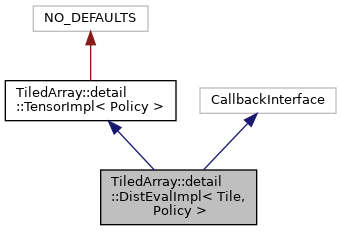
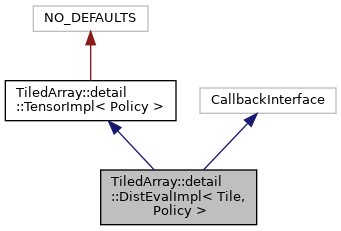
Documentation
template<typename Tile, typename Policy>
class TiledArray::detail::DistEvalImpl< Tile, Policy >
Distributed evaluator implementation object.
This class is used as the base class for other distributed evaluation implementation classes. It has several pure virtual function that are used by derived classes to implement the distributed evaluate. This class can also handles permutation of result tiles if necessary.
- Template Parameters
-
Tile The output tile type Policy The tensor policy class
Definition at line 45 of file dist_eval.h.
Public Types | |
typedef DistEvalImpl< Tile, Policy > | DistEvalImpl_ |
This object type. More... | |
typedef TiledArray::detail::TensorImpl< Policy > | TensorImpl_ |
Tensor implementation base class. More... | |
typedef TensorImpl_::ordinal_type | ordinal_type |
Ordinal type. More... | |
typedef TensorImpl_::trange_type | trange_type |
Tiled range type for this object. More... | |
typedef TensorImpl_::range_type | range_type |
Range type this tensor. More... | |
typedef TensorImpl_::shape_type | shape_type |
Shape type. More... | |
typedef TensorImpl_::pmap_interface | pmap_interface |
process map interface type More... | |
typedef Tile | value_type |
Tile type. More... | |
typedef eval_trait< value_type >::type | eval_type |
Tile evaluation type. More... | |
![]() | |
typedef TensorImpl< Policy > | TensorImpl_ |
typedef Policy | policy_type |
Policy type. More... | |
typedef Policy::trange_type | trange_type |
Tiled range type. More... | |
typedef Policy::range_type | range_type |
Element/tile range type. More... | |
typedef Policy::index1_type | index1_type |
1-index type More... | |
typedef Policy::ordinal_type | ordinal_type |
Ordinal type. More... | |
typedef Policy::shape_type | shape_type |
Tensor shape type. More... | |
typedef Policy::pmap_interface | pmap_interface |
Process map interface type. More... | |
Public Member Functions | |
DistEvalImpl (World &world, const trange_type &trange, const shape_type &shape, const std::shared_ptr< pmap_interface > &pmap, const Permutation &perm) | |
Constructor. More... | |
virtual | ~DistEvalImpl () |
const madness::uniqueidT & | id () const |
Unique object id accessor. More... | |
virtual Future< value_type > | get_tile (ordinal_type i) const =0 |
Get tile at index i . More... | |
virtual void | discard_tile (ordinal_type i) const =0 |
Discard a tile that is not needed. More... | |
void | set_tile (ordinal_type i, const value_type &value) |
Set tensor value. More... | |
void | set_tile (ordinal_type i, Future< value_type > f) |
Set tensor value with a future. More... | |
virtual void | notify () |
Tile set notification. More... | |
void | wait () const |
Wait for all tiles to be assigned. More... | |
void | eval () |
Evaluate this tensor expression object. More... | |
![]() | |
TensorImpl (World &world, const trange_type &trange, const shape_type &shape, const std::shared_ptr< pmap_interface > &pmap) | |
Constructor. More... | |
virtual | ~TensorImpl () |
Virtual destructor. More... | |
const std::shared_ptr< pmap_interface > & | pmap () const |
Tensor process map accessor. More... | |
const range_type & | tiles_range () const |
Tiles range accessor. More... | |
ordinal_type | size () const |
Tensor tile volume accessor. More... | |
ordinal_type | local_size () const |
Local element count. More... | |
template<typename Index > | |
ProcessID | owner (const Index &i) const |
Query a tile owner. More... | |
template<typename Index > | |
bool | is_local (const Index &i) const |
Query for a locally owned tile. More... | |
template<typename Index > | |
bool | is_zero (const Index &i) const |
Query for a zero tile. More... | |
bool | is_dense () const |
Query the density of the tensor. More... | |
const shape_type & | shape () const |
Tensor shape accessor. More... | |
const trange_type & | trange () const |
Tiled range accessor. More... | |
World & | get_world () const |
World & | world () const |
World accessor. More... | |
Protected Member Functions | |
ordinal_type | perm_index_to_target (ordinal_type index) const |
Permute index from a source index to a target index. More... | |
ordinal_type | perm_index_to_source (ordinal_type index) const |
Permute index from a target index to a source index. More... | |
Member Typedef Documentation
◆ DistEvalImpl_
typedef DistEvalImpl<Tile, Policy> TiledArray::detail::DistEvalImpl< Tile, Policy >::DistEvalImpl_ |
This object type.
Definition at line 48 of file dist_eval.h.
◆ eval_type
typedef eval_trait<value_type>::type TiledArray::detail::DistEvalImpl< Tile, Policy >::eval_type |
Tile evaluation type.
Definition at line 62 of file dist_eval.h.
◆ ordinal_type
typedef TensorImpl_::ordinal_type TiledArray::detail::DistEvalImpl< Tile, Policy >::ordinal_type |
Ordinal type.
Definition at line 52 of file dist_eval.h.
◆ pmap_interface
typedef TensorImpl_::pmap_interface TiledArray::detail::DistEvalImpl< Tile, Policy >::pmap_interface |
process map interface type
Definition at line 59 of file dist_eval.h.
◆ range_type
typedef TensorImpl_::range_type TiledArray::detail::DistEvalImpl< Tile, Policy >::range_type |
Range type this tensor.
Definition at line 56 of file dist_eval.h.
◆ shape_type
typedef TensorImpl_::shape_type TiledArray::detail::DistEvalImpl< Tile, Policy >::shape_type |
Shape type.
Definition at line 57 of file dist_eval.h.
◆ TensorImpl_
typedef TiledArray::detail::TensorImpl<Policy> TiledArray::detail::DistEvalImpl< Tile, Policy >::TensorImpl_ |
Tensor implementation base class.
Definition at line 49 of file dist_eval.h.
◆ trange_type
typedef TensorImpl_::trange_type TiledArray::detail::DistEvalImpl< Tile, Policy >::trange_type |
Tiled range type for this object.
Definition at line 54 of file dist_eval.h.
◆ value_type
typedef Tile TiledArray::detail::DistEvalImpl< Tile, Policy >::value_type |
Tile type.
Definition at line 60 of file dist_eval.h.
Constructor & Destructor Documentation
◆ DistEvalImpl()
|
inline |
Constructor.
- Parameters
-
world The world where the tensor lives trange The tiled range object shape The tensor shape object pmap The tile-process map perm The permutation that is applied to tile indices
- Note
trange
andshape
will be permuted byperm
before storing the data.
Definition at line 109 of file dist_eval.h.
◆ ~DistEvalImpl()
|
inlinevirtual |
Definition at line 128 of file dist_eval.h.
Member Function Documentation
◆ discard_tile()
|
pure virtual |
Discard a tile that is not needed.
This function handles the cleanup for tiles that are not needed in subsequent computation.
- Parameters
-
i The index of the tile
Implemented in TiledArray::detail::ArrayEvalImpl< Array, Op, Policy >, TiledArray::detail::UnaryEvalImpl< Arg, Op, Policy >, TiledArray::detail::Summa< Left, Right, Op, Policy >, and TiledArray::detail::BinaryEvalImpl< Left, Right, Op, Policy >.

