Namespaces | |
blas | |
linalg | |
Classes | |
struct | BinaryReduceWrapper |
Binary reduction wrapper class that handles lazy tile evaluation. More... | |
struct | BinaryReduceWrapper< typename Op::first_argument_type, typename Op::second_argument_type, Op > |
Binary reduction operation wrapper. More... | |
class | Block |
class | GemmHelper |
Contraction to *GEMM helper. More... | |
class | GemmTask |
class | MatrixBlockTask |
class | OuterVectorOpUnwind |
Outer algorithm automatic loop unwinding. More... | |
class | OuterVectorOpUnwind< 0 > |
class | PartialReduceUnwind |
Partial reduce algorithm automatic loop unwinding. More... | |
class | PartialReduceUnwind< 0 > |
class | TransposeUnwind |
Partial transpose algorithm automatic loop unwinding. More... | |
class | TransposeUnwind< 0 > |
class | UnaryReduceWrapper |
Unary reduction wrapper class that handles lazy tile evaluation. More... | |
class | UnaryReduceWrapper< typename Op::argument_type, Op > |
Unary reduction wrapper class that handles lazy tile evaluation. More... | |
struct | VectorOpUnwind |
Vector loop unwind helper class. More... | |
struct | VectorOpUnwind< 0ul > |
Vector loop unwind helper class. More... | |
Typedefs | |
typedef OuterVectorOpUnwind< TILEDARRAY_LOOP_UNWIND - 1 > | OuterVectorOpUnwindN |
typedef PartialReduceUnwind< TILEDARRAY_LOOP_UNWIND - 1 > | PartialReduceUnwindN |
typedef TransposeUnwind< TILEDARRAY_LOOP_UNWIND - 1 > | TransposeUnwindN |
typedef std::integral_constant< std::size_t, TILEDARRAY_CACHELINE_SIZE/sizeof(double)> | LoopUnwind |
typedef std::integral_constant< std::size_t, ~std::size_t(TILEDARRAY_LOOP_UNWIND - 1ul)> | index_mask |
typedef VectorOpUnwind< TILEDARRAY_LOOP_UNWIND - 1 > | VecOpUnwindN |
Functions | |
template<typename X , typename Y , typename A , typename Op > | |
void | outer_fill (const std::size_t m, const std::size_t n, const X *const x, const Y *const y, A *a, const Op &op) |
Compute and store outer of x and y in a . More... | |
template<typename X , typename Y , typename A , typename Op > | |
void | outer (const std::size_t m, const std::size_t n, const X *const x, const Y *const y, A *a, const Op &op) |
Compute the outer of x and y to modify a . More... | |
template<typename X , typename Y , typename A , typename B , typename Op > | |
void | outer_fill (const std::size_t m, const std::size_t n, const X *MADNESS_RESTRICT const x, const Y *MADNESS_RESTRICT const y, const A *MADNESS_RESTRICT a, B *MADNESS_RESTRICT b, const Op &op) |
Compute the outer of x , y , and a , and store the result in b . More... | |
template<typename Left , typename Right , typename Result , typename Op > | |
void | row_reduce (const std::size_t m, const std::size_t n, const Left *MADNESS_RESTRICT const left, const Right *MADNESS_RESTRICT const right, Result *MADNESS_RESTRICT const result, const Op &op) |
Reduce the rows of a matrix. More... | |
template<typename Arg , typename Result , typename Op > | |
void | row_reduce (const std::size_t m, const std::size_t n, const Arg *MADNESS_RESTRICT const arg, Result *MADNESS_RESTRICT const result, const Op &op) |
Reduce the rows of a matrix. More... | |
template<typename Left , typename Right , typename Result , typename Op > | |
void | col_reduce (const std::size_t m, const std::size_t n, const Left *MADNESS_RESTRICT const left, const Right *MADNESS_RESTRICT const right, Result *MADNESS_RESTRICT const result, const Op &op) |
Reduce the columns of a matrix. More... | |
template<typename Arg , typename Result , typename Op > | |
void | col_reduce (const std::size_t m, const std::size_t n, const Arg *MADNESS_RESTRICT const arg, Result *MADNESS_RESTRICT const result, const Op &op) |
Reduce the columns of a matrix. More... | |
template<typename InputOp , typename OutputOp , typename Result , typename... Args> | |
TILEDARRAY_FORCE_INLINE void | transpose_block (InputOp &&input_op, OutputOp &&output_op, const std::size_t result_stride, Result *const result, const std::size_t arg_stride, const Args *const ... args) |
template<typename InputOp , typename OutputOp , typename Result , typename... Args> | |
TILEDARRAY_FORCE_INLINE void | transpose_block (InputOp &&input_op, OutputOp &&output_op, const std::size_t m, const std::size_t n, const std::size_t result_stride, Result *MADNESS_RESTRICT const result, const std::size_t arg_stride, const Args *MADNESS_RESTRICT const ... args) |
template<typename InputOp , typename OutputOp , typename Result , typename... Args> | |
void | transpose (InputOp &&input_op, OutputOp &&output_op, const std::size_t m, const std::size_t n, const std::size_t result_stride, Result *result, const std::size_t arg_stride, const Args *const ... args) |
Matrix transpose and initialization. More... | |
template<typename Op , typename Result , typename... Args> | |
TILEDARRAY_FORCE_INLINE void | for_each_block (Op &&op, Result *const result, const Args *const ... args) |
template<typename Op , typename Result , typename... Args> | |
TILEDARRAY_FORCE_INLINE void | for_each_block (Op &&op, Block< Result > &result, Block< Args > &&... args) |
template<typename Op , typename Result , typename... Args> | |
TILEDARRAY_FORCE_INLINE void | for_each_block_n (Op &&op, const std::size_t n, Result *MADNESS_RESTRICT const result, const Args *MADNESS_RESTRICT const ... args) |
template<typename Op , typename Result , typename... Args> | |
TILEDARRAY_FORCE_INLINE std::enable_if<(sizeof...(Args) >=0)>::type | for_each_block_ptr (Op &&op, Result *const result, const Args *const ... args) |
template<typename Op , typename Result , typename... Args> | |
TILEDARRAY_FORCE_INLINE std::enable_if<(sizeof...(Args) > 0)>::type | for_each_block_ptr (Op &&op, Result *const result, Block< Args > &&... args) |
template<typename Op , typename Result , typename... Args> | |
TILEDARRAY_FORCE_INLINE void | for_each_block_ptr_n (Op &&op, const std::size_t n, Result *MADNESS_RESTRICT const result, const Args *MADNESS_RESTRICT const ... args) |
template<typename Op , typename Result , typename... Args> | |
TILEDARRAY_FORCE_INLINE void | reduce_block (Op &&op, Result &result, const Args *const ... args) |
template<typename Op , typename Result , typename... Args> | |
TILEDARRAY_FORCE_INLINE void | reduce_block (Op &&op, Result &result, Block< Args > &&... args) |
template<typename Op , typename Result , typename... Args> | |
TILEDARRAY_FORCE_INLINE void | reduce_block_n (Op &&op, const std::size_t n, Result &MADNESS_RESTRICT result, const Args *MADNESS_RESTRICT const ... args) |
template<typename Result , typename Arg > | |
TILEDARRAY_FORCE_INLINE void | copy_block (Result *const result, const Arg *const arg) |
template<typename Arg , typename Result > | |
TILEDARRAY_FORCE_INLINE void | copy_block_n (std::size_t n, Result *const result, const Arg *const arg) |
template<typename Arg , typename Result > | |
TILEDARRAY_FORCE_INLINE void | scatter_block (Result *const result, const std::size_t stride, const Arg *const arg) |
template<typename Result , typename Arg > | |
TILEDARRAY_FORCE_INLINE void | scatter_block_n (const std::size_t n, Result *result, const std::size_t stride, const Arg *const arg) |
template<typename Result , typename Arg > | |
TILEDARRAY_FORCE_INLINE void | gather_block (Result *const result, const Arg *const arg, const std::size_t stride) |
template<typename Arg , typename Result > | |
TILEDARRAY_FORCE_INLINE void | gather_block_n (const std::size_t n, Result *const result, const Arg *const arg, const std::size_t stride) |
template<typename Op , typename Result , typename... Args, typename std::enable_if< std::is_void< typename std::result_of< Op(Result &, Args...)>::type >::value >::type * = nullptr> | |
void | inplace_vector_op_serial (Op &&op, const std::size_t n, Result *const result, const Args *const ... args) |
template<typename Op , typename Result , typename... Args, typename std::enable_if< std::is_void< typename std::result_of< Op(Result &, Args...)>::type >::value >::type * = nullptr> | |
void | inplace_vector_op (Op &&op, const std::size_t n, Result *const result, const Args *const ... args) |
template<typename Op , typename Result , typename... Args, typename std::enable_if<!std::is_void< typename std::result_of< Op(Args...)>::type >::value >::type * = nullptr> | |
void | vector_op_serial (Op &&op, const std::size_t n, Result *const result, const Args *const ... args) |
template<typename Op , typename Result , typename... Args, typename std::enable_if<!std::is_void< typename std::result_of< Op(Args...)>::type >::value >::type * = nullptr> | |
void | vector_op (Op &&op, const std::size_t n, Result *const result, const Args *const ... args) |
template<typename Op , typename Result , typename... Args> | |
void | vector_ptr_op_serial (Op &&op, const std::size_t n, Result *const result, const Args *const ... args) |
template<typename Op , typename Result , typename... Args> | |
void | vector_ptr_op (Op &&op, const std::size_t n, Result *const result, const Args *const ... args) |
template<typename Op , typename Result , typename... Args> | |
void | reduce_op_serial (Op &&op, const std::size_t n, Result &result, const Args *const ... args) |
template<typename ReduceOp , typename JoinOp , typename Result , typename... Args> | |
void | reduce_op (ReduceOp &&reduce_op, JoinOp &&join_op, const Result &identity, const std::size_t n, Result &result, const Args *const ... args) |
template<typename Arg , typename Result > | |
std::enable_if<!(std::is_same< Arg, Result >::value &&detail::is_scalar_v< Arg >)>::type | copy_vector (const std::size_t n, const Arg *const arg, Result *const result) |
template<typename T > | |
std::enable_if< detail::is_scalar_v< T > >::type | copy_vector (const std::size_t n, const T *const arg, T *const result) |
template<typename Arg , typename Result > | |
void | fill_vector (const std::size_t n, const Arg &arg, Result *const result) |
template<typename Arg , typename Result > | |
std::enable_if<!(detail::is_scalar_v< Arg > &&detail::is_scalar_v< Result >)>::type | uninitialized_copy_vector (const std::size_t n, const Arg *const arg, Result *const result) |
template<typename Arg , typename Result > | |
std::enable_if< detail::is_scalar_v< Arg > &&detail::is_scalar_v< Result > >::type | uninitialized_copy_vector (const std::size_t n, const Arg *const arg, Result *const result) |
template<typename Arg , typename Result > | |
std::enable_if<!(detail::is_scalar_v< Arg > &&detail::is_scalar_v< Result >)>::type | uninitialized_fill_vector (const std::size_t n, const Arg &arg, Result *const result) |
template<typename Arg , typename Result > | |
std::enable_if< detail::is_scalar_v< Arg > &&detail::is_scalar_v< Result > >::type | uninitialized_fill_vector (const std::size_t n, const Arg &arg, Result *const result) |
template<typename Arg > | |
std::enable_if<!detail::is_scalar_v< Arg > >::type | destroy_vector (const std::size_t n, Arg *const arg) |
template<typename Arg > | |
std::enable_if< detail::is_scalar_v< Arg > >::type | destroy_vector (const std::size_t, const Arg *const) |
template<typename Arg , typename Result , typename Op > | |
std::enable_if<!(detail::is_scalar_v< Arg > &&detail::is_scalar_v< Result >)>::type | uninitialized_unary_vector_op (const std::size_t n, const Arg *const arg, Result *const result, Op &&op) |
template<typename Arg , typename Result , typename Op > | |
std::enable_if< detail::is_scalar_v< Arg > &&detail::is_scalar_v< Result > >::type | uninitialized_unary_vector_op (const std::size_t n, const Arg *const arg, Result *const result, Op &&op) |
template<typename Left , typename Right , typename Result , typename Op > | |
std::enable_if<!(detail::is_scalar_v< Left > &&detail::is_scalar_v< Right > &&detail::is_scalar_v< Result >)>::type | uninitialized_binary_vector_op (const std::size_t n, const Left *const left, const Right *const right, Result *const result, Op &&op) |
template<typename Left , typename Right , typename Result , typename Op > | |
std::enable_if< detail::is_scalar_v< Left > &&detail::is_scalar_v< Right > &&detail::is_scalar_v< Result > >::type | uninitialized_binary_vector_op (const std::size_t n, const Left *const left, const Right *const right, Result *const result, Op &&op) |
Typedef Documentation
◆ index_mask
typedef std::integral_constant<std::size_t, ~std::size_t(TILEDARRAY_LOOP_UNWIND - 1ul)> TiledArray::math::index_mask |
Definition at line 54 of file vector_op.h.
◆ LoopUnwind
typedef std::integral_constant<std::size_t, TILEDARRAY_CACHELINE_SIZE / sizeof(double)> TiledArray::math::LoopUnwind |
Definition at line 51 of file vector_op.h.
◆ OuterVectorOpUnwindN
◆ PartialReduceUnwindN
Definition at line 179 of file partial_reduce.h.
◆ TransposeUnwindN
Definition at line 106 of file transpose.h.
◆ VecOpUnwindN
Definition at line 157 of file vector_op.h.
Function Documentation
◆ col_reduce() [1/2]
void TiledArray::math::col_reduce | ( | const std::size_t | m, |
const std::size_t | n, | ||
const Arg *MADNESS_RESTRICT const | arg, | ||
Result *MADNESS_RESTRICT const | result, | ||
const Op & | op | ||
) |
Reduce the columns of a matrix.
op(result[j], arg[i][j])
.
- Template Parameters
-
Arg The argument vector element type Result The a matrix element type Op The operator type
- Parameters
-
[in] m The number of rows in left [in] n The size of the right-hand vector [in] arg An m*n matrix [out] result The result vector of size n [in] op The operation that will reduce the columns of left
Definition at line 393 of file partial_reduce.h.

