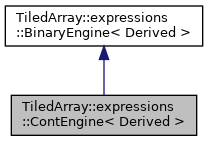
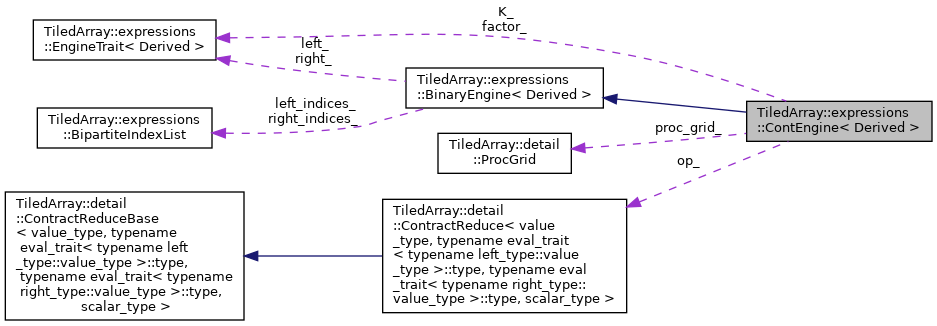
Documentation
template<typename Derived>
class TiledArray::expressions::ContEngine< Derived >
Multiplication expression engine.
- Template Parameters
-
Derived The derived engine type
Definition at line 50 of file cont_engine.h.
Public Types | |
typedef ContEngine< Derived > | ContEngine_ |
This class type. More... | |
typedef BinaryEngine< Derived > | BinaryEngine_ |
Binary base class type. More... | |
typedef ExprEngine< Derived > | ExprEngine_ |
Expression engine base class type. More... | |
typedef EngineTrait< Derived >::left_type | left_type |
The left-hand expression type. More... | |
typedef EngineTrait< Derived >::right_type | right_type |
The right-hand expression type. More... | |
typedef EngineTrait< Derived >::value_type | value_type |
The result tile type. More... | |
typedef EngineTrait< Derived >::scalar_type | scalar_type |
Tile scalar type. More... | |
typedef TiledArray::detail::ContractReduce< value_type, typename eval_trait< typename left_type::value_type >::type, typename eval_trait< typename right_type::value_type >::type, scalar_type > | op_type |
The tile operation type. More... | |
typedef EngineTrait< Derived >::policy | policy |
The result policy type. More... | |
typedef EngineTrait< Derived >::dist_eval_type | dist_eval_type |
The distributed evaluator type. More... | |
typedef EngineTrait< Derived >::size_type | size_type |
Size type. More... | |
typedef EngineTrait< Derived >::trange_type | trange_type |
Tiled range type. More... | |
typedef EngineTrait< Derived >::shape_type | shape_type |
Shape type. More... | |
typedef EngineTrait< Derived >::pmap_interface | pmap_interface |
Process map interface type. More... | |
![]() | |
typedef BinaryEngine< Derived > | BinaryEngine_ |
This class type. More... | |
typedef ExprEngine< Derived > | ExprEngine_ |
Base class type. More... | |
typedef EngineTrait< Derived >::left_type | left_type |
The left-hand expression type. More... | |
typedef EngineTrait< Derived >::right_type | right_type |
The right-hand expression type. More... | |
typedef EngineTrait< Derived >::value_type | value_type |
The result tile type. More... | |
typedef EngineTrait< Derived >::op_type | op_type |
The tile operation type. More... | |
typedef EngineTrait< Derived >::policy | policy |
The result policy type. More... | |
typedef EngineTrait< Derived >::dist_eval_type | dist_eval_type |
The distributed evaluator type. More... | |
typedef EngineTrait< Derived >::size_type | size_type |
Size type. More... | |
typedef EngineTrait< Derived >::trange_type | trange_type |
Tiled range type. More... | |
typedef EngineTrait< Derived >::shape_type | shape_type |
Shape type. More... | |
typedef EngineTrait< Derived >::pmap_interface | pmap_interface |
Process map interface type. More... | |
Public Member Functions | |
template<typename L , typename R > | |
ContEngine (const MultExpr< L, R > &expr) | |
Constructor. More... | |
template<typename L , typename R , typename S > | |
ContEngine (const ScalMultExpr< L, R, S > &expr) | |
Constructor. More... | |
void | perm_indices (const BipartiteIndexList &target_indices) |
Set the index list for this expression. More... | |
void | init_indices (bool children_initialized=false) |
Initialize the index list of this expression. More... | |
void | init_indices (const BipartiteIndexList &target_indices) |
Initialize the index list of this expression. More... | |
void | init_struct (const BipartiteIndexList &target_indices) |
Initialize result tensor structure. More... | |
void | init_distribution (World *world, std::shared_ptr< pmap_interface > pmap) |
Initialize result tensor distribution. More... | |
trange_type | make_trange (const Permutation &perm={}) const |
Tiled range factory function. More... | |
shape_type | make_shape () const |
Non-permuting shape factory function. More... | |
