Documentation
template<typename Tile = Tensor<double, Eigen::aligned_allocator<double>>, typename Policy = DensePolicy>
class TiledArray::DistArray< Tile, Policy >
Forward declarations.
A (multidimensional) tiled array.
DistArray is the local representation of a global object. This means that the local array object will only contain a portion of the data. It may be used to construct distributed tensor algebraic operations.
- Template Parameters
-
T The element type of for array tiles Tile The tile type [ Default = Tensor<T>
]
Definition at line 57 of file dist_array.h.
Public Types | |
typedef DistArray< Tile, Policy > | DistArray_ |
This object's type. More... | |
typedef TiledArray::detail::ArrayImpl< Tile, Policy > | impl_type |
The type of the PIMPL. More... | |
typedef impl_type::policy_type | policy_type |
Policy type. More... | |
typedef detail::numeric_type< Tile >::type | numeric_type |
typedef detail::scalar_type< Tile >::type | scalar_type |
typedef impl_type::trange_type | trange_type |
Tile range type. More... | |
typedef impl_type::range_type | range_type |
Elements/tiles range type. More... | |
typedef impl_type::shape_type | shape_type |
Shape type for array tiling. More... | |
typedef impl_type::range_type::index1_type | index1_type |
1-index type More... | |
typedef impl_type::range_type::index | index |
Array coordinate index type. More... | |
typedef impl_type::ordinal_type | ordinal_type |
Ordinal type. More... | |
typedef impl_type::value_type | value_type |
Tile type. More... | |
typedef impl_type::eval_type | eval_type |
The tile evaluation type. More... | |
typedef impl_type::reference | future |
Future of value_type . More... | |
typedef impl_type::reference | reference |
future type More... | |
typedef impl_type::const_reference | const_reference |
future type More... | |
typedef impl_type::iterator | iterator |
Local tile iterator. More... | |
typedef impl_type::const_iterator | const_iterator |
Local tile const iterator. More... | |
typedef impl_type::pmap_interface | pmap_interface |
Process map interface type. More... | |
typedef value_type::value_type | element_type |
template<typename OtherTile > | |
using | is_my_type = std::is_same< DistArray_, DistArray< OtherTile, Policy > > |
template<typename OtherTile > | |
using | enable_if_not_my_type = std::enable_if_t< not is_my_type< OtherTile >::value > |
template<typename Index > | |
using | enable_if_is_integral_or_integral_range = std::enable_if_t< is_integral_or_integral_range_v< Index > > |
template<typename Index > | |
using | enable_if_is_integral = std::enable_if_t< std::is_integral_v< Index > > |
Public Member Functions | |
DistArray () | |
Default constructor. More... | |
DistArray (const DistArray_ &other) | |
Copy constructor. More... | |
DistArray (World &world, const trange_type &trange, const std::shared_ptr< pmap_interface > &pmap=std::shared_ptr< pmap_interface >()) | |
Dense array constructor. More... | |
DistArray (World &world, const trange_type &trange, const shape_type &shape, const std::shared_ptr< pmap_interface > &pmap=std::shared_ptr< pmap_interface >()) | |
Sparse array constructor. More... | |
template<typename OtherTile , typename = enable_if_not_my_type<OtherTile>> | |
DistArray (const DistArray< OtherTile, Policy > &other) | |
converting copy constructor More... | |
template<typename OtherTile , typename Op > | |
DistArray (const DistArray< OtherTile, Policy > &other, Op &&op) | |
Unary transform constructor. More... | |
~DistArray () | |
Destructor. More... | |
DistArray_ | clone () const |
Create a deep copy of this array. More... | |
std::shared_ptr< const impl_type > | pimpl () const |
Accessor for the (shared_ptr to) implementation object. More... | |
std::shared_ptr< impl_type > | pimpl () |
Accessor for the (shared_ptr to) implementation object. More... | |
std::weak_ptr< const impl_type > | weak_pimpl () const |
Accessor for the (weak_ptr to) implementation object. More... | |
std::weak_ptr< impl_type > | weak_pimpl () |
Accessor for the (shared_ptr to) implementation object. More... | |
void | wait_for_lazy_cleanup () const |
Wait for lazy tile cleanup. More... | |
DistArray_ & | operator= (const DistArray_ &other) |
Copy assignment. More... | |
madness::uniqueidT | id () const |
Global object id. More... | |
iterator | begin () |
Begin iterator factory function. More... | |
const_iterator | begin () const |
Begin const iterator factory function. More... | |
iterator | end () |
End iterator factory function. More... | |
const_iterator | end () const |
End const iterator factory function. More... | |
template<typename Index , typename = enable_if_is_integral_or_integral_range<Index>> | |
Future< value_type > | find (const Index &i) const |
Find local or remote tile by index. More... | |
template<typename Integer , typename = enable_if_is_integral<Integer>> | |
Future< value_type > | find (const std::initializer_list< Integer > &i) const |
Find local or remote tile. More... | |
template<typename Index , typename = std::enable_if_t<std::is_integral_v<Index> || detail::is_integral_range_v<Index>>> | |
const Future< value_type > & | find_local (const Index &i) const |
Find local tile. More... | |
template<typename Integer , typename = std::enable_if_t<(std::is_integral_v<Integer>)>> | |
const Future< value_type > & | find_local (const std::initializer_list< Integer > &i) const |
Find local tile. More... | |
template<typename Index , typename = std::enable_if_t<std::is_integral_v<Index> || detail::is_integral_range_v<Index>>> | |
Future< value_type > & | find_local (const Index &i) |
Find local tile. More... | |
template<typename Integer , typename = std::enable_if_t<(std::is_integral_v<Integer>)>> | |
Future< value_type > & | find_local (const std::initializer_list< Integer > &i) |
Find local tile. More... | |
template<typename Index , typename InIter , typename = std::enable_if_t<is_integral_or_integral_range_v<Index> && detail::is_input_iterator<InIter>::value>> | |
void | set (const Index &i, InIter first) |
template<typename Integer , typename InIter , typename = std::enable_if_t<(std::is_integral_v<Integer>)&&detail:: is_input_iterator<InIter>::value>> | |
std::enable_if< detail::is_input_iterator< InIter >::value >::type | set (const std::initializer_list< Integer > &i, InIter first) |
template<typename Index , typename = enable_if_is_integral_or_integral_range<Index>> | |
void | set (const Index &i, const element_type &value=element_type()) |
Set a tile and fill it using a value. More... | |
template<typename Integer , typename = enable_if_is_integral<Integer>> | |
void | set (const std::initializer_list< Integer > &i, const element_type &value=element_type()) |
Set every element of a tile to a specified value. More... | |
template<typename Index , typename Value , typename = std::enable_if_t<(is_integral_or_integral_range_v<Index> && is_value_or_future_to_value_v<Value>)>> | |
void | set (const Index &i, Value &&v) |
Set a tile directly using a future to a tile. More... | |
template<typename Index , typename Value , typename = std::enable_if_t< (std::is_integral_v<Index>)&&is_value_or_future_to_value_v<Value>>> | |
void | set (const std::initializer_list< Index > &i, Value &&v) |
Set a tile directly using a future to a tile. More... | |
void | fill_local (const element_type &value=element_type(), bool skip_set=false) |
Fill all local tiles with the specified value. More... | |
void | fill (const element_type &value=numeric_type(), bool skip_set=false) |
Fill all local tiles with the specified value. More... | |
template<typename T = element_type, typename = detail::enable_if_can_make_random_t<T>> | |
void | fill_random (bool skip_set=false) |
template<typename Op > | |
void | init_tiles (Op &&op, bool skip_set=false) |
Initialize (local) tiles with a user provided functor. More... | |
template<typename Op > | |
void | init_elements (Op &&op, bool skip_set=false) |
Initialize elements of local, non-zero tiles with a user provided functor. More... | |
const trange_type & | trange () const |
Tiled range accessor. More... | |
const range_type & | range () const |
Tile range accessor. More... | |
const range_type & | tiles_range () const |
Tile range accessor. More... | |
const trange_type::range_type & | elements () const |
const trange_type::range_type & | elements_range () const |
Element range accessor. More... | |
auto | size () const |
Returns the number of tiles in the tensor. More... | |
TiledArray::expressions::TsrExpr< const DistArray_, true > | operator() (const std::string &vars) const |
Create a tensor expression. More... | |
TiledArray::expressions::TsrExpr< DistArray_, true > | operator() (const std::string &vars) |
Create a tensor expression. More... | |
World & | get_world () const |
World & | world () const |
World accessor. More... | |
const std::shared_ptr< pmap_interface > & | get_pmap () const |
const std::shared_ptr< pmap_interface > & | pmap () const |
Process map accessor. More... | |
bool | is_dense () const |
Check dense/sparse. More... | |
const shape_type & | get_shape () const |
const shape_type & | shape () const |
Shape accessor. More... | |
template<typename Index , typename = enable_if_is_integral_or_integral_range<Index>> | |
ProcessID | owner (const Index &i) const |
Tile ownership. More... | |
template<typename Index , typename = enable_if_is_integral<Index>> | |
ProcessID | owner (const std::initializer_list< Index > &i) const |
Tile ownership. More... | |
template<typename Index , typename = enable_if_is_integral_or_integral_range<Index>> | |
bool | is_local (const Index &i) const |
Check if the tile at index i is stored locally. More... | |
template<typename Index , typename = enable_if_is_integral<Index>> | |
bool | is_local (const std::initializer_list< Index > &i) const |
Check if the tile at index i is stored locally. More... | |
template<typename Index , typename = enable_if_is_integral_or_integral_range<Index>> | |
bool | is_zero (const Index &i) const |
Check for zero tiles. More... | |
template<typename Index , typename = enable_if_is_integral<Index>> | |
bool | is_zero (const std::initializer_list< Index > &i) const |
Check for zero tiles. More... | |
void | swap (DistArray_ &other) |
Swap this array with other . More... | |
void | make_replicated () |
void | truncate (typename shape_type::value_type thresh=shape_type::threshold()) |
bool | is_initialized () const |
Check if the array is initialized. More... | |
operator bool () const | |
Conversion to bool is equivalent to DistArray::is_initialized() More... | |
template<typename Archive , typename = std::enable_if_t< !Archive::is_parallel_archive && madness::archive::is_output_archive<Archive>::value>> | |
void | serialize (const Archive &ar) const |
serialize local contents of a DistArray to an Archive object More... | |
template<typename Archive , typename = std::enable_if_t< !Archive::is_parallel_archive && madness::archive::is_input_archive<Archive>::value>> | |
void | serialize (const Archive &ar) |
deserialize local contents of a DistArray from an Archive object More... | |
template<typename Archive > | |
void | load (World &world, Archive &ar) |
template<typename Archive > | |
void | store (Archive &ar) const |
Stores this array to an Archive object. More... | |
Initializer list constructors | |
Creates a new tensor containing the elements in the provided | |
template<typename T > | |
DistArray (World &world, detail::vector_il< T > il) | |
template<typename T > | |
DistArray (World &world, detail::matrix_il< T > il) | |
template<typename T > | |
DistArray (World &world, detail::tensor3_il< T > il) | |
template<typename T > | |
DistArray (World &world, detail::tensor4_il< T > il) | |
template<typename T > | |
DistArray (World &world, detail::tensor5_il< T > il) | |
template<typename T > | |
DistArray (World &world, detail::tensor6_il< T > il) | |
Tiling initializer list constructors | |
Constructs a new tensor containing the elements in the provided | |
template<typename T > | |
DistArray (World &world, const trange_type &trange, detail::vector_il< T > il) | |
template<typename T > | |
DistArray (World &world, const trange_type &trange, detail::matrix_il< T > il) | |
template<typename T > | |
DistArray (World &world, const trange_type &trange, detail::tensor3_il< T > il) | |
template<typename T > | |
DistArray (World &world, const trange_type &trange, detail::tensor4_il< T > il) | |
template<typename T > | |
DistArray (World &world, const trange_type &trange, detail::tensor5_il< T > il) | |
template<typename T > | |
DistArray (World &world, const trange_type &trange, detail::tensor6_il< T > il) | |
Static Public Member Functions | |
static void | wait_for_lazy_cleanup (World &world, const double=60.0) |
Wait for lazy tile cleanup. More... | |
static void | register_set_notifier (std::function< void(const impl_type &, int64_t)> notifier={}) |
Debugging/tracing instrumentation. More... | |
Static Public Attributes | |
template<typename Index > | |
static constexpr bool | is_integral_or_integral_range_v |
template<typename Value > | |
static constexpr bool | is_value_or_future_to_value_v |
Member Typedef Documentation
◆ const_iterator
typedef impl_type::const_iterator TiledArray::DistArray< Tile, Policy >::const_iterator |
Local tile const iterator.
Definition at line 95 of file dist_array.h.
◆ const_reference
typedef impl_type::const_reference TiledArray::DistArray< Tile, Policy >::const_reference |
future
type
Definition at line 92 of file dist_array.h.
◆ DistArray_
typedef DistArray<Tile, Policy> TiledArray::DistArray< Tile, Policy >::DistArray_ |
This object's type.
Definition at line 59 of file dist_array.h.
◆ element_type
typedef value_type::value_type TiledArray::DistArray< Tile, Policy >::element_type |
The type of the elements in the tile. For a tile of type Tensor<T> this is T. Note that if the tile type is Tensor<Tensor<double>> this typedef will be Tensor<double> and NOT double like numeric_type.
Definition at line 102 of file dist_array.h.
