Documentation
template<typename opT>
class TiledArray::detail::ReduceTask< opT >
Reduce task.
This task will reduce an arbitrary number of objects. It is optimized for reduction of data that is the result of other tasks or remote data. Also, it is assumed that individual reduction operations require a substantial amount of work (i.e. your reduction operation should reduce a vector of data, not two numbers). The reduction arguments are reduced as they become ready, which results in non-deterministic reduction order. This is much faster than a simple binary tree reduction since the reduction tasks do not have to wait for specific pairs of data. Though data that is not stored in a future can be used, it may not be the best choice in that case.
The reduction operation must have the following form:
For example, a vector sum function might look like:
- Note
- There is no need to add this object to the MADNESS task queue. It will be handled internally by the object. Simply call
submit()
to add this task to the task queue.
- Template Parameters
-
opT The reduction operation type
Definition at line 200 of file reduce_task.h.
Public Member Functions | |
ReduceTask () | |
Default constructor. More... | |
ReduceTask (World &world, const opT &op=opT(), madness::CallbackInterface *callback=nullptr) | |
Constructor. More... | |
ReduceTask (ReduceTask< opT > &&other) noexcept | |
Move constructor. More... | |
~ReduceTask () | |
Destructor. More... | |
ReduceTask< opT > & | operator= (ReduceTask< opT > &&other) noexcept |
Move assignment operator. More... | |
ReduceTask (const ReduceTask< opT > &)=delete | |
ReduceTask< opT > & | operator= (const ReduceTask< opT > &)=delete |
template<typename Arg > | |
int | add (const Arg &arg, madness::CallbackInterface *callback=nullptr) |
Add an argument to the reduction task. More... | |
int | count () const |
Argument count. More... | |
Future< result_type > | submit () |
Submit the reduction task to the task queue. More... | |
operator bool () const | |
Type conversion operator. More... | |
Constructor & Destructor Documentation
◆ ReduceTask() [1/4]
|
inline |
Default constructor.
Definition at line 734 of file reduce_task.h.
◆ ReduceTask() [2/4]
|
inline |
Constructor.
- Parameters
-
world The world that owns this task op The reduction operation [ default = opT() ] callback The callback that will be invoked when this task is complete
Definition at line 742 of file reduce_task.h.
◆ ReduceTask() [3/4]
|
inlinenoexcept |
Move constructor.
- Parameters
-
other The object to be moved
Definition at line 749 of file reduce_task.h.
◆ ~ReduceTask()
|
inline |
Destructor.
If the reduction has not been submitted or destroy()
has not been called, it will be submitted when the destructor is called.
Definition at line 759 of file reduce_task.h.
◆ ReduceTask() [4/4]
|
delete |
Member Function Documentation
◆ add()
|
inline |
Add an argument to the reduction task.
arg
may be of the argument type of opT
, a Future
to the argument type, or RemoteReference<FutureImpl>
to the argument type.
- Template Parameters
-
Arg The argument type
- Parameters
-
arg The argument that will be reduced callback The callback that will be invoked when this argument pair has been reduced [ default = nullptr ]
Definition at line 786 of file reduce_task.h.
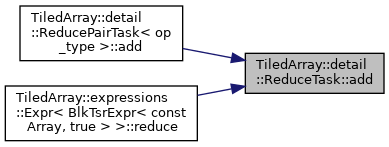
◆ count()
|
inline |
Argument count.
- Returns
- The total number of arguments added to this task
Definition at line 796 of file reduce_task.h.
◆ operator bool()
|
inline |
Type conversion operator.
- Returns
true
if the task object is initialized.
Definition at line 821 of file reduce_task.h.
◆ operator=() [1/2]
|
delete |
◆ operator=() [2/2]
|
inlinenoexcept |
Move assignment operator.
- Parameters
-
other The object to be moved
Definition at line 764 of file reduce_task.h.

◆ submit()
|
inline |
Submit the reduction task to the task queue.
- Returns
- The result of the reduction
- Note
- Arguments can no longer be added to the reduction after calling
submit()
.
Definition at line 803 of file reduce_task.h.

The documentation for this class was generated from the following file:
- TiledArray/reduce_task.h