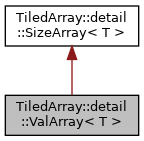
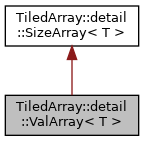
Documentation
template<typename T>
class TiledArray::detail::ValArray< T >
Value array.
This is minimal wrapper around a dynamically allocated array. The array may be shared and includes a reference counter.
- Template Parameters
-
T The element type of the array
Definition at line 44 of file val_array.h.
Public Types | |
typedef ValArray< T > | ValArray_ |
This object type. More... | |
typedef SizeArray< T >::size_type | size_type |
size type More... | |
typedef SizeArray< T >::value_type | value_type |
Element type. More... | |
typedef SizeArray< T >::reference | reference |
Reference type. More... | |
typedef SizeArray< T >::const_reference | const_reference |
Const reference type. More... | |
typedef SizeArray< T >::pointer | pointer |
Data pointer type. More... | |
typedef SizeArray< T >::const_pointer | const_pointer |
Const data pointer type. More... | |
typedef SizeArray< T >::difference_type | difference_type |
Difference type. More... | |
typedef SizeArray< T >::iterator | iterator |
Iterator type. More... | |
typedef SizeArray< T >::const_iterator | const_iterator |
Const iterator type. More... | |
Public Member Functions | |
ValArray ()=default | |
ValArray (const size_type n) | |
template<typename Value , typename std::enable_if< std::is_convertible< value_type, Value >::value >::type * = nullptr> | |
ValArray (const size_type n, const Value &value) | |
template<typename Arg > | |
ValArray (const size_type n, const Arg *const arg) | |
template<typename Arg , typename Op > | |
ValArray (const size_type n, const Arg *MADNESS_RESTRICT const arg, const Op &op) | |
template<typename U , typename Op > | |
ValArray (const ValArray< U > &arg, const Op &op) | |
template<typename Left , typename Right , typename Op > | |
ValArray (const size_type n, const Left *MADNESS_RESTRICT const left, const Right *MADNESS_RESTRICT const right, const Op &op) | |
template<typename U , typename V , typename Op > | |
ValArray (const ValArray< U > &left, const ValArray< V > &right, const Op &op) | |
ValArray (const ValArray_ &other) | |
ValArray_ & | operator= (const ValArray_ &other) |
~ValArray () | |
template<typename U , typename Op > | |
void | binary (const ValArray< U > &arg, const Op &op) |
Binary vector operation. More... | |
template<typename U , typename V , typename Op > | |
void | binary (const ValArray< U > &left, const ValArray< V > &right, const Op &op) |
Binary vector operation. More... | |
template<typename U , typename Op > | |
void | unary (const ValArray< U > &arg, const Op &op) |
Unary vector operation. More... | |
template<typename U , typename Result , typename Op > | |
Result | reduce (const ValArray< U > &arg, Result &result, const Op &op) |
Binary reduce operation. More... | |
template<typename U , typename V , typename Op > | |
void | row_reduce (const ValArray< U > &left, const ValArray< V > &right, const Op &op) |
Reduce row operation. More... | |
template<typename U , typename Op > | |
void | row_reduce (const ValArray< U > &arg, const Op &op) |
Reduce row operation. More... | |
template<typename U , typename V , typename Op > | |
void | col_reduce (const ValArray< U > &left, const ValArray< V > &right, const Op &op) |
Reduce column operation. More... | |
template<typename U , typename Op > | |
void | col_reduce (const ValArray< U > &arg, const Op &op) |
Reduce column operation. More... | |
template<typename U , typename V , typename Op > | |
void | outer (const ValArray< U > &left, const ValArray< V > &right, const Op &op) |
Outer operation. More... | |