◆ enable_if_is_integral
using TiledArray::DistArray< Tile, Policy >::enable_if_is_integral = std::enable_if_t<std::is_integral_v<Index> > |
Definition at line 125 of file dist_array.h.
◆ enable_if_is_integral_or_integral_range
using TiledArray::DistArray< Tile, Policy >::enable_if_is_integral_or_integral_range = std::enable_if_t<is_integral_or_integral_range_v<Index> > |
Definition at line 121 of file dist_array.h.
◆ enable_if_not_my_type
using TiledArray::DistArray< Tile, Policy >::enable_if_not_my_type = std::enable_if_t<not is_my_type<OtherTile>::value> |
Definition at line 113 of file dist_array.h.
◆ eval_type
typedef impl_type::eval_type TiledArray::DistArray< Tile, Policy >::eval_type |
The tile evaluation type.
Definition at line 88 of file dist_array.h.
◆ future
typedef impl_type::reference TiledArray::DistArray< Tile, Policy >::future |
Future of value_type
.
Definition at line 89 of file dist_array.h.
◆ impl_type
typedef TiledArray::detail::ArrayImpl<Tile, Policy> TiledArray::DistArray< Tile, Policy >::impl_type |
The type of the PIMPL.
Definition at line 61 of file dist_array.h.
◆ index
typedef impl_type::range_type::index TiledArray::DistArray< Tile, Policy >::index |
Array coordinate index type.
Definition at line 84 of file dist_array.h.
◆ index1_type
typedef impl_type::range_type::index1_type TiledArray::DistArray< Tile, Policy >::index1_type |
1-index type
Definition at line 82 of file dist_array.h.
◆ is_my_type
using TiledArray::DistArray< Tile, Policy >::is_my_type = std::is_same<DistArray_, DistArray<OtherTile, Policy> > |
These are some of the types and compile-time values needed for SFINAE. They probably should be factored out into a header file so they can be used elsewhere too.
Definition at line 110 of file dist_array.h.
◆ iterator
typedef impl_type::iterator TiledArray::DistArray< Tile, Policy >::iterator |
Local tile iterator.
Definition at line 93 of file dist_array.h.
◆ numeric_type
typedef detail::numeric_type<Tile>::type TiledArray::DistArray< Tile, Policy >::numeric_type |
Type used to hold the components of the tensors in the array. For DistArray<Tensor<double>> this will be double. Similarly for DistArray<Tensor<Tensor<double>> this will also be double. Notably for complex elements of type std::complex<T> numeric_type will be std::complex<T> and scalar_type will be T.
Definition at line 69 of file dist_array.h.
◆ ordinal_type
typedef impl_type::ordinal_type TiledArray::DistArray< Tile, Policy >::ordinal_type |
Ordinal type.
Definition at line 85 of file dist_array.h.
◆ pmap_interface
typedef impl_type::pmap_interface TiledArray::DistArray< Tile, Policy >::pmap_interface |
Process map interface type.
Definition at line 97 of file dist_array.h.
◆ policy_type
typedef impl_type::policy_type TiledArray::DistArray< Tile, Policy >::policy_type |
Policy type.
Definition at line 62 of file dist_array.h.
◆ range_type
typedef impl_type::range_type TiledArray::DistArray< Tile, Policy >::range_type |
Elements/tiles range type.
Definition at line 78 of file dist_array.h.
◆ reference
typedef impl_type::reference TiledArray::DistArray< Tile, Policy >::reference |
future
type
Definition at line 90 of file dist_array.h.
◆ scalar_type
typedef detail::scalar_type<Tile>::type TiledArray::DistArray< Tile, Policy >::scalar_type |
Type used to hold the scalar components of numeric_type. For real-valued arrays scalar_type will be the same as numeric_type; however, for arrays with elements of type std::complex<T> scalar_type will be T.
Definition at line 74 of file dist_array.h.
◆ shape_type
typedef impl_type::shape_type TiledArray::DistArray< Tile, Policy >::shape_type |
Shape type for array tiling.
Definition at line 80 of file dist_array.h.
◆ trange_type
typedef impl_type::trange_type TiledArray::DistArray< Tile, Policy >::trange_type |
Tile range type.
Definition at line 76 of file dist_array.h.
◆ value_type
typedef impl_type::value_type TiledArray::DistArray< Tile, Policy >::value_type |
Tile type.
Definition at line 86 of file dist_array.h.
Constructor & Destructor Documentation
◆ DistArray() [1/18]
|
inline |
Default constructor.
Constructs an uninitialized array object. Uninitialized arrays contain no tile or meta data. Most of the functions are not available when the array is uninitialized, but these arrays may be assign via a tensor expression assignment or the copy construction.
Definition at line 242 of file dist_array.h.
◆ DistArray() [2/18]
|
inline |
Copy constructor.
This is a shallow copy, that is no data is copied.
- Parameters
-
other The array to be copied
Definition at line 248 of file dist_array.h.
◆ DistArray() [3/18]
|
inline |
Dense array constructor.
Constructs an array with the given meta data. This constructor only initializes the array meta data; the array tiles are empty and must be assigned by the user.
- Parameters
-
world The world where the array will live. trange The tiled range object that will be used to set the array tiling. pmap The tile index -> process map
Definition at line 258 of file dist_array.h.
◆ DistArray() [4/18]
|
inline |
Sparse array constructor.
Constructs an array with the given meta data. This constructor only initializes the array meta data; the array tiles are empty and must be assigned by the user.
- Parameters
-
world The world where the array will live. trange The tiled range object that will be used to set the array tiling. shape The array shape that defines zero and non-zero tiles pmap The tile index -> process map
Definition at line 272 of file dist_array.h.
◆ DistArray() [5/18]
|
inline |
This ctor will create an array comprised of a single tile. The array will have a rank equal to the nesting of il
and the elements will be those in the provided std::initializer_list
. This ctor can not be used to create an empty tensor (attempts to do so will raise an error).
- Template Parameters
-
T The types of the elements in the std::initializer_list
. Must be implicitly convertible to numeric_type.
- Parameters
-
[in] world The world where the resulting array will live. [in] il The initial values for the elements in the array. The elements are assumed to be listed in row-major order.
- Exceptions
-
TiledArray::Exception if il
contains no elements. If an exception is raisedworld
andil
are unchanged (strong throw guarantee).TiledArray::Exception If the provided std::initializer_list
is not rectangular (e.g., attempting to initialize a matrix with the value{{1, 2}, {3, 4, 5}}
). If an exception is raisedworld
andil
are unchanged.
Definition at line 303 of file dist_array.h.
◆ DistArray() [6/18]
|
inline |
This ctor will create an array comprised of a single tile. The array will have a rank equal to the nesting of il
and the elements will be those in the provided std::initializer_list
. This ctor can not be used to create an empty tensor (attempts to do so will raise an error).
- Template Parameters
-
T The types of the elements in the std::initializer_list
. Must be implicitly convertible to numeric_type.
- Parameters
-
[in] world The world where the resulting array will live. [in] il The initial values for the elements in the array. The elements are assumed to be listed in row-major order.
- Exceptions
-
TiledArray::Exception if il
contains no elements. If an exception is raisedworld
andil
are unchanged (strong throw guarantee).TiledArray::Exception If the provided std::initializer_list
is not rectangular (e.g., attempting to initialize a matrix with the value{{1, 2}, {3, 4, 5}}
). If an exception is raisedworld
andil
are unchanged.
Definition at line 307 of file dist_array.h.
◆ DistArray() [7/18]
|
inline |
This ctor will create an array comprised of a single tile. The array will have a rank equal to the nesting of il
and the elements will be those in the provided std::initializer_list
. This ctor can not be used to create an empty tensor (attempts to do so will raise an error).
- Template Parameters
-
T The types of the elements in the std::initializer_list
. Must be implicitly convertible to numeric_type.
- Parameters
-
[in] world The world where the resulting array will live. [in] il The initial values for the elements in the array. The elements are assumed to be listed in row-major order.
- Exceptions
-
TiledArray::Exception if il
contains no elements. If an exception is raisedworld
andil
are unchanged (strong throw guarantee).TiledArray::Exception If the provided std::initializer_list
is not rectangular (e.g., attempting to initialize a matrix with the value{{1, 2}, {3, 4, 5}}
). If an exception is raisedworld
andil
are unchanged.
Definition at line 311 of file dist_array.h.
◆ DistArray() [8/18]
|
inline |
This ctor will create an array comprised of a single tile. The array will have a rank equal to the nesting of il
and the elements will be those in the provided std::initializer_list
. This ctor can not be used to create an empty tensor (attempts to do so will raise an error).
- Template Parameters
-
T The types of the elements in the std::initializer_list
. Must be implicitly convertible to numeric_type.
- Parameters
-
[in] world The world where the resulting array will live. [in] il The initial values for the elements in the array. The elements are assumed to be listed in row-major order.
- Exceptions
-
TiledArray::Exception if il
contains no elements. If an exception is raisedworld
andil
are unchanged (strong throw guarantee).TiledArray::Exception If the provided std::initializer_list
is not rectangular (e.g., attempting to initialize a matrix with the value{{1, 2}, {3, 4, 5}}
). If an exception is raisedworld
andil
are unchanged.
Definition at line 315 of file dist_array.h.
◆ DistArray() [9/18]
|
inline |
This ctor will create an array comprised of a single tile. The array will have a rank equal to the nesting of il
and the elements will be those in the provided std::initializer_list
. This ctor can not be used to create an empty tensor (attempts to do so will raise an error).
- Template Parameters
-
T The types of the elements in the std::initializer_list
. Must be implicitly convertible to numeric_type.
- Parameters
-
[in] world The world where the resulting array will live. [in] il The initial values for the elements in the array. The elements are assumed to be listed in row-major order.
- Exceptions
-
TiledArray::Exception if il
contains no elements. If an exception is raisedworld
andil
are unchanged (strong throw guarantee).TiledArray::Exception If the provided std::initializer_list
is not rectangular (e.g., attempting to initialize a matrix with the value{{1, 2}, {3, 4, 5}}
). If an exception is raisedworld
andil
are unchanged.
Definition at line 319 of file dist_array.h.
◆ DistArray() [10/18]
|
inline |
This ctor will create an array comprised of a single tile. The array will have a rank equal to the nesting of il
and the elements will be those in the provided std::initializer_list
. This ctor can not be used to create an empty tensor (attempts to do so will raise an error).
- Template Parameters
-
T The types of the elements in the std::initializer_list
. Must be implicitly convertible to numeric_type.
- Parameters
-
[in] world The world where the resulting array will live. [in] il The initial values for the elements in the array. The elements are assumed to be listed in row-major order.
- Exceptions
-
TiledArray::Exception if il
contains no elements. If an exception is raisedworld
andil
are unchanged (strong throw guarantee).TiledArray::Exception If the provided std::initializer_list
is not rectangular (e.g., attempting to initialize a matrix with the value{{1, 2}, {3, 4, 5}}
). If an exception is raisedworld
andil
are unchanged.
Definition at line 323 of file dist_array.h.
◆ DistArray() [11/18]
|
inline |
This ctor will create an array using the provided TiledRange instance whose values will be initialized from the provided std::initializer_list
il
. This ctor can not be used to create an empty tensor (attempts to do so will raise an error).
- Template Parameters
-
T The types of the elements in the std::initializer_list
. Must be implicitly convertible to numeric_type.
- Parameters
-
[in] world The world where the resulting array will live. [in] trange The tiling to use for the resulting array. [in] il The initial values for the elements in the array. The elements are assumed to be listed in row-major order.
- Exceptions
-
TiledArray::Exception if il
contains no elements. If an exception is raisedworld
andil
are unchanged (strong throw guarantee).TiledArray::Exception If the provided std::initializer_list
is not rectangular (e.g., attempting to initialize a matrix with the value{{1, 2}, {3, 4, 5}}
). If an exception is raisedworld
andil
are unchanged.
Definition at line 354 of file dist_array.h.
◆ DistArray() [12/18]
|
inline |
This ctor will create an array using the provided TiledRange instance whose values will be initialized from the provided std::initializer_list
il
. This ctor can not be used to create an empty tensor (attempts to do so will raise an error).
- Template Parameters
-
T The types of the elements in the std::initializer_list
. Must be implicitly convertible to numeric_type.
- Parameters
-
[in] world The world where the resulting array will live. [in] trange The tiling to use for the resulting array. [in] il The initial values for the elements in the array. The elements are assumed to be listed in row-major order.
- Exceptions
-
TiledArray::Exception if il
contains no elements. If an exception is raisedworld
andil
are unchanged (strong throw guarantee).TiledArray::Exception If the provided std::initializer_list
is not rectangular (e.g., attempting to initialize a matrix with the value{{1, 2}, {3, 4, 5}}
). If an exception is raisedworld
andil
are unchanged.
Definition at line 358 of file dist_array.h.
◆ DistArray() [13/18]
|
inline |
This ctor will create an array using the provided TiledRange instance whose values will be initialized from the provided std::initializer_list
il
. This ctor can not be used to create an empty tensor (attempts to do so will raise an error).
- Template Parameters
-
T The types of the elements in the std::initializer_list
. Must be implicitly convertible to numeric_type.
- Parameters
-
[in] world The world where the resulting array will live. [in] trange The tiling to use for the resulting array. [in] il The initial values for the elements in the array. The elements are assumed to be listed in row-major order.
- Exceptions
-
TiledArray::Exception if il
contains no elements. If an exception is raisedworld
andil
are unchanged (strong throw guarantee).TiledArray::Exception If the provided std::initializer_list
is not rectangular (e.g., attempting to initialize a matrix with the value{{1, 2}, {3, 4, 5}}
). If an exception is raisedworld
andil
are unchanged.
Definition at line 362 of file dist_array.h.
◆ DistArray() [14/18]
|
inline |
This ctor will create an array using the provided TiledRange instance whose values will be initialized from the provided std::initializer_list
il
. This ctor can not be used to create an empty tensor (attempts to do so will raise an error).
- Template Parameters
-
T The types of the elements in the std::initializer_list
. Must be implicitly convertible to numeric_type.
- Parameters
-
[in] world The world where the resulting array will live. [in] trange The tiling to use for the resulting array. [in] il The initial values for the elements in the array. The elements are assumed to be listed in row-major order.
- Exceptions
-
TiledArray::Exception if il
contains no elements. If an exception is raisedworld
andil
are unchanged (strong throw guarantee).TiledArray::Exception If the provided std::initializer_list
is not rectangular (e.g., attempting to initialize a matrix with the value{{1, 2}, {3, 4, 5}}
). If an exception is raisedworld
andil
are unchanged.
Definition at line 366 of file dist_array.h.
◆ DistArray() [15/18]
|
inline |
This ctor will create an array using the provided TiledRange instance whose values will be initialized from the provided std::initializer_list
il
. This ctor can not be used to create an empty tensor (attempts to do so will raise an error).
- Template Parameters
-
T The types of the elements in the std::initializer_list
. Must be implicitly convertible to numeric_type.
- Parameters
-
[in] world The world where the resulting array will live. [in] trange The tiling to use for the resulting array. [in] il The initial values for the elements in the array. The elements are assumed to be listed in row-major order.
- Exceptions
-
TiledArray::Exception if il
contains no elements. If an exception is raisedworld
andil
are unchanged (strong throw guarantee).TiledArray::Exception If the provided std::initializer_list
is not rectangular (e.g., attempting to initialize a matrix with the value{{1, 2}, {3, 4, 5}}
). If an exception is raisedworld
andil
are unchanged.
Definition at line 370 of file dist_array.h.
◆ DistArray() [16/18]
|
inline |
This ctor will create an array using the provided TiledRange instance whose values will be initialized from the provided std::initializer_list
il
. This ctor can not be used to create an empty tensor (attempts to do so will raise an error).
- Template Parameters
-
T The types of the elements in the std::initializer_list
. Must be implicitly convertible to numeric_type.
- Parameters
-
[in] world The world where the resulting array will live. [in] trange The tiling to use for the resulting array. [in] il The initial values for the elements in the array. The elements are assumed to be listed in row-major order.
- Exceptions
-
TiledArray::Exception if il
contains no elements. If an exception is raisedworld
andil
are unchanged (strong throw guarantee).TiledArray::Exception If the provided std::initializer_list
is not rectangular (e.g., attempting to initialize a matrix with the value{{1, 2}, {3, 4, 5}}
). If an exception is raisedworld
andil
are unchanged.
Definition at line 374 of file dist_array.h.
◆ DistArray() [17/18]
|
inlineexplicit |
converting copy constructor
This constructor uses the meta data of other
to initialize the meta data of the new array. In addition, the tiles of the new array are also initialized using TiledArray::Cast<Tile,OtherTile>
- Parameters
-
other The array to be copied
Definition at line 385 of file dist_array.h.
◆ DistArray() [18/18]
|
inline |
Unary transform constructor.
This constructor uses the meta data of other
to initialize the meta data of the new array. In addition, the tiles of the new array are also initialized using the op
function/functor, which transforms each tile in other
using op
- Parameters
-
other The array to be copied
Definition at line 399 of file dist_array.h.
◆ ~DistArray()
|
inline |
Destructor.
This is a distributed lazy destructor. The object will only be deleted after the last reference to the world object on all nodes has been destroyed.
Definition at line 408 of file dist_array.h.
Member Function Documentation
◆ begin() [1/2]
|
inline |
Begin iterator factory function.
- Returns
- An iterator to the first local tile.
- Exceptions
-
TiledArray::Exception if the PIMPL has not been initialized. Strong throw guarantee.
Definition at line 484 of file dist_array.h.
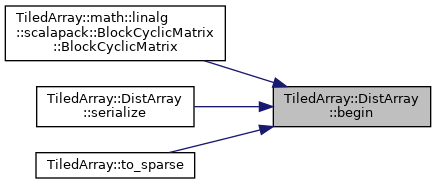
◆ begin() [2/2]
|
inline |
Begin const iterator factory function.
- Returns
- A const iterator to the first local tile.
- Exceptions
-
Tiledarray::Exception if the PIMPL has not been initialized. Strong throw guarantee.
Definition at line 491 of file dist_array.h.
◆ clone()
|
inline |
Create a deep copy of this array.
- Returns
- An array that is equal to this array
Definition at line 413 of file dist_array.h.
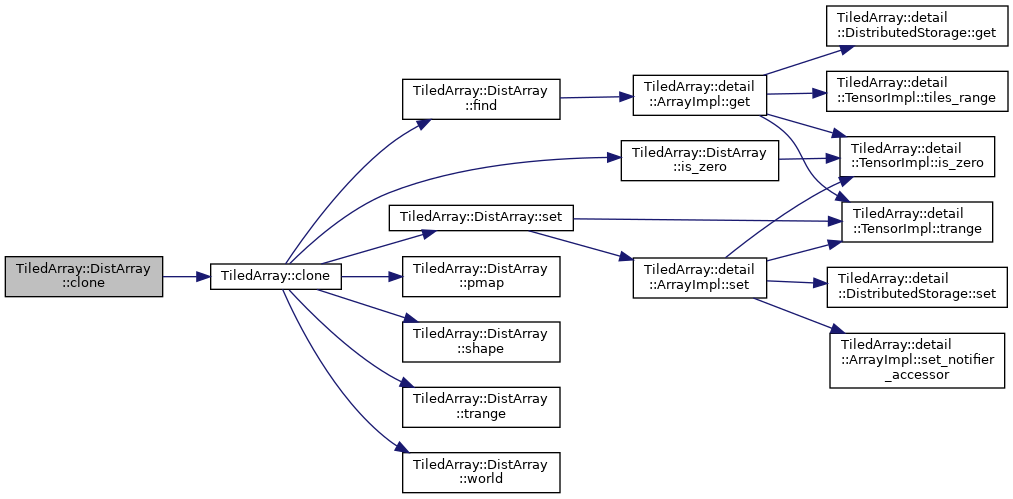
◆ elements()
|
inline |
◆ elements_range()
|
inline |
Element range accessor.
This function returns a Range object which can be used to iterate over the indices of the tiles' elements. This should not be confused with trange()
which returns an object containing the tiling information (e.g., where the tile boundaries are, or which element is in which tile) or range()
which returns an object for iterating over the indices of the tiles.
- Returns
- A const reference to the range object for the array's elements
- Exceptions
-
TiledArray::Exception if the PIMPL is not initialized. Strong throw guarantee.
Definition at line 958 of file dist_array.h.

◆ end() [1/2]
|
inline |
End iterator factory function.
- Returns
- An iterator to one past the last local tile.
- Exceptions
-
TiledArray::Exception if the PIMPL has not been initialized. Strong throw guarantee.
Definition at line 498 of file dist_array.h.
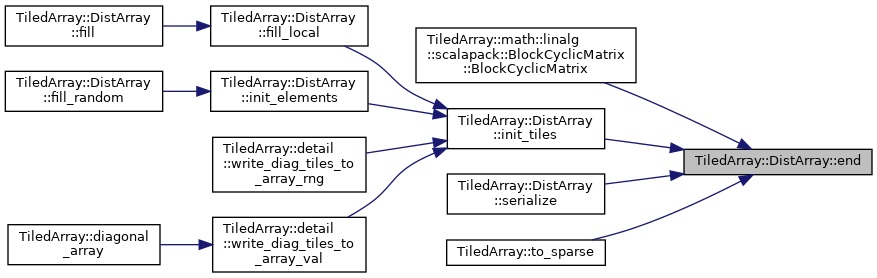
◆ end() [2/2]
|
inline |
End const iterator factory function.
- Returns
- A const iterator to one past the last local tile.
- Exceptions
-
TiledArray::Exception if the PIMPL has not been initialized. Strong throw guarantee.
Definition at line 505 of file dist_array.h.
◆ fill()
|
inline |
Fill all local tiles with the specified value.
- Parameters
-
[in] value What each local tile should be filled with. [in] skip_set If false, will throw if any tiles are already set
- Exceptions
-
TiledArray::Exception if the PIMPL is uninitialized. Strong throw guarantee. TiledArray::Exception if skip_set is false and a local tile is already set. Weak throw guarantee.
Definition at line 765 of file dist_array.h.
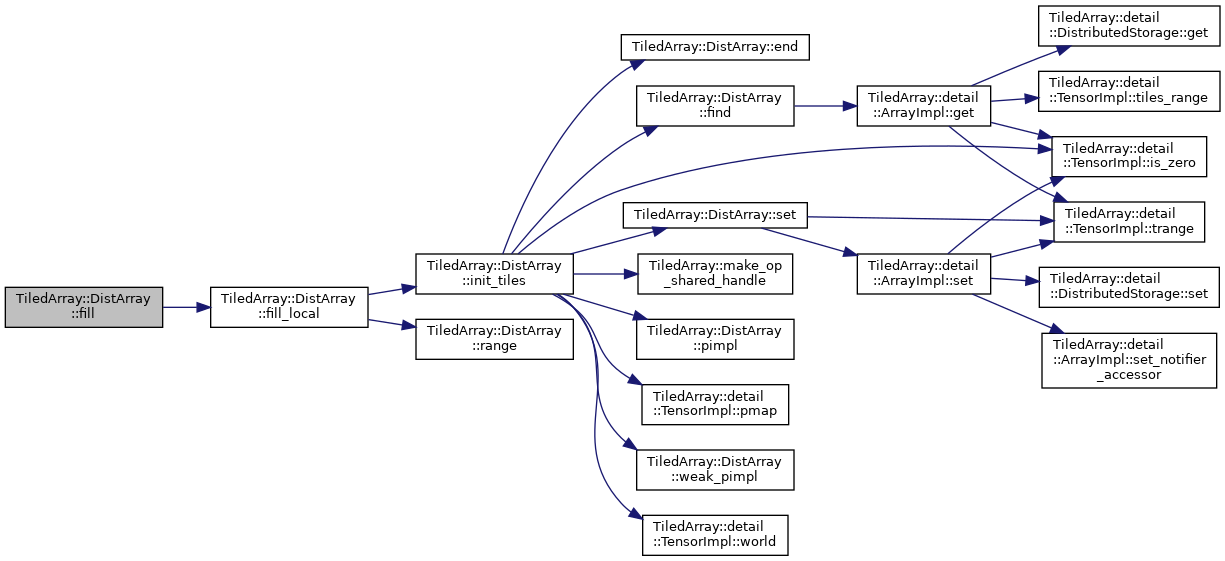
◆ fill_local()
|
inline |
Fill all local tiles with the specified value.
- Parameters
-
[in] value What each local tile should be filled with. [in] skip_set If false, will throw if any tiles are already set
- Exceptions
-
TiledArray::Exception if the PIMPL is uninitialized. Strong throw guarantee. TiledArray::Exception if skip_set is false and a local tile is already set. Weak throw guarantee.
Definition at line 750 of file dist_array.h.
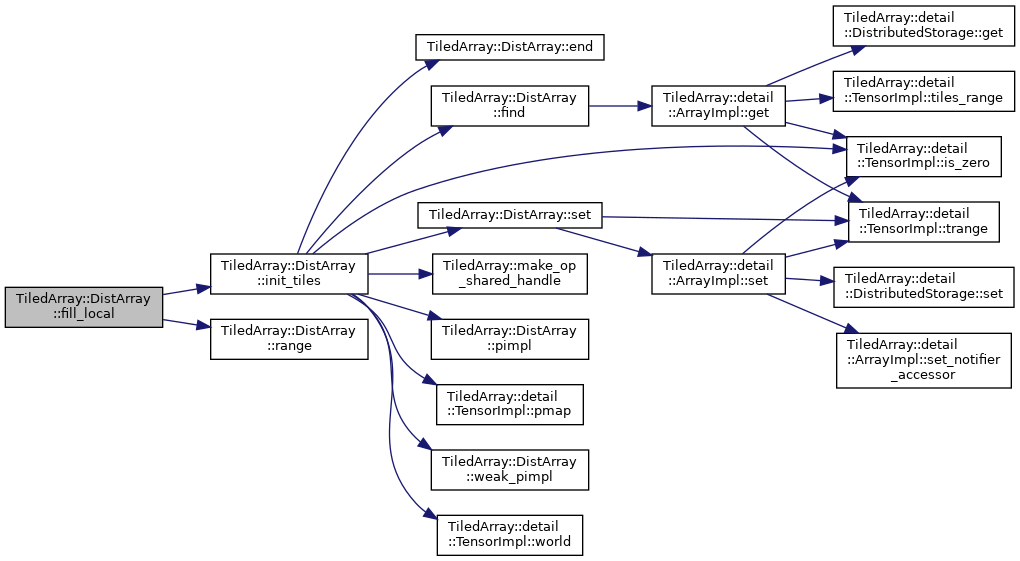

◆ fill_random()
|
inline |
Fill all local tiles with random values
This function will fill all local tiles with random values. The random values are generated by calling TiledArray::detail::MakeRandom, which can be specialized to determine how the random values for a given type are generated. It should be noted that if MakeRandom does not know how to generate random values of type T this function will be disabled via SFINAE and attempting to use it will lead to a compile-time error.
- Template Parameters
-
T The type of random value to generate. Defaults to element_type. <anonymous> A template type parameter which will be deduced as void only if MakeRandom knows how to generate random values of type T. If MakeRandom does not know how to generate random values of type T, SFINAE will disable this function.
- Parameters
-
[in] skip_set If false, will throw if any tiles are already set
- Exceptions
-
TiledArray::Exception if the PIMPL is not initialized. Strong throw guarantee. TiledArray::Exception if skip_set is false and a local tile is already initialized. Weak throw guarantee.
Definition at line 792 of file dist_array.h.
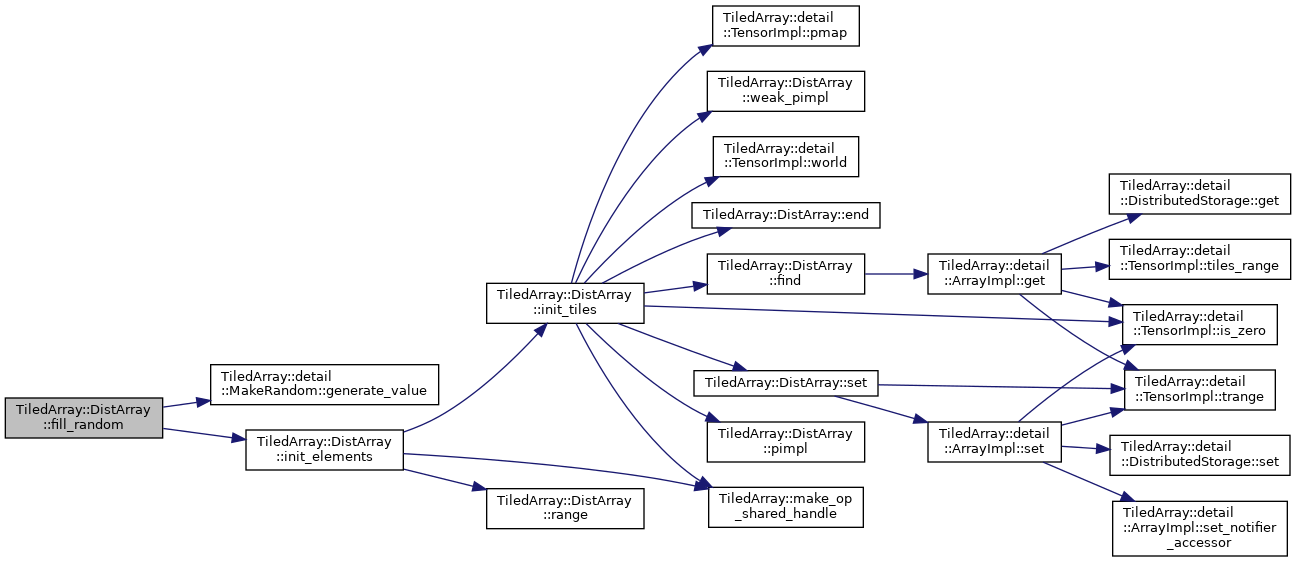
◆ find() [1/2]
|
inline |
Find local or remote tile by index.
- Template Parameters
-
Index The type of the index. Should be an integral type for an ordinal index, a type satisfying container of integral instances for a coordinate index, or an integral range type.
- Parameters
-
[in] i The ordinal or coordinate index of the desired tile or a range or indices.
- Returns
- A
future
to tilei
- Exceptions
-
TiledArray::Exception When tile i
is zeroTiledArray::Exception If PIMPL is not initialized. Strong throw guarantee. TiledArray::Exception if index i
is out of bounds. Strong throw guarantee.TiledArray::Exception if index i
is a coordinate index with the wrong rank. Strong throw guarantee.
Definition at line 524 of file dist_array.h.
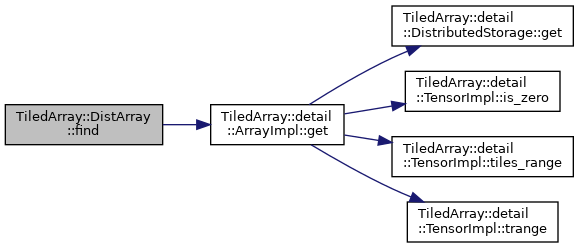
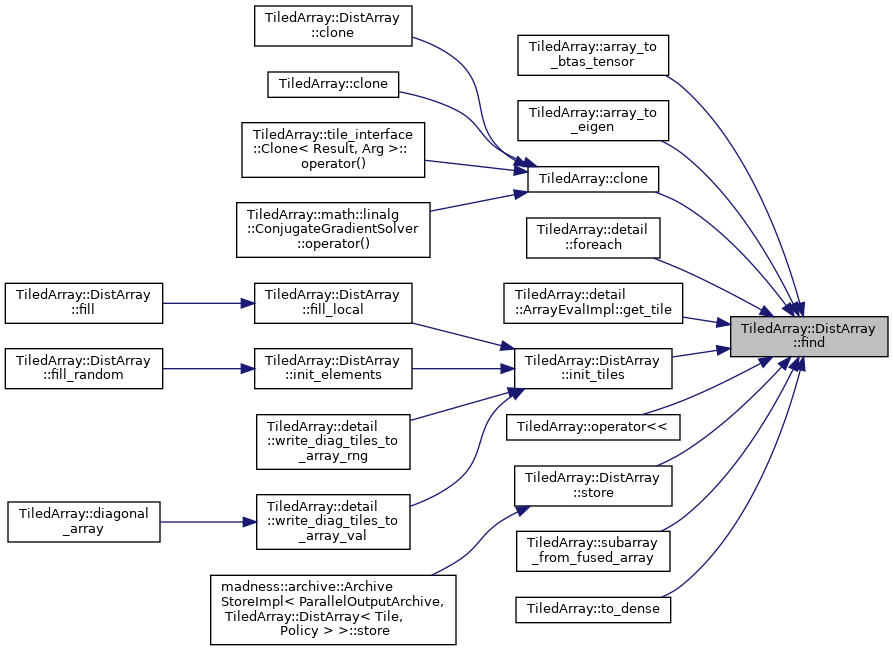
◆ find() [2/2]
|
inline |
Find local or remote tile.
- Template Parameters
-
Integer An integer type
- Parameters
-
i An std::initializer_list<Integer>
indicating the coordinate index of the requested tile.
- Returns
- A
future
to tilei
- Exceptions
-
TiledArray::Exception When tile i
is zeroTiledArray::Exception If PIMPL is not initialized. Strong throw guarantee. TiledArray::Exception if index i
is out of bounds. Strong throw guarantee.TiledArray::Exception if index i
is a coordinate index with the wrong rank. Strong throw guarantee.
Definition at line 543 of file dist_array.h.
◆ find_local() [1/4]
|
inline |
Find local tile.
- Template Parameters
-
Index An integral or integral range type
- Parameters
-
i The tile index
- Returns
- A
future
to tilei
- Exceptions
-
TiledArray::Exception When tile i
is zero or not local
Definition at line 583 of file dist_array.h.
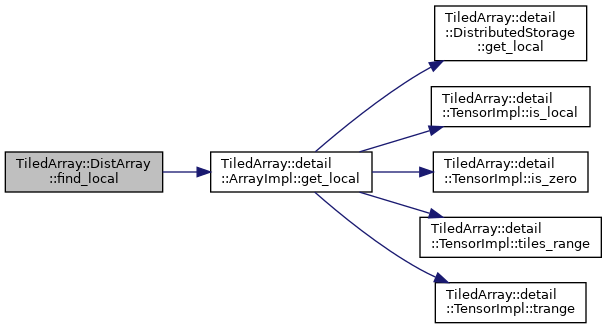
◆ find_local() [2/4]
|
inline |
Find local tile.
- Template Parameters
-
Index An integral or integral range type
- Parameters
-
i The tile index
- Returns
- A
future
to tilei
- Exceptions
-
TiledArray::Exception When tile i
is zero or not local
Definition at line 556 of file dist_array.h.
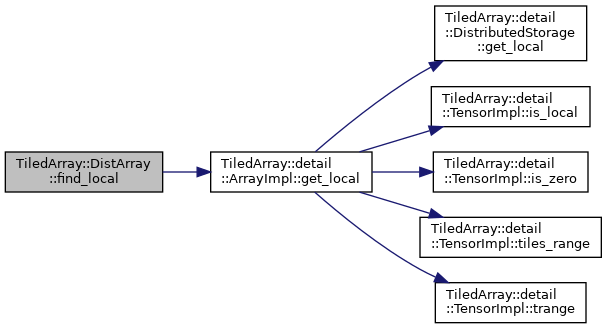
◆ find_local() [3/4]
|
inline |
Find local tile.
- Template Parameters
-
Integer An integral type
- Parameters
-
i The tile index, as an std::initializer_list<Integer>
- Returns
- A
future
to tilei
- Exceptions
-
TiledArray::Exception When tile i
is zero or not local
Definition at line 596 of file dist_array.h.
◆ find_local() [4/4]
|
inline |
Find local tile.
- Template Parameters
-
Integer An integral type
- Parameters
-
i The tile index, as an std::initializer_list<Integer>
- Returns
- A
future
to tilei
- Exceptions
-
TiledArray::Exception When tile i
is zero or not local
Definition at line 569 of file dist_array.h.
◆ get_pmap()
|
inline |
◆ get_shape()
|
inline |
◆ get_world()
|
inline |
◆ id()
|
inline |
Global object id.
- Returns
- A globally unique identifier.
- Exceptions
-
TiledArray::Exception if the PIMPL has not been initialized.
- Note
- This function is primarily used for debugging purposes. Users should not rely on this function.
Definition at line 477 of file dist_array.h.
◆ init_elements()
|
inline |
Initialize elements of local, non-zero tiles with a user provided functor.
This function is used to initialize the elements of the local, non-zero tiles via a function (or functor). The work is done in parallel, therefore op
must be a thread safe function/functor. The signature of the functor should be:
For example, in the following code, the array elements are initialized with random numbers from 0 to 1:
- Template Parameters
-
Op Type of the function/functor which will generate the elements.
- Parameters
-
[in] op The operation used to generate elements [in] skip_set If false, will throw if any tiles are already set
- Exceptions
-
TiledArray::Exception if the PIMPL is not initialized. Strong throw guarnatee. TiledArray::Exception if skip_set is false and a local, non-zero tile is already initialized. Weak throw guarantee.
Definition at line 890 of file dist_array.h.
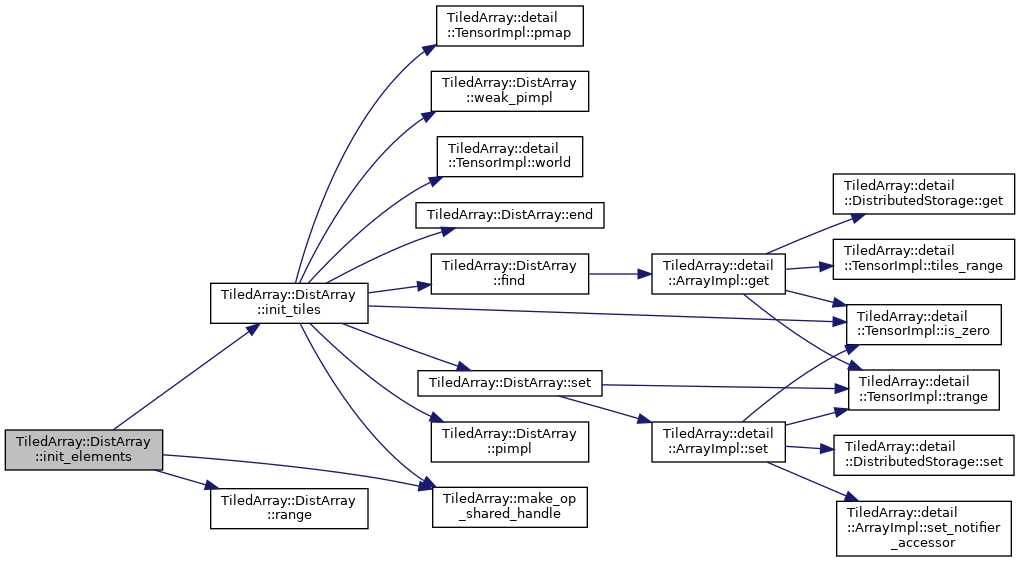

◆ init_tiles()
|
inline |
Initialize (local) tiles with a user provided functor.
This function is used to initialize the local, non-zero tiles of the array via a function (or functor). The work is done in parallel, therefore op
must be a thread safe function/functor. The signature of the functor should be:
For example, in the following code, the array tiles are initialized with random numbers from 0 to 1:
- Template Parameters
-
Op The type of the functor/function
- Parameters
-
[in] op The operation used to generate tiles [in] skip_set If false, will throw if any tiles are already set
- Exceptions
-
TiledArray::Exception if the PIMPL is not set. Strong throw guarantee. TiledArray::Exception if a tile is already set and skip_set is false. Weak throw guarantee.
Definition at line 834 of file dist_array.h.
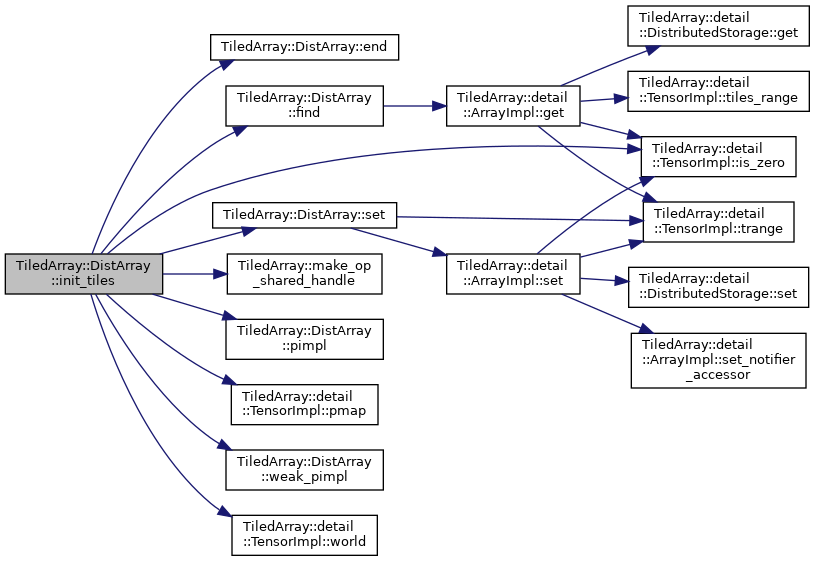
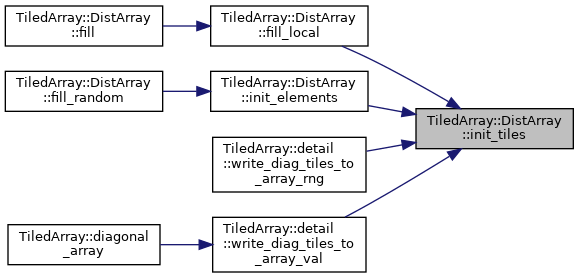
◆ is_dense()
|
inline |
Check dense/sparse.
- Returns
true
whenArray
is dense,false
otherwise.
- Exceptions
-
TiledArray::Exception if the PIMPL is not initialized. Strong throw guarantee.
Definition at line 1028 of file dist_array.h.
◆ is_initialized()
|
inline |
Check if the array is initialized.
- Returns
false
if the array has been default initialized, otherwisetrue
.
- Exceptions
-
None No throw guarantee.
Definition at line 1206 of file dist_array.h.

◆ is_local() [1/2]
|
inline |
Check if the tile at index i
is stored locally.
- Template Parameters
-
Index An integral type, a type satisfying container of integral types, or an integral range type.
- Parameters
-
[in] i The index of the tile whose locality is being questioned. The index may be either a coordinate or ordinal index.
- Returns
true
ifowner(i)
is equal to the MPI process rank, otherwisefalse
.
- Exceptions
-
TiledArray::Exception if the PIMPL is not initialized. Strong throw guarantee. TiledArray::Exception if the index is out of bounds. Strong throw guarantee. TiledArray::Exception if i
is a coordinate index with the wrong rank. Strong throw guarantee.
Definition at line 1098 of file dist_array.h.


◆ is_local() [2/2]
|
inline |
Check if the tile at index i
is stored locally.
- Template Parameters
-
Index An integral type
- Parameters
-
[in] i The coordinate index of the tile whose locality is being questioned.
- Returns
true
ifowner(i)
is equal to the MPI process rank, otherwisefalse
.
- Exceptions
-
TiledArray::Exception if the PIMPL is not initialized. Strong throw guarantee. TiledArray::Exception if the index is out of bounds. Strong throw guarantee. TiledArray::Exception if i
has the wrong rank. Strong throw guarantee.
Definition at line 1117 of file dist_array.h.
◆ is_zero() [1/2]
|
inline |
Check for zero tiles.
- Template Parameters
-
Index An integral type, a type satisfying container of integral types, or an integral range type.
- Parameters
-
[in] i The index of the tile whose nothingness is being contemplated. Can be either an ordinal or coordinate index.
- Returns
true
if tile at indexi
is zero, false if the tile is non-zero or remote existence data is not available.
- Exceptions
-
TiledArray::Exception if the PIMPL is not initialized. Strong throw guarantee. TiledArray::Exception if i
is not in the range of valid tile indices. Strong throw guarantee.TiledArray::Exception if i
is a coordinate index with the wrong rank. Strong throw guarantee.
Definition at line 1137 of file dist_array.h.

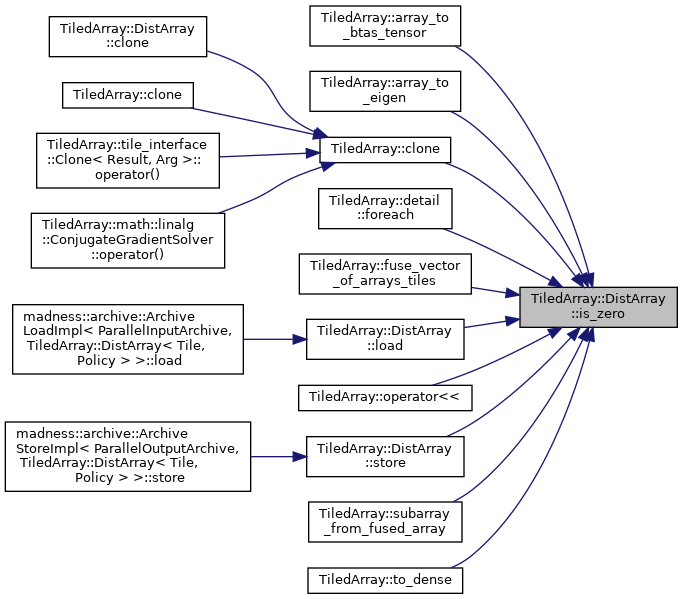
◆ is_zero() [2/2]
|
inline |
Check for zero tiles.
- Template Parameters
-
Index An integral type
- Parameters
-
[in] i The coordinate index of the tile whose nothingness is being contemplated.
- Returns
true
if tile at indexi
is zero, false if the tile is non-zero or remote existence data is not available.
- Exceptions
-
TiledArray::Exception if the PIMPL is not initialized. Strong throw guarantee. TiledArray::Exception if i
is not in the range of valid tile indices. Strong throw guarantee.TiledArray::Exception if i
has the wrong rank. Strong throw guarantee.
Definition at line 1156 of file dist_array.h.
◆ load()
|
inline |
Replaces this array with one loaded from an Archive object using the default processor map
- Template Parameters
-
Archive a parallel MADWorld Archive type
- Parameters
-
world a World object with which this object will be associated ar an Archive object from which this object's data will be read
- Note
- The & operator for serializing will only work with parallel MADWorld archives.
-
This is a collective operation that fences before and after completion, if
ar.dofence()
is true
Definition at line 1300 of file dist_array.h.
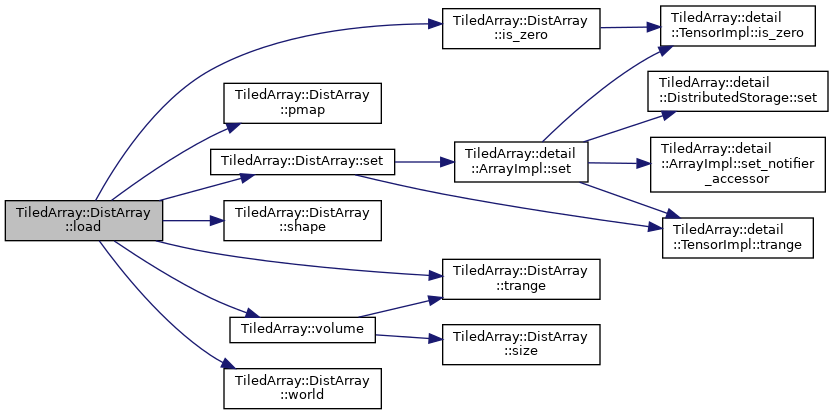

◆ make_replicated()
|
inline |
Convert a distributed array into a replicated array
- Exceptions
-
TiledArray::Exception if the PIMPL is not initialized. Strong throw guarantee.
Definition at line 1169 of file dist_array.h.
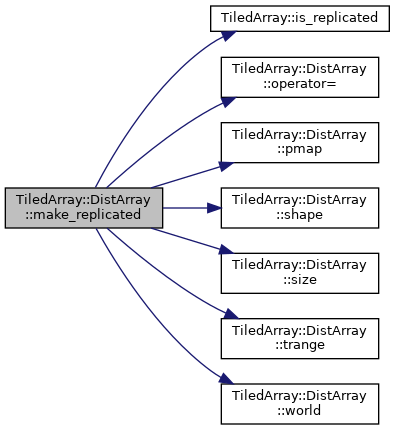
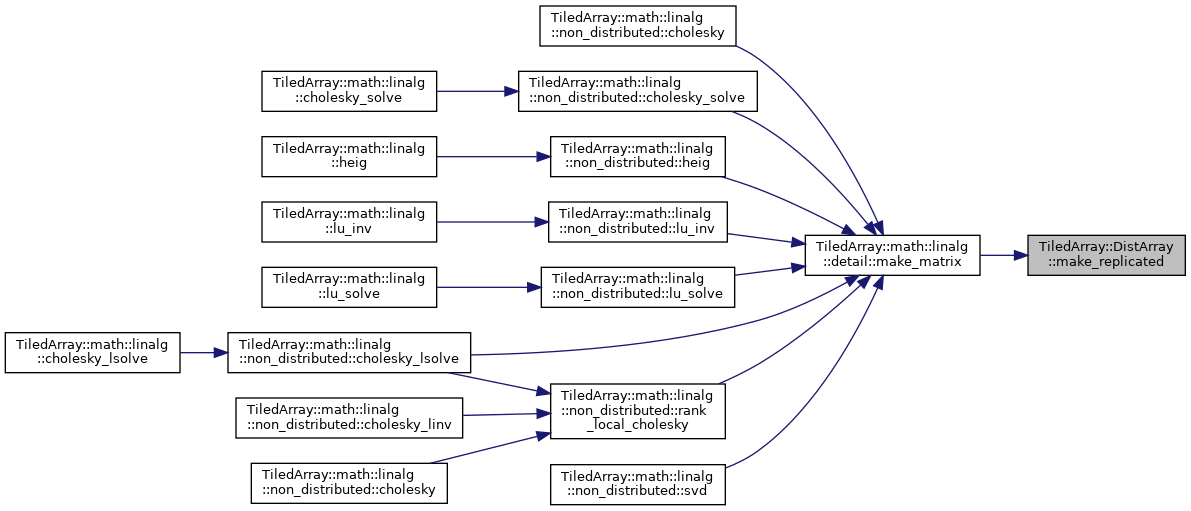
◆ operator bool()
|
inlineexplicit |
Conversion to bool is equivalent to DistArray::is_initialized()
- Returns
false
if the array has been default initialized, otherwisetrue
.
- Exceptions
-
None No throw guarantee.
Definition at line 1213 of file dist_array.h.

◆ operator()() [1/2]
|
inline |
Create a tensor expression.
- Parameters
-
vars A string with a comma-separated list of variables
- Returns
- A non-const tensor expression object
- Note
- size and contents of
vars
are validated using DistArray::check_str_index()
Definition at line 993 of file dist_array.h.
◆ operator()() [2/2]
|
inline |
Create a tensor expression.
- Parameters
-
vars A string with a comma-separated list of variables
- Returns
- A const tensor expression object
- Note
- size and contents of
vars
are validated using DistArray::check_str_index()
Definition at line 980 of file dist_array.h.
◆ operator=()
|
inline |
Copy assignment.
This is a shallow copy, that is no data is copied.
- Parameters
-
other The array to be copied
Definition at line 465 of file dist_array.h.
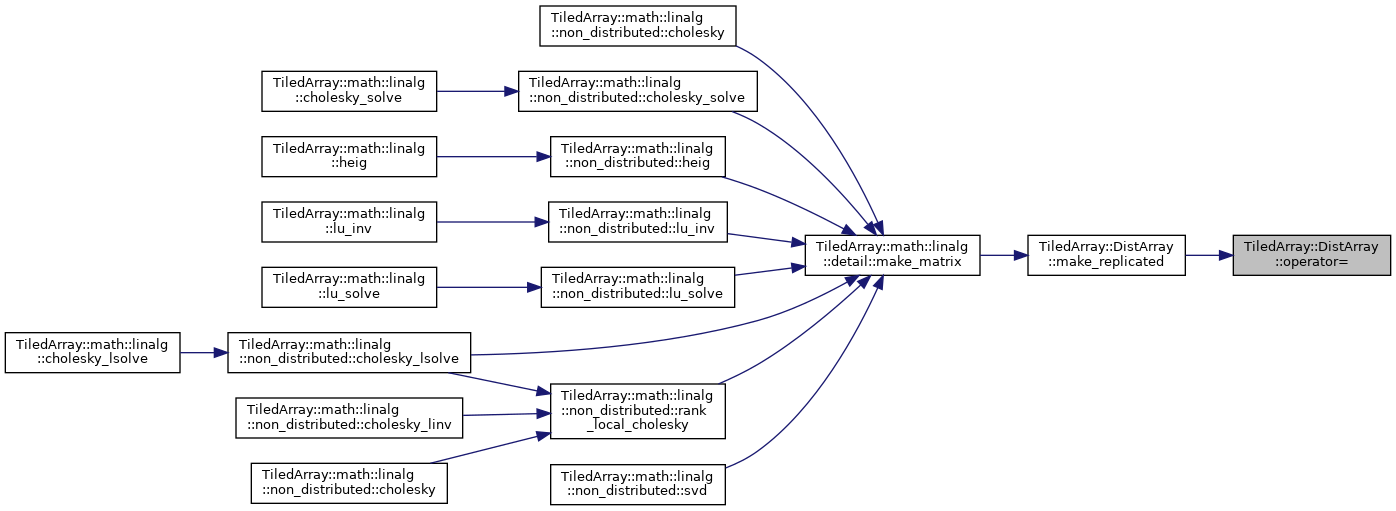
◆ owner() [1/2]
|
inline |
Tile ownership.
- Template Parameters
-
Index An integral type, a type satisfying container of integral types, or an integral range type.
- Parameters
-
[in] i The coordinate or ordinal index of the tile or a range of tiles whose ownership is in question.
- Returns
- The process ID of the owner of a tile.
- Note
- This does not indicate whether a tile exists or not. Only, the rank of the process that would own it if it does exist.
- Exceptions
-
TiledArray::Exception if the PIMPL is not initialized. Strong throw guarantee. TiledArray::Exception if the index is out of bounds. Strong throw guarantee. TiledArray::Exception if i
is a coordinate index with the wrong rank. Strong throw guarantee.
Definition at line 1058 of file dist_array.h.

◆ owner() [2/2]
|
inline |
Tile ownership.
- Template Parameters
-
Index An integral type
- Parameters
-
[in] i The coordinate index of the tile whose ownership is in question.
- Returns
- The process ID of the owner of a tile.
- Note
- This does not indicate whether a tile exists or not. Only, the rank of the process that would own it if it does exist.
- Exceptions
-
TiledArray::Exception if the PIMPL is not initialized. Strong throw guarantee. TiledArray::Exception if the index is out of bounds. Strong throw guarantee. TiledArray::Exception if i
has the wrong rank. Strong throw guarantee.
Definition at line 1078 of file dist_array.h.
◆ pimpl() [1/2]
|
inline |
Accessor for the (shared_ptr to) implementation object.
- Returns
- std::shared_ptr to the nonconst implementation object
Definition at line 423 of file dist_array.h.
◆ pimpl() [2/2]
|
inline |
Accessor for the (shared_ptr to) implementation object.
- Returns
- std::shared_ptr to the const implementation object
Definition at line 418 of file dist_array.h.
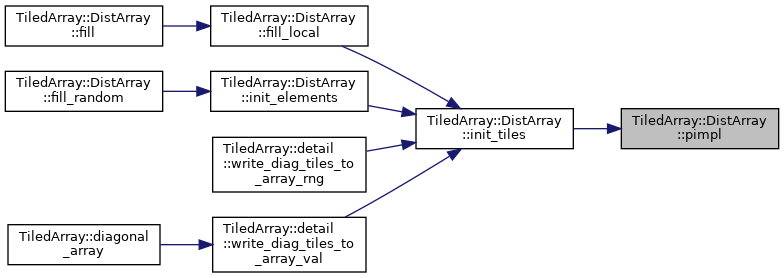
◆ pmap()
|
inline |
Process map accessor.
- Returns
- A reference to the process map that owns this array.
- Exceptions
-
TiledArray::Exception if the PIMPL is not initialized. Strong throw guarantee.
Definition at line 1019 of file dist_array.h.
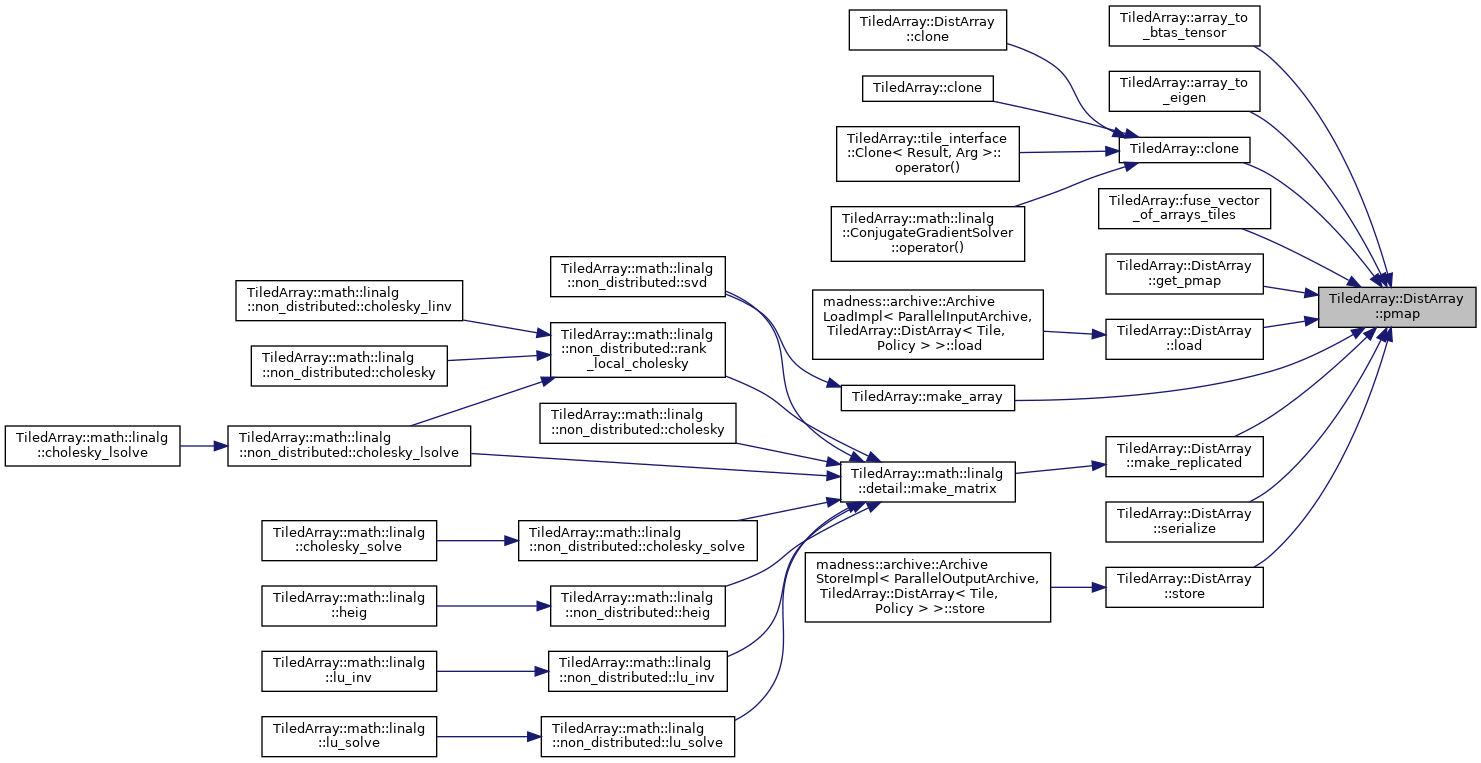
◆ range()
|
inline |
Tile range accessor.
This function returns a range object which can be used to iterate over the indices of the tiles. This should be contrasted with trange()
which gives an object holding the tiling information (number of tiles, which elements belong to which tiles, etc.) and elements_range()
which returns a range object that can be used to iterate over element indices.
- Returns
- A const reference to the range object for the array's tiles
- Exceptions
-
TiledArray::Exception if the PIMPL is not initialized. Strong throw guarantee.
Definition at line 932 of file dist_array.h.
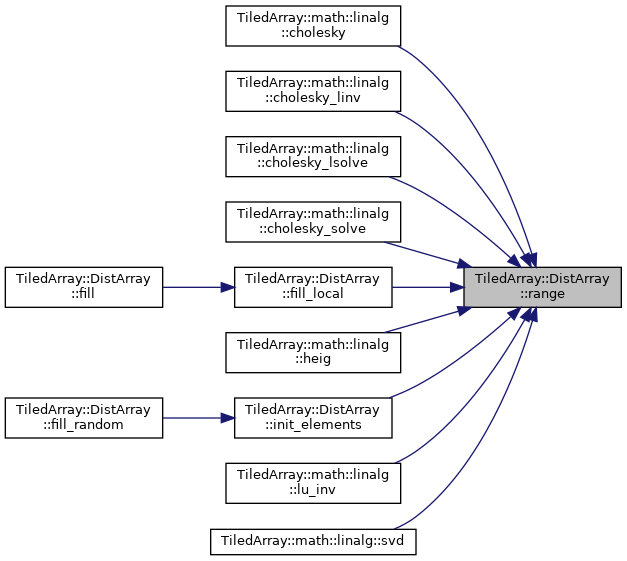
◆ register_set_notifier()
|
inlinestatic |
Debugging/tracing instrumentation.
registers notifier for set() calls
- Parameters
-
notifier the notifier callable that accepts ref to the implementation object that received set call and the corresponding ordinal index
Definition at line 1432 of file dist_array.h.
◆ serialize() [1/2]
|
inline |
deserialize local contents of a DistArray from an Archive object
- Note
- use Parallel{Input,Output}Archive for parallel serialization
- Template Parameters
-
Archive an Archive type
- Warning
- this does not fence; it is user's responsibility to do that
Definition at line 1244 of file dist_array.h.
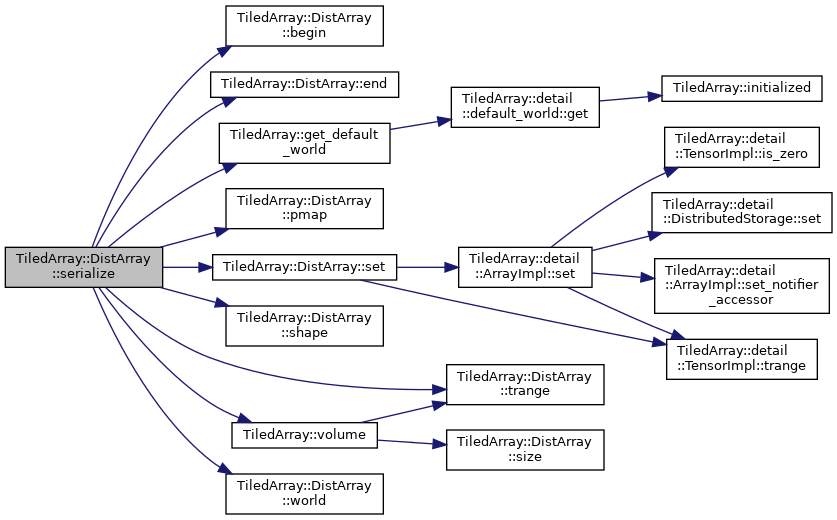
◆ serialize() [2/2]
|
inline |
serialize local contents of a DistArray to an Archive object
- Note
- use Parallel{Input,Output}Archive for parallel serialization
- Template Parameters
-
Archive an Archive type
- Warning
- this does not fence; it is user's responsibility to do that
Definition at line 1224 of file dist_array.h.
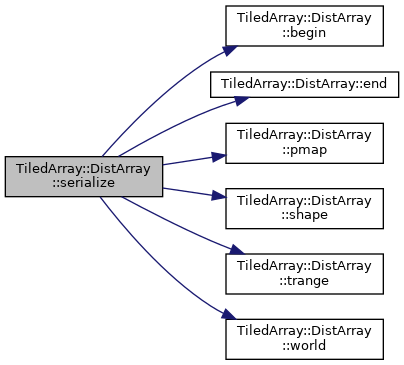
◆ set() [1/6]
|
inline |
Set a tile and fill it using a value.
This function sets each element of a tile to the specified value. For normal, non-nested, tiles this amounts to setting each scalar component of the tile to the provided value. For nested tile types this function sets the elements of the outer most tile (so the input value would be of type Tensor<T>, assuming a tile type of Tensor<Tensor<T>>).
- Template Parameters
-
Index An integral type, a type satisfying the concept of a container of integral types, or an integral range type.
- Parameters
-
[in] i Either the ordinal or coordinate index of the tile to be set. [in] value What each element of the tile will be set to.
- Exceptions
-
TiledArray::Exception If PIMPL is not initialized. Strong throw guarantee. TiledArray::Exception if index i
is out of bounds. Strong throw guarantee.TiledArray::Exception if index i
is a coordinate index with the wrong rank. Strong throw guarantee.TiledArray::Exception if tile i
is already set.
Definition at line 668 of file dist_array.h.
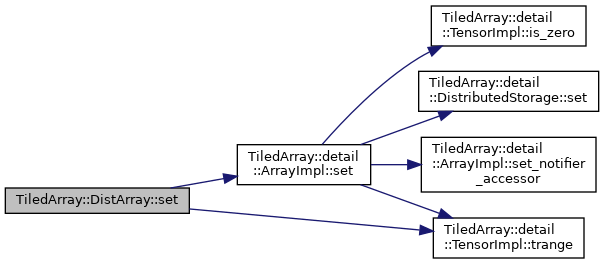
◆ set() [2/6]
|
inline |
Set a tile and fill it using a sequence
This function will set an uninitialized tile to the provided value. The tile may be specified either by ordinal or coordinate index.
- Template Parameters
-
Index Either an integral type, a container type with integral objects, or an integral range type. InIter Type satisfying input iterator
- Parameters
-
[in] i The index or the ordinal of the tile to be set. i may be either an ordinal or coordinate index. [in] first The iterator that points to the start of the input sequence. It is assumed that the container pointed to by first minimally contains the same number of elements as the tile.
- Exceptions
-
TiledArray::Exception if the tile is already initialized.
Definition at line 619 of file dist_array.h.
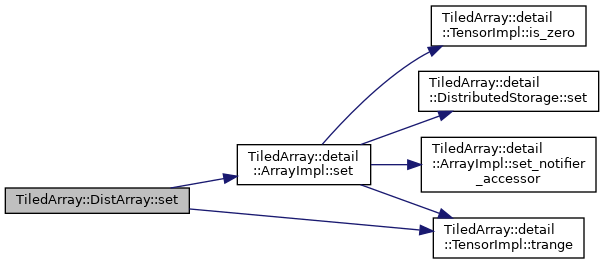
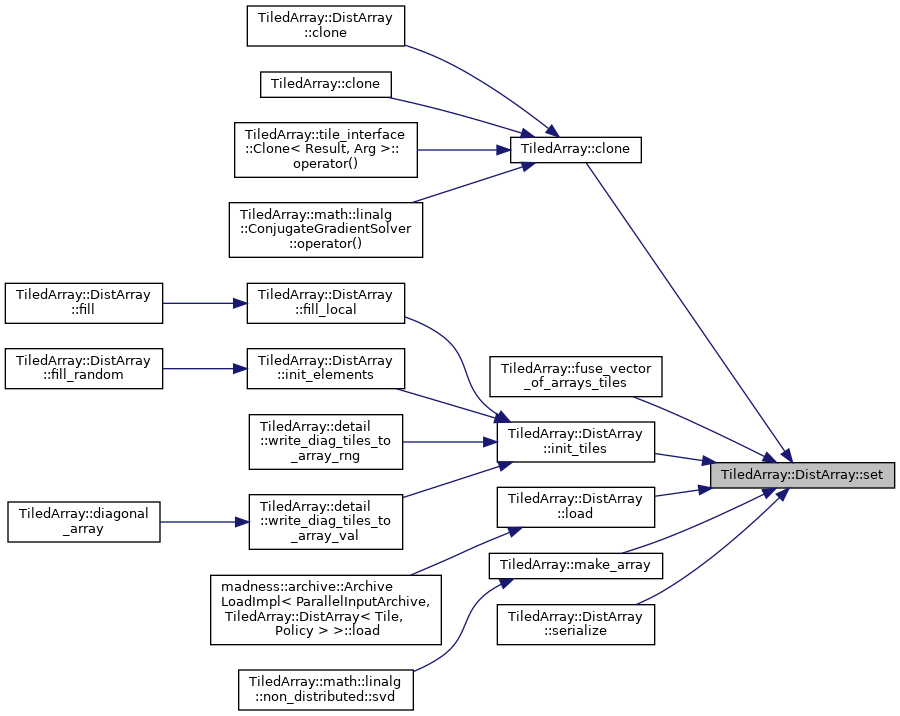
◆ set() [3/6]
|
inline |
Set a tile directly using a future to a tile.
- Template Parameters
-
Index For an ordinal index should be an integral type and for a coordinate index should be a type satisfying container of integral types. Can also be a range type.
- Parameters
-
[in] i The ordinal or coordinate index of the tile to set [in] v The tile's new value
- Exceptions
-
TiledArray::Exception If PIMPL is not initialized. Strong throw guarantee. TiledArray::Exception if index i
is out of bounds. Strong throw guarantee.TiledArray::Exception if index i
is a coordinate index with the wrong rank. Strong throw guarantee.TiledArray::Exception if tile i
is already set.
Definition at line 716 of file dist_array.h.
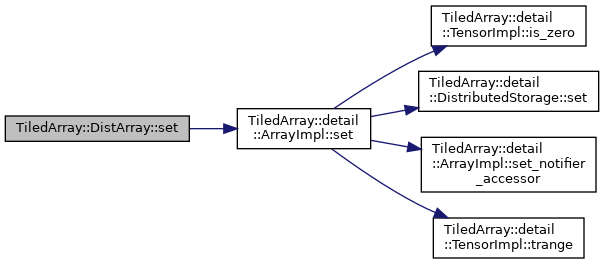
◆ set() [4/6]
|
inline |
Set a tile directly using a future to a tile.
- Template Parameters
-
Integer An integral type
- Parameters
-
[in] i The coordinate index of the tile to set, as an std::initializer_list<Integer>
[in] f A future to the tile's new value
- Exceptions
-
TiledArray::Exception If PIMPL is not initialized. Strong throw guarantee. TiledArray::Exception if index i
is out of bounds. Strong throw guarantee.TiledArray::Exception if index i
has the wrong rank. Strong throw guarantee.TiledArray::Exception if tile i
is already set.
Definition at line 738 of file dist_array.h.
◆ set() [5/6]
|
inline |
Set every element of a tile to a specified value.
This function sets each element of a tile to the specified value. For normal, non-nested, tiles this amounts to setting each scalar component of the tile to the provided value. For nested tile types this function sets the elements of the outer most tile (so the input value would be of type Tensor<T>, assuming a tile type of Tensor<Tensor<T>>).
- Template Parameters
-
Integer An integral type
- Parameters
-
[in] i The coordinate index, as an std::initializer_list<Integer>
for the tile.[in] value What the tile elements should be set to.
- Exceptions
-
TiledArray::Exception If PIMPL is not initialized. Strong throw guarantee. TiledArray::Exception if index i
is out of bounds. Strong throw guarantee.TiledArray::Exception if index i
has the wrong rank. Strong throw guarantee.TiledArray::Exception if tile i
is already set.
Definition at line 693 of file dist_array.h.
◆ set() [6/6]
|
inline |
Set a tile and fill it using a sequence
This function will set an uninitialized tile to the provided value. This overload allows the user to specify the coordinate index with an initializer list.
- Template Parameters
-
Integer An integral type InIter Type satisfying input iterator
- Parameters
-
[in] i The tile's coordinate index, as an std::initializer_list<Integer>
[in] first The iterator that points to the start of the input sequence. It is assumed that the container pointed to by first minimally contains the same number of elements as the tile.
- Exceptions
-
TiledArray::Exception if the tile is already initialized.
Definition at line 642 of file dist_array.h.
◆ shape()
|
inline |
Shape accessor.
Returns shape object. No communication is required.
- Returns
- reference to the shape object.
- Exceptions
-
TiledArray::Exception if the PIMPL is not initialized. Strong throw guarantee.
Definition at line 1039 of file dist_array.h.
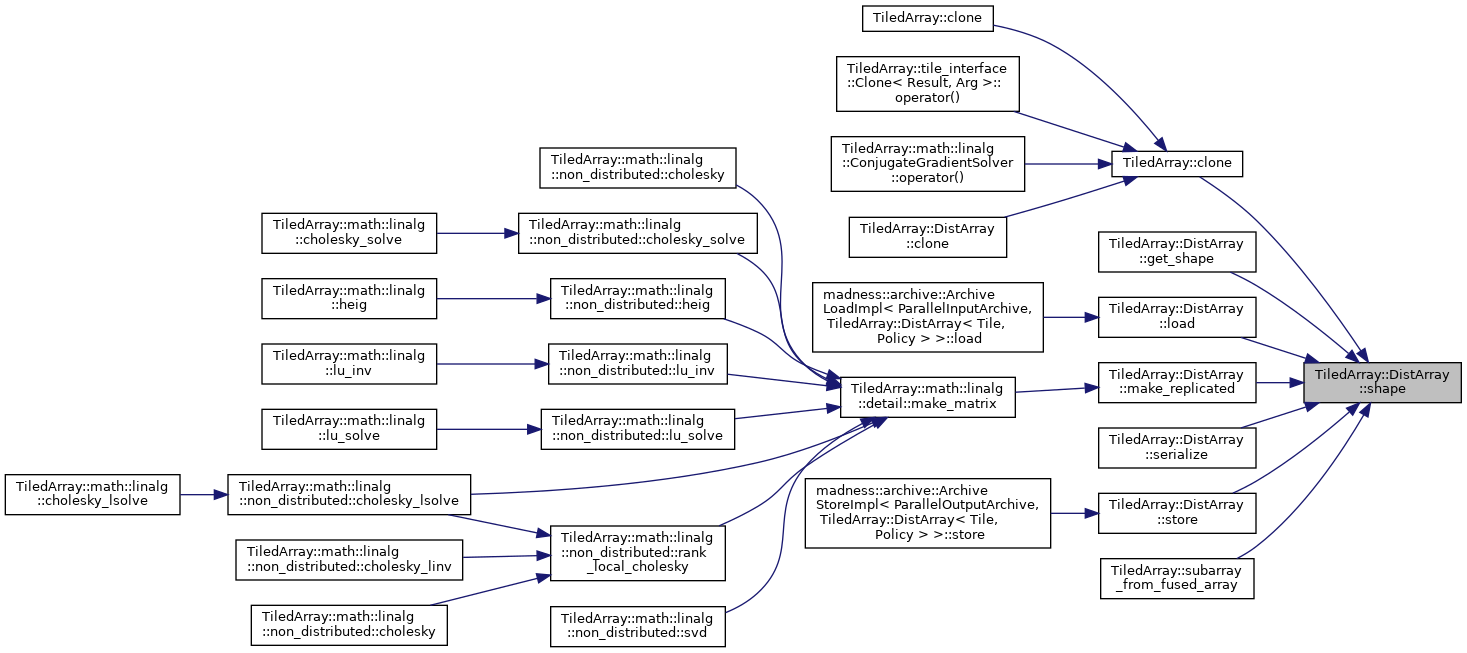
◆ size()
|
inline |
Returns the number of tiles in the tensor.
This function returns the number of tiles in the tensor. This is usually not the same as the volume of the tensor (i.e., the number of elements in the tensor; they are the same only if each tile contains a single element).
- Returns
- The number of tiles in the tensor.
- Exceptions
-
TiledArray::Exception if the PIMPL has not been set. Strong throw guarantee.
Definition at line 972 of file dist_array.h.
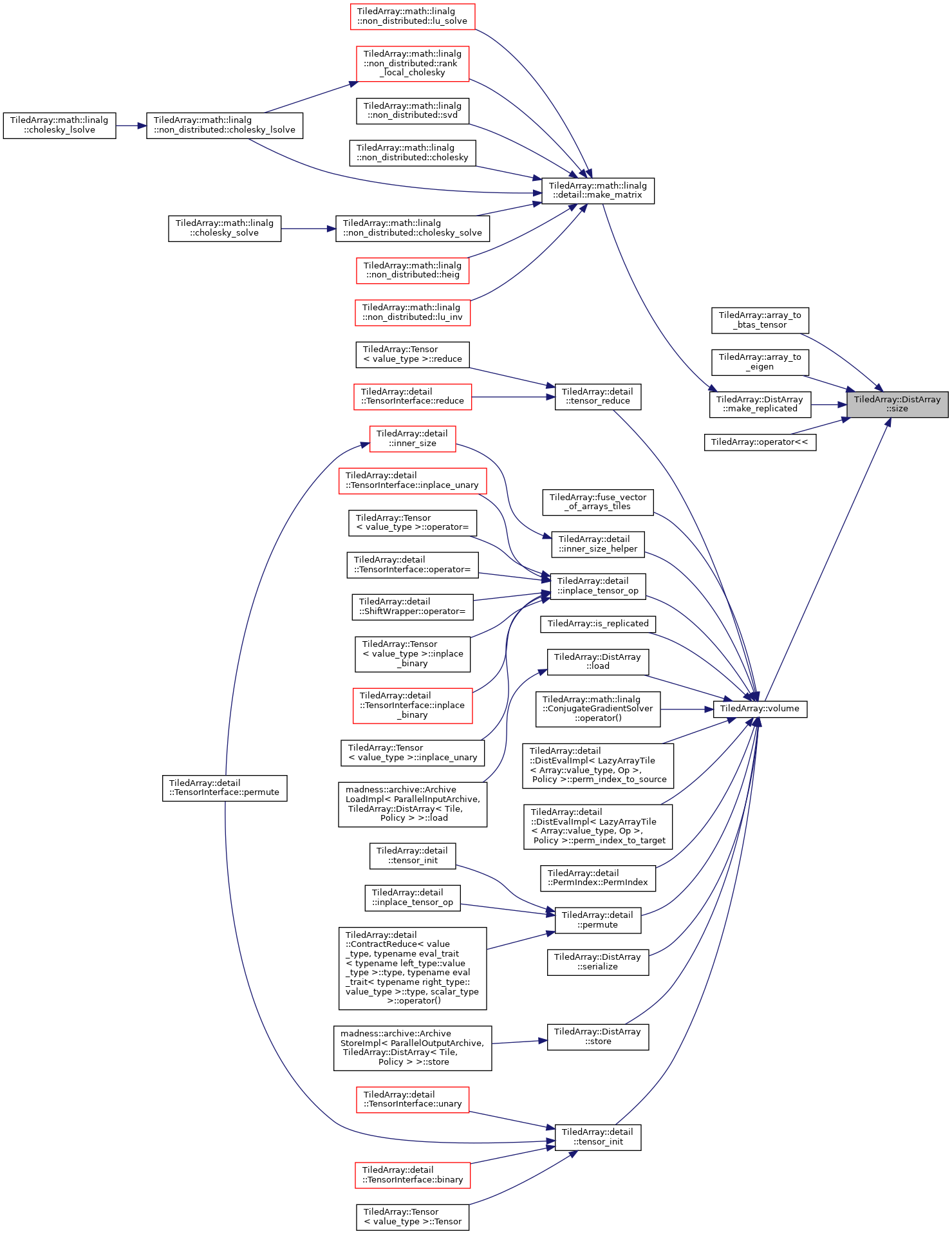
◆ store()
|
inline |
Stores this array to an Archive object.
