Documentation
template<typename T>
class TiledArray::Tile< T >
An N-dimensional shallow copy wrapper for tile objects.
Tile
represents a block of an Array
. The rank of the tile block is the same as the owning Array
object. In order for a user defined tensor object to be used in TiledArray expressions, users must also define the following functions:
add
add_to
subt
subt_to
mult
mult_to
scale
scale_to
gemm
neg
permute
empty
shift
shift_to
trace
sum
product
squared_norm
norm
min
max
abs_min
abs_max
dot
as for the intrusive or non-instrusive interface. See the non-intrusive tile interface documentation for more details.- Template Parameters
-
T The tensor type used to represent tile data
Public Types | |
typedef Tile< T > | Tile_ |
This object type. More... | |
typedef T | tensor_type |
Tensor type used to represent tile data. More... | |
using | value_type = typename tensor_type::value_type |
value type More... | |
using | range_type = typename tensor_type::range_type |
Tensor range type. More... | |
using | index1_type = typename tensor_type::index1_type |
1-index type More... | |
using | size_type = typename tensor_type::ordinal_type |
using | reference = typename tensor_type::reference |
Element reference type. More... | |
using | const_reference = typename tensor_type::const_reference |
Element reference type. More... | |
using | iterator = typename tensor_type::iterator |
Element iterator type. More... | |
using | const_iterator = typename tensor_type::const_iterator |
Element const iterator type. More... | |
using | pointer = typename tensor_type::pointer |
Element pointer type. More... | |
using | const_pointer = typename tensor_type::const_pointer |
Element const pointer type. More... | |
using | numeric_type = typename TiledArray::detail::numeric_type< tensor_type >::type |
the numeric type that supports T More... | |
using | scalar_type = typename TiledArray::detail::scalar_type< tensor_type >::type |
the scalar type that supports T More... | |
Public Member Functions | |
Tile ()=default | |
Tile (const Tile_ &)=default | |
Tile (Tile_ &&)=default | |
template<typename Arg , typename = typename std::enable_if< not detail::is_same_or_derived<Tile_, Arg>::value && not std::is_convertible<Arg, Tile_>::value && not TiledArray::detail::is_explicitly_convertible< Arg, Tile_>::value>::type> | |
Tile (Arg &&arg) | |
Forwarding ctor. More... | |
template<typename Arg1 , typename Arg2 , typename... Args> | |
Tile (Arg1 &&arg1, Arg2 &&arg2, Args &&... args) | |
~Tile ()=default | |
Tile_ & | operator= (Tile_ &&)=default |
Tile_ & | operator= (const Tile_ &)=default |
Tile_ & | operator= (const tensor_type &tensor) |
Tile_ & | operator= (tensor_type &&tensor) |
bool | empty () const |
tensor_type & | tensor () |
const tensor_type & | tensor () const |
decltype(auto) | begin () |
Iterator factory. More... | |
decltype(auto) | begin () const |
Iterator factory. More... | |
decltype(auto) | end () |
Iterator factory. More... | |
decltype(auto) | end () const |
Iterator factory. More... | |
decltype(auto) | data () |
Data direct access. More... | |
decltype(auto) | data () const |
Data direct access. More... | |
decltype(auto) | size () const |
Size accessors. More... | |
decltype(auto) | range () const |
Range accessor. More... | |
template<typename Ordinal , std::enable_if_t< std::is_integral< Ordinal >::value > * = nullptr> | |
const_reference | operator[] (const Ordinal ord) const |
Const element accessor. More... | |
template<typename Ordinal , std::enable_if_t< std::is_integral< Ordinal >::value > * = nullptr> | |
