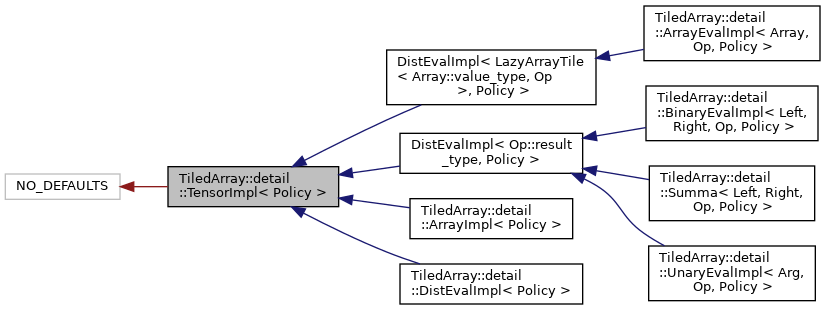
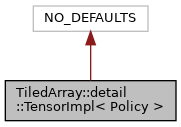
Documentation
template<typename Policy>
class TiledArray::detail::TensorImpl< Policy >
Tensor implementation and base for other tensor implementation objects.
This implementation object holds the meta data for tensor object, which includes tiled range, shape, and process map.
- Note
- The process map must be set before data elements can be set.
- It is the users responsibility to ensure the process maps on all nodes are identical.
Definition at line 39 of file tensor_impl.h.
Public Types | |
typedef TensorImpl< Policy > | TensorImpl_ |
typedef Policy | policy_type |
Policy type. More... | |
typedef Policy::trange_type | trange_type |
Tiled range type. More... | |
typedef Policy::range_type | range_type |
Element/tile range type. More... | |
typedef Policy::index1_type | index1_type |
1-index type More... | |
typedef Policy::ordinal_type | ordinal_type |
Ordinal type. More... | |
typedef Policy::shape_type | shape_type |
Tensor shape type. More... | |
typedef Policy::pmap_interface | pmap_interface |
Process map interface type. More... | |
Public Member Functions | |
TensorImpl (World &world, const trange_type &trange, const shape_type &shape, const std::shared_ptr< pmap_interface > &pmap) | |
Constructor. More... | |
virtual | ~TensorImpl () |
Virtual destructor. More... | |
const std::shared_ptr< pmap_interface > & | pmap () const |
Tensor process map accessor. More... | |
const range_type & | tiles_range () const |
Tiles range accessor. More... | |
ordinal_type | size () const |
Tensor tile volume accessor. More... | |
ordinal_type | local_size () const |
Local element count. More... | |
template<typename Index > | |
ProcessID | owner (const Index &i) const |
Query a tile owner. More... | |
template<typename Index > | |
bool | is_local (const Index &i) const |
Query for a locally owned tile. More... | |
template<typename Index > | |
bool | is_zero (const Index &i) const |
Query for a zero tile. More... | |
bool | is_dense () const |
Query the density of the tensor. More... | |
const shape_type & | shape () const |
Tensor shape accessor. More... | |
const trange_type & | trange () const |
Tiled range accessor. More... | |
World & | get_world () const |
World & | world () const |
World accessor. More... | |
Member Typedef Documentation
◆ index1_type
typedef Policy::index1_type TiledArray::detail::TensorImpl< Policy >::index1_type |
1-index type
Definition at line 45 of file tensor_impl.h.
◆ ordinal_type
typedef Policy::ordinal_type TiledArray::detail::TensorImpl< Policy >::ordinal_type |
Ordinal type.
Definition at line 46 of file tensor_impl.h.
◆ pmap_interface
typedef Policy::pmap_interface TiledArray::detail::TensorImpl< Policy >::pmap_interface |
Process map interface type.
Definition at line 49 of file tensor_impl.h.
◆ policy_type
typedef Policy TiledArray::detail::TensorImpl< Policy >::policy_type |
Policy type.
Definition at line 42 of file tensor_impl.h.