- Template Parameters
-
Archive a parallel MADWorld Archive type
- Parameters
-
ar an Archive object that will contain this object's data
- Note
- The & operator for serializing will only work with parallel MADWorld archives.
-
this is a collective operation that fences before and after completion if
ar.dofence()
is true
Definition at line 1392 of file dist_array.h.
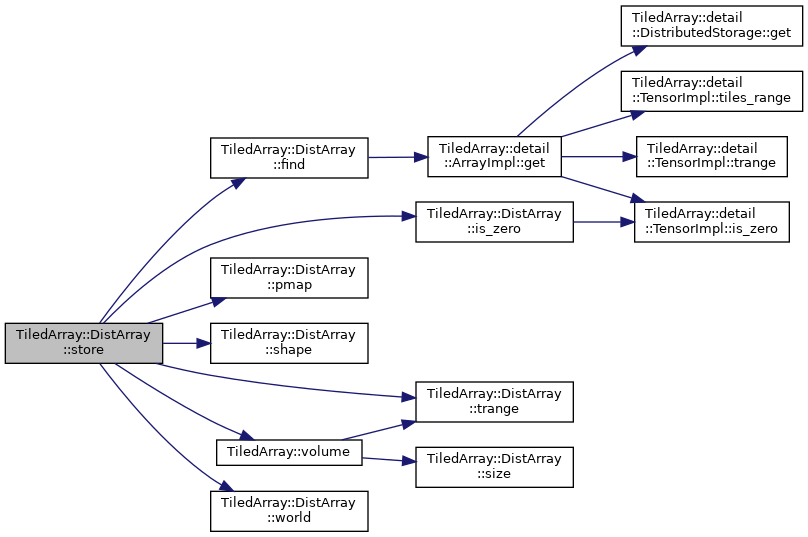

◆ swap()
|
inline |
Swap this array with other
.
- Parameters
-
other The array to be swapped with this array.
- Exceptions
-
None no throw guarantee.
Definition at line 1164 of file dist_array.h.

◆ tiles_range()
|
inline |
Tile range accessor.
- Returns
- A const reference to the range object for the array tiles
Definition at line 937 of file dist_array.h.
◆ trange()
|
inline |
Tiled range accessor.
This function returns an object containing the tiling information of the array (e.g., the tile boundaries, or which elements go to which tile). This should not be confused with range
which returns a Range object for iterating over the tile indices or elements_range
which returns a Range object for iterating over the elements.
- Returns
- A const reference to the tiled range object for the array
- Exceptions
-
TiledArray::Exception if the PIMPL is not initialized. Strong throw guarantee.
Definition at line 917 of file dist_array.h.
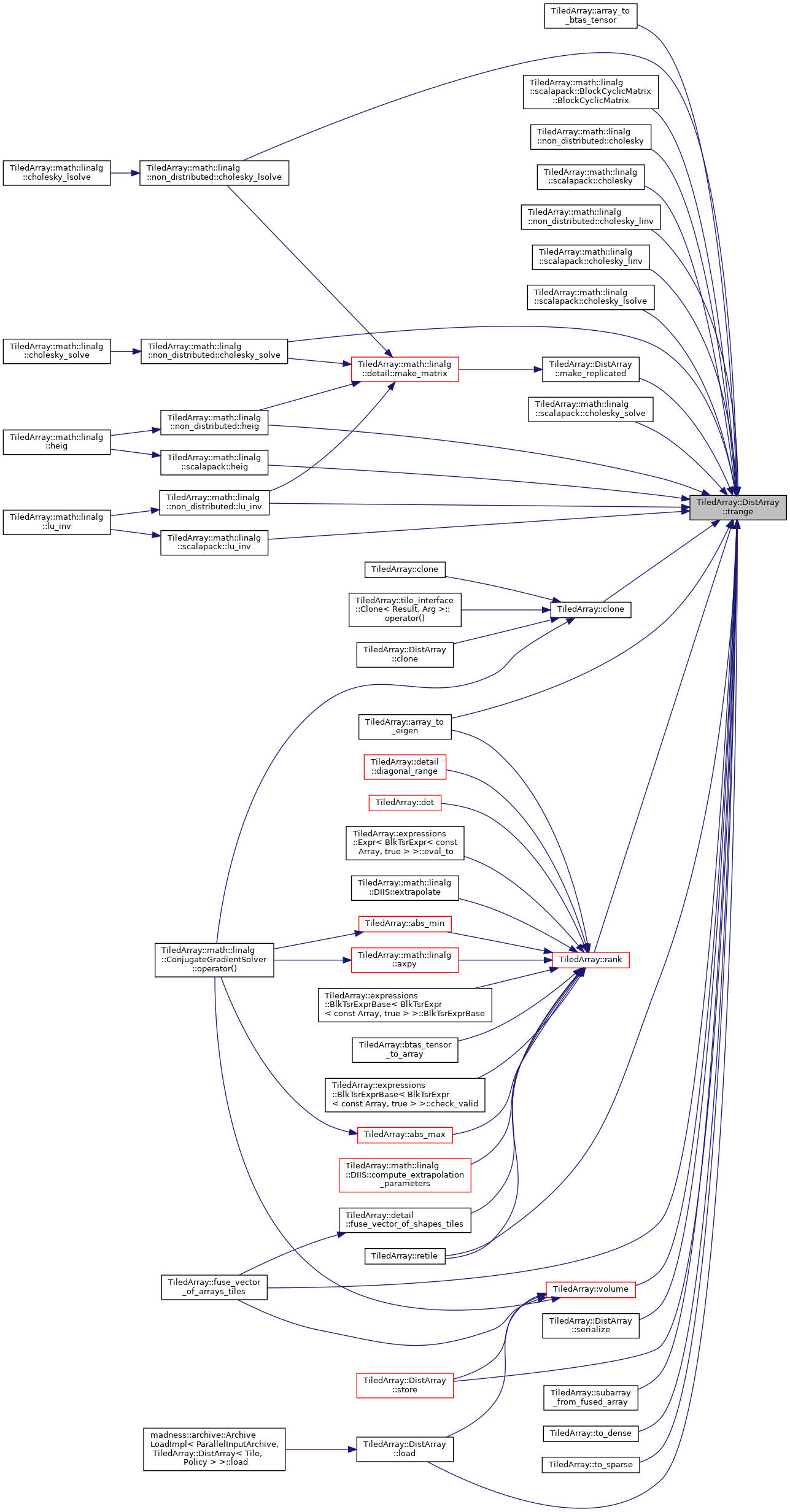
◆ truncate()
|
inline |
Update shape data and remove tiles that are below the zero threshold
- Parameters
-
[in] thresh the threshold below which the tiles are considered to be zero (only for sparse arrays will such tiles be discarded)
- See also
- SparseShape::is_zero
- Note
- This is a collective operation
- This function is a no-op for dense arrays.
Definition at line 1196 of file dist_array.h.

◆ wait_for_lazy_cleanup() [1/2]
|
inline |
Wait for lazy tile cleanup.
The member version of the static DistArray::wait_for_lazy_cleanup
Definition at line 457 of file dist_array.h.

◆ wait_for_lazy_cleanup() [2/2]
|
inlinestatic |
Wait for lazy tile cleanup.
This function will wait for cleanup of tile data that has been scheduled for lazy deletion. Ready tasks will be executed by this function while waiting for cleanup. This function will timeout if the wait time exceeds the timeout specified in the MAD_WAIT_TIMEOUT
environment variable. The default timeout is 900 seconds.
- Parameters
-
world The world that to be used to execute ready tasks.
- Exceptions
-
madness::MadnessException When timeout has been exceeded.
Definition at line 444 of file dist_array.h.

◆ weak_pimpl() [1/2]
|
inline |
Accessor for the (shared_ptr to) implementation object.
- Returns
- std::weak_ptr to the nonconst implementation object
Definition at line 433 of file dist_array.h.
◆ weak_pimpl() [2/2]
|
inline |
Accessor for the (weak_ptr to) implementation object.
- Returns
- std::weak_ptr to the const implementation object
Definition at line 428 of file dist_array.h.
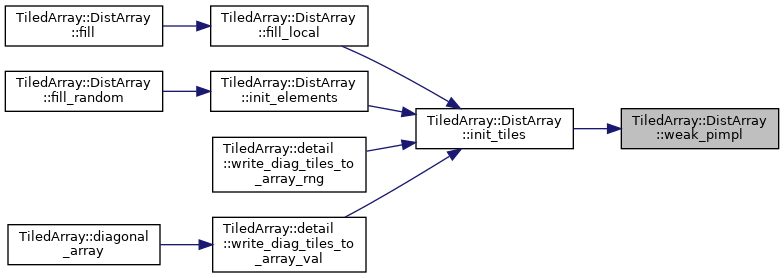
◆ world()
|
inline |
World accessor.
- Returns
- A reference to the world that owns this array.
- Exceptions
-
TiledArray::Exception if the PIMPL is not initialized. Strong throw guarantee.
Definition at line 1007 of file dist_array.h.
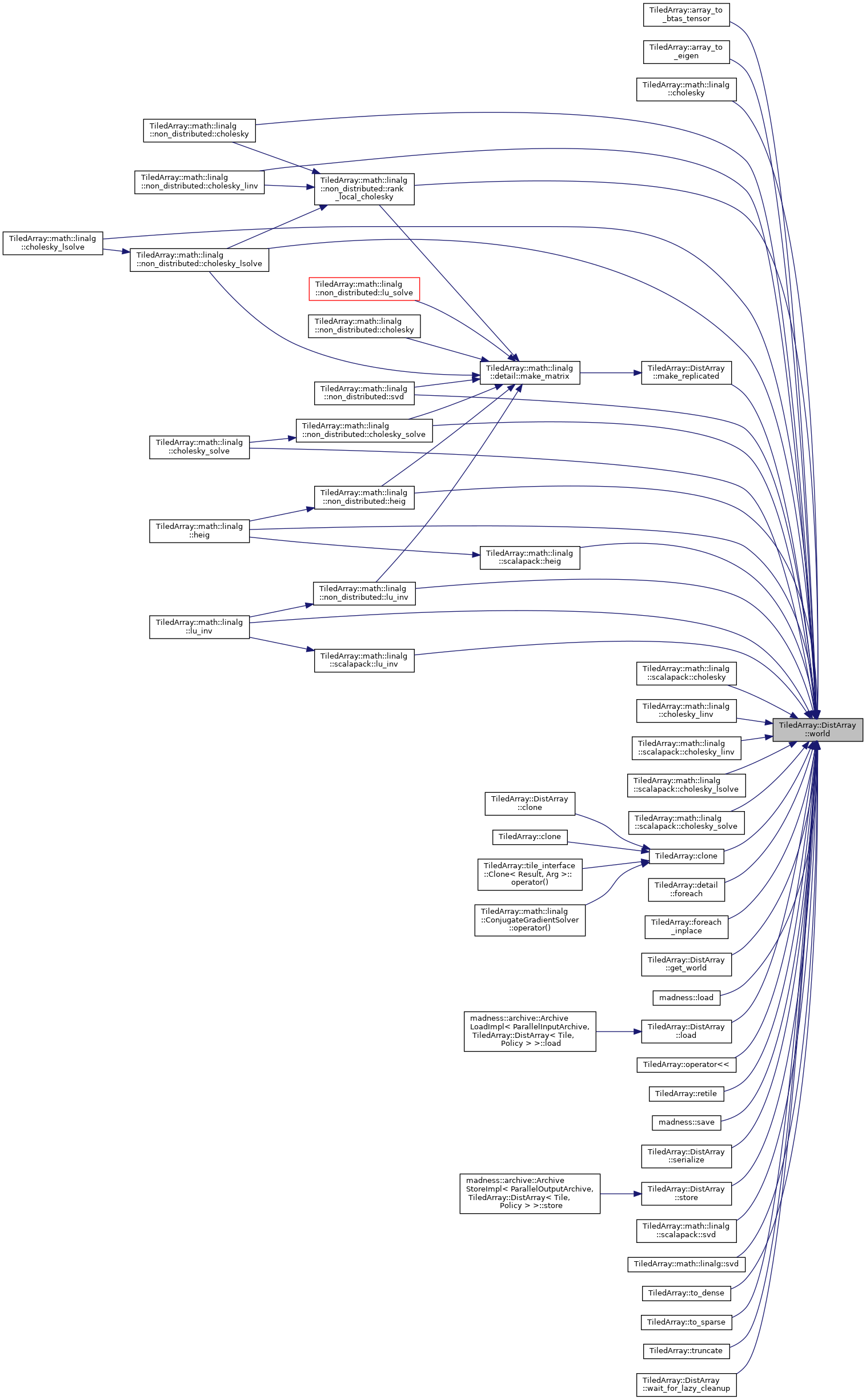
Member Data Documentation
◆ is_integral_or_integral_range_v
|
staticconstexpr |
Definition at line 117 of file dist_array.h.
◆ is_value_or_future_to_value_v
|
staticconstexpr |
Definition at line 128 of file dist_array.h.
The documentation for this class was generated from the following files:
- TiledArray/conversions/clone.h
- TiledArray/dist_array.h