
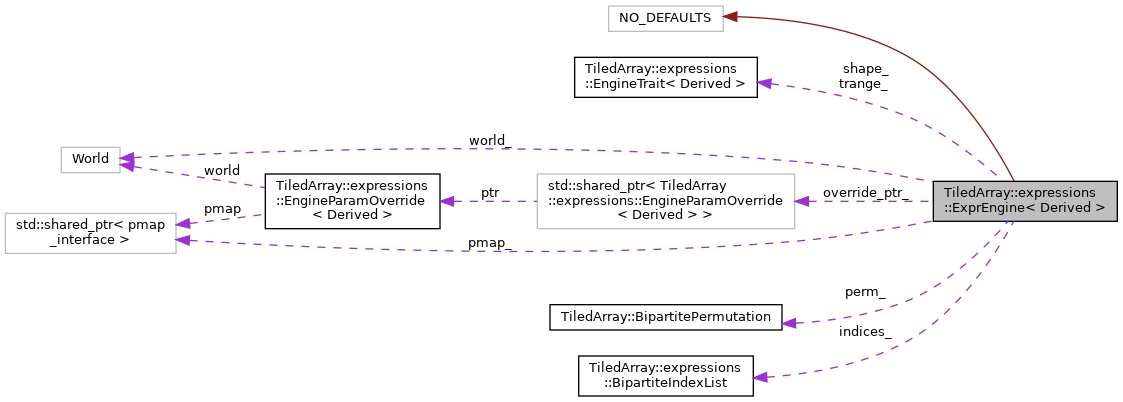
Documentation
template<typename Derived>
class TiledArray::expressions::ExprEngine< Derived >
Expression engine.
Definition at line 45 of file expr_engine.h.
Public Types | |
typedef ExprEngine< Derived > | ExprEngine_ |
typedef Derived | derived_type |
The derived object type. More... | |
typedef EngineTrait< Derived >::value_type | value_type |
Tensor value type. More... | |
typedef EngineTrait< Derived >::op_type | op_type |
Tile operation type. More... | |
typedef EngineTrait< Derived >::policy | policy |
The result policy type. More... | |
typedef EngineTrait< Derived >::dist_eval_type | dist_eval_type |
This expression's distributed evaluator type. More... | |
typedef EngineTrait< Derived >::size_type | size_type |
Size type. More... | |
typedef EngineTrait< Derived >::trange_type | trange_type |
Tiled range type type. More... | |
typedef EngineTrait< Derived >::shape_type | shape_type |
Tensor shape type. More... | |
typedef EngineTrait< Derived >::pmap_interface | pmap_interface |
Process map interface type. More... | |
Public Member Functions | |
template<typename D > | |
ExprEngine (const Expr< D > &expr) | |
Default constructor. More... | |
void | init (World &world, std::shared_ptr< pmap_interface > pmap, const BipartiteIndexList &target_indices) |
Construct and initialize the expression engine. More... | |
void | init_struct (const BipartiteIndexList &target_indices) |
Initialize result tensor structure. More... | |
void | init_distribution (World *world, const std::shared_ptr< pmap_interface > &pmap) |
Initialize result tensor distribution. More... | |
BipartitePermutation | make_perm (const BipartiteIndexList &target_indices) const |
Permutation factory function. More... | |
op_type | make_op () const |
Tile operation factory function. More... | |
derived_type & | derived () |
Cast this object to its derived type. More... | |
const derived_type & | derived () const |
Cast this object to its derived type. More... | |
World * | world () const |
World accessor. More... | |
const BipartiteIndexList & | indices () const |
Index list accessor. More... | |
const BipartitePermutation & | perm () const |
Permutation accessor. More... | |
const trange_type & | trange () const |
Tiled range accessor. More... | |
const shape_type & | shape () const |
Shape accessor. More... | |
const std::shared_ptr< pmap_interface > & | pmap () const |
Process map accessor. More... | |
void | permute_tiles (const bool status) |
Set the permute tiles flag. More... | |
void | print (ExprOStream &os, const BipartiteIndexList &target_indices) const |
Expression print. More... | |
const char * | make_tag () const |
Expression identification tag. More... | |
Protected Attributes | |
World * | world_ |
The world where this expression will be evaluated. More... | |
BipartiteIndexList | indices_ |
bool | permute_tiles_ |
BipartitePermutation | perm_ |
The permutation that will be applied to the outer tensor of tensors. More... | |
trange_type | trange_ |
The tiled range of the result tensor. More... | |
shape_type | shape_ |
The shape of the result tensor. More... | |
std::shared_ptr< pmap_interface > | pmap_ |
The process map for the result tensor. More... | |
std::shared_ptr< EngineParamOverride< Derived > > | override_ptr_ |
The engine params overriding the default. More... | |
Member Typedef Documentation
◆ derived_type
typedef Derived TiledArray::expressions::ExprEngine< Derived >::derived_type |
The derived object type.
