Documentation
Range data of a tiled array.
TiledRange is a direct (Cartesian) product of 1-dimensional tiled ranges (TiledRange1)
Definition at line 32 of file tiled_range.h.
Public Types | |
typedef TiledRange | TiledRange_ |
typedef Range | range_type |
typedef range_type::index_type | index_type |
typedef range_type::ordinal_type | ordinal_type |
typedef range_type::index1_type | index1_type |
typedef container::svector< TiledRange1 > | Ranges |
Public Member Functions | |
TiledRange () | |
Default constructor. More... | |
template<typename InIter > | |
TiledRange (InIter first, InIter last) | |
template<typename TRange1Range , typename = std::enable_if_t< detail::is_range_v<TRange1Range> && std::is_same_v<detail::value_t<TRange1Range>, TiledRange1>>> | |
TiledRange (const TRange1Range &range_of_trange1s) | |
template<typename Integer , typename = std::enable_if_t<std::is_integral_v<Integer>>> | |
TiledRange (const std::initializer_list< std::initializer_list< Integer >> &list) | |
Constructs using a list of lists convertible to TiledRange1. More... | |
TiledRange (const std::initializer_list< TiledRange1 > &list) | |
Constructed with an initializer_list of TiledRange1's. More... | |
TiledRange (const TiledRange_ &other) | |
Copy constructor. More... | |
TiledRange_ & | operator= (const TiledRange_ &other) |
TiledRange assignment operator. More... | |
TiledRange_ & | operator*= (const Permutation &p) |
In place permutation of this range. More... | |
const range_type & | tiles_range () const |
Access the tile range. More... | |
const range_type & | tiles () const |
Access the tile range. More... | |
const range_type & | elements_range () const |
Access the element range. More... | |
const range_type & | elements () const |
Access the element range. More... | |
range_type | tile (const index1_type &i) const |
Constructs a range for the tile indexed by the given ordinal index. More... | |
range_type | make_tile_range (const index1_type &i) const |
Construct a range for the tile indexed by the given ordinal index. More... | |
template<typename Index > | |
std::enable_if_t< detail::is_integral_range_v< Index >, range_type > | tile (const Index &index) const |
Construct a range for the tile indexed by the given index. More... | |
template<typename Index > | |
std::enable_if_t< detail::is_integral_range_v< Index >, range_type > | make_tile_range (const Index &index) const |
Construct a range for the tile indexed by the given index. More... | |
template<typename Integer , typename = std::enable_if_t<std::is_integral_v<Integer>>> | |
range_type | tile (const std::initializer_list< Integer > &index) const |
Construct a range for the tile indexed by the given index. More... | |
template<typename Integer , typename = std::enable_if_t<std::is_integral_v<Integer>>> | |
range_type | make_tile_range (const std::initializer_list< Integer > &index) const |
Construct a range for the tile indexed by the given index. More... | |
template<typename Index > | |
std::enable_if_t< detail::is_integral_range_v< Index >, typename range_type::index > | element_to_tile (const Index &index) const |
Convert an element index to a tile index. More... | |
std::size_t | rank () const |
The rank accessor. More... | |
const TiledRange1 & | dim (std::size_t d) const |
Accessor of the tiled range for one of the dimensions. More... | |
const Ranges & | data () const |
Tile dimension boundary array accessor. More... | |
void | swap (TiledRange_ &other) |
template<typename Archive , typename std::enable_if< madness::archive::is_input_archive< Archive >::value >::type * = nullptr> | |
void | serialize (const Archive &ar) |
template<typename Archive , typename std::enable_if< madness::archive::is_output_archive< Archive >::value >::type * = nullptr> | |
void | serialize (const Archive &ar) const |
Member Typedef Documentation
◆ index1_type
Definition at line 68 of file tiled_range.h.
◆ index_type
Definition at line 66 of file tiled_range.h.
◆ ordinal_type
Definition at line 67 of file tiled_range.h.
◆ range_type
Definition at line 65 of file tiled_range.h.
◆ Ranges
Definition at line 70 of file tiled_range.h.
◆ TiledRange_
Definition at line 64 of file tiled_range.h.
Constructor & Destructor Documentation
◆ TiledRange() [1/6]
|
inline |
Default constructor.
Definition at line 73 of file tiled_range.h.

◆ TiledRange() [2/6]
|
inlineexplicit |
Constructs using a range of TiledRange1 objects
- Parameters
-
first the iterator pointing to the front of the range last the iterator pointing past the back of the range
Definition at line 79 of file tiled_range.h.
◆ TiledRange() [3/6]
|
inlineexplicit |
Constructs using a range of TiledRange1 objects
- Parameters
-
range_of_trange1s a range of TiledRange1 objects
Definition at line 90 of file tiled_range.h.
◆ TiledRange() [4/6]
|
inlineexplicit |
Constructs using a list of lists convertible to TiledRange1.
- Template Parameters
-
Integer An integral type
- Parameters
-
list a list of lists of integers that can be converted to TiledRange1
Definition at line 104 of file tiled_range.h.
◆ TiledRange() [5/6]
|
inlineexplicit |
Constructed with an initializer_list of TiledRange1's.
Definition at line 115 of file tiled_range.h.
◆ TiledRange() [6/6]
|
inline |
Copy constructor.
Definition at line 121 of file tiled_range.h.
Member Function Documentation
◆ data()
|
inline |
Tile dimension boundary array accessor.
- Returns
- A reference to the array of Range1 objects.
- Exceptions
-
nothing
Definition at line 292 of file tiled_range.h.
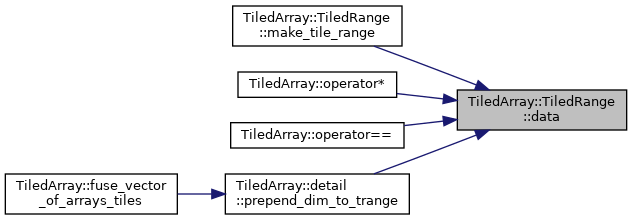
◆ dim()
|
inline |
Accessor of the tiled range for one of the dimensions.
- Parameters
-
d the dimension index, a nonnegative integer less than rank()
- Returns
- TiledRange1 object for dimension
d
Definition at line 283 of file tiled_range.h.


◆ element_to_tile()
|
inline |
Convert an element index to a tile index.
- Template Parameters
-
Index An integral range type
- Parameters
-
index The element index to convert
- Returns
- The tile index that corresponds to the given element index
Definition at line 259 of file tiled_range.h.
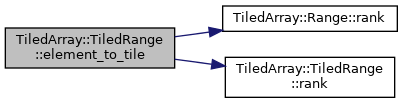

◆ elements()
|
inline |
Access the element range.
- Returns
- A const reference to the element range object
- Deprecated:
- use TiledRange::elements_range() instead
Definition at line 164 of file tiled_range.h.

◆ elements_range()
|
inline |
Access the element range.
- Returns
- A const reference to the element range object
Definition at line 158 of file tiled_range.h.
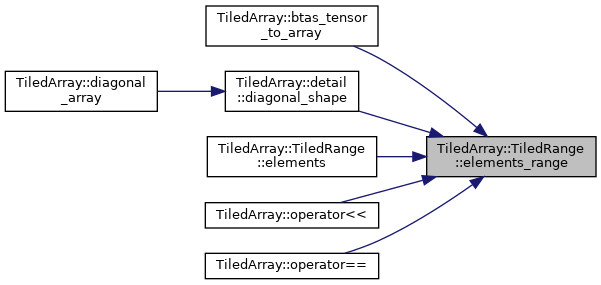
◆ make_tile_range() [1/3]
|
inline |
Construct a range for the tile indexed by the given index.
- Template Parameters
-
Index An integral range type
- Parameters
-
index The index of the tile range to be constructed
- Exceptions
-
std::runtime_error Throws if i is not included in the range
- Returns
- The constructed range object
Definition at line 207 of file tiled_range.h.
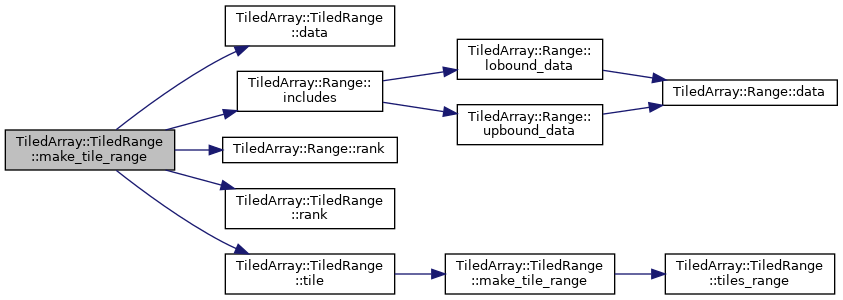
◆ make_tile_range() [2/3]
|
inline |
Construct a range for the tile indexed by the given ordinal index.
- Parameters
-
i The ordinal index of the tile range to be constructed
- Exceptions
-
std::runtime_error Throws if i is not included in the range
- Returns
- The constructed range object
Definition at line 180 of file tiled_range.h.


◆ make_tile_range() [3/3]
|
inline |
Construct a range for the tile indexed by the given index.
- Template Parameters
-
Integer An integral type
- Parameters
-
index The tile index, given as a std::initializer_list
- Exceptions
-
std::runtime_error Throws if i is not included in the range
- Returns
- The constructed range object
Definition at line 246 of file tiled_range.h.
◆ operator*=()
|
inline |
In place permutation of this range.
- Returns
- A reference to this object
Definition at line 137 of file tiled_range.h.
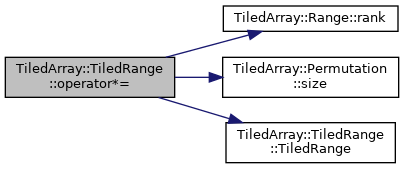
◆ operator=()
|
inline |
TiledRange assignment operator.
- Returns
- A reference to this object
Definition at line 129 of file tiled_range.h.

◆ rank()
|
inline |
The rank accessor.
- Returns
- the rank (=number of dimensions) of this object
Definition at line 277 of file tiled_range.h.
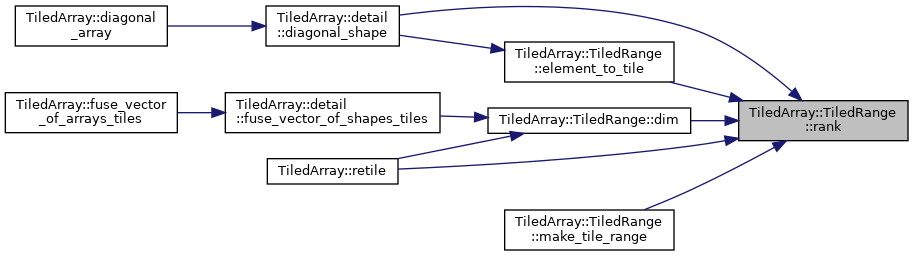
◆ serialize() [1/2]
|
inline |
Definition at line 303 of file tiled_range.h.
◆ serialize() [2/2]
|
inline |
Definition at line 310 of file tiled_range.h.
◆ swap()
|
inline |
Definition at line 294 of file tiled_range.h.

