm , typename = std::enable_if_t<detail::is_permutation_v<Perm>>> | |
decltype(auto) | TiledArray::binary (const Tile< Left > &left, const Tile< Right > &right, Op &&op, const Perm &perm) |
Binary element-wise transform producing a new tile. More... | |
template<typename Left , typename Right , typename Op > | |
Tile< Left > & | TiledArray::inplace_binary (Tile< Left > &left, const Tile< Right > &right, Op &&op) |
Binary element-wise in-place transform. More... | |
template<typename Arg , typename Scalar , typename std::enable_if< detail::is_numeric_v< Scalar >>::type * = nullptr> | |
decltype(auto) | TiledArray::scale (const Tile< Arg > &arg, const Scalar factor) |
Scalar the tile argument. More... | |
template<typename Arg , typename Scalar , typename Perm , typename std::enable_if< detail::is_numeric_v< Scalar > &&detail::is_permutation_v< Perm >>::type * = nullptr> | |
decltype(auto) | TiledArray::scale (const Tile< Arg > &arg, const Scalar factor, const Perm &perm) |
Scale and permute tile argument. More... | |
template<typename Result , typename Scalar , typename std::enable_if< detail::is_numeric_v< Scalar >>::type * = nullptr> | |
Tile< Result > & | TiledArray::scale_to (Tile< Result > &result, const Scalar factor) |
Scale to the result tile. More... | |
template<typename Arg > | |
decltype(auto) | TiledArray::neg (const Tile< Arg > &arg) |
Negate the tile argument. More... | |
template<typename Arg , typename Perm , typename = std::enable_if_t<detail::is_permutation_v<Perm>>> | |
decltype(auto) | TiledArray::neg (const Tile< Arg > &arg, const Perm &perm) |
Negate and permute tile argument. More... | |
template<typename Result > | |
Tile< Result > & | TiledArray::neg_to (Tile< Result > &result) |
In-place negate tile. More... | |
template<typename Arg > | |
decltype(auto) | TiledArray::conj (const Tile< Arg > &arg) |
Create a complex conjugated copy of a tile. More... | |
template<typename Arg , typename Scalar , typename std::enable_if< TiledArray::detail::is_numeric_v< Scalar >>::type * = nullptr> | |
decltype(auto) | TiledArray::conj (const Tile< Arg > &arg, const Scalar factor) |
Create a complex conjugated and scaled copy of a tile. More... | |
template<typename Arg , typename Perm , typename = std::enable_if_t<detail::is_permutation_v<Perm>>> | |
decltype(auto) | TiledArray::conj (const Tile< Arg > &arg, const Perm &perm) |
Create a complex conjugated and permuted copy of a tile. More... | |
template<typename Arg , typename Scalar , typename Perm , typename std::enable_if< TiledArray::detail::is_numeric_v< Scalar > &&detail::is_permutation_v< Perm >>::type * = nullptr> | |
decltype(auto) | TiledArray::conj (const Tile< Arg > &arg, const Scalar factor, const Perm &perm) |
Create a complex conjugated, scaled, and permuted copy of a tile. More... | |
template<typename Result > | |
Tile< Result > & | TiledArray::conj_to (Tile< Result > &result) |
In-place complex conjugate a tile. More... | |
template<typename Result , typename Scalar , typename std::enable_if< TiledArray::detail::is_numeric_v< Scalar >>::type * = nullptr> | |
Tile< Result > & | TiledArray::conj_to (Tile< Result > &result, const Scalar factor) |
In-place complex conjugate and scale a tile. More... | |
template<typename Arg , typename Op > | |
decltype(auto) | TiledArray::unary (const Tile< Arg > &arg, Op &&op) |
Unary element-wise transform producing a new tile. More... | |
template<typename Arg , typename Op , typename Perm , typename = std::enable_if_t<detail::is_permutation_v<Perm>>> | |
decltype(auto) | TiledArray::unary (const Tile< Arg > &arg, Op &&op, const Perm &perm) |
Unary element-wise transform producing a new tile. More... | |
template<typename Result , typename Op > | |
Tile< Result > & | TiledArray::inplace_unary (Tile< Result > &arg, Op &&op) |
Unary element-wise in-place transform. More... | |
template<typename Left , typename Right , typename Scalar , typename std::enable_if< detail::is_numeric_v< Scalar >>::type * = nullptr> | |
decltype(auto) | TiledArray::gemm (const Tile< Left > &left, const Tile< Right > &right, const Scalar factor, const math::GemmHelper &gemm_config) |
Contract 2 tensors over head/tail modes and scale the product. More... | |
template<typename Result , typename Left , typename Right , typename Scalar , typename std::enable_if< detail::is_numeric_v< Scalar >>::type * = nullptr> | |
Tile< Result > & | TiledArray::gemm (Tile< Result > &result, const Tile< Left > &left, const Tile< Right > &right, const Scalar factor, const math::GemmHelper &gemm_config) |
template<typename Result , typename Left , typename Right , typename ElementMultiplyAddOp , typename std::enable_if< std::is_invocable_r_v< void, std::remove_reference_t< ElementMultiplyAddOp >, typename Result::value_type &, const typename Left::value_type &, const typename Right::value_type & >>::type * = nullptr> | |
Tile< Result > & | TiledArray::gemm (Tile< Result > &result, const Tile< Left > &left, const Tile< Right > &right, const math::GemmHelper &gemm_config, ElementMultiplyAddOp &&element_multiplyadd_op) |
template<typename Arg > | |
decltype(auto) | TiledArray::trace (const Tile< Arg > &arg) |
Sum the hyper-diagonal elements a tile. More... | |
template<typename Arg > | |
decltype(auto) | TiledArray::sum (const Tile< Arg > &arg) |
Sum the elements of a tile. More... | |
template<typename Arg > | |
decltype(auto) | TiledArray::product (const Tile< Arg > &arg) |
Multiply the elements of a tile. More... | |
template<typename Arg > | |
decltype(auto) | TiledArray::squared_norm (const Tile< Arg > &arg) |
Squared vector 2-norm of the elements of a tile. More... | |
template<typename Arg > | |
decltype(auto) | TiledArray::norm (const Tile< Arg > &arg) |
Vector 2-norm of a tile. More... | |
template<typename Arg , typename ResultType > | |
void | TiledArray::norm (const Tile< Arg > &arg, ResultType &result) |
Vector 2-norm of a tile. More... | |
template<typename Arg > | |
decltype(auto) | TiledArray::max (const Tile< Arg > &arg) |
Maximum element of a tile. More... | |
template<typename Arg > | |
decltype(auto) | TiledArray::min (const Tile< Arg > &arg) |
Minimum element of a tile. More... | |
template<typename Arg > | |
decltype(auto) | TiledArray::abs_max (const Tile< Arg > &arg) |
Absolute maximum element of a tile. More... | |
template<typename Arg > | |
decltype(auto) | TiledArray::abs_min (const Tile< Arg > &arg) |
Absolute mainimum element of a tile. More... | |
template<typename Left , typename Right > | |
decltype(auto) | TiledArray::dot (const Tile< Left > &left, const Tile< Right > &right) |
Vector dot product of a tile. More... | |
template<typename Left , typename Right > | |
decltype(auto) | TiledArray::inner_product (const Tile< Left > &left, const Tile< Right > &right) |
Vector inner product of a tile. More... | |
template<typename T > | |
std::ostream & | TiledArray::operator<< (std::ostream &os, const Tile< T > &tile) |
Variables | |
template<typename T > | |
constexpr const bool | TiledArray::is_lazy_tile_v = is_lazy_tile<T>::value |
is_lazy_tile_v<T> is an alias for is_lazy_tile<T>::value More... | |
template<typename T > | |
constexpr const bool | TiledArray::is_consumable_tile_v = is_consumable_tile<T>::value |
is_consumable_tile_v<T> is an alias for is_consumable_tile<T>::value More... | |
Function Documentation
◆ abs_max()
|
inline |
◆ abs_min()
|
inline |
◆ add() [1/6]
|
inline |
Add a constant scalar to tile argument.
- Template Parameters
-
Arg The tile argument type Scalar A scalar type
- Parameters
-
arg The left-hand argument to be added value The constant scalar to be added
- Returns
- A tile that is equal to
arg + value
Definition at line 801 of file tile.h.

