Search Results
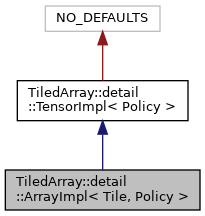
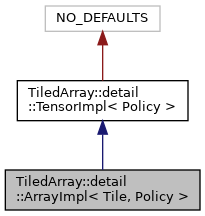
Documentation
template<typename Tile, typename Policy>
class TiledArray::detail::ArrayImpl< Tile, Policy >
Tensor implementation and base for other tensor implementation objects.
This implementation object holds the data for tensor object, which includes tiled range, shape, and tiles. The tiles are held in a distributed container, stored according to a given process map.
Definition at line 410 of file array_impl.h.
Public Types | |
typedef ArrayImpl< Tile, Policy > ArrayImpl_ | |
This object type. More... | |
typedef TensorImpl< Policy > TensorImpl_ | |
The base class of this object. More... | |
typedef TensorImpl_::index1_type index1_type | |
1-index type More... | |
typedef TensorImpl_::ordinal_type ordinal_type | |
Ordinal type. More... | |
typedef TensorImpl_::policy_type policy_type | |
Policy type for this object. More... | |
typedef TensorImpl_::trange_type trange_type | |
Tiled range type for this object. More... | |
typedef TensorImpl_::range_type range_type | |
Elements/tiles range type. More... | |
typedef TensorImpl_::shape_type shape_type | |
Shape type. More... | |
typedef TensorImpl_::pmap_interface pmap_interface | |
process map interface type More... | |
typedef Tile value_type | |
Tile or data type. More... | |
typedef eval_trait< Tile >::type eval_type | |
The tile evaluation type. More... | |
typedef numeric_type< value_type >::type numeric_type | |
the numeric type that supports Tile More... | |
typedef DistributedStorage< value_type > storage_type | |
The data container type. More... | |
typedef storage_type::future future | |
Future tile type. More... | |
typedef TileReference< ArrayImpl_ > reference | |
Tile reference type. More... | |
typedef TileConstReference< ArrayImpl_ > const_reference | |
Tile constant reference type. More... | |
typedef ArrayIterator< ArrayImpl_, reference > iterator | |
Iterator type. More... | |
typedef ArrayIterator< const ArrayImpl_, const_reference > const_iterator | |
Constant iterator type. More... | |
![]() | |
typedef TensorImpl< Policy > TensorImpl_ | |
typedef Policy policy_type | |
Policy type. More... | |
typedef Policy::trange_type trange_type | |
Tiled range type. More... | |
typedef Policy::range_type range_type | |
Element/tile range type. More... | |
typedef Policy::index1_type index1_type | |
1-index type More... | |
typedef Policy::ordinal_type ordinal_type | |
Ordinal type. More... | |
typedef Policy::shape_type shape_type | |
Tensor shape type. More... | |
typedef Policy::pmap_interface pmap_interface | |
Process map interface type. More... | |
Public Member Functions | |
ArrayImpl (World &world, const trange_type &trange, const shape_type &shape, const std::shared_ptr< pmap_interface > &pmap) | |
Constructor. More... | |
virtual ~ArrayImpl () | |
Virtual destructor. More... | |
template<typename Index , typename = std::enable_if_t<std::is_integral_v<Index> || detail::is_integral_range_v<Index>>> | |
future get (const Index &i) const | |
Tile future accessor. More... | |
template<typename Integer , typename = std::enable_if_t<std::is_integral_v<Integer>>> | |
future get (const std::initializer_list< Integer > &i) const | |
Tile future accessor. More... | |
template<typename Index , typename = std::enable_if_t<std::is_integral_v<Index> || detail::is_integral_range_v<Index>>> | |
const future & get_local (const Index &i) const | |
Local tile future accessor. More... | |
template<typename Integer , typename = std::enable_if_t<std::is_integral_v<Integer>>> | |
const future & get_local (const std::initializer_list< Integer > &i) const | |
Local tile future accessor. More... | |
template<typename Index , typename = std::enable_if_t<std::is_integral_v<Index> || detail::is_integral_range_v<Index>>> | |
future & get_local (const Index &i) | |
Local tile future accessor. More... | |
template<typename Integer , typename = std::enable_if_t<std::is_integral_v<Integer>>> | |
future & get_local (const std::initializer_list< Integer > &i) | |
Local tile future accessor. More... | |