◆ col_reduce() [2/2]
void TiledArray::math::col_reduce | ( | const std::size_t | m, |
const std::size_t | n, | ||
const Left *MADNESS_RESTRICT const | left, | ||
const Right *MADNESS_RESTRICT const | right, | ||
Result *MADNESS_RESTRICT const | result, | ||
const Op & | op | ||
) |
Reduce the columns of a matrix.
op(result[j], left[i][j], right[i])
.
- Template Parameters
-
Left The left-hand vector element type Right The right-hand vector element type Result The a matrix element type Op The operator type
- Parameters
-
[in] m The number of rows in left [in] n The size of the right-hand vector [in] left An m*n matrix [in] right A vector of size m [out] result The result vector of size n [in] op The operation that will reduce the columns of left
Definition at line 322 of file partial_reduce.h.


◆ copy_block()
TILEDARRAY_FORCE_INLINE void TiledArray::math::copy_block | ( | Result *const | result, |
const Arg *const | arg | ||
) |
Definition at line 219 of file vector_op.h.

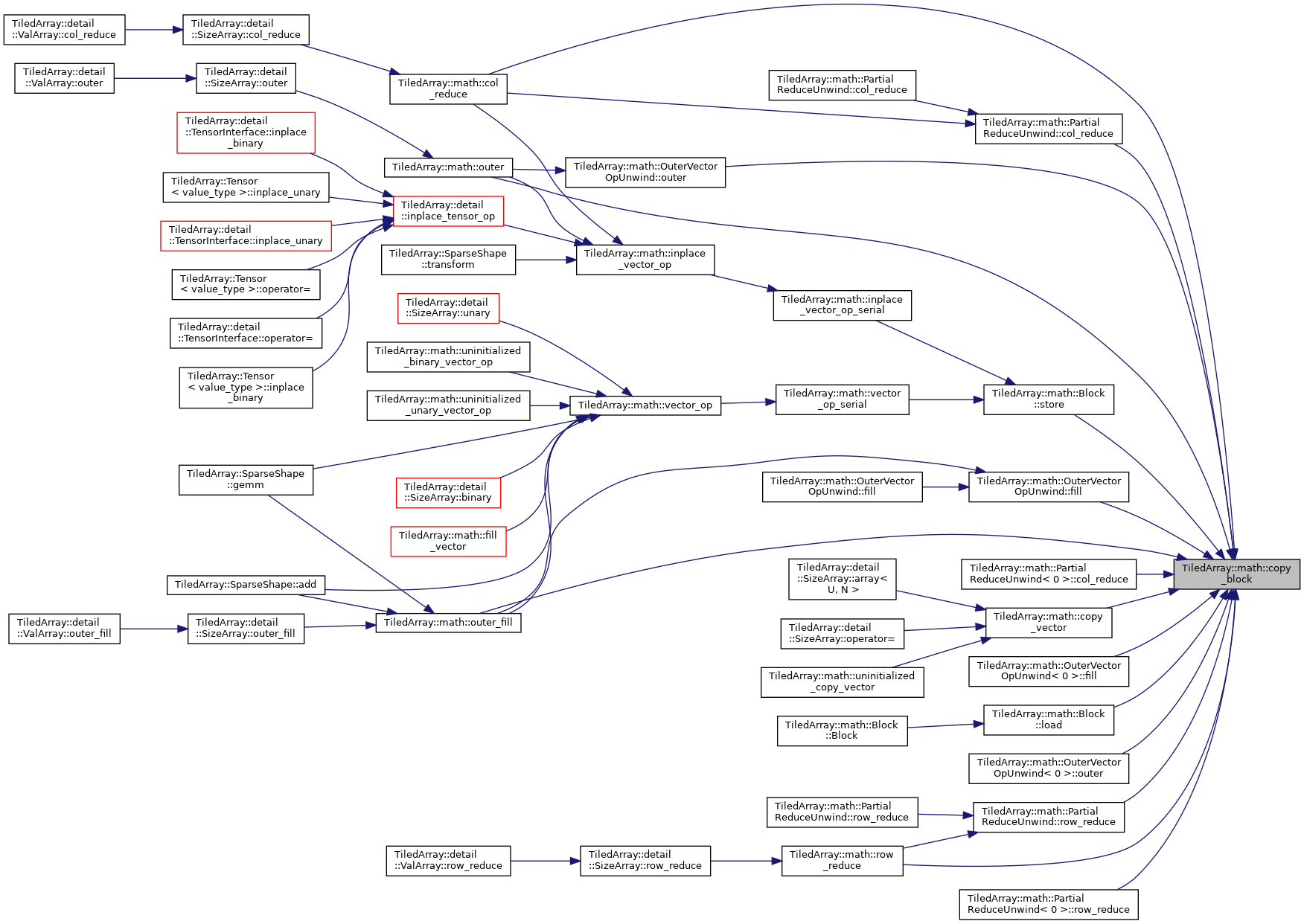
◆ copy_block_n()
TILEDARRAY_FORCE_INLINE void TiledArray::math::copy_block_n | ( | std::size_t | n, |
Result *const | result, | ||
const Arg *const | arg | ||
) |
Definition at line 226 of file vector_op.h.

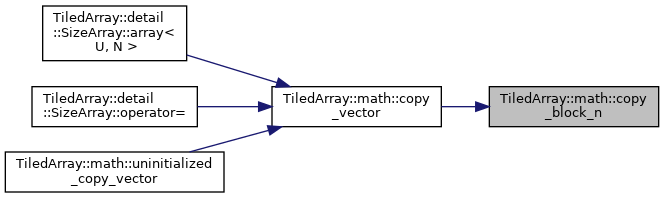
◆ copy_vector() [1/2]
std::enable_if<!(std::is_same<Arg, Result>::value && detail::is_scalar_v<Arg>)>::type TiledArray::math::copy_vector | ( | const std::size_t | n, |
const Arg *const | arg, | ||
Result *const | result | ||
) |
Definition at line 648 of file vector_op.h.

