

Documentation
template<typename Left, typename Right, typename Op, typename Policy>
class TiledArray::detail::BinaryEvalImpl< Left, Right, Op, Policy >
Binary, distributed tensor evaluator.
This object is used to evaluate the tiles of a distributed binary expression.
- Template Parameters
-
Left The left argument type Right The right argument type Op The binary transform operator type Policy The tensor policy class
Definition at line 40 of file binary_eval.h.
Public Types | |
typedef BinaryEvalImpl< Left, Right, Op, Policy > | BinaryEvalImpl_ |
This object type. More... | |
typedef DistEvalImpl< typename Op::result_type, Policy > | DistEvalImpl_ |
The base class type. More... | |
typedef DistEvalImpl_::TensorImpl_ | TensorImpl_ |
The base, base class type. More... | |
typedef Left | left_type |
The left-hand argument type. More... | |
typedef Right | right_type |
The right-hand argument type. More... | |
typedef DistEvalImpl_::ordinal_type | ordinal_type |
Ordinal type. More... | |
typedef DistEvalImpl_::range_type | range_type |
Range type. More... | |
typedef DistEvalImpl_::shape_type | shape_type |
Shape type. More... | |
typedef DistEvalImpl_::pmap_interface | pmap_interface |
Process map interface type. More... | |
typedef DistEvalImpl_::trange_type | trange_type |
Tiled range type. More... | |
typedef DistEvalImpl_::value_type | value_type |
Tile type. More... | |
typedef DistEvalImpl_::eval_type | eval_type |
Tile evaluation type. More... | |
typedef Op | op_type |
Tile evaluation operator type. More... | |
![]() | |
typedef DistEvalImpl< Op::result_type, Policy > | DistEvalImpl_ |
This object type. More... | |
typedef TiledArray::detail::TensorImpl< Policy > | TensorImpl_ |
Tensor implementation base class. More... | |
typedef TensorImpl_::ordinal_type | ordinal_type |
Ordinal type. More... | |
typedef TensorImpl_::trange_type | trange_type |
Tiled range type for this object. More... | |
typedef TensorImpl_::range_type | range_type |
Range type this tensor. More... | |
typedef TensorImpl_::shape_type | shape_type |
Shape type. More... | |
typedef TensorImpl_::pmap_interface | pmap_interface |
process map interface type More... | |
typedef Op::result_type | value_type |
Tile type. More... | |
typedef eval_trait< value_type >::type | eval_type |
Tile evaluation type. More... | |
![]() | |
typedef TensorImpl< Policy > | TensorImpl_ |
typedef Policy | policy_type |
Policy type. More... | |
typedef Policy::trange_type | trange_type |
Tiled range type. More... | |
typedef Policy::range_type | range_type |
Element/tile range type. More... | |
typedef Policy::index1_type | index1_type |
1-index type More... | |
typedef Policy::ordinal_type | ordinal_type |
Ordinal type. More... | |
typedef Policy::shape_type | shape_type |
Tensor shape type. More... | |
typedef Policy::pmap_interface | pmap_interface |
Process map interface type. More... | |
Public Member Functions | |
template<typename Perm , typename = std::enable_if_t< TiledArray::detail::is_permutation_v<Perm>>> | |
BinaryEvalImpl (const left_type &left, const right_type &right, World &world, const trange_type &trange, const shape_type &shape, const std::shared_ptr< pmap_interface > &pmap, const Perm &perm, const op_type &op) | |
Construct a binary evaluator. More... | |
virtual | ~BinaryEvalImpl () |
virtual Future< value_type > | get_tile (ordinal_type i) const |
Get tile at index i . More... | |
virtual void | discard_tile (ordinal_type i) const |
Discard a tile that is not needed. More... | |
![]() | |
DistEvalImpl (World &world, const trange_type &trange, const shape_type &shape, const std::shared_ptr< pmap_interface > &pmap, const Permutation &perm) | |
Constructor. More... | |
virtual | ~DistEvalImpl () |
const madness::uniqueidT & | id () const |
Unique object id accessor. More... | |
void | set_tile (ordinal_type i, const value_type &value) |
Set tensor value. More... | |
void | set_tile (ordinal_type i, Future< value_type > f) |
Set tensor value with a future. More... | |
virtual void | notify () |
Tile set notification. More... | |
void | wait () const |
Wait for all tiles to be assigned. More... | |
void | eval () |
Evaluate this tensor expression object. More... | |
![]() | |
TensorImpl (World &world, const trange_type &trange, const shape_type &shape, const std::shared_ptr< pmap_interface > &pmap) | |
Constructor. More... | |
virtual | ~TensorImpl () |
Virtual destructor. More... | |
const std::shared_ptr< pmap_interface > & | pmap () const |
Tensor process map accessor. More... | |
const range_type & | tiles_range () const |
Tiles range accessor. More... | |
ordinal_type | size () const |
Tensor tile volume accessor. More... | |
ordinal_type | local_size () const |
Local element count. More... | |
template<typename Index > | |
ProcessID | owner (const Index &i) const |
Query a tile owner. More... | |
template<typename Index > | |
bool | is_local (const Index &i) const |
Query for a locally owned tile. More... | |
template<typename Index > | |
bool | is_zero (const Index &i) const |
Query for a zero tile. More... | |
bool | is_dense () const |
Query the density of the tensor. More... | |
const shape_type & | shape () const |
Tensor shape accessor. More... | |
const trange_type & | trange () const |
Tiled range accessor. More... | |
World & | get_world () const |
World & | world () const |
World accessor. More... | |
Additional Inherited Members | |
![]() | |
ordinal_type | perm_index_to_target (ordinal_type index) const |
Permute index from a source index to a target index. More... | |
ordinal_type | perm_index_to_source (ordinal_type index) const |
Permute index from a target index to a source index. More... | |
Member Typedef Documentation
◆ BinaryEvalImpl_
typedef BinaryEvalImpl<Left, Right, Op, Policy> TiledArray::detail::BinaryEvalImpl< Left, Right, Op, Policy >::BinaryEvalImpl_ |
This object type.
