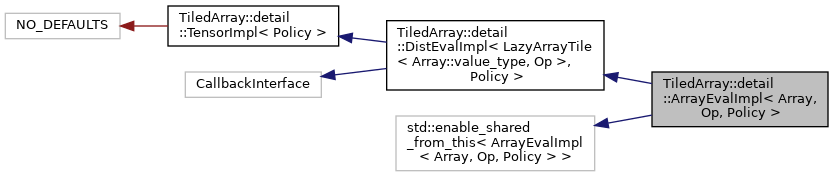
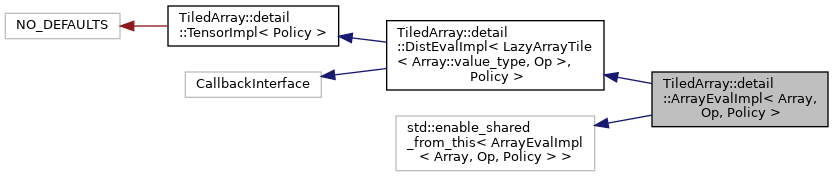
Documentation
template<typename Array, typename Op, typename Policy>
class TiledArray::detail::ArrayEvalImpl< Array, Op, Policy >
Distributed evaluator for TiledArray::Array
objects.
This distributed evaluator applies modifications to Array that will be used as input to other distributed evaluators. Common operations that may be applied to array objects are scaling, permutation, and lazy tile evaluation. It also serves as an abstraction layer between TiledArray::Array
objects and internal evaluation of expressions. The main purpose of this evaluator is to do a lazy evaluation of input tiles so that the resulting data is only evaluated when the tile is needed by subsequent operations.
- Template Parameters
-
Policy The evaluator policy type
Definition at line 170 of file array_eval.h.
Public Member Functions | |
template<typename Perm , typename = std::enable_if_t< TiledArray::detail::is_permutation_v<Perm>>> | |
ArrayEvalImpl (const array_type &array, World &world, const trange_type &trange, const shape_type &shape, const std::shared_ptr< pmap_interface > &pmap, const Perm &perm, const op_type &op) | |
Construct with full array range. More... | |
template<typename Index1 , typename Index2 , typename Perm , typename = std::enable_if_t< TiledArray::detail::is_integral_range_v<Index1> && TiledArray::detail::is_integral_range_v<Index2> && TiledArray::detail::is_permutation_v<Perm>>> | |
ArrayEvalImpl (const array_type &array, World &world, const trange_type &trange, const shape_type &shape, const std::shared_ptr< pmap_interface > &pmap, const Perm &perm, const op_type &op, const Index1 &lower_bound, const Index2 &upper_bound) | |
Constructor with sub-block range. More... | |
virtual | ~ArrayEvalImpl () |
Virtual destructor. More... | |
virtual Future< value_type > | get_tile (ordinal_type i) const |
Get tile at index i . More... | |
virtual void | discard_tile (ordinal_type) const |
Discard a tile that is not needed. More... | |
![]() | |
DistEvalImpl (World &world, const trange_type &trange, const shape_type &shape, const std::shared_ptr< pmap_interface > &pmap, const Permutation &perm) | |
Constructor. More... | |
virtual | ~DistEvalImpl () |
const madness::uniqueidT & | id () const |
Unique object id accessor. More... | |
void | set_tile (ordinal_type i, const value_type &value) |
Set tensor value. More... | |
void | set_tile (ordinal_type i, Future< value_type > f) |
Set tensor value with a future. More... | |
virtual void | notify () |
Tile set notification. More... | |
void | wait () const |
Wait for all tiles to be assigned. More... | |
void | eval () |
Evaluate this tensor expression object. More... | |
![]() | |
TensorImpl (World &world, const trange_type &trange, const shape_type &shape, const std::shared_ptr< pmap_interface > &pmap) | |
Constructor. More... | |
virtual | ~TensorImpl () |
Virtual destructor. More... | |
const std::shared_ptr< pmap_interface > & | pmap () const |
Tensor process map accessor. More... | |
const range_type & | tiles_range () const |
Tiles range accessor. More... | |
ordinal_type | size () const |
Tensor tile volume accessor. More... | |
ordinal_type | local_size () const |
Local element count. More... | |
template<typename Index > | |
ProcessID | owner (const Index &i) const |
Query a tile owner. More... | |
template<typename Index > | |
bool | is_local (const Index &i) const |
Query for a locally owned tile. More... | |
template<typename Index > | |
bool | is_zero (const Index &i) const |
Query for a zero tile. More... | |
bool | is_dense () const |
Query the density of the tensor. More... | |
const shape_type & | shape () const |
Tensor shape accessor. More... | |
const trange_type & | trange () const |
Tiled range accessor. More... | |
World & | get_world () const |
World & | world () const |
World accessor. More... | |
Additional Inherited Members | |
![]() | |
ordinal_type | perm_index_to_target (ordinal_type index) const |
Permute index from a source index to a target index. More... | |
ordinal_type | perm_index_to_source (ordinal_type index) const |
Permute index from a target index to a source index. More... | |
Member Typedef Documentation
◆ array_type
typedef Array TiledArray::detail::ArrayEvalImpl< Array, Op, Policy >::array_type |
The array type.
Definition at line 181 of file array_eval.h.
◆ ArrayEvalImpl_
typedef ArrayEvalImpl<Array, Op, Policy> TiledArray::detail::ArrayEvalImpl< Array, Op, Policy >::ArrayEvalImpl_ |
This object type.
Definition at line 176 of file array_eval.h.
