Documentation
template<typename T, typename A>
class TiledArray::Tensor< T, A >
An N-dimensional tensor object.
- Template Parameters
-
T the value type of this tensor A The allocator type for the data
Public Types | |
typedef Tensor< T, A > | Tensor_ |
This class type. More... | |
typedef Range | range_type |
Tensor range type. More... | |
typedef range_type::index1_type | index1_type |
1-index type More... | |
typedef range_type::ordinal_type | ordinal_type |
Ordinal type. More... | |
typedef range_type::ordinal_type | size_type |
Size type (to meet the container concept) More... | |
typedef A | allocator_type |
Allocator type. More... | |
typedef allocator_type::value_type | value_type |
Array element type. More... | |
typedef allocator_type::reference | reference |
Element reference type. More... | |
typedef allocator_type::const_reference | const_reference |
Element reference type. More... | |
typedef allocator_type::pointer | pointer |
Element pointer type. More... | |
typedef allocator_type::const_pointer | const_pointer |
Element const pointer type. More... | |
typedef allocator_type::difference_type | difference_type |
Difference type. More... | |
typedef pointer | iterator |
Element iterator type. More... | |
typedef const_pointer | const_iterator |
Element const iterator type. More... | |
typedef TiledArray::detail::numeric_type< T >::type | numeric_type |
the numeric type that supports T More... | |
typedef TiledArray::detail::scalar_type< T >::type | scalar_type |
the scalar type that supports T More... | |
Public Member Functions | |
Tensor () | |
Tensor (const Tensor_ &other) | |
Tensor (Tensor_ &&other) | |
~Tensor () | |
Tensor_ & | operator= (const Tensor_ &other) |
Tensor_ & | operator= (Tensor_ &&other) |
Tensor (const range_type &range) | |
Construct tensor. More... | |
template<typename Value , typename std::enable_if< std::is_same< Value, value_type >::value &&detail::is_tensor< Value >::value >::type * = nullptr> | |
Tensor (const range_type &range, const Value &value) | |
Construct a tensor with a fill value. More... | |
template<typename Value , typename std::enable_if< detail::is_numeric_v< Value >>::type * = nullptr> | |
Tensor (const range_type &range, const Value &value) | |
Construct a tensor with a fill value. More... | |
template<typename InIter , typename std::enable_if< TiledArray::detail::is_input_iterator< InIter >::value &&!std::is_pointer< InIter >::value >::type * = nullptr> | |
Tensor (const range_type &range, InIter it) | |
Construct an evaluated tensor. More... | |
template<typename U > | |
Tensor (const Range &range, const U *u) | |
Tensor (const Range &range, std::initializer_list< T > il) | |
template<typename T1 , typename std::enable_if< is_tensor< T1 >::value &&!std::is_same< T1, Tensor_ >::value &&!detail::has_conversion_operator_v< T1, Tensor_ >>::type * = nullptr> | |
Tensor (const T1 &other) | |
Construct a copy of a tensor interface object. More... | |
template<typename T1 , typename Perm , typename std::enable_if< is_tensor< T1 >::value &&detail::is_permutation_v< Perm >>::type * = nullptr> | |
Tensor (const T1 &other, const Perm &perm) | |
Construct a permuted tensor copy. More... | |
template<typename T1 , typename Op , typename std::enable_if_t< is_tensor< T1 >::value &&!detail::is_permutation_v< std::decay_t< Op >>> * = nullptr> | |
Tensor (const T1 &other, Op &&op) | |
Copy and modify the data from other . More... | |
template<typename T1 , typename Op , typename Perm , typename std::enable_if_t< is_tensor< T1 >::value &&detail::is_permutation_v< Perm >> * = nullptr> | |
Tensor (const T1 &other, Op &&op, const Perm &perm) | |
Copy, modify, and permute the data from other . More... | |
template<typename T1 , typename T2 , typename Op , typename std::enable_if< is_tensor< T1, T2 >::value >::type * = nullptr> | |
Tensor (const T1 &left, const T2 &right, Op &&op) | |
Copy and modify the data from left , and right . More... | |
template<typename T1 , typename T2 , typename Op , typename Perm , typename std::enable_if< is_tensor< T1, T2 >::value &&detail::is_permutation_v< Perm >>::type * = nullptr> | |
Tensor (const T1 &left, const T2 &right, Op &&op, const Perm &perm) | |
Copy, modify, and permute the data from left , and right . More... | |
Tensor_ | clone () const |
template<typename T1 , typename std::enable_if< is_tensor< T1 >::value >::type * = nullptr> | |
Tensor_ & | operator= (const T1 &other) |
const range_type & | range () const |
Tensor range object accessor. More... | |
range_type & | range () |
Tensor range object mutable accessor. More... | |
ordinal_type | size () const |
Tensor dimension size accessor. More... | |
template<typename Ordinal , std::enable_if_t< std::is_integral< Ordinal >::value > * = nullptr> | |
const_reference | operator[] (const Ordinal ord) const |
Const element accessor. More... | |
template<typename Ordinal , std::enable_if_t< std::is_integral< Ordinal >::value > * = nullptr> | |
reference | operator[] (const Ordinal ord) |
Element accessor. More... | |
template<typename Index , std::enable_if_t< detail::is_integral_range_v< Index >> * = nullptr> | |
const_reference | operator[] (const Index &i) const |
Const element accessor. More... | |
template<typename Index , std::enable_if_t< detail::is_integral_range_v< Index >> * = nullptr> | |
reference | operator[] (const Index &i) |
Element accessor. More... | |
template<typename Integer , std::enable_if_t< std::is_integral_v< Integer >> * = nullptr> | |
const_reference | operator[] (const std::initializer_list< Integer > &i) const |
Const element accessor. More... | |
template<typename Integer , std::enable_if_t< std::is_integral_v< Integer >> * = nullptr> | |
reference | operator[] (const std::initializer_list< Integer > &i) |
Element accessor. More... | |
template<typename Index , std::enable_if_t< detail::is_integral_range_v< Index >> * = nullptr> | |
const_reference | operator() (const Index &i) const |
Const element accessor. More... | |
template<typename Index , std::enable_if_t< detail::is_integral_range_v< Index >> * = nullptr> | |
reference | operator() (const Index &i) |
Element accessor. More... | |
template<typename Integer , std::enable_if_t< std::is_integral_v< Integer >> * = nullptr> | |
