Typedefs | |
using | integer = math::blas::integer |
using | Job = ::lapack::Job |
template<typename T , int Options = ::Eigen::ColMajor> | |
using | Matrix = ::Eigen::Matrix< T, ::Eigen::Dynamic, ::Eigen::Dynamic, Options > |
Functions | |
template<typename T > | |
void | cholesky (Matrix< T > &A) |
template<typename T > | |
void | cholesky_linv (Matrix< T > &A) |
template<typename T > | |
void | cholesky_solve (Matrix< T > &A, Matrix< T > &X) |
template<typename T > | |
void | cholesky_lsolve (Op transpose, Matrix< T > &A, Matrix< T > &X) |
template<typename T > | |
void | heig (Matrix< T > &A, std::vector< T > &W) |
template<typename T > | |
void | heig (Matrix< T > &A, Matrix< T > &B, std::vector< T > &W) |
template<typename T > | |
void | svd (Job jobu, Job jobvt, Matrix< T > &A, std::vector< T > &S, Matrix< T > *U, Matrix< T > *VT) |
template<typename T > | |
void | lu_solve (Matrix< T > &A, Matrix< T > &B) |
template<typename T > | |
void | lu_inv (Matrix< T > &A) |
TA_LAPACK_EXPLICIT (Matrix< double >, std::vector< double >) | |
TA_LAPACK_EXPLICIT (Matrix< float >, std::vector< float >) | |
template<typename T > | |
void | svd (Matrix< T > &A, std::vector< T > &S, Matrix< T > *U, Matrix< T > *VT) |
Typedef Documentation
◆ integer
using TiledArray::math::linalg::rank_local::integer = typedef math::blas::integer |
Definition at line 69 of file rank-local.cpp.
◆ Job
using TiledArray::math::linalg::rank_local::Job = typedef ::lapack::Job |
Definition at line 13 of file rank-local.h.
◆ Matrix
template<typename T , int Options = ::Eigen::ColMajor>
using TiledArray::math::linalg::rank_local::Matrix = typedef ::Eigen::Matrix<T, ::Eigen::Dynamic, ::Eigen::Dynamic, Options> |
Definition at line 16 of file rank-local.h.
Function Documentation
◆ cholesky()
template<typename T >
void TiledArray::math::linalg::rank_local::cholesky | ( | Matrix< T > & | A | ) |
◆ cholesky_linv()
template<typename T >
void TiledArray::math::linalg::rank_local::cholesky_linv | ( | Matrix< T > & | A | ) |
◆ cholesky_lsolve()
template<typename T >
void TiledArray::math::linalg::rank_local::cholesky_lsolve | ( | Op | transpose, |
Matrix< T > & | A, | ||
Matrix< T > & | X | ||
) |
Definition at line 103 of file rank-local.cpp.
Here is the call graph for this function:

Here is the caller graph for this function:

◆ cholesky_solve()
◆ heig() [1/2]
template<typename T >
void TiledArray::math::linalg::rank_local::heig | ( | Matrix< T > & | A, |
Matrix< T > & | B, | ||
std::vector< T > & | W | ||
) |
Definition at line 128 of file rank-local.cpp.
◆ heig() [2/2]
template<typename T >
void TiledArray::math::linalg::rank_local::heig | ( | Matrix< T > & | A, |
std::vector< T > & | W | ||
) |
◆ lu_inv()
template<typename T >
void TiledArray::math::linalg::rank_local::lu_inv | ( | Matrix< T > & | A | ) |
◆ lu_solve()
◆ svd() [1/2]
template<typename T >
void TiledArray::math::linalg::rank_local::svd | ( | Job | jobu, |
Job | jobvt, | ||
Matrix< T > & | A, | ||
std::vector< T > & | S, | ||
Matrix< T > * | U, | ||
Matrix< T > * | VT | ||
) |
Definition at line 143 of file rank-local.cpp.
Here is the call graph for this function:

Here is the caller graph for this function:
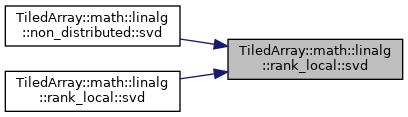
◆ svd() [2/2]
◆ TA_LAPACK_EXPLICIT() [1/2]
TiledArray::math::linalg::rank_local::TA_LAPACK_EXPLICIT | ( | Matrix< double > | , |
std::vector< double > | |||
) |
◆ TA_LAPACK_EXPLICIT() [2/2]
TiledArray::math::linalg::rank_local::TA_LAPACK_EXPLICIT | ( | Matrix< float > | , |
std::vector< float > | |||
) |