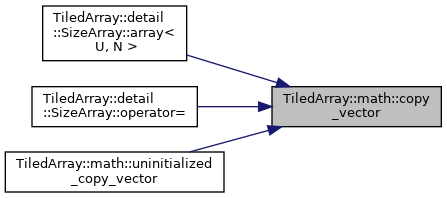
◆ copy_vector() [2/2]
|
inline |
Definition at line 660 of file vector_op.h.
◆ destroy_vector() [1/2]
std::enable_if<!detail::is_scalar_v<Arg> >::type TiledArray::math::destroy_vector | ( | const std::size_t | n, |
Arg *const | arg | ||
) |
◆ destroy_vector() [2/2]
|
inline |
Definition at line 713 of file vector_op.h.
◆ fill_vector()
void TiledArray::math::fill_vector | ( | const std::size_t | n, |
const Arg & | arg, | ||
Result *const | result | ||
) |
Definition at line 666 of file vector_op.h.

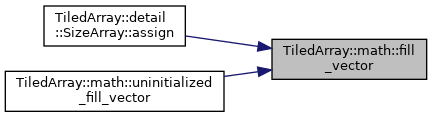
◆ for_each_block() [1/2]
◆ for_each_block() [2/2]
TILEDARRAY_FORCE_INLINE void TiledArray::math::for_each_block | ( | Op && | op, |
Result *const | result, | ||
const Args *const ... | args | ||
) |
Definition at line 162 of file vector_op.h.

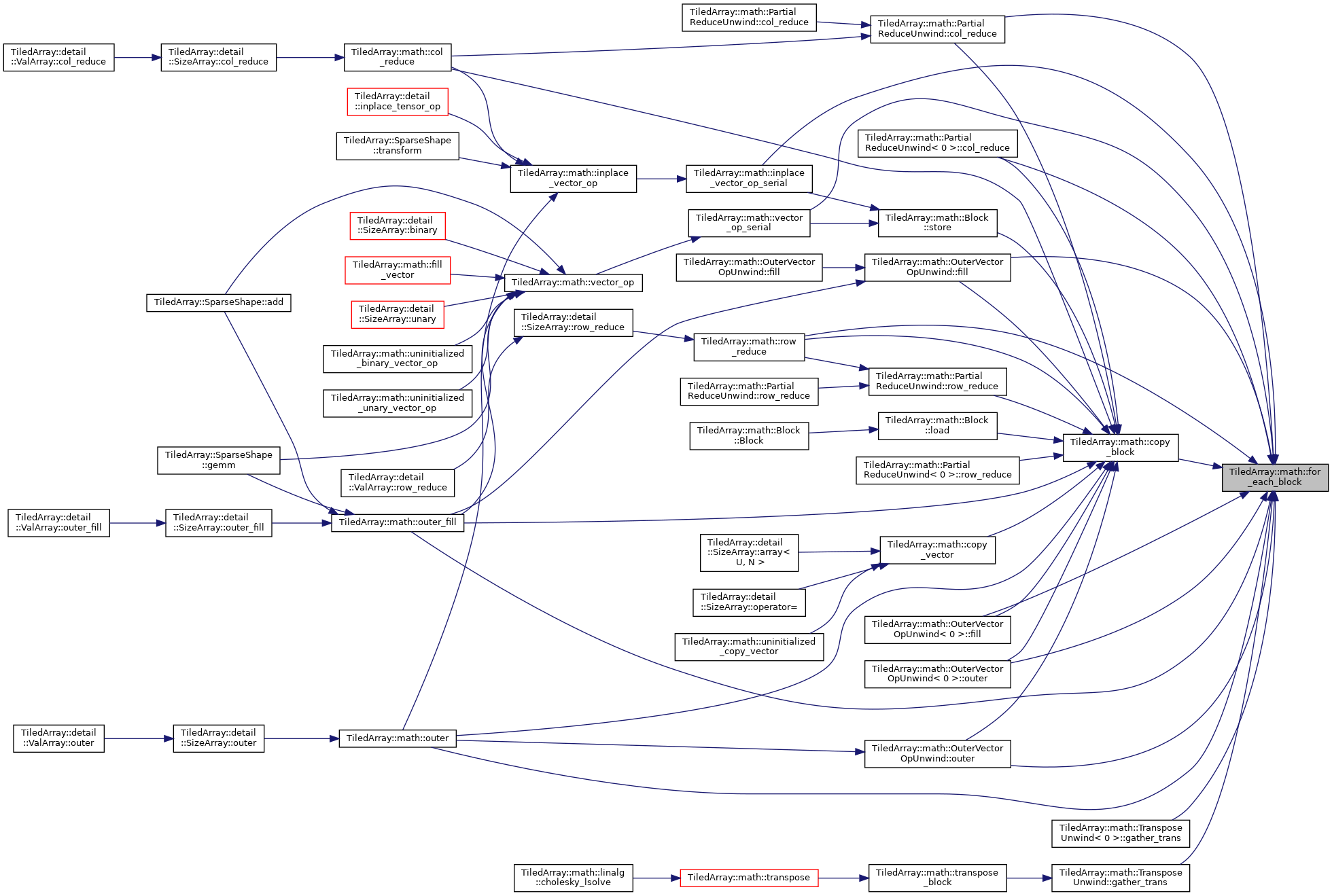
◆ for_each_block_n()
TILEDARRAY_FORCE_INLINE void TiledArray::math::for_each_block_n | ( | Op && | op, |
const std::size_t | n, | ||
Result *MADNESS_RESTRICT const | result, | ||
const Args *MADNESS_RESTRICT const ... | args | ||
) |
◆ for_each_block_ptr() [1/2]
TILEDARRAY_FORCE_INLINE std::enable_if<(sizeof...(Args) > 0)>::type TiledArray::math::for_each_block_ptr | ( | Op && | op, |
Result *const | result, | ||
Block< Args > &&... | args | ||
) |
◆ for_each_block_ptr() [2/2]
TILEDARRAY_FORCE_INLINE std::enable_if<(sizeof...(Args) >= 0)>::type TiledArray::math::for_each_block_ptr | ( | Op && | op, |
Result *const | result, | ||
const Args *const ... | args | ||
) |
Definition at line 182 of file vector_op.h.

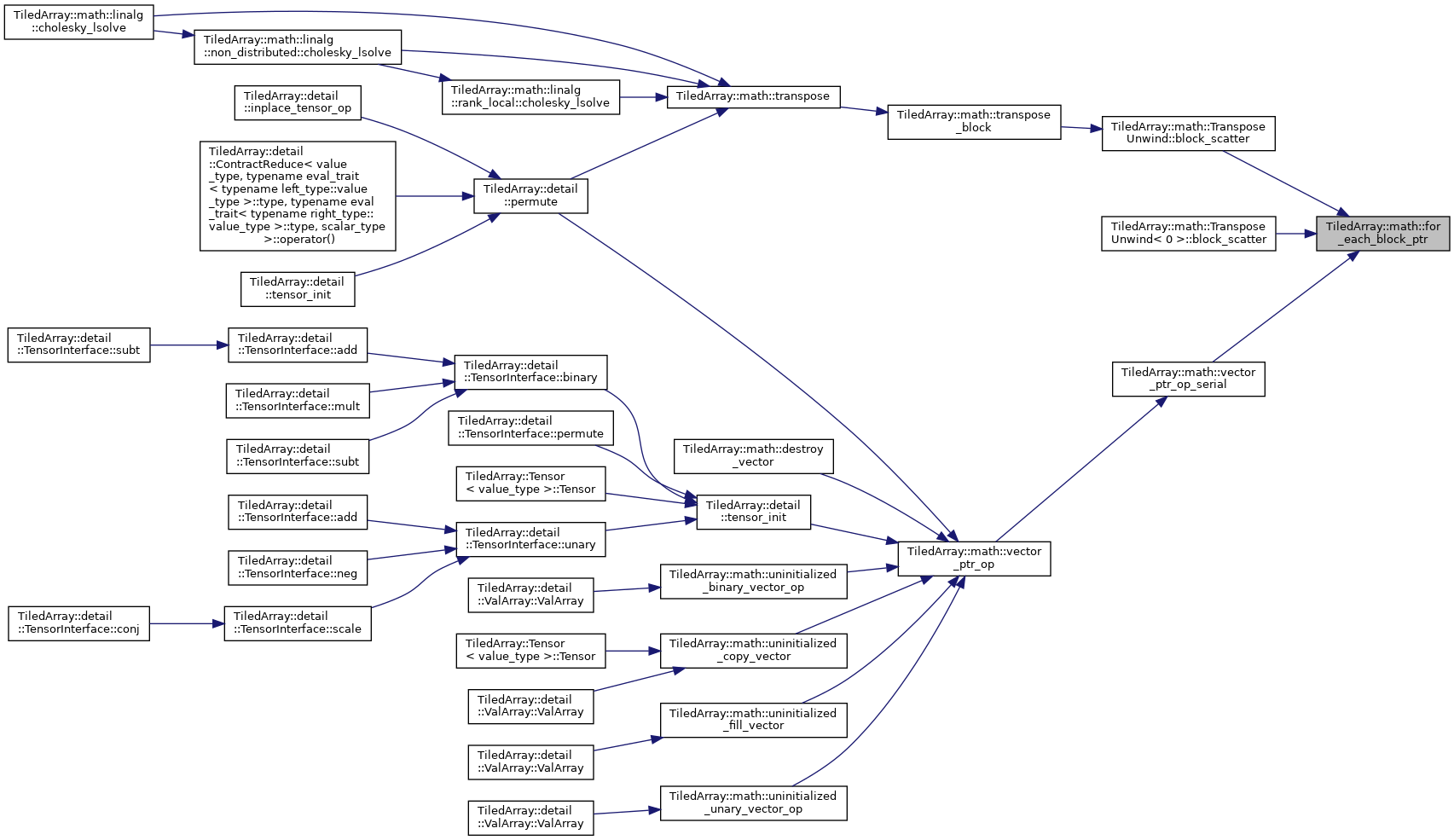
◆ for_each_block_ptr_n()
TILEDARRAY_FORCE_INLINE void TiledArray::math::for_each_block_ptr_n | ( | Op && | op, |
const std::size_t | n, | ||
Result *MADNESS_RESTRICT const | result, | ||
const Args *MADNESS_RESTRICT const ... | args | ||
) |
◆ gather_block()
TILEDARRAY_FORCE_INLINE void TiledArray::math::gather_block | ( | Result *const | result, |
const Arg *const | arg, | ||
const std::size_t | stride | ||
) |
Definition at line 248 of file vector_op.h.