const_reference | operator() (const std::initializer_list< Integer > &i) const |
Const element accessor. More... | |
template<typename Integer , std::enable_if_t< std::is_integral_v< Integer >> * = nullptr> | |
reference | operator() (const std::initializer_list< Integer > &i) |
Element accessor. More... | |
template<typename... Index, std::enable_if_t< detail::is_integral_list< Index... >::value > * = nullptr> | |
const_reference | operator() (const Index &... i) const |
Const element accessor. More... | |
template<typename... Index, std::enable_if_t< detail::is_integral_list< Index... >::value > * = nullptr> | |
reference | operator() (const Index &... i) |
Element accessor. More... | |
const_iterator | begin () const |
Iterator factory. More... | |
iterator | begin () |
Iterator factory. More... | |
const_iterator | end () const |
Iterator factory. More... | |
iterator | end () |
Iterator factory. More... | |
const_pointer | data () const |
Data direct access. More... | |
pointer | data () |
Data direct access. More... | |
bool | empty () const |
Test if the tensor is empty. More... | |
template<typename Archive , typename std::enable_if< madness::archive::is_output_archive< Archive >::value >::type * = nullptr> | |
void | serialize (Archive &ar) |
Output serialization function. More... | |
template<typename Archive , typename std::enable_if< madness::archive::is_input_archive< Archive >::value >::type * = nullptr> | |
void | serialize (Archive &ar) |
Input serialization function. More... | |
void | swap (Tensor_ &other) |
Swap tensor data. More... | |
template<typename Perm , typename = std::enable_if_t<detail::is_permutation_v<Perm>>> | |
Tensor_ | permute (const Perm &perm) const |
Create a permuted copy of this tensor. More... | |
template<typename Index , std::enable_if_t< detail::is_integral_range_v< Index >> * = nullptr> | |
Tensor_ & | shift_to (const Index &bound_shift) |
Shift the lower and upper bound of this tensor. More... | |
template<typename Integer , std::enable_if_t< std::is_integral_v< Integer >> * = nullptr> | |
Tensor_ & | shift_to (const std::initializer_list< Integer > &bound_shift) |
Shift the lower and upper bound of this tensor. More... | |
template<typename Index , std::enable_if_t< detail::is_integral_range_v< Index >> * = nullptr> | |
Tensor_ | shift (const Index &bound_shift) const |
Shift the lower and upper bound of this range. More... | |
template<typename Integer , std::enable_if_t< std::is_integral_v< Integer >> * = nullptr> | |
Tensor_ | shift (const std::initializer_list< Integer > &bound_shift) const |
Shift the lower and upper bound of this range. More... | |
template<typename Right , typename Op , typename std::enable_if< is_tensor< Right >::value >::type * = nullptr> | |
Tensor_ | binary (const Right &right, Op &&op) const |
Use a binary, element wise operation to construct a new tensor. More... | |
template<typename Right , typename Op , typename Perm , typename std::enable_if< is_tensor< Right >::value &&detail::is_permutation_v< Perm >>::type * = nullptr> | |
Tensor_ | binary (const Right &right, Op &&op, const Perm &perm) const |
Use a binary, element wise operation to construct a new, permuted tensor. More... | |
template<typename Right , typename Op , typename std::enable_if< is_tensor< Right >::value >::type * = nullptr> | |
Tensor_ & | inplace_binary (const Right &right, Op &&op) |
Use a binary, element wise operation to modify this tensor. More... | |
template<typename Op > | |
Tensor_ | unary (Op &&op) const |
Use a unary, element wise operation to construct a new tensor. More... | |
template<typename Op , typename Perm , typename = std::enable_if_t<detail::is_permutation_v<Perm>>> | |
Tensor_ | unary (Op &&op, const Perm &perm) const |
Use a unary, element wise operation to construct a new, permuted tensor. More... | |
template<typename Op > | |
Tensor_ & | inplace_unary (Op &&op) |
Use a unary, element wise operation to modify this tensor. More... | |
template<typename Scalar , typename std::enable_if< detail::is_numeric_v< Scalar >>::type * = nullptr> | |
Tensor_ | scale (const Scalar factor) const |
Construct a scaled copy of this tensor. More... | |
template<typename Scalar , typename Perm , typename = std::enable_if_t<detail::is_numeric_v<Scalar> && detail::is_permutation_v<Perm>>> | |
Tensor_ | scale (const Scalar factor, const Perm &perm) const |
Construct a scaled and permuted copy of this tensor. More... | |
template<typename Scalar , typename std::enable_if< detail::is_numeric_v< Scalar >>::type * = nullptr> | |
Tensor_ & | scale_to (const Scalar factor) |
Scale this tensor. More... | |
template<typename Right , typename std::enable_if< is_tensor< Right >::value >::type * = nullptr> | |
Tensor_ | add (const Right &right) const |
Add this and other to construct a new tensors. More... | |
template<typename Right , typename Perm , typename std::enable_if< is_tensor< Right >::value &&detail::is_permutation_v< Perm >>::type * = nullptr> | |
Tensor_ | add (const Right &right, const Perm &perm) const |
Add this and other to construct a new, permuted tensor. More... | |
template<typename Right , typename Scalar , typename std::enable_if< is_tensor< Right >::value &&detail::is_numeric_v< Scalar >>::type * = nullptr> | |
Tensor_ | add (const Right &right, const Scalar factor) const |
Scale and add this and other to construct a new tensor. More... | |
template<typename Right , typename Scalar , typename Perm , typename std::enable_if< is_tensor< Right >::value &&detail::is_numeric_v< Scalar > &&detail::is_permutation_v< Perm >>::type * = nullptr> | |
Tensor_ | add (const Right &right, const Scalar factor, const Perm &perm) const |
Scale and add this and other to construct a new, permuted tensor. More... | |
Tensor_ | add (const numeric_type value) const |
Add a constant to a copy of this tensor. More... | |
template<typename Perm , typename = std::enable_if_t<detail::is_permutation_v<Perm>>> | |
Tensor_ | add (const numeric_type value, const Perm &perm) const |
Add a constant to a permuted copy of this tensor. More... | |
template<typename Right , typename std::enable_if< is_tensor< Right >::value >::type * = nullptr> | |
Tensor_ & | add_to (const Right &right) |
Add other to this tensor. More... | |