◆ range_type
typedef Policy::range_type TiledArray::detail::TensorImpl< Policy >::range_type |
Element/tile range type.
Definition at line 44 of file tensor_impl.h.
◆ shape_type
typedef Policy::shape_type TiledArray::detail::TensorImpl< Policy >::shape_type |
Tensor shape type.
Definition at line 47 of file tensor_impl.h.
◆ TensorImpl_
typedef TensorImpl<Policy> TiledArray::detail::TensorImpl< Policy >::TensorImpl_ |
Definition at line 41 of file tensor_impl.h.
◆ trange_type
typedef Policy::trange_type TiledArray::detail::TensorImpl< Policy >::trange_type |
Tiled range type.
Definition at line 43 of file tensor_impl.h.
Constructor & Destructor Documentation
◆ TensorImpl()
|
inline |
Constructor.
The size of shape must be equal to the volume of the tiled range tiles.
- Parameters
-
world The world where this tensor will live trange The tiled range for this tensor shape The shape of this tensor pmap The tile-process map
- Exceptions
-
TiledArray::Exception When the size of shape is not equal to zero
Definition at line 67 of file tensor_impl.h.
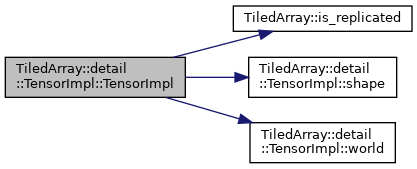
◆ ~TensorImpl()
|
inlinevirtual |
Virtual destructor.
Definition at line 83 of file tensor_impl.h.
Member Function Documentation
◆ get_world()
|
inline |
Definition at line 170 of file tensor_impl.h.
◆ is_dense()
|
inline |
Query the density of the tensor.
- Returns
true
if the tensor is dense, otherwise false
- Exceptions
-
nothing
Definition at line 156 of file tensor_impl.h.

◆ is_local()
|
inline |
Query for a locally owned tile.
- Template Parameters
-
Index The index type
- Parameters
-
i The tile index to query
- Returns
true
if the tile is owned by this node, otherwisefalse
- Exceptions
-
TiledArray::Exception When the process map has not been set
Definition at line 134 of file tensor_impl.h.
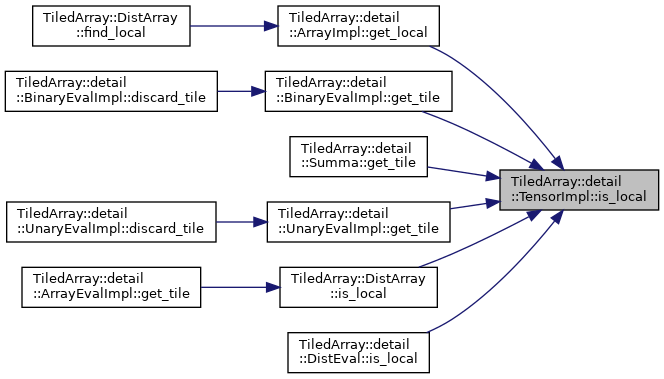
◆ is_zero()
|
inline |
Query for a zero tile.
- Template Parameters
-
Index The index type
- Parameters
-
i The tile index to query
- Returns
true
if the tile is zero, otherwisefalse
- Exceptions
-
TiledArray::Exception When i
is outside the tiled range tile range
Definition at line 147 of file tensor_impl.h.