template<typename U , typename V , typename Op > | |
void | outer_fill (const ValArray< U > &left, const ValArray< V > &right, const Op &op) |
Outer fill operation. More... | |
template<typename U , typename V , typename A , typename Op > | |
void | outer_fill (const ValArray< U > &left, const ValArray< V > &right, const ValArray< A > &a, const Op &op) |
Outer operation. More... | |
void | swap (ValArray_ &other) |
template<typename U > | |
bool | operator== (const ValArray< U > &other) const |
template<typename U > | |
bool | operator!= (const ValArray< U > &other) const |
template<typename Archive , typename = std::enable_if_t< madness::archive::is_output_archive<Archive>::value>> | |
void | serialize (Archive &ar) const |
Serialization. More... | |
template<typename Archive , typename = std::enable_if_t< madness::archive::is_input_archive<Archive>::value>> | |
void | serialize (Archive &ar) |
(De)serialization More... | |
Static Public Attributes | |
static const std::size_t | alignment = TILEDARRAY_DEFAULT_ALIGNMENT |
Member Typedef Documentation
◆ const_iterator
typedef SizeArray<T>::const_iterator TiledArray::detail::ValArray< T >::const_iterator |
Const iterator type.
Definition at line 59 of file val_array.h.
◆ const_pointer
typedef SizeArray<T>::const_pointer TiledArray::detail::ValArray< T >::const_pointer |
Const data pointer type.
Definition at line 54 of file val_array.h.
◆ const_reference
typedef SizeArray<T>::const_reference TiledArray::detail::ValArray< T >::const_reference |
Const reference type.
Definition at line 51 of file val_array.h.
◆ difference_type
typedef SizeArray<T>::difference_type TiledArray::detail::ValArray< T >::difference_type |
Difference type.
Definition at line 56 of file val_array.h.
◆ iterator
typedef SizeArray<T>::iterator TiledArray::detail::ValArray< T >::iterator |
Iterator type.
Definition at line 57 of file val_array.h.
◆ pointer
typedef SizeArray<T>::pointer TiledArray::detail::ValArray< T >::pointer |
Data pointer type.
Definition at line 52 of file val_array.h.
◆ reference
typedef SizeArray<T>::reference TiledArray::detail::ValArray< T >::reference |
Reference type.
Definition at line 49 of file val_array.h.
◆ size_type
typedef SizeArray<T>::size_type TiledArray::detail::ValArray< T >::size_type |
size type
Definition at line 47 of file val_array.h.
◆ ValArray_
typedef ValArray<T> TiledArray::detail::ValArray< T >::ValArray_ |
This object type.
Definition at line 46 of file val_array.h.
◆ value_type
typedef SizeArray<T>::value_type TiledArray::detail::ValArray< T >::value_type |
Element type.
Definition at line 48 of file val_array.h.
Constructor & Destructor Documentation
◆ ValArray() [1/9]
|
default |
◆ ValArray() [2/9]
|
inlineexplicit |
Definition at line 122 of file val_array.h.
◆ ValArray() [3/9]
|
inline |
◆ ValArray() [4/9]
|
inline |
◆ ValArray() [5/9]
|
inline |
◆ ValArray() [6/9]
|
inline |
◆ ValArray() [7/9]
|
inline |
◆ ValArray() [8/9]
|
inline |
◆ ValArray() [9/9]
|
inline |
Definition at line 170 of file val_array.h.
◆ ~ValArray()
|
inline |
Definition at line 198 of file val_array.h.
Member Function Documentation
◆ binary() [1/2]
|
inline |
Binary vector operation.
Perform a binary vector operation where this array is the left-hand argument and arg is the right-hand argument. The data elements are modified by: op(*this[i], arg[i])
.
- Template Parameters
-
U The element type of arg
Op The binary operation
- Parameters
-
arg The right-hand argument op The binary operation
- Exceptions
-
TiledArray::Exception When the size of arg
is not equal to the size of this array.
Definition at line 237 of file val_array.h.