◆ add() [2/6]
|
inline |
Add a constant scalar and permute tile argument.
- Template Parameters
-
Arg The tile argument type Scalar A scalar type Perm A permutation tile
- Parameters
-
arg The left-hand argument to be added value The constant scalar value to be added perm The permutation to be applied to the result
- Returns
- A tile that is equal to
perm ^ (arg + value)
Definition at line 818 of file tile.h.

◆ add() [3/6]
|
inline |
Add tile arguments.
- Template Parameters
-
Left The left-hand tile type Right The right-hand tile type
- Parameters
-
left The left-hand argument to be added right The right-hand argument to be added
- Returns
- A tile that is equal to
(left + right)
Definition at line 734 of file tile.h.

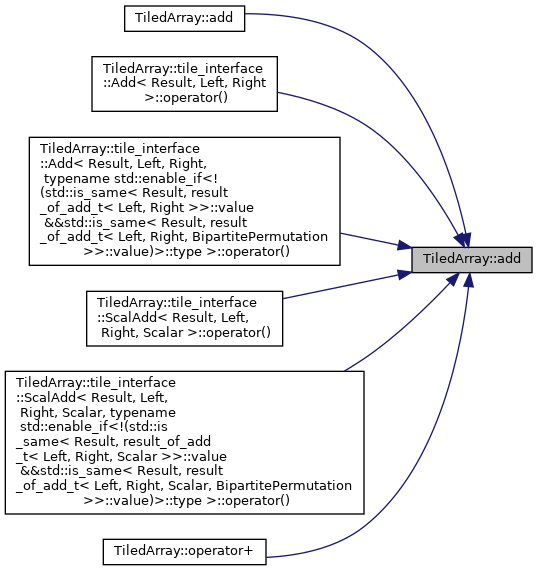
◆ add() [4/6]
|
inline |
Add and permute tile arguments.
- Template Parameters
-
Left The left-hand tile type Right The right-hand tile type Perm A permutation type
- Parameters
-
left The left-hand argument to be added right The right-hand argument to be added perm The permutation to be applied to the result
- Returns
- A tile that is equal to
perm * (left + right)
Definition at line 766 of file tile.h.

◆ add() [5/6]
|
inline |
Add and scale tile arguments.
- Template Parameters
-
Left The left-hand tile type Right The right-hand tile type Scalar A scalar type
- Parameters
-
left The left-hand argument to be added right The right-hand argument to be added factor The scaling factor
- Returns
- A tile that is equal to
(left + right) * factor
Definition at line 750 of file tile.h.

◆ add() [6/6]
|
inline |
Add, scale, and permute tile arguments.
- Template Parameters
-
Left The left-hand tile type Right The right-hand tile type Scalar A scalar type Perm A permutation tile
- Parameters
-
left The left-hand argument to be added right The right-hand argument to be added factor The scaling factor perm The permutation to be applied to the result
- Returns
- A tile that is equal to
perm ^ (left + right) * factor
Definition at line 786 of file tile.h.

◆ add_to() [1/3]
|
inline |
Add constant scalar to the result tile.
- Template Parameters
-
Result The result tile type Scalar A scalar type
- Parameters
-
result The result tile value The constant scalar to be added to result
- Returns
- A tile that is equal to
(result[i] += arg[i]) *= factor
Definition at line 864 of file tile.h.

◆ add_to() [2/3]
|
inline |
Add to the result tile.
- Template Parameters
-
Result The result tile type Arg The argument tile type
- Parameters
-
result The result tile arg The argument to be added to the result
- Returns
- A tile that is equal to
result[i] += arg[i]
Definition at line 831 of file tile.h.