template<typename Index , typename Value , typename = std::enable_if_t<std::is_integral_v<Index> || detail::is_integral_range_v<Index>>> | |
void set (const Index &i, Value &&value) | |
Set tile. More... | |
template<typename Index , typename Value , typename = std::enable_if_t<std::is_integral_v<Index>>> | |
void set (const std::initializer_list< Index > &i, Value &&value) | |
Set tile. More... | |
iterator begin () | |
Array begin iterator. More... | |
const_iterator cbegin () const | |
Array begin iterator. More... | |
iterator end () | |
Array end iterator. More... | |
const_iterator cend () const | |
Array end iterator. More... | |
const madness::uniqueidT & id () const | |
Unique object id accessor. More... | |
![]() | |
TensorImpl (World &world, const trange_type &trange, const shape_type &shape, const std::shared_ptr< pmap_interface > &pmap) | |
Constructor. More... | |
virtual ~TensorImpl () | |
Virtual destructor. More... | |
const std::shared_ptr< pmap_interface > & pmap () const | |
Tensor process map accessor. More... | |
const range_type & tiles_range () const | |
Tiles range accessor. More... | |
ordinal_type size () const | |
Tensor tile volume accessor. More... | |
ordinal_type local_size () const | |
Local element count. More... | |
template<typename Index > | |
ProcessID owner (const Index &i) const | |
Query a tile owner. More... | |
template<typename Index > | |
bool is_local (const Index &i) const | |
Query for a locally owned tile. More... | |
template<typename Index > | |
bool is_zero (const Index &i) const | |
Query for a zero tile. More... | |
bool is_dense () const | |
Query the density of the tensor. More... | |
const shape_type & shape () const | |
Tensor shape accessor. More... | |
const trange_type & trange () const | |
Tiled range accessor. More... | |
World & get_world () const | |
World & world () const | |
World accessor. More... | |
Static Public Member Functions | |
static std::function< void(const ArrayImpl_ &, int64_t)> & set_notifier_accessor () | |
Member Typedef Documentation
◆ ArrayImpl_
typedef ArrayImpl<Tile, Policy> TiledArray::detail::ArrayImpl< Tile, Policy >::ArrayImpl_ |
This object type.
Definition at line 412 of file array_impl.h.
◆ const_iterator
typedef ArrayIterator<const ArrayImpl_, const_reference> TiledArray::detail::ArrayImpl< Tile, Policy >::const_iterator |
Constant iterator type.
Definition at line 438 of file array_impl.h.
◆ const_reference
typedef TileConstReference<ArrayImpl_> TiledArray::detail::ArrayImpl< Tile, Policy >::const_reference |
Tile constant reference type.
Definition at line 435 of file array_impl.h.
◆ eval_type
typedef eval_trait<Tile>::type TiledArray::detail::ArrayImpl< Tile, Policy >::eval_type |
The tile evaluation type.
Definition at line 427 of file array_impl.h.
◆ future
typedef storage_type::future TiledArray::detail::ArrayImpl< Tile, Policy >::future |
Future tile type.
Definition at line 432 of file array_impl.h.
◆ index1_type
typedef TensorImpl_::index1_type TiledArray::detail::ArrayImpl< Tile, Policy >::index1_type |
1-index type
Definition at line 414 of file array_impl.h.
◆ iterator
typedef ArrayIterator<ArrayImpl_, reference> TiledArray::detail::ArrayImpl< Tile, Policy >::iterator |
Iterator type.
Definition at line 436 of file array_impl.h.
◆ numeric_type
typedef numeric_type<value_type>::type TiledArray::detail::ArrayImpl< Tile, Policy >::numeric_type |
the numeric type that supports Tile
Definition at line 429 of file array_impl.h.
◆ ordinal_type
typedef TensorImpl_::ordinal_type TiledArray::detail::ArrayImpl< Tile, Policy >::ordinal_type |
Ordinal type.
Definition at line 415 of file array_impl.h.
◆ pmap_interface
typedef TensorImpl_::pmap_interface TiledArray::detail::ArrayImpl< Tile, Policy >::pmap_interface |
process map interface type
Definition at line 424 of file array_impl.h.
◆ policy_type
typedef TensorImpl_::policy_type TiledArray::detail::ArrayImpl< Tile, Policy >::policy_type |
Policy type for this object.
Definition at line 417 of file array_impl.h.
◆ range_type
typedef TensorImpl_::range_type TiledArray::detail::ArrayImpl< Tile, Policy >::range_type |
Elements/tiles range type.
Definition at line 421 of file array_impl.h.