◆ binary() [2/2]
|
inline |
Binary vector operation.
Perform a binary vector operation with left
and right
, and store the result in this array. The data elements are given by: *this[i] = op(left[i], right[i])
.
- Template Parameters
-
U The element type of arg
Op The binary operation
- Parameters
-
left The left-hand argument right The right-hand argument op The binary operation
- Exceptions
-
TiledArray::Exception When the sizes of left and right are not equal to the size of this array.
Definition at line 255 of file val_array.h.

◆ col_reduce() [1/2]
|
inline |
Reduce column operation.
Reduce columns of arg
to this array, where a columns have arg.size()/size
() elements. The reduced result is computed by op(*this[i], arg[i][j])
.
- Template Parameters
-
U The element type of arg
Op The reduction operation
- Parameters
-
arg The array to be reduced op The reduction operation
- Exceptions
-
TiledArray::Exception When (arg.size() % size()) != 0
.
Definition at line 363 of file val_array.h.

◆ col_reduce() [2/2]
|
inline |
Reduce column operation.
Reduce columns of left
to this array, where columns have right.size()
elements. The reduced result is computed by op(*this[j], left[i][j], right[i])
.
- Template Parameters
-
U The element type of left
V The element type of right
Op The reduction operation
- Parameters
-
left The array to be reduced of size size()*right
.size()right The right-hand array op The reduction operation
- Exceptions
-
TiledArray::Exception When left.size() != (size() * right.size())
.
Definition at line 346 of file val_array.h.

◆ operator!=()
|
inline |
◆ operator=()
|
inline |
◆ operator==()
|
inline |
◆ outer()
|
inline |
Outer operation.
This function use two arrays, left
and right
, to modify this array ( which is treated as a matrix of size left.size() * right.size()
). Elements of this array are modified by op(*this[i][j], left[i], right[j])
.
- Template Parameters
-
U The left-hand argument type V The right-hand argument type
- Parameters
-
left The left-hand array right The right-hand array op The outer operation
- Exceptions
-
TiledArray::Exception When size() != (left.size() * right.size())
.
Definition at line 382 of file val_array.h.
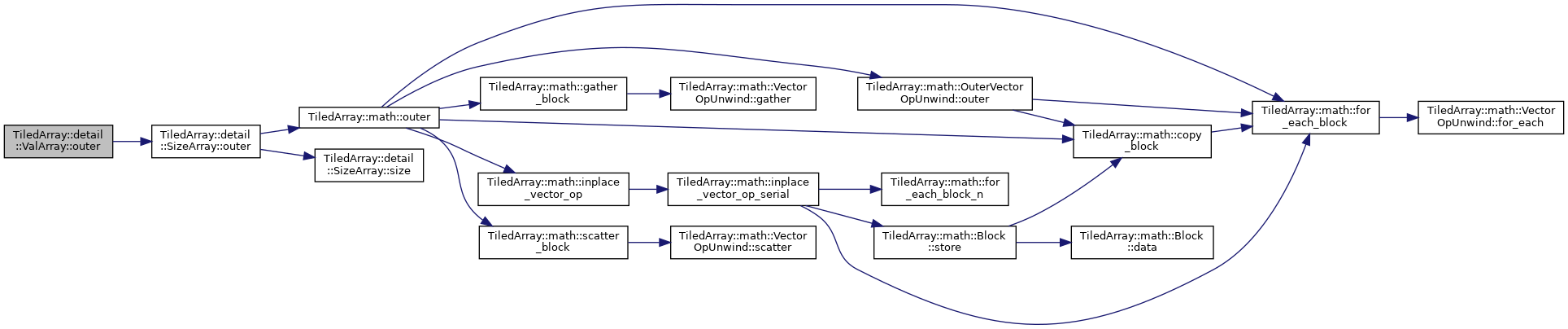
◆ outer_fill() [1/2]
|
inline |
Outer fill operation.
This function use two arrays, left
and right
, to fill this array ( which is treated as a matrix of size left.size() * right.size()
). Elements of this array are filled by op(*this[i][j], left[i], right[j])
.
- Template Parameters
-
U The left-hand argument type V The right-hand argument type
- Parameters
-
left The left-hand array right The right-hand array op The outer operation
- Exceptions
-
TiledArray::Exception When size() != (left.size() * right.size())
.
Definition at line 402 of file val_array.h.