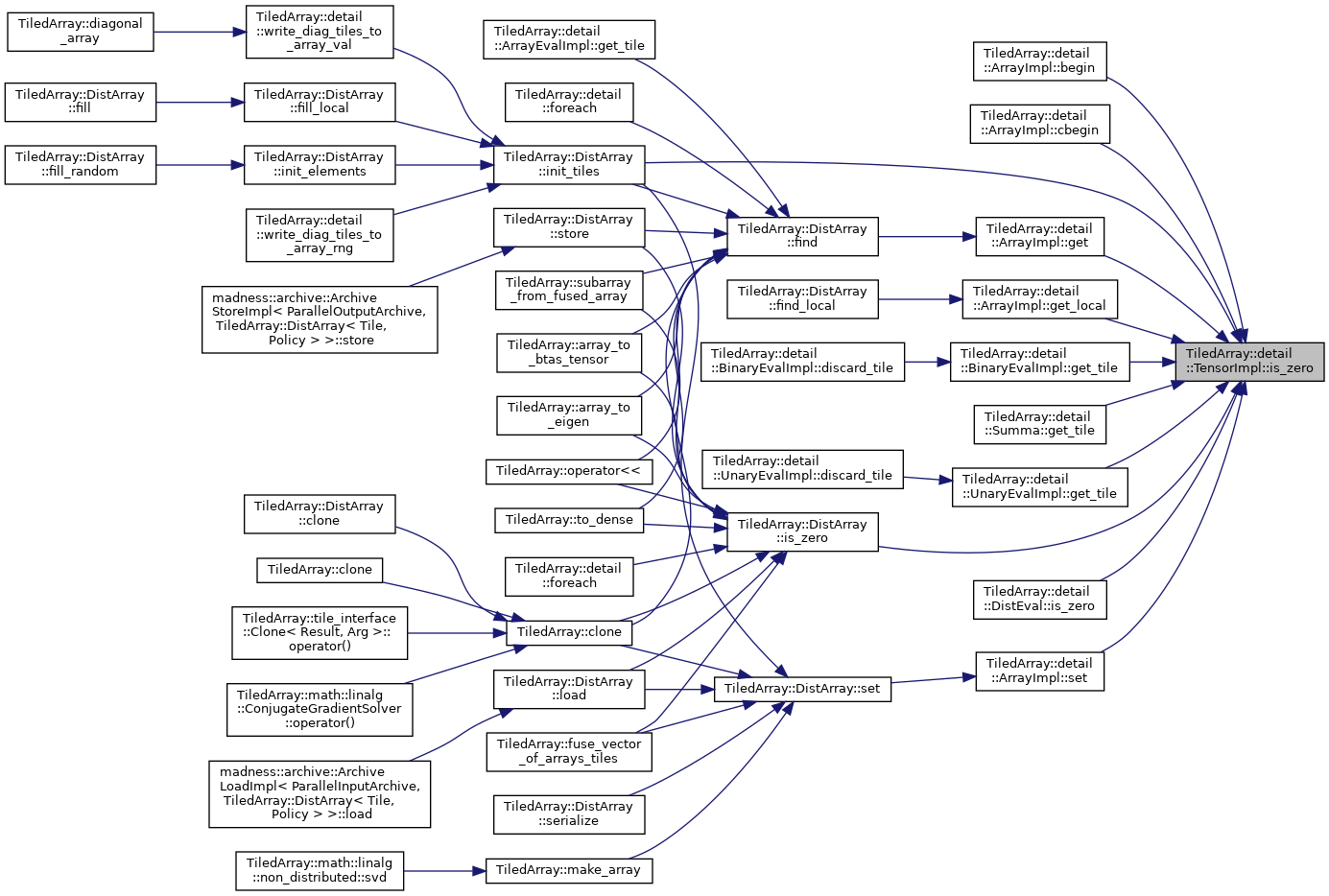
◆ local_size()
|
inline |
Local element count.
This function is primarily available for debugging purposes. The returned value is volatile and may change at any time; you should not rely on it in your algorithms.
- Returns
- The current number of local tiles stored in the tensor.
Definition at line 109 of file tensor_impl.h.
◆ owner()
|
inline |
Query a tile owner.
- Template Parameters
-
Index The index type
- Parameters
-
i The tile index to query
- Returns
- The process ID of the node that owns tile
i
- Exceptions
-
TiledArray::Exception When i
is outside the tiled range tile rangeTiledArray::Exception When the process map has not been set
Definition at line 122 of file tensor_impl.h.
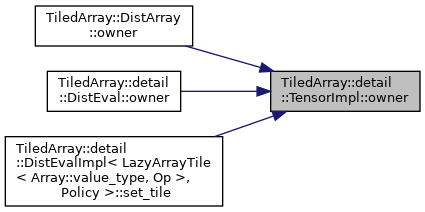
◆ pmap()
|
inline |
Tensor process map accessor.
- Returns
- A shared pointer to the process map of this tensor
- Exceptions
-
nothing
Definition at line 89 of file tensor_impl.h.
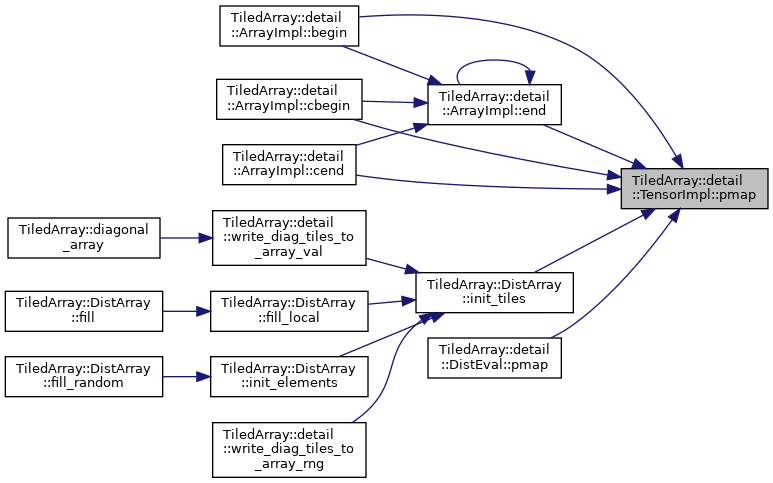
◆ shape()
|
inline |
Tensor shape accessor.
- Returns
- A reference to the tensor shape map
- Exceptions
-
TiledArray::Exception When this tensor is dense
Definition at line 162 of file tensor_impl.h.
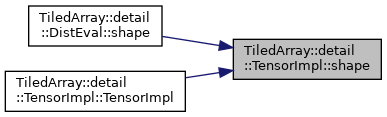
◆ size()
|
inline |
Tensor tile volume accessor.
- Returns
- The number of tiles in the tensor
- Exceptions
-
nothing
Definition at line 101 of file tensor_impl.h.

◆ tiles_range()
|
inline |
Tiles range accessor.
- Returns
- The range of tile indices
- Exceptions
-
nothing
Definition at line 95 of file tensor_impl.h.
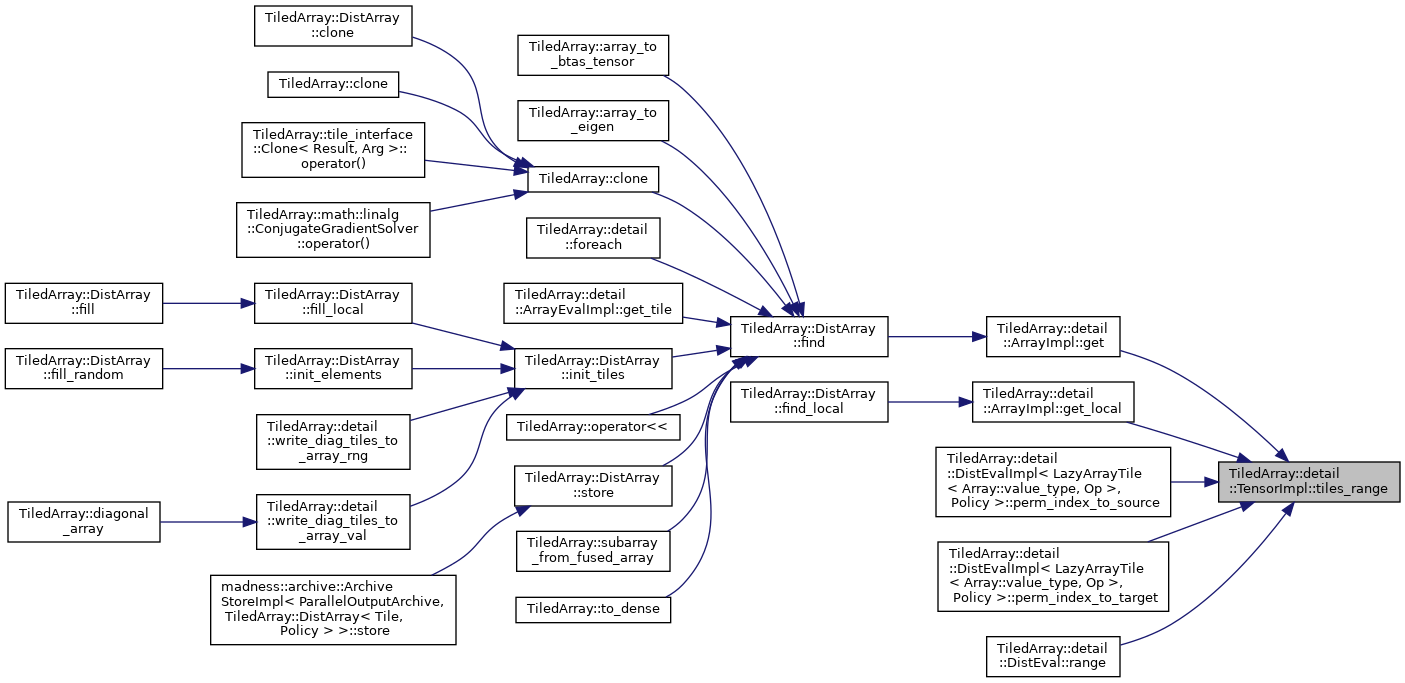
◆ trange()
|