reference | operator[] (const Ordinal ord) |
Element accessor. More... | |
template<typename Index , std::enable_if_t< detail::is_integral_range_v< Index >> * = nullptr> | |
const_reference | operator[] (const Index &i) const |
Const element accessor. More... | |
template<typename Index , std::enable_if_t< detail::is_integral_range_v< Index >> * = nullptr> | |
reference | operator[] (const Index &i) |
Element accessor. More... | |
template<typename Index , typename = std::enable_if_t<std::is_integral_v<Index>>> | |
const_reference | operator[] (const std::initializer_list< Index > &i) const |
Const element accessor. More... | |
template<typename Index , typename = std::enable_if_t<std::is_integral_v<Index>>> | |
reference | operator[] (const std::initializer_list< Index > &i) |
Element accessor. More... | |
template<typename Index , std::enable_if_t< detail::is_integral_range_v< Index >> * = nullptr> | |
const_reference | operator() (const Index &i) const |
Const element accessor. More... | |
template<typename Index , std::enable_if_t< detail::is_integral_range_v< Index >> * = nullptr> | |
reference | operator() (const Index &i) |
Element accessor. More... | |
template<typename Index , typename = std::enable_if_t<std::is_integral_v<Index>>> | |
const_reference | operator() (const std::initializer_list< Index > &i) const |
Const element accessor. More... | |
template<typename Index , typename = std::enable_if_t<std::is_integral_v<Index>>> | |
reference | operator() (const std::initializer_list< Index > &i) |
Element accessor. More... | |
template<typename... Index, std::enable_if_t< detail::is_integral_list< Index... >::value > * = nullptr> | |
const_reference | operator() (const Index &... i) const |
Const element accessor. More... | |
template<typename... Index, std::enable_if_t< detail::is_integral_list< Index... >::value > * = nullptr> | |
reference | operator() (const Index &... i) |
Element accessor. More... | |
template<typename Archive , typename std::enable_if< madness::archive::is_output_archive< Archive >::value >::type * = nullptr> | |
void | serialize (Archive &ar) const |
template<typename Archive , typename std::enable_if< madness::archive::is_input_archive< Archive >::value >::type * = nullptr> | |
void | serialize (Archive &ar) |
template<typename Index1 , typename Index2 , typename = std::enable_if_t<detail::is_integral_range_v<Index1> && detail::is_integral_range_v<Index2>>> | |
decltype(auto) | block (const Index1 &lower_bound, const Index2 &upper_bound) |
Constructs a view of the block defined by lower_bound and upper_bound . More... | |
template<typename Index1 , typename Index2 , typename = std::enable_if_t<detail::is_integral_range_v<Index1> && detail::is_integral_range_v<Index2>>> | |
decltype(auto) | block (const Index1 &lower_bound, const Index2 &upper_bound) const |
Constructs a view of the block defined by lower_bound and upper_bound . More... | |
template<typename Index1 , typename Index2 , typename = std::enable_if_t<std::is_integral_v<Index1> && std::is_integral_v<Index2>>> | |
decltype(auto) | block (const std::initializer_list< Index1 > &lower_bound, const std::initializer_list< Index2 > &upper_bound) |
Constructs a view of the block defined by lower_bound and upper_bound . More... | |
template<typename Index1 , typename Index2 , typename = std::enable_if_t<std::is_integral_v<Index1> && std::is_integral_v<Index2>>> | |
decltype(auto) | block (const std::initializer_list< Index1 > &lower_bound, const std::initializer_list< Index2 > &upper_bound) const |
