Search Results
Documentation
template<typename Left, typename Right, typename Scalar, typename Result>
class TiledArray::expressions::ScalMultEngine< Left, Right, Scalar, Result >
Scaled multiplication expression engine.
Similar to MultEngine but implements the result of MultEngine scaled by a constant.
Definition at line 474 of file mult_engine.h.
Public Member Functions | |
template<typename L , typename R , typename S > | |
ScalMultEngine (const ScalMultExpr< L, R, S > &expr) | |
Constructor. More... | |
void perm_indices (const BipartiteIndexList &target_indices) | |
Set the index list for this expression. More... | |
void init_indices (const BipartiteIndexList &target_indices) | |
Initialize the index list of this expression. More... | |
void init_indices () | |
Initialize the index list of this expression. More... | |
void init_struct (const BipartiteIndexList &target_indices) | |
Initialize result tensor structure. More... | |
void init_distribution (World *world, std::shared_ptr< pmap_interface > pmap) | |
Initialize result tensor distribution. More... | |
dist_eval_type make_dist_eval () const | |
Construct the distributed evaluator for this expression. More... | |
trange_type make_trange () const | |
Non-permuting tiled range factory function. More... | |
trange_type make_trange (const Permutation &perm) const | |
Permuting tiled range factory function. More... | |
shape_type make_shape () const | |
Non-permuting shape factory function. More... | |
shape_type make_shape (const Permutation &perm) const | |
Permuting shape factory function. More... | |
op_type make_tile_op () const | |
Non-permuting tile operation factory function. More... | |
template<typename Perm , typename = std::enable_if_t< TiledArray::detail::is_permutation_v<Perm>>> | |
op_type make_tile_op (const Perm &perm) const | |
Permuting tile operation factory function. More... | |
std::string make_tag () const | |
Expression identification tag. More... | |
void print (ExprOStream os, const BipartiteIndexList &target_indices) const | |
Expression print. More... | |
Member Typedef Documentation
◆ BinaryEngine_
typedef BinaryEngine<ScalMultEngine_> TiledArray::expressions::ScalMultEngine< Left, Right, Scalar, Result >::BinaryEngine_ |
Binary base class type.
Definition at line 483 of file mult_engine.h.
◆ ContEngine_
typedef ContEngine<ScalMultEngine_> TiledArray::expressions::ScalMultEngine< Left, Right, Scalar, Result >::ContEngine_ |
Contraction engine base class.
Definition at line 481 of file mult_engine.h.
◆ dist_eval_type
typedef EngineTrait<ScalMultEngine_>::dist_eval_type TiledArray::expressions::ScalMultEngine< Left, Right, Scalar, Result >::dist_eval_type |
The distributed evaluator type.
Definition at line 505 of file mult_engine.h.
◆ ExprEngine_
typedef BinaryEngine<ScalMultEngine_> TiledArray::expressions::ScalMultEngine< Left, Right, Scalar, Result >::ExprEngine_ |
Expression engine base class type.
Definition at line 485 of file mult_engine.h.
◆ left_type
typedef EngineTrait<ScalMultEngine_>::left_type TiledArray::expressions::ScalMultEngine< Left, Right, Scalar, Result >::left_type |
The left-hand expression type.
Definition at line 489 of file mult_engine.h.
◆ op_base_type
typedef EngineTrait<ScalMultEngine_>::op_base_type TiledArray::expressions::ScalMultEngine< Left, Right, Scalar, Result >::op_base_type |
The tile operation type.
Definition at line 499 of file mult_engine.h.
◆ op_type
typedef EngineTrait<ScalMultEngine_>::op_type TiledArray::expressions::ScalMultEngine< Left, Right, Scalar, Result >::op_type |
The tile operation type.
Definition at line 501 of file mult_engine.h.
◆ pmap_interface
typedef EngineTrait<ScalMultEngine_>::pmap_interface TiledArray::expressions::ScalMultEngine< Left, Right, Scalar, Result >::pmap_interface |
Process map interface type.
Definition at line 515 of file mult_engine.h.