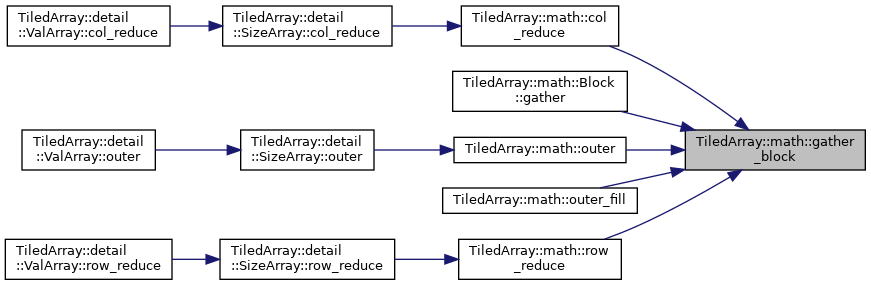
◆ gather_block_n()
TILEDARRAY_FORCE_INLINE void TiledArray::math::gather_block_n | ( | const std::size_t | n, |
Result *const | result, | ||
const Arg *const | arg, | ||
const std::size_t | stride | ||
) |
Definition at line 255 of file vector_op.h.
◆ inplace_vector_op()
void TiledArray::math::inplace_vector_op | ( | Op && | op, |
const std::size_t | n, | ||
Result *const | result, | ||
const Args *const ... | args | ||
) |
Definition at line 391 of file vector_op.h.

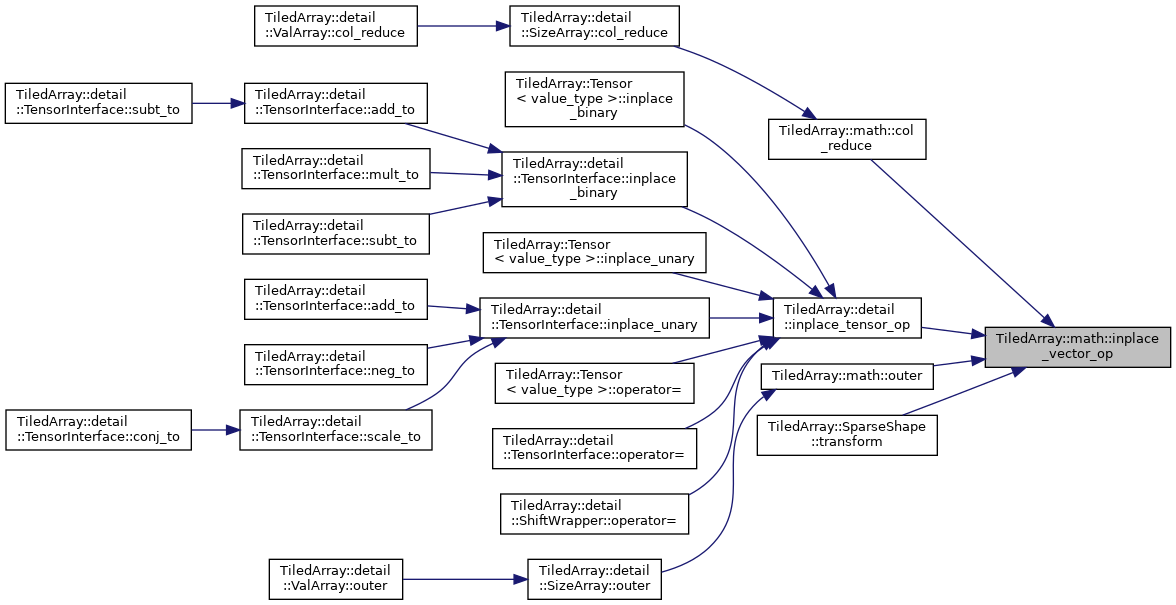
◆ inplace_vector_op_serial()
void TiledArray::math::inplace_vector_op_serial | ( | Op && | op, |
const std::size_t | n, | ||
Result *const | result, | ||
const Args *const ... | args | ||
) |
Definition at line 341 of file vector_op.h.

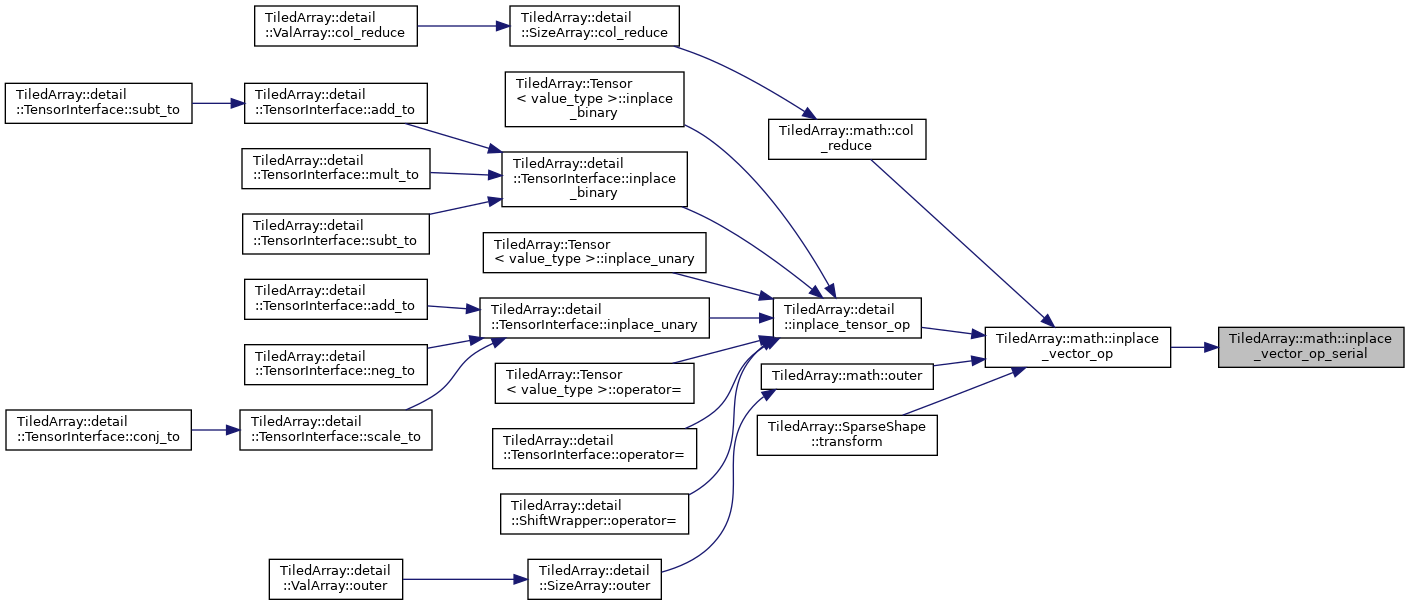
◆ outer()
void TiledArray::math::outer | ( | const std::size_t | m, |
const std::size_t | n, | ||
const X *const | x, | ||
const Y *const | y, | ||
A * | a, | ||
const Op & | op | ||
) |
Compute the outer of x
and y
to modify a
.
Compute op(a[i][j], x[i], y[j])
for each i
and j
pair, where a
[i][j] is modified by op
.
- Template Parameters
-
X The left hand vector element type Y The right-hand vector element type A The a matrix element type Op The operation that will compute outer product elements
- Parameters
-
[in] m The size of the left-hand vector [in] n The size of the right-hand vector [in] x The left-hand vector [in] y The right-hand vector [in,out] a The result matrix of size m*n
[in] op The operation used to generate the result
Definition at line 239 of file outer.h.
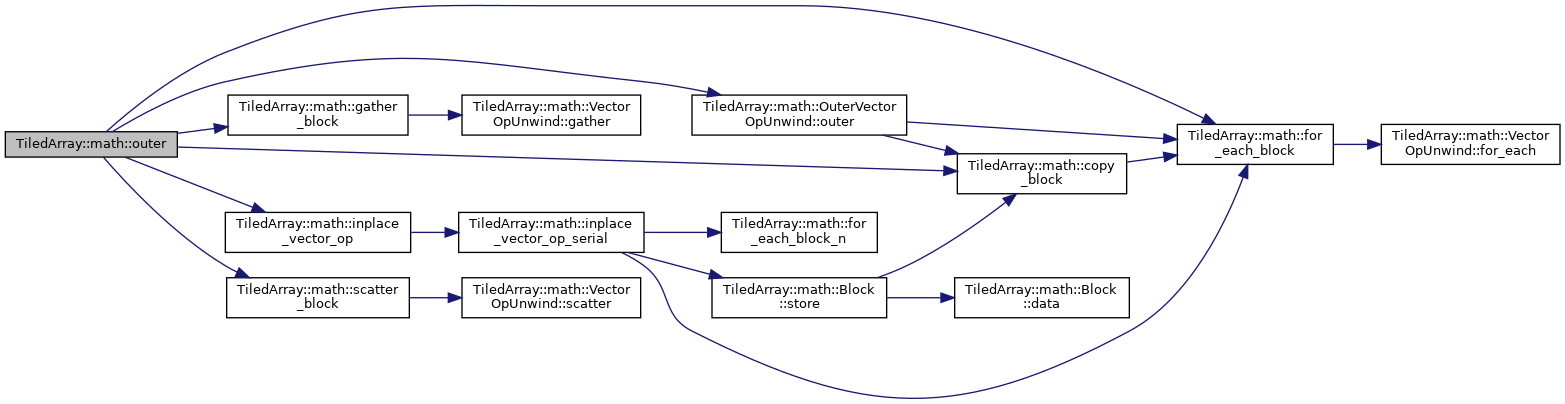