◆ outer_fill() [2/2]
|
inline |
Outer operation.
This function use two arrays, left
and right
, to modify this array ( which is treated as a matrix of size left.size() * right.size()
). Elements of this array are modified by op(*this[i][j], left[i], right[j])
.
- Template Parameters
-
U The left-hand argument type V The right-hand argument type
- Parameters
-
[in] left The left-hand array [in] right The right-hand array [out] a The array that will hold the result [in] op The outer operation
- Exceptions
-
TiledArray::Exception When size() != (left.size() * right.size())
.
Definition at line 424 of file val_array.h.

◆ reduce()
|
inline |
Binary reduce operation.
Binary reduction operation where this object is the left-hand argument type. The reduced result is computed by op(result, *this[i], arg[i])
.
- Template Parameters
-
U The element type of arg
Result The result type of the reduction Op The reduction operation.
- Parameters
-
arg The right-hand array argument result The initial value of the reduction op The binary reduction operation
- Returns
- The reduced value
- Exceptions
-
TiledArray::Exception When arg.size() != size()
.
Definition at line 291 of file val_array.h.

◆ row_reduce() [1/2]
|
inline |
Reduce row operation.
Reduce rows of arg
to this array, where a row have arg.size()/size
() elements. The reduced result is computed by op(*this[i], arg[i][j])
.
- Template Parameters
-
U The element type of arg
Op The reduction operation
- Parameters
-
arg The array to be reduced op The reduction operation
- Exceptions
-
TiledArray::Exception When (arg.size() % size()) != 0
.
Definition at line 327 of file val_array.h.

◆ row_reduce() [2/2]
|
inline |
Reduce row operation.
Reduce rows of left
to this array, where the size of rows is equal to right.size()
. The reduced result is computed by op(*this[i], left[i][j], right[j])
.
- Template Parameters
-
U The element type of left
V The element type of right
Op The reduction operation
- Parameters
-
left The array to be reduced of size size()*right
.size()right The right-hand array op The reduction operation
- Exceptions
-
TiledArray::Exception When left.size() != (size() * right.size())
.
Definition at line 310 of file val_array.h.

◆ serialize() [1/2]
|
inline |
(De)serialization
- Template Parameters
-
Archive An input archive type
- Parameters
-
[out] ar an Archive object
Definition at line 470 of file val_array.h.
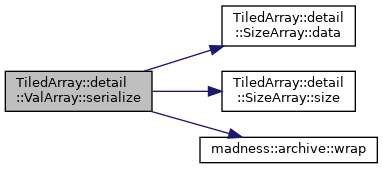
◆ serialize() [2/2]
|
inline |
Serialization.
- Template Parameters
-
Archive An output archive type
- Parameters
-
[out] ar an Archive object
Definition at line 458 of file val_array.h.
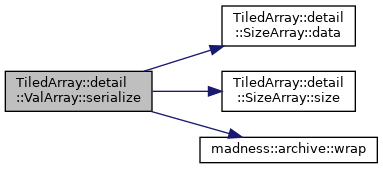
◆ swap()
|
inline |
◆ unary()
|
inline |
Unary vector operation.
Perform a unary vector operation, and store the result in this array. The data elements are given by: *this[i] = op(arg[i])
.
- Template Parameters
-
U The element type of arg
Op The binary operation
- Parameters
-
arg The right-hand argument op The binary operation
- Exceptions
-
TiledArray::Exception When the size of arg
is not equal to the size of this array.
Definition at line 272 of file val_array.h.

Member Data Documentation
◆ alignment
|
static |
Definition at line 61 of file val_array.h.
The documentation for this class was generated from the following file:
- TiledArray/val_array.h