template<typename Right , typename Scalar , typename std::enable_if< is_tensor< Right >::value &&detail::is_numeric_v< Scalar >>::type * = nullptr> | |
Tensor_ & | add_to (const Right &right, const Scalar factor) |
Add other to this tensor, and scale the result. More... | |
Tensor_ & | add_to (const numeric_type value) |
Add a constant to this tensor. More... | |
template<typename Right , typename std::enable_if< is_tensor< Right >::value >::type * = nullptr> | |
Tensor_ | subt (const Right &right) const |
Subtract right from this and return the result. More... | |
template<typename Right , typename Perm , typename std::enable_if< is_tensor< Right >::value &&detail::is_permutation_v< Perm >>::type * = nullptr> | |
Tensor_ | subt (const Right &right, const Perm &perm) const |
Subtract right from this and return the result permuted by perm . More... | |
template<typename Right , typename Scalar , typename std::enable_if< is_tensor< Right >::value &&detail::is_numeric_v< Scalar >>::type * = nullptr> | |
Tensor_ | subt (const Right &right, const Scalar factor) const |
template<typename Right , typename Scalar , typename Perm , typename std::enable_if< is_tensor< Right >::value &&detail::is_numeric_v< Scalar > &&detail::is_permutation_v< Perm >>::type * = nullptr> | |
Tensor_ | subt (const Right &right, const Scalar factor, const Perm &perm) const |
Tensor_ | subt (const numeric_type value) const |
Subtract a constant from a copy of this tensor. More... | |
template<typename Perm , typename = std::enable_if_t<detail::is_permutation_v<Perm>>> | |
Tensor_ | subt (const numeric_type value, const Perm &perm) const |
Subtract a constant from a permuted copy of this tensor. More... | |
template<typename Right , typename std::enable_if< is_tensor< Right >::value >::type * = nullptr> | |
Tensor_ & | subt_to (const Right &right) |
Subtract right from this tensor. More... | |
template<typename Right , typename Scalar , typename std::enable_if< is_tensor< Right >::value &&detail::is_numeric_v< Scalar >>::type * = nullptr> | |
Tensor_ & | subt_to (const Right &right, const Scalar factor) |
Subtract right from and scale this tensor. More... | |
Tensor_ & | subt_to (const numeric_type value) |
Subtract a constant from this tensor. More... | |
template<typename Right , typename std::enable_if< is_tensor< Right >::value >::type * = nullptr> | |
Tensor_ | mult (const Right &right) const |
Multiply this by right to create a new tensor. More... | |
template<typename Right , typename Perm , typename std::enable_if< is_tensor< Right >::value &&detail::is_permutation_v< Perm >>::type * = nullptr> | |
Tensor_ | mult (const Right &right, const Perm &perm) const |
Multiply this by right to create a new, permuted tensor. More... | |
template<typename Right , typename Scalar , typename std::enable_if< is_tensor< Right >::value &&detail::is_numeric_v< Scalar >>::type * = nullptr> | |
Tensor_ | mult (const Right &right, const Scalar factor) const |
Scale and multiply this by right to create a new tensor. More... | |
template<typename Right , typename Scalar , typename Perm , typename std::enable_if< is_tensor< Right >::value &&detail::is_numeric_v< Scalar > &&detail::is_permutation_v< Perm >>::type * = nullptr> | |
Tensor_ | mult (const Right &right, const Scalar factor, const Perm &perm) const |
Scale and multiply this by right to create a new, permuted tensor. More... | |
template<typename Right , typename std::enable_if< is_tensor< Right >::value >::type * = nullptr> | |
Tensor_ & | mult_to (const Right &right) |
Multiply this tensor by right . More... | |
template<typename Right , typename Scalar , typename std::enable_if< is_tensor< Right >::value &&detail::is_numeric_v< Scalar >>::type * = nullptr> | |
Tensor_ & | mult_to (const Right &right, const Scalar factor) |
Scale and multiply this tensor by right . More... | |
Tensor_ | neg () const |
Create a negated copy of this tensor. More... | |
template<typename Perm , typename = std::enable_if_t<detail::is_permutation_v<Perm>>> | |
Tensor_ | neg (const Perm &perm) const |
Create a negated and permuted copy of this tensor. More... | |
Tensor_ & | neg_to () |
Negate elements of this tensor. More... | |
Tensor_ | conj () const |
Create a complex conjugated copy of this tensor. More... | |
template<typename Scalar , typename std::enable_if< detail::is_numeric_v< Scalar >>::type * = nullptr> | |
Tensor_ | conj (const Scalar factor) const |
Create a complex conjugated and scaled copy of this tensor. More... | |
template<typename Perm , typename = std::enable_if_t<detail::is_permutation_v<Perm>>> | |
Tensor_ | conj (const Perm &perm) const |
Create a complex conjugated and permuted copy of this tensor. More... | |
template<typename Scalar , typename Perm , typename std::enable_if< detail::is_numeric_v< Scalar > &&detail::is_permutation_v< Perm >>::type * = nullptr> | |
Tensor_ | conj (const Scalar factor, const Perm &perm) const |
Create a complex conjugated, scaled, and permuted copy of this tensor. More... | |
Tensor_ & | conj_to () |
Complex conjugate this tensor. More... | |
template<typename Scalar , typename std::enable_if< detail::is_numeric_v< Scalar >>::type * = nullptr> | |
Tensor_ & | conj_to (const Scalar factor) |
Complex conjugate and scale this tensor. More... | |
template<typename U , typename AU , typename V > | |
Tensor_ | gemm (const Tensor< U, AU > &other, const V factor, const math::GemmHelper &gemm_helper) const |
Contract this tensor with other . More... | |
template<typename U , typename AU , typename V , typename AV , typename W > | |
Tensor_ & | gemm (const Tensor< U, AU > &left, const Tensor< V, AV > &right, const W factor, const math::GemmHelper &gemm_helper) |
Contract two tensors and accumulate the scaled result to this tensor. More... | |
template<typename U , typename AU , typename V , typename AV , typename ElementMultiplyAddOp , typename = std::enable_if_t<std::is_invocable_r_v< void, std::remove_reference_t<ElementMultiplyAddOp>, value_type&, const U&, const V&>>> | |
Tensor_ & | gemm (const Tensor< U, AU > &left, const Tensor< V, AV > &right, const math::GemmHelper &gemm_helper, ElementMultiplyAddOp &&elem_muladd_op) |
template<typename TileType = Tensor_, typename = detail::enable_if_trace_is_defined_t<TileType>> | |
decltype(auto) | trace () const |