◆ policy
typedef EngineTrait<ScalMultEngine_>::policy TiledArray::expressions::ScalMultEngine< Left, Right, Scalar, Result >::policy |
The result policy type.
Definition at line 503 of file mult_engine.h.
◆ right_type
typedef EngineTrait<ScalMultEngine_>::right_type TiledArray::expressions::ScalMultEngine< Left, Right, Scalar, Result >::right_type |
The right-hand expression type.
Definition at line 491 of file mult_engine.h.
◆ scalar_type
typedef EngineTrait<ScalMultEngine_>::scalar_type TiledArray::expressions::ScalMultEngine< Left, Right, Scalar, Result >::scalar_type |
Tile scalar type.
Definition at line 497 of file mult_engine.h.
◆ ScalMultEngine_
typedef ScalMultEngine<Left, Right, Scalar, Result> TiledArray::expressions::ScalMultEngine< Left, Right, Scalar, Result >::ScalMultEngine_ |
This class type.
Definition at line 479 of file mult_engine.h.
◆ shape_type
typedef EngineTrait<ScalMultEngine_>::shape_type TiledArray::expressions::ScalMultEngine< Left, Right, Scalar, Result >::shape_type |
Shape type.
Definition at line 513 of file mult_engine.h.
◆ size_type
typedef EngineTrait<ScalMultEngine_>::size_type TiledArray::expressions::ScalMultEngine< Left, Right, Scalar, Result >::size_type |
Size type.
Definition at line 509 of file mult_engine.h.
◆ trange_type
typedef EngineTrait<ScalMultEngine_>::trange_type TiledArray::expressions::ScalMultEngine< Left, Right, Scalar, Result >::trange_type |
Tiled range type.
Definition at line 511 of file mult_engine.h.
◆ value_type
typedef EngineTrait<ScalMultEngine_>::value_type TiledArray::expressions::ScalMultEngine< Left, Right, Scalar, Result >::value_type |
The result tile type.
Definition at line 495 of file mult_engine.h.
Constructor & Destructor Documentation
◆ ScalMultEngine()
|
inline |
Constructor.
- Template Parameters
-
L The left-hand argument expression type R The right-hand argument expression type S The expression scalar type
- Parameters
-
expr The parent expression
Definition at line 525 of file mult_engine.h.
Member Function Documentation
◆ init_distribution()
|
inline |
Initialize result tensor distribution.
This function will initialize the world and process map for the result tensor.
- Parameters
-
world The world were the result will be distributed pmap The process map for the result tensor tiles
Definition at line 602 of file mult_engine.h.
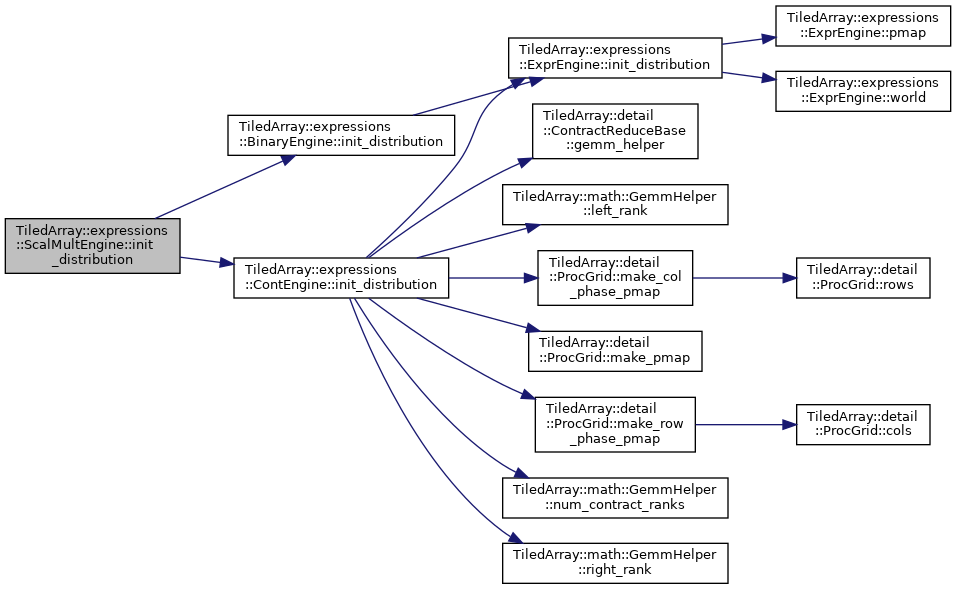
◆ init_indices() [1/2]
|
inline |
Initialize the index list of this expression.