shape_type | make_shape (const Permutation &perm) const |
Permuting shape factory function. More... | |
dist_eval_type | make_dist_eval () const |
std::string | make_tag () const |
Expression identification tag. More... | |
void | print (ExprOStream os, const BipartiteIndexList &target_indices) const |
Expression print. More... | |
derived_type & | derived () |
Cast this object to its derived type. More... | |
const derived_type & | derived () const |
Cast this object to its derived type. More... | |
const BipartiteIndexList & | indices () const |
Index list accessor. More... | |
![]() | |
template<typename D > | |
BinaryEngine (const BinaryExpr< D > &expr) | |
void | perm_indices (const BipartiteIndexList &target_indices) |
Set the index list for this expression. More... | |
void | init_indices (const BipartiteIndexList &target_indices) |
Initialize the index list of this expression. More... | |
void | init_indices (bool children_initialized=false) |
Initialize the index list of this expression. More... | |
void | init_struct (const BipartiteIndexList &target_indices) |
Initialize result tensor structure. More... | |
void | init_distribution (World *world, const std::shared_ptr< pmap_interface > &pmap) |
Initialize result tensor distribution. More... | |
trange_type | make_trange () const |
Non-permuting tiled range factory function. More... | |
trange_type | make_trange (const Permutation &perm) const |
Permuting tiled range factory function. More... | |
dist_eval_type | make_dist_eval () const |
Construct the distributed evaluator for this expression. More... | |
void | print (ExprOStream os, const BipartiteIndexList &target_indices) const |
Expression print. More... | |
Protected Types | |
using | tile_element_type = typename value_type::value_type |
Protected Member Functions | |
TensorProduct | product_type () const |
TensorProduct | inner_product_type () const |
void | init_inner_tile_op (const IndexList &inner_target_indices) |
![]() | |
template<TensorProduct ProductType> | |
void | init_indices_ (const BipartiteIndexList &target_indices={}) |
Static Protected Member Functions | |
static unsigned int | find (const BipartiteIndexList &indices, const std::string &index_label, unsigned int i, const unsigned int n) |
Protected Attributes | |
scalar_type | factor_ |
Contraction scaling factor. More... | |
op_type | op_ |
Tile operation. More... | |
std::function< void(tile_element_type &, const tile_element_type &, const tile_element_type &)> | inner_tile_nonreturn_op_ |
std::function< tile_element_type(const tile_element_type &, const tile_element_type &)> | inner_tile_return_op_ |
TiledArray::detail::ProcGrid | proc_grid_ |
Process grid for the contraction. More... | |
size_type | K_ = 1 |
Inner dimension size. More... | |
TensorProduct | product_type_ = TensorProduct::Invalid |
TensorProduct | inner_product_type_ = TensorProduct::Invalid |
left_type | left_ |
The left-hand argument. More... | |
BipartiteIndexList | left_indices_ |
Target left-hand index list. More... | |
PermutationType | left_inner_permtype_ |
Left-hand permutation type. More... | |
PermutationType | left_outer_permtype_ |
Left-hand permutation type. More... | |
right_type | right_ |
The right-hand argument. More... | |
BipartiteIndexList | right_indices_ |
Target right-hand index list. More... | |
PermutationType | right_inner_permtype_ |
Right-hand permutation type. More... | |
PermutationType | right_outer_permtype_ |
Right-hand permutation type. More... | |
BipartiteIndexList | indices_ |
BipartitePermutation | perm_ |
The permutation that will be applied to the outer tensor of tensors. More... | |
bool | permute_tiles_ |
std::shared_ptr< pmap_interface > | pmap_ |
The process map for the result tensor. More... | |
shape_type | shape_ |
The shape of the result tensor. More... | |
trange_type | trange_ |
The tiled range of the result tensor. More... | |
World * | world_ |