Constructs a view of the block defined by lower_bound and upper_bound . More... | |
template<typename PairRange , typename = std::enable_if_t<detail::is_gpair_range_v<PairRange>>> | |
decltype(auto) | block (const PairRange &bounds) |
Constructs a view of the block defined by its bounds . More... | |
template<typename PairRange , typename = std::enable_if_t<detail::is_gpair_range_v<PairRange>>> | |
decltype(auto) | block (const PairRange &bounds) const |
Constructs a view of the block defined by its bounds . More... | |
template<typename Index , typename = std::enable_if_t<std::is_integral_v<Index>>> | |
decltype(auto) | block (const std::initializer_list< std::initializer_list< Index >> &bounds) |
Constructs a view of the block defined by its bounds . More... | |
template<typename Index , typename = std::enable_if_t<std::is_integral_v<Index>>> | |
decltype(auto) | block (const std::initializer_list< std::initializer_list< Index >> &bounds) const |
Constructs a view of the block defined by its bounds . More... | |
Member Typedef Documentation
◆ const_iterator
using TiledArray::Tile< T >::const_iterator = typename tensor_type::const_iterator |
◆ const_pointer
using TiledArray::Tile< T >::const_pointer = typename tensor_type::const_pointer |
◆ const_reference
using TiledArray::Tile< T >::const_reference = typename tensor_type::const_reference |
◆ index1_type
using TiledArray::Tile< T >::index1_type = typename tensor_type::index1_type |
◆ iterator
using TiledArray::Tile< T >::iterator = typename tensor_type::iterator |
◆ numeric_type
using TiledArray::Tile< T >::numeric_type = typename TiledArray::detail::numeric_type< tensor_type>::type |
◆ pointer
using TiledArray::Tile< T >::pointer = typename tensor_type::pointer |
◆ range_type
using TiledArray::Tile< T >::range_type = typename tensor_type::range_type |
◆ reference
using TiledArray::Tile< T >::reference = typename tensor_type::reference |
◆ scalar_type
using TiledArray::Tile< T >::scalar_type = typename TiledArray::detail::scalar_type< tensor_type>::type |
◆ size_type
using TiledArray::Tile< T >::size_type = typename tensor_type::ordinal_type |
◆ tensor_type
typedef T TiledArray::Tile< T >::tensor_type |
◆ Tile_
typedef Tile<T> TiledArray::Tile< T >::Tile_ |
◆ value_type
using TiledArray::Tile< T >::value_type = typename tensor_type::value_type |
Constructor & Destructor Documentation
◆ Tile() [1/5]
|
default |
◆ Tile() [2/5]
|
default |
◆ Tile() [3/5]
|
default |
◆ Tile() [4/5]
|
inlineexplicit |
Forwarding ctor.
To simplify construction, Tile provides ctors that all forward their args to T. To avoid clashing with copy and move ctors need conditional instantiation – e.g. see http://ericniebler.com/2013/08/07/universal-references-and-the-copy-constructo/ NB For Arg that can be converted to Tile also use the copy/move ctors.
◆ Tile() [5/5]
|
inline |
◆ ~Tile()
|
default |
Member Function Documentation
◆ begin() [1/2]
|
inline |
◆ begin() [2/2]
|
inline |
◆ block() [1/8]
|
inline |
Constructs a view of the block defined by lower_bound
and upper_bound
.
Examples of using this:
- Template Parameters
-
Index1 An integral range type Index2 An integral range type
- Parameters
-
lower_bound The lower bound upper_bound The upper bound
- Returns
- a {const,mutable} view of the block defined by
lower_bound
andupper_bound
- Exceptions
-
TiledArray::Exception When the size of lower_bound
is not equal to that ofupper_bound
.TiledArray::Exception When lower_bound[i] >= upper_bound[i]
Definition at line 423 of file tile.h.
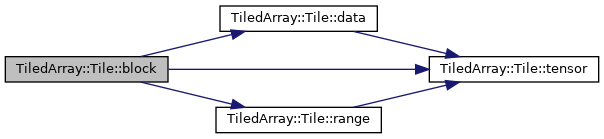