◆ outer_fill() [1/2]
void TiledArray::math::outer_fill | ( | const std::size_t | m, |
const std::size_t | n, | ||
const X *const | x, | ||
const Y *const | y, | ||
A * | a, | ||
const Op & | op | ||
) |
Compute and store outer of x
and y
in a
.
a[i][j] = op(x[i], y[j])
.
- Template Parameters
-
X The left-hand vector element type Y The right-hand vector element type A The a matrix element type
- Parameters
-
[in] m The size of the left-hand vector [in] n The size of the right-hand vector [in] x The left-hand vector [in] y The right-hand vector [out] a The result matrix of size m*n
[in] op The operation that will compute the outer product elements
Definition at line 175 of file outer.h.
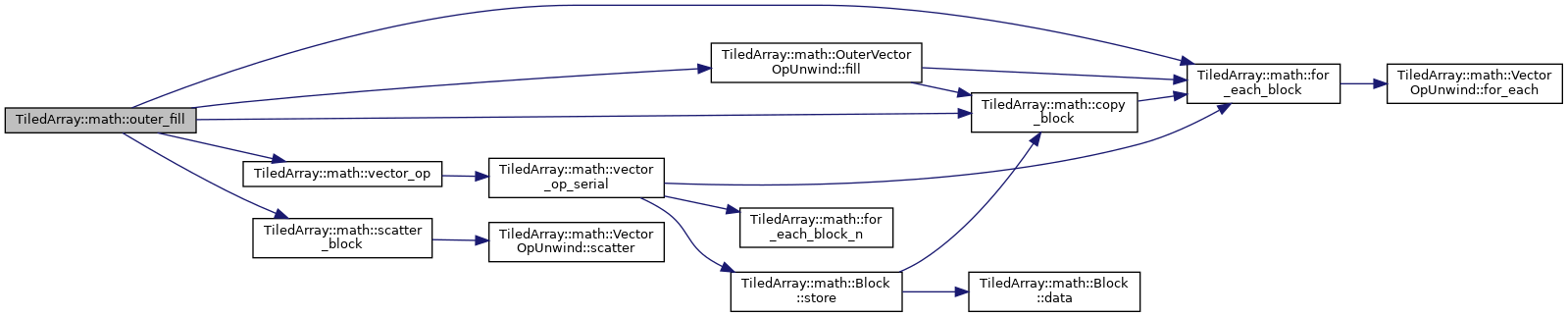
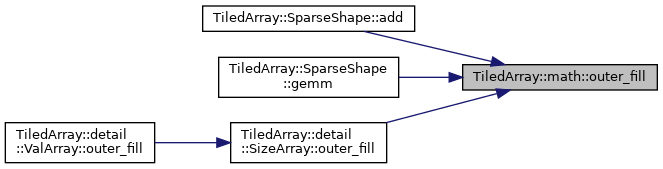
◆ outer_fill() [2/2]
void TiledArray::math::outer_fill | ( | const std::size_t | m, |
const std::size_t | n, | ||
const X *MADNESS_RESTRICT const | x, | ||
const Y *MADNESS_RESTRICT const | y, | ||
const A *MADNESS_RESTRICT | a, | ||
B *MADNESS_RESTRICT | b, | ||
const Op & | op | ||
) |
Compute the outer of x
, y
, and a
, and store the result in b
.
Store a modified copy of a
in b
, where modified elements are generated using the following algorithm:
for each unique pair of i
and j
.
- Template Parameters
-
X The left hand vector element type Y The right-hand vector element type A The a matrix element type B The b matrix element type Op The operation that will compute outer product elements
- Parameters
-
[in] m The size of the left-hand vector [in] n The size of the right-hand vector [in] x The left-hand vector [in] y The right-hand vector [in] a The input matrix of size m*n
[out] b The output matrix of size m*n
[in] op The operation that will compute the outer product elements
Definition at line 315 of file outer.h.
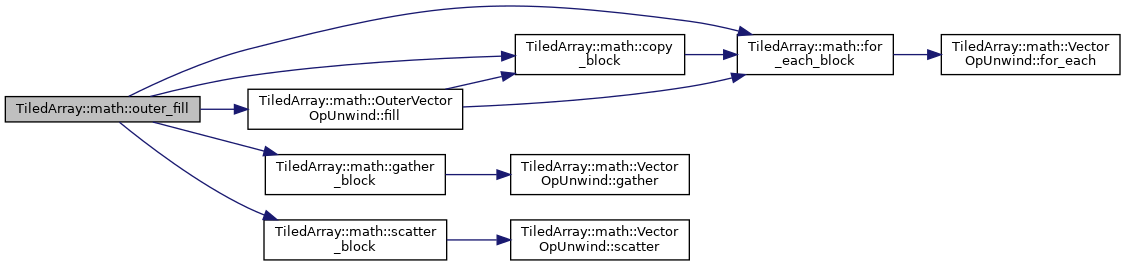
◆ reduce_block() [1/2]
TILEDARRAY_FORCE_INLINE void TiledArray::math::reduce_block | ( | Op && | op, |
Result & | result, | ||
Block< Args > &&... | args | ||
) |
◆ reduce_block() [2/2]
TILEDARRAY_FORCE_INLINE void TiledArray::math::reduce_block | ( | Op && | op, |
Result & | result, | ||
const Args *const ... | args | ||
) |
Definition at line 200 of file vector_op.h.

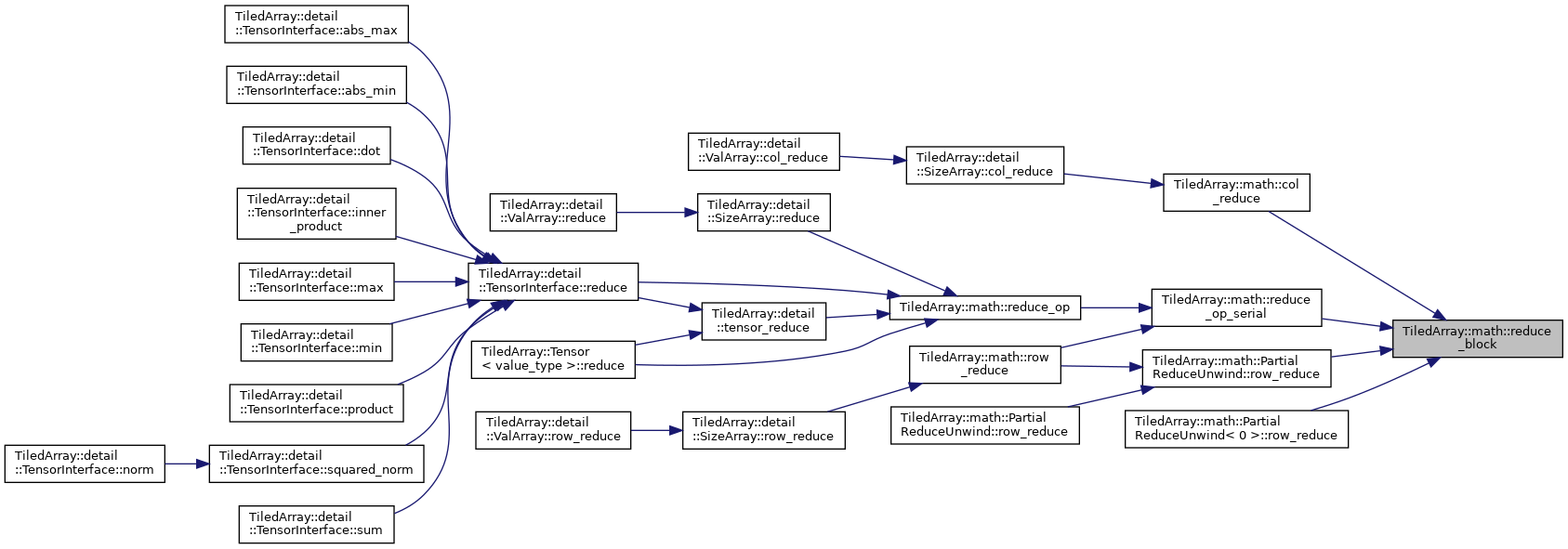
◆ reduce_block_n()
TILEDARRAY_FORCE_INLINE void TiledArray::math::reduce_block_n | ( | Op && | op, |
const std::size_t | n, | ||
Result &MADNESS_RESTRICT | result, | ||
const Args *MADNESS_RESTRICT const ... | args | ||
) |
◆ reduce_op()
void TiledArray::math::reduce_op | ( | ReduceOp && | reduce_op, |
JoinOp && | join_op, | ||
const Result & | identity, | ||
const std::size_t | n, | ||
Result & | result, | ||
const Args *const ... | args | ||
) |
Definition at line 628 of file vector_op.h.

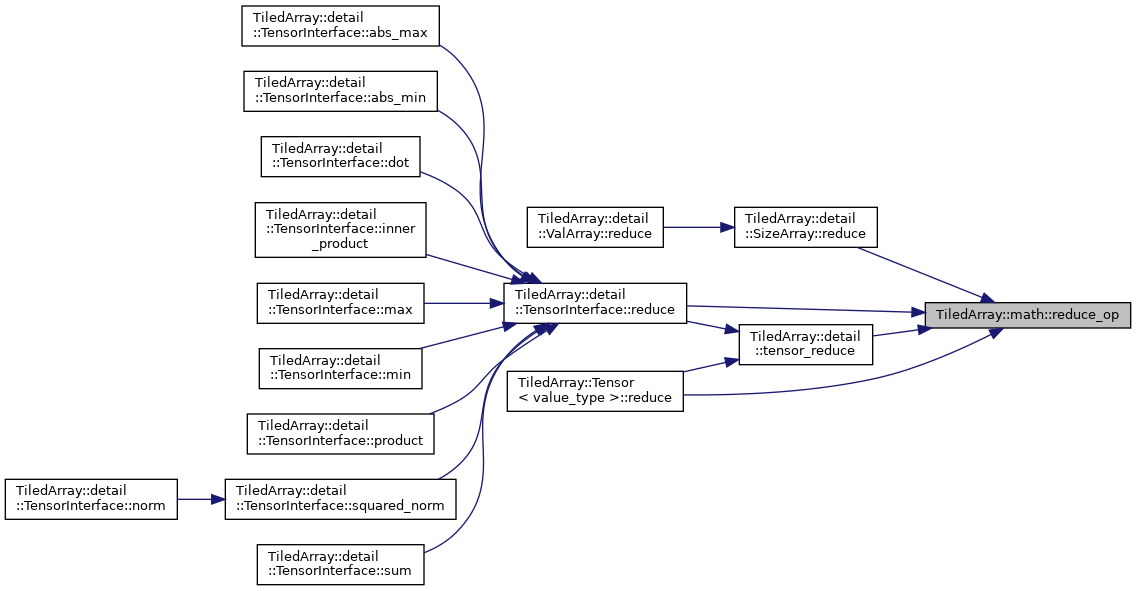
◆ reduce_op_serial()
void TiledArray::math::reduce_op_serial | ( | Op && | op, |
const std::size_t | n, | ||
Result & | result, | ||
const Args *const ... | args | ||
) |
Definition at line 562 of file vector_op.h.