◆ eval()
|
inline |
Evaluate this tensor expression object.
This function will evaluate the children of this distributed evaluator and evaluate the tiles for this distributed evaluator. It will block until the tasks for the children are evaluated (not for the tasks of this object).
Definition at line 231 of file dist_eval.h.

◆ get_tile()
|
pure virtual |
Get tile at index i
.
- Parameters
-
i The index of the tile
- Returns
- Tile at index i
Implemented in TiledArray::detail::UnaryEvalImpl< Arg, Op, Policy >, TiledArray::detail::Summa< Left, Right, Op, Policy >, TiledArray::detail::BinaryEvalImpl< Left, Right, Op, Policy >, and TiledArray::detail::ArrayEvalImpl< Array, Op, Policy >.

◆ id()
|
inline |
Unique object id accessor.
- Returns
- This object's unique identifier
Definition at line 133 of file dist_eval.h.
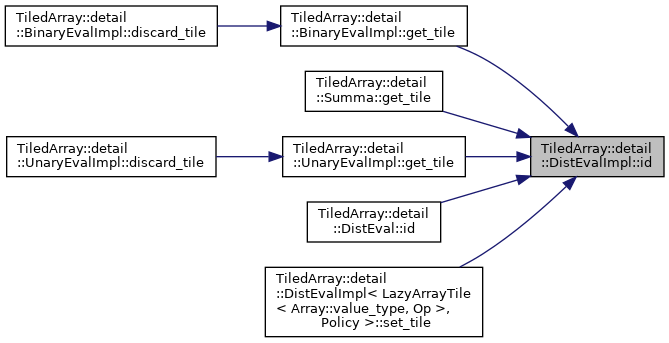
◆ notify()
|
inlinevirtual |
Tile set notification.
Definition at line 179 of file dist_eval.h.

◆ perm_index_to_source()
|
inlineprotected |
Permute index
from a target index to a source index.
- Parameters
-
index An ordinal index in the target index space
- Returns
- The ordinal index in the source index space
Definition at line 94 of file dist_eval.h.
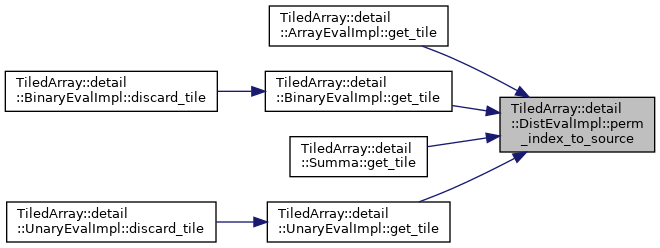
◆ perm_index_to_target()
|
inlineprotected |
Permute index
from a source index to a target index.
- Parameters
-
index An ordinal index in the source index space
- Returns
- The ordinal index in the target index space
Definition at line 85 of file dist_eval.h.
◆ set_tile() [1/2]
|
inline |
Set tensor value.
This will store value
at ordinal index i
. Typically, this function should be called by a task function.
- Parameters
-
i The index in the result space where value will be stored value The value to be stored at index i
Definition at line 154 of file dist_eval.h.
◆ set_tile() [2/2]
|
inline |
Set tensor value with a future.
This will store value
at ordinal index i
. Typically, this function should be called by a task function.
- Parameters
-
i The index in the result space where value will be stored f The future value to be stored at index i
Definition at line 169 of file dist_eval.h.
◆ wait()
|
inline |
Wait for all tiles to be assigned.
Definition at line 182 of file dist_eval.h.

The documentation for this class was generated from the following file:
- TiledArray/dist_eval/dist_eval.h