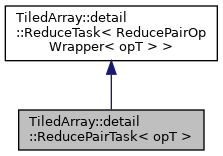
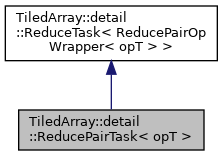
Documentation
template<typename opT>
class TiledArray::detail::ReducePairTask< opT >
Reduce pair task.
This task will reduce an arbitrary number of pairs of objects. This task is optimized for reduction of data that is the result of other tasks or remote data. Also, it is assumed that individual reduction operations require a substantial amount of work (i.e. your reduction operation should reduce a vector of data, not two numbers). The reduction arguments are reduced as they become ready, which results in non- deterministic reduction order. This is much faster than a simple binary tree reduction since the reduction tasks do not have to wait for specific pairs of data. Though data that is not stored in a future can be used, it may not be the best choice in that case.
The reduction operation must have the following form:
For example, a dot product function might look like:
- Note
- There is no need to add this object to the MADNESS task queue. It will be handled internally by the object. Simply call
submit()
to add this task to the task queue.
- Template Parameters
-
opT The reduction operation type
Definition at line 910 of file reduce_task.h.
Public Member Functions | |
ReducePairTask () | |
Default constructor. More... | |
ReducePairTask (World &world, const opT &op=opT(), madness::CallbackInterface *callback=nullptr) | |
Constructor. More... | |
ReducePairTask (ReducePairTask< opT > &&other) noexcept | |
Move constructor. More... | |
ReducePairTask< opT > & | operator= (ReducePairTask< opT > &&other) noexcept |
Move assignment operator. More... | |
ReducePairTask (const ReducePairTask< opT > &)=delete | |
Non-copyable. More... | |
ReducePairTask< opT > | operator= (const ReducePairTask< opT > &)=delete |
template<typename L , typename R > | |
void | add (const L &left, const R &right, madness::CallbackInterface *callback=nullptr) |
Add a pair of arguments to the reduction task. More... | |
![]() | |
ReduceTask () | |
Default constructor. More... | |
ReduceTask (World &world, const ReducePairOpWrapper< opT > &op=ReducePairOpWrapper< opT >(), madness::CallbackInterface *callback=nullptr) | |
Constructor. More... | |
ReduceTask (ReduceTask< ReducePairOpWrapper< opT > > &&other) noexcept | |
Move constructor. More... | |
ReduceTask (const ReduceTask< ReducePairOpWrapper< opT > > &)=delete | |
~ReduceTask () | |
Destructor. More... | |
ReduceTask< ReducePairOpWrapper< opT > > & | operator= (ReduceTask< ReducePairOpWrapper< opT > > &&other) noexcept |
Move assignment operator. More... | |
ReduceTask< ReducePairOpWrapper< opT > > & | operator= (const ReduceTask< ReducePairOpWrapper< opT > > &)=delete |
int | add (const Arg &arg, madness::CallbackInterface *callback=nullptr) |
Add an argument to the reduction task. More... | |
int | count () const |
Argument count. More... | |
Future< result_type > | submit () |
Submit the reduction task to the task queue. More... | |
operator bool () const | |
Type conversion operator. More... | |
Constructor & Destructor Documentation
◆ ReducePairTask() [1/4]
|
inline |
Default constructor.
Definition at line 923 of file reduce_task.h.
◆ ReducePairTask() [2/4]
|
inline |
Constructor.
- Parameters
-
world The world that owns this task op The pair reduction operation [ default = opT() ] callback The callback that will be invoked when this task is complete
Definition at line 931 of file reduce_task.h.
◆ ReducePairTask() [3/4]
|
inlinenoexcept |
Move constructor.
- Parameters
-
other The object to be moved
Definition at line 938 of file reduce_task.h.
◆ ReducePairTask() [4/4]
|
delete |
Non-copyable.
Member Function Documentation
◆ add()
|
inline |
Add a pair of arguments to the reduction task.
left
and right
may be of the argument types of opT
, a Future
to the argument types, RemoteReference<FutureImpl>
to the argument types, or any combination of the above.
- Template Parameters
-
L The left-hand object type R The right-hand object type
- Parameters
-
left The left-hand argument that will be reduced right The right-hand argument that will be reduced callback The callback that will be invoked when this argument pair has been reduced [ default = nullptr ]
Definition at line 966 of file reduce_task.h.

◆ operator=() [1/2]
|
delete |
◆ operator=() [2/2]
|
inlinenoexcept |
Move assignment operator.
- Parameters
-
other The object to be moved
Definition at line 944 of file reduce_task.h.
The documentation for this class was generated from the following file:
- TiledArray/reduce_task.h