The world where this expression will be evaluated. More... | |
![]() | |
left_type | left_ |
The left-hand argument. More... | |
right_type | right_ |
The right-hand argument. More... | |
BipartiteIndexList | left_indices_ |
Target left-hand index list. More... | |
BipartiteIndexList | right_indices_ |
Target right-hand index list. More... | |
PermutationType | left_outer_permtype_ |
Left-hand permutation type. More... | |
PermutationType | right_outer_permtype_ |
Right-hand permutation type. More... | |
PermutationType | left_inner_permtype_ |
Left-hand permutation type. More... | |
PermutationType | right_inner_permtype_ |
Right-hand permutation type. More... | |
BipartiteIndexList | indices_ |
BipartitePermutation | perm_ |
The permutation that will be applied to the outer tensor of tensors. More... | |
bool | permute_tiles_ |
std::shared_ptr< pmap_interface > | pmap_ |
The process map for the result tensor. More... | |
shape_type | shape_ |
The shape of the result tensor. More... | |
trange_type | trange_ |
The tiled range of the result tensor. More... | |
World * | world_ |
The world where this expression will be evaluated. More... | |
Additional Inherited Members | |
![]() | |
static constexpr bool | consumable = EngineTrait<Derived>::consumable |
static constexpr unsigned int | leaves = EngineTrait<Derived>::leaves |
Member Typedef Documentation
◆ BinaryEngine_
typedef BinaryEngine<Derived> TiledArray::expressions::ContEngine< Derived >::BinaryEngine_ |
Binary base class type.
Definition at line 54 of file cont_engine.h.
◆ ContEngine_
typedef ContEngine<Derived> TiledArray::expressions::ContEngine< Derived >::ContEngine_ |
This class type.
Definition at line 53 of file cont_engine.h.
◆ dist_eval_type
typedef EngineTrait<Derived>::dist_eval_type TiledArray::expressions::ContEngine< Derived >::dist_eval_type |
The distributed evaluator type.
Definition at line 77 of file cont_engine.h.
◆ ExprEngine_
typedef ExprEngine<Derived> TiledArray::expressions::ContEngine< Derived >::ExprEngine_ |
Expression engine base class type.
Definition at line 56 of file cont_engine.h.
◆ left_type
typedef EngineTrait<Derived>::left_type TiledArray::expressions::ContEngine< Derived >::left_type |
The left-hand expression type.
Definition at line 60 of file cont_engine.h.
◆ op_type
typedef TiledArray::detail::ContractReduce< value_type, typename eval_trait<typename left_type::value_type>::type, typename eval_trait<typename right_type::value_type>::type, scalar_type> TiledArray::expressions::ContEngine< Derived >::op_type |
The tile operation type.
Definition at line 73 of file cont_engine.h.
◆ pmap_interface
typedef EngineTrait<Derived>::pmap_interface TiledArray::expressions::ContEngine< Derived >::pmap_interface |
Process map interface type.
Definition at line 85 of file cont_engine.h.
◆ policy
typedef EngineTrait<Derived>::policy TiledArray::expressions::ContEngine< Derived >::policy |
The result policy type.
Definition at line 75 of file cont_engine.h.
◆ right_type
typedef EngineTrait<Derived>::right_type TiledArray::expressions::ContEngine< Derived >::right_type |
The right-hand expression type.
Definition at line 62 of file cont_engine.h.
◆ scalar_type
typedef EngineTrait<Derived>::scalar_type TiledArray::expressions::ContEngine< Derived >::scalar_type |
Tile scalar type.
Definition at line 68 of file cont_engine.h.
◆ shape_type
typedef EngineTrait<Derived>::shape_type TiledArray::expressions::ContEngine< Derived >::shape_type |
Shape type.
Definition at line 83 of file cont_engine.h.
◆ size_type
typedef EngineTrait<Derived>::size_type TiledArray::expressions::ContEngine< Derived >::size_type |
Size type.
Definition at line 80 of file cont_engine.h.
◆ tile_element_type
|
protected |
Definition at line 110 of file cont_engine.h.
◆ trange_type
typedef EngineTrait<Derived>::trange_type TiledArray::expressions::ContEngine< Derived >::trange_type |
Tiled range type.