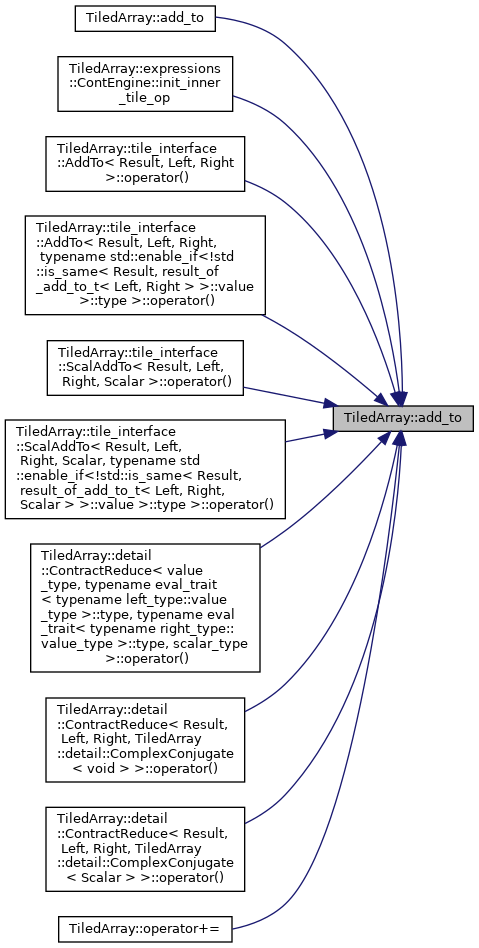
◆ add_to() [3/3]
|
inline |
Add and scale to the result tile.
- Template Parameters
-
Result The result tile type Arg The argument tile type Scalar A scalar type
- Parameters
-
result The result tile arg The argument to be added to result
factor The scaling factor
- Returns
- A tile that is equal to
(result[i] += arg[i]) * factor
Definition at line 848 of file tile.h.

◆ binary() [1/2]
|
inline |
Binary element-wise transform producing a new tile.
- Template Parameters
-
Left The left-hand tile type Right The right-hand tile type Op An element-wise operation type
- Parameters
-
[in] left The left-hand argument to the transform [in] right The right-hand argument to the transform op An element-wise operation
- Returns
result
where for eachi
inleft.range()
result
[i]==op(left[i],right[i])
Definition at line 1118 of file tile.h.


◆ binary() [2/2]
|
inline |
Binary element-wise transform producing a new tile.
- Template Parameters
-
Left The left-hand tile type Right The right-hand tile type Op An element-wise operation type Perm A permutation type
- Parameters
-
[in] left The left-hand argument to the transform [in] right The right-hand argument to the transform op An element-wise operation perm The permutation to be applied to the result
- Returns
perm^result
where for eachi
inleft.range()
result
[i]==op(left[i],right[i])
Definition at line 1139 of file tile.h.

◆ clone()
◆ conj() [1/4]
|
inline |
◆ conj() [2/4]
|
inline |
Create a complex conjugated and permuted copy of a tile.
- Template Parameters
-
Arg The tile argument type Perm A permutation tile
- Parameters
-
arg The tile to be conjugated perm The permutation to be applied to arg
- Returns
- A complex conjugated and permuted copy of
arg
Definition at line 1283 of file tile.h.

◆ conj() [3/4]
|
inline |
Create a complex conjugated and scaled copy of a tile.
- Template Parameters
-
Arg The tile argument type Scalar A scalar type
- Parameters
-
arg The tile to be conjugated factor The scaling factor
- Returns
- A complex conjugated and scaled copy of
arg
Definition at line 1270 of file tile.h.

◆ conj() [4/4]
|
inline |
Create a complex conjugated, scaled, and permuted copy of a tile.
- Template Parameters
-
Arg The tile argument type Scalar A scalar type Perm A permutation tile
- Parameters
-
arg The argument to be conjugated factor The scaling factor perm The permutation to be applied to arg
- Returns
- A complex conjugated, scaled, and permuted copy of
arg
Definition at line 1300 of file tile.h.

◆ conj_to() [1/2]
◆ conj_to() [2/2]
◆ dot()
|
inline |
Vector dot product of a tile.
- Template Parameters
-
Left The left-hand argument type Right The right-hand argument type
- Parameters
-
left The left-hand argument tile to be contracted right The right-hand argument tile to be contracted
- Returns
- A scalar that is equal to
sum_i left[i] * right[i]
Definition at line 1591 of file tile.h.

◆ empty()
|
inline |
◆ gemm() [1/3]
|
inline |
Contract 2 tensors over head/tail modes and scale the product.
The contraction is done via a GEMM operation with fused indices as defined by gemm_config
.
- Template Parameters
-
Left The left-hand tile type Right The right-hand tile type Scalar A numeric type
- Parameters
-
left The left-hand argument to be contracted right The right-hand argument to be contracted factor The scaling factor gemm_config A helper object used to simplify gemm operations
- Returns
- A tile that is equal to
(left * right) * factor
Definition at line 1396 of file tile.h.