◆ block() [2/8]
|
inline |
Constructs a view of the block defined by lower_bound
and upper_bound
.
Examples of using this:
- Template Parameters
-
Index1 An integral range type Index2 An integral range type
- Parameters
-
lower_bound The lower bound upper_bound The upper bound
- Returns
- a {const,mutable} view of the block defined by
lower_bound
andupper_bound
- Exceptions
-
TiledArray::Exception When the size of lower_bound
is not equal to that ofupper_bound
.TiledArray::Exception When lower_bound[i] >= upper_bound[i]
Definition at line 433 of file tile.h.
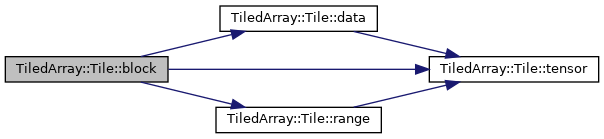
◆ block() [3/8]
|
inline |
Constructs a view of the block defined by its bounds
.
Examples of using this:
- Template Parameters
-
PairRange Type representing a range of generalized pairs (see TiledArray::detail::is_gpair_v )
- Parameters
-
bounds The block bounds
- Returns
- a {const,mutable} view of the block defined by its
bounds
- Exceptions
-
TiledArray::Exception When the size of lower_bound
is not equal to that ofupper_bound
.TiledArray::Exception When get<0>(bounds[i]) >= get<1>(bounds[i])
Definition at line 520 of file tile.h.
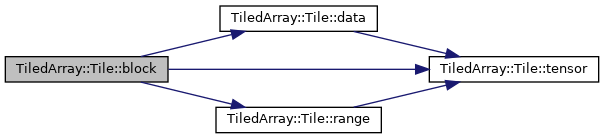
◆ block() [4/8]
|
inline |
Constructs a view of the block defined by its bounds
.
Examples of using this:
- Template Parameters
-
PairRange Type representing a range of generalized pairs (see TiledArray::detail::is_gpair_v )
- Parameters
-
bounds The block bounds
- Returns
- a {const,mutable} view of the block defined by its
bounds
- Exceptions
-
TiledArray::Exception When the size of lower_bound
is not equal to that ofupper_bound
.TiledArray::Exception When get<0>(bounds[i]) >= get<1>(bounds[i])
Definition at line 528 of file tile.h.
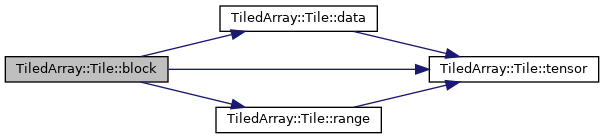
◆ block() [5/8]
|
inline |
Constructs a view of the block defined by lower_bound
and upper_bound
.
Examples of using this:
- Template Parameters
-
Index1 An integral type Index2 An integral type
- Parameters
-
lower_bound The lower bound upper_bound The upper bound
- Returns
- a {const,mutable} view of the block defined by
lower_bound
andupper_bound
- Exceptions
-
TiledArray::Exception When the size of lower_bound
is not equal to that ofupper_bound
.TiledArray::Exception When lower_bound[i] >= upper_bound[i]
Definition at line 464 of file tile.h.
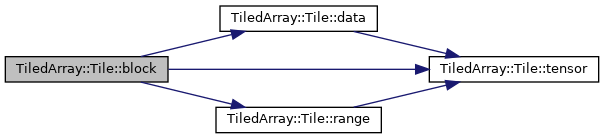
◆ block() [6/8]
|
inline |
Constructs a view of the block defined by lower_bound
and upper_bound
.
Examples of using this:
- Template Parameters
-
Index1 An integral type Index2 An integral type
- Parameters
-
lower_bound The lower bound upper_bound The upper bound
- Returns
- a {const,mutable} view of the block defined by
lower_bound
andupper_bound
- Exceptions
-
TiledArray::Exception When the size of lower_bound
is not equal to that ofupper_bound
.TiledArray::Exception When lower_bound[i] >= upper_bound[i]
Definition at line 475 of file tile.h.
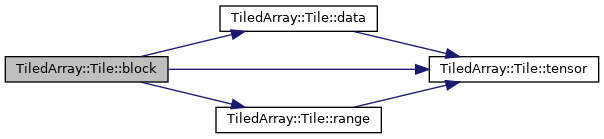
◆ block() [7/8]
|
inline |
Constructs a view of the block defined by its bounds
.
Examples of using this:
- Template Parameters
-
Index An integral type
- Parameters
-
bounds The block bounds
- Returns
- a {const,mutable} view of the block defined by its
bounds
- Exceptions
-
TiledArray::Exception When the size of lower_bound
is not equal to that ofupper_bound
.TiledArray::Exception When get<0>(bounds[i]) >= get<1>(bounds[i])
Definition at line 552 of file tile.h.
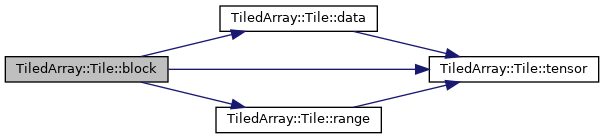
◆ block() [8/8]
|
inline |
Constructs a view of the block defined by its bounds
.
Examples of using this:
- Template Parameters
-
Index An integral type
- Parameters
-
bounds The block bounds
- Returns
- a {const,mutable} view of the block defined by its
bounds
- Exceptions
-
TiledArray::Exception When the size of lower_bound
is not equal to that ofupper_bound
.TiledArray::Exception When get<0>(bounds[i]) >= get<1>(bounds[i])
Definition at line 561 of file tile.h.
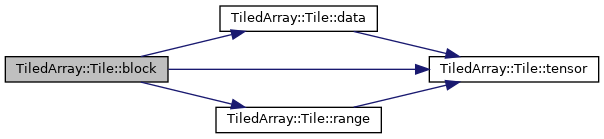
◆ data() [1/2]
|
inline |
◆ data() [2/2]
|
inline |
◆ empty()
|
inline |
◆ end() [1/2]
|
inline |
◆ end() [2/2]
|
inline |
◆ operator()() [1/6]
|
inline |
Element accessor.
- Template Parameters
-
Index an integral list ( see TiledArray::detail::is_integral_list )
- Parameters
-
[in] i an index
- Returns
- Reference to the element at position
i
.
- Note
- This asserts (using TA_ASSERT) that this is not empty and ord is included in the range
Definition at line 391 of file tile.h.