inline |
Tiled range accessor.
- Returns
- The tiled range of the tensor
Definition at line 167 of file tensor_impl.h.
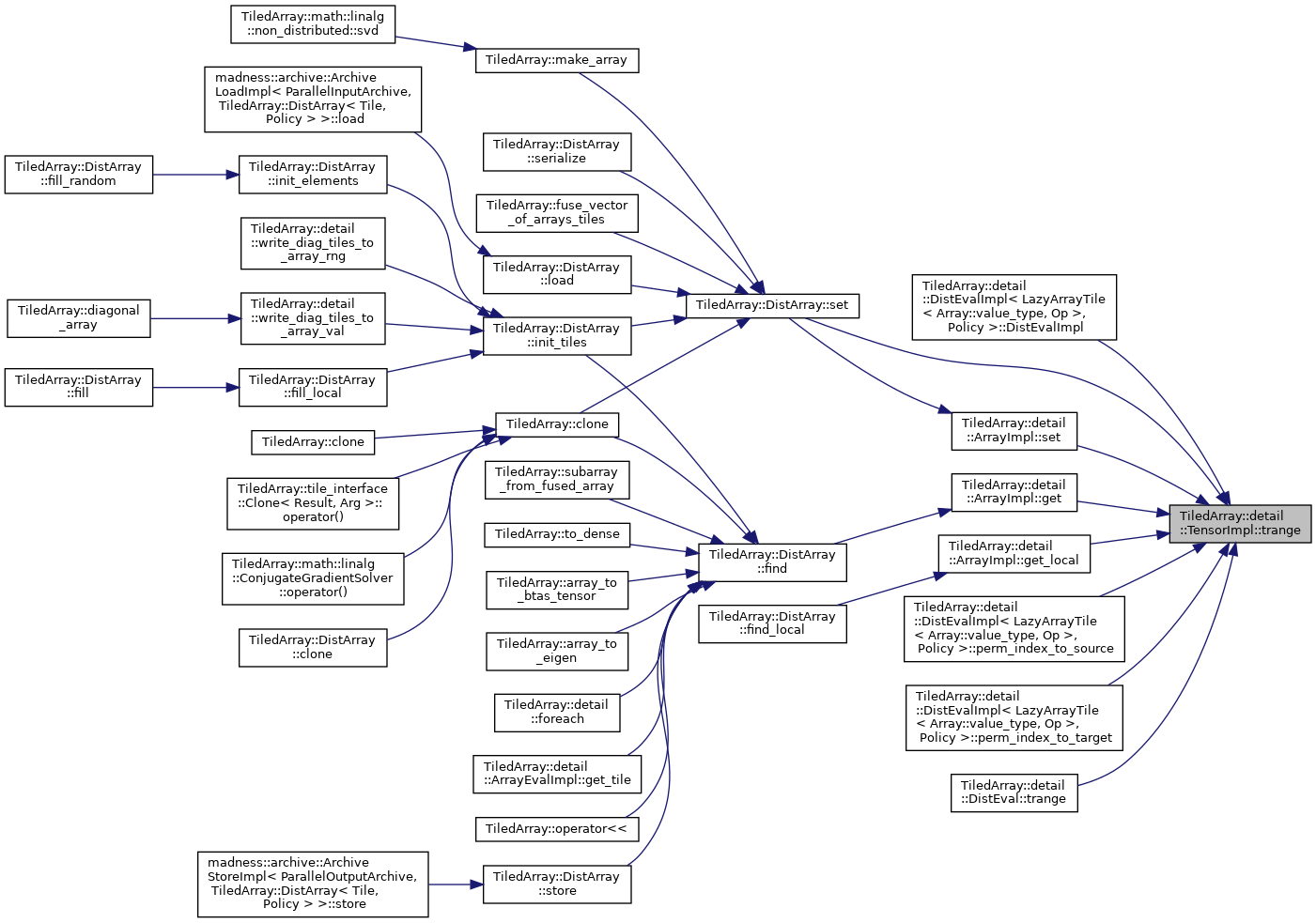
◆ world()
|
inline |
World accessor.
- Returns
- A reference to the world that contains this tensor
Definition at line 175 of file tensor_impl.h.
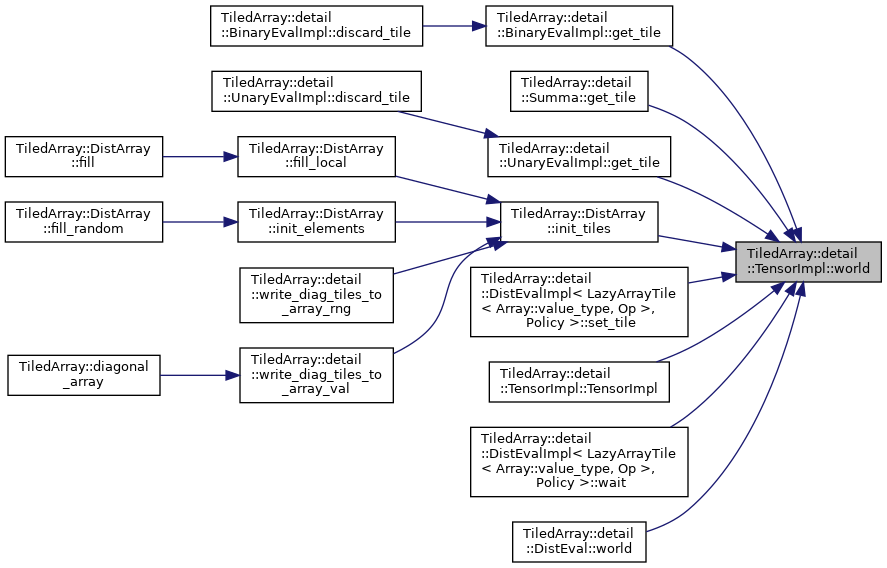
The documentation for this class was generated from the following file:
- TiledArray/tensor_impl.h