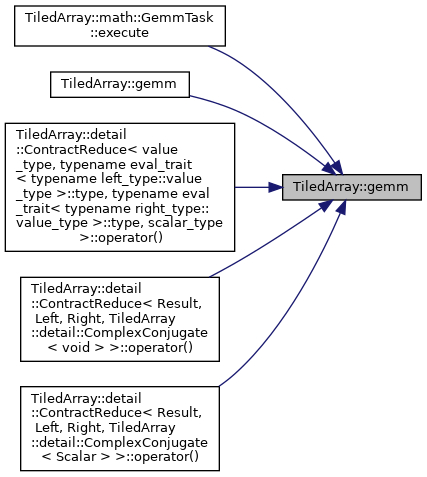
◆ gemm() [2/3]
|
inline |
Contract 2 tensors over head/tail modes and accumulate into result
using a custom element-wise multiply-add op The contraction is done via a GEMM operation with fused indices as defined by gemm_config
.
- Template Parameters
-
Result The result tile type Left The left-hand tile type Right The right-hand tile type ElementMultiplyAddOp a callable type with signature that implements custom multiply-add operation:void (Result::value_type& result, Left::value_type const& left,Right::value_type const& right)
- Parameters
-
result The contracted result left The left-hand argument to be contracted right The right-hand argument to be contracted factor The scaling factor gemm_config A helper object used to simplify gemm operations element_multiplyadd_op a custom multiply op operation for tensor elements
- Returns
- A tile whose element
result[i,j]
obtained by executingforeach k: element_multiplyadd_op(result[i,j], left[i,k], right[k,j])
Definition at line 1460 of file tile.h.

◆ gemm() [3/3]
|
inline |
Contract 2 tensors over head/tail modes, scale the product, and add to result
The contraction is done via a GEMM operation with fused indices as defined by gemm_config
.
- Template Parameters
-
Result The result tile type Left The left-hand tile type Right The right-hand tile type Scalar A numeric type
- Parameters
-
result The contracted result left The left-hand argument to be contracted right The right-hand argument to be contracted factor The scaling factor gemm_config A helper object used to simplify gemm operations
- Returns
- A tile that is equal to
result + (left * right) * factor
Definition at line 1421 of file tile.h.

◆ inner_product()
|
inline |
Vector inner product of a tile.
- Template Parameters
-
Left The left-hand argument type Right The right-hand argument type
- Parameters
-
left The left-hand argument tile to be contracted right The right-hand argument tile to be contracted
Definition at line 1602 of file tile.h.

◆ inplace_binary()
|
inline |
Binary element-wise in-place transform.
- Template Parameters
-
Left The left-hand tile type Right The right-hand tile type Op An element-wise operation type
- Parameters
-
[in,out] left The left-hand argument to the transform; output contains the result of binary(left,right,op)
[in] right The right-hand argument to the transform op An element-wise operation
- Returns
- reference to
left
Definition at line 1157 of file tile.h.

◆ inplace_unary()
|
inline |
Unary element-wise in-place transform.
- Template Parameters
-
Arg The tile argument type Op An element-wise operation type
- Parameters
-
[in,out] arg The tile to be transformed, on output for each i
inarg.range()
arg
[i] containsop(arg[i])
op An element-wise operation
- Returns
reference
toarg
Definition at line 1374 of file tile.h.

◆ max()
|
inline |
◆ min()
|
inline |
◆ mult() [1/4]
|
inline |
Multiplication tile arguments.
- Template Parameters
-
Left The left-hand tile type Right The right-hand tile type
- Parameters
-
left The left-hand argument to be multiplied right The right-hand argument to be multiplied
- Returns
- A tile that is equal to
(left * right)
Definition at line 1018 of file tile.h.

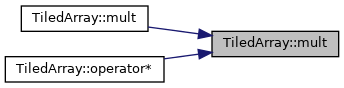
◆ mult() [2/4]
|
inline |
Multiplication and permute tile arguments.
- Template Parameters
-
Left The left-hand tile type Right The right-hand tile type Perm A permutation tile
- Parameters
-
left The left-hand argument to be multiplied right The right-hand argument to be multiplied perm The permutation to be applied to the result
- Returns
- A tile that is equal to
perm ^ (left * right)
Definition at line 1049 of file tile.h.

