Namespaces | |
detail | |
Classes | |
class | Permutation |
Permutation of a sequence of objects indexed by base-0 indices. More... | |
class | PermutationGroup |
Permutation group. More... | |
class | Representation |
class | SymmetricGroup |
Symmetric group. More... | |
Functions | |
bool | operator== (const Permutation &p1, const Permutation &p2) |
Permutation equality operator. More... | |
bool | operator!= (const Permutation &p1, const Permutation &p2) |
Permutation inequality operator. More... | |
bool | operator< (const Permutation &p1, const Permutation &p2) |
Permutation less-than operator. More... | |
std::ostream & | operator<< (std::ostream &output, const Permutation &p) |
Add permutation to an output stream. More... | |
Permutation | operator- (const Permutation &perm) |
Inverse permutation operator. More... | |
Permutation | operator* (const Permutation &p1, const Permutation &p2) |
Permutation multiplication operator. More... | |
Permutation & | operator*= (Permutation &p1, const Permutation &p2) |
return *this ^ other More... | |
Permutation | operator^ (const Permutation &perm, int n) |
Raise perm to the n-th power. More... | |
template<typename T , std::size_t N> | |
std::array< T, N > | operator* (const Permutation &perm, const std::array< T, N > &a) |
Permute a std::array . More... | |
template<typename T , std::size_t N> | |
std::array< T, N > & | operator*= (std::array< T, N > &a, const Permutation &perm) |
In-place permute a std::array . More... | |
template<typename T , typename A > | |
std::vector< T > | operator* (const Permutation &perm, const std::vector< T, A > &v) |
permute a std::vector<T> More... | |
template<typename T , typename A > | |
std::vector< T, A > & | operator*= (std::vector< T, A > &v, const Permutation &perm) |
In-place permute a std::array . More... | |
template<typename T , std::size_t N> | |
boost::container::small_vector< T, N > | operator* (const Permutation &perm, const boost::container::small_vector< T, N > &v) |
permute a boost::container::small_vector<T> More... | |
template<typename T , std::size_t N> | |
boost::container::small_vector< T, N > & | operator*= (boost::container::small_vector< T, N > &v, const Permutation &perm) |
In-place permute a boost::container::small_vector . More... | |
bool | operator== (const PermutationGroup &p1, const PermutationGroup &p2) |
PermutationGroup equality operator. More... | |
bool | operator!= (const PermutationGroup &p1, const PermutationGroup &p2) |
PermutationGroup inequality operator. More... | |
bool | operator< (const PermutationGroup &p1, const PermutationGroup &p2) |
PermutationGroup less-than operator. More... | |
std::ostream & | operator<< (std::ostream &output, const PermutationGroup &p) |
Add permutation group to an output stream. More... | |
template<typename MultiIndex > | |
bool | is_lexicographically_smallest (const MultiIndex &idx, const PermutationGroup &pg) |
PermutationGroup | conjugate (const PermutationGroup &G, const PermutationGroup::Permutation &h) |
Computes conjugate permutation group obtained by the action of a permutation. More... | |
PermutationGroup | intersect (const PermutationGroup &G1, const PermutationGroup &G2) |
template<typename Set > | |
PermutationGroup | stabilizer (const PermutationGroup &G, const Set &f) |
template<typename T > | |
T | identity () |
identity for group of objects of type T More... | |
Function Documentation
◆ identity()
T TiledArray::symmetry::identity | ( | ) |
identity for group of objects of type T
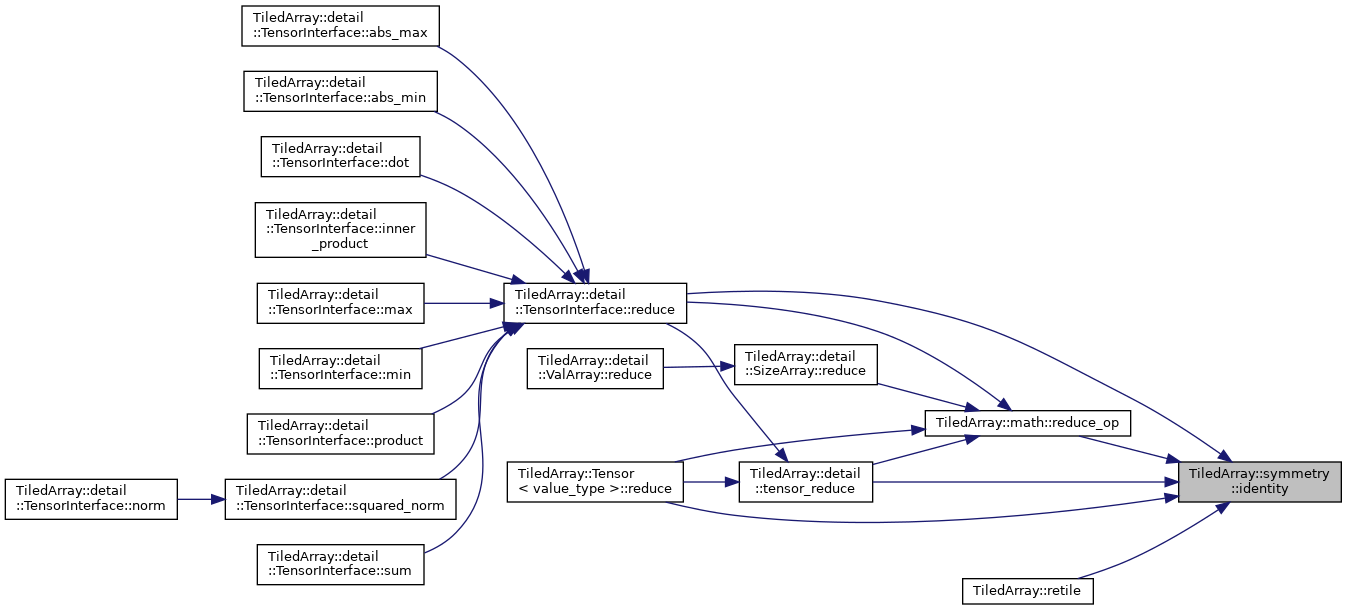
◆ operator!=()
|
inline |
Permutation inequality operator.
- Parameters
-
p1 The left-hand permutation to be compared p2 The right-hand permutation to be compared
- Returns
true
if any element ofp1
is not equal to that ofp2
, otherwisefalse
.
Definition at line 460 of file permutation.h.

◆ operator*() [1/4]
|
inline |
Permutation multiplication operator.
- Parameters
-
p1 The left-hand permutation p2 The right-hand permutation
- Returns
- The product of p1 and p2 (which is the permutation of
p2
byp1
).
Definition at line 500 of file permutation.h.

◆ operator*() [2/4]
|
inline |
permute a boost::container::small_vector<T>
- Template Parameters
-
T The element type of the vector N The max static size of the vector
- Parameters
-
perm The permutation v The vector to be permuted
- Returns
- A permuted copy of
v
- Exceptions
-
TiledArray::Exception When the dimension of the permutation is not equal to the size of v
.
Definition at line 630 of file permutation.h.

◆ operator*() [3/4]
|
inline |
Permute a std::array
.
- Template Parameters
-
T The element type of the array N The size of the array
- Parameters
-
perm The permutation a The array to be permuted
- Returns
- A permuted copy of
a
- Exceptions
-
TiledArray::Exception When the dimension of the permutation is not equal to the size of a
.
Definition at line 562 of file permutation.h.

◆ operator*() [4/4]
|
inline |
permute a std::vector<T>
- Template Parameters
-
T The element type of the vector A The allocator type of the vector
- Parameters
-
perm The permutation v The vector to be permuted
- Returns
- A permuted copy of
v
- Exceptions
-
TiledArray::Exception When the dimension of the permutation is not equal to the size of v
.
Definition at line 596 of file permutation.h.

◆ operator*=() [1/4]
|
inline |
In-place permute a boost::container::small_vector
.
- Template Parameters
-
T The element type of the vector N The max static size of the vector
- Parameters
-
[out] v The vector to be permuted [in] perm The permutation
- Returns
- A reference to
v
- Exceptions
-
TiledArray::Exception When the dimension of the permutation is not equal to the size of v
.
Definition at line 647 of file permutation.h.

◆ operator*=() [2/4]
|
inline |
return *this ^ other
Definition at line 505 of file permutation.h.
◆ operator*=() [3/4]
|
inline |
In-place permute a std::array
.
- Template Parameters
-
T The element type of the array N The size of the array
- Parameters
-
[out] a The array to be permuted [in] perm The permutation
- Returns
- A reference to
a
- Exceptions
-
TiledArray::Exception When the dimension of the permutation is not equal to the size of a
.
Definition at line 579 of file permutation.h.

◆ operator*=() [4/4]
|
inline |
In-place permute a std::array
.
- Template Parameters
-
T The element type of the vector A The allocator type of the vector
- Parameters
-
[out] v The vector to be permuted [in] perm The permutation
- Returns
- A reference to
v
- Exceptions
-
TiledArray::Exception When the dimension of the permutation is not equal to the size of v
.
Definition at line 613 of file permutation.h.

◆ operator-()
|
inline |
Inverse permutation operator.
- Parameters
-
perm The permutation to be inverted
- Returns
perm.inverse()
Definition at line 492 of file permutation.h.

◆ operator<()
|
inline |
Permutation less-than operator.
- Parameters
-
p1 The left-hand permutation to be compared p2 The right-hand permutation to be compared
- Returns
true
if the elements ofp1
are lexicographically less than that ofp2
, otherwisefalse
.
Definition at line 470 of file permutation.h.

◆ operator<<()
|
inline |
Add permutation to an output stream.
- Parameters
-
[out] output The output stream [in] p The permutation to be added to the output stream
- Returns
- The output stream
Definition at line 481 of file permutation.h.

◆ operator==()
|
inline |
Permutation equality operator.
- Parameters
-
p1 The left-hand permutation to be compared p2 The right-hand permutation to be compared
- Returns
true
if all elements ofp1
andp2
are equal and in the same order, otherwisefalse
.
Definition at line 450 of file permutation.h.
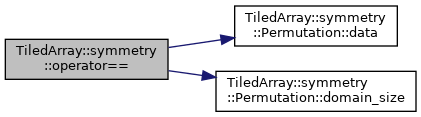

◆ operator^()
|
inline |
Raise perm
to the n-th power.
Constructs the permutation , where
is the permutation
perm
.
- Parameters
-
perm The base permutation n Exponent value
- Returns
- This permutation raised to the n-th power
Definition at line 516 of file permutation.h.