Generalized tensor trace. More... | |
template<typename ReduceOp , typename JoinOp , typename Scalar > | |
decltype(auto) | reduce (ReduceOp &&reduce_op, JoinOp &&join_op, Scalar identity) const |
Unary reduction operation. More... | |
template<typename Right , typename ReduceOp , typename JoinOp , typename Scalar , typename std::enable_if< is_tensor< Right >::value >::type * = nullptr> | |
decltype(auto) | reduce (const Right &other, ReduceOp &&reduce_op, JoinOp &&join_op, Scalar identity) const |
Binary reduction operation. More... | |
numeric_type | sum () const |
Sum of elements. More... | |
numeric_type | product () const |
Product of elements. More... | |
scalar_type | squared_norm () const |
Square of vector 2-norm. More... | |
template<typename ResultType = scalar_type> | |
ResultType | norm () const |
Vector 2-norm. More... | |
template<typename Numeric = numeric_type> | |
numeric_type | min (typename std::enable_if< detail::is_strictly_ordered< Numeric >::value >::type *=nullptr) const |
Minimum element. More... | |
template<typename Numeric = numeric_type> | |
numeric_type | max (typename std::enable_if< detail::is_strictly_ordered< Numeric >::value >::type *=nullptr) const |
Maximum element. More... | |
scalar_type | abs_min () const |
Absolute minimum element. More... | |
scalar_type | abs_max () const |
Absolute maximum element. More... | |
template<typename Right , typename std::enable_if< is_tensor< Right >::value >::type * = nullptr> | |
numeric_type | dot (const Right &other) const |
Vector dot (not inner!) product. More... | |
template<typename Right , typename std::enable_if< is_tensor< Right >::value >::type * = nullptr> | |
numeric_type | inner_product (const Right &other) const |
Vector inner product. More... | |
template<typename Index1 , typename Index2 , typename = std::enable_if_t<detail::is_integral_range_v<Index1> && detail::is_integral_range_v<Index2>>> | |
detail::TensorInterface< T, BlockRange > | block (const Index1 &lower_bound, const Index2 &upper_bound) |
Constructs a view of the block defined by lower_bound and upper_bound . More... | |
template<typename Index1 , typename Index2 , typename = std::enable_if_t<detail::is_integral_range_v<Index1> && detail::is_integral_range_v<Index2>>> | |
detail::TensorInterface< const T, BlockRange > | block (const Index1 &lower_bound, const Index2 &upper_bound) const |
Constructs a view of the block defined by lower_bound and upper_bound . More... | |
template<typename Index1 , typename Index2 , typename = std::enable_if_t<std::is_integral_v<Index1> && std::is_integral_v<Index2>>> | |
detail::TensorInterface< T, BlockRange > | block (const std::initializer_list< Index1 > &lower_bound, const std::initializer_list< Index2 > &upper_bound) |
Constructs a view of the block defined by lower_bound and upper_bound . More... | |
template<typename Index1 , typename Index2 , typename = std::enable_if_t<std::is_integral_v<Index1> && std::is_integral_v<Index2>>> | |
detail::TensorInterface< const T, BlockRange > | block (const std::initializer_list< Index1 > &lower_bound, const std::initializer_list< Index2 > &upper_bound) const |
Constructs a view of the block defined by lower_bound and upper_bound . More... | |
template<typename PairRange , typename = std::enable_if_t<detail::is_gpair_range_v<PairRange>>> | |
detail::TensorInterface< const T, BlockRange > | block (const PairRange &bounds) const |
Constructs a view of the block defined by its bounds . More... | |
template<typename PairRange , typename = std::enable_if_t<detail::is_gpair_range_v<PairRange>>> | |
detail::TensorInterface< T, BlockRange > | block (const PairRange &bounds) |
Constructs a view of the block defined by its bounds . More... | |
template<typename Index , typename = std::enable_if_t<std::is_integral_v<Index>>> | |
detail::TensorInterface< const T, BlockRange > | block (const std::initializer_list< std::initializer_list< Index >> &bounds) const |
Constructs a view of the block defined by its bounds . More... | |
template<typename Index , typename = std::enable_if_t<std::is_integral_v<Index>>> | |
detail::TensorInterface< T, BlockRange > | block (const std::initializer_list< std::initializer_list< Index >> &bounds) |
Constructs a view of the block defined by its bounds . More... | |
Member Typedef Documentation
◆ allocator_type
typedef A TiledArray::Tensor< T, A >::allocator_type |
◆ const_iterator
typedef const_pointer TiledArray::Tensor< T, A >::const_iterator |
◆ const_pointer
typedef allocator_type::const_pointer TiledArray::Tensor< T, A >::const_pointer |
◆ const_reference
typedef allocator_type::const_reference TiledArray::Tensor< T, A >::const_reference |
◆ difference_type
typedef allocator_type::difference_type TiledArray::Tensor< T, A >::difference_type |
◆ index1_type
typedef range_type::index1_type TiledArray::Tensor< T, A >::index1_type |
◆ iterator
typedef pointer TiledArray::Tensor< T, A >::iterator |
◆ numeric_type
typedef TiledArray::detail::numeric_type<T>::type TiledArray::Tensor< T, A >::numeric_type |
◆ ordinal_type
typedef range_type::ordinal_type TiledArray::Tensor< T, A >::ordinal_type |
◆ pointer
typedef allocator_type::pointer TiledArray::Tensor< T, A >::pointer |
◆ range_type
typedef Range TiledArray::Tensor< T, A >::range_type |
◆ reference
typedef allocator_type::reference TiledArray::Tensor< T, A >::reference |
◆ scalar_type
typedef TiledArray::detail::scalar_type<T>::type TiledArray::Tensor< T, A >::scalar_type |
◆ size_type
typedef range_type::ordinal_type TiledArray::Tensor< T, A >::size_type |
◆ Tensor_
typedef Tensor<T, A> TiledArray::Tensor< T, A >::Tensor_ |
◆ value_type
typedef allocator_type::value_type TiledArray::Tensor< T, A >::value_type |
Constructor & Destructor Documentation
◆ Tensor() [1/15]
|
inline |
◆ Tensor() [2/15]
|
inline |
◆ Tensor() [3/15]
|
inline |
◆ ~Tensor()
|
inline |
◆ Tensor() [4/15]
|
inlineexplicit |
◆ Tensor() [5/15]
|
inline |
◆ Tensor() [6/15]
|
inline |
◆ Tensor() [7/15]
|
inline |
◆ Tensor() [8/15]
|
inline |
◆ Tensor() [9/15]
|
inline |
◆ Tensor() [10/15]
|
inlineexplicit |
◆ Tensor() [11/15]
|
inline |
◆ Tensor() [12/15]
|
inline |
◆ Tensor() [13/15]
|
inline |
◆ Tensor() [14/15]
|
inline |
◆ Tensor() [15/15]
|
inline |
Copy, modify, and permute the data from left
, and right
.