Definition at line 48 of file expr_engine.h.
◆ dist_eval_type
typedef EngineTrait<Derived>::dist_eval_type TiledArray::expressions::ExprEngine< Derived >::dist_eval_type |
This expression's distributed evaluator type.
Definition at line 58 of file expr_engine.h.
◆ ExprEngine_
typedef ExprEngine<Derived> TiledArray::expressions::ExprEngine< Derived >::ExprEngine_ |
Definition at line 47 of file expr_engine.h.
◆ op_type
typedef EngineTrait<Derived>::op_type TiledArray::expressions::ExprEngine< Derived >::op_type |
Tile operation type.
Definition at line 54 of file expr_engine.h.
◆ pmap_interface
typedef EngineTrait<Derived>::pmap_interface TiledArray::expressions::ExprEngine< Derived >::pmap_interface |
Process map interface type.
Definition at line 67 of file expr_engine.h.
◆ policy
typedef EngineTrait<Derived>::policy TiledArray::expressions::ExprEngine< Derived >::policy |
The result policy type.
Definition at line 56 of file expr_engine.h.
◆ shape_type
typedef EngineTrait<Derived>::shape_type TiledArray::expressions::ExprEngine< Derived >::shape_type |
Tensor shape type.
Definition at line 65 of file expr_engine.h.
◆ size_type
typedef EngineTrait<Derived>::size_type TiledArray::expressions::ExprEngine< Derived >::size_type |
Size type.
Definition at line 61 of file expr_engine.h.
◆ trange_type
typedef EngineTrait<Derived>::trange_type TiledArray::expressions::ExprEngine< Derived >::trange_type |
Tiled range type type.
Definition at line 63 of file expr_engine.h.
◆ value_type
typedef EngineTrait<Derived>::value_type TiledArray::expressions::ExprEngine< Derived >::value_type |
Tensor value type.
Definition at line 52 of file expr_engine.h.
Constructor & Destructor Documentation
◆ ExprEngine()
|
inline |
Default constructor.
All data members are initialized to NULL values.
Definition at line 93 of file expr_engine.h.
Member Function Documentation
◆ derived() [1/2]
|
inline |
Cast this object to its derived type.
Definition at line 209 of file expr_engine.h.
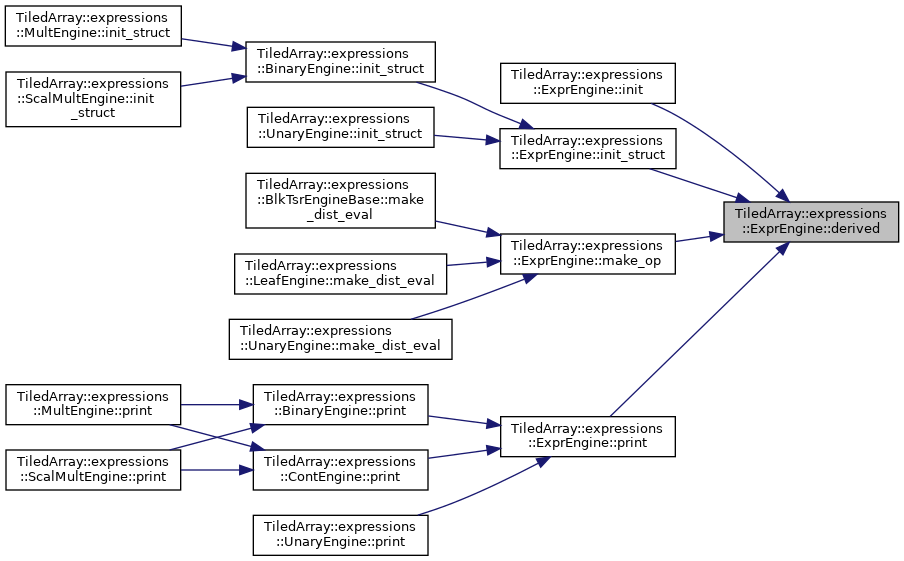
◆ derived() [2/2]
|
inline |
Cast this object to its derived type.
Definition at line 212 of file expr_engine.h.
◆ indices()
|
inline |
Index list accessor.
- Returns
- A const reference to the index list
Definition at line 224 of file expr_engine.h.

◆ init()
|
inline |
Construct and initialize the expression engine.
This function will initialize all expression engines in the expression graph. The init_indices()
, init_struct()
, and init_distribution()
will be called for each node and leaf of the graph in that order.
- Parameters
-
world The world where the expression will be evaluated pmap The process map for the result tensor (may be NULL) target_indices The target index list of the result tensor
Definition at line 112 of file expr_engine.h.
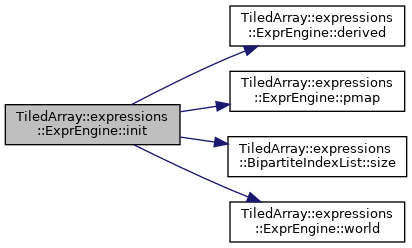
◆ init_distribution()
|
inline |
Initialize result tensor distribution.
This function will initialize the world and process map for the result tensor. Derived classes may customize this function by providing their own implementation it.
- Parameters
-
world The world were the result will be distributed pmap The process map for the result tensor tiles
Definition at line 171 of file expr_engine.h.
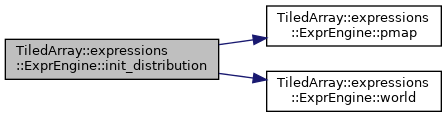
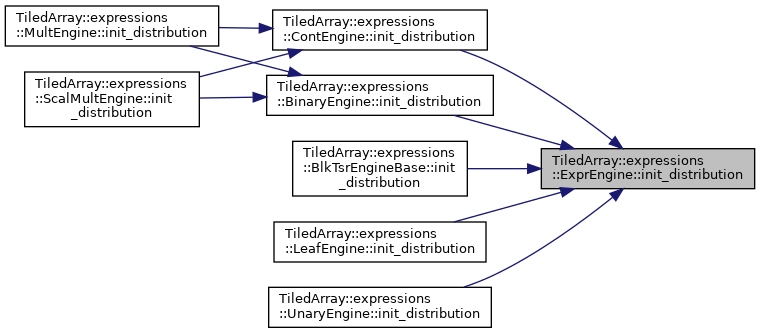
◆ init_struct()
|
inline |
Initialize result tensor structure.
This function will initialize the permutation, tiled range, and shape for the result tensor. These members are initialized with the make_perm()
, make_trange()
, and make_shape() functions. Derived classes may customize the structure initialization by providing their own implementation of this function or any of the above initialization. functions.
- Parameters
-
target_indices The target index list for the result tensor
Definition at line 150 of file expr_engine.h.
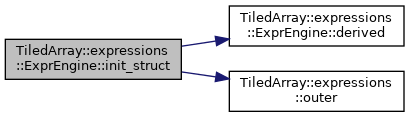
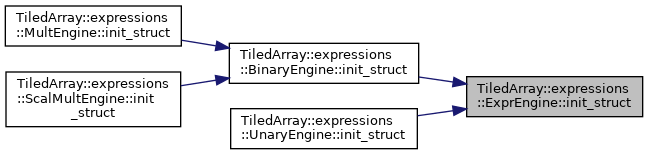
◆ make_op()
|
inline |
Tile operation factory function.
This function will generate the tile operations by calling make_tile_op()
. The permuting or non-permuting version of the tile operation will be selected based on permute_tiles(). Derived classes may customize this function by providing their own implementation it.
Definition at line 199 of file expr_engine.h.

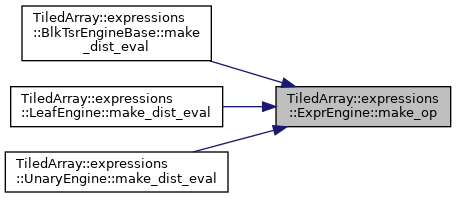
◆ make_perm()
|
inline |
Permutation factory function.
This function will generate the permutation that will be applied to the result tensor. Derived classes may customize this function by providing their own implementation it.
Definition at line 188 of file expr_engine.h.

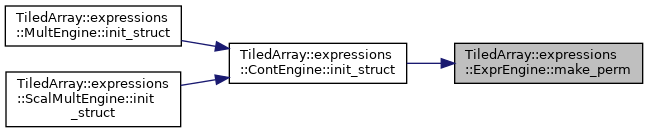
◆ make_tag()
|
inline |
Expression identification tag.
- Returns
- An expression tag used to identify this expression
Definition at line 269 of file expr_engine.h.
◆ perm()
|
inline |
Permutation accessor.
- Returns
- A const reference to the permutation
Definition at line 229 of file expr_engine.h.
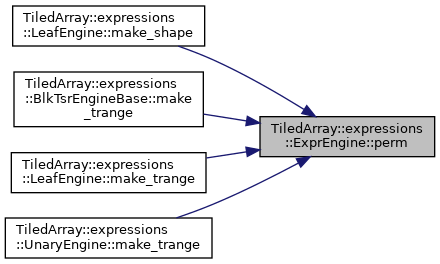
◆ permute_tiles()
|
inline |
Set the permute tiles flag.
- Parameters
-
status The new status for permute tiles (true == permute result tiles)
Definition at line 250 of file expr_engine.h.
◆ pmap()
|
inline |
Process map accessor.
- Returns
- A const reference to the process map
Definition at line 244 of file expr_engine.h.
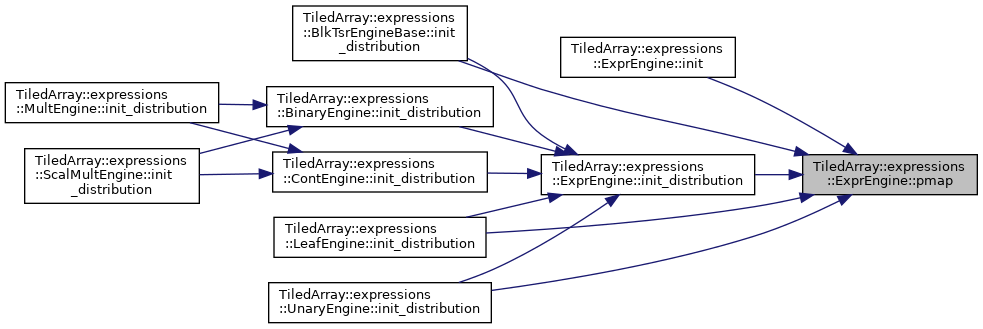
◆ print()
|
inline |
Expression print.
- Parameters
-
os The output stream target_indices The target index list for this expression
Definition at line 256 of file expr_engine.h.

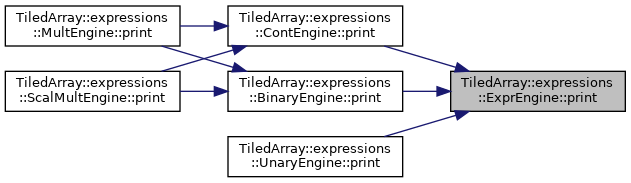
◆ shape()
|
inline |
Shape accessor.
- Returns
- A const reference to the tiled range
Definition at line 239 of file expr_engine.h.
◆ trange()
|
inline |
Tiled range accessor.
- Returns
- A const reference to the tiled range
Definition at line 234 of file expr_engine.h.

◆ world()
|
inline |
World accessor.
- Returns
- A pointer to world
Definition at line 219 of file expr_engine.h.
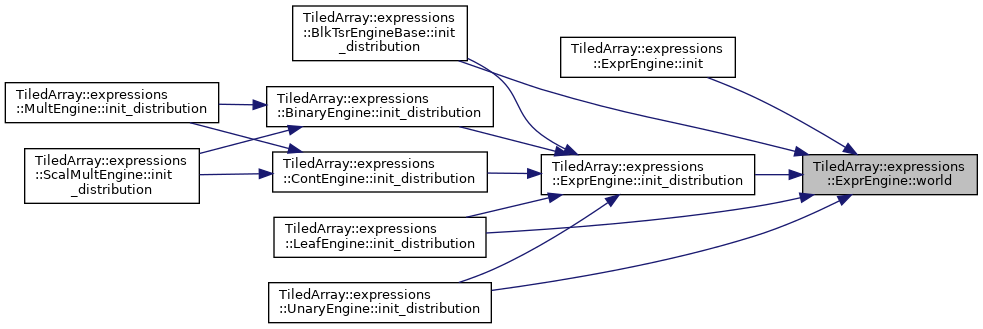
Member Data Documentation
◆ indices_
|
protected |
The index list of this expression; bipartite due to need to support recursive tensors (i.e. Tensor-of-Tensor)
Definition at line 75 of file expr_engine.h.
◆ override_ptr_
|
protected |
The engine params overriding the default.
Definition at line 86 of file expr_engine.h.
◆ perm_
|
protected |
The permutation that will be applied to the outer tensor of tensors.
Definition at line 80 of file expr_engine.h.
◆ permute_tiles_
|
protected |
Result tile permutation flag (true
== permute tile)
Definition at line 77 of file expr_engine.h.
◆ pmap_
|
protected |
The process map for the result tensor.
Definition at line 84 of file expr_engine.h.
◆ shape_
|
protected |
The shape of the result tensor.
Definition at line 82 of file expr_engine.h.
◆ trange_
|
protected |
The tiled range of the result tensor.
Definition at line 81 of file expr_engine.h.
◆ world_
|
protected |
The world where this expression will be evaluated.
Definition at line 73 of file expr_engine.h.
The documentation for this class was generated from the following file:
- TiledArray/expressions/expr_engine.h