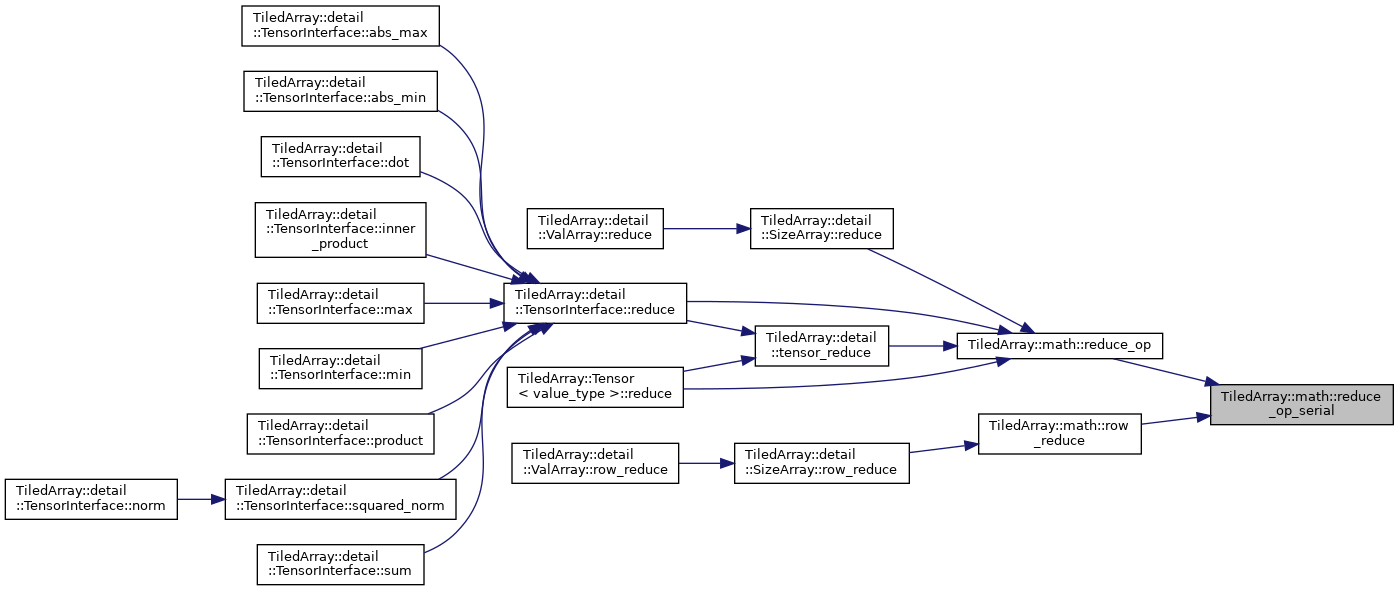
◆ row_reduce() [1/2]
void TiledArray::math::row_reduce | ( | const std::size_t | m, |
const std::size_t | n, | ||
const Arg *MADNESS_RESTRICT const | arg, | ||
Result *MADNESS_RESTRICT const | result, | ||
const Op & | op | ||
) |
Reduce the rows of a matrix.
op(result[i], arg[i][j])
.
- Template Parameters
-
Arg The left-hand vector element type Result The a matrix element type Op The operator type
- Parameters
-
[in] m The number of rows in left [in] n The size of the right-hand vector [in] arg An m*n matrix [out] result The result vector of size m [in] op The operation that will reduce the rows of left
Definition at line 264 of file partial_reduce.h.
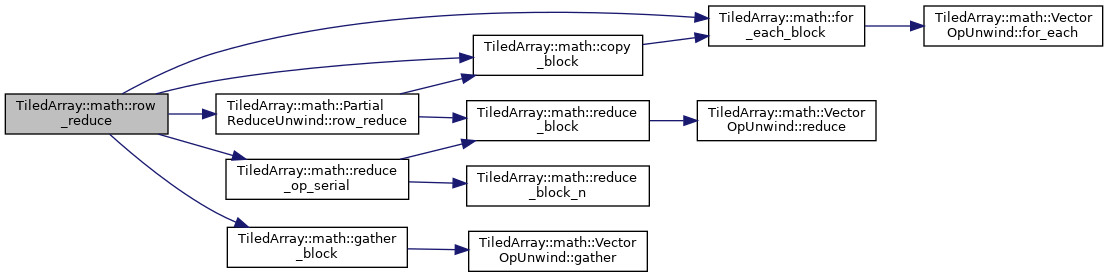
◆ row_reduce() [2/2]
void TiledArray::math::row_reduce | ( | const std::size_t | m, |
const std::size_t | n, | ||
const Left *MADNESS_RESTRICT const | left, | ||
const Right *MADNESS_RESTRICT const | right, | ||
Result *MADNESS_RESTRICT const | result, | ||
const Op & | op | ||
) |
Reduce the rows of a matrix.
op(result[i], left[i][j], right[j])
.
- Template Parameters
-
Left The left-hand matrix element type Right The right-hand vector element type Result The result vector element type
- Parameters
-
[in] m The number of rows in left [in] n The size of the right-hand vector [in] left An m*n matrix [in] right A vector of size n [out] result The result vector of size m [in] op The operation that will reduce the rows of left
Definition at line 195 of file partial_reduce.h.
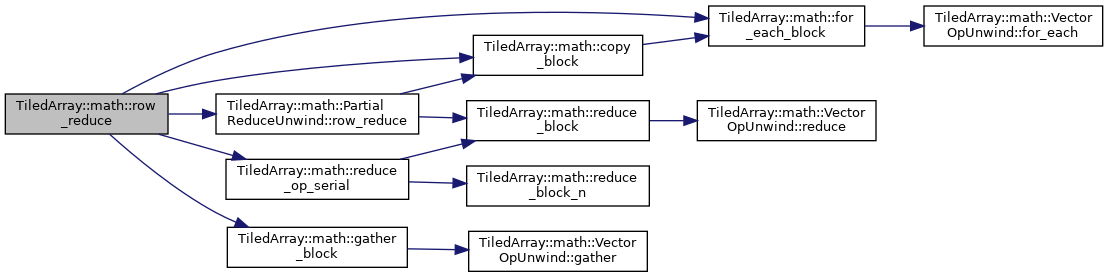

◆ scatter_block()
TILEDARRAY_FORCE_INLINE void TiledArray::math::scatter_block | ( | Result *const | result, |
const std::size_t | stride, | ||
const Arg *const | arg | ||
) |
Definition at line 233 of file vector_op.h.

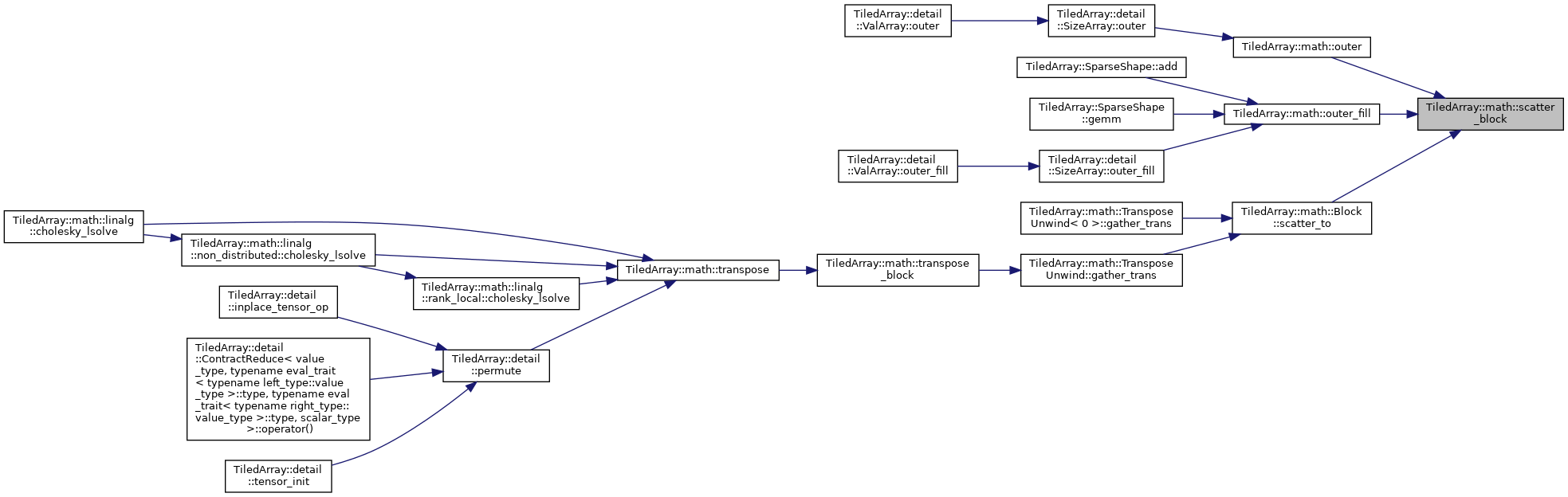
◆ scatter_block_n()
TILEDARRAY_FORCE_INLINE void TiledArray::math::scatter_block_n | ( | const std::size_t | n, |
Result * | result, | ||
const std::size_t | stride, | ||
const Arg *const | arg | ||
) |
Definition at line 240 of file vector_op.h.
◆ transpose()
void TiledArray::math::transpose | ( | InputOp && | input_op, |
OutputOp && | output_op, | ||
const std::size_t | m, | ||
const std::size_t | n, | ||
const std::size_t | result_stride, | ||
Result * | result, | ||
const std::size_t | arg_stride, | ||
const Args *const ... | args | ||
) |
Matrix transpose and initialization.
This function will transpose and transform argument matrices into an uninitialized block of memory
- Template Parameters
-
InputOp The input transform operation type OutputOp The output transform operation type Result The result element type Args The argument element type
- Parameters
-
[in] input_op The transformation operation applied to input arguments [in] output_op The transformation operation used to set the result [in] m The number of rows in the argument matrix [in] n The number of columns in the argument matrix [in] result_stride THe stride between result rows [out] result A pointer to the first element of the result matrix [in] arg_stride The stride between argument rows [in] args A pointer to the first element of the argument matrix
- Note
- The data layout is expected to be row-major.
Definition at line 178 of file transpose.h.