- Template Parameters
-
T1 A tensor type T2 A tensor type Op An element-wise operation type Perm A permutation tile
- Parameters
-
left The left-hand tensor argument right The right-hand tensor argument op The element-wise operation perm The permutation that will be applied to the arguments
Member Function Documentation
◆ abs_max()
|
inline |
◆ abs_min()
|
inline |
◆ add() [1/6]
|
inline |
◆ add() [2/6]
|
inline |
Add a constant to a permuted copy of this tensor.
- Template Parameters
-
Perm A permutation tile
- Parameters
-
value The constant to be added to this tensor perm The permutation to be applied to this tensor
- Returns
- A new tensor where the elements are the sum of the elements of
this
andvalue
◆ add() [3/6]
|
inline |
Add this and other
to construct a new tensors.
- Template Parameters
-
Right The right-hand tensor type
- Parameters
-
right The tensor that will be added to this tensor
- Returns
- A new tensor where the elements are the sum of the elements of
this
andother
Definition at line 1121 of file tensor.h.

◆ add() [4/6]
|
inline |
Add this and other
to construct a new, permuted tensor.
- Template Parameters
-
Right The right-hand tensor type Perm A permutation tile
- Parameters
-
right The tensor that will be added to this tensor perm The permutation to be applied to this tensor
- Returns
- A new tensor where the elements are the sum of the elements of
this
andother
◆ add() [5/6]
|
inline |
Scale and add this and other
to construct a new tensor.
- Template Parameters
-
Right The right-hand tensor type Scalar A scalar type
- Parameters
-
right The tensor that will be added to this tensor factor The scaling factor
- Returns
- A new tensor where the elements are the sum of the elements of
this
andother
, scaled byfactor
◆ add() [6/6]
|
inline |
Scale and add this and other
to construct a new, permuted tensor.
- Template Parameters
-
Right The right-hand tensor type Scalar A scalar type Perm A permutation tile
- Parameters
-
right The tensor that will be added to this tensor factor The scaling factor perm The permutation to be applied to this tensor
- Returns
- A new tensor where the elements are the sum of the elements of
this
andother
, scaled byfactor
◆ add_to() [1/3]
|
inline |
◆ add_to() [2/3]
|
inline |
◆ add_to() [3/3]
|
inline |
◆ begin() [1/2]
|
inline |
◆ begin() [2/2]
|
inline |
◆ binary() [1/2]
|
inline |
Use a binary, element wise operation to construct a new tensor.
- Template Parameters
-
Right The right-hand tensor type Op The binary operation type
- Parameters
-
right The right-hand argument in the binary operation op The binary, element-wise operation
- Returns
- A tensor where element
i
of the new tensor is equal toop(*this[i],other[i])
Definition at line 950 of file tensor.h.
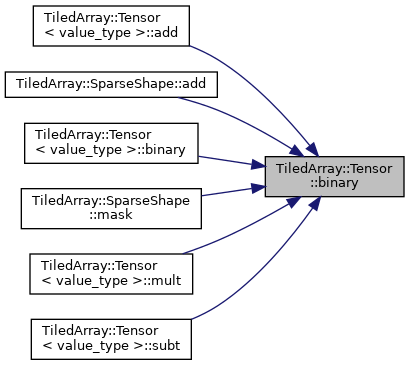
◆ binary() [2/2]
|
inline |
Use a binary, element wise operation to construct a new, permuted tensor.
- Template Parameters
-
Right The right-hand tensor type Op The binary operation type Perm A permutation tile
- Parameters
-
right The right-hand argument in the binary operation op The binary, element-wise operation perm The permutation to be applied to this tensor
- Returns
- A tensor where element
i
of the new tensor is equal toop(*this[i],other[i])
◆ block() [1/8]
|
inline |
Constructs a view of the block defined by lower_bound
and upper_bound
.
Examples of using this:
- Template Parameters
-
Index1 An integral range type Index2 An integral range type
- Parameters
-
lower_bound The lower bound upper_bound The upper bound
- Returns
- a {const,mutable} view of the block defined by
lower_bound
andupper_bound
- Exceptions
-
TiledArray::Exception When the size of lower_bound
is not equal to that ofupper_bound
.TiledArray::Exception When lower_bound[i] >= upper_bound[i]
Definition at line 704 of file tensor.h.

◆ block() [2/8]
|
inline |
Constructs a view of the block defined by lower_bound
and upper_bound
.