◆ reference
typedef TileReference<ArrayImpl_> TiledArray::detail::ArrayImpl< Tile, Policy >::reference |
Tile reference type.
Definition at line 433 of file array_impl.h.
◆ shape_type
typedef TensorImpl_::shape_type TiledArray::detail::ArrayImpl< Tile, Policy >::shape_type |
Shape type.
Definition at line 422 of file array_impl.h.
◆ storage_type
typedef DistributedStorage<value_type> TiledArray::detail::ArrayImpl< Tile, Policy >::storage_type |
The data container type.
Definition at line 431 of file array_impl.h.
◆ TensorImpl_
typedef TensorImpl<Policy> TiledArray::detail::ArrayImpl< Tile, Policy >::TensorImpl_ |
The base class of this object.
Definition at line 413 of file array_impl.h.
◆ trange_type
typedef TensorImpl_::trange_type TiledArray::detail::ArrayImpl< Tile, Policy >::trange_type |
Tiled range type for this object.
Definition at line 419 of file array_impl.h.
◆ value_type
typedef Tile TiledArray::detail::ArrayImpl< Tile, Policy >::value_type |
Tile or data type.
Definition at line 425 of file array_impl.h.
Constructor & Destructor Documentation
◆ ArrayImpl()
|
inline |
Constructor.
The size of shape must be equal to the volume of the tiled range tiles.
- Parameters
-
world The world where this tensor will live trange The tiled range for this tensor shape The shape of this tensor pmap The tile-process map
- Exceptions
-
TiledArray::Exception When the size of shape is not equal to zero
Definition at line 453 of file array_impl.h.
◆ ~ArrayImpl()
|
inlinevirtual |
Virtual destructor.
Definition at line 459 of file array_impl.h.
Member Function Documentation
◆ begin()
|
inline |
Array begin iterator.
- Returns
- A const iterator to the first local element of the array.
Definition at line 583 of file array_impl.h.
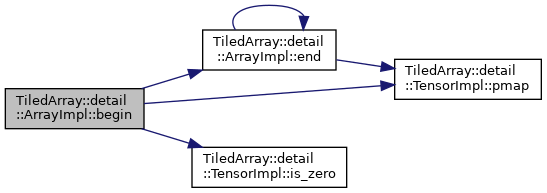
◆ cbegin()
|
inline |
Array begin iterator.
- Returns
- A const iterator to the first local element of the array.
Definition at line 599 of file array_impl.h.
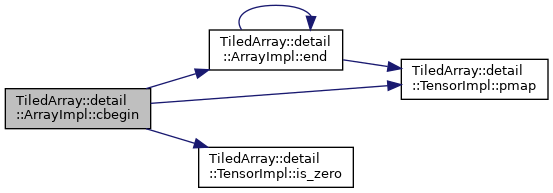
◆ cend()
|
inline |
Array end iterator.
- Returns
- A const iterator to one past the last local element of the array.
Definition at line 620 of file array_impl.h.
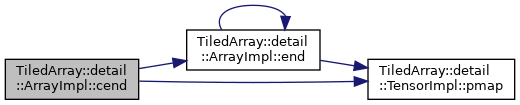
◆ end()
|
inline |
Array end iterator.
- Returns
- A const iterator to one past the last local element of the array.
Definition at line 615 of file array_impl.h.
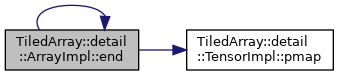
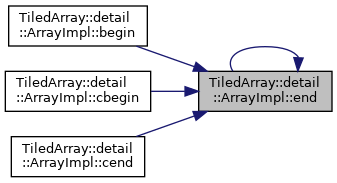
◆ get() [1/2]
|
inline |
Tile future accessor.
- Template Parameters
-
Index An integral or integral range type
- Parameters
-
i The tile index or ordinal
- Returns
- A
future
to tilei
- Exceptions
-
TiledArray::Exception When tile i
is zero
Definition at line 470 of file array_impl.h.
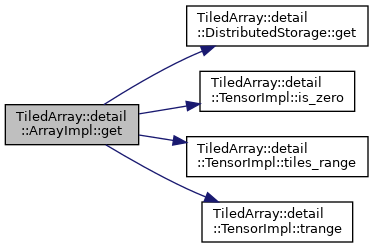
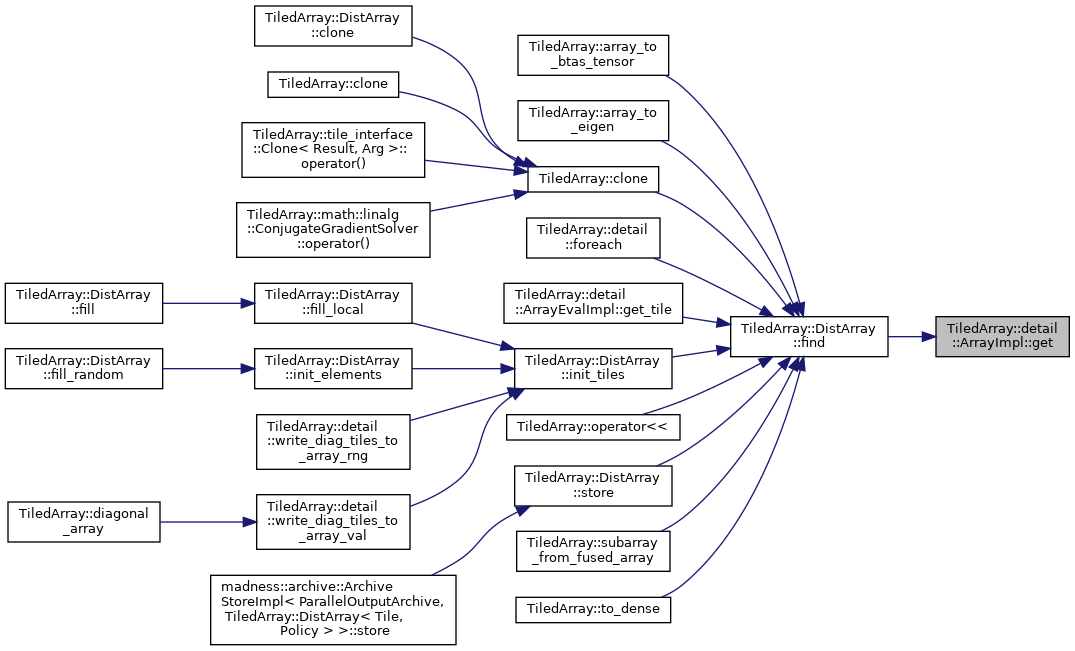
◆ get() [2/2]
|
inline |
Tile future accessor.
- Template Parameters
-
Integer An integral type
- Parameters
-
i The tile index, as an std::initializer_list<Integer>
- Returns
- A
future
to tilei
- Exceptions
-
TiledArray::Exception When tile i
is zero
Definition at line 483 of file array_impl.h.
◆ get_local() [1/4]
|
inline |
Local tile future accessor.
- Template Parameters
-
Index An integral or integral range type
- Parameters
-
i The tile index or ordinal
- Returns
- A
future
to tilei
- Exceptions
-
TiledArray::Exception When tile i
is zero or not local
Definition at line 522 of file array_impl.h.
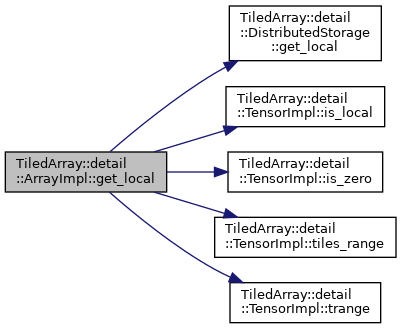
◆ get_local() [2/4]
|
inline |
Local tile future accessor.
- Template Parameters
-
Index An integral or integral range type
- Parameters
-
i The tile index or ordinal
- Returns
- A
future
to tilei
- Exceptions
-
TiledArray::Exception When tile i
is zero or not local
Definition at line 496 of file array_impl.h.
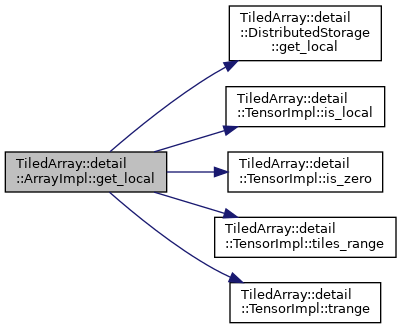

◆ get_local() [3/4]
|
inline |
Local tile future accessor.
- Template Parameters
-
Integer An integral type
- Parameters
-
i The tile index, as an std::initializer_list<Integer>
- Returns
- A
future
to tilei
- Exceptions
-
TiledArray::Exception When tile i
is zero or not local
Definition at line 535 of file array_impl.h.
◆ get_local() [4/4]
|
inline |
Local tile future accessor.
- Template Parameters
-
Integer An integral type
- Parameters
-
i The tile index, as an std::initializer_list<Integer>
- Returns
- A
future
to tilei
- Exceptions
-
TiledArray::Exception When tile i
is zero or not local
Definition at line 509 of file array_impl.h.
◆ id()
|
inline |
Unique object id accessor.
- Returns
- A const reference to this object unique id
Definition at line 627 of file array_impl.h.
◆ set() [1/2]
|
inline |
Set tile.
Set the tile at i
with value
. Value
type may be value_type
, Future<value_type>
, or madness::detail::MoveWrapper<value_type>
.
- Template Parameters
-
Index An integral or integral range type Value The value type
- Parameters
-
i The index of the tile to be set value The object tat contains the tile value
Definition at line 551 of file array_impl.h.
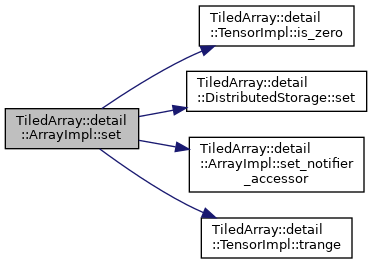
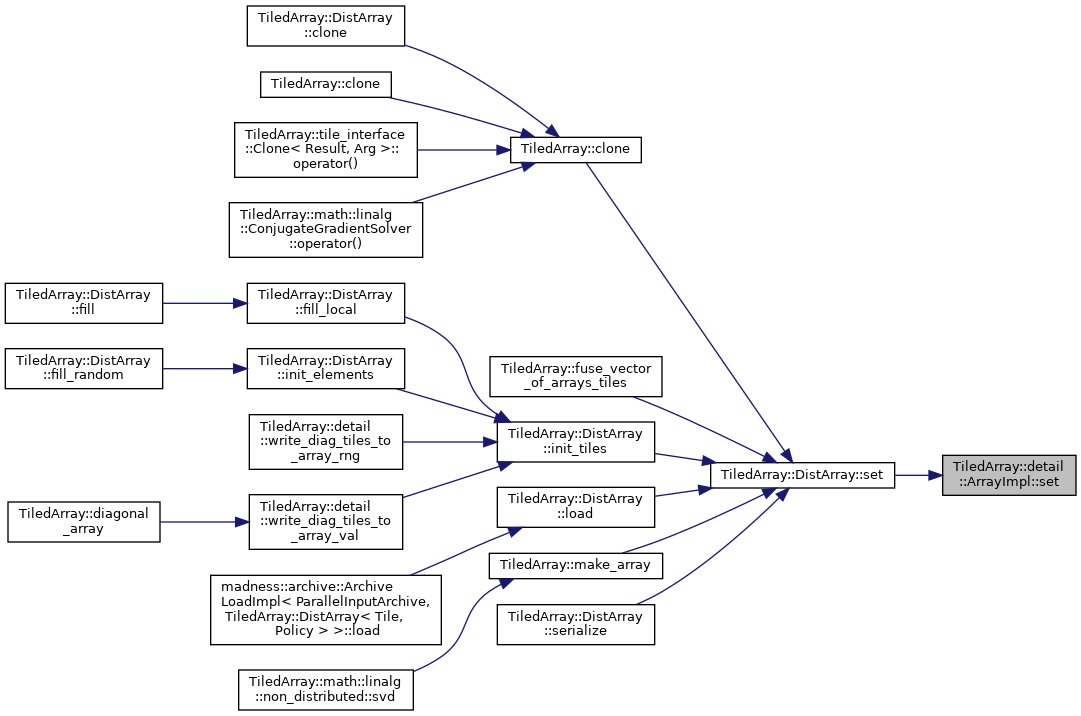
◆ set() [2/2]
|
inline |
Set tile.
Set the tile at i
with value
. Value
type may be value_type
, Future<value_type>
, or madness::detail::MoveWrapper<value_type>
.
- Template Parameters
-
Index An integral type Value The value type
- Parameters
-
i The index of the tile to be set value The object tat contains the tile value
Definition at line 571 of file array_impl.h.
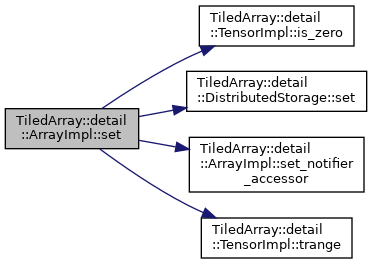
◆ set_notifier_accessor()
|
inlinestatic |
The documentation for this class was generated from the following file:
- TiledArray/array_impl.h