◆ operator()() [2/6]
|
inline |
Const element accessor.
- Template Parameters
-
Index an integral list ( see TiledArray::detail::is_integral_list )
- Parameters
-
[in] i an index
- Returns
- Const reference to the element at position
i
.
- Note
- This asserts (using TA_ASSERT) that this is not empty and ord is included in the range
Definition at line 376 of file tile.h.

◆ operator()() [3/6]
|
inline |
Element accessor.
- Template Parameters
-
Index An integral range type
- Parameters
-
[in] i an index
- Returns
- Reference to the element at position
i
.
- Note
- This asserts (using TA_ASSERT) that this is not empty and ord is included in the range
Definition at line 331 of file tile.h.

◆ operator()() [4/6]
|
inline |
Const element accessor.
- Template Parameters
-
Index An integral range type
- Parameters
-
[in] i an index
- Returns
- Const reference to the element at position
i
.
- Note
- This asserts (using TA_ASSERT) that this is not empty and ord is included in the range
Definition at line 316 of file tile.h.

◆ operator()() [5/6]
|
inline |
Element accessor.
- Template Parameters
-
Index An integral type
- Parameters
-
[in] i an index
- Returns
- Reference to the element at position
i
.
- Note
- This asserts (using TA_ASSERT) that this is not empty and ord is included in the range
Definition at line 361 of file tile.h.

◆ operator()() [6/6]
|
inline |
Const element accessor.
- Template Parameters
-
Index An integral type
- Parameters
-
[in] i an index
- Returns
- Const reference to the element at position
i
.
- Note
- This asserts (using TA_ASSERT) that this is not empty and ord is included in the range
Definition at line 346 of file tile.h.

◆ operator=() [1/4]
|
inline |
◆ operator=() [2/4]
|
default |
◆ operator=() [3/4]
|
inline |
◆ operator=() [4/4]
|
default |
◆ operator[]() [1/6]
|
inline |
Element accessor.
- Template Parameters
-
Index An integral range type
- Parameters
-
[in] i an index
- Returns
- Reference to the element at position
i
.
- Note
- This asserts (using TA_ASSERT) that this is not empty and ord is included in the range
Definition at line 271 of file tile.h.

◆ operator[]() [2/6]
|
inline |
Const element accessor.
- Template Parameters
-
Index An integral range type
- Parameters
-
[in] i an index
- Returns
- Const reference to the element at position
i
.
- Note
- This asserts (using TA_ASSERT) that this is not empty and ord is included in the range
Definition at line 256 of file tile.h.

◆ operator[]() [3/6]
|
inline |
Element accessor.
- Template Parameters
-
Ordinal an integer type that represents an ordinal
- Parameters
-
[in] ord an ordinal index
- Returns
- Reference to the element at position
ord
.
- Note
- This asserts (using TA_ASSERT) that this is not empty and ord is included in the range
Definition at line 241 of file tile.h.

◆ operator[]() [4/6]
|
inline |
Const element accessor.
- Template Parameters
-
Ordinal an integer type that represents an ordinal
- Parameters
-
[in] ord an ordinal index
- Returns
- Const reference to the element at position
ord
.
- Note
- This asserts (using TA_ASSERT) that this is not empty and ord is included in the range
Definition at line 226 of file tile.h.

◆ operator[]() [5/6]
|
inline |
Element accessor.
- Template Parameters
-
Index An integral type
- Parameters
-
[in] i an index
- Returns
- Reference to the element at position
i
.
- Note
- This asserts (using TA_ASSERT) that this is not empty and ord is included in the range
Definition at line 301 of file tile.h.

◆ operator[]() [6/6]
|
inline |
Const element accessor.
- Template Parameters
-
Index An integral type
- Parameters
-
[in] i an index
- Returns
- Const reference to the element at position
i
.
- Note
- This asserts (using TA_ASSERT) that this is not empty and ord is included in the range
Definition at line 286 of file tile.h.

◆ range()
|
inline |
◆ serialize() [1/2]
|
inline |
◆ serialize() [2/2]
|
inline |
◆ size()
|
inline |
◆ tensor() [1/2]
|
inline |
◆ tensor() [2/2]
|
inline |
The documentation for this class was generated from the following files:
- TiledArray/tensor/type_traits.h
- TiledArray/tile.h