Definition at line 562 of file mult_engine.h.
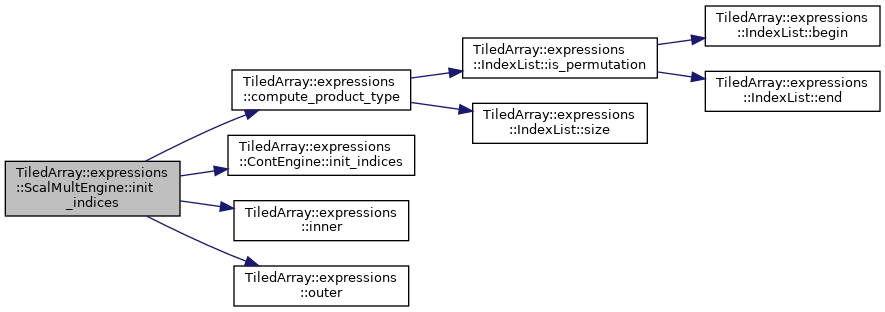
◆ init_indices() [2/2]
|
inline |
Initialize the index list of this expression.
- Parameters
-
target_indices The target index list for this expression
Definition at line 545 of file mult_engine.h.
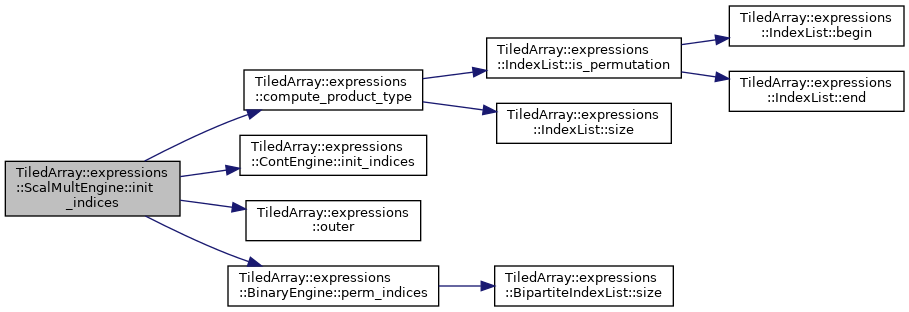
◆ init_struct()
|
inline |
Initialize result tensor structure.
This function will initialize the permutation, tiled range, and shape for the result tensor.
- Parameters
-
target_indices The target index list for the result tensor
Definition at line 588 of file mult_engine.h.
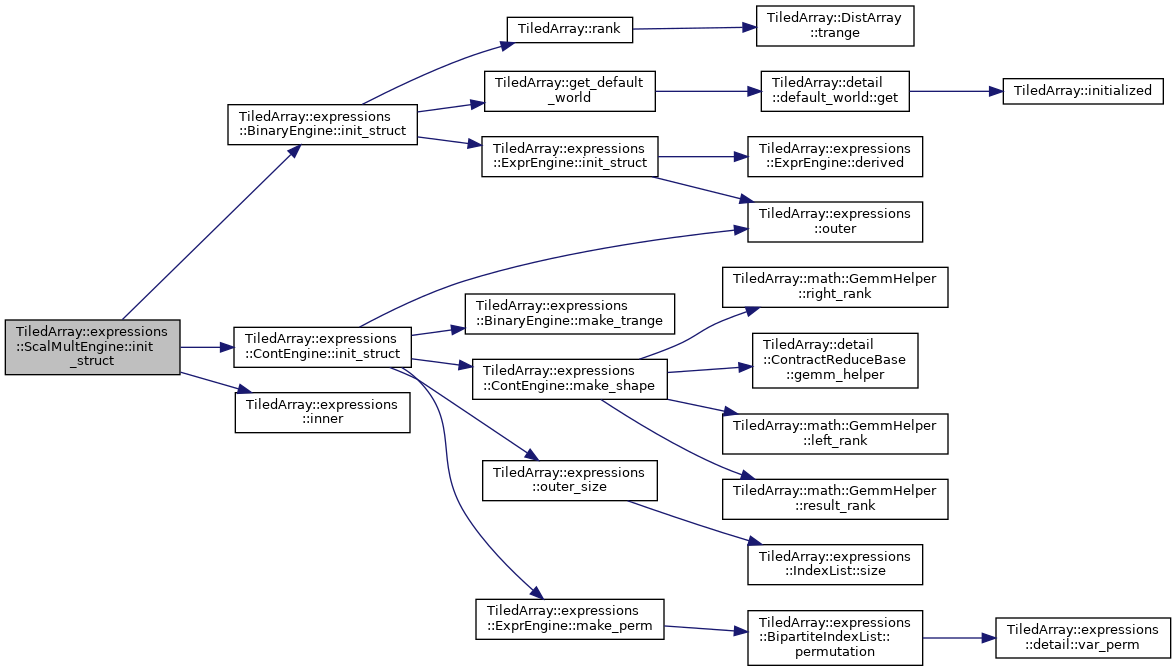
◆ make_dist_eval()
|
inline |
Construct the distributed evaluator for this expression.
- Returns
- The distributed evaluator that will evaluate this expression
Definition at line 612 of file mult_engine.h.
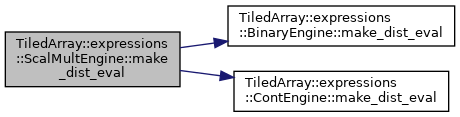
◆ make_shape() [1/2]
|
inline |
Non-permuting shape factory function.
- Returns
- The result shape
Definition at line 643 of file mult_engine.h.
◆ make_shape() [2/2]
|
inline |
Permuting shape factory function.
- Parameters
-
perm The permutation to be applied to the array
- Returns
- The result shape
Definition at line 652 of file mult_engine.h.
◆ make_tag()
|
inline |
Expression identification tag.
- Returns
- An expression tag used to identify this expression
Definition at line 677 of file mult_engine.h.
◆ make_tile_op() [1/2]
|
inline |
Non-permuting tile operation factory function.