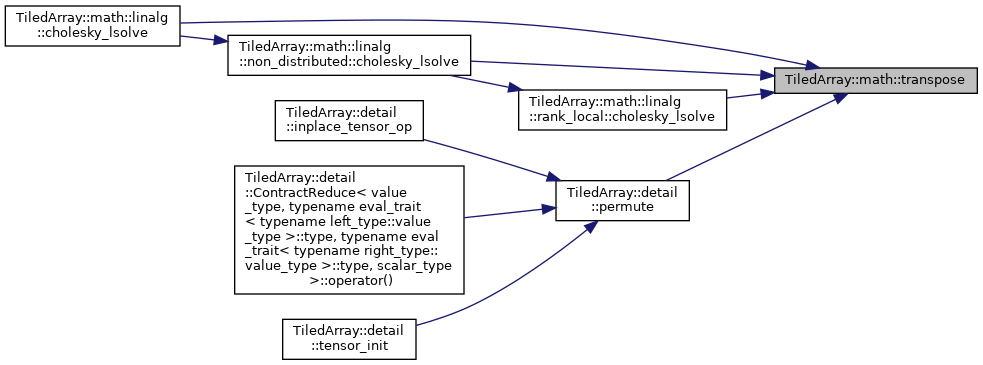
◆ transpose_block() [1/2]
TILEDARRAY_FORCE_INLINE void TiledArray::math::transpose_block | ( | InputOp && | input_op, |
OutputOp && | output_op, | ||
const std::size_t | m, | ||
const std::size_t | n, | ||
const std::size_t | result_stride, | ||
Result *MADNESS_RESTRICT const | result, | ||
const std::size_t | arg_stride, | ||
const Args *MADNESS_RESTRICT const ... | args | ||
) |
Definition at line 130 of file transpose.h.
◆ transpose_block() [2/2]
TILEDARRAY_FORCE_INLINE void TiledArray::math::transpose_block | ( | InputOp && | input_op, |
OutputOp && | output_op, | ||
const std::size_t | result_stride, | ||
Result *const | result, | ||
const std::size_t | arg_stride, | ||
const Args *const ... | args | ||
) |
Definition at line 110 of file transpose.h.
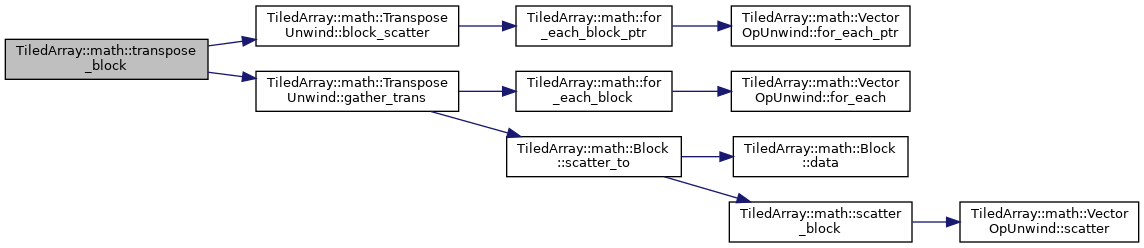
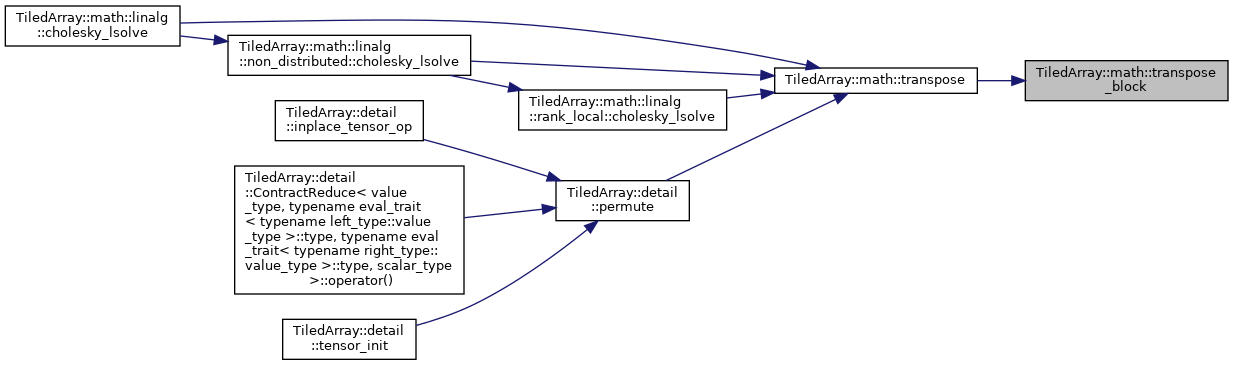
◆ uninitialized_binary_vector_op() [1/2]
std::enable_if<!(detail::is_scalar_v<Left> && detail::is_scalar_v<Right> && detail::is_scalar_v<Result>)>::type TiledArray::math::uninitialized_binary_vector_op | ( | const std::size_t | n, |
const Left *const | left, | ||
const Right *const | right, | ||
Result *const | result, | ||
Op && | op | ||
) |
Definition at line 739 of file vector_op.h.


◆ uninitialized_binary_vector_op() [2/2]
std::enable_if<detail::is_scalar_v<Left> && detail::is_scalar_v<Right> && detail::is_scalar_v<Result> >::type TiledArray::math::uninitialized_binary_vector_op | ( | const std::size_t | n, |
const Left *const | left, | ||
const Right *const | right, | ||
Result *const | result, | ||
Op && | op | ||
) |
◆ uninitialized_copy_vector() [1/2]
std::enable_if<!(detail::is_scalar_v<Arg> && detail::is_scalar_v<Result>)>::type TiledArray::math::uninitialized_copy_vector | ( | const std::size_t | n, |
const Arg *const | arg, | ||
Result *const | result | ||
) |
Definition at line 674 of file vector_op.h.

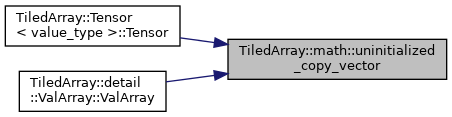
◆ uninitialized_copy_vector() [2/2]
|
inline |
◆ uninitialized_fill_vector() [1/2]
std::enable_if<!(detail::is_scalar_v<Arg> && detail::is_scalar_v<Result>)>::type TiledArray::math::uninitialized_fill_vector | ( | const std::size_t | n, |
const Arg & | arg, | ||
Result *const | result | ||
) |
Definition at line 691 of file vector_op.h.


◆ uninitialized_fill_vector() [2/2]
|
inline |
◆ uninitialized_unary_vector_op() [1/2]
std::enable_if<!(detail::is_scalar_v<Arg> && detail::is_scalar_v<Result>)>::type TiledArray::math::uninitialized_unary_vector_op | ( | const std::size_t | n, |
const Arg *const | arg, | ||
Result *const | result, | ||
Op && | op | ||
) |
Definition at line 719 of file vector_op.h.


◆ uninitialized_unary_vector_op() [2/2]
|
inline |
◆ vector_op()
void TiledArray::math::vector_op | ( | Op && | op, |
const std::size_t | n, | ||
Result *const | result, | ||
const Args *const ... | args | ||
) |
Definition at line 472 of file vector_op.h.

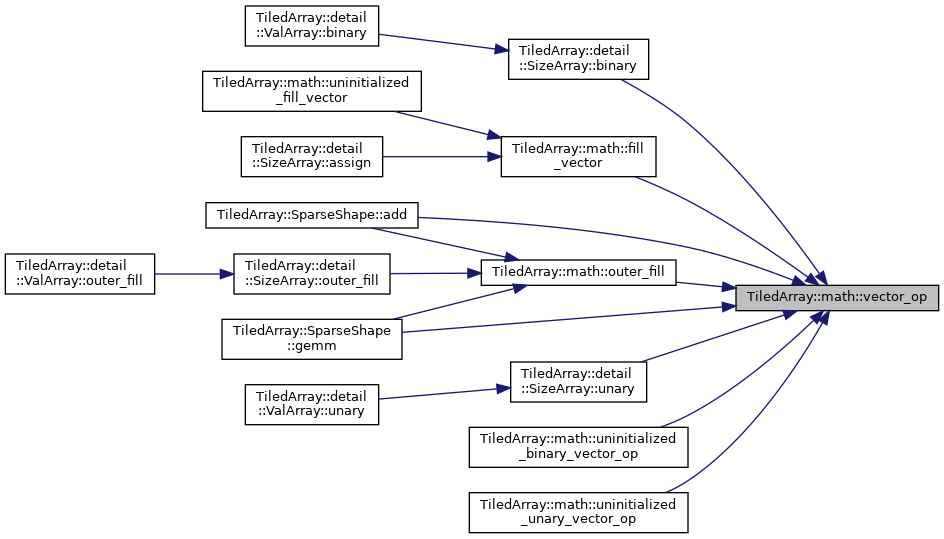
◆ vector_op_serial()
void TiledArray::math::vector_op_serial | ( | Op && | op, |
const std::size_t | n, | ||
Result *const | result, | ||
const Args *const ... | args | ||
) |
Definition at line 418 of file vector_op.h.