Definition at line 82 of file cont_engine.h.
◆ value_type
typedef EngineTrait<Derived>::value_type TiledArray::expressions::ContEngine< Derived >::value_type |
The result tile type.
Definition at line 66 of file cont_engine.h.
Constructor & Destructor Documentation
◆ ContEngine() [1/2]
|
inline |
Constructor.
- Template Parameters
-
L The left-hand argument expression type R The right-hand argument expression type
- Parameters
-
expr The parent expression
Definition at line 163 of file cont_engine.h.
◆ ContEngine() [2/2]
|
inline |
Constructor.
- Template Parameters
-
L The left-hand argument expression type R The right-hand argument expression type S The expression scalar type
- Parameters
-
expr The parent expression
Definition at line 172 of file cont_engine.h.
Member Function Documentation
◆ derived() [1/2]
|
inline |
Cast this object to its derived type.
Definition at line 209 of file expr_engine.h.
◆ derived() [2/2]
|
inline |
Cast this object to its derived type.
Definition at line 212 of file expr_engine.h.
◆ find()
|
inlinestaticprotected |
◆ indices()
|
inline |
Index list accessor.
- Returns
- A const reference to the index list
Definition at line 224 of file expr_engine.h.

◆ init_distribution()
|
inline |
Initialize result tensor distribution.
This function will initialize the world and process map for the result tensor.
- Parameters
-
world The world were the result will be distributed pmap The process map for the result tensor tiles
Definition at line 304 of file cont_engine.h.
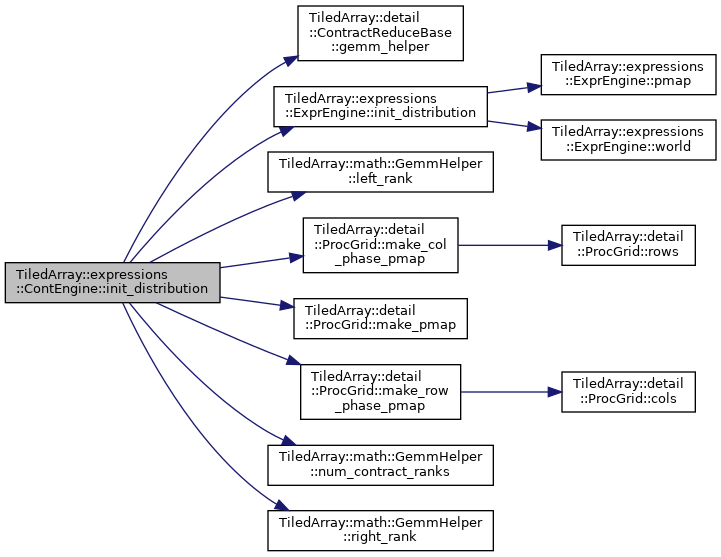
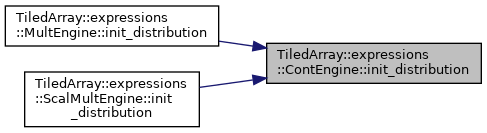
◆ init_indices() [1/2]
|
inline |
Initialize the index list of this expression.
- Note
- This function does not initialize the child data as is done in
BinaryEngine
. Instead they are initialized inMultEngine
andScalMultEngine
since they need child indices to determine the type of product
Definition at line 212 of file cont_engine.h.
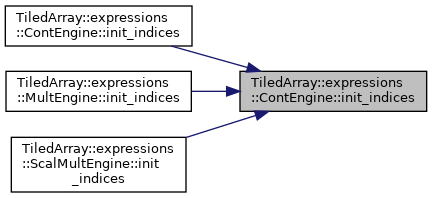
◆ init_indices() [2/2]
|
inline |
Initialize the index list of this expression.
- Parameters
-
target_indices the target index list
- Note
- This function does not initialize the child data as is done in
BinaryEngine
. Instead they are initialized inMultEngine
andScalMultEngine
since they need child indices to determine the type of product
Definition at line 228 of file cont_engine.h.
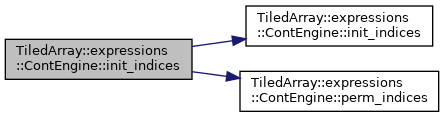
◆ init_inner_tile_op()
|
inlineprotected |
◆ init_struct()
|
inline |
Initialize result tensor structure.