Examples of using this:
- Template Parameters
-
Index1 An integral range type Index2 An integral range type
- Parameters
-
lower_bound The lower bound upper_bound The upper bound
- Returns
- a {const,mutable} view of the block defined by
lower_bound
andupper_bound
- Exceptions
-
TiledArray::Exception When the size of lower_bound
is not equal to that ofupper_bound
.TiledArray::Exception When lower_bound[i] >= upper_bound[i]
◆ block() [3/8]
|
inline |
Constructs a view of the block defined by its bounds
.
Examples of using this:
- Template Parameters
-
PairRange Type representing a range of generalized pairs (see TiledArray::detail::is_gpair_v )
- Parameters
-
bounds The block bounds
- Returns
- a {const,mutable} view of the block defined by its
bounds
- Exceptions
-
TiledArray::Exception When the size of lower_bound
is not equal to that ofupper_bound
.TiledArray::Exception When get<0>(bounds[i]) >= get<1>(bounds[i])
◆ block() [4/8]
|
inline |
Constructs a view of the block defined by its bounds
.
Examples of using this:
- Template Parameters
-
PairRange Type representing a range of generalized pairs (see TiledArray::detail::is_gpair_v )
- Parameters
-
bounds The block bounds
- Returns
- a {const,mutable} view of the block defined by its
bounds
- Exceptions
-
TiledArray::Exception When the size of lower_bound
is not equal to that ofupper_bound
.TiledArray::Exception When get<0>(bounds[i]) >= get<1>(bounds[i])
◆ block() [5/8]
|
inline |
Constructs a view of the block defined by lower_bound
and upper_bound
.
Examples of using this:
- Template Parameters
-
Index1 An integral type Index2 An integral type
- Parameters
-
lower_bound The lower bound upper_bound The upper bound
- Returns
- a {const,mutable} view of the block defined by
lower_bound
andupper_bound
- Exceptions
-
TiledArray::Exception When the size of lower_bound
is not equal to that ofupper_bound
.TiledArray::Exception When lower_bound[i] >= upper_bound[i]
◆ block() [6/8]
|
inline |
Constructs a view of the block defined by lower_bound
and upper_bound
.
Examples of using this:
- Template Parameters
-
Index1 An integral type Index2 An integral type
- Parameters
-
lower_bound The lower bound upper_bound The upper bound
- Returns
- a {const,mutable} view of the block defined by
lower_bound
andupper_bound
- Exceptions
-
TiledArray::Exception When the size of lower_bound
is not equal to that ofupper_bound
.TiledArray::Exception When lower_bound[i] >= upper_bound[i]
◆ block() [7/8]
|
inline |
Constructs a view of the block defined by its bounds
.
Examples of using this:
- Template Parameters
-
Index An integral type
- Parameters
-
bounds The block bounds
- Returns
- a {const,mutable} view of the block defined by its
bounds
- Exceptions
-
TiledArray::Exception When the size of lower_bound
is not equal to that ofupper_bound
.TiledArray::Exception When get<0>(bounds[i]) >= get<1>(bounds[i])
◆ block() [8/8]
|
inline |
Constructs a view of the block defined by its bounds
.
Examples of using this:
- Template Parameters
-
Index An integral type
- Parameters
-
bounds The block bounds
- Returns
- a {const,mutable} view of the block defined by its
bounds
- Exceptions
-
TiledArray::Exception When the size of lower_bound
is not equal to that ofupper_bound
.TiledArray::Exception When get<0>(bounds[i]) >= get<1>(bounds[i])
◆ clone()
|
inline |
◆ conj() [1/4]
|
inline |
◆ conj() [2/4]
|
inline |
◆ conj() [3/4]
|
inline |
◆ conj() [4/4]
|
inline |
Create a complex conjugated, scaled, and permuted copy of this tensor.
- Template Parameters
-
Scalar A scalar type Perm A permutation type
- Parameters
-
factor The scaling factor perm The permutation to be applied to this tensor
- Returns
- A permuted copy of this tensor that contains the complex conjugate values
◆ conj_to() [1/2]
|
inline |
◆ conj_to() [2/2]
|
inline |
◆ data() [1/2]
|
inline |
◆ data() [2/2]
|
inline |
◆ dot()
|
inline |
Vector dot (not inner!) product.
- Template Parameters
-
Right The right-hand tensor type
- Parameters
-
other The right-hand tensor to be reduced
- Returns
- The dot product of the this and
other
If numeric_type is real, this is equivalent to inner product
- See also
- Tensor::inner_product
◆ empty()
|
inline |
◆ end() [1/2]
|
inline |
◆ end() [2/2]
|
inline |
◆ gemm() [1/3]
|
inline |
◆ gemm() [2/3]
|
inline |
Contract two tensors and accumulate the scaled result to this tensor.
GEMM is limited to matrix like contractions. For example, the following contractions are supported:
Notice that in the above contractions, the inner and outer indices of the arguments for exactly two contiguous groups in each tensor and that each group is in the same order in all tensors. That is, the indices of the tensors must fit the one of the following patterns:
This allows use of optimized BLAS functions to evaluate tensor contractions. Tensor contractions that do not fit this pattern require one or more tensor permutation so that the tensors fit the required pattern.
- Template Parameters
-
U The left-hand tensor element type AU The left-hand tensor allocator type V The right-hand tensor element type AV The right-hand tensor allocator type W The type of the scaling factor
- Parameters
-
left The left-hand tensor that will be contracted right The right-hand tensor that will be contracted factor The contraction result will be scaling by this value, then accumulated into this@param
gemm_helper The *GEMM operation meta data
- Returns
- A reference to
this
- Note
- if this is uninitialized, i.e., if
this->empty()==true
will this is equivalent toreturn (*this = left.gemm(right, factor, gemm_helper));
◆ gemm() [3/3]
|
inline |
Contract this tensor with other
.
- Template Parameters
-
U The other tensor element type AU The other tensor allocator type V The type of factor
scalar
- Parameters
-
other The tensor that will be contracted with this tensor factor Multiply the result by this constant gemm_helper The *GEMM operation meta data
- Returns
- A new tensor which is the result of contracting this tensor with
other
and scaled byfactor
Definition at line 1609 of file tensor.h.