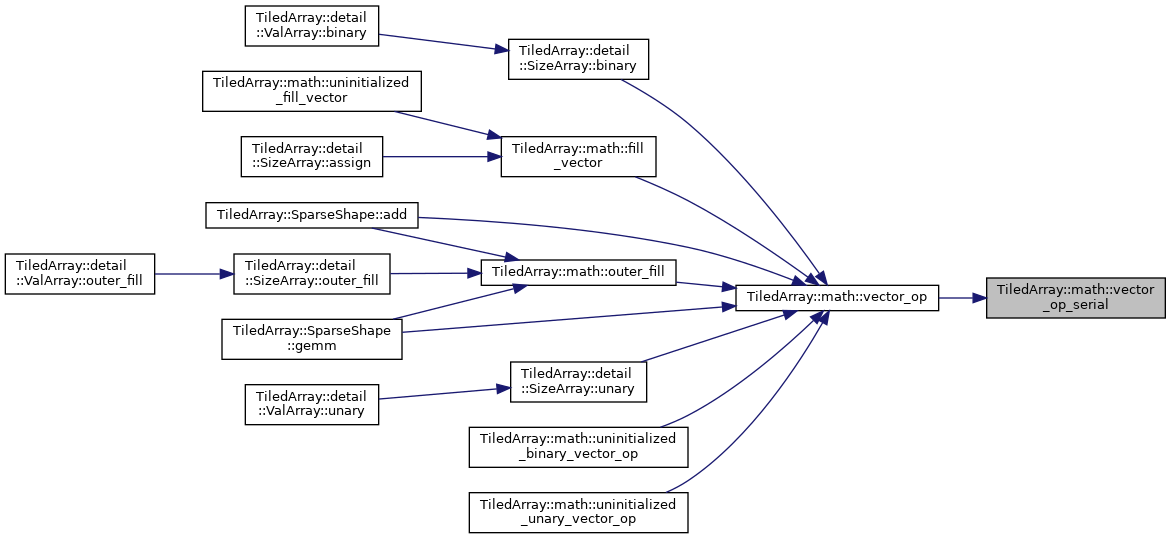
◆ vector_ptr_op()
void TiledArray::math::vector_ptr_op | ( | Op && | op, |
const std::size_t | n, | ||
Result *const | result, | ||
const Args *const ... | args | ||
) |
Definition at line 538 of file vector_op.h.

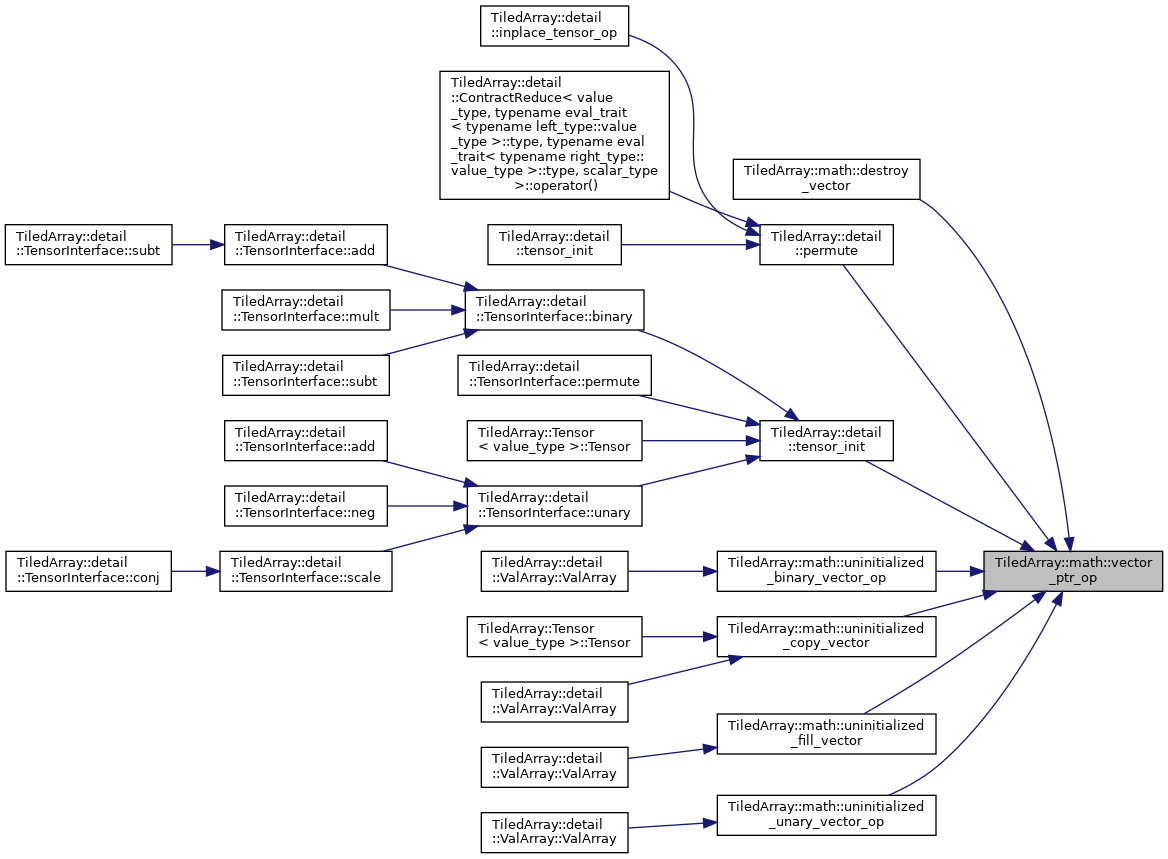
◆ vector_ptr_op_serial()
void TiledArray::math::vector_ptr_op_serial | ( | Op && | op, |
const std::size_t | n, | ||
Result *const | result, | ||
const Args *const ... | args | ||
) |
Definition at line 496 of file vector_op.h.

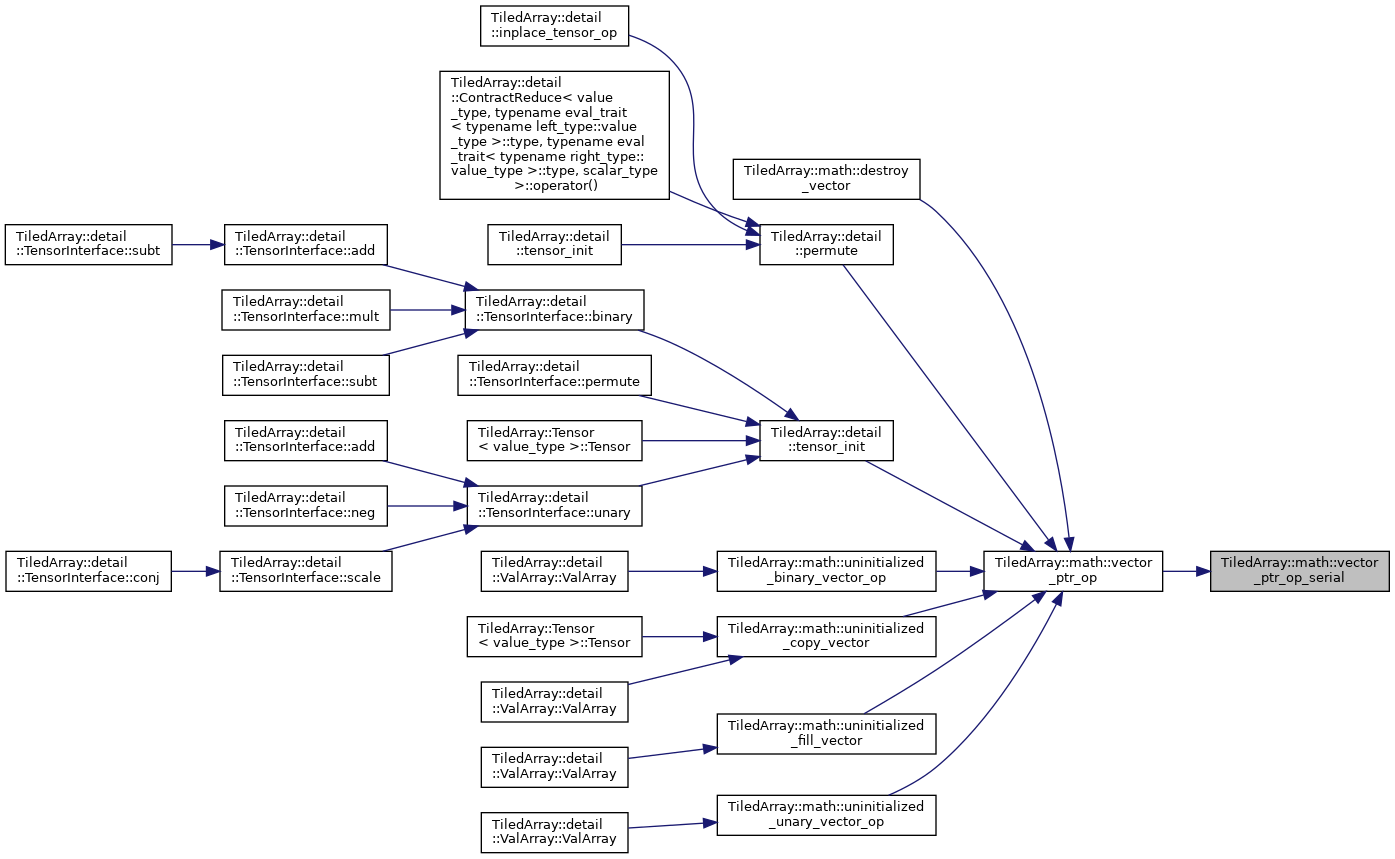