mpqc::lcao::ReferenceFockBuilder< Tile, Policy, Integral > Class Template Reference
Collaboration diagram for mpqc::lcao::ReferenceFockBuilder< Tile, Policy, Integral >:
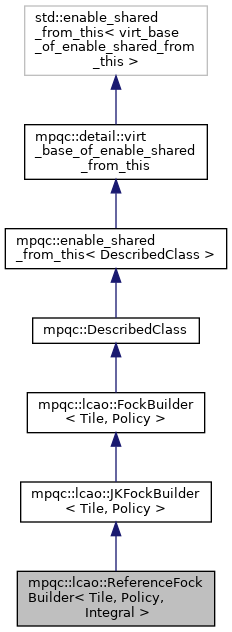
Documentation
template<typename Tile, typename Policy, typename Integral>
class mpqc::lcao::ReferenceFockBuilder< Tile, Policy, Integral >
ReferenceFockBuilder is a reference implementation that uses 4-center (stored or direct) integrals.
- Warning
- This is a very inefficient builder when used with direct integrals: the number of integrals it evaluates is roughly 6 to 16 times greater than optimal (this depends on whether the integral arrays account for any permutational symmetry). It is only useful for reference computation
Public Types | |
using | base_type = JKFockBuilder< Tile, Policy > |
using | array_type = typename base_type::array_type |
using | arrayvec_type = typename base_type::arrayvec_type |
using | result_type = typename base_type::result_type |
![]() | |
using | array_type = TA::DistArray< Tile, Policy > |
using | arrayvec_type = TA::DistArrayVector< Tile, Policy > |
using | result_type = std::tuple< arrayvec_type, std::optional< double > > |
![]() | |
using | array_type = TA::DistArray< Tile, Policy > |
using | arrayvec_type = TA::DistArrayVector< Tile, Policy > |
using | result_type = std::tuple< arrayvec_type, std::optional< double > > |
![]() | |
typedef std::shared_ptr< DescribedClass >(* | keyval_ctor_wrapper_type) (const KeyVal &) |
Public Member Functions | |
ReferenceFockBuilder (Integral const &eri4_J, Integral const &eri4_K) | |
result_type | compute (arrayvec_type const &D, arrayvec_type const &LMO, double scale_J_by=1, double scale_K_by=1, double target_precision=std::numeric_limits< double >::epsilon()) override |
computes the J+K part of the Fock matrix with variable fraction of J and K More... | |
void | register_fock (const arrayvec_type &fock, FormulaRegistry< array_type > ®istry) const override |
![]() | |
virtual | ~JKFockBuilder ()=default |
result_type | operator() (arrayvec_type const &D, arrayvec_type const &C, double target_precision=std::numeric_limits< double >::epsilon()) override final |
computes the J+K contribution of the Fock matrix More... | |
![]() | |
virtual | ~FockBuilder () |
virtual void | log_iter (std::ostream &os=ExEnv::out0()) const |
![]() | |
DescribedClass ()=default | |
virtual | ~DescribedClass () |
std::string | class_key () const |
![]() | |
virtual | ~enable_shared_from_this ()=default |
std::shared_ptr< DescribedClass > | shared_from_this () |
returns the pointer to this object More... | |
std::shared_ptr< std::add_const_t< DescribedClass > > | shared_from_this () const |
returns the pointer to this object More... | |
![]() | |
virtual | ~virt_base_of_enable_shared_from_this ()=default |
bool | shared_from_this_possible () const |
template<typename Target , typename = std::enable_if_t<!std::is_const_v<Target>>> | |
std::shared_ptr< Target > | cast_shared_from_this_to () |
returns the pointer to this cast to a particular type More... | |
template<typename Target > | |
std::shared_ptr< std::add_const_t< Target > > | cast_shared_from_this_to () const |
returns the pointer to this cast to a particular type More... | |
Additional Inherited Members | |
![]() | |
static keyval_ctor_wrapper_type | type_to_keyval_ctor (const std::string &type_name) |
template<typename T > | |
static void | register_keyval_ctor () |
template<typename T > | |
static bool | is_registered () |
template<typename T > | |
static std::string | class_key () |
static const keyval_ctor_registry_type & | keyval_ctor_registry () |
returns const ref to the keyval ctor registry More... | |
Member Typedef Documentation
◆ array_type
template<typename Tile , typename Policy , typename Integral >
using mpqc::lcao::ReferenceFockBuilder< Tile, Policy, Integral >::array_type = typename base_type::array_type |
◆ arrayvec_type
template<typename Tile , typename Policy , typename Integral >
using mpqc::lcao::ReferenceFockBuilder< Tile, Policy, Integral >::arrayvec_type = typename base_type::arrayvec_type |
◆ base_type
template<typename Tile , typename Policy , typename Integral >
using mpqc::lcao::ReferenceFockBuilder< Tile, Policy, Integral >::base_type = JKFockBuilder<Tile, Policy> |
◆ result_type
template<typename Tile , typename Policy , typename Integral >
using mpqc::lcao::ReferenceFockBuilder< Tile, Policy, Integral >::result_type = typename base_type::result_type |
Constructor & Destructor Documentation
◆ ReferenceFockBuilder()
template<typename Tile , typename Policy , typename Integral >
|
inline |
Member Function Documentation
◆ compute()
template<typename Tile , typename Policy , typename Integral >
|
inlineoverridevirtual |
computes the J+K part of the Fock matrix with variable fraction of J and K
- Parameters
-
D the (1-particle) density matrix C the occupied orbital AO coefficient matrix scale_J_by the coefficient of J in the result scale_K_by the coefficient of K in the result target_precision the (absolute) target precision of the Fock matrix; this precision is not in general guaranteed, but the actual precision of the Fock matrix is usually proportional to this value.
- Returns
- the J+K contribution to the Fock matrix an optional energy correction
Implements mpqc::lcao::JKFockBuilder< Tile, Policy >.
◆ register_fock()
template<typename Tile , typename Policy , typename Integral >
|
inlineoverridevirtual |
Implements mpqc::lcao::FockBuilder< Tile, Policy >.
The documentation for this class was generated from the following file:
- mpqc/chemistry/qc/lcao/scf/traditional_four_center_fock_builder.h