Definition at line 45 of file binary_eval.h.
◆ DistEvalImpl_
typedef DistEvalImpl<typename Op::result_type, Policy> TiledArray::detail::BinaryEvalImpl< Left, Right, Op, Policy >::DistEvalImpl_ |
The base class type.
Definition at line 47 of file binary_eval.h.
◆ eval_type
typedef DistEvalImpl_::eval_type TiledArray::detail::BinaryEvalImpl< Left, Right, Op, Policy >::eval_type |
Tile evaluation type.
Definition at line 61 of file binary_eval.h.
◆ left_type
typedef Left TiledArray::detail::BinaryEvalImpl< Left, Right, Op, Policy >::left_type |
The left-hand argument type.
Definition at line 50 of file binary_eval.h.
◆ op_type
typedef Op TiledArray::detail::BinaryEvalImpl< Left, Right, Op, Policy >::op_type |
Tile evaluation operator type.
Definition at line 62 of file binary_eval.h.
◆ ordinal_type
typedef DistEvalImpl_::ordinal_type TiledArray::detail::BinaryEvalImpl< Left, Right, Op, Policy >::ordinal_type |
Ordinal type.
Definition at line 52 of file binary_eval.h.
◆ pmap_interface
typedef DistEvalImpl_::pmap_interface TiledArray::detail::BinaryEvalImpl< Left, Right, Op, Policy >::pmap_interface |
Process map interface type.
Definition at line 56 of file binary_eval.h.
◆ range_type
typedef DistEvalImpl_::range_type TiledArray::detail::BinaryEvalImpl< Left, Right, Op, Policy >::range_type |
Range type.
Definition at line 53 of file binary_eval.h.
◆ right_type
typedef Right TiledArray::detail::BinaryEvalImpl< Left, Right, Op, Policy >::right_type |
The right-hand argument type.
Definition at line 51 of file binary_eval.h.
◆ shape_type
typedef DistEvalImpl_::shape_type TiledArray::detail::BinaryEvalImpl< Left, Right, Op, Policy >::shape_type |
Shape type.
Definition at line 54 of file binary_eval.h.
◆ TensorImpl_
typedef DistEvalImpl_::TensorImpl_ TiledArray::detail::BinaryEvalImpl< Left, Right, Op, Policy >::TensorImpl_ |
The base, base class type.
Definition at line 49 of file binary_eval.h.
◆ trange_type
typedef DistEvalImpl_::trange_type TiledArray::detail::BinaryEvalImpl< Left, Right, Op, Policy >::trange_type |
Tiled range type.
Definition at line 58 of file binary_eval.h.
◆ value_type
typedef DistEvalImpl_::value_type TiledArray::detail::BinaryEvalImpl< Left, Right, Op, Policy >::value_type |
Tile type.
Definition at line 59 of file binary_eval.h.
Constructor & Destructor Documentation
◆ BinaryEvalImpl()
|
inline |
Construct a binary evaluator.
- Parameters
-
left The left-hand argument right The right-hand argument world The world where the tensor lives trange The tiled range object shape The tensor shape object pmap The tile-process map perm The permutation that is applied to tile indices op The tile transform operation
Definition at line 84 of file binary_eval.h.
◆ ~BinaryEvalImpl()
|
inlinevirtual |
Definition at line 95 of file binary_eval.h.
Member Function Documentation
◆ discard_tile()
|
inlinevirtual |
Discard a tile that is not needed.
This function handles the cleanup for tiles that are not needed in subsequent computation.
- Parameters
-
i The index of the tile
Implements TiledArray::detail::DistEvalImpl< Op::result_type, Policy >.
Definition at line 121 of file binary_eval.h.
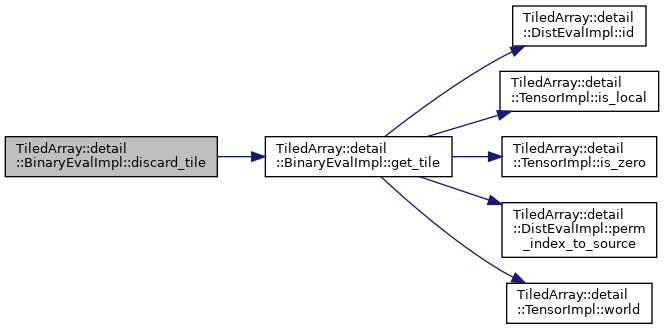
◆ get_tile()
|
inlinevirtual |
Get tile at index i
.
- Parameters
-
i The index of the tile
- Returns
- A
Future
to the tile at index i
- Exceptions
-
TiledArray::Exception When tile i
is owned by a remote node.TiledArray::Exception When tile i
a zero tile.
Implements TiledArray::detail::DistEvalImpl< Op::result_type, Policy >.
Definition at line 103 of file binary_eval.h.
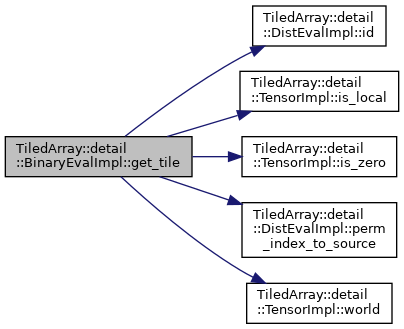

The documentation for this class was generated from the following file:
- TiledArray/dist_eval/binary_eval.h