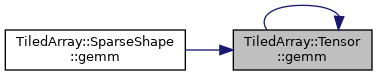
◆ inner_product()
|
inline |
Vector inner product.
- Template Parameters
-
Right The right-hand tensor type
- Parameters
-
other The right-hand tensor to be reduced
- Returns
- The dot product of the this and
other
If numeric_type is real, this is equivalent to dot product
- See also
- Tensor::dot
◆ inplace_binary()
|
inline |
Use a binary, element wise operation to modify this tensor.
- Template Parameters
-
Right The right-hand tensor type Op The binary operation type
- Parameters
-
right The right-hand argument in the binary operation op The binary, element-wise operation
- Returns
- A reference to this object
- Exceptions
-
TiledArray::Exception When this tensor is empty. TiledArray::Exception When other
is empty.TiledArray::Exception When the range of this tensor is not equal to the range of other
.TiledArray::Exception When this and other
are the same.
Definition at line 1005 of file tensor.h.
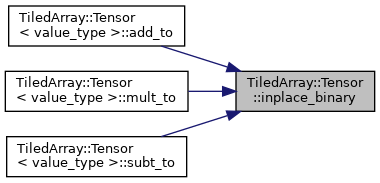
◆ inplace_unary()
|
inline |
Use a unary, element wise operation to modify this tensor.
- Template Parameters
-
Op The unary operation type
- Parameters
-
op The unary, element-wise operation
- Returns
- A reference to this object
- Exceptions
-
TiledArray::Exception When this tensor is empty.
Definition at line 1062 of file tensor.h.
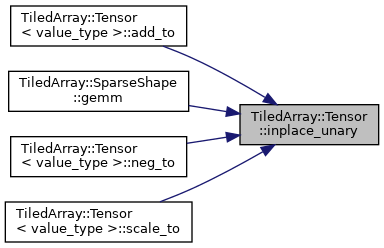
◆ max()
|
inline |
◆ min()
|
inline |
◆ mult() [1/4]
|
inline |
Multiply this by right
to create a new tensor.
- Template Parameters
-
Right The right-hand tensor type
- Parameters
-
right The tensor that will be multiplied by this tensor
- Returns
- A new tensor where the elements are the product of the elements of
this
andright
Definition at line 1398 of file tensor.h.

◆ mult() [2/4]
|
inline |
Multiply this by right
to create a new, permuted tensor.
- Template Parameters
-
Right The right-hand tensor type Perm a permutation type
- Parameters
-
right The tensor that will be multiplied by this tensor perm The permutation to be applied to this tensor
- Returns
- A new tensor where the elements are the product of the elements of
this
andright
◆ mult() [3/4]
|
inline |
Scale and multiply this by right
to create a new tensor.
- Template Parameters
-
Right The right-hand tensor type Scalar A scalar type
- Parameters
-
right The tensor that will be multiplied by this tensor factor The scaling factor
- Returns
- A new tensor where the elements are the product of the elements of
this
andright
, scaled byfactor
◆ mult() [4/4]
|
inline |
Scale and multiply this by right
to create a new, permuted tensor.
- Template Parameters
-
Right The right-hand tensor type Scalar A scalar type Perm A permutation type
- Parameters
-
right The tensor that will be multiplied by this tensor factor The scaling factor perm The permutation to be applied to this tensor
- Returns
- A new tensor where the elements are the product of the elements of
this
andright
, scaled byfactor
◆ mult_to() [1/2]
|
inline |
◆ mult_to() [2/2]
|
inline |
◆ neg() [1/2]
|
inline |
◆ neg() [2/2]
|
inline |
◆ neg_to()
|
inline |
◆ norm()
|
inline |
Vector 2-norm.
- Template Parameters
-
ResultType return type
- Note
- This evaluates
std::sqrt
(ResultType(this->squared_norm()))
- Returns
- The vector norm of this tensor
◆ operator()() [1/6]
|
inline |
Element accessor.
- Template Parameters
-
Index an integral list ( see TiledArray::detail::is_integral_list )
- Parameters
-
[in] i an index
- Returns
- Reference to the element at position
i
.
- Note
- This asserts (using TA_ASSERT) that this is not empty and ord is included in the range
◆ operator()() [2/6]
|
inline |
Const element accessor.
- Template Parameters
-
Index an integral list ( see TiledArray::detail::is_integral_list )
- Parameters
-
[in] i an index
- Returns
- Const reference to the element at position
i
.
- Note
- This asserts (using TA_ASSERT) that this is not empty and ord is included in the range
◆ operator()() [3/6]
|
inline |
◆ operator()() [4/6]
|
inline |
◆ operator()() [5/6]
|
inline |
◆ operator()() [6/6]
|
inline |
◆ operator=() [1/3]
|
inline |
◆ operator=() [2/3]
|
inline |
◆ operator=() [3/3]
|
inline |
◆ operator[]() [1/6]
|
inline |
◆ operator[]() [2/6]
|
inline |
◆ operator[]() [3/6]
|
inline |
Element accessor.
- Template Parameters
-
Ordinal an integer type that represents an ordinal
- Parameters
-
[in] ord an ordinal index
- Returns
- Reference to the element at position
ord
.
- Note
- This asserts (using TA_ASSERT) that this is not empty and ord is included in the range
◆ operator[]() [4/6]
|
inline |
Const element accessor.
- Template Parameters
-
Ordinal an integer type that represents an ordinal
- Parameters
-
[in] ord an ordinal index
- Returns
- Const reference to the element at position
ord
.
- Note
- This asserts (using TA_ASSERT) that this is not empty and ord is included in the range
◆ operator[]() [5/6]
|
inline |
◆ operator[]() [6/6]
|
inline |
◆ permute()
|
inline |
◆ product()
|
inline |
◆ range() [1/2]
|
inline |
◆ range() [2/2]
|
inline |
◆ reduce() [1/2]
|
inline |
Binary reduction operation.
Perform an element-wise binary reduction of the data of this
and other
by executing join_op(result, reduce_op(*this[i], other[i]))
for each i
in the index range of this
. result
is initialized to identity
. If HAVE_INTEL_TBB is defined, and this is a contiguous tensor, the reduction will be executed in an undefined order, otherwise will execute in the order of increasing i
.