◆ DistEvalImpl_
typedef DistEvalImpl<LazyArrayTile<typename Array::value_type, Op>, Policy> TiledArray::detail::ArrayEvalImpl< Array, Op, Policy >::DistEvalImpl_ |
The base class type.
Definition at line 178 of file array_eval.h.
◆ op_type
typedef Op TiledArray::detail::ArrayEvalImpl< Array, Op, Policy >::op_type |
Tile evaluation operator type.
Definition at line 191 of file array_eval.h.
◆ ordinal_type
typedef DistEvalImpl_::ordinal_type TiledArray::detail::ArrayEvalImpl< Array, Op, Policy >::ordinal_type |
Ordinal type.
Definition at line 182 of file array_eval.h.
◆ pmap_interface
typedef DistEvalImpl_::pmap_interface TiledArray::detail::ArrayEvalImpl< Array, Op, Policy >::pmap_interface |
Process map interface type.
Definition at line 186 of file array_eval.h.
◆ range_type
typedef DistEvalImpl_::range_type TiledArray::detail::ArrayEvalImpl< Array, Op, Policy >::range_type |
Range type.
Definition at line 183 of file array_eval.h.
◆ shape_type
typedef DistEvalImpl_::shape_type TiledArray::detail::ArrayEvalImpl< Array, Op, Policy >::shape_type |
Shape type.
Definition at line 184 of file array_eval.h.
◆ TensorImpl_
typedef DistEvalImpl_::TensorImpl_ TiledArray::detail::ArrayEvalImpl< Array, Op, Policy >::TensorImpl_ |
The base, base class type.
Definition at line 180 of file array_eval.h.
◆ trange_type
typedef DistEvalImpl_::trange_type TiledArray::detail::ArrayEvalImpl< Array, Op, Policy >::trange_type |
tiled range type
Definition at line 188 of file array_eval.h.
◆ value_type
typedef DistEvalImpl_::value_type TiledArray::detail::ArrayEvalImpl< Array, Op, Policy >::value_type |
value type = LazyArrayTile
Definition at line 190 of file array_eval.h.
Constructor & Destructor Documentation
◆ ArrayEvalImpl() [1/2]
|
inline |
Construct with full array range.
- Parameters
-
array The array that will be evaluated world The world where array will be evaluated trange The tiled range of the result tensor shape The shape of the result tensor pmap The process map for the result tensor tiles perm The permutation that is applied to the tile coordinate index op The operation that will be used to evaluate the tiles of array
Definition at line 213 of file array_eval.h.
◆ ArrayEvalImpl() [2/2]
|
inline |
Constructor with sub-block range.
- Template Parameters
-
Index1 An integral range type Index2 An integral range type
- Parameters
-
array The array that will be evaluated world The world where array will be evaluated trange The tiled range of the result tensor shape The shape of the result tensor pmap The process map for the result tensor tiles perm The permutation that is applied to the tile coordinate index op The operation that will be used to evaluate the tiles of array lower_bound The sub-block lower bound upper_bound The sub-block upper bound
Definition at line 240 of file array_eval.h.
◆ ~ArrayEvalImpl()
|
inlinevirtual |
Virtual destructor.
Definition at line 251 of file array_eval.h.
Member Function Documentation
◆ discard_tile()
|
inlinevirtual |
Discard a tile that is not needed.
This function handles the cleanup for tiles that are not needed in subsequent computation.
Implements TiledArray::detail::DistEvalImpl< LazyArrayTile< Array::value_type, Op >, Policy >.
Definition at line 273 of file array_eval.h.

◆ get_tile()
|
inlinevirtual |
Get tile at index i
.
- Parameters
-
i The index of the tile
- Returns
- Tile at index i
Implements TiledArray::detail::DistEvalImpl< LazyArrayTile< Array::value_type, Op >, Policy >.
Definition at line 253 of file array_eval.h.
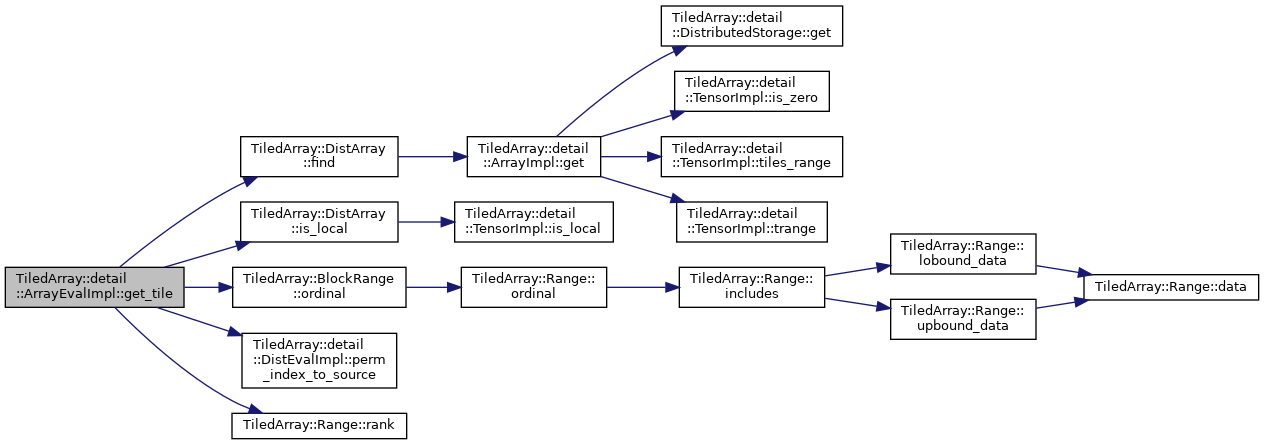
The documentation for this class was generated from the following file:
- TiledArray/dist_eval/array_eval.h