◆ mult() [3/4]
|
inline |
Multiplication and scale tile arguments.
- Template Parameters
-
Left The left-hand tile type Right The right-hand tile type
- Parameters
-
left The left-hand argument to be multiplied right The right-hand argument to be multiplied factor The scaling factor
- Returns
- A tile that is equal to
(left * right) * factor
Definition at line 1033 of file tile.h.

◆ mult() [4/4]
|
inline |
Multiplication, scale, and permute tile arguments.
- Template Parameters
-
Left The left-hand tile type Right The right-hand tile type Perm A permutation tile
- Parameters
-
left The left-hand argument to be multiplied right The right-hand argument to be multiplied factor The scaling factor perm The permutation to be applied to the result
- Returns
- A tile that is equal to
perm ^ (left * right) * factor
Definition at line 1068 of file tile.h.

◆ mult_to() [1/2]
|
inline |
Multiply to the result tile.
- Template Parameters
-
Result The result tile type Arg The argument tile type
- Parameters
-
result The result tile to be multiplied arg The argument to be multiplied by the result
- Returns
- A tile that is equal to
result *= arg
Definition at line 1081 of file tile.h.

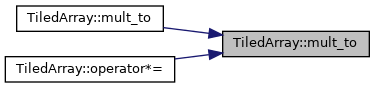
◆ mult_to() [2/2]
|
inline |
Multiply and scale to the result tile.
- Template Parameters
-
Result The result tile type Arg The argument tile type
- Parameters
-
result The result tile to be multiplied arg The argument to be multiplied by result
factor The scaling factor
- Returns
- A tile that is equal to
(result *= arg) *= factor
Definition at line 1097 of file tile.h.

◆ neg() [1/2]
|
inline |
Negate the tile argument.
- Template Parameters
-
Arg The tile argument type
- Parameters
-
arg The argument to be negated
- Returns
- A tile that is equal to
-arg
- Note
- equivalent to
scale
(arg,-1)
Definition at line 1218 of file tile.h.

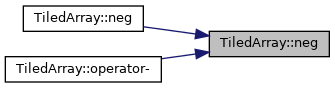
◆ neg() [2/2]
|
inline |
Negate and permute tile argument.
- Template Parameters
-
Arg The tile argument type Perm A permutation tile
- Parameters
-
arg The argument to be negated perm The permutation to be applied to the result
- Returns
- A tile that is equal to
perm ^ -arg
- Note
- equivalent to
scale
(arg,-1,perm)
Definition at line 1232 of file tile.h.

◆ neg_to()
◆ norm() [1/2]
|
inline |
◆ norm() [2/2]
|
inline |
Vector 2-norm of a tile.
- Template Parameters
-
Arg The tile argument type ResultType The result type
- Parameters
-
[in,out] arg The argument to be multiplied and summed; on output will contain the vector 2-norm of arg
, i.e.sqrt(sum_i arg[i] * arg[i])
Definition at line 1539 of file tile.h.
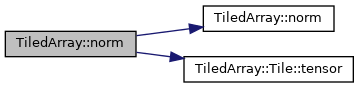
◆ operator<<()
|
inline |
◆ permute()
|
inline |
Create a permuted copy of arg
.
- Template Parameters
-
Arg The tile argument type Perm A permutation tile
- Parameters
-
arg The tile argument to be permuted perm The permutation to be applied to the result
- Returns
- A tile that is equal to
perm ^ arg
Definition at line 662 of file tile.h.

◆ product()
|
inline |
◆ scale() [1/2]
|
inline |
Scalar the tile argument.
- Template Parameters
-
Arg The tile argument type
- Parameters
-
arg The left-hand argument to be scaled factor The scaling factor
- Returns
- A tile that is equal to
arg * factor
Definition at line 1174 of file tile.h.

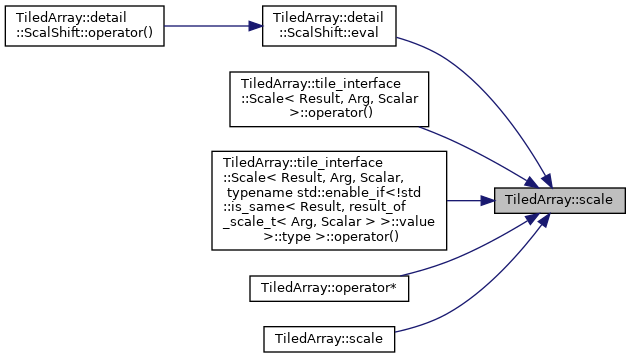
◆ scale() [2/2]
|
inline |
Scale and permute tile argument.
- Template Parameters
-
Arg The tile argument type Perm A permutation tile
- Parameters
-
arg The left-hand argument to be scaled factor The scaling factor perm The permutation to be applied to the result
- Returns
- A tile that is equal to
perm ^ (arg * factor)
Definition at line 1190 of file tile.h.

