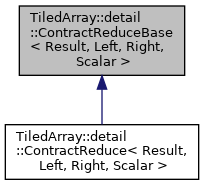
Documentation
template<typename Result, typename Left, typename Right, typename Scalar>
class TiledArray::detail::ContractReduceBase< Result, Left, Right, Scalar >
Contract and (sum) reduce base.
This implementation class is used to provide shallow copy semantics for ContractReduce. It encodes a binary tensor contraction mapped to a GEMM, as well as the sum reduction and post-processing.
- Template Parameters
-
Result The result tile type Left The left-hand tile type Right The right-hand tile type Scalar The scaling factor type
Definition at line 52 of file contract_reduce.h.
Public Types | |
typedef ContractReduceBase< Result, Left, Right, Scalar > | ContractReduceBase_ |
This class type. More... | |
typedef const Left & | first_argument_type |
The left tile type. More... | |
typedef const Right & | second_argument_type |
The right tile type. More... | |
typedef Result | result_type |
The result type. More... | |
typedef Scalar | scalar_type |
The scaling factor type. More... | |
using | left_value_type = typename Left::value_type |
using | right_value_type = typename Right::value_type |
using | result_value_type = typename Result::value_type |
using | elem_muladd_op_type = void(result_value_type &, const left_value_type &, const right_value_type &) |
Public Member Functions | |
ContractReduceBase ()=default | |
ContractReduceBase (const ContractReduceBase_ &)=default | |
ContractReduceBase (ContractReduceBase_ &&)=default | |
~ContractReduceBase ()=default | |
ContractReduceBase_ & | operator= (const ContractReduceBase_ &)=default |
ContractReduceBase_ & | operator= (ContractReduceBase_ &&)=default |
template<typename Perm = BipartitePermutation, typename ElemMultAddOp = TiledArray::function_ref<elem_muladd_op_type>, typename = std::enable_if_t< TiledArray::detail::is_permutation_v<Perm> && std::is_invocable_r_v<void, std::remove_reference_t<ElemMultAddOp>, result_value_type&, const left_value_type&, const right_value_type&>>> | |
ContractReduceBase (const math::blas::Op left_op, const math::blas::Op right_op, const scalar_type alpha, const unsigned int result_rank, const unsigned int left_rank, const unsigned int right_rank, const Perm &perm={}, ElemMultAddOp &&elem_muladd_op={}) | |
Construct contract/reduce functor. More... | |
const math::GemmHelper & | gemm_helper () const |
Gemm meta data accessor. More... | |
const BipartitePermutation & | perm () const |
Permutation accessor. More... | |
scalar_type | factor () const |
Scaling factor accessor. More... | |
const auto & | elem_muladd_op () const |
Element multiply-add op accessor. More... | |
unsigned int | num_contract_ranks () const |
Compute the number of contracted ranks. More... | |
unsigned int | result_rank () const |
Result rank accessor. More... | |
unsigned int | left_rank () const |
Left-hand argument rank accessor. More... | |
unsigned int | right_rank () const |
Right-hand argument rank accessor. More... | |
Static Public Attributes | |
static constexpr bool | plain_tensors |
Member Typedef Documentation
◆ ContractReduceBase_
typedef ContractReduceBase<Result, Left, Right, Scalar> TiledArray::detail::ContractReduceBase< Result, Left, Right, Scalar >::ContractReduceBase_ |
This class type.
Definition at line 55 of file contract_reduce.h.
◆ elem_muladd_op_type
using TiledArray::detail::ContractReduceBase< Result, Left, Right, Scalar >::elem_muladd_op_type = void(result_value_type&, const left_value_type&, const right_value_type&) |
Definition at line 64 of file contract_reduce.h.
◆ first_argument_type
typedef const Left& TiledArray::detail::ContractReduceBase< Result, Left, Right, Scalar >::first_argument_type |
The left tile type.
Definition at line 56 of file contract_reduce.h.
◆ left_value_type
using TiledArray::detail::ContractReduceBase< Result, Left, Right, Scalar >::left_value_type = typename Left::value_type |
Definition at line 61 of file contract_reduce.h.
◆ result_type
typedef Result TiledArray::detail::ContractReduceBase< Result, Left, Right, Scalar >::result_type |
The result type.
Definition at line 58 of file contract_reduce.h.
◆ result_value_type
using TiledArray::detail::ContractReduceBase< Result, Left, Right, Scalar >::result_value_type = typename Result::value_type |
Definition at line 63 of file contract_reduce.h.
◆ right_value_type
using TiledArray::detail::ContractReduceBase< Result, Left, Right, Scalar >::right_value_type = typename Right::value_type |
Definition at line 62 of file contract_reduce.h.
◆ scalar_type
typedef Scalar TiledArray::detail::ContractReduceBase< Result, Left, Right, Scalar >::scalar_type |
The scaling factor type.
Definition at line 59 of file contract_reduce.h.
◆ second_argument_type
typedef const Right& TiledArray::detail::ContractReduceBase< Result, Left, Right, Scalar >::second_argument_type |
The right tile type.
Definition at line 57 of file contract_reduce.h.
Constructor & Destructor Documentation
◆ ContractReduceBase() [1/4]
|
default |
◆ ContractReduceBase() [2/4]
|
default |
◆ ContractReduceBase() [3/4]
|
default |
◆ ~ContractReduceBase()
|
default |
◆ ContractReduceBase() [4/4]
|
inline |
Construct contract/reduce functor.
- Template Parameters
-
Perm a permutation type ElemMultAddOp a callable with signature elem_muladd_op_type
- Parameters
-
left_op The left-hand BLAS matrix operation right_op The right-hand BLAS matrix operation alpha The scaling factor applied to the contracted tiles result_rank The rank of the result tensor left_rank The rank of the left-hand tensor right_rank The rank of the right-hand tensor perm The permutation to be applied to the result tensor (default = no permute) elem_muladd_op The element multiply-add op
Definition at line 145 of file contract_reduce.h.
Member Function Documentation
◆ elem_muladd_op()
|
inline |
Element multiply-add op accessor.
- Returns
- A const reference to the element multiply-add op function_ref
Definition at line 182 of file contract_reduce.h.
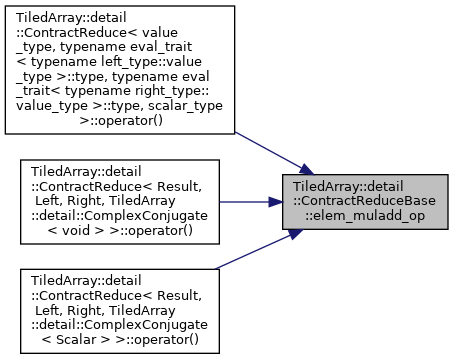
◆ factor()
|
inline |
Scaling factor accessor.
- Returns
- The scaling factor for this operation
Definition at line 174 of file contract_reduce.h.
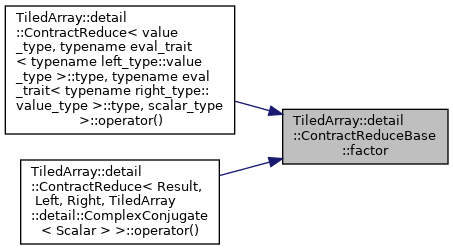
◆ gemm_helper()
|
inline |
Gemm meta data accessor.
- Returns
- A const reference to the gemm helper object
Definition at line 158 of file contract_reduce.h.
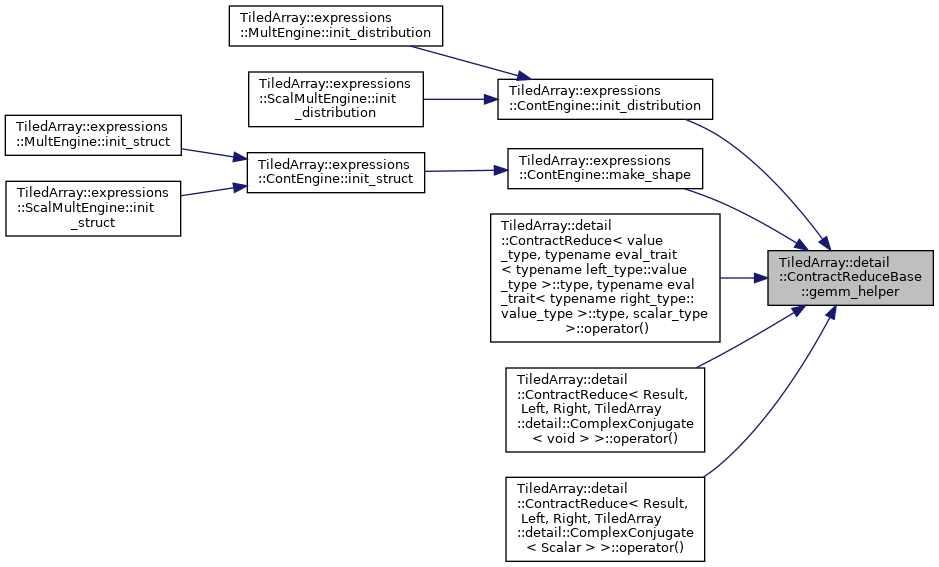
◆ left_rank()
|
inline |
Left-hand argument rank accessor.
- Returns
- The rank of the left-hand tile
Definition at line 208 of file contract_reduce.h.
◆ num_contract_ranks()
|
inline |
Compute the number of contracted ranks.
- Returns
- The number of ranks that are summed by this operation
Definition at line 192 of file contract_reduce.h.
◆ operator=() [1/2]
|
default |
◆ operator=() [2/2]
|
default |
◆ perm()
|
inline |
Permutation accessor.
- Returns
- A const reference to the permutation for this operation
Definition at line 166 of file contract_reduce.h.
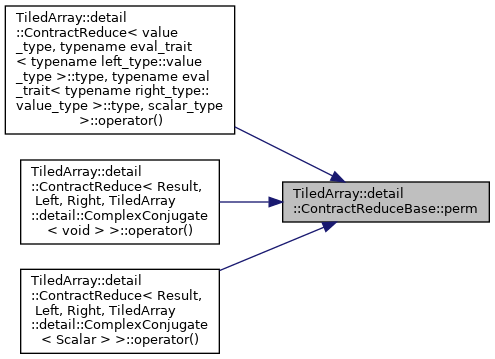
◆ result_rank()
|
inline |
Result rank accessor.
- Returns
- The rank of the result tile
Definition at line 200 of file contract_reduce.h.
◆ right_rank()
|
inline |
Right-hand argument rank accessor.
- Returns
- The rank of the right-hand tile
Definition at line 216 of file contract_reduce.h.
Member Data Documentation
◆ plain_tensors
|
staticconstexpr |
Definition at line 74 of file contract_reduce.h.
The documentation for this class was generated from the following file:
- TiledArray/tile_op/contract_reduce.h