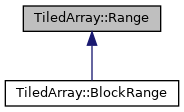
Documentation
A (hyperrectangular) interval on , space of integer
-indices.
This object is a range of integers on a -dimensional, hyperrectangular domain, where rank (aka order, number of dimensions)
. It is fully specified by its lower and upper bounds. It also provides zero-cost access to extent (size) and stride for each mode (dimension). Range is a regular type with null default state.
- Warning
- Range is with rank 0 is null, i.e. invalid. There are many reasons that rank-0 Range is not supported; summarily, rank-0 Range is not a special case of rank-
Range as many invariants of nonzero-rank Range are not observed by it. E.g. for any nonempty nonzero-rank Range the lower bound differs from its upper bound. To define the 0-dimensional limit of array/tensor to be a scalar, the volume of rank-0 Range should be 1, but clearly its lower and upper bounds are equal.
Public Types | |
typedef Range | Range_ |
This object type. More... | |
typedef TA_1INDEX_TYPE | index1_type |
typedef container::svector< index1_type > | index_type |
typedef index_type | index |
Coordinate index type (deprecated) More... | |
typedef detail::SizeArray< const index1_type > | index_view_type |
Non-owning variant of index_type. More... | |
typedef index_type | extent_type |
Range extent type, to conform to TWG spec. More... | |
typedef std::size_t | ordinal_type |
Ordinal type, to conform to TWG spec. More... | |
typedef std::make_signed_t< ordinal_type > | distance_type |
Distance type. More... | |
typedef ordinal_type | size_type |
Size type (deprecated) More... | |
typedef detail::RangeIterator< index1_type, Range_ > | const_iterator |
Coordinate iterator. More... | |
Public Member Functions | |
Range () | |
Default constructor. More... | |
template<typename Index1 , typename Index2 , typename std::enable_if_t< detail::is_integral_sized_range_v< Index1 > &&detail::is_integral_sized_range_v< Index2 >> * = nullptr> | |
Range (const Index1 &lower_bound, const Index2 &upper_bound) | |
Construct range defined by upper and lower bound ranges. More... | |
template<typename Index1 , typename Index2 , typename = std::enable_if_t<std::is_integral_v<Index1> && std::is_integral_v<Index2>>> | |
Range (const std::initializer_list< Index1 > &lower_bound, const std::initializer_list< Index2 > &upper_bound) | |
Construct range defined by the upper and lower bound ranges. More... | |
template<typename Index , typename std::enable_if_t< detail::is_integral_sized_range_v< Index >> * = nullptr> | |
Range (const Index &extent) | |
Range constructor from a range of extents. More... | |
template<typename Index , typename = std::enable_if_t<std::is_integral_v<Index>>> | |
Range (const std::initializer_list< Index > &extent) | |
Range constructor from an initializer list of extents. More... | |
template<typename PairRange , typename = std::enable_if_t<detail::is_sized_range_v<PairRange> && detail::is_gpair_range_v<PairRange>>> | |
Range (const PairRange &bounds) | |
Construct Range defined by a range of {lower,upper} bound pairs. More... | |
template<typename GPair > | |
Range (const std::initializer_list< GPair > &bounds, std::enable_if_t< detail::is_gpair_v< GPair >> *=nullptr) | |
Construct range defined by an initializer_list of {lower,upper} bounds for each dimension given as a generalized pair. More... | |
template<typename Index , typename = std::enable_if_t<std::is_integral_v<Index>>> | |
Range (const std::initializer_list< std::initializer_list< Index >> &bounds) | |
Construct range defined by an initializer_list of std::initializer_list{lower,upper} bounds. More... | |
template<typename... Index, typename std::enable_if< detail::is_integral_list< Index... >::value >::type * = nullptr> | |
Range (const Index... extents) | |
Range constructor from a pack of extents for each dimension. More... | |
template<typename... IndexPairs, std::enable_if_t< detail::is_integral_pair_list_v< IndexPairs... >> * = nullptr> | |
Range (const IndexPairs... bounds) | |
Range (const Range_ &other)=default | |
Copy Constructor. More... | |
Range (Range_ &&other) | |
Move Constructor. More... | |
Range (const Permutation &perm, const Range_ &other) | |
Permuting copy constructor. More... | |
~Range ()=default | |
Destructor. More... | |
Range_ & | operator= (const Range_ &other)=default |
Copy assignment operator. More... | |
Range_ & | operator= (Range_ &&other) |
Move assignment operator. More... | |
operator bool () const | |
Conversion to bool. More... | |
unsigned int | rank () const |
Rank accessor. More... | |
std::pair< index1_type, index1_type > | dim (std::size_t d) const |
Accessor of the d-th dimension of the range. More... | |
const index1_type * | lobound_data () const |
Range lower bound data accessor. More... | |
index_view_type | lobound () const |
Range lower bound accessor. More... | |
index1_type | lobound (size_t dim) const |
Range lower bound element accessor. More... | |
const index1_type * | upbound_data () const |
Range upper bound data accessor. More... | |
index_view_type | upbound () const |
Range upper bound accessor. More... | |
index1_type | upbound (size_t dim) const |
Range upped bound element accessor. More... | |
const index1_type * | extent_data () const |
Range extent data accessor. More... | |
index_view_type | extent () const |
Range extent accessor. More... | |
index1_type | extent (size_t dim) const |
Range extent element accessor. More... | |
const index1_type * | stride_data () const |
Range stride data accessor. More... | |
index_view_type | stride () const |
Range stride accessor. More... | |
index1_type | stride (size_t dim) const |
Range stride element accessor. More... | |
ordinal_type | volume () const |
Range volume accessor. More... | |
ordinal_type | area () const |
distance_type | offset () const |
Range offset. More... | |
const_iterator | begin () const |
Index iterator factory. More... | |
const_iterator | end () const |
Index iterator factory. More... | |
template<typename Index , typename std::enable_if< detail::is_integral_range_v< Index >, bool >::type * = nullptr> | |
bool | includes (const Index &index) const |
Check the coordinate to make sure it is within the range. More... | |
template<typename Index , typename = std::enable_if_t<std::is_integral_v<Index>>> | |
bool | includes (const std::initializer_list< Index > &index) const |
Check the coordinate to make sure it is within the range. More... | |
template<typename Ordinal > | |
std::enable_if< std::is_integral_v< Ordinal >, bool >::type | includes (Ordinal i) const |
Check the ordinal index to make sure it is within the range. More... | |
template<typename... Index> | |
std::enable_if_t<(sizeof...(Index) > 1ul) &&(std::is_integral_v< Index > &&...), bool > | includes (const Index &... index) const |
Range_ & | operator*= (const Permutation &perm) |
Permute this range. More... | |
template<typename Index1 , typename Index2 , typename = std::enable_if_t<detail::is_integral_sized_range_v<Index1> && detail::is_integral_sized_range_v<Index2>>> | |
Range_ & | resize (const Index1 &lower_bound, const Index2 &upper_bound) |
Resize range to a new upper and lower bound. More... | |
template<typename Index , typename = std::enable_if_t<detail::is_integral_range_v<Index>>> | |
Range_ & | inplace_shift (const Index &bound_shift) |
Shift the lower and upper bound of this range. More... | |
template<typename Index , typename = std::enable_if_t<std::is_integral_v<Index>>> | |
Range_ & | inplace_shift (const std::initializer_list< Index > &bound_shift) |
Shift the lower and upper bound of this range. More... | |
template<typename Index , typename = std::enable_if_t<detail::is_integral_range_v<Index>>> | |
Range_ | shift (const Index &bound_shift) |
Create a Range with shiften lower and upper bounds. More... | |
template<typename Index , typename = std::enable_if_t<std::is_integral_v<Index>>> | |
Range_ | shift (const std::initializer_list< Index > &bound_shift) |
Create a Range with shiften lower and upper bounds. More... | |
ordinal_type | ordinal (const ordinal_type index) const |
calculate the ordinal index of i More... | |
template<typename Index , typename std::enable_if_t< detail::is_integral_range_v< Index >> * = nullptr> | |
ordinal_type | ordinal (const Index &index) const |
calculate the ordinal index of index More... | |
template<typename Index , typename = std::enable_if_t<std::is_integral_v<Index>>> | |
ordinal_type | ordinal (const std::initializer_list< Index > &index) const |
calculate the ordinal index of index More... | |
template<typename... Index, typename std::enable_if_t<(sizeof...(Index) > 1ul) &&(std::is_integral_v< Index > &&...)> * = nullptr> | |
ordinal_type | ordinal (const Index &... index) const |
calculate the ordinal index of index More... | |
index_type | idx (ordinal_type index) const |
calculate the coordinate index of the ordinal index, index . More... | |
template<typename Index , typename std::enable_if_t< detail::is_integral_range_v< Index >> * = nullptr> | |
const Index & | idx (const Index &i) const |
calculate the index of i More... | |
template<typename Archive > | |
void | serialize (Archive &ar) |
void | swap (Range_ &other) |
Protected Member Functions | |
void | init_datavec (unsigned int rank) |
const index1_type * | data () const |
index1_type * | data () |
index1_type * | lobound_data_nc () |
index1_type * | upbound_data_nc () |
index1_type * | extent_data_nc () |
index1_type * | stride_data_nc () |
Protected Attributes | |
container::svector< index1_type, 4 *TA_MAX_SOO_RANK_METADATA > | datavec_ |
distance_type | offset_ = 0l |
Ordinal index offset correction. More... | |
ordinal_type | volume_ = 0ul |
Total number of elements. More... | |
unsigned int | rank_ = 0u |
The rank (or number of dimensions) in the range. More... | |
Friends | |
class | detail::RangeIterator< index1_type, Range_ > |
Member Typedef Documentation
◆ const_iterator
◆ distance_type
typedef std::make_signed_t<ordinal_type> TiledArray::Range::distance_type |
◆ extent_type
◆ index
typedef index_type TiledArray::Range::index |
◆ index1_type
typedef TA_1INDEX_TYPE TiledArray::Range::index1_type |
◆ index_type
◆ index_view_type
typedef detail::SizeArray<const index1_type> TiledArray::Range::index_view_type |
◆ ordinal_type
typedef std::size_t TiledArray::Range::ordinal_type |
◆ Range_
typedef Range TiledArray::Range::Range_ |
◆ size_type
Constructor & Destructor Documentation
◆ Range() [1/13]
|
inline |
◆ Range() [2/13]
|
inline |
Construct range defined by upper and lower bound ranges.
Construct a range defined by lower_bound
and upper_bound
. Examples of using this constructor:
- Template Parameters
-
Index1 An integral sized range type Index2 An integral sized range type
- Parameters
-
lower_bound A sequence of lower bounds for each dimension upper_bound A sequence of upper bounds for each dimension
- Exceptions
-
TiledArray::Exception When the size of lower_bound
is not equal to that ofupper_bound
.TiledArray::Exception When lower_bound[i] >= upper_bound[i]
Definition at line 355 of file range.h.

◆ Range() [3/13]
|
inline |
Construct range defined by the upper and lower bound ranges.
Construct a range defined by lower_bound
and upper_bound
. Examples of using this constructor:
- Template Parameters
-
Index1 An integral type Index2 An integral type
- Parameters
-
lower_bound An initializer list of lower bounds for each dimension upper_bound An initializer list of upper bounds for each dimension
- Warning
- do not use uniform initialization syntax ("curly braces") to invoke this
- Exceptions
-
TiledArray::Exception When the size of lower_bound
is not equal to that ofupper_bound
.TiledArray::Exception When lower_bound[i] >= upper_bound[i]
Definition at line 389 of file range.h.

◆ Range() [4/13]
|
inlineexplicit |
Range constructor from a range of extents.
Construct a range with a lower bound of zero and an upper bound equal to extents
. Examples of using this constructor:
- Template Parameters
-
Index A vector type
- Parameters
-
extent A vector that defines the size of each dimension
Definition at line 414 of file range.h.

◆ Range() [5/13]
|
inlineexplicit |
Range constructor from an initializer list of extents.
Construct a range with a lower bound of zero and an upper bound equal to extent
. Examples of using this constructor:
- Template Parameters
-
Index An integral type
- Parameters
-
extent An initializer list that defines the size of each dimension
Definition at line 437 of file range.h.

◆ Range() [6/13]
|
inlineexplicit |
Construct Range defined by a range of {lower,upper} bound pairs.
Examples of using this constructor:
- Template Parameters
-
PairRange Type representing a sized range of generalized pairs (see TiledArray::detail::is_gpair_v )
- Parameters
-
bound A range of {lower,upper} bounds for each dimension
- Exceptions
-
TiledArray::Exception When bound[i].lower>=bound[i].upper
for anyi
.
Definition at line 480 of file range.h.

◆ Range() [7/13]
|
inlineexplicit |
Construct range defined by an initializer_list of {lower,upper} bounds for each dimension given as a generalized pair.
Examples of using this constructor:
- Template Parameters
-
GPair a generalized pair of integral types
- Parameters
-
bound A sequence of {lower,upper} bounds for each dimension
- Exceptions
-
TiledArray::Exception When bound
[i].lower>=bound[i].upper for anyi
.
Definition at line 502 of file range.h.

◆ Range() [8/13]
|
inlineexplicit |
Construct range defined by an initializer_list of std::initializer_list{lower,upper} bounds.
Examples of using this constructor:
- Template Parameters
-
Index An integral type
- Parameters
-
bound A sequence of {lower,upper} bounds for each dimension
- Exceptions
-
TiledArray::Exception When bound[i].lower>=bound[i].upper
for anyi
.
Definition at line 537 of file range.h.

◆ Range() [9/13]
|
inlineexplicit |
◆ Range() [10/13]
|
inlineexplicit |
Range constructor from a pack of std::pair{lo,up} bounds for each dimension Examples of using this constructor:
- Template Parameters
-
IndexPairs Pack of std::pair's of integer types
- Parameters
-
extents A pack of pairs of lobound and upbound for each dimension
◆ Range() [11/13]
|
default |
Copy Constructor.
- Parameters
-
other The range to be copied
◆ Range() [12/13]
|
inline |
◆ Range() [13/13]
|
inline |
◆ ~Range()
|
default |
Destructor.
Member Function Documentation
◆ area()
|
inline |
◆ begin()
|
inline |
Index iterator factory.
The iterator dereferences to an index. The order of iteration matches the data layout of a row-major tensor.
- Returns
- An iterator that holds the lower bound index of a tensor (unless it has zero volume, then it returns same result as end())
- Exceptions
-
nothing
Definition at line 803 of file range.h.

◆ data() [1/2]
|
inlineprotected |
◆ data() [2/2]
|
inlineprotected |
◆ dim()
|
inline |
◆ end()
|
inline |
Index iterator factory.
The iterator dereferences to an index. The order of iteration matches the data layout of a row-major tensor.
- Returns
- An iterator that holds the upper bound element index of a tensor
- Exceptions
-
nothing
Definition at line 813 of file range.h.


◆ extent() [1/2]
|
inline |
◆ extent() [2/2]
|
inline |
◆ extent_data()
|
inline |
Range extent data accessor.
- Returns
- A pointer to the extent data (see Range::extent() )
- Note
- Not necessarily nullptr for rank-0 or null Range
- Exceptions
-
nothing
Definition at line 735 of file range.h.

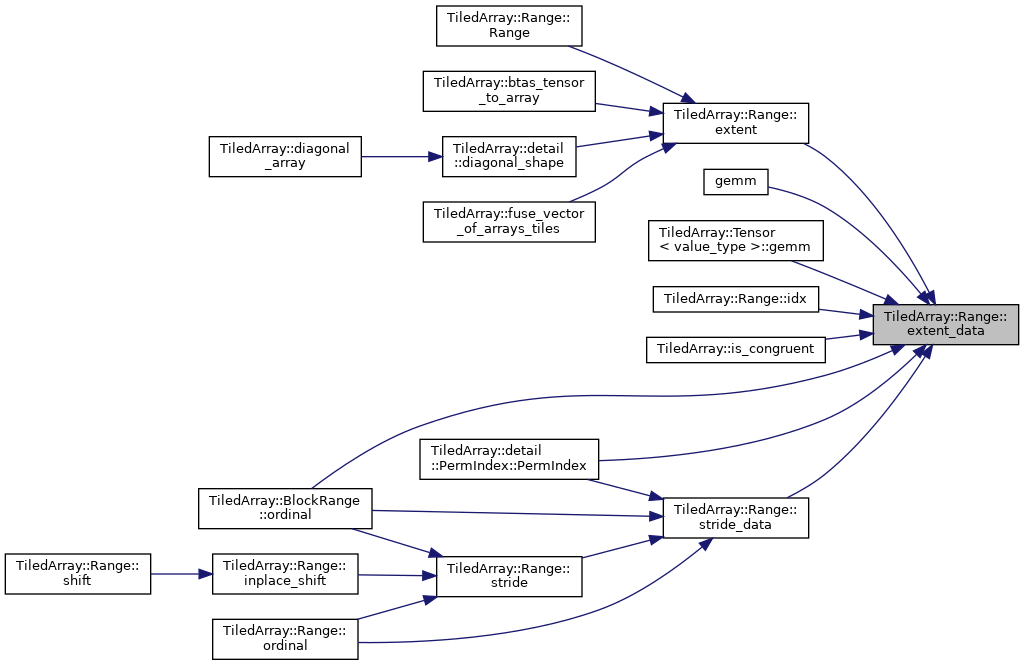
◆ extent_data_nc()
|
inlineprotected |
◆ idx() [1/2]
|
inline |
calculate the index of i
This function is just a pass-through so the user can call idx()
on a template parameter that can be an index or an ordinal_type.
- Template Parameters
-
Index An integral range type
- Parameters
-
i The index
- Returns
i
(unchanged)
Definition at line 1114 of file range.h.

◆ idx() [2/2]
|
inline |
calculate the coordinate index of the ordinal index, index
.
Convert an ordinal index to a coordinate index.
- Parameters
-
index Ordinal index
- Returns
- The index of the ordinal index
- Exceptions
-
TiledArray::Exception When index
is not included in this range
Definition at line 1076 of file range.h.
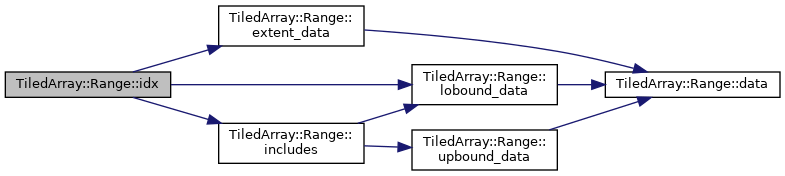
◆ includes() [1/4]
|
inline |
◆ includes() [2/4]
|
inline |
Check the coordinate to make sure it is within the range.
- Template Parameters
-
Index An integral range type
- Parameters
-
index The coordinate index to check for inclusion in the range
- Returns
true
wheni >= lobound
andi < upbound
, otherwisefalse
- Exceptions
-
TiledArray::Exception When the rank of this range is not equal to the size of the index.
Definition at line 826 of file range.h.
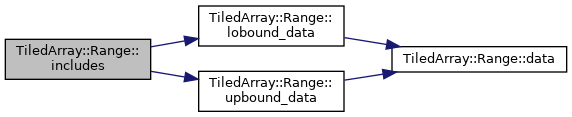
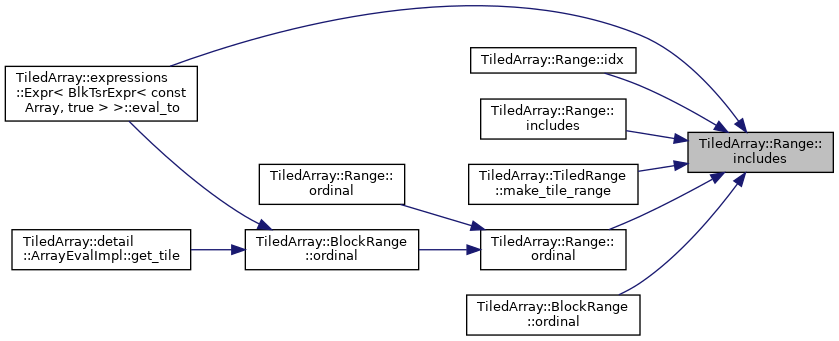
◆ includes() [3/4]
|
inline |
Check the coordinate to make sure it is within the range.
- Template Parameters
-
Index An integral type
- Parameters
-
index The element index whose presence in the range is queried
- Returns
true
wheni >= lobound
andi < upbound
, otherwisefalse
- Exceptions
-
TiledArray::Exception When the rank of this range is not equal to the size of the index.
◆ includes() [4/4]
|
inline |
◆ init_datavec()
|
inlineprotected |
◆ inplace_shift() [1/2]
|
inline |
Shift the lower and upper bound of this range.
- Template Parameters
-
Index An integral range type
- Parameters
-
bound_shift The shift to be applied to the range
- Returns
- A reference to this range
Definition at line 933 of file range.h.


◆ inplace_shift() [2/2]
|
inline |
◆ lobound() [1/2]
|
inline |
◆ lobound() [2/2]
|
inline |
◆ lobound_data()
|
inline |
Range lower bound data accessor.
- Returns
- A pointer to the lower bound data (see Range::lobound() )
- Note
- Not necessarily nullptr for rank-0 or null Range
- Exceptions
-
nothing
Definition at line 685 of file range.h.

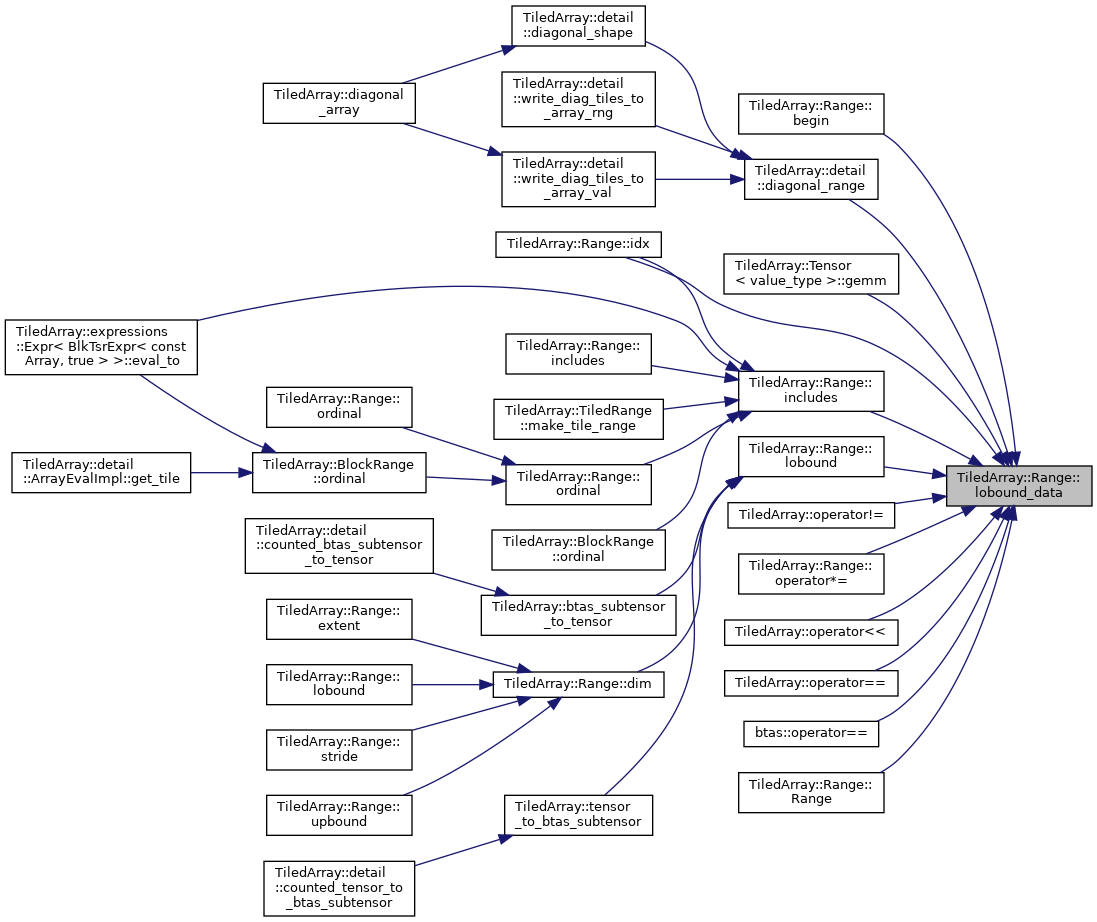
◆ lobound_data_nc()
|
inlineprotected |
◆ offset()
|
inline |
◆ operator bool()
|
inlineexplicit |
◆ operator*=()
|
inline |
Permute this range.
- Parameters
-
perm The permutation to be applied to this range
- Returns
- A reference to this range
- Exceptions
-
TiledArray::Exception When the rank of this range is not equal to the rank of the permutation.
Definition at line 1218 of file range.h.
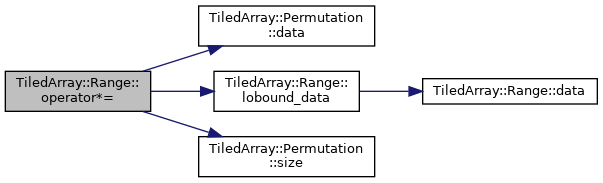
◆ operator=() [1/2]
Copy assignment operator.
- Parameters
-
other The range to be copied
- Returns
- A reference to this object
◆ operator=() [2/2]
◆ ordinal() [1/4]
|
inline |
calculate the ordinal index of index
Convert a coordinate index to an ordinal index.
- Template Parameters
-
Index A pack of integral types
- Parameters
-
index The index to be converted to an ordinal index
- Returns
- The ordinal index of
index
- Exceptions
-
When index
is not included in this range.
Definition at line 1064 of file range.h.

◆ ordinal() [2/4]
|
inline |
calculate the ordinal index of index
Convert a coordinate index to an ordinal index.
- Template Parameters
-
Index An integral range type
- Parameters
-
index The index to be converted to an ordinal index
- Returns
- The ordinal index of
index
- Exceptions
-
When index
is not included in this range.
Definition at line 1022 of file range.h.

◆ ordinal() [3/4]
|
inline |
calculate the ordinal index of i
This function is just a pass-through so the user can call ordinal()
on a template parameter that can be a coordinate index or an integral.
- Parameters
-
index Ordinal index
- Returns
index
(unchanged)
- Exceptions
-
When index
is not included in this range
Definition at line 1008 of file range.h.

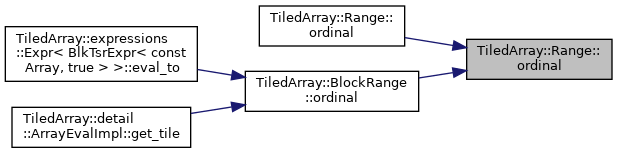
◆ ordinal() [4/4]
|
inline |
calculate the ordinal index of index
Convert a coordinate index to an ordinal index.
- Template Parameters
-
Index An integral type
- Parameters
-
index The index to be converted to an ordinal index
- Returns
- The ordinal index of
index
- Exceptions
-
When index
is not included in this range.
◆ rank()
|
inline |
◆ resize()
|
inline |
Resize range to a new upper and lower bound.
- Template Parameters
-
Index1 An integral sized range type Index2 An integral sized range type
- Parameters
-
lower_bound The lower bounds of the N-dimensional range upper_bound The upper bound of the N-dimensional range
- Returns
- A reference to this range
- Exceptions
-
TiledArray::Exception When the size of lower_bound
is not equal to that ofupper_bound
.TiledArray::Exception When lower_bound[i] >= upper_bound[i]
Definition at line 908 of file range.h.

◆ serialize()
|
inline |
◆ shift() [1/2]
|
inline |
◆ shift() [2/2]
|
inline |
◆ stride() [1/2]
|
inline |
◆ stride() [2/2]
|
inline |
◆ stride_data()
|
inline |
Range stride data accessor.
- Returns
- A pointer to the stride data (see Range::stride() )
- Note
- Not necessarily nullptr for rank-0 or null Range
- Exceptions
-
nothing
Definition at line 759 of file range.h.

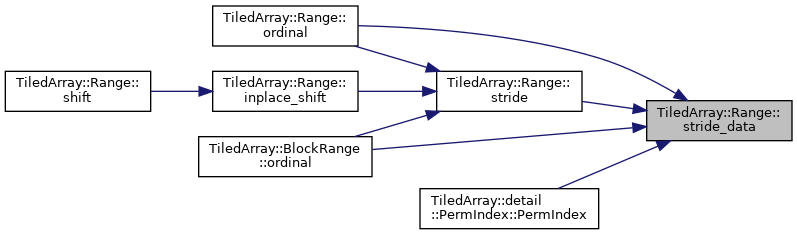
◆ stride_data_nc()
|
inlineprotected |
◆ swap()
|
inline |
◆ upbound() [1/2]
|
inline |
◆ upbound() [2/2]
|
inline |
◆ upbound_data()
|
inline |
Range upper bound data accessor.
- Returns
- A pointer to the upper bound data (see Range::upbound() )
- Note
- Not necessarily nullptr for rank-0 or null Range
- Exceptions
-
nothing
Definition at line 710 of file range.h.

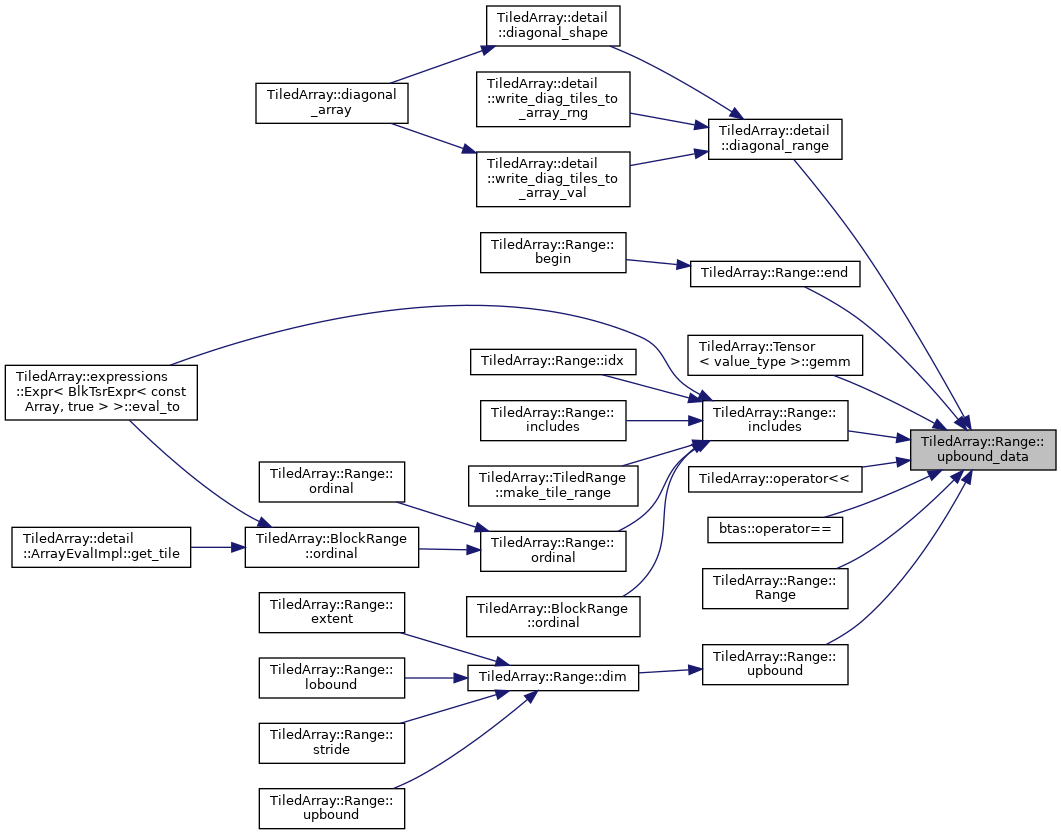
◆ upbound_data_nc()
|
inlineprotected |
◆ volume()
|
inline |
Friends And Related Function Documentation
◆ detail::RangeIterator< index1_type, Range_ >
|
friend |
Member Data Documentation
◆ datavec_
|
protected |
◆ offset_
|
protected |
◆ rank_
|
protected |
◆ volume_
|
protected |
The documentation for this class was generated from the following file:
- TiledArray/range.h