◆ scale_to()
|
inline |
Scale to the result tile.
- Template Parameters
-
Result The result tile type
- Parameters
-
result The result tile to be scaled factor The scaling factor
- Returns
- A tile that is equal to
result *= factor
Definition at line 1204 of file tile.h.

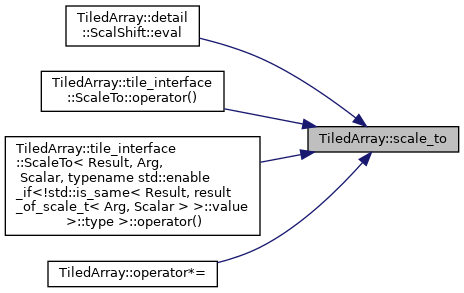
◆ shift() [1/2]
|
inline |
Shift the range of arg
.
- Template Parameters
-
Arg The tensor argument type Index An integral range type
- Parameters
-
arg The tile argument to be shifted range_shift The offset to be applied to the argument range
- Returns
- A copy of the tile with a new range
Definition at line 677 of file tile.h.
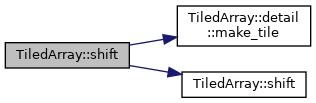
◆ shift() [2/2]
|
inline |
Shift the range of arg
.
- Template Parameters
-
Arg The tensor argument type Index An integral type
- Parameters
-
arg The tile argument to be shifted range_shift The offset to be applied to the argument range
- Returns
- A copy of the tile with a new range
Definition at line 690 of file tile.h.
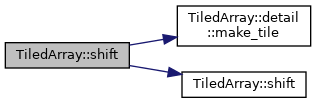
◆ shift_to() [1/2]
|
inline |
Shift the range of arg
in place.
- Template Parameters
-
Arg The tensor argument type Index An integral range type
- Parameters
-
arg The tile argument to be shifted range_shift The offset to be applied to the argument range
- Returns
- A copy of the tile with a new range
Definition at line 704 of file tile.h.

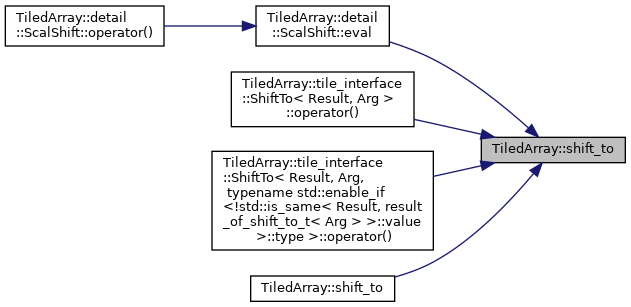
◆ shift_to() [2/2]
|
inline |
Shift the range of arg
in place.
- Template Parameters
-
Arg The tensor argument type Index An integral type
- Parameters
-
arg The tile argument to be shifted range_shift The offset to be applied to the argument range
- Returns
- A copy of the tile with a new range
Definition at line 718 of file tile.h.

◆ squared_norm()
|
inline |
Squared vector 2-norm of the elements of a tile.
- Template Parameters
-
Arg The tile argument type
- Parameters
-
arg The argument to be multiplied and summed
- Returns
- The sum of the squared elements of
arg
-
A scalar that is equal to
sum_i arg[i] * arg[i]
Definition at line 1517 of file tile.h.

◆ subt() [1/6]
|
inline |
Subtract a scalar constant from the tile argument.
- Template Parameters
-
Arg The tile argument type
- Parameters
-
arg The left-hand argument to be subtracted value The constant scalar to be subtracted
- Returns
- A tile that is equal to
arg - value
Definition at line 943 of file tile.h.