This function will initialize the permutation, tiled range, and shape for the result tensor as well as the tile operation.
- Parameters
-
target_indices The target index list for the result tensor
Definition at line 238 of file cont_engine.h.
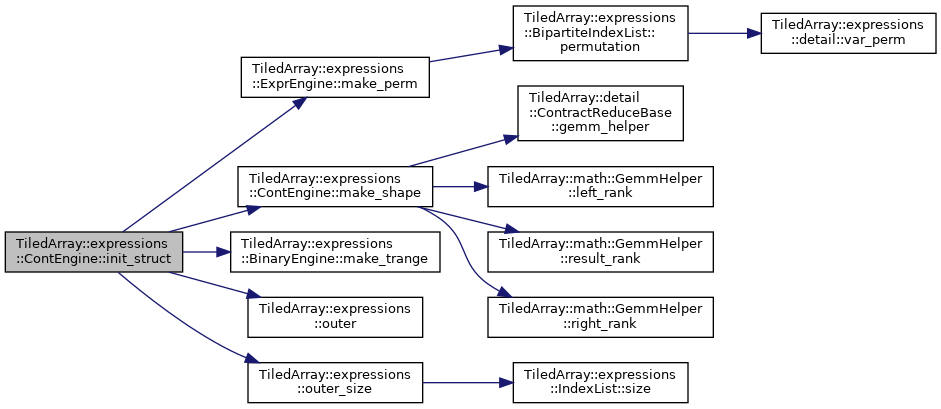
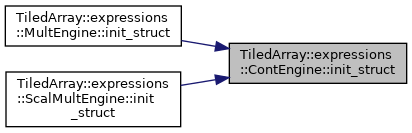
◆ inner_product_type()
|
inlineprotected |
- Returns
- the inner product type
only Hadamard and contraction are supported now
Definition at line 147 of file cont_engine.h.

◆ make_dist_eval()
|
inline |
◆ make_shape() [1/2]
|
inline |
Non-permuting shape factory function.
- Returns
- The result shape
Definition at line 398 of file cont_engine.h.
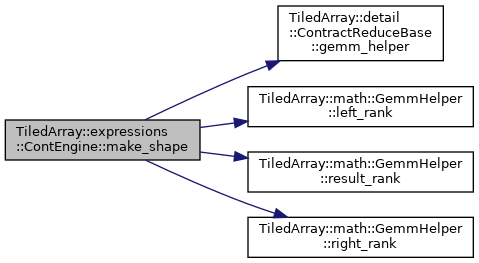
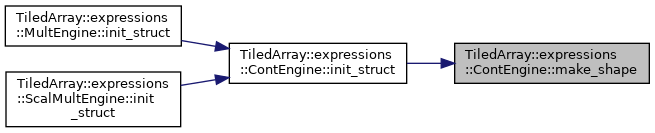
◆ make_shape() [2/2]
|
inline |
Permuting shape factory function.
- Parameters
-
perm The permutation to be applied to the array
- Returns
- The result shape
Definition at line 410 of file cont_engine.h.
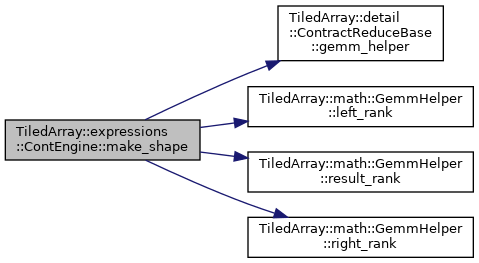
◆ make_tag()
|
inline |
Expression identification tag.
- Returns
- An expression tag used to identify this expression
Definition at line 438 of file cont_engine.h.
◆ make_trange()
|
inline |
Tiled range factory function.
- Parameters
-
perm The permutation to be applied to the array
- Returns
- The result tiled range
Definition at line 349 of file cont_engine.h.
◆ perm_indices()
|
inline |
Set the index list for this expression.
If arguments can be permuted freely and target_indices
are well-partitioned into left and right args' indices it is possible to permute left and right args to order their free indices the order that target_indices
requires.
- Parameters
-
target_indices The target index list for this expression
- Warning
- this does not take into account the ranks of the args to decide whether it makes sense to permute them or the result
- Todo:
- take into account the ranks of the args to decide whether it makes sense to permute them or the result
Definition at line 190 of file cont_engine.h.