- Returns
- The tile operation
Definition at line 660 of file mult_engine.h.
◆ make_tile_op() [2/2]
|
inline |
Permuting tile operation factory function.
- Parameters
-
perm The permutation to be applied to tiles
- Returns
- The tile operation
Definition at line 670 of file mult_engine.h.
◆ make_trange() [1/2]
|
inline |
Non-permuting tiled range factory function.
- Returns
- The result tiled range object
Definition at line 622 of file mult_engine.h.

◆ make_trange() [2/2]
|
inline |
Permuting tiled range factory function.
- Parameters
-
perm The permutation to be applied to the array
- Returns
- The result tiled range object
Definition at line 633 of file mult_engine.h.

◆ perm_indices()
|
inline |
Set the index list for this expression.
This function will set the index list for this expression and its children such that the number of permutations is minimized. The final index list may not be set to target, which indicates that the result of this expression will be permuted to match target_indices
.
- Parameters
-
target_indices The target index list for this expression
Definition at line 534 of file mult_engine.h.

◆ print()
|
inline |
Expression print.
- Parameters
-
os The output stream target_indices The target index list for this expression
Definition at line 687 of file mult_engine.h.
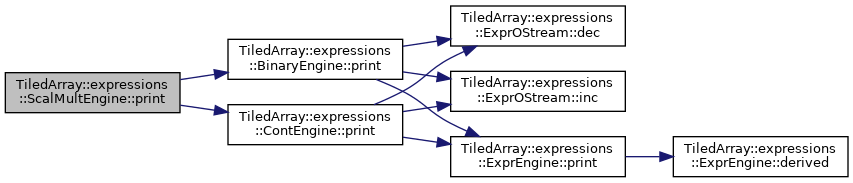
The documentation for this class was generated from the following file:
- TiledArray/expressions/mult_engine.h