◆ subt() [2/6]
|
inline |
Subtract a constant scalar and permute tile argument.
- Template Parameters
-
Arg The tile argument type Perm A permutation tile
- Parameters
-
arg The left-hand argument to be subtracted value The constant scalar value to be subtracted perm The permutation to be applied to the result
- Returns
- A tile that is equal to
perm ^ (arg - value)
Definition at line 959 of file tile.h.

◆ subt() [3/6]
|
inline |
Subtract tile arguments.
- Template Parameters
-
Left The left-hand tile type Right The right-hand tile type
- Parameters
-
left The left-hand argument to be subtracted right The right-hand argument to be subtracted
- Returns
- A tile that is equal to
(left - right)
Definition at line 879 of file tile.h.

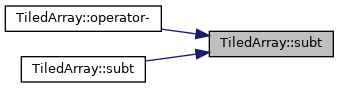
◆ subt() [4/6]
|
inline |
Subtract and permute tile arguments.
- Template Parameters
-
Left The left-hand tile type Right The right-hand tile type Perm A permutation tile
- Parameters
-
left The left-hand argument to be subtracted right The right-hand argument to be subtracted perm The permutation to be applied to the result
- Returns
- A tile that is equal to
perm ^ (left - right)
Definition at line 910 of file tile.h.

◆ subt() [5/6]
|
inline |
Subtract and scale tile arguments.
- Template Parameters
-
Left The left-hand tile type Right The right-hand tile type
- Parameters
-
left The left-hand argument to be subtracted right The right-hand argument to be subtracted factor The scaling factor
- Returns
- A tile that is equal to
(left - right) * factor
Definition at line 894 of file tile.h.

◆ subt() [6/6]
|
inline |
Subtract, scale, and permute tile arguments.
- Template Parameters
-
Left The left-hand tile type Right The right-hand tile type Perm A permutation tile
- Parameters
-
left The left-hand argument to be subtracted right The right-hand argument to be subtracted factor The scaling factor perm The permutation to be applied to the result
- Returns
- A tile that is equal to
perm ^ (left - right) * factor
Definition at line 929 of file tile.h.

◆ subt_to() [1/3]
|
inline |
Subtract constant scalar from the result tile.
- Template Parameters
-
Result The result tile type
- Parameters
-
result The result tile value The constant scalar to be subtracted from result
- Returns
- A tile that is equal to
(result -= arg) *= factor
Definition at line 1003 of file tile.h.

◆ subt_to() [2/3]
|
inline |
Subtract from the result tile.
- Template Parameters
-
Result The result tile type Arg The argument tile type
- Parameters
-
result The result tile arg The argument to be subtracted from the result
- Returns
- A tile that is equal to
result[i] -= arg[i]
Definition at line 972 of file tile.h.

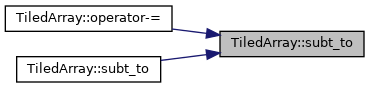
◆ subt_to() [3/3]
|
inline |
Subtract and scale from the result tile.
- Template Parameters
-
Result The result tile type Arg The argument tile type
- Parameters
-
result The result tile arg The argument to be subtracted from result
factor The scaling factor
- Returns
- A tile that is equal to
(result -= arg) *= factor
Definition at line 988 of file tile.h.

◆ sum()
|
inline |
◆ trace()
|
inline |
◆ unary() [1/2]
|
inline |
Unary element-wise transform producing a new tile.
- Template Parameters
-
Arg The tile argument type Op An element-wise operation type
- Parameters
-
[in] arg The tile to be transformed op An element-wise operation
- Returns
result
where for eachi
inarg.range()
result
[i]==op(arg[i])
Definition at line 1344 of file tile.h.


◆ unary() [2/2]
|
inline |
Unary element-wise transform producing a new tile.
- Template Parameters
-
Arg The tile argument type Op An element-wise operation type
- Parameters
-
[in] arg The tile to be transformed op An element-wise operation perm The permutation to be applied to the result of the transform
- Returns
perm^result
where for eachi
inarg.range()
result
[i]==op(arg[i])
Definition at line 1360 of file tile.h.

Variable Documentation
◆ is_consumable_tile_v
|
constexpr |
is_consumable_tile_v<T>
is an alias for is_consumable_tile<T>::value
Definition at line 618 of file type_traits.h.
◆ is_lazy_tile_v
|
constexpr |
is_lazy_tile_v<T>
is an alias for is_lazy_tile<T>::value
Definition at line 598 of file type_traits.h.