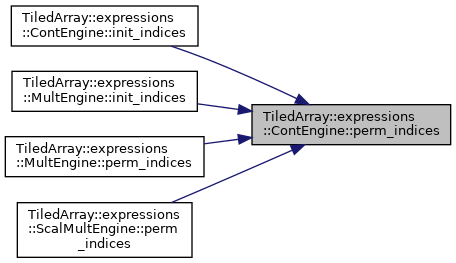
◆ print()
|
inline |
Expression print.
- Parameters
-
os The output stream target_indices The target index list for this expression
Definition at line 449 of file cont_engine.h.
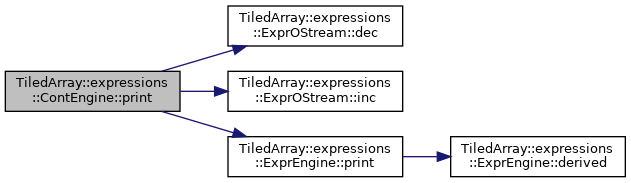
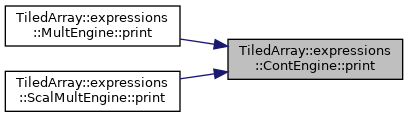
◆ product_type()
|
inlineprotected |
- Returns
- the product type
only Hadamard and contraction are supported now
Definition at line 137 of file cont_engine.h.

Member Data Documentation
◆ factor_
|
protected |
Contraction scaling factor.
Definition at line 106 of file cont_engine.h.
◆ indices_
|
protected |
The index list of this expression; bipartite due to need to support recursive tensors (i.e. Tensor-of-Tensor)
Definition at line 75 of file expr_engine.h.
◆ inner_product_type_
|
protected |
Definition at line 134 of file cont_engine.h.
◆ inner_tile_nonreturn_op_
|
protected |
Tile element operation (only non-null for nested tensor expressions)
Definition at line 113 of file cont_engine.h.
◆ inner_tile_return_op_
|
protected |
Same as inner_tile_nonreturn_op_ but returns the result
Definition at line 117 of file cont_engine.h.
◆ K_
|
protected |
Inner dimension size.
Definition at line 121 of file cont_engine.h.
◆ left_
|
protected |
The left-hand argument.
Definition at line 86 of file binary_engine.h.
◆ left_indices_
|
protected |
Target left-hand index list.
Definition at line 88 of file binary_engine.h.
◆ left_inner_permtype_
|
protected |
Left-hand permutation type.
Definition at line 94 of file binary_engine.h.
◆ left_outer_permtype_
|
protected |
Left-hand permutation type.
Definition at line 90 of file binary_engine.h.
◆ op_
|
protected |
Tile operation.
Definition at line 109 of file cont_engine.h.
◆ perm_
|
protected |
The permutation that will be applied to the outer tensor of tensors.
Definition at line 80 of file expr_engine.h.
◆ permute_tiles_
|
protected |
Result tile permutation flag (true
== permute tile)
Definition at line 77 of file expr_engine.h.
◆ pmap_
|
protected |
The process map for the result tensor.
Definition at line 84 of file expr_engine.h.
◆ proc_grid_
|
protected |
Process grid for the contraction.
Definition at line 120 of file cont_engine.h.
◆ product_type_
|
protected |
Definition at line 133 of file cont_engine.h.
◆ right_
|
protected |
The right-hand argument.
Definition at line 87 of file binary_engine.h.
◆ right_indices_
|
protected |
Target right-hand index list.
Definition at line 89 of file binary_engine.h.
◆ right_inner_permtype_
|
protected |
Right-hand permutation type.
Definition at line 96 of file binary_engine.h.
◆ right_outer_permtype_
|
protected |
Right-hand permutation type.
Definition at line 92 of file binary_engine.h.
◆ shape_
|
protected |
The shape of the result tensor.
Definition at line 82 of file expr_engine.h.
◆ trange_
|
protected |
The tiled range of the result tensor.
Definition at line 81 of file expr_engine.h.
◆ world_
|
protected |
The world where this expression will be evaluated.
Definition at line 73 of file expr_engine.h.
The documentation for this class was generated from the following file:
- TiledArray/expressions/cont_engine.h