- Template Parameters
-
Right The right-hand argument tensor type ReduceOp The reduction operation type JoinOp The join operation type
- Parameters
-
other The right-hand argument of the binary reduction reduce_op The element-wise reduction operation join_op The join result operation identity The identity value of the reduction
- Returns
- The reduced value
◆ reduce() [2/2]
|
inline |
Unary reduction operation.
Perform an element-wise reduction of the data by executing join_op(result, reduce_op(*this[i]))
for each i
in the index range of this
. result
is initialized to identity
. If HAVE_INTEL_TBB is defined, and this is a contiguous tensor, the reduction will be executed in an undefined order, otherwise will execute in the order of increasing i
.
- Template Parameters
-
ReduceOp The reduction operation type JoinOp The join operation type
- Parameters
-
reduce_op The element-wise reduction operation join_op The join result operation identity The identity value of the reduction
- Returns
- The reduced value
Definition at line 1996 of file tensor.h.
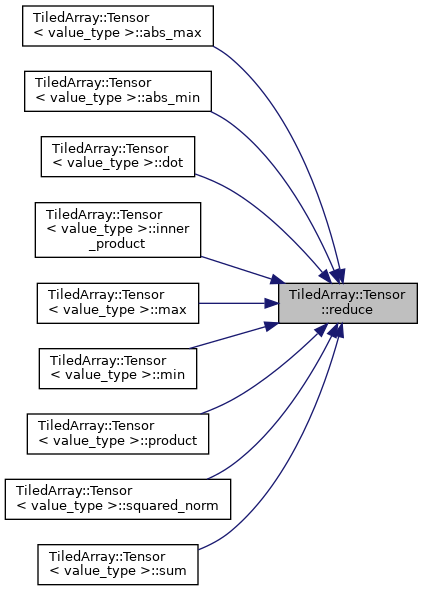
◆ scale() [1/2]
|
inline |
◆ scale() [2/2]
|
inline |
Construct a scaled and permuted copy of this tensor.
- Template Parameters
-
Scalar A scalar type Perm A permutation tile
- Parameters
-
factor The scaling factor perm The permutation to be applied to this tensor
- Returns
- A new tensor where the elements of this tensor are scaled by
factor
and permuted
◆ scale_to()
|
inline |
◆ serialize() [1/2]
|
inline |
◆ serialize() [2/2]
|
inline |
◆ shift() [1/2]
|
inline |
◆ shift() [2/2]
|
inline |
◆ shift_to() [1/2]
|
inline |
◆ shift_to() [2/2]
|
inline |
◆ size()
|
inline |
◆ squared_norm()
|
inline |
◆ subt() [1/6]
|
inline |
◆ subt() [2/6]
|
inline |
Subtract a constant from a permuted copy of this tensor.
- Template Parameters
-
Perm A permutation tile
- Parameters
-
value The constant to be subtracted perm The permutation to be applied to this tensor
- Returns
- A new tensor where the elements are the different between the elements of
this
andvalue
◆ subt() [3/6]
|
inline |
Subtract right
from this and return the result.
- Template Parameters
-
Right The right-hand tensor type
- Parameters
-
right The tensor that will be subtracted from this tensor
- Returns
- A new tensor where the elements are the different between the elements of
this
andright
◆ subt() [4/6]
|
inline |
Subtract right
from this and return the result permuted by perm
.
- Template Parameters
-
Right The right-hand tensor type Perm A permutation type
- Parameters
-
right The tensor that will be subtracted from this tensor perm The permutation to be applied to this tensor
- Returns
- A new tensor where the elements are the different between the elements of
this
andright
◆ subt() [5/6]
|
inline |
Subtract right
from this and return the result scaled by a scaling factor
- Template Parameters
-
Right The right-hand tensor type Scalar A scalar type
- Parameters
-
right The tensor that will be subtracted from this tensor factor The scaling factor
- Returns
- A new tensor where the elements are the different between the elements of
this
andright
, scaled byfactor
◆ subt() [6/6]
|
inline |
Subtract right
from this and return the result scaled by a scaling factor
and permuted by perm
- Template Parameters
-
Right The right-hand tensor type Scalar A scalar type Perm A permutation type
- Parameters
-
right The tensor that will be subtracted from this tensor factor The scaling factor perm The permutation to be applied to this tensor
- Returns
- A new tensor where the elements are the different between the elements of
this
andright
, scaled byfactor
◆ subt_to() [1/3]
|
inline |
◆ subt_to() [2/3]
|
inline |
◆ subt_to() [3/3]
|
inline |
◆ sum()
|
inline |
◆ swap()
|
inline |
◆ trace()
|
inline |
Generalized tensor trace.
This function will compute the sum of the hyper diagonal elements of tensor.
- Returns
- The trace of this tensor
- Exceptions
-
TiledArray::Exception When this tensor is empty.
◆ unary() [1/2]
|
inline |
Use a unary, element wise operation to construct a new tensor.
- Template Parameters
-
Op The unary operation type
- Parameters
-
op The unary, element-wise operation
- Returns
- A tensor where element
i
of the new tensor is equal toop(*this[i])
- Exceptions
-
TiledArray::Exception When this tensor is empty.
Definition at line 1018 of file tensor.h.
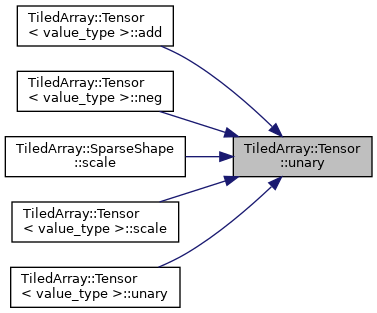
◆ unary() [2/2]
|
inline |
Use a unary, element wise operation to construct a new, permuted tensor.
- Template Parameters
-
Op The unary operation type Perm A permutation tile
- Parameters
-
op The unary operation perm The permutation to be applied to this tensor
- Returns
- A permuted tensor with elements that have been modified by
op
- Exceptions
-
TiledArray::Exception When this tensor is empty. TiledArray::Exception The dimension of perm
does not match that of this tensor.
The documentation for this class was generated from the following files: