Documentation
TiledRange1 class defines a non-uniformly-tiled, contiguous, one-dimensional range. The tiling data is constructed with and stored in an array with the format {a0, a1, a2, ...}, where 0 <= a0 < a1 < a2 < ... Each tile is defined as [a0,a1), [a1,a2), ... The number of tiles in the range will be equal to one less than the number of elements in the array.
Definition at line 50 of file tiled_range1.h.
Public Types | |
typedef TA_1INDEX_TYPE | index1_type |
typedef std::pair< index1_type, index1_type > | range_type |
typedef std::vector< range_type >::const_iterator | const_iterator |
Public Member Functions | |
TiledRange1 () | |
template<typename RandIter , typename std::enable_if< detail::is_random_iterator< RandIter >::value >::type * = nullptr> | |
TiledRange1 (RandIter first, RandIter last) | |
TiledRange1 (const TiledRange1 &rng)=default | |
Copy constructor. More... | |
TiledRange1 (TiledRange1 &&rng)=default | |
Move constructor. More... | |
template<typename... _sizes> | |
TiledRange1 (const index1_type &t0, const _sizes &... t_rest) | |
Construct a 1D tiled range. More... | |
template<typename Integer , typename = std::enable_if_t<std::is_integral_v<Integer>>> | |
TiledRange1 (const std::initializer_list< Integer > &list) | |
Construct a 1D tiled range. More... | |
TiledRange1 & | operator= (const TiledRange1 &rng)=default |
Copy assignment operator. More... | |
TiledRange1 & | operator= (TiledRange1 &&rng)=default |
Move assignment operator. More... | |
const_iterator | begin () const |
Returns an iterator to the first tile in the range. More... | |
const_iterator | end () const |
Returns an iterator to the end of the range. More... | |
bool | empty () const |
Returns true if this range is empty (i.e. has no tiles) More... | |
const_iterator | find (const index1_type &e) const |
Return tile iterator associated with ordinal_index. More... | |
const range_type & | tiles () const |
const range_type & | tiles_range () const |
Tile range accessor. More... | |
const range_type & | elements () const |
const range_type & | elements_range () const |
Elements range accessor. More... | |
index1_type | tile_extent () const |
Tile range extent accessor. More... | |
index1_type | extent () const |
Elements range extent accessor. More... | |
const range_type & | tile (const index1_type i) const |
Tile range accessor. More... | |
const index1_type & | element_to_tile (const index1_type &i) const |
Maps element index to tile index. More... | |
const index1_type & | element2tile (const index1_type &i) const |
void | swap (TiledRange1 &other) |
swapper More... | |
template<typename Archive , typename std::enable_if< madness::archive::is_input_archive< Archive >::value >::type * = nullptr> | |
void | serialize (const Archive &ar) |
template<typename Archive , typename std::enable_if< madness::archive::is_output_archive< Archive >::value >::type * = nullptr> | |
void | serialize (const Archive &ar) const |
Friends | |
std::ostream & | operator<< (std::ostream &, const TiledRange1 &) |
TiledRange1 ostream operator. More... | |
Member Typedef Documentation
◆ const_iterator
typedef std::vector<range_type>::const_iterator TiledArray::TiledRange1::const_iterator |
Definition at line 57 of file tiled_range1.h.
◆ index1_type
typedef TA_1INDEX_TYPE TiledArray::TiledRange1::index1_type |
Definition at line 55 of file tiled_range1.h.
◆ range_type
typedef std::pair<index1_type, index1_type> TiledArray::TiledRange1::range_type |
Definition at line 56 of file tiled_range1.h.
Constructor & Destructor Documentation
◆ TiledRange1() [1/6]
|
inline |
Default constructor creates an empty range (tile and element ranges are both [0,0))
- Postcondition
- TiledRange1 tr;assert(tr.tiles_range() == (TiledRange1::range_type{0,0}));assert(tr.elements_range() == (TiledRange1::range_type{0,0}));assert(tr.begin() == tr.end());
Definition at line 66 of file tiled_range1.h.
◆ TiledRange1() [2/6]
|
inlineexplicit |
Constructs a range with the boundaries provided by the range [ first
, last
).
- Note
- validity of the [
first
,last
) range is checked using TA_ASSERT() only if preprocessor macroNDEBUG
is not defined
Definition at line 76 of file tiled_range1.h.
◆ TiledRange1() [3/6]
|
default |
Copy constructor.
◆ TiledRange1() [4/6]
|
default |
Move constructor.
◆ TiledRange1() [5/6]
|
inlineexplicit |
Construct a 1D tiled range.
This will construct a 1D tiled range with tile boundaries {t0
, t_rest
... } The number of tile boundaries is n + 1, where n is the number of tiles. Tiles are defined as [t0
, t1), [t1, t2), [t2, t3), ...
- Parameters
-
t0 The starting index of the first tile t_rest The rest of tile boundaries
- Note
- validity of the {
t0
,t_rest
... } range is checked using TA_ASSERT() only if preprocessor macroNDEBUG
is not defined
Definition at line 98 of file tiled_range1.h.
◆ TiledRange1() [6/6]
|
inlineexplicit |
Construct a 1D tiled range.
This will construct a 1D tiled range with tile boundaries {t0
, t_rest
... } The number of tile boundaries is n + 1, where n is the number of tiles. Tiles are defined as [t0
, t1), [t1, t2), [t2, t3), ... Tiles are indexed starting with 0.
- Template Parameters
-
Integer An integral type
- Parameters
-
list The list of tile boundaries in order from smallest to largest
- Note
- validity of the {
t0
,t_rest
... } range is checked using TA_ASSERT() only if preprocessor macroNDEBUG
is not defined
Definition at line 117 of file tiled_range1.h.
Member Function Documentation
◆ begin()
|
inline |
Returns an iterator to the first tile in the range.
Definition at line 128 of file tiled_range1.h.
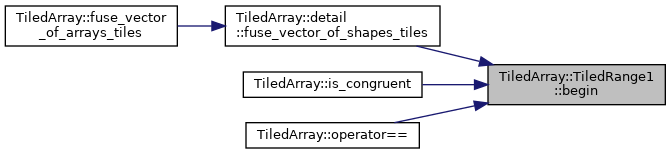
◆ element2tile()
|
inline |
◆ element_to_tile()
|
inline |
Maps element index to tile index.
- Parameters
-
i element index
- Returns
- tile index
- Precondition
- assert(i >= elements_range().first && i < elements_range().second);
- Note
- element->tile map is memoized, thus complexity of the first call is linear in the number of elements, complexity of subsequent calls is constant. Note that the memoization assumes infrequent (or serial) use as it is serialized across ALL TiledRange1 instances.
Definition at line 194 of file tiled_range1.h.
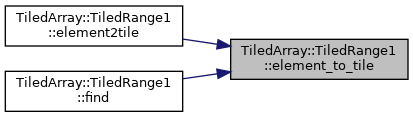
◆ elements()
|
inline |
Definition at line 153 of file tiled_range1.h.
◆ elements_range()
|
inline |
Elements range accessor.
- Returns
- a reference to the element index range
Definition at line 158 of file tiled_range1.h.
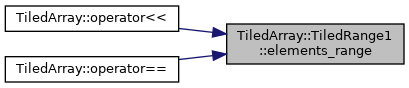
◆ empty()
|
inline |
Returns true if this range is empty (i.e. has no tiles)
Definition at line 134 of file tiled_range1.h.

◆ end()
|
inline |
Returns an iterator to the end of the range.
Definition at line 131 of file tiled_range1.h.

◆ extent()
|
inline |
Elements range extent accessor.
Definition at line 164 of file tiled_range1.h.
◆ find()
|
inline |
Return tile iterator associated with ordinal_index.
Definition at line 137 of file tiled_range1.h.

◆ operator=() [1/2]
|
default |
Copy assignment operator.
◆ operator=() [2/2]
|
default |
Move assignment operator.
◆ serialize() [1/2]
|
inline |
Definition at line 221 of file tiled_range1.h.
◆ serialize() [2/2]
|
inline |
Definition at line 228 of file tiled_range1.h.
◆ swap()
|
inline |
swapper
- Parameters
-
other the range with which the contents of this range will be swapped
Definition at line 211 of file tiled_range1.h.


◆ tile()
|
inline |
Tile range accessor.
- Parameters
-
i tile index
- Returns
- A const reference to the range of tile
i
- Precondition
- assert(i >= tiles_range().first && i < tiles_range().second);
Definition at line 176 of file tiled_range1.h.

◆ tile_extent()
|
inline |
Tile range extent accessor.
Definition at line 161 of file tiled_range1.h.

◆ tiles()
|
inline |
Definition at line 145 of file tiled_range1.h.
◆ tiles_range()
|
inline |
Tile range accessor.
- Returns
- a reference to the tile index range
Definition at line 150 of file tiled_range1.h.
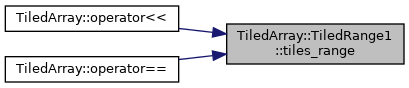
Friends And Related Function Documentation
◆ operator<<
|
friend |
TiledRange1 ostream operator.
Definition at line 323 of file tiled_range1.h.
The documentation for this class was generated from the following file:
- TiledArray/tiled_range1.h