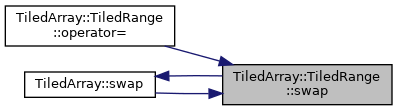
◆ tile() [1/3]
|
inline |
Construct a range for the tile indexed by the given index.
- Template Parameters
-
Index An integral range type
- Parameters
-
index The index of the tile range to be constructed
- Exceptions
-
std::runtime_error Throws if i is not included in the range
- Returns
- The constructed range object
- Note
- alias to TiledRange::make_tile_range() , introduced for consitency with TiledRange1::tile()
Definition at line 195 of file tiled_range.h.

◆ tile() [2/3]
|
inline |
Constructs a range for the tile indexed by the given ordinal index.
- Parameters
-
i The ordinal index of the tile range to be constructed
- Exceptions
-
std::runtime_error Throws if i is not included in the range
- Returns
- The constructed range object
- Note
- alias to TiledRange::make_tile_range() , introduced for consitency with TiledRange1::tile()
Definition at line 173 of file tiled_range.h.


◆ tile() [3/3]
|
inline |
Construct a range for the tile indexed by the given index.
- Template Parameters
-
Integer An integral type
- Parameters
-
index The tile index, given as a std::initializer_list
- Exceptions
-
std::runtime_error Throws if i is not included in the range
- Returns
- The constructed range object
- Note
- alias to TiledRange::make_tile_range() , introduced for consitency with TiledRange1::tile()
Definition at line 234 of file tiled_range.h.

◆ tiles()
|
inline |
Access the tile range.
- Returns
- A const reference to the tile range object
- Deprecated:
- use TiledRange::tiles_range() instead
Definition at line 153 of file tiled_range.h.

◆ tiles_range()
|
inline |
Access the tile range.
- Returns
- A const reference to the tile range object
Definition at line 147 of file tiled_range.h.
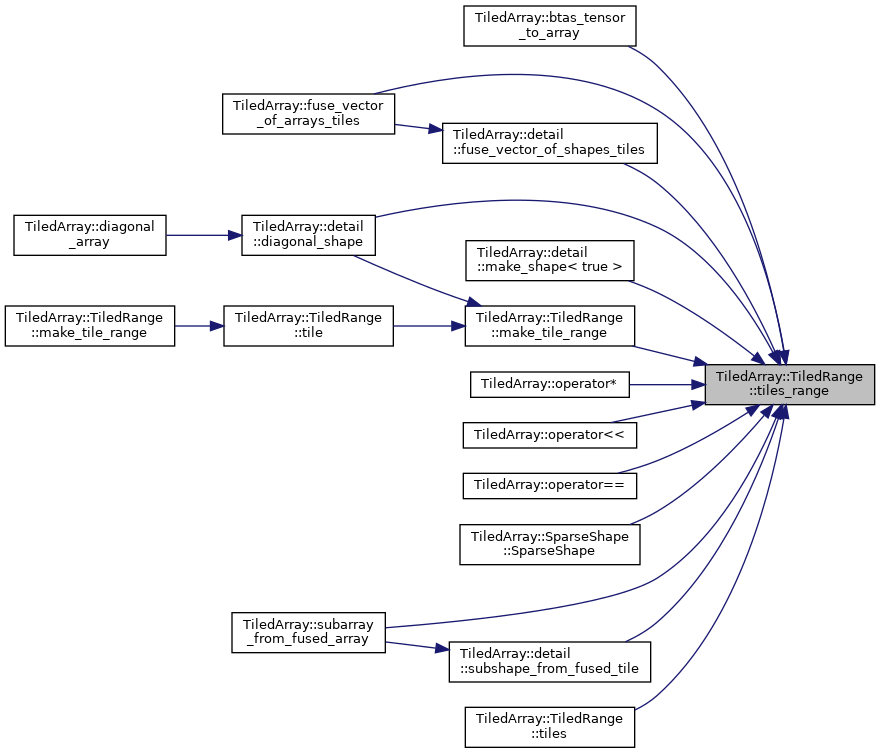
The documentation for this class was generated from the following file:
- TiledArray/tiled_range.h