Documentation
template<typename Array, bool Alias>
class TiledArray::expressions::TsrExpr< Array, Alias >
Expression wrapper for array objects.
- Template Parameters
-
Array The TiledArray::Array
typeAlias If true, the array tiles should be evaluated as temporaries before assignment; if false, can reuse the result tiles
Definition at line 83 of file tsr_expr.h.
Public Types | |
typedef TsrExpr< Array, Alias > | TsrExpr_ |
This class type. More... | |
typedef Expr< TsrExpr_ > | Expr_ |
Base class type. More... | |
typedef ExprTrait< TsrExpr_ >::array_type | array_type |
The array type. More... | |
typedef ExprTrait< TsrExpr_ >::engine_type | engine_type |
Expression engine type. More... | |
using | index1_type = TA_1INDEX_TYPE |
Public Member Functions | |
TsrExpr ()=default | |
TsrExpr (const TsrExpr_ &)=default | |
TsrExpr (TsrExpr_ &&)=default | |
~TsrExpr ()=default | |
TsrExpr (array_type &array, const std::string &annotation) | |
Constructor. More... | |
array_type & | operator= (TsrExpr_ &other) |
Expression assignment operator. More... | |
template<typename D > | |
array_type & | operator= (const Expr< D > &other) |
Expression assignment operator. More... | |
template<typename D > | |
array_type & | operator+= (const Expr< D > &other) |
Expression plus-assignment operator. More... | |
template<typename D > | |
array_type & | operator-= (const Expr< D > &other) |
Expression minus-assignment operator. More... | |
template<typename D > | |
array_type & | operator*= (const Expr< D > &other) |
Expression multiply-assignment operator. More... | |
array_type & | array () const |
Array accessor. More... | |
TsrExpr< Array, false > | no_alias () const |
Flag this tensor expression for a non-aliasing assignment. More... | |
template<typename Index1 , typename Index2 , typename = std::enable_if_t< TiledArray::detail::is_integral_range_v<Index1> && TiledArray::detail::is_integral_range_v<Index2>>> | |
BlkTsrExpr< const Array, Alias > | block (const Index1 &lower_bound, const Index2 &upper_bound) const |
immutable Block expression factory More... | |
template<typename Index1 , typename Index2 , typename = std::enable_if_t<std::is_integral_v<Index1> && std::is_integral_v<Index2>>> | |
BlkTsrExpr< const Array, Alias > | block (const std::initializer_list< Index1 > &lower_bound, const std::initializer_list< Index2 > &upper_bound) const |
immutable Block expression factory More... | |
template<typename PairRange , typename = std::enable_if_t< TiledArray::detail::is_gpair_range_v<PairRange>>> | |
BlkTsrExpr< const Array, Alias > | block (const PairRange &bounds) const |
immutable Block expression factory More... | |
template<typename Index , typename = std::enable_if_t<std::is_integral_v<Index>>> | |
BlkTsrExpr< const Array, Alias > | block (const std::initializer_list< std::initializer_list< Index >> &bounds) const |
immutable Block expression factory More... | |
template<typename Index1 , typename Index2 , typename = std::enable_if_t< TiledArray::detail::is_integral_range_v<Index1> && TiledArray::detail::is_integral_range_v<Index2>>> | |
BlkTsrExpr< Array, Alias > | block (const Index1 &lower_bound, const Index2 &upper_bound) |
mutable Block expression factory More... | |
template<typename Index1 , typename Index2 , typename = std::enable_if_t<std::is_integral_v<Index1> && std::is_integral_v<Index2>>> | |
BlkTsrExpr< Array, Alias > | block (const std::initializer_list< Index1 > &lower_bound, const std::initializer_list< Index2 > &upper_bound) |
mutable Block expression factory More... | |
template<typename PairRange , typename = std::enable_if_t< TiledArray::detail::is_gpair_range_v<PairRange>>> | |
BlkTsrExpr< Array, Alias > | block (const PairRange &bounds) |
mutable Block expression factory More... | |
template<typename Index , typename = std::enable_if_t<std::is_integral_v<Index>>> | |
BlkTsrExpr< Array, Alias > | block (const std::initializer_list< std::initializer_list< Index >> &bounds) |
mutable Block expression factory More... | |
ConjTsrExpr< Array > | conj () const |
Conjugated-tensor expression factor. More... | |
const std::string & | annotation () const |
Tensor annotation accessor. More... | |
Member Typedef Documentation
◆ array_type
typedef ExprTrait<TsrExpr_>::array_type TiledArray::expressions::TsrExpr< Array, Alias >::array_type |
The array type.
Definition at line 88 of file tsr_expr.h.
◆ engine_type
typedef ExprTrait<TsrExpr_>::engine_type TiledArray::expressions::TsrExpr< Array, Alias >::engine_type |
Expression engine type.
Definition at line 90 of file tsr_expr.h.
◆ Expr_
typedef Expr<TsrExpr_> TiledArray::expressions::TsrExpr< Array, Alias >::Expr_ |
Base class type.
Definition at line 86 of file tsr_expr.h.
◆ index1_type
using TiledArray::expressions::TsrExpr< Array, Alias >::index1_type = TA_1INDEX_TYPE |
Definition at line 91 of file tsr_expr.h.
◆ TsrExpr_
typedef TsrExpr<Array, Alias> TiledArray::expressions::TsrExpr< Array, Alias >::TsrExpr_ |
This class type.
Definition at line 85 of file tsr_expr.h.
Constructor & Destructor Documentation
◆ TsrExpr() [1/4]
|
default |
◆ TsrExpr() [2/4]
|
default |
◆ TsrExpr() [3/4]
|
default |
◆ ~TsrExpr()
|
default |
◆ TsrExpr() [4/4]
|
inline |
Constructor.
- Parameters
-
array The array object annotation The annotation for array
Definition at line 108 of file tsr_expr.h.
Member Function Documentation
◆ annotation()
|
inline |
Tensor annotation accessor.
- Returns
- A const reference to the annotation for this tensor
Definition at line 304 of file tsr_expr.h.
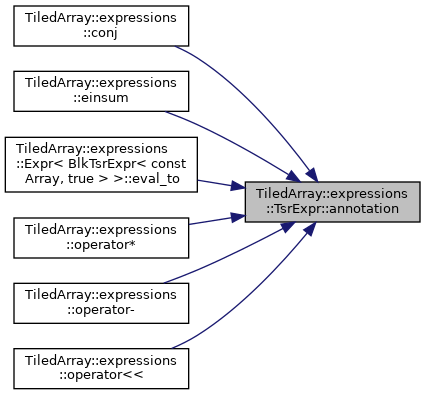
◆ array()
|
inline |
Array accessor.
- Returns
- a const reference to this array
Definition at line 175 of file tsr_expr.h.
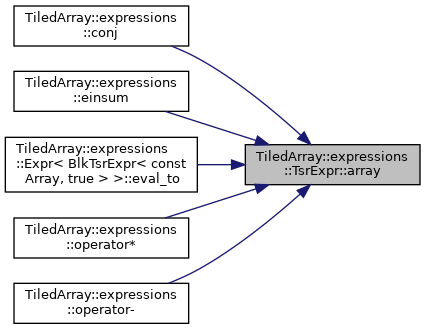
◆ block() [1/8]
|
inline |
mutable Block expression factory
- Template Parameters
-
Index1 An integral range type Index2 An integral range type
- Parameters
-
lower_bound The lower_bound of the block upper_bound The upper_bound of the block
Definition at line 249 of file tsr_expr.h.
◆ block() [2/8]
|
inline |
immutable Block expression factory
- Template Parameters
-
Index1 An integral range type Index2 An integral range type
- Parameters
-
lower_bound The lower_bound of the block upper_bound The upper_bound of the block
Definition at line 194 of file tsr_expr.h.
◆ block() [3/8]
|
inline |
mutable Block expression factory
- Template Parameters
-
PairRange Type representing a range of generalized pairs (see TiledArray::detail::is_gpair_v )
- Parameters
-
bounds The {lower,upper} bounds of the block
Definition at line 279 of file tsr_expr.h.
◆ block() [4/8]
|
inline |
immutable Block expression factory
- Template Parameters
-
PairRange Type representing a range of generalized pairs (see TiledArray::detail::is_gpair_v )
- Parameters
-
bounds The {lower,upper} bounds of the block
Definition at line 224 of file tsr_expr.h.
◆ block() [5/8]
|
inline |
mutable Block expression factory
- Template Parameters
-
Index1 An integral type Index2 An integral type
- Parameters
-
lower_bound The lower_bound of the block upper_bound The upper_bound of the block
Definition at line 264 of file tsr_expr.h.
◆ block() [6/8]
|
inline |
immutable Block expression factory
- Template Parameters
-
Index1 An integral type Index2 An integral type
- Parameters
-
lower_bound The lower_bound of the block upper_bound The upper_bound of the block
Definition at line 209 of file tsr_expr.h.
◆ block() [7/8]
|
inline |
mutable Block expression factory
- Template Parameters
-
Index An integral type
- Parameters
-
bounds The {lower,upper} bounds of the block
Definition at line 289 of file tsr_expr.h.
◆ block() [8/8]
|
inline |
immutable Block expression factory
- Template Parameters
-
Index An integral type
- Parameters
-
bounds The {lower,upper} bounds of the block
Definition at line 234 of file tsr_expr.h.
◆ conj()
|
inline |
Conjugated-tensor expression factor.
- Returns
- A conjugated expression object
Definition at line 297 of file tsr_expr.h.

◆ no_alias()
|
inline |
Flag this tensor expression for a non-aliasing assignment.
- Returns
- A non-aliased tensor expression
Definition at line 180 of file tsr_expr.h.
◆ operator*=()
|
inline |
Expression multiply-assignment operator.
- Template Parameters
-
D The derived expression type
- Parameters
-
other The expression that will scale this array
Definition at line 164 of file tsr_expr.h.
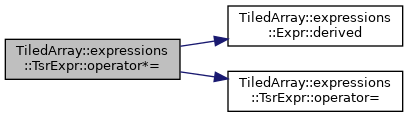
◆ operator+=()
|
inline |
Expression plus-assignment operator.
- Template Parameters
-
D The derived expression type
- Parameters
-
other The expression that will be added to this array
Definition at line 138 of file tsr_expr.h.
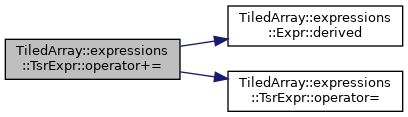
◆ operator-=()
|
inline |
Expression minus-assignment operator.
- Template Parameters
-
D The derived expression type
- Parameters
-
other The expression that will be subtracted from this array
Definition at line 151 of file tsr_expr.h.
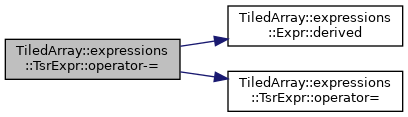
◆ operator=() [1/2]
|
inline |
Expression assignment operator.
- Template Parameters
-
D The derived expression type
- Parameters
-
other The expression that will be assigned to this array
Definition at line 124 of file tsr_expr.h.

◆ operator=() [2/2]
|
inline |
Expression assignment operator.
- Parameters
-
other The expression that will be assigned to this array
Definition at line 114 of file tsr_expr.h.
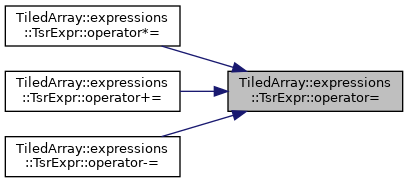
The documentation for this class was generated from the following files:
- TiledArray/dist_array.h
- TiledArray/expressions/tsr_expr.h