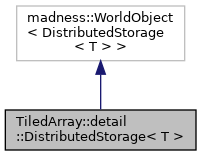
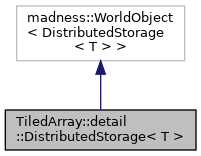
Documentation
template<typename T>
class TiledArray::detail::DistributedStorage< T >
Distributed storage container.
Each element in this container is owned by a single node, but any node may request a copy of the element in the form of a Future
. The owner of each element is defined by a process map (pmap), which is passed to the constructor. Elements do not need to be explicitly initialized because they will be added to the container when the element is first accessed, though you may manually initialize an element with the insert()
function. All elements are stored in Future
, which may be set only once.
- Note
- This object is derived from
WorldObject
, which means the order of construction of object must be the same on all nodes. This can easily be achieved by only constructing world objects in the main thread. DO NOT construct world objects within tasks where the order of execution is nondeterministic.
Definition at line 44 of file distributed_storage.h.
Public Types | |
typedef DistributedStorage< T > | DistributedStorage_ |
This object type. More... | |
typedef madness::WorldObject< DistributedStorage_ > | WorldObject_ |
Base object type. More... | |
typedef std::size_t | size_type |
size type More... | |
typedef size_type | key_type |
element key type More... | |
typedef T | value_type |
Element type. More... | |
typedef Future< value_type > | future |
Element container type. More... | |
typedef Pmap | pmap_interface |
Process map interface type. More... | |
typedef madness::ConcurrentHashMap< key_type, future > | container_type |
Local container type. More... | |
typedef container_type::accessor | accessor |
Local element accessor type. More... | |
typedef container_type::const_accessor | const_accessor |
Local element const accessor type. More... | |
Public Member Functions | |
DistributedStorage (World &world, size_type max_size, const std::shared_ptr< pmap_interface > &pmap) | |
virtual | ~DistributedStorage () |
const std::shared_ptr< pmap_interface > & | pmap () const |
Process map accessor. More... | |
ProcessID | owner (size_type i) const |
Element owner. More... | |
bool | is_local (size_type i) const |
Local element query. More... | |
size_type | size () const |
Number of local elements. More... | |
size_type | max_size () const |
Max size accessor. More... | |
future | get (size_type i) const |
Get local or remote element. More... | |
const future & | get_local (const size_type i) const |
Get local element. More... | |
future & | get_local (const size_type i) |
Get local element. More... | |
void | set (size_type i, const value_type &value) |
Set element i with value . More... | |
void | set (size_type i, const future &f) |
Set element i with a Future f . More... | |
Friends | |
struct | DelayedSet |
Member Typedef Documentation
◆ accessor
typedef container_type::accessor TiledArray::detail::DistributedStorage< T >::accessor |
Local element accessor type.
Definition at line 58 of file distributed_storage.h.
◆ const_accessor
typedef container_type::const_accessor TiledArray::detail::DistributedStorage< T >::const_accessor |
Local element const accessor type.
Definition at line 60 of file distributed_storage.h.
◆ container_type
typedef madness::ConcurrentHashMap<key_type, future> TiledArray::detail::DistributedStorage< T >::container_type |
Local container type.
Definition at line 56 of file distributed_storage.h.
◆ DistributedStorage_
typedef DistributedStorage<T> TiledArray::detail::DistributedStorage< T >::DistributedStorage_ |
This object type.
Definition at line 46 of file distributed_storage.h.
◆ future
typedef Future<value_type> TiledArray::detail::DistributedStorage< T >::future |
Element container type.
Definition at line 53 of file distributed_storage.h.
◆ key_type
typedef size_type TiledArray::detail::DistributedStorage< T >::key_type |
element key type
Definition at line 51 of file distributed_storage.h.
◆ pmap_interface
typedef Pmap TiledArray::detail::DistributedStorage< T >::pmap_interface |
Process map interface type.
Definition at line 54 of file distributed_storage.h.
◆ size_type
typedef std::size_t TiledArray::detail::DistributedStorage< T >::size_type |
size type
Definition at line 50 of file distributed_storage.h.
◆ value_type
typedef T TiledArray::detail::DistributedStorage< T >::value_type |
Element type.
Definition at line 52 of file distributed_storage.h.
◆ WorldObject_
typedef madness::WorldObject<DistributedStorage_> TiledArray::detail::DistributedStorage< T >::WorldObject_ |
Base object type.
Definition at line 48 of file distributed_storage.h.
Constructor & Destructor Documentation
◆ DistributedStorage()
|
inline |
Makes an initialized, empty container with default data distribution (no communication) A unique ID is associated with every distributed container within a world. In order to avoid synchronization when making a container, we have to assume that all processes execute this constructor in the same order (does not apply to the non-initializing, default constructor).
- Parameters
-
world The world where the distributed container lives max_size The maximum capacity of this container pmap The process map for the container (default = null pointer)
Definition at line 127 of file distributed_storage.h.
◆ ~DistributedStorage()
|
inlinevirtual |
Definition at line 142 of file distributed_storage.h.
Member Function Documentation
◆ get()
|
inline |
Get local or remote element.
- Parameters
-
i The element to get
- Returns
- A future to element
i
- Exceptions
-
TiledArray::Exception If i
is greater than or equal tomax_size()
.
Definition at line 202 of file distributed_storage.h.
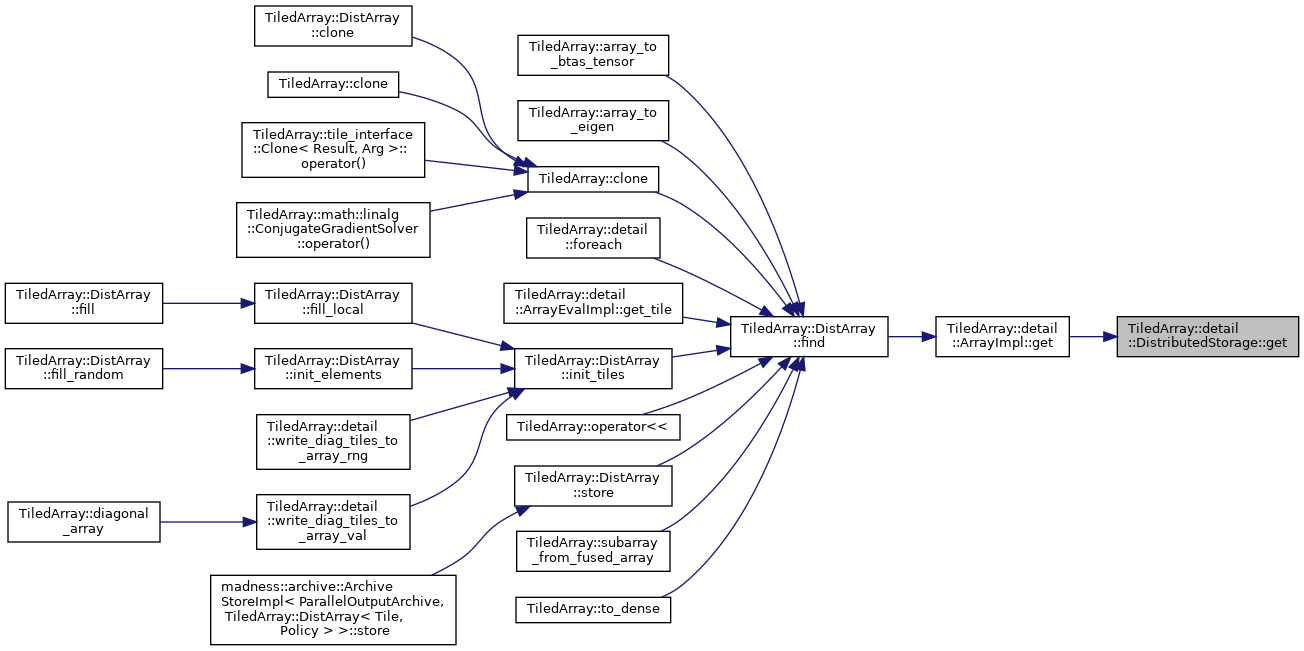
◆ get_local() [1/2]
|
inline |
Get local element.
- Parameters
-
i The element to get
- Returns
- A reference to element
i
- Exceptions
-
TiledArray::Exception If i
is greater than or equal to max_size() ori
is not local.
Definition at line 238 of file distributed_storage.h.
◆ get_local() [2/2]
|
inline |
Get local element.
- Parameters
-
i The element to get
- Returns
- A const reference to element
i
- Exceptions
-
TiledArray::Exception If i
is greater than or equal to max_size() ori
is not local.
Definition at line 223 of file distributed_storage.h.
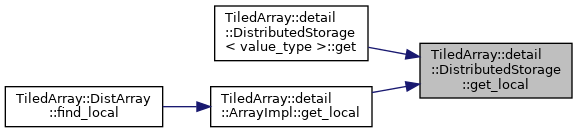
◆ is_local()
|
inline |
Local element query.
Check if element i
belongs to this node. The element may or may not be stored. Use find
to determine if an element is present.
- Parameters
-
i The element to check.
- Returns
true
when the element is stored locally, otherwisefalse
.
Definition at line 175 of file distributed_storage.h.
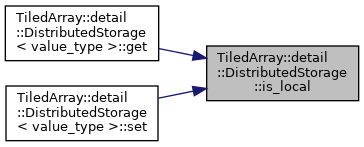
◆ max_size()
|
inline |
Max size accessor.
The maximum size is the total number of elements that can be held by this container on all nodes, not on each node.
- Returns
- The maximum number of elements.
- Exceptions
-
nothing
Definition at line 194 of file distributed_storage.h.

◆ owner()
|
inline |
Element owner.
- Returns
- The process that owns element
i
Definition at line 163 of file distributed_storage.h.

◆ pmap()
|
inline |
Process map accessor.
- Returns
- A shared pointer to the process map.
- Exceptions
-
nothing
Definition at line 158 of file distributed_storage.h.
◆ set() [1/2]
|
inline |
Set element i
with a Future
f
.
The owner of i
may be local or remote. If i
is remote, a task is spawned on the owning node after the local future has been assigned. If i
is not already in the container, it will be inserted.
- Parameters
-
i The element to be set f The future for element i
- Exceptions
-
madness::MadnessException If i
has already been set.TiledArray::Exception If i
is greater than or equal tomax_size()
.
Definition at line 272 of file distributed_storage.h.
◆ set() [2/2]
|
inline |
Set element i
with value
.
- Parameters
-
i The element to be set value The value of element i
- Exceptions
-
TiledArray::Exception If i
is greater than or equal tomax_size()
.madness::MadnessException If i
has already been set.
Definition at line 254 of file distributed_storage.h.
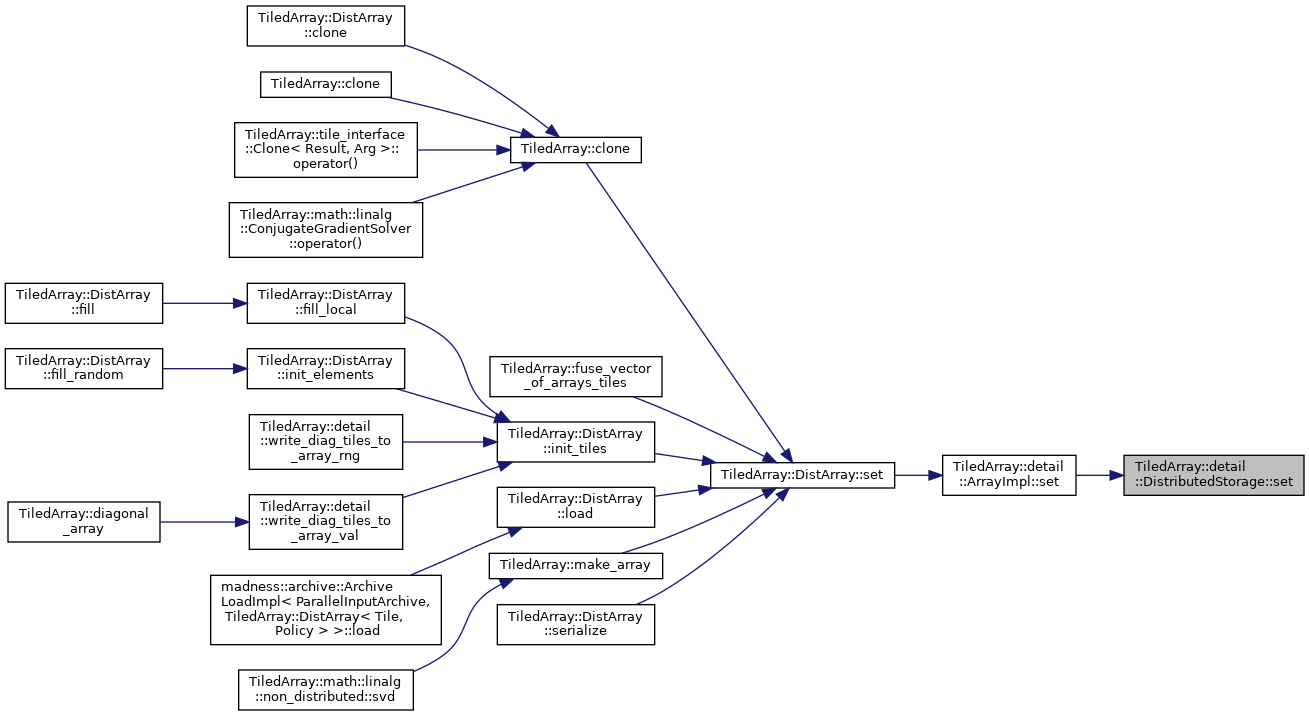
◆ size()
|
inline |
Number of local elements.
No communication.
- Returns
- The number of local elements stored by the container.
- Exceptions
-
nothing
Definition at line 186 of file distributed_storage.h.
Friends And Related Function Documentation
◆ DelayedSet
|
friend |
Definition at line 114 of file distributed_storage.h.
The documentation for this class was generated from the following file:
- TiledArray/distributed_storage.h