Documentation
template<typename T, typename R, typename OpResult>
class TiledArray::detail::TensorInterface< T, R, OpResult >
Tensor interface for external data.
This class allows users to construct a tensor object using data from an external source. TensorInterface
objects can be used with each other and Tensor
objects in any of the arithmetic operations.
- Warning
- No ownership of the data pointer used to construct
TensorInterface
objects. Therefore, it is the user's responsibility to manage the lifetime of the data. -
This is not appropriate for use as a tile object as it does not own the data it references. Use
Tensor
for that purpose.
- Template Parameters
-
T The tensor value type R The range type OpResult tensor type used as the return type from operations that produce a tensor
Definition at line 82 of file tensor_interface.h.
Public Types | |
typedef TensorInterface< T, R, OpResult > | TensorInterface_ |
This class type. More... | |
typedef R | range_type |
Tensor range type. More... | |
typedef range_type::index1_type | index1_type |
1-index type More... | |
typedef range_type::ordinal_type | ordinal_type |
Ordinal type. More... | |
using | size_type = ordinal_type |
typedef std::remove_const< T >::type | value_type |
Array element type. More... | |
typedef std::add_lvalue_reference< T >::type | reference |
Element reference type. More... | |
typedef std::add_lvalue_reference< typename std::add_const< T >::type >::type | const_reference |
Element reference type. More... | |
typedef std::add_pointer< T >::type | pointer |
Element pointer type. More... | |
typedef std::add_pointer< typename std::add_const< T >::type >::type | const_pointer |
Element pointer type. More... | |
typedef std::ptrdiff_t | difference_type |
Difference type. More... | |
typedef detail::numeric_type< value_type >::type | numeric_type |
the numeric type that supports T More... | |
typedef detail::scalar_type< value_type >::type | scalar_type |
the scalar type that supports T More... | |
typedef OpResult | result_tensor |
Tensor type used as the return type from operations that produce a tensor. More... | |
Public Member Functions | |
TensorInterface ()=delete | |
Compiler generated functions. More... | |
~TensorInterface ()=default | |
TensorInterface (const TensorInterface_ &)=default | |
TensorInterface (TensorInterface_ &&)=default | |
TensorInterface_ & | operator= (const TensorInterface_ &)=delete |
TensorInterface_ & | operator= (TensorInterface_ &&)=delete |
template<typename U , typename UOpResult , typename std::enable_if< std::is_convertible< typename TensorInterface< U, R, UOpResult >::pointer, pointer >::value >::type * = nullptr> | |
TensorInterface (const TensorInterface< U, R, UOpResult > &other) | |
Type conversion copy constructor. More... | |
template<typename U , typename UOpResult , typename std::enable_if< std::is_convertible< typename TensorInterface< U, R, UOpResult >::pointer, pointer >::value >::type * = nullptr> | |
TensorInterface (TensorInterface< U, R, UOpResult > &&other) | |
Type conversion move constructor. More... | |
TensorInterface (const range_type &range, pointer data) | |
Construct a new view of tensor . More... | |
TensorInterface (range_type &&range, pointer data) | |
Construct a new view of tensor . More... | |
template<typename T1 , typename std::enable_if< detail::is_tensor< T1 >::value >::type * = nullptr> | |
TensorInterface_ & | operator= (const T1 &other) |
const range_type & | range () const |
Tensor range object accessor. More... | |
ordinal_type | size () const |
Tensor dimension size accessor. More... | |
pointer | data () const |
Data pointer accessor. More... | |
const_reference | operator[] (const ordinal_type index) const |
Element subscript accessor. More... | |
reference | operator[] (const ordinal_type index) |
Element subscript accessor. More... | |
template<typename... Index> | |
reference | operator() (const Index &... idx) |
Element accessor. More... | |
template<typename... Index> | |
const_reference | operator() (const Index &... idx) const |
Element accessor. More... | |
constexpr bool | empty () const |
Check for empty view. More... | |
void | swap (TensorInterface_ &other) |
Swap tensor views. More... | |
template<typename Index , typename = std::enable_if_t<detail::is_integral_range_v<Index>>> | |
TensorInterface_ & | shift_to (const Index &bound_shift) |
Shift the lower and upper bound of this tensor view. More... | |
template<typename Index , typename = std::enable_if_t<std::is_integral_v<Index>>> | |
TensorInterface_ & | shift_to (const std::initializer_list< Index > &bound_shift) |
Shift the lower and upper bound of this tensor view. More... | |
template<typename Index , typename = std::enable_if_t<detail::is_integral_range_v<Index>>> | |
result_tensor | shift (const Index &bound_shift) const |
Make a copy of this view shited by bound_shift . More... | |
template<typename Index , typename = std::enable_if_t<std::is_integral_v<Index>>> | |
result_tensor | shift (const std::initializer_list< Index > &bound_shift) const |
Make a copy of this view shited by bound_shift . More... | |
template<typename Right , typename Op , typename std::enable_if< is_tensor< Right >::value >::type * = nullptr> | |
result_tensor | binary (const Right &right, Op &&op) const |
Use a binary, element wise operation to construct a new tensor. More... | |
template<typename Right , typename Op , typename Perm , typename std::enable_if< is_tensor< Right >::value &&detail::is_permutation_v< Perm >>::type * = nullptr> | |
result_tensor | binary (const Right &right, Op &&op, const Perm &perm) const |
Use a binary, element wise operation to construct a new, permuted tensor. More... | |
template<typename Right , typename Op , typename std::enable_if< is_tensor< Right >::value >::type * = nullptr> | |
TensorInterface_ & | inplace_binary (const Right &right, Op &&op) |
Use a binary, element wise operation to modify this tensor. More... | |
template<typename Op > | |
result_tensor | unary (Op &&op) const |
Use a unary, element wise operation to construct a new tensor. More... | |
template<typename Op , typename Perm , typename = std::enable_if_t<detail::is_permutation_v<Perm>>> | |
result_tensor | unary (Op &&op, const Perm &perm) const |
Use a unary, element wise operation to construct a new, permuted tensor. More... | |
template<typename Op > | |
TensorInterface_ & | inplace_unary (Op &&op) |
Use a unary, element wise operation to modify this tensor. More... | |
template<typename Perm , typename = std::enable_if_t<detail::is_permutation_v<Perm>>> | |
result_tensor | permute (const Perm &perm) const |
template<typename Scalar , typename std::enable_if< detail::is_numeric_v< Scalar >>::type * = nullptr> | |
result_tensor | scale (const Scalar factor) const |
Construct a scaled copy of this tensor. More... | |
template<typename Scalar , typename Perm , typename std::enable_if< detail::is_numeric_v< Scalar > &&detail::is_permutation_v< Perm >>::type * = nullptr> | |
result_tensor | scale (const Scalar factor, const Perm &perm) const |
Construct a scaled and permuted copy of this tensor. More... | |
template<typename Scalar , typename std::enable_if< detail::is_numeric_v< Scalar >>::type * = nullptr> | |
TensorInterface_ & | scale_to (const Scalar factor) |
Scale this tensor. More... | |
template<typename Right , typename std::enable_if< is_tensor< Right >::value >::type * = nullptr> | |
result_tensor | add (const Right &right) const |
Add this and other to construct a new tensors. More... | |
template<typename Right , typename Perm , typename std::enable_if< is_tensor< Right >::value &&detail::is_permutation_v< Perm >>::type * = nullptr> | |
result_tensor | add (const Right &right, const Perm &perm) const |
Add this and other to construct a new, permuted tensor. More... | |
template<typename Right , typename Scalar , typename std::enable_if< is_tensor< Right >::value &&detail::is_numeric_v< Scalar >>::type * = nullptr> | |
result_tensor | add (const Right &right, const Scalar factor) const |
Scale and add this and other to construct a new tensor. More... | |
template<typename Right , typename Scalar , typename Perm , typename std::enable_if< is_tensor< Right >::value &&detail::is_numeric_v< Scalar > &&detail::is_permutation_v< Perm >>::type * = nullptr> | |
result_tensor | add (const Right &right, const Scalar factor, const Perm &perm) const |
Scale and add this and other to construct a new, permuted tensor. More... | |
result_tensor | add (const numeric_type value) const |
Add a constant to a copy of this tensor. More... | |
template<typename Perm , typename = std::enable_if_t<detail::is_permutation_v<Perm>>> | |
result_tensor | add (const numeric_type value, const Perm &perm) const |
Add a constant to a permuted copy of this tensor. More... | |
template<typename Right , typename std::enable_if< is_tensor< Right >::value >::type * = nullptr> | |
TensorInterface_ & | add_to (const Right &right) |
Add other to this tensor. More... | |
template<typename Right , typename Scalar , typename std::enable_if< is_tensor< Right >::value &&detail::is_numeric_v< Scalar >>::type * = nullptr> | |
TensorInterface_ & | add_to (const Right &right, const Scalar factor) |
Add other to this tensor, and scale the result. More... | |
TensorInterface_ & | add_to (const numeric_type value) |
Add a constant to this tensor. More... | |
template<typename Right , typename std::enable_if< is_tensor< Right >::value >::type * = nullptr> | |
result_tensor | subt (const Right &right) const |
Subtract this and right to construct a new tensor. More... | |
template<typename Right , typename Perm , typename std::enable_if< is_tensor< Right >::value &&detail::is_permutation_v< Perm >>::type * = nullptr> | |
result_tensor | subt (const Right &right, const Perm &perm) const |
Subtract this and right to construct a new, permuted tensor. More... | |
template<typename Right , typename Scalar , typename std::enable_if< is_tensor< Right >::value &&detail::is_numeric_v< Scalar >>::type * = nullptr> | |
result_tensor | subt (const Right &right, const Scalar factor) const |
Scale and subtract this and right to construct a new tensor. More... | |
template<typename Right , typename Scalar , typename Perm , typename std::enable_if< is_tensor< Right >::value &&detail::is_numeric_v< Scalar > &&detail::is_permutation_v< Perm >>::type * = nullptr> | |
result_tensor | subt (const Right &right, const Scalar factor, const Perm &perm) const |
Scale and subtract this and right to construct a new, permuted tensor. More... | |
result_tensor | subt (const numeric_type value) const |
Subtract a constant from a copy of this tensor. More... | |
template<typename Perm , typename = std::enable_if_t<detail::is_permutation_v<Perm>>> | |
result_tensor | subt (const numeric_type value, const Perm &perm) const |
Subtract a constant from a permuted copy of this tensor. More... | |
template<typename Right , typename std::enable_if< is_tensor< Right >::value >::type * = nullptr> | |
TensorInterface_ & | subt_to (const Right &right) |
Subtract right from this tensor. More... | |
template<typename Right , typename Scalar , typename std::enable_if< is_tensor< Right >::value &&detail::is_numeric_v< Scalar >>::type * = nullptr> | |
TensorInterface_ & | subt_to (const Right &right, const Scalar factor) |
Subtract right from and scale this tensor. More... | |
TensorInterface_ & | subt_to (const numeric_type value) |
Subtract a constant from this tensor. More... | |
template<typename Right , typename std::enable_if< is_tensor< Right >::value >::type * = nullptr> | |
result_tensor | mult (const Right &right) const |
Multiply this by right to create a new tensor. More... | |
template<typename Right , typename Perm , typename std::enable_if< is_tensor< Right >::value &&detail::is_permutation_v< Perm >>::type * = nullptr> | |
result_tensor | mult (const Right &right, const Perm &perm) const |
Multiply this by right to create a new, permuted tensor. More... | |
template<typename Right , typename Scalar , typename std::enable_if< is_tensor< Right >::value &&detail::is_numeric_v< Scalar >>::type * = nullptr> | |
result_tensor | mult (const Right &right, const Scalar factor) const |
Scale and multiply this by right to create a new tensor. More... | |
template<typename Right , typename Scalar , typename Perm , typename std::enable_if< is_tensor< Right >::value &&detail::is_numeric_v< Scalar > &&detail::is_permutation_v< Perm >>::type * = nullptr> | |
result_tensor | mult (const Right &right, const Scalar factor, const Perm &perm) const |
Scale and multiply this by right to create a new, permuted tensor. More... | |
template<typename Right , typename std::enable_if< is_tensor< Right >::value >::type * = nullptr> | |
TensorInterface_ & | mult_to (const Right &right) |
Multiply this tensor by right . More... | |
template<typename Right , typename Scalar , typename std::enable_if< is_tensor< Right >::value &&detail::is_numeric_v< Scalar >>::type * = nullptr> | |
TensorInterface_ & | mult_to (const Right &right, const Scalar factor) |
Scale and multiply this tensor by right . More... | |
result_tensor | neg () const |
Create a negated copy of this tensor. More... | |
template<typename Perm , typename = std::enable_if_t<detail::is_permutation_v<Perm>>> | |
result_tensor | neg (const Perm &perm) const |
Create a negated and permuted copy of this tensor. More... | |
TensorInterface_ & | neg_to () |
Negate elements of this tensor. More... | |
result_tensor | conj () const |
Create a complex conjugated copy of this tensor. More... | |
template<typename Scalar , typename std::enable_if< detail::is_numeric_v< Scalar >>::type * = nullptr> | |
result_tensor | conj (const Scalar factor) const |
Create a complex conjugated and scaled copy of this tensor. More... | |
template<typename Perm , typename = std::enable_if_t<detail::is_permutation_v<Perm>>> | |
result_tensor | conj (const Perm &perm) const |
Create a complex conjugated and permuted copy of this tensor. More... | |
template<typename Scalar , typename Perm , typename std::enable_if< detail::is_numeric_v< Scalar > &&detail::is_permutation_v< Perm >>::type * = nullptr> | |
result_tensor | conj (const Scalar factor, const Perm &perm) const |
Create a complex conjugated, scaled, and permuted copy of this tensor. More... | |
TensorInterface_ & | conj_to () |
Complex conjugate this tensor. More... | |
template<typename Scalar , typename std::enable_if< detail::is_numeric_v< Scalar >>::type * = nullptr> | |
TensorInterface_ & | conj_to (const Scalar factor) |
Complex conjugate and scale this tensor. More... | |
template<typename ReduceOp , typename JoinOp > | |
numeric_type | reduce (ReduceOp &&reduce_op, JoinOp &&join_op, const numeric_type identity) const |
Unary reduction operation. More... | |
template<typename Right , typename ReduceOp , typename JoinOp , typename std::enable_if< is_tensor< Right >::value >::type * = nullptr> | |
numeric_type | reduce (const Right &other, ReduceOp &&reduce_op, JoinOp &&join_op, const numeric_type identity) const |
Binary reduction operation. More... | |
numeric_type | sum () const |
Sum of elements. More... | |
numeric_type | product () const |
Product of elements. More... | |
scalar_type | squared_norm () const |
Square of vector 2-norm. More... | |
template<typename ResultType = numeric_type> | |
ResultType | norm () const |
Vector 2-norm. More... | |
numeric_type | min () const |
Minimum element. More... | |
numeric_type | max () const |
Maximum element. More... | |
numeric_type | abs_min () const |
Absolute minimum element. More... | |
numeric_type | abs_max () const |
Absolute maximum element. More... | |
template<typename Right , typename std::enable_if< is_tensor< Right >::value >::type * = nullptr> | |
numeric_type | dot (const Right &other) const |
Vector dot product. More... | |
template<typename Right , typename std::enable_if< is_tensor< Right >::value >::type * = nullptr> | |
numeric_type | inner_product (const Right &other) const |
Friends | |
template<typename , typename , typename > | |
class | TensorInterface |
template<typename U , typename Range_ , typename OpResult_ , typename Index1 , typename Index2 > | |
void | TiledArray::remap (detail::TensorInterface< U, Range_, OpResult_ > &, U *const, const Index1 &, const Index2 &) |
template<typename U , typename Range_ , typename OpResult_ , typename Index1 , typename Index2 > | |
void | TiledArray::remap (detail::TensorInterface< const U, Range_, OpResult_ > &, U *const, const Index1 &, const Index2 &) |
template<typename U , typename Range_ , typename OpResult_ , typename Index1 , typename Index2 > | |
void | TiledArray::remap (detail::TensorInterface< U, Range_, OpResult_ > &, U *const, const std::initializer_list< Index1 > &, const std::initializer_list< Index2 > &) |
template<typename U , typename Range_ , typename OpResult_ , typename Index1 , typename Index2 > | |
void | TiledArray::remap (detail::TensorInterface< const U, Range_, OpResult_ > &, U *const, const std::initializer_list< Index1 > &, const std::initializer_list< Index2 > &) |
Member Typedef Documentation
◆ const_pointer
typedef std::add_pointer<typename std::add_const<T>::type>::type TiledArray::detail::TensorInterface< T, R, OpResult >::const_pointer |
Element pointer type.
Definition at line 99 of file tensor_interface.h.
◆ const_reference
typedef std::add_lvalue_reference<typename std::add_const<T>::type>::type TiledArray::detail::TensorInterface< T, R, OpResult >::const_reference |
Element reference type.
Definition at line 96 of file tensor_interface.h.
◆ difference_type
typedef std::ptrdiff_t TiledArray::detail::TensorInterface< T, R, OpResult >::difference_type |
Difference type.
Definition at line 100 of file tensor_interface.h.
◆ index1_type
typedef range_type::index1_type TiledArray::detail::TensorInterface< T, R, OpResult >::index1_type |
1-index type
Definition at line 87 of file tensor_interface.h.
◆ numeric_type
typedef detail::numeric_type<value_type>::type TiledArray::detail::TensorInterface< T, R, OpResult >::numeric_type |
the numeric type that supports T
Definition at line 102 of file tensor_interface.h.
◆ ordinal_type
typedef range_type::ordinal_type TiledArray::detail::TensorInterface< T, R, OpResult >::ordinal_type |
Ordinal type.
Definition at line 88 of file tensor_interface.h.
◆ pointer
typedef std::add_pointer<T>::type TiledArray::detail::TensorInterface< T, R, OpResult >::pointer |
Element pointer type.
Definition at line 97 of file tensor_interface.h.
◆ range_type
typedef R TiledArray::detail::TensorInterface< T, R, OpResult >::range_type |
Tensor range type.
Definition at line 86 of file tensor_interface.h.
◆ reference
typedef std::add_lvalue_reference<T>::type TiledArray::detail::TensorInterface< T, R, OpResult >::reference |
Element reference type.
Definition at line 93 of file tensor_interface.h.
◆ result_tensor
typedef OpResult TiledArray::detail::TensorInterface< T, R, OpResult >::result_tensor |
Tensor type used as the return type from operations that produce a tensor.
Definition at line 106 of file tensor_interface.h.
◆ scalar_type
typedef detail::scalar_type<value_type>::type TiledArray::detail::TensorInterface< T, R, OpResult >::scalar_type |
the scalar type that supports T
Definition at line 104 of file tensor_interface.h.
◆ size_type
using TiledArray::detail::TensorInterface< T, R, OpResult >::size_type = ordinal_type |
Definition at line 89 of file tensor_interface.h.
◆ TensorInterface_
typedef TensorInterface<T, R, OpResult> TiledArray::detail::TensorInterface< T, R, OpResult >::TensorInterface_ |
This class type.
Definition at line 85 of file tensor_interface.h.
◆ value_type
typedef std::remove_const<T>::type TiledArray::detail::TensorInterface< T, R, OpResult >::value_type |
Array element type.
Definition at line 91 of file tensor_interface.h.
Constructor & Destructor Documentation
◆ TensorInterface() [1/7]
|
delete |
Compiler generated functions.
◆ ~TensorInterface()
|
default |
◆ TensorInterface() [2/7]
|
default |
◆ TensorInterface() [3/7]
|
default |
◆ TensorInterface() [4/7]
|
inline |
Type conversion copy constructor.
- Template Parameters
-
U The value type of the other view
- Parameters
-
other The other tensor view to be copied
Definition at line 157 of file tensor_interface.h.
◆ TensorInterface() [5/7]
|
inline |
Type conversion move constructor.
- Template Parameters
-
U The value type of the other tensor view
- Parameters
-
other The other tensor view to be moved
Definition at line 168 of file tensor_interface.h.
◆ TensorInterface() [6/7]
|
inline |
Construct a new view of tensor
.
- Parameters
-
range The range of this tensor data The data pointer for this tensor
Definition at line 177 of file tensor_interface.h.

◆ TensorInterface() [7/7]
|
inline |
Construct a new view of tensor
.
- Parameters
-
range The range of this tensor data The data pointer for this tensor
Definition at line 186 of file tensor_interface.h.

Member Function Documentation
◆ abs_max()
|
inline |
Absolute maximum element.
- Returns
- The maximum elements of this tensor
Definition at line 1093 of file tensor_interface.h.
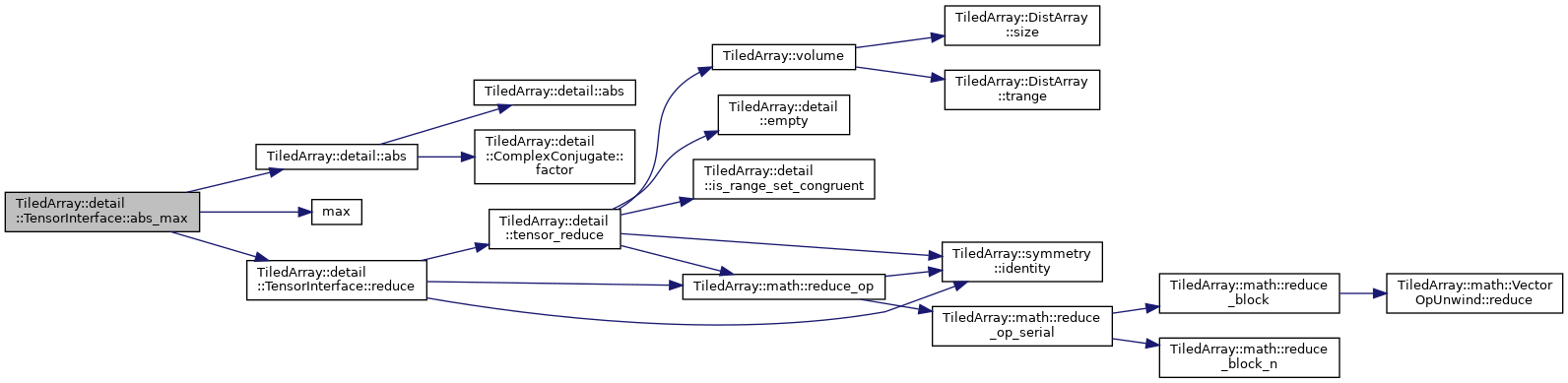
◆ abs_min()
|
inline |
Absolute minimum element.
- Returns
- The minimum elements of this tensor
Definition at line 1080 of file tensor_interface.h.
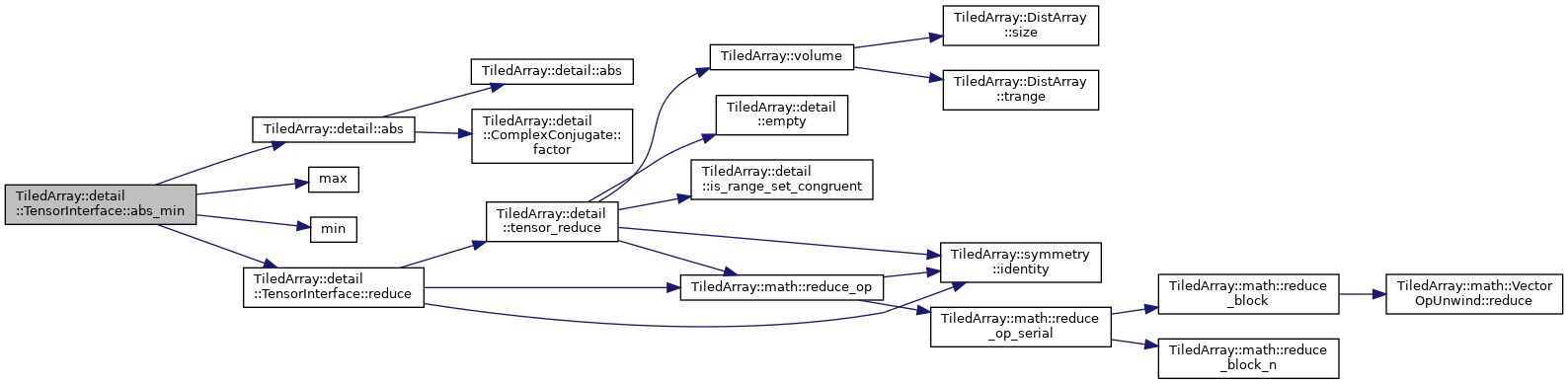
◆ add() [1/6]
|
inline |
Add a constant to a copy of this tensor.
- Parameters
-
value The constant to be added to this tensor
- Returns
- A new tensor where the elements are the sum of the elements of
this
andvalue
Definition at line 595 of file tensor_interface.h.
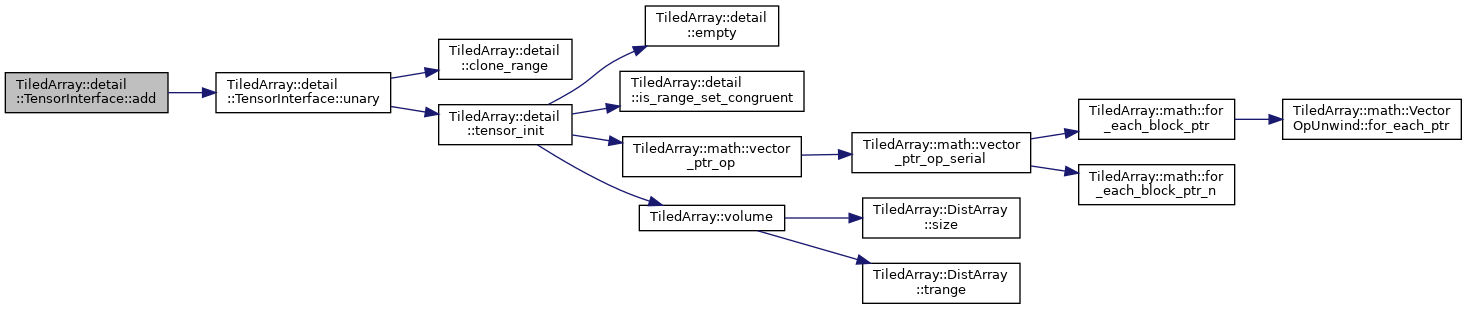
◆ add() [2/6]
|
inline |
Add a constant to a permuted copy of this tensor.
- Parameters
-
value The constant to be added to this tensor perm The permutation to be applied to this tensor
- Returns
- A new tensor where the elements are the sum of the elements of
this
andvalue
Definition at line 608 of file tensor_interface.h.
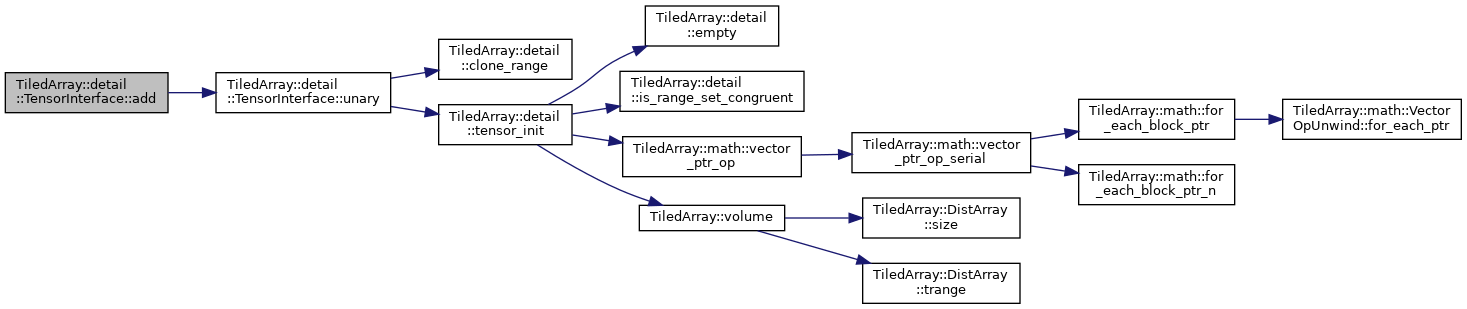
◆ add() [3/6]
|
inline |
Add this and other
to construct a new tensors.
- Template Parameters
-
Right The right-hand tensor type
- Parameters
-
right The tensor that will be added to this tensor
- Returns
- A new tensor where the elements are the sum of the elements of
this
andother
Definition at line 522 of file tensor_interface.h.
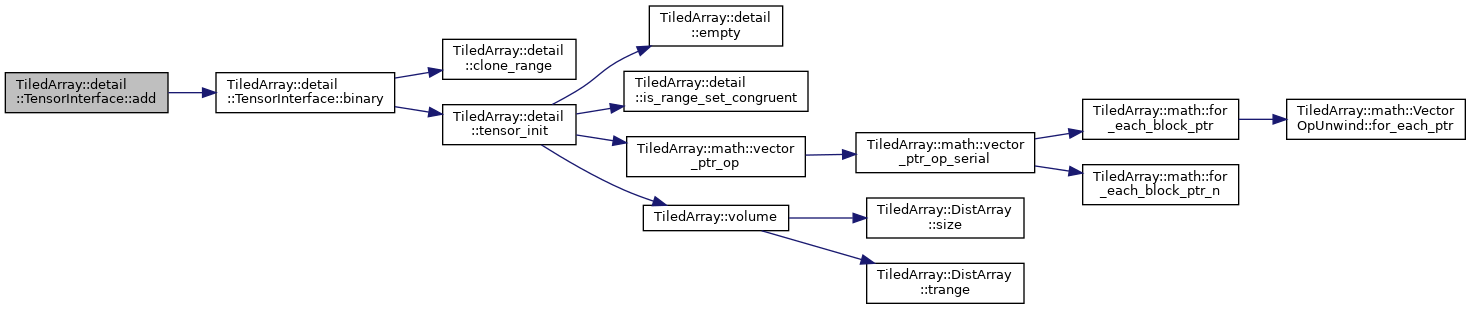

◆ add() [4/6]
|
inline |
Add this and other
to construct a new, permuted tensor.
- Template Parameters
-
Right The right-hand tensor type
- Parameters
-
right The tensor that will be added to this tensor perm The permutation to be applied to this tensor
- Returns
- A new tensor where the elements are the sum of the elements of
this
andother
Definition at line 541 of file tensor_interface.h.
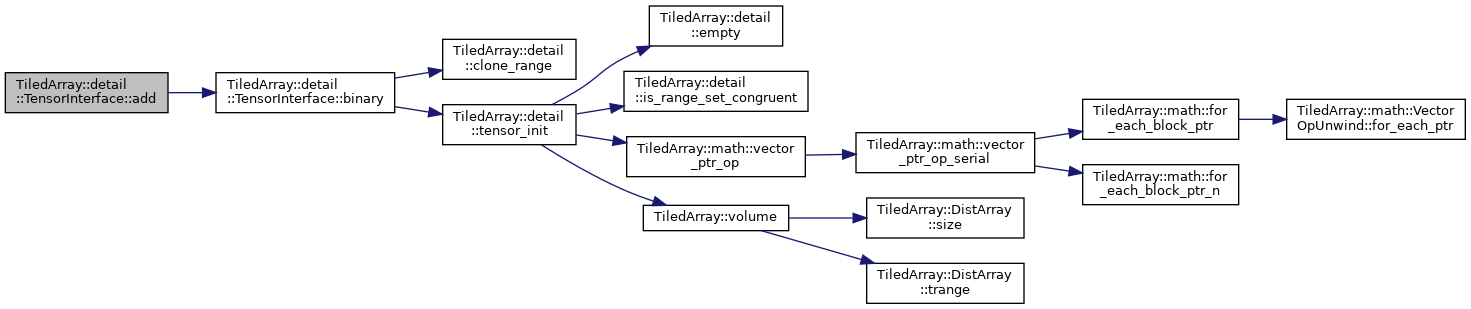
◆ add() [5/6]
|
inline |
Scale and add this and other
to construct a new tensor.
- Template Parameters
-
Right The right-hand tensor type Scalar A scalar type
- Parameters
-
right The tensor that will be added to this tensor factor The scaling factor
- Returns
- A new tensor where the elements are the sum of the elements of
this
andother
, scaled byfactor
Definition at line 562 of file tensor_interface.h.
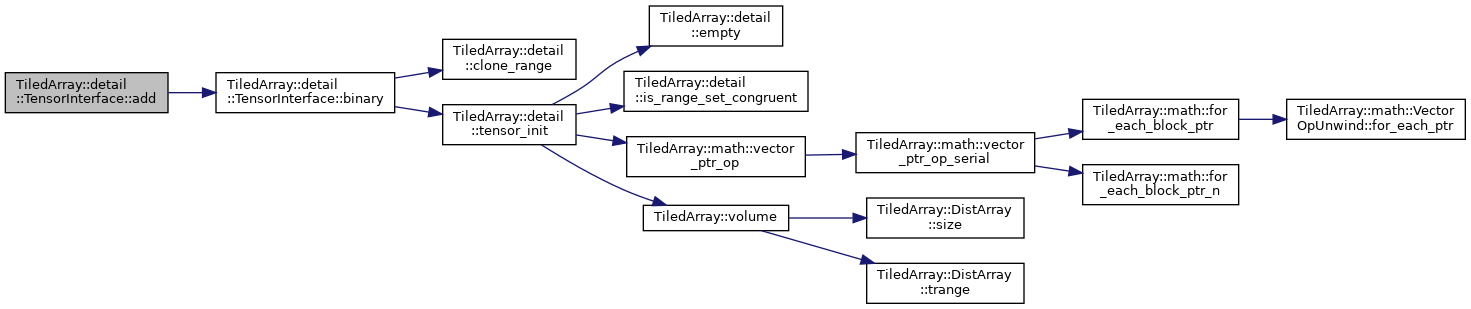
◆ add() [6/6]
|
inline |
Scale and add this and other
to construct a new, permuted tensor.
- Template Parameters
-
Right The right-hand tensor type Scalar A scalar type
- Parameters
-
right The tensor that will be added to this tensor factor The scaling factor perm The permutation to be applied to this tensor
- Returns
- A new tensor where the elements are the sum of the elements of
this
andother
, scaled byfactor
Definition at line 581 of file tensor_interface.h.
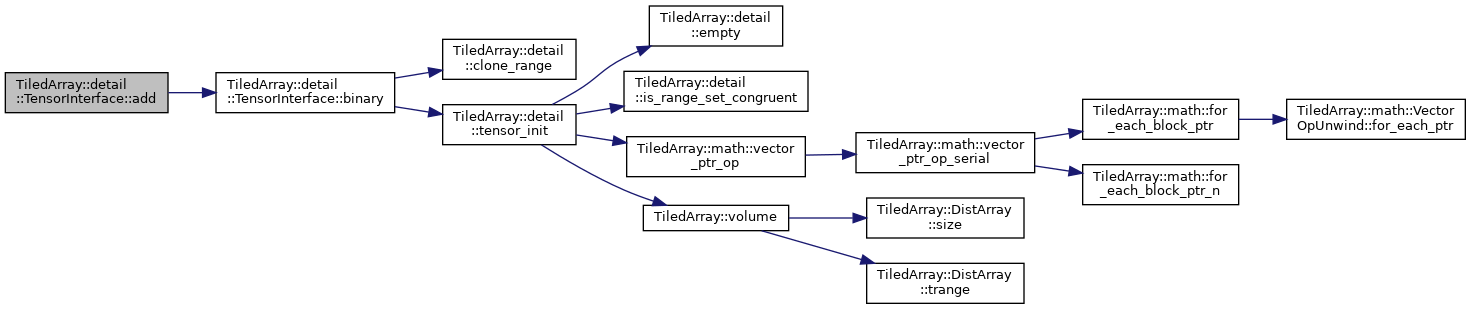
◆ add_to() [1/3]
|
inline |
Add a constant to this tensor.
- Parameters
-
value The constant to be added
- Returns
- A reference to this tensor
Definition at line 647 of file tensor_interface.h.

◆ add_to() [2/3]
|
inline |
Add other
to this tensor.
- Template Parameters
-
Right The right-hand tensor type
- Parameters
-
right The tensor that will be added to this tensor
- Returns
- A reference to this tensor
Definition at line 621 of file tensor_interface.h.


◆ add_to() [3/3]
|
inline |
Add other
to this tensor, and scale the result.
- Template Parameters
-
Right The right-hand tensor type Scalar A scalar type
- Parameters
-
right The tensor that will be added to this tensor factor The scaling factor
- Returns
- A reference to this tensor
Definition at line 637 of file tensor_interface.h.

◆ binary() [1/2]
|
inline |
Use a binary, element wise operation to construct a new tensor.
- Template Parameters
-
Right The right-hand tensor type Op The binary operation type
- Parameters
-
right The right-hand argument in the binary operation op The binary, element-wise operation
- Returns
- A tensor where element
i
of the new tensor is equal toop(*this[i],other[i])
Definition at line 327 of file tensor_interface.h.
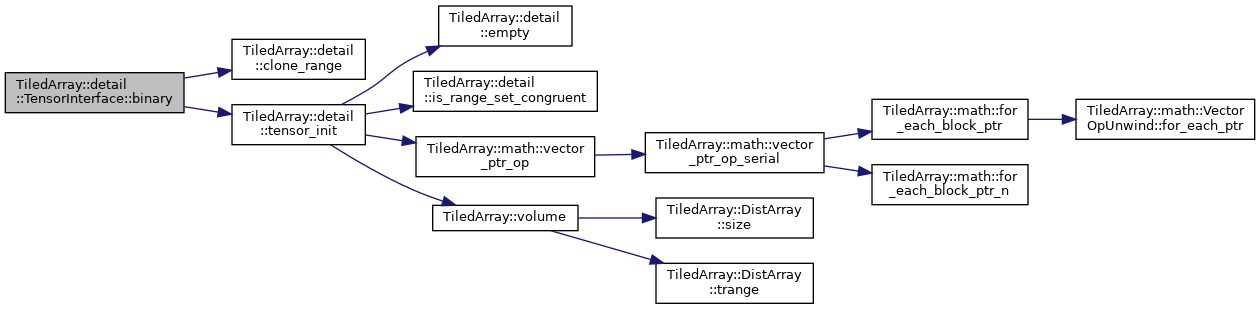
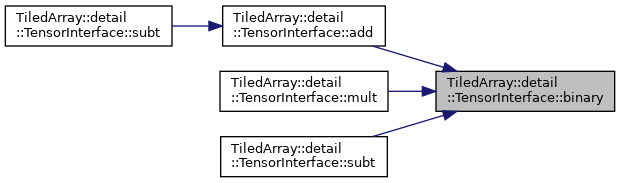
◆ binary() [2/2]
|
inline |
Use a binary, element wise operation to construct a new, permuted tensor.
- Template Parameters
-
Right The right-hand tensor type Op The binary operation type
- Parameters
-
right The right-hand argument in the binary operation op The binary, element-wise operation perm The permutation to be applied to this tensor
- Returns
- A tensor where element
i
of the new tensor is equal toop(*this[i],other[i])
Definition at line 347 of file tensor_interface.h.
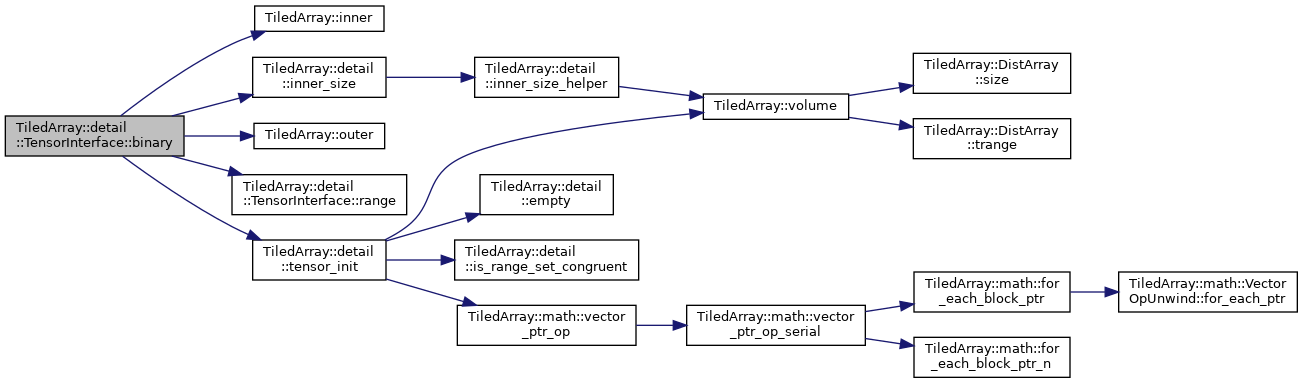
◆ conj() [1/4]
|
inline |
Create a complex conjugated copy of this tensor.
- Returns
- A copy of this tensor that contains the complex conjugate the values
Definition at line 919 of file tensor_interface.h.

◆ conj() [2/4]
|
inline |
Create a complex conjugated and permuted copy of this tensor.
- Parameters
-
perm The permutation to be applied to this tensor
- Returns
- A permuted copy of this tensor that contains the complex conjugate values
Definition at line 940 of file tensor_interface.h.

◆ conj() [3/4]
|
inline |
Create a complex conjugated and scaled copy of this tensor.
- Template Parameters
-
Scalar A scalar type
- Parameters
-
factor The scaling factor
- Returns
- A copy of this tensor that contains the scaled complex conjugate the values
Definition at line 929 of file tensor_interface.h.

◆ conj() [4/4]
|
inline |
Create a complex conjugated, scaled, and permuted copy of this tensor.
- Template Parameters
-
Scalar A scalar type
- Parameters
-
factor The scaling factor perm The permutation to be applied to this tensor
- Returns
- A permuted copy of this tensor that contains the complex conjugate values
Definition at line 955 of file tensor_interface.h.

◆ conj_to() [1/2]
|
inline |
Complex conjugate this tensor.
- Returns
- A reference to this tensor
Definition at line 962 of file tensor_interface.h.

◆ conj_to() [2/2]
|
inline |
Complex conjugate and scale this tensor.
- Template Parameters
-
Scalar A scalar type
- Parameters
-
factor The scaling factor
- Returns
- A reference to this tensor
Definition at line 971 of file tensor_interface.h.

◆ data()
|
inline |
Data pointer accessor.
- Returns
- The data pointer of the parent tensor
Definition at line 216 of file tensor_interface.h.
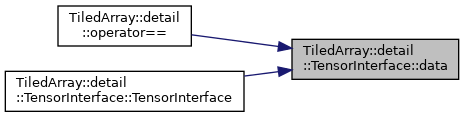
◆ dot()
|
inline |
Vector dot product.
- Template Parameters
-
Right The right-hand tensor type
- Parameters
-
other The right-hand tensor to be reduced
- Returns
- The inner product of the this and
other
Definition at line 1110 of file tensor_interface.h.
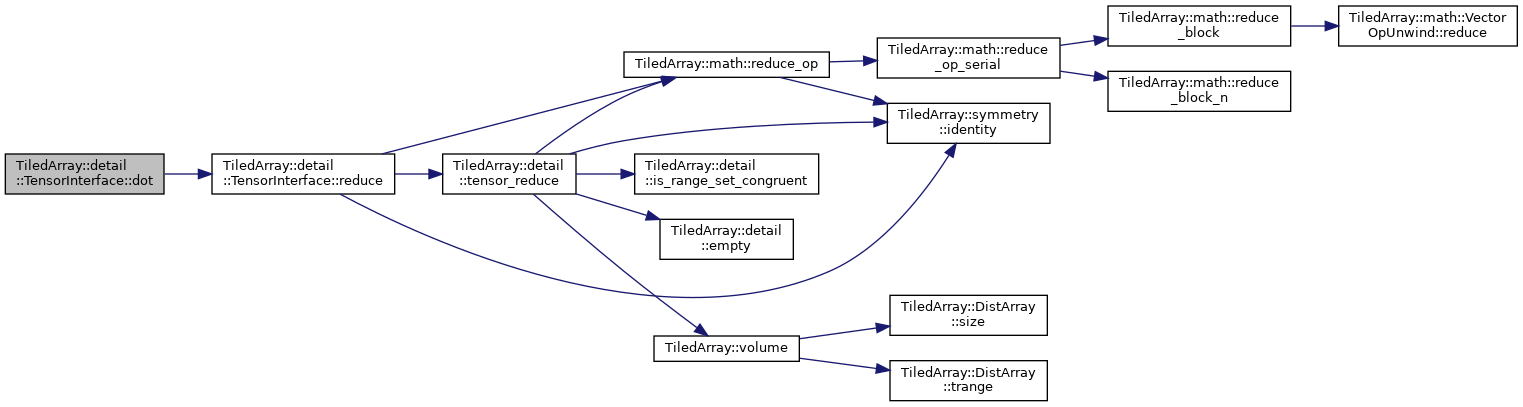
◆ empty()
|
inlineconstexpr |
◆ inner_product()
|
inline |
Vector inner product
- Template Parameters
-
Right The right-hand tensor type
- Parameters
-
other The right-hand tensor to be reduced
- Returns
- The dot product of the this and
other
If numeric_type is real, this is equivalent to dot product
- See also
- Tensor::dot
Definition at line 1126 of file tensor_interface.h.

◆ inplace_binary()
|
inline |
Use a binary, element wise operation to modify this tensor.
- Template Parameters
-
Right The right-hand tensor type Op The binary operation type
- Parameters
-
right The right-hand argument in the binary operation op The binary, element-wise operation
- Returns
- A reference to this object
- Exceptions
-
TiledArray::Exception When this tensor is empty. TiledArray::Exception When other
is empty.TiledArray::Exception When the range of this tensor is not equal to the range of other
.TiledArray::Exception When this and other
are the same.
Definition at line 382 of file tensor_interface.h.

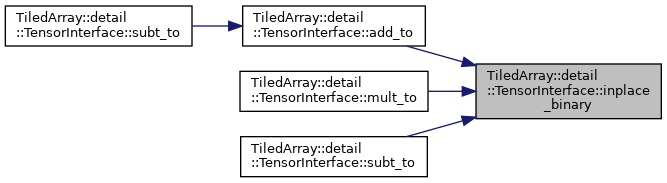
◆ inplace_unary()
|
inline |
Use a unary, element wise operation to modify this tensor.
- Template Parameters
-
Op The unary operation type
- Parameters
-
op The unary, element-wise operation
- Returns
- A reference to this object
- Exceptions
-
TiledArray::Exception When this tensor is empty.
Definition at line 439 of file tensor_interface.h.

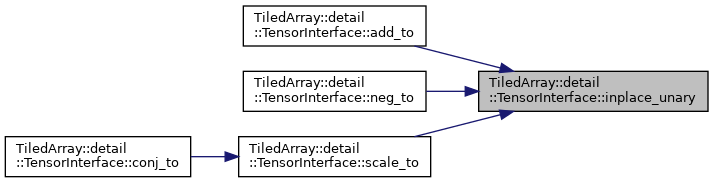
◆ max()
|
inline |
Maximum element.
- Returns
- The maximum elements of this tensor
Definition at line 1071 of file tensor_interface.h.
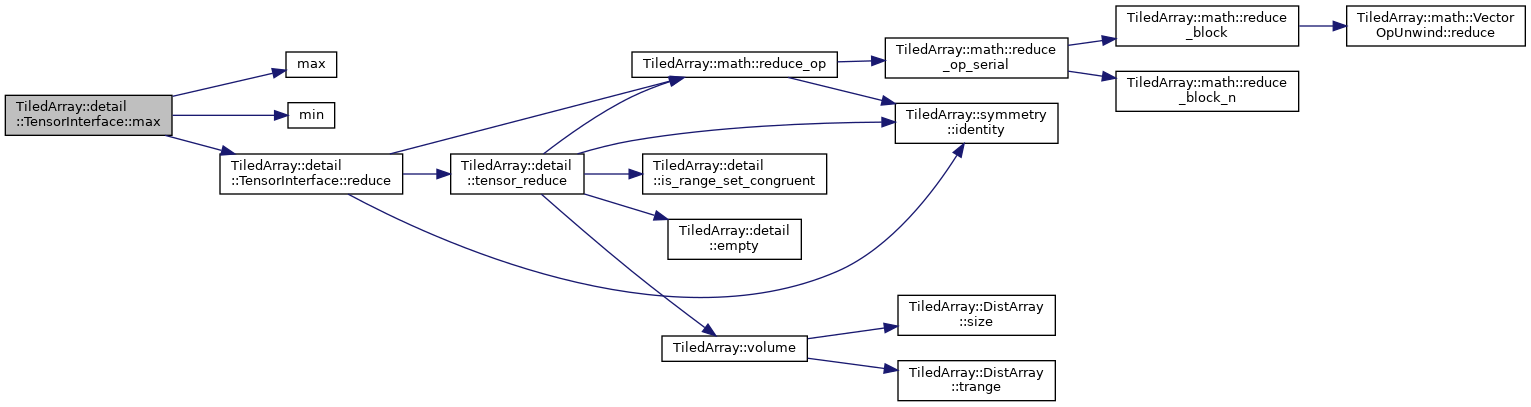
◆ min()
|
inline |
Minimum element.
- Returns
- The minimum elements of this tensor
Definition at line 1062 of file tensor_interface.h.
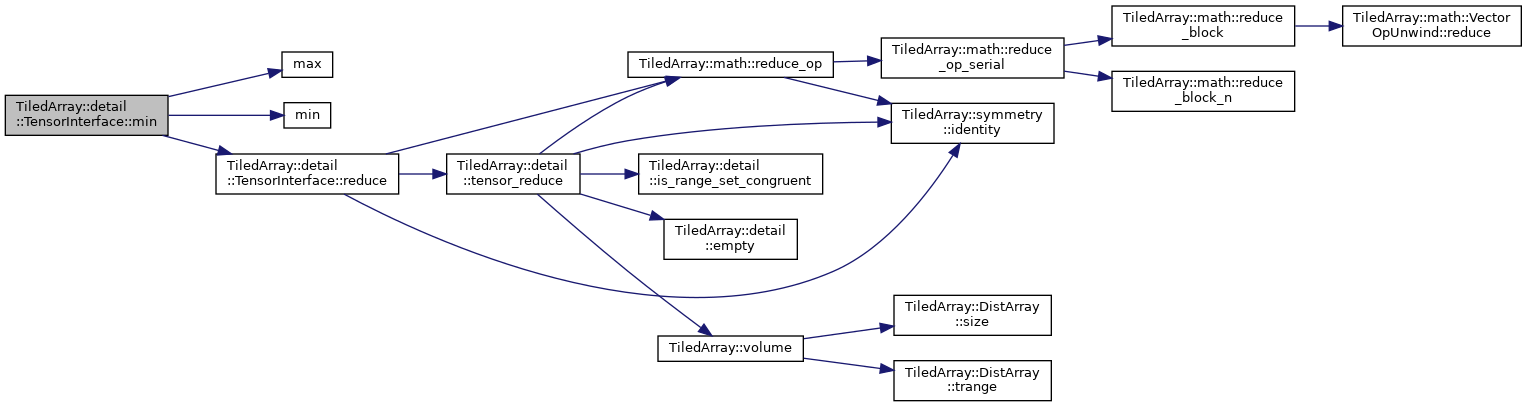
◆ mult() [1/4]
|
inline |
Multiply this by right
to create a new tensor.
- Template Parameters
-
Right The right-hand tensor type
- Parameters
-
right The tensor that will be multiplied by this tensor
- Returns
- A new tensor where the elements are the product of the elements of
this
andright
Definition at line 792 of file tensor_interface.h.
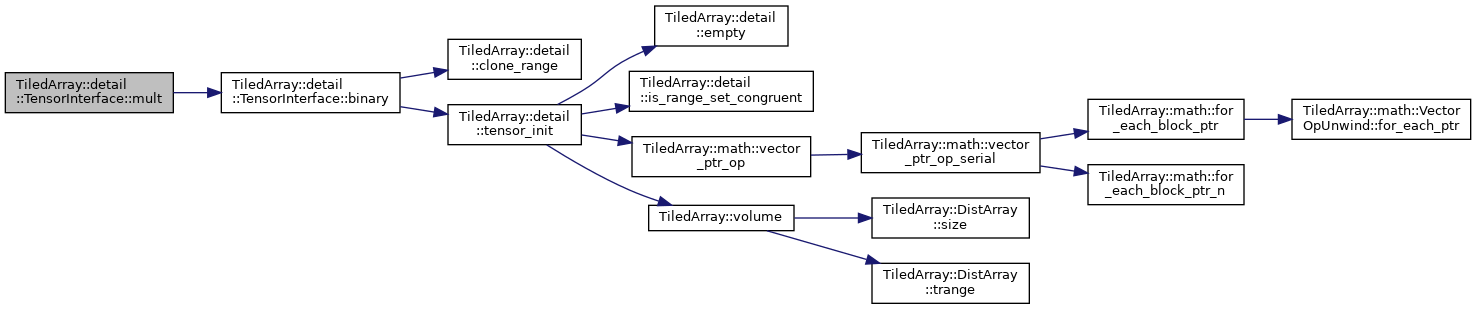
◆ mult() [2/4]
|
inline |
Multiply this by right
to create a new, permuted tensor.
- Template Parameters
-
Right The right-hand tensor type
- Parameters
-
right The tensor that will be multiplied by this tensor perm The permutation to be applied to this tensor
- Returns
- A new tensor where the elements are the product of the elements of
this
andright
Definition at line 811 of file tensor_interface.h.
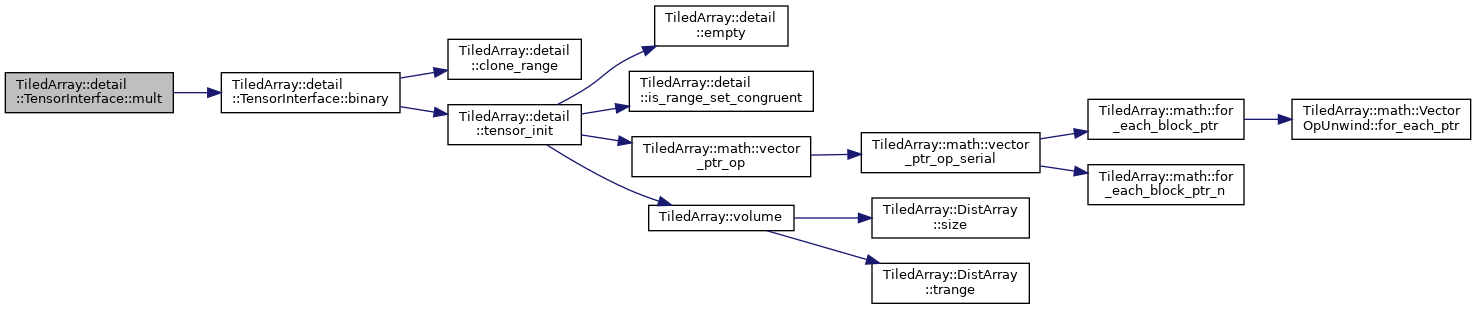
◆ mult() [3/4]
|
inline |
Scale and multiply this by right
to create a new tensor.
- Template Parameters
-
Right The right-hand tensor type Scalar A scalar type
- Parameters
-
right The tensor that will be multiplied by this tensor factor The scaling factor
- Returns
- A new tensor where the elements are the product of the elements of
this
andright
, scaled byfactor
Definition at line 832 of file tensor_interface.h.
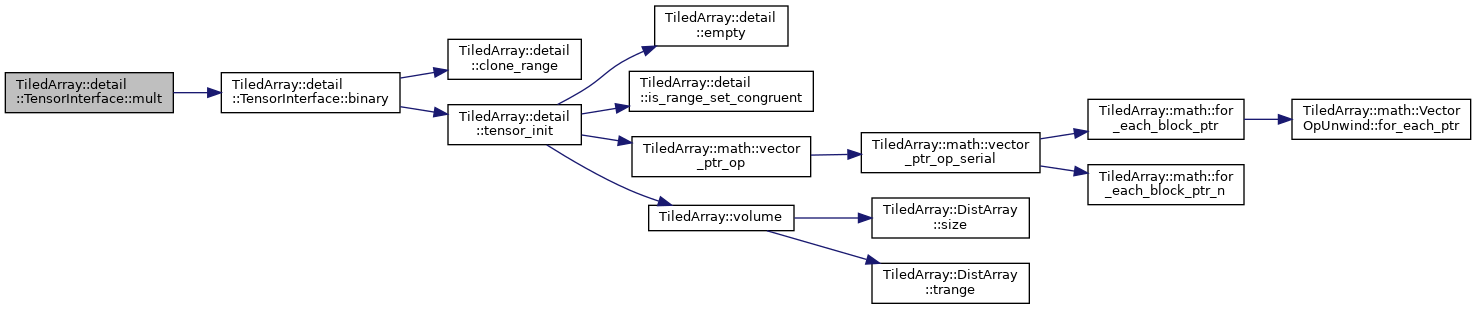
◆ mult() [4/4]
|
inline |
Scale and multiply this by right
to create a new, permuted tensor.
- Template Parameters
-
Right The right-hand tensor type Scalar A scalar type
- Parameters
-
right The tensor that will be multiplied by this tensor factor The scaling factor perm The permutation to be applied to this tensor
- Returns
- A new tensor where the elements are the product of the elements of
this
andright
, scaled byfactor
Definition at line 851 of file tensor_interface.h.
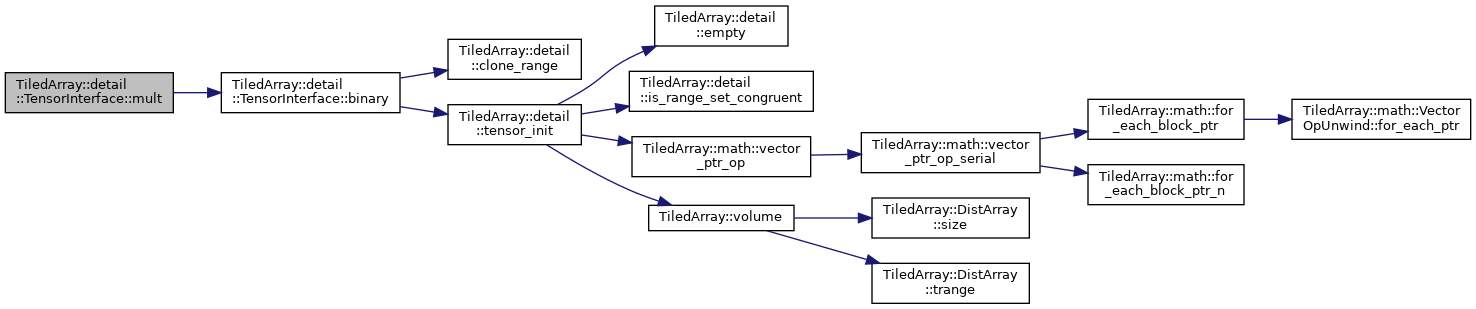
◆ mult_to() [1/2]
|
inline |
Multiply this tensor by right
.
- Template Parameters
-
Right The right-hand tensor type
- Parameters
-
right The tensor that will be multiplied by this tensor
- Returns
- A reference to this tensor
Definition at line 867 of file tensor_interface.h.

◆ mult_to() [2/2]
|
inline |
Scale and multiply this tensor by right
.
- Template Parameters
-
Right The right-hand tensor type Scalar A scalar type
- Parameters
-
right The tensor that will be multiplied by this tensor factor The scaling factor
- Returns
- A reference to this tensor
Definition at line 883 of file tensor_interface.h.

◆ neg() [1/2]
|
inline |
Create a negated copy of this tensor.
- Returns
- A new tensor that contains the negative values of this tensor
Definition at line 894 of file tensor_interface.h.
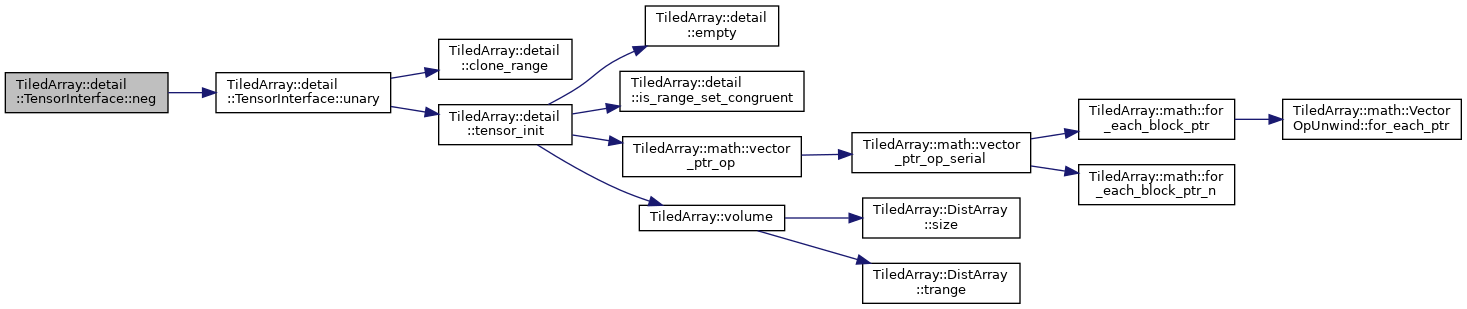
◆ neg() [2/2]
|
inline |
Create a negated and permuted copy of this tensor.
- Parameters
-
perm The permutation to be applied to this tensor
- Returns
- A new tensor that contains the negative values of this tensor
Definition at line 904 of file tensor_interface.h.
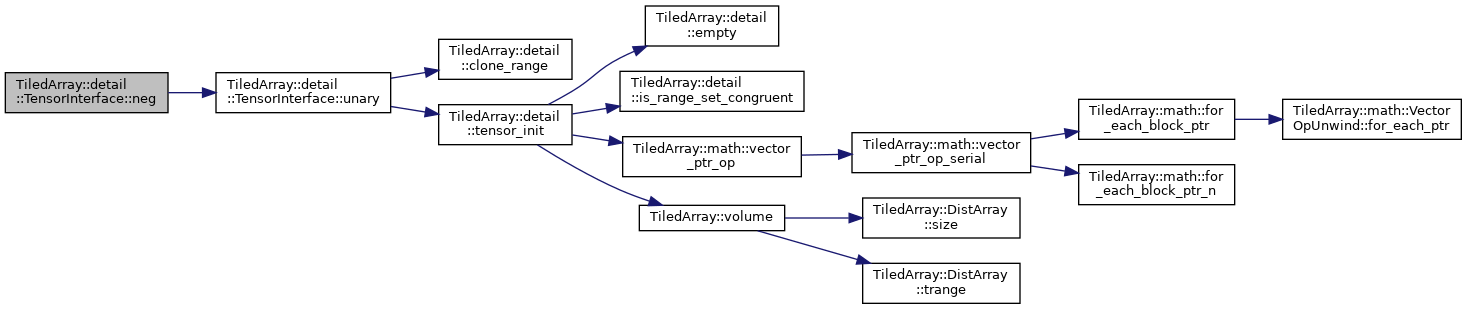
◆ neg_to()
|
inline |
Negate elements of this tensor.
- Returns
- A reference to this tensor
Definition at line 911 of file tensor_interface.h.

◆ norm()
|
inline |
Vector 2-norm.
- Template Parameters
-
ResultType the return type
- Note
- This evaluates
std::sqrt
(ResultType(this->squared_norm()))
- Returns
- The vector norm of this tensor
Definition at line 1055 of file tensor_interface.h.
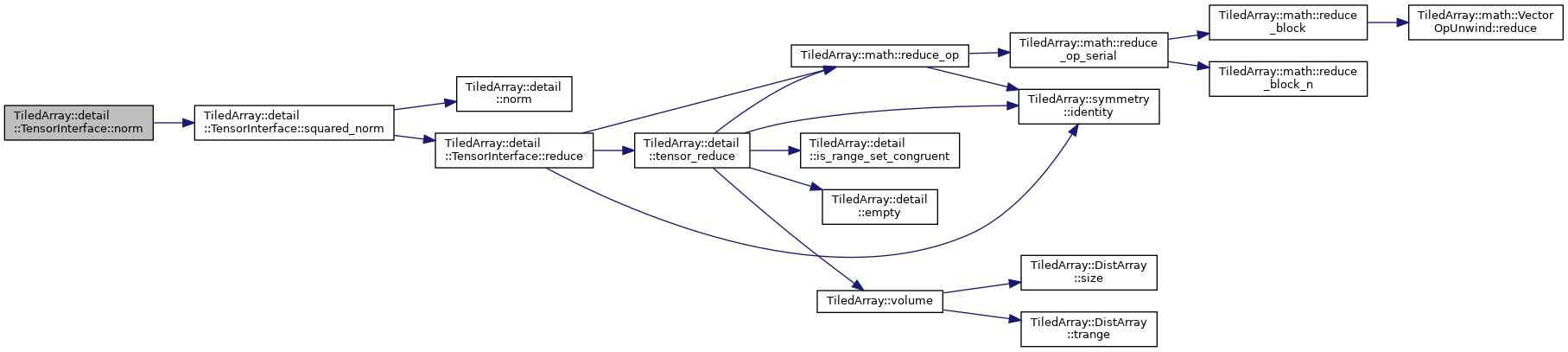
◆ operator()() [1/2]
|
inline |
Element accessor.
- Template Parameters
-
Index An integral type pack or a single coodinate index type
- Parameters
-
idx The index pack
Definition at line 241 of file tensor_interface.h.
◆ operator()() [2/2]
|
inline |
Element accessor.
- Template Parameters
-
Index An integral type pack or a single coodinate index type
- Parameters
-
idx The index pack
Definition at line 251 of file tensor_interface.h.
◆ operator=() [1/3]
|
inline |
◆ operator=() [2/3]
|
delete |
◆ operator=() [3/3]
|
delete |
◆ operator[]() [1/2]
|
inline |
Element subscript accessor.
- Parameters
-
index The ordinal element index
- Returns
- A const reference to the element at
index
.
Definition at line 231 of file tensor_interface.h.
◆ operator[]() [2/2]
|
inline |
Element subscript accessor.
- Parameters
-
index The ordinal element index
- Returns
- A const reference to the element at
index
.
Definition at line 222 of file tensor_interface.h.
◆ permute()
|
inline |
◆ product()
|
inline |
Product of elements.
- Returns
- The product of all elements of this tensor
Definition at line 1029 of file tensor_interface.h.
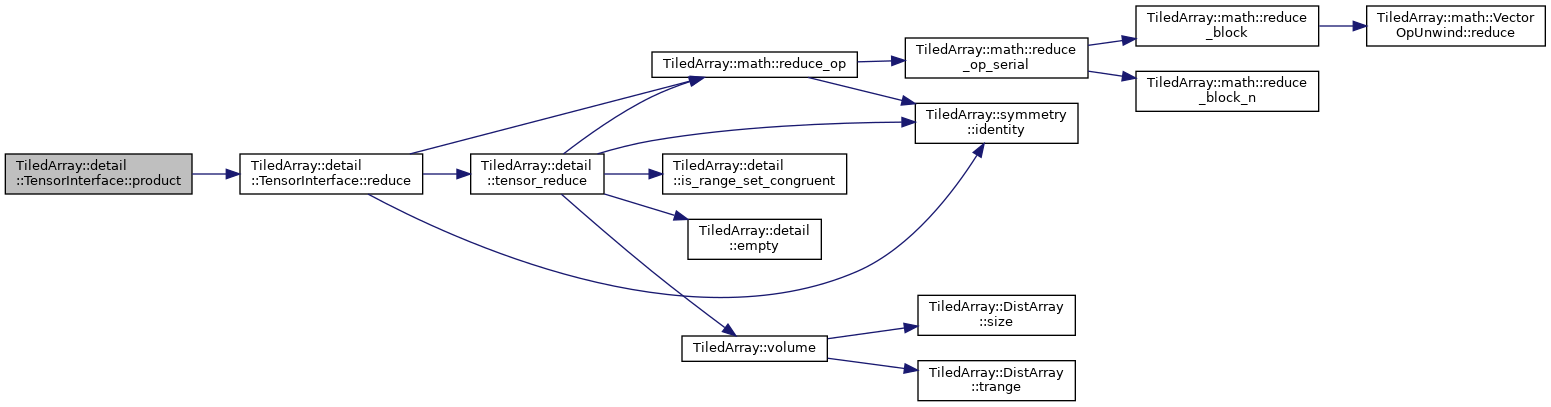
◆ range()
|
inline |
Tensor range object accessor.
- Returns
- The tensor range object
Definition at line 206 of file tensor_interface.h.
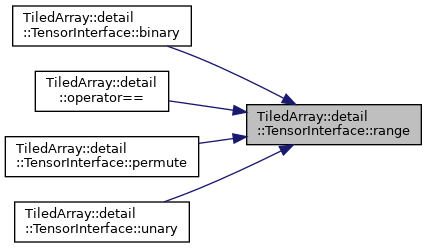
◆ reduce() [1/2]
|
inline |
Binary reduction operation.
Perform an element-wise binary reduction of the data of this
and other
by executing join_op(result, reduce_op(*this[i], other[i]))
for each i
in the index range of this
. result
is initialized to identity
. If HAVE_INTEL_TBB is defined, and this is a contiguous tensor, the reduction will be executed in an undefined order, otherwise will execute in the order of increasing i
.
- Template Parameters
-
Right The right-hand argument tensor type ReduceOp The reduction operation type JoinOp The join operation type
- Parameters
-
other The right-hand argument of the binary reduction reduce_op The element-wise reduction operation join_op The join result operation identity The identity value of the reduction
- Returns
- The reduced value
Definition at line 1010 of file tensor_interface.h.
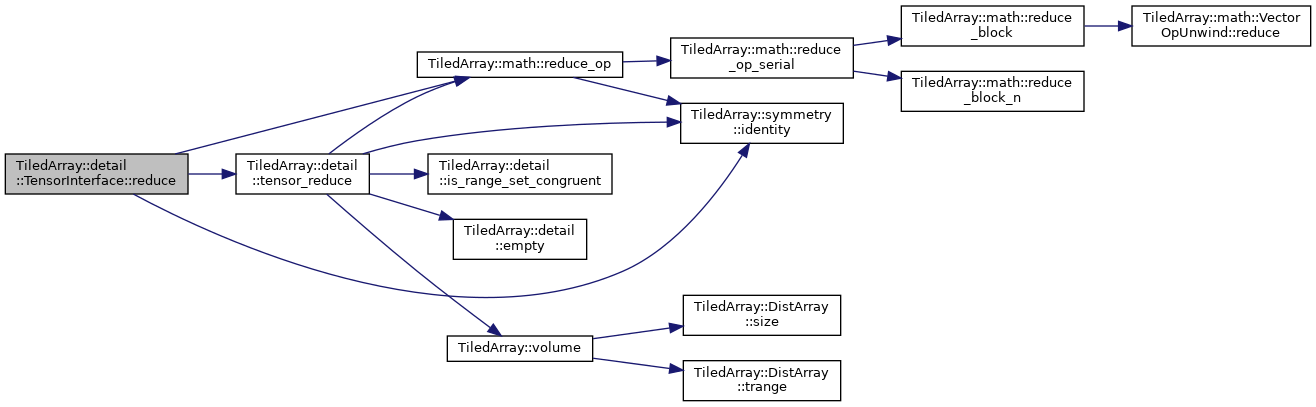
◆ reduce() [2/2]
|
inline |
Unary reduction operation.
Perform an element-wise reduction of the data by executing join_op(result, reduce_op(*this[i]))
for each i
in the index range of this
. result
is initialized to identity
. If HAVE_INTEL_TBB is defined, and this is a contiguous tensor, the reduction will be executed in an undefined order, otherwise will execute in the order of increasing i
.
- Template Parameters
-
ReduceOp The reduction operation type JoinOp The join operation type
- Parameters
-
reduce_op The element-wise reduction operation join_op The join result operation identity The identity value of the reduction
- Returns
- The reduced value
Definition at line 988 of file tensor_interface.h.
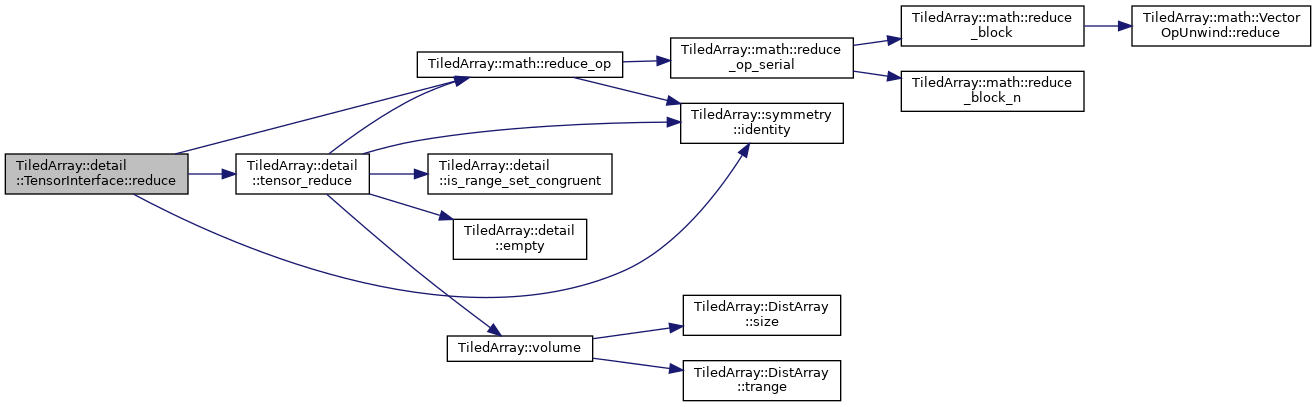
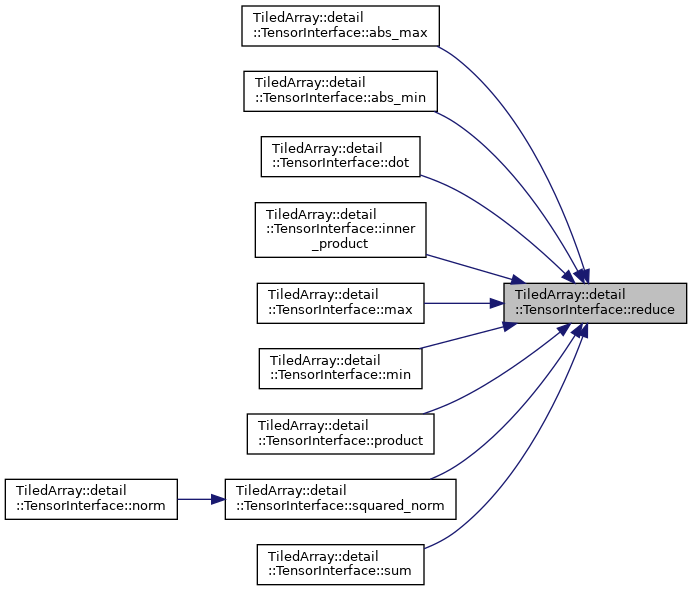
◆ scale() [1/2]
|
inline |
Construct a scaled copy of this tensor.
- Template Parameters
-
Scalar A scalar type
- Parameters
-
factor The scaling factor
- Returns
- A new tensor where the elements of this tensor are scaled by
factor
Definition at line 478 of file tensor_interface.h.
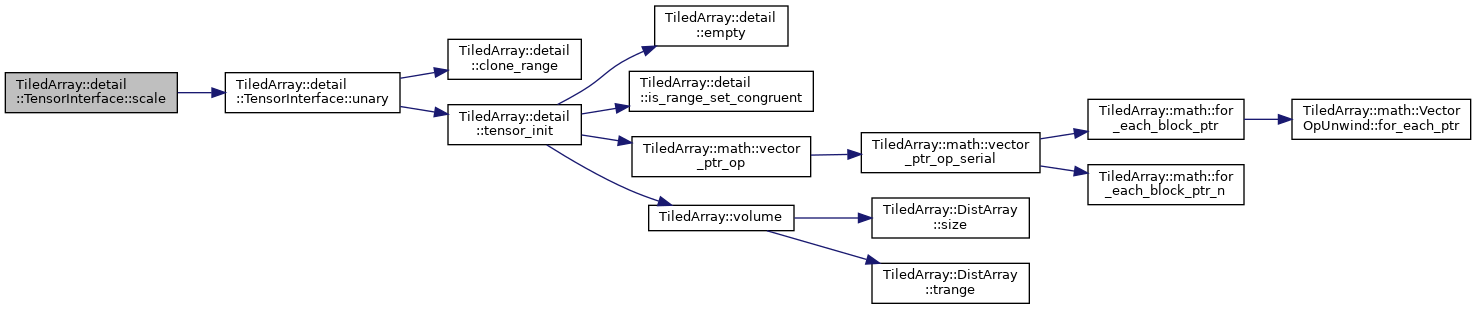

◆ scale() [2/2]
|
inline |
Construct a scaled and permuted copy of this tensor.
- Template Parameters
-
Scalar A scalar type
- Parameters
-
factor The scaling factor perm The permutation to be applied to this tensor
- Returns
- A new tensor where the elements of this tensor are scaled by
factor
and permuted
Definition at line 494 of file tensor_interface.h.
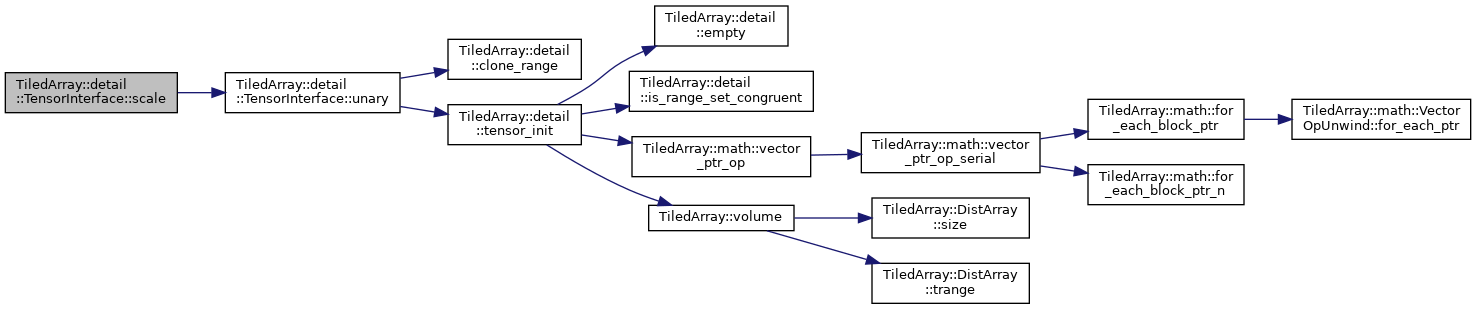
◆ scale_to()
|
inline |
Scale this tensor.
- Template Parameters
-
Scalar A scalar type
- Parameters
-
factor The scaling factor
- Returns
- A reference to this tensor
Definition at line 507 of file tensor_interface.h.


◆ shift() [1/2]
|
inline |
Make a copy of this view shited by bound_shift
.
- Template Parameters
-
Index An integral range type
- Parameters
-
bound_shift The shift to be applied to the tensor range
- Returns
- A copy of the shifted view
Definition at line 300 of file tensor_interface.h.
◆ shift() [2/2]
|
inline |
Make a copy of this view shited by bound_shift
.
- Template Parameters
-
Index An integral range type
- Parameters
-
bound_shift The shift to be applied to the tensor range
- Returns
- A copy of the shifted view
Definition at line 311 of file tensor_interface.h.
◆ shift_to() [1/2]
|
inline |
Shift the lower and upper bound of this tensor view.
- Template Parameters
-
Index An integral range type
- Parameters
-
bound_shift The shift to be applied to the tensor range
- Returns
- Reference to
this
Definition at line 276 of file tensor_interface.h.
◆ shift_to() [2/2]
|
inline |
Shift the lower and upper bound of this tensor view.
- Template Parameters
-
Index An integral type
- Parameters
-
bound_shift The shift to be applied to the tensor range
- Returns
- Reference to
this
Definition at line 288 of file tensor_interface.h.
◆ size()
|
inline |
Tensor dimension size accessor.
- Returns
- The number of elements in the tensor
Definition at line 211 of file tensor_interface.h.
◆ squared_norm()
|
inline |
Square of vector 2-norm.
- Returns
- The vector norm of this tensor
Definition at line 1038 of file tensor_interface.h.
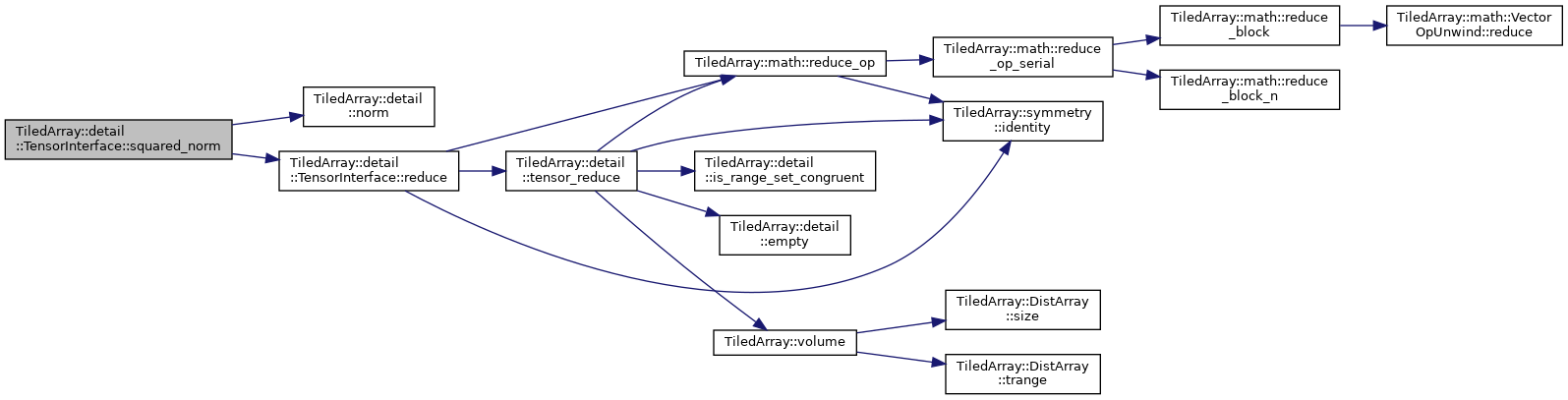

◆ subt() [1/6]
|
inline |
Subtract a constant from a copy of this tensor.
- Returns
- A new tensor where the elements are the different between the elements of
this
andvalue
Definition at line 734 of file tensor_interface.h.

◆ subt() [2/6]
|
inline |
Subtract a constant from a permuted copy of this tensor.
- Parameters
-
value The constant to be subtracted perm The permutation to be applied to this tensor
- Returns
- A new tensor where the elements are the different between the elements of
this
andvalue
Definition at line 744 of file tensor_interface.h.

◆ subt() [3/6]
|
inline |
Subtract this and right
to construct a new tensor.
- Template Parameters
-
Right The right-hand tensor type
- Parameters
-
right The tensor that will be subtracted from this tensor
- Returns
- A new tensor where the elements are the different between the elements of
this
andright
Definition at line 662 of file tensor_interface.h.
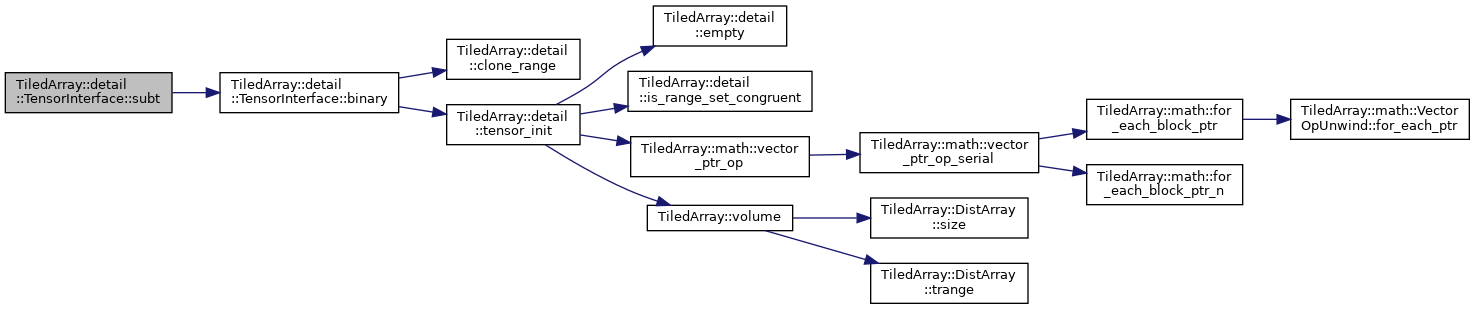
◆ subt() [4/6]
|
inline |
Subtract this and right
to construct a new, permuted tensor.
- Template Parameters
-
Right The right-hand tensor type
- Parameters
-
right The tensor that will be subtracted from this tensor perm The permutation to be applied to this tensor
- Returns
- A new tensor where the elements are the different between the elements of
this
andright
Definition at line 681 of file tensor_interface.h.
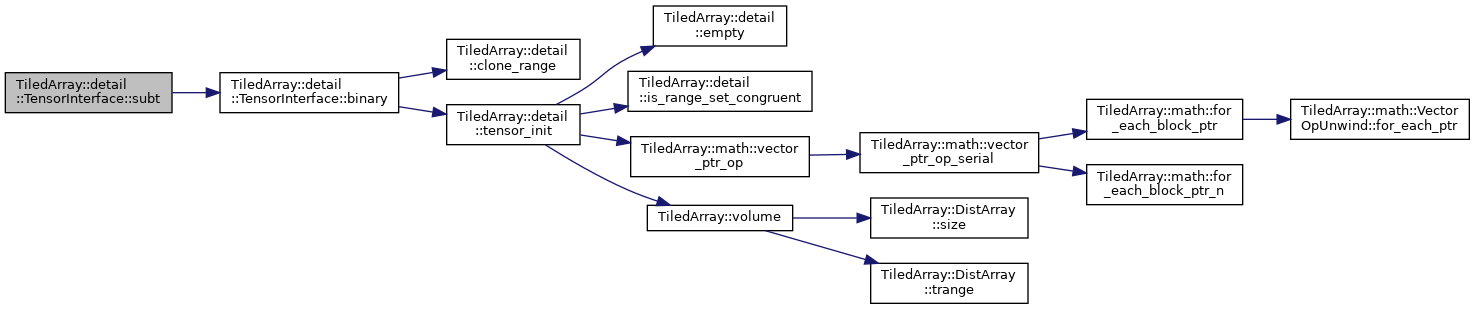
◆ subt() [5/6]
|
inline |
Scale and subtract this and right
to construct a new tensor.
- Template Parameters
-
Right The right-hand tensor type Scalar A scalar type
- Parameters
-
right The tensor that will be subtracted from this tensor factor The scaling factor
- Returns
- A new tensor where the elements are the different between the elements of
this
andright
, scaled byfactor
Definition at line 702 of file tensor_interface.h.
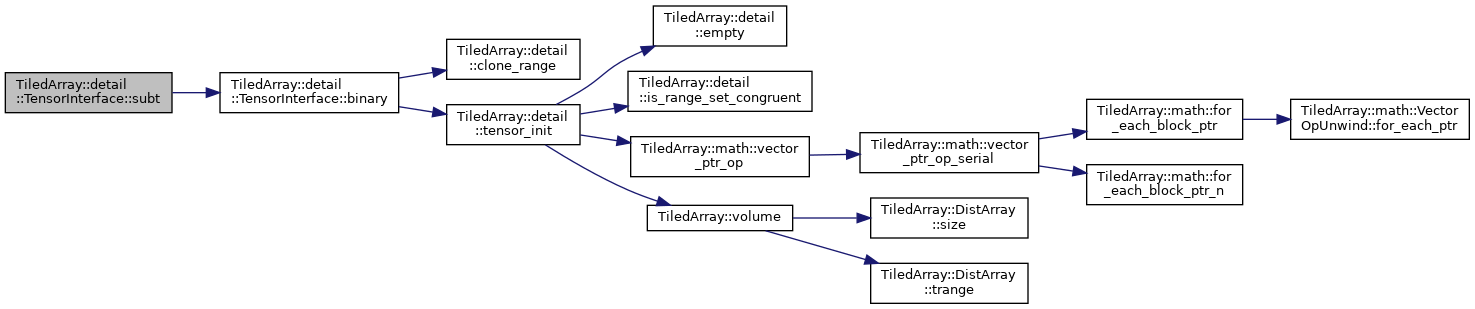
◆ subt() [6/6]
|
inline |
Scale and subtract this and right
to construct a new, permuted tensor.
- Template Parameters
-
Right The right-hand tensor type Scalar A scalar type
- Parameters
-
right The tensor that will be subtracted from this tensor factor The scaling factor perm The permutation to be applied to this tensor
- Returns
- A new tensor where the elements are the different between the elements of
this
andright
, scaled byfactor
Definition at line 721 of file tensor_interface.h.
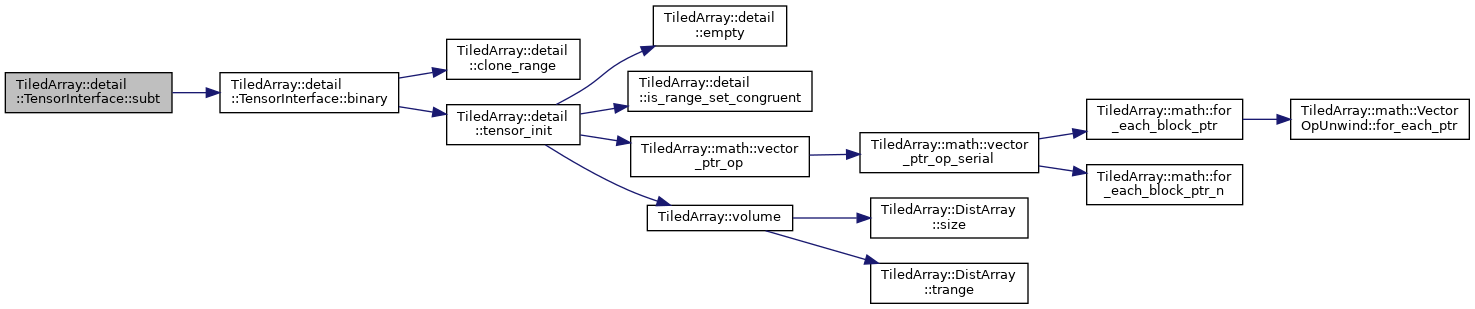
◆ subt_to() [1/3]
|
inline |
Subtract a constant from this tensor.
- Returns
- A reference to this tensor
Definition at line 780 of file tensor_interface.h.

◆ subt_to() [2/3]
|
inline |
Subtract right
from this tensor.
- Template Parameters
-
Right The right-hand tensor type
- Parameters
-
right The tensor that will be subtracted from this tensor
- Returns
- A reference to this tensor
Definition at line 755 of file tensor_interface.h.

◆ subt_to() [3/3]
|
inline |
Subtract right
from and scale this tensor.
- Template Parameters
-
Right The right-hand tensor type Scalar A scalar type
- Parameters
-
right The tensor that will be subtracted from this tensor factor The scaling factor
- Returns
- A reference to this tensor
Definition at line 771 of file tensor_interface.h.

◆ sum()
|
inline |
Sum of elements.
- Returns
- The sum of all elements of this tensor
Definition at line 1020 of file tensor_interface.h.
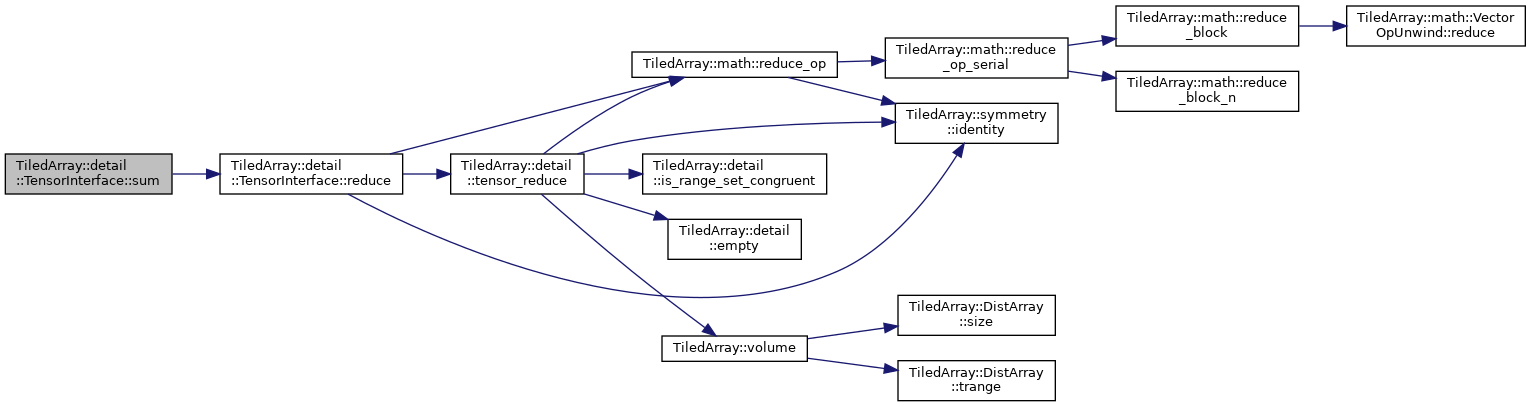
◆ swap()
|
inline |
Swap tensor views.
- Parameters
-
other The view to be swapped
Definition at line 264 of file tensor_interface.h.

◆ unary() [1/2]
|
inline |
Use a unary, element wise operation to construct a new tensor.
- Template Parameters
-
Op The unary operation type
- Parameters
-
op The unary, element-wise operation
- Returns
- A tensor where element
i
of the new tensor is equal toop(*this[i])
- Exceptions
-
TiledArray::Exception When this tensor is empty.
Definition at line 395 of file tensor_interface.h.
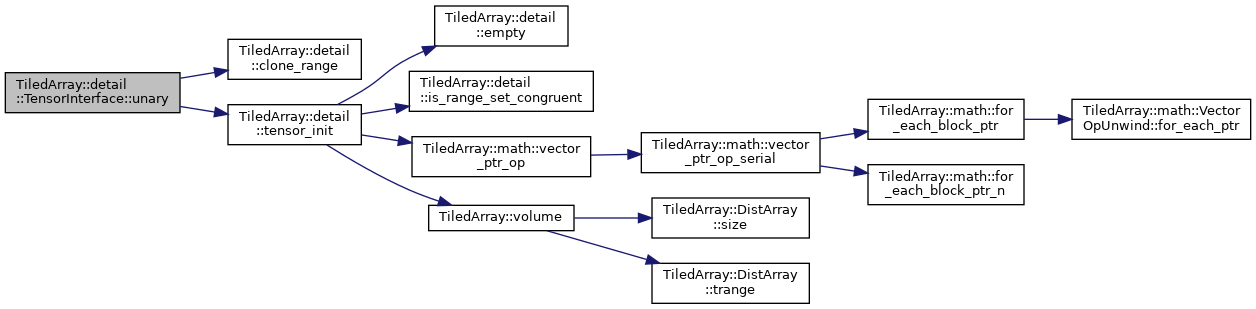
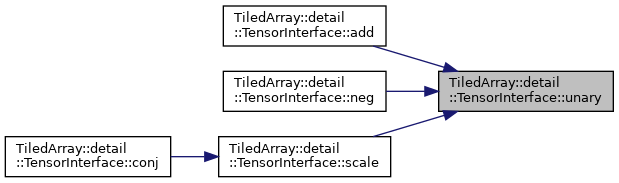
◆ unary() [2/2]
|
inline |
Use a unary, element wise operation to construct a new, permuted tensor.
- Template Parameters
-
Op The unary operation type
- Parameters
-
op The unary operation perm The permutation to be applied to this tensor
- Returns
- A permuted tensor with elements that have been modified by
op
- Exceptions
-
TiledArray::Exception When this tensor is empty. TiledArray::Exception The dimension of perm
does not match that of this tensor.
Definition at line 413 of file tensor_interface.h.
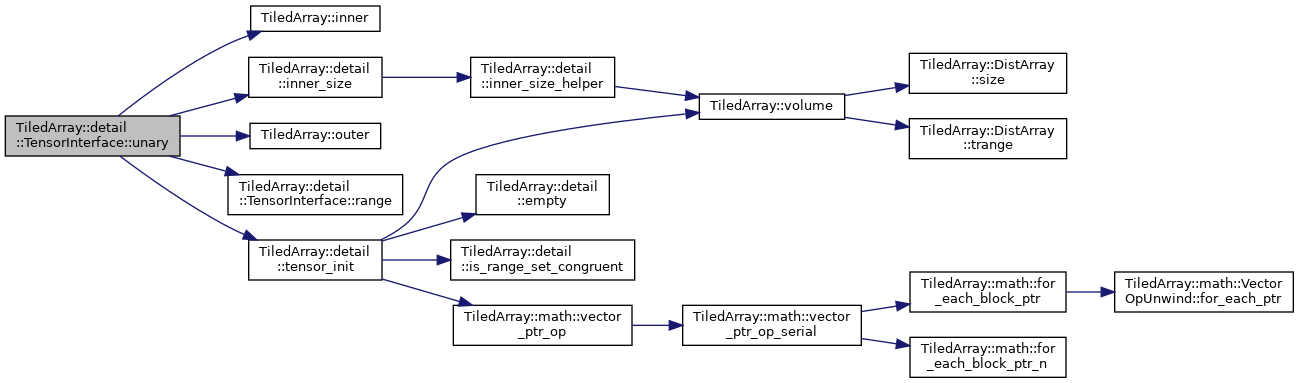
Friends And Related Function Documentation
◆ TensorInterface
|
friend |
Definition at line 114 of file tensor_interface.h.
◆ TiledArray::remap [1/4]
|
friend |
◆ TiledArray::remap [2/4]
|
friend |
◆ TiledArray::remap [3/4]
|
friend |
◆ TiledArray::remap [4/4]
|
friend |
The documentation for this class was generated from the following file:
- TiledArray/tensor/tensor_interface.h