Documentation
template<typename Op>
class TiledArray::detail::BinaryWrapper< Op >
Binary tile operation wrapper.
This wrapper class handles evaluation of lazily evaluated tiles in binary operations and forwards the evaluated arguments to the base operation object.
The base binary operation class must have the following interface.
- Template Parameters
-
Op The base binary operation type
Definition at line 94 of file binary_wrapper.h.
Public Types | |
typedef BinaryWrapper< Op > | BinaryWrapper_ |
typedef Op::left_type | left_type |
Left-hand argument type. More... | |
typedef Op::right_type | right_type |
Right-hand argument type. More... | |
typedef Op::result_type | result_type |
The result tile type. More... | |
template<typename T > | |
using | eval_t = typename eval_trait< std::decay_t< T > >::type |
Public Member Functions | |
BinaryWrapper (const BinaryWrapper< Op > &)=default | |
BinaryWrapper (BinaryWrapper< Op > &&)=default | |
~BinaryWrapper ()=default | |
BinaryWrapper< Op > & | operator= (const BinaryWrapper< Op > &)=default |
BinaryWrapper< Op > & | operator= (BinaryWrapper< Op > &&)=default |
template<typename Perm , typename = std::enable_if_t< TiledArray::detail::is_permutation_v<Perm>>> | |
BinaryWrapper (const Op &op, const Perm &perm) | |
BinaryWrapper (const Op &op) | |
template<typename L , typename R , std::enable_if_t< !(is_lazy_tile_v< L >||is_lazy_tile_v< R >)&&!std::is_same< std::decay_t< L >, ZeroTensor >::value &&!std::is_same< std::decay_t< R >, ZeroTensor >::value > * = nullptr> | |
auto | operator() (L &&left, R &&right) const |
Evaluate two non-zero tiles and possibly permute. More... | |
template<typename R , std::enable_if_t<!is_lazy_tile_v< R >> * = nullptr> | |
auto | operator() (const ZeroTensor &left, R &&right) const |
Evaluate a zero tile to a non-zero tiles and possibly permute. More... | |
template<typename L , std::enable_if_t<!is_lazy_tile_v< L >> * = nullptr> | |
auto | operator() (L &&left, const ZeroTensor &right) const |
Evaluate a non-zero tiles to a zero tile and possibly permute. More... | |
template<typename L , typename R , std::enable_if_t< is_lazy_tile_v< L > &&is_lazy_tile_v< R > &&(left_is_consumable||right_is_consumable)> * = nullptr> | |
auto | operator() (L &&left, R &&right) const |
Evaluate two lazy tiles. More... | |
template<typename L , typename R , std::enable_if_t< is_lazy_tile_v< L > &&(!is_lazy_tile_v< R >)&&(left_is_consumable||right_is_consumable)> * = nullptr> | |
auto | operator() (L &&left, R &&right) const |
Evaluate lazy and non-lazy tiles. More... | |
template<typename L , typename R , std::enable_if_t<(!is_lazy_tile_v< L >)&&is_lazy_tile_v< R > &&(left_is_consumable||right_is_consumable)> * = nullptr> | |
auto | operator() (L &&left, R &&right) const |
Evaluate non-lazy and lazy tiles. More... | |
template<typename L , typename R , std::enable_if_t< is_array_tile_v< L > &&is_array_tile_v< R > &&!(left_is_consumable||right_is_consumable)> * = nullptr> | |
auto | operator() (L &&left, R &&right) const |
Evaluate two lazy-array tiles. More... | |
template<typename L , typename R , std::enable_if_t< is_array_tile_v< L > &&(!is_lazy_tile_v< R >)&&!(left_is_consumable||right_is_consumable)> * = nullptr> | |
auto | operator() (L &&left, R &&right) const |
template<typename L , typename R , std::enable_if_t< is_array_tile_v< L > &&is_nonarray_lazy_tile_v< R > &&!(left_is_consumable||right_is_consumable)> * = nullptr> | |
auto | operator() (L &&left, R &&right) const |
template<typename L , typename R , std::enable_if_t<(!is_lazy_tile_v< L >)&&is_array_tile_v< R > &&!(left_is_consumable||right_is_consumable)> * = nullptr> | |
auto | operator() (L &&left, R &&right) const |
template<typename L , typename R , std::enable_if_t< is_nonarray_lazy_tile_v< L > &&is_array_tile_v< R > &&!(left_is_consumable||right_is_consumable)> * = nullptr> | |
auto | operator() (L &&left, R &&right) const |
Static Public Attributes | |
static constexpr bool | left_is_consumable = Op::left_is_consumable |
Boolean value that indicates the left-hand argument can always be consumed. More... | |
static constexpr bool | right_is_consumable = Op::right_is_consumable |
template<typename T > | |
static constexpr bool | is_lazy_tile_v = is_lazy_tile<std::decay_t<T>>::value |
template<typename T > | |
static constexpr bool | is_array_tile_v = is_array_tile<std::decay_t<T>>::value |
template<typename T > | |
static constexpr bool | is_nonarray_lazy_tile_v |
Member Typedef Documentation
◆ BinaryWrapper_
typedef BinaryWrapper<Op> TiledArray::detail::BinaryWrapper< Op >::BinaryWrapper_ |
Definition at line 96 of file binary_wrapper.h.
◆ eval_t
using TiledArray::detail::BinaryWrapper< Op >::eval_t = typename eval_trait<std::decay_t<T> >::type |
Definition at line 118 of file binary_wrapper.h.
◆ left_type
typedef Op::left_type TiledArray::detail::BinaryWrapper< Op >::left_type |
Left-hand argument type.
Definition at line 97 of file binary_wrapper.h.
◆ result_type
typedef Op::result_type TiledArray::detail::BinaryWrapper< Op >::result_type |
The result tile type.
Definition at line 99 of file binary_wrapper.h.
◆ right_type
typedef Op::right_type TiledArray::detail::BinaryWrapper< Op >::right_type |
Right-hand argument type.
Definition at line 98 of file binary_wrapper.h.
Constructor & Destructor Documentation
◆ BinaryWrapper() [1/4]
|
default |
◆ BinaryWrapper() [2/4]
|
default |
◆ ~BinaryWrapper()
|
default |
◆ BinaryWrapper() [3/4]
|
inline |
Definition at line 134 of file binary_wrapper.h.
◆ BinaryWrapper() [4/4]
|
inline |
Definition at line 136 of file binary_wrapper.h.
Member Function Documentation
◆ operator()() [1/11]
|
inline |
Evaluate a zero tile to a non-zero tiles and possibly permute.
Evaluate the result tile using the appropriate Derived
class evaluation kernel.
- Parameters
-
left The left-hand argument right The right-hand argument
- Returns
- The result tile from the binary operation applied to the
left
andright
arguments.
Definition at line 172 of file binary_wrapper.h.
◆ operator()() [2/11]
|
inline |
Evaluate a non-zero tiles to a zero tile and possibly permute.
Evaluate the result tile using the appropriate Derived
class evaluation kernel.
- Parameters
-
left The left-hand argument right The right-hand argument
- Returns
- The result tile from the binary operation applied to the
left
andright
arguments.
Definition at line 190 of file binary_wrapper.h.
◆ operator()() [3/11]
|
inline |
Evaluate two non-zero tiles and possibly permute.
Evaluate the result tile using the appropriate Derived
class evaluation kernel.
- Parameters
-
left The left-hand argument right The right-hand argument
- Returns
- The result tile from the binary operation applied to the
left
andright
arguments.
Definition at line 151 of file binary_wrapper.h.
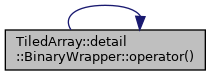
◆ operator()() [4/11]
|
inline |
Evaluate two lazy tiles.
This function will evaluate the left
and right
, then pass the evaluated tiles to the appropriate BinaryInterfaceBase_::operator()
function.
- Template Parameters
-
L The left-hand, lazy tile type R The right-hand, lazy tile type
- Parameters
-
left The left-hand, lazy tile argument right The right-hand, lazy tile argument
- Returns
- The result tile from the binary operation applied to the evaluated
left
andright
.
Definition at line 217 of file binary_wrapper.h.

◆ operator()() [5/11]
|
inline |
Evaluate lazy and non-lazy tiles.
This function will evaluate the left
, then pass the evaluated tile and right
to the appropriate BinaryInterfaceBase_::operator()
function.
- Template Parameters
-
L The left-hand, lazy tile type R The right-hand, non-lazy tile type
- Parameters
-
left The left-hand, lazy tile argument right The right-hand, non-lazy tile argument
- Returns
- The result tile from the binary operation applied to the evaluated
left
andright
.
Definition at line 244 of file binary_wrapper.h.

◆ operator()() [6/11]
|
inline |
Evaluate non-lazy and lazy tiles.
This function will evaluate the right
, then pass the evaluated tile and left
to the appropriate BinaryInterfaceBase_::operator()
function.
- Template Parameters
-
L The left-hand, non-lazy tile type R The right-hand, lazy tile type
- Parameters
-
left The left-hand, non-lazy tile argument right The right-hand, lazy tile argument
- Returns
- The result tile from the binary operation applied to the evaluated
left
andright
.
Definition at line 268 of file binary_wrapper.h.

◆ operator()() [7/11]
|
inline |
Evaluate two lazy-array tiles.
This function will evaluate the left
and right
, then pass the evaluated tiles to the appropriate Derived
class evaluation kernel.
- Template Parameters
-
L The left-hand, lazy-array tile type R The right-hand, lazy-array tile type
- Parameters
-
left The left-hand, non-lazy tile argument right The right-hand, lazy tile argument
- Returns
- The result tile from the binary operation applied to the evaluated
left
andright
.
Definition at line 291 of file binary_wrapper.h.

◆ operator()() [8/11]
|
inline |
◆ operator()() [9/11]
|
inline |
◆ operator()() [10/11]
|
inline |
◆ operator()() [11/11]
|
inline |
◆ operator=() [1/2]
|
default |
◆ operator=() [2/2]
|
default |
Member Data Documentation
◆ is_array_tile_v
|
staticconstexpr |
Definition at line 111 of file binary_wrapper.h.
◆ is_lazy_tile_v
|
staticconstexpr |
Definition at line 108 of file binary_wrapper.h.
◆ is_nonarray_lazy_tile_v
|
staticconstexpr |
Definition at line 114 of file binary_wrapper.h.
◆ left_is_consumable
|
staticconstexpr |
Boolean value that indicates the left-hand argument can always be consumed.
Definition at line 102 of file binary_wrapper.h.
◆ right_is_consumable
|
staticconstexpr |
Boolean value that indicates the right-hand argument can always be consumed
Definition at line 105 of file binary_wrapper.h.
The documentation for this class was generated from the following file:
- TiledArray